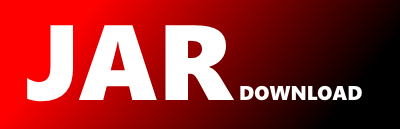
com.gs.collections.impl.map.mutable.UnmodifiableMutableMap Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2015 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.map.mutable;
import java.util.Collection;
import java.util.Comparator;
import java.util.Iterator;
import java.util.Map;
import com.gs.collections.api.LazyIterable;
import com.gs.collections.api.RichIterable;
import com.gs.collections.api.bag.MutableBag;
import com.gs.collections.api.bag.primitive.MutableBooleanBag;
import com.gs.collections.api.bag.primitive.MutableByteBag;
import com.gs.collections.api.bag.primitive.MutableCharBag;
import com.gs.collections.api.bag.primitive.MutableDoubleBag;
import com.gs.collections.api.bag.primitive.MutableFloatBag;
import com.gs.collections.api.bag.primitive.MutableIntBag;
import com.gs.collections.api.bag.primitive.MutableLongBag;
import com.gs.collections.api.bag.primitive.MutableShortBag;
import com.gs.collections.api.bag.sorted.MutableSortedBag;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function0;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.function.primitive.BooleanFunction;
import com.gs.collections.api.block.function.primitive.ByteFunction;
import com.gs.collections.api.block.function.primitive.CharFunction;
import com.gs.collections.api.block.function.primitive.DoubleFunction;
import com.gs.collections.api.block.function.primitive.DoubleObjectToDoubleFunction;
import com.gs.collections.api.block.function.primitive.FloatFunction;
import com.gs.collections.api.block.function.primitive.FloatObjectToFloatFunction;
import com.gs.collections.api.block.function.primitive.IntFunction;
import com.gs.collections.api.block.function.primitive.IntObjectToIntFunction;
import com.gs.collections.api.block.function.primitive.LongFunction;
import com.gs.collections.api.block.function.primitive.LongObjectToLongFunction;
import com.gs.collections.api.block.function.primitive.ShortFunction;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.block.procedure.Procedure2;
import com.gs.collections.api.block.procedure.primitive.ObjectIntProcedure;
import com.gs.collections.api.collection.primitive.MutableBooleanCollection;
import com.gs.collections.api.collection.primitive.MutableByteCollection;
import com.gs.collections.api.collection.primitive.MutableCharCollection;
import com.gs.collections.api.collection.primitive.MutableDoubleCollection;
import com.gs.collections.api.collection.primitive.MutableFloatCollection;
import com.gs.collections.api.collection.primitive.MutableIntCollection;
import com.gs.collections.api.collection.primitive.MutableLongCollection;
import com.gs.collections.api.collection.primitive.MutableShortCollection;
import com.gs.collections.api.list.MutableList;
import com.gs.collections.api.map.ImmutableMap;
import com.gs.collections.api.map.MutableMap;
import com.gs.collections.api.map.primitive.ObjectDoubleMap;
import com.gs.collections.api.map.primitive.ObjectLongMap;
import com.gs.collections.api.map.sorted.MutableSortedMap;
import com.gs.collections.api.multimap.MutableMultimap;
import com.gs.collections.api.multimap.bag.MutableBagMultimap;
import com.gs.collections.api.multimap.set.MutableSetMultimap;
import com.gs.collections.api.ordered.OrderedIterable;
import com.gs.collections.api.partition.bag.PartitionMutableBag;
import com.gs.collections.api.set.MutableSet;
import com.gs.collections.api.set.sorted.MutableSortedSet;
import com.gs.collections.api.tuple.Pair;
import com.gs.collections.impl.UnmodifiableIteratorAdapter;
import com.gs.collections.impl.UnmodifiableMap;
import com.gs.collections.impl.factory.Maps;
import com.gs.collections.impl.tuple.AbstractImmutableEntry;
import com.gs.collections.impl.utility.LazyIterate;
/**
* An unmodifiable view of a map.
*
* @see MutableMap#asUnmodifiable()
*/
public class UnmodifiableMutableMap
extends UnmodifiableMap
implements MutableMap
{
private static final long serialVersionUID = 1L;
protected UnmodifiableMutableMap(MutableMap map)
{
super(map);
}
/**
* This method will take a MutableMap and wrap it directly in a UnmodifiableMutableMap. It will
* take any other non-GS-map and first adapt it will a MapAdapter, and then return a
* UnmodifiableMutableMap that wraps the adapter.
*/
public static > UnmodifiableMutableMap of(M map)
{
if (map == null)
{
throw new IllegalArgumentException("cannot create a UnmodifiableMutableMap for null");
}
return new UnmodifiableMutableMap(MapAdapter.adapt(map));
}
public MutableMap newEmpty()
{
return this.getMutableMap().newEmpty();
}
public boolean notEmpty()
{
return this.getMutableMap().notEmpty();
}
public void forEachValue(Procedure super V> procedure)
{
this.getMutableMap().forEachValue(procedure);
}
public void forEachKey(Procedure super K> procedure)
{
this.getMutableMap().forEachKey(procedure);
}
public void forEachKeyValue(Procedure2 super K, ? super V> procedure)
{
this.getMutableMap().forEachKeyValue(procedure);
}
public MutableMap flipUniqueValues()
{
return this.getMutableMap().flipUniqueValues();
}
public MutableMap collectKeysAndValues(
Iterable iterable,
Function super E, ? extends K> keyFunction,
Function super E, ? extends V> valueFunction)
{
throw new UnsupportedOperationException("Cannot call collectKeysAndValues() on " + this.getClass().getSimpleName());
}
public V removeKey(K key)
{
throw new UnsupportedOperationException("Cannot call removeKey() on " + this.getClass().getSimpleName());
}
public V updateValue(K key, Function0 extends V> factory, Function super V, ? extends V> function)
{
throw new UnsupportedOperationException("Cannot call updateValue() on " + this.getClass().getSimpleName());
}
public V updateValueWith(
K key,
Function0 extends V> factory,
Function2 super V, ? super P, ? extends V> function,
P parameter)
{
throw new UnsupportedOperationException("Cannot call updateValueWith() on " + this.getClass().getSimpleName());
}
public V getIfAbsentPut(K key, Function0 extends V> function)
{
V result = this.get(key);
if (this.isAbsent(result, key))
{
throw new UnsupportedOperationException("Cannot mutate " + this.getClass().getSimpleName());
}
return result;
}
public V getIfAbsentPut(K key, V value)
{
V result = this.get(key);
if (this.isAbsent(result, key))
{
throw new UnsupportedOperationException("Cannot mutate " + this.getClass().getSimpleName());
}
return result;
}
public V getIfAbsentPutWithKey(K key, Function super K, ? extends V> function)
{
return this.getIfAbsentPutWith(key, function, key);
}
public
V getIfAbsentPutWith(
K key,
Function super P, ? extends V> function,
P parameter)
{
V result = this.get(key);
if (this.isAbsent(result, key))
{
throw new UnsupportedOperationException("Cannot mutate " + this.getClass().getSimpleName());
}
return result;
}
public V getIfAbsent(K key, Function0 extends V> function)
{
V result = this.get(key);
if (this.isAbsent(result, key))
{
return function.value();
}
return result;
}
public V getIfAbsentValue(K key, V value)
{
V result = this.get(key);
if (this.isAbsent(result, key))
{
return value;
}
return result;
}
public