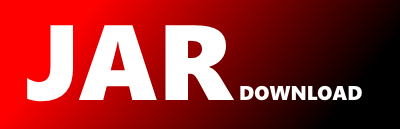
com.gs.collections.impl.multimap.bag.TreeBagMultimap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gs-collections Show documentation
Show all versions of gs-collections Show documentation
GS Collections is a collections framework for Java. It has JDK-compatible List, Set and Map
implementations with a rich API and set of utility classes that work with any JDK compatible Collections,
Arrays, Maps or Strings. The iteration protocol was inspired by the Smalltalk collection framework.
/*
* Copyright 2015 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.multimap.bag;
import java.io.Externalizable;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.Comparator;
import com.gs.collections.api.bag.sorted.ImmutableSortedBag;
import com.gs.collections.api.bag.sorted.MutableSortedBag;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.block.procedure.Procedure2;
import com.gs.collections.api.map.MutableMap;
import com.gs.collections.api.multimap.Multimap;
import com.gs.collections.api.multimap.bag.MutableBagMultimap;
import com.gs.collections.api.multimap.sortedbag.ImmutableSortedBagMultimap;
import com.gs.collections.api.multimap.sortedbag.MutableSortedBagMultimap;
import com.gs.collections.api.multimap.sortedbag.SortedBagMultimap;
import com.gs.collections.api.tuple.Pair;
import com.gs.collections.impl.bag.sorted.mutable.TreeBag;
import com.gs.collections.impl.map.mutable.UnifiedMap;
import com.gs.collections.impl.multimap.AbstractMutableMultimap;
import com.gs.collections.impl.multimap.bag.sorted.immutable.ImmutableSortedBagMultimapImpl;
import com.gs.collections.impl.multimap.list.FastListMultimap;
import com.gs.collections.impl.utility.Iterate;
/**
* @deprecated in 5.0. Use {@link com.gs.collections.impl.multimap.bag.sorted.mutable.TreeBagMultimap} instead.
*/
@Deprecated
public final class TreeBagMultimap
extends AbstractMutableMultimap>
implements MutableSortedBagMultimap, Externalizable
{
private static final long serialVersionUID = 1L;
private Comparator super V> comparator;
public TreeBagMultimap()
{
this.comparator = null;
}
public TreeBagMultimap(Comparator super V> comparator)
{
this.comparator = comparator;
}
public TreeBagMultimap(Multimap extends K, ? extends V> multimap)
{
super(Math.max(multimap.keysView().size() * 2, 16));
this.comparator = multimap instanceof SortedBagMultimap, ?> ? ((SortedBagMultimap) multimap).comparator() : null;
this.putAll(multimap);
}
public TreeBagMultimap(Pair... pairs)
{
super(pairs);
this.comparator = null;
}
public static TreeBagMultimap newMultimap()
{
return new TreeBagMultimap();
}
public static TreeBagMultimap newMultimap(Multimap extends K, ? extends V> multimap)
{
return new TreeBagMultimap(multimap);
}
public static TreeBagMultimap newMultimap(Comparator super V> comparator)
{
return new TreeBagMultimap(comparator);
}
public static TreeBagMultimap newMultimap(Pair... pairs)
{
return new TreeBagMultimap(pairs);
}
@Override
protected MutableMap> createMap()
{
return UnifiedMap.newMap();
}
@Override
protected MutableMap> createMapWithKeyCount(int keyCount)
{
return UnifiedMap.newMap(keyCount);
}
@Override
protected MutableSortedBag createCollection()
{
return TreeBag.newBag(this.comparator);
}
public TreeBagMultimap newEmpty()
{
return new TreeBagMultimap(this.comparator);
}
public Comparator super V> comparator()
{
return this.comparator;
}
public MutableSortedBagMultimap toMutable()
{
return new TreeBagMultimap(this);
}
public ImmutableSortedBagMultimap toImmutable()
{
final MutableMap> map = UnifiedMap.newMap();
this.map.forEachKeyValue(new Procedure2>()
{
public void value(K key, MutableSortedBag bag)
{
map.put(key, bag.toImmutable());
}
});
return new ImmutableSortedBagMultimapImpl(map, this.comparator());
}
@Override
public void writeExternal(ObjectOutput out) throws IOException
{
out.writeObject(this.comparator());
super.writeExternal(out);
}
@Override
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException
{
this.comparator = (Comparator super V>) in.readObject();
super.readExternal(in);
}
public MutableBagMultimap flip()
{
return Iterate.flip(this);
}
public TreeBagMultimap selectKeysValues(Predicate2 super K, ? super V> predicate)
{
return this.selectKeysValues(predicate, this.newEmpty());
}
public TreeBagMultimap rejectKeysValues(Predicate2 super K, ? super V> predicate)
{
return this.rejectKeysValues(predicate, this.newEmpty());
}
public TreeBagMultimap selectKeysMultiValues(Predicate2 super K, ? super Iterable> predicate)
{
return this.selectKeysMultiValues(predicate, this.newEmpty());
}
public TreeBagMultimap rejectKeysMultiValues(Predicate2 super K, ? super Iterable> predicate)
{
return this.rejectKeysMultiValues(predicate, this.newEmpty());
}
public HashBagMultimap collectKeysValues(Function2 super K, ? super V, Pair> function)
{
return this.collectKeysValues(function, HashBagMultimap.newMultimap());
}
public FastListMultimap collectValues(Function super V, ? extends V2> function)
{
return this.collectValues(function, FastListMultimap.newMultimap());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy