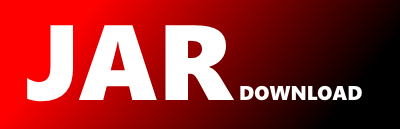
com.gs.collections.impl.utility.internal.RandomAccessListIterate Maven / Gradle / Ivy
Show all versions of gs-collections Show documentation
/*
* Copyright 2015 Goldman Sachs.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gs.collections.impl.utility.internal;
import java.io.IOException;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Collection;
import java.util.Comparator;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
import java.util.RandomAccess;
import com.gs.collections.api.RichIterable;
import com.gs.collections.api.block.HashingStrategy;
import com.gs.collections.api.block.function.Function;
import com.gs.collections.api.block.function.Function0;
import com.gs.collections.api.block.function.Function2;
import com.gs.collections.api.block.function.Function3;
import com.gs.collections.api.block.function.primitive.BooleanFunction;
import com.gs.collections.api.block.function.primitive.ByteFunction;
import com.gs.collections.api.block.function.primitive.CharFunction;
import com.gs.collections.api.block.function.primitive.DoubleFunction;
import com.gs.collections.api.block.function.primitive.DoubleObjectToDoubleFunction;
import com.gs.collections.api.block.function.primitive.FloatFunction;
import com.gs.collections.api.block.function.primitive.FloatObjectToFloatFunction;
import com.gs.collections.api.block.function.primitive.IntFunction;
import com.gs.collections.api.block.function.primitive.IntObjectToIntFunction;
import com.gs.collections.api.block.function.primitive.LongFunction;
import com.gs.collections.api.block.function.primitive.LongObjectToLongFunction;
import com.gs.collections.api.block.function.primitive.ShortFunction;
import com.gs.collections.api.block.predicate.Predicate;
import com.gs.collections.api.block.predicate.Predicate2;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.block.procedure.Procedure2;
import com.gs.collections.api.block.procedure.primitive.ObjectIntProcedure;
import com.gs.collections.api.collection.primitive.MutableBooleanCollection;
import com.gs.collections.api.collection.primitive.MutableByteCollection;
import com.gs.collections.api.collection.primitive.MutableCharCollection;
import com.gs.collections.api.collection.primitive.MutableDoubleCollection;
import com.gs.collections.api.collection.primitive.MutableFloatCollection;
import com.gs.collections.api.collection.primitive.MutableIntCollection;
import com.gs.collections.api.collection.primitive.MutableLongCollection;
import com.gs.collections.api.collection.primitive.MutableShortCollection;
import com.gs.collections.api.list.MutableList;
import com.gs.collections.api.list.primitive.MutableBooleanList;
import com.gs.collections.api.list.primitive.MutableByteList;
import com.gs.collections.api.list.primitive.MutableCharList;
import com.gs.collections.api.list.primitive.MutableDoubleList;
import com.gs.collections.api.list.primitive.MutableFloatList;
import com.gs.collections.api.list.primitive.MutableIntList;
import com.gs.collections.api.list.primitive.MutableLongList;
import com.gs.collections.api.list.primitive.MutableShortList;
import com.gs.collections.api.map.MutableMap;
import com.gs.collections.api.map.primitive.ObjectDoubleMap;
import com.gs.collections.api.map.primitive.ObjectLongMap;
import com.gs.collections.api.multimap.MutableMultimap;
import com.gs.collections.api.ordered.OrderedIterable;
import com.gs.collections.api.partition.list.PartitionMutableList;
import com.gs.collections.api.set.MutableSet;
import com.gs.collections.api.tuple.Pair;
import com.gs.collections.api.tuple.Twin;
import com.gs.collections.impl.block.factory.Functions0;
import com.gs.collections.impl.block.factory.HashingStrategies;
import com.gs.collections.impl.block.procedure.MutatingAggregationProcedure;
import com.gs.collections.impl.block.procedure.NonMutatingAggregationProcedure;
import com.gs.collections.impl.factory.Lists;
import com.gs.collections.impl.list.mutable.FastList;
import com.gs.collections.impl.list.mutable.primitive.BooleanArrayList;
import com.gs.collections.impl.list.mutable.primitive.ByteArrayList;
import com.gs.collections.impl.list.mutable.primitive.CharArrayList;
import com.gs.collections.impl.list.mutable.primitive.DoubleArrayList;
import com.gs.collections.impl.list.mutable.primitive.FloatArrayList;
import com.gs.collections.impl.list.mutable.primitive.IntArrayList;
import com.gs.collections.impl.list.mutable.primitive.LongArrayList;
import com.gs.collections.impl.list.mutable.primitive.ShortArrayList;
import com.gs.collections.impl.map.mutable.UnifiedMap;
import com.gs.collections.impl.map.mutable.primitive.ObjectDoubleHashMap;
import com.gs.collections.impl.map.mutable.primitive.ObjectLongHashMap;
import com.gs.collections.impl.multimap.list.FastListMultimap;
import com.gs.collections.impl.partition.list.PartitionFastList;
import com.gs.collections.impl.set.mutable.UnifiedSet;
import com.gs.collections.impl.set.strategy.mutable.UnifiedSetWithHashingStrategy;
import com.gs.collections.impl.tuple.Tuples;
import com.gs.collections.impl.utility.Iterate;
import com.gs.collections.impl.utility.ListIterate;
/**
* The ListIterate class provides a few of the methods from the Smalltalk Collection Protocol for use with ArrayLists.
* This includes do:, select:, reject:, collect:, inject:into:, detect:, detect:ifNone:, anySatisfy: and allSatisfy:
*/
public final class RandomAccessListIterate
{
private RandomAccessListIterate()
{
throw new AssertionError("Suppress default constructor for noninstantiability");
}
public static void toArray(List list, T[] target, int startIndex, int sourceSize)
{
for (int i = 0; i < sourceSize; i++)
{
target[startIndex + i] = list.get(i);
}
}
/**
* @see Iterate#select(Iterable, Predicate)
*/
public static MutableList select(List list, Predicate super T> predicate)
{
return RandomAccessListIterate.select(list, predicate, FastList.newList());
}
/**
* @see Iterate#selectWith(Iterable, Predicate2, Object)
*/
public static MutableList selectWith(
List list,
Predicate2 super T, ? super IV> predicate,
IV injectedValue)
{
return RandomAccessListIterate.selectWith(list, predicate, injectedValue, FastList.newList());
}
/**
* @see Iterate#select(Iterable, Predicate, Collection)
*/
public static > R select(
List list,
Predicate super T> predicate,
R targetCollection)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
T item = list.get(i);
if (predicate.accept(item))
{
targetCollection.add(item);
}
}
return targetCollection;
}
/**
* @see Iterate#selectWith(Iterable, Predicate2, Object, Collection)
*/
public static > R selectWith(
List list,
Predicate2 super T, ? super P> predicate,
P parameter,
R targetCollection)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
T item = list.get(i);
if (predicate.accept(item, parameter))
{
targetCollection.add(item);
}
}
return targetCollection;
}
/**
* @see Iterate#selectInstancesOf(Iterable, Class)
*/
public static MutableList selectInstancesOf(
List> list,
Class clazz)
{
int size = list.size();
FastList result = FastList.newList(size);
for (int i = 0; i < size; i++)
{
Object item = list.get(i);
if (clazz.isInstance(item))
{
result.add((T) item);
}
}
result.trimToSize();
return result;
}
/**
* @see Iterate#count(Iterable, Predicate)
*/
public static int count(
List list,
Predicate super T> predicate)
{
int count = 0;
int size = list.size();
for (int i = 0; i < size; i++)
{
if (predicate.accept(list.get(i)))
{
count++;
}
}
return count;
}
public static int countWith(
List list,
Predicate2 super T, ? super IV> predicate,
IV injectedValue)
{
int count = 0;
int size = list.size();
for (int i = 0; i < size; i++)
{
if (predicate.accept(list.get(i), injectedValue))
{
count++;
}
}
return count;
}
/**
* @see Iterate#collectIf(Iterable, Predicate, Function)
*/
public static MutableList collectIf(
List list,
Predicate super T> predicate,
Function super T, ? extends A> function)
{
return RandomAccessListIterate.collectIf(list, predicate, function, FastList.newList());
}
/**
* @see Iterate#collectIf(Iterable, Predicate, Function, Collection)
*/
public static > R collectIf(
List list,
Predicate super T> predicate,
Function super T, ? extends A> function,
R targetCollection)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
T item = list.get(i);
if (predicate.accept(item))
{
targetCollection.add(function.valueOf(item));
}
}
return targetCollection;
}
/**
* @see Iterate#reject(Iterable, Predicate)
*/
public static MutableList reject(List list, Predicate super T> predicate)
{
return RandomAccessListIterate.reject(list, predicate, FastList.newList());
}
/**
* @see Iterate#rejectWith(Iterable, Predicate2, Object)
*/
public static MutableList rejectWith(
List list,
Predicate2 super T, ? super IV> predicate,
IV injectedValue)
{
return RandomAccessListIterate.rejectWith(list, predicate, injectedValue, FastList.newList());
}
/**
* @see Iterate#reject(Iterable, Predicate, Collection)
*/
public static > R reject(
List list,
Predicate super T> predicate,
R targetCollection)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
T item = list.get(i);
if (!predicate.accept(item))
{
targetCollection.add(item);
}
}
return targetCollection;
}
/**
* @see Iterate#reject(Iterable, Predicate, Collection)
*/
public static > R rejectWith(
List list,
Predicate2 super T, ? super P> predicate,
P parameter,
R targetCollection)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
T item = list.get(i);
if (!predicate.accept(item, parameter))
{
targetCollection.add(item);
}
}
return targetCollection;
}
/**
* @see Iterate#collect(Iterable, Function)
*/
public static MutableList collect(
List list,
Function super T, ? extends A> function)
{
return RandomAccessListIterate.collect(list, function, FastList.newList(list.size()));
}
/**
* @see Iterate#collect(Iterable, Function, Collection)
*/
public static > R collect(
List list,
Function super T, ? extends A> function,
R targetCollection)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
targetCollection.add(function.valueOf(list.get(i)));
}
return targetCollection;
}
/**
* @see Iterate#collectBoolean(Iterable, BooleanFunction)
*/
public static MutableBooleanList collectBoolean(
List list,
BooleanFunction super T> booleanFunction)
{
return RandomAccessListIterate.collectBoolean(list, booleanFunction, new BooleanArrayList(list.size()));
}
/**
* @see Iterate#collectBoolean(Iterable, BooleanFunction)
*/
public static R collectBoolean(
List list,
BooleanFunction super T> booleanFunction,
R target)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
target.add(booleanFunction.booleanValueOf(list.get(i)));
}
return target;
}
/**
* @see Iterate#collectByte(Iterable, ByteFunction)
*/
public static MutableByteList collectByte(
List list,
ByteFunction super T> byteFunction)
{
return RandomAccessListIterate.collectByte(list, byteFunction, new ByteArrayList(list.size()));
}
/**
* @see Iterate#collectByte(Iterable, ByteFunction)
*/
public static R collectByte(
List list,
ByteFunction super T> byteFunction,
R target)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
target.add(byteFunction.byteValueOf(list.get(i)));
}
return target;
}
/**
* @see Iterate#collectChar(Iterable, CharFunction)
*/
public static MutableCharList collectChar(
List list,
CharFunction super T> charFunction)
{
return RandomAccessListIterate.collectChar(list, charFunction, new CharArrayList(list.size()));
}
/**
* @see Iterate#collectChar(Iterable, CharFunction)
*/
public static R collectChar(
List list,
CharFunction super T> charFunction,
R target)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
target.add(charFunction.charValueOf(list.get(i)));
}
return target;
}
/**
* @see Iterate#collectDouble(Iterable, DoubleFunction)
*/
public static MutableDoubleList collectDouble(
List list,
DoubleFunction super T> doubleFunction)
{
return RandomAccessListIterate.collectDouble(list, doubleFunction, new DoubleArrayList(list.size()));
}
/**
* @see Iterate#collectDouble(Iterable, DoubleFunction)
*/
public static R collectDouble(
List list,
DoubleFunction super T> doubleFunction,
R target)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
target.add(doubleFunction.doubleValueOf(list.get(i)));
}
return target;
}
/**
* @see Iterate#collectFloat(Iterable, FloatFunction)
*/
public static MutableFloatList collectFloat(
List list,
FloatFunction super T> floatFunction)
{
return RandomAccessListIterate.collectFloat(list, floatFunction, new FloatArrayList(list.size()));
}
/**
* @see Iterate#collectFloat(Iterable, FloatFunction)
*/
public static R collectFloat(
List list,
FloatFunction super T> floatFunction,
R target)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
target.add(floatFunction.floatValueOf(list.get(i)));
}
return target;
}
/**
* @see Iterate#collectInt(Iterable, IntFunction)
*/
public static MutableIntList collectInt(
List list,
IntFunction super T> intFunction)
{
return RandomAccessListIterate.collectInt(list, intFunction, new IntArrayList(list.size()));
}
/**
* @see Iterate#collectInt(Iterable, IntFunction)
*/
public static R collectInt(
List list,
IntFunction super T> intFunction,
R target)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
target.add(intFunction.intValueOf(list.get(i)));
}
return target;
}
/**
* @see Iterate#collectLong(Iterable, LongFunction)
*/
public static MutableLongList collectLong(
List list,
LongFunction super T> longFunction)
{
return RandomAccessListIterate.collectLong(list, longFunction, new LongArrayList(list.size()));
}
/**
* @see Iterate#collectLong(Iterable, LongFunction)
*/
public static R collectLong(
List list,
LongFunction super T> longFunction,
R target)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
target.add(longFunction.longValueOf(list.get(i)));
}
return target;
}
/**
* @see Iterate#collectShort(Iterable, ShortFunction)
*/
public static MutableShortList collectShort(
List list,
ShortFunction super T> shortFunction)
{
return RandomAccessListIterate.collectShort(list, shortFunction, new ShortArrayList(list.size()));
}
/**
* @see Iterate#collectShort(Iterable, ShortFunction)
*/
public static R collectShort(
List list,
ShortFunction super T> shortFunction,
R target)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
target.add(shortFunction.shortValueOf(list.get(i)));
}
return target;
}
/**
* @see Iterate#flatCollect(Iterable, Function)
*/
public static MutableList flatCollect(
List list,
Function super T, ? extends Iterable> function)
{
return RandomAccessListIterate.flatCollect(list, function, FastList.newList(list.size()));
}
/**
* @see Iterate#flatCollect(Iterable, Function, Collection)
*/
public static > R flatCollect(
List list,
Function super T, ? extends Iterable> function,
R targetCollection)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
Iterate.addAllTo(function.valueOf(list.get(i)), targetCollection);
}
return targetCollection;
}
/**
* Returns the last element of a list.
*/
public static T getLast(List collection)
{
return Iterate.isEmpty(collection) ? null : collection.get(collection.size() - 1);
}
/**
* @see Iterate#forEach(Iterable, Procedure)
*/
public static void forEach(List list, Procedure super T> procedure)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
procedure.value(list.get(i));
}
}
/**
* Iterates over the section of the list covered by the specified indexes. The indexes are both inclusive. If the
* from is less than the to, the list is iterated in forward order. If the from is greater than the to, then the
* list is iterated in the reverse order.
*
*
e.g.
* MutableList people = FastList.newListWith(ted, mary, bob, sally);
* ListIterate.forEach(people, 0, 1, new Procedure()
* {
* public void value(Person person)
* {
* LOGGER.info(person.getName());
* }
* });
*
*
* This code would output ted and mary's names.
*/
public static void forEach(List list, int from, int to, Procedure super T> procedure)
{
ListIterate.rangeCheck(from, to, list.size());
if (from <= to)
{
for (int i = from; i <= to; i++)
{
procedure.value(list.get(i));
}
}
else
{
for (int i = from; i >= to; i--)
{
procedure.value(list.get(i));
}
}
}
/**
* Iterates over the section of the list covered by the specified indexes. The indexes are both inclusive. If the
* from is less than the to, the list is iterated in forward order. If the from is greater than the to, then the
* list is iterated in the reverse order. The index passed into the ObjectIntProcedure is the actual index of the
* range.
*
*
e.g.
* MutableList people = FastList.newListWith(ted, mary, bob, sally);
* ListIterate.forEachWithIndex(people, 0, 1, new ObjectIntProcedure()
* {
* public void value(Person person, int index)
* {
* LOGGER.info(person.getName() + " at index: " + index);
* }
* });
*
*
* This code would output ted and mary's names.
*/
public static void forEachWithIndex(List list, int from, int to, ObjectIntProcedure super T> objectIntProcedure)
{
ListIterate.rangeCheck(from, to, list.size());
if (from <= to)
{
for (int i = from; i <= to; i++)
{
objectIntProcedure.value(list.get(i), i);
}
}
else
{
for (int i = from; i >= to; i--)
{
objectIntProcedure.value(list.get(i), i);
}
}
}
/**
* For each element in both of the Lists, operation is evaluated with both elements as parameters.
*/
public static void forEachInBoth(List list1, List list2, Procedure2 super T1, ? super T2> procedure)
{
if (list1 != null && list2 != null)
{
int size1 = list1.size();
int size2 = list2.size();
if (size1 == size2)
{
for (int i = 0; i < size1; i++)
{
procedure.value(list1.get(i), list2.get(i));
}
}
else
{
throw new IllegalArgumentException("Attempt to call forEachInBoth with two Lists of different sizes :"
+ size1
+ ':'
+ size2);
}
}
}
/**
* Iterates over a collection passing each element and the current relative int index to the specified instance of
* ObjectIntProcedure.
*/
public static void forEachWithIndex(List list, ObjectIntProcedure super T> objectIntProcedure)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
objectIntProcedure.value(list.get(i), i);
}
}
public static IV injectInto(IV injectValue, List list, Function2 super IV, ? super T, ? extends IV> function)
{
IV result = injectValue;
int size = list.size();
for (int i = 0; i < size; i++)
{
result = function.value(result, list.get(i));
}
return result;
}
public static int injectInto(int injectValue, List list, IntObjectToIntFunction super T> function)
{
int result = injectValue;
int size = list.size();
for (int i = 0; i < size; i++)
{
result = function.intValueOf(result, list.get(i));
}
return result;
}
public static long injectInto(long injectValue, List list, LongObjectToLongFunction super T> function)
{
long result = injectValue;
int size = list.size();
for (int i = 0; i < size; i++)
{
result = function.longValueOf(result, list.get(i));
}
return result;
}
public static double injectInto(double injectValue, List list, DoubleObjectToDoubleFunction super T> function)
{
double result = injectValue;
int size = list.size();
for (int i = 0; i < size; i++)
{
result = function.doubleValueOf(result, list.get(i));
}
return result;
}
public static float injectInto(float injectValue, List list, FloatObjectToFloatFunction super T> function)
{
float result = injectValue;
int size = list.size();
for (int i = 0; i < size; i++)
{
result = function.floatValueOf(result, list.get(i));
}
return result;
}
public static long sumOfInt(List list, IntFunction super T> function)
{
long result = 0;
for (int i = 0; i < list.size(); i++)
{
result += (long) function.intValueOf(list.get(i));
}
return result;
}
public static long sumOfLong(List list, LongFunction super T> function)
{
long result = 0L;
for (int i = 0; i < list.size(); i++)
{
result += function.longValueOf(list.get(i));
}
return result;
}
public static double sumOfFloat(List list, FloatFunction super T> function)
{
double sum = 0.0d;
double compensation = 0.0d;
for (int i = 0; i < list.size(); i++)
{
double adjustedValue = (double) function.floatValueOf(list.get(i)) - compensation;
double nextSum = sum + adjustedValue;
compensation = nextSum - sum - adjustedValue;
sum = nextSum;
}
return sum;
}
public static double sumOfDouble(List list, DoubleFunction super T> function)
{
double sum = 0.0d;
double compensation = 0.0d;
for (int i = 0; i < list.size(); i++)
{
double adjustedValue = function.doubleValueOf(list.get(i)) - compensation;
double nextSum = sum + adjustedValue;
compensation = nextSum - sum - adjustedValue;
sum = nextSum;
}
return sum;
}
public static BigDecimal sumOfBigDecimal(List list, Function super T, BigDecimal> function)
{
BigDecimal result = BigDecimal.ZERO;
int size = list.size();
for (int i = 0; i < size; i++)
{
result = result.add(function.valueOf(list.get(i)));
}
return result;
}
public static BigInteger sumOfBigInteger(List list, Function super T, BigInteger> function)
{
BigInteger result = BigInteger.ZERO;
int size = list.size();
for (int i = 0; i < size; i++)
{
result = result.add(function.valueOf(list.get(i)));
}
return result;
}
public static MutableMap sumByBigDecimal(List list, Function groupBy, final Function super T, BigDecimal> function)
{
MutableMap result = UnifiedMap.newMap();
int size = list.size();
for (int i = 0; i < size; i++)
{
final T item = list.get(i);
result.updateValue(groupBy.valueOf(item), Functions0.zeroBigDecimal(), new Function()
{
public BigDecimal valueOf(BigDecimal original)
{
return original.add(function.valueOf(item));
}
});
}
return result;
}
public static MutableMap sumByBigInteger(List list, Function groupBy, final Function super T, BigInteger> function)
{
MutableMap result = UnifiedMap.newMap();
int size = list.size();
for (int i = 0; i < size; i++)
{
final T item = list.get(i);
result.updateValue(groupBy.valueOf(item), Functions0.zeroBigInteger(), new Function()
{
public BigInteger valueOf(BigInteger original)
{
return original.add(function.valueOf(item));
}
});
}
return result;
}
public static boolean shortCircuit(
List list,
Predicate super T> predicate,
boolean expected,
boolean onShortCircuit,
boolean atEnd)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
if (predicate.accept(list.get(i)) == expected)
{
return onShortCircuit;
}
}
return atEnd;
}
public static boolean shortCircuitWith(
List list,
Predicate2 super T, ? super P> predicate2,
P parameter,
boolean expected,
boolean onShortCircuit,
boolean atEnd)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
if (predicate2.accept(list.get(i), parameter) == expected)
{
return onShortCircuit;
}
}
return atEnd;
}
public static boolean corresponds(List list, OrderedIterable other, Predicate2 super T, ? super P> predicate)
{
if (!(list instanceof RandomAccess))
{
throw new IllegalArgumentException();
}
int size = list.size();
if (size != other.size())
{
return false;
}
if (other instanceof RandomAccess)
{
List
otherList = (List
) other;
for (int index = 0; index < size; index++)
{
if (!predicate.accept(list.get(index), otherList.get(index)))
{
return false;
}
}
return true;
}
Iterator
iterator = other.iterator();
for (int index = 0; index < size; index++)
{
if (!predicate.accept(list.get(index), iterator.next()))
{
return false;
}
}
return true;
}
public static boolean anySatisfy(List list, Predicate super T> predicate)
{
return RandomAccessListIterate.shortCircuit(list, predicate, true, true, false);
}
public static boolean anySatisfyWith(List list, Predicate2 super T, ? super P> predicate, P parameter)
{
return RandomAccessListIterate.shortCircuitWith(list, predicate, parameter, true, true, false);
}
public static boolean allSatisfy(List list, Predicate super T> predicate)
{
return RandomAccessListIterate.shortCircuit(list, predicate, false, false, true);
}
public static boolean allSatisfyWith(List list, Predicate2 super T, ? super P> predicate, P parameter)
{
return RandomAccessListIterate.shortCircuitWith(list, predicate, parameter, false, false, true);
}
public static boolean noneSatisfy(List list, Predicate super T> predicate)
{
return RandomAccessListIterate.shortCircuit(list, predicate, true, false, true);
}
public static boolean noneSatisfyWith(List list, Predicate2 super T, ? super P> predicate, P parameter)
{
return RandomAccessListIterate.shortCircuitWith(list, predicate, parameter, true, false, true);
}
public static T detect(List list, Predicate super T> predicate)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
T each = list.get(i);
if (predicate.accept(each))
{
return each;
}
}
return null;
}
public static T detectWith(List list, Predicate2 super T, ? super P> predicate, P parameter)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
T each = list.get(i);
if (predicate.accept(each, parameter))
{
return each;
}
}
return null;
}
public static Twin> selectAndRejectWith(
List list,
Predicate2 super T, ? super IV> predicate,
IV injectedValue)
{
MutableList positiveResult = Lists.mutable.empty();
MutableList negativeResult = Lists.mutable.empty();
int size = list.size();
for (int i = 0; i < size; i++)
{
T item = list.get(i);
(predicate.accept(item, injectedValue) ? positiveResult : negativeResult).add(item);
}
return Tuples.twin(positiveResult, negativeResult);
}
public static PartitionMutableList partition(List list, Predicate super T> predicate)
{
PartitionFastList partitionFastList = new PartitionFastList();
int size = list.size();
for (int i = 0; i < size; i++)
{
T each = list.get(i);
MutableList bucket = predicate.accept(each) ? partitionFastList.getSelected() : partitionFastList.getRejected();
bucket.add(each);
}
return partitionFastList;
}
public static PartitionMutableList partitionWith(List list, Predicate2 super T, ? super P> predicate, P parameter)
{
PartitionFastList partitionFastList = new PartitionFastList();
int size = list.size();
for (int i = 0; i < size; i++)
{
T each = list.get(i);
MutableList bucket = predicate.accept(each, parameter) ? partitionFastList.getSelected() : partitionFastList.getRejected();
bucket.add(each);
}
return partitionFastList;
}
public static boolean removeIf(List list, Predicate super T> predicate)
{
boolean changed = false;
for (int i = 0; i < list.size(); i++)
{
T each = list.get(i);
if (predicate.accept(each))
{
list.remove(i--);
changed = true;
}
}
return changed;
}
public static boolean removeIfWith(List list, Predicate2 super T, ? super P> predicate, P parameter)
{
boolean changed = false;
for (int i = 0; i < list.size(); i++)
{
T each = list.get(i);
if (predicate.accept(each, parameter))
{
list.remove(i--);
changed = true;
}
}
return changed;
}
public static boolean removeIf(List list, Predicate super T> predicate, Procedure super T> procedure)
{
boolean changed = false;
for (int i = 0; i < list.size(); i++)
{
T each = list.get(i);
if (predicate.accept(each))
{
procedure.value(each);
list.remove(i--);
changed = true;
}
}
return changed;
}
public static boolean removeIfWith(List list, Predicate2 super T, ? super P> predicate, P parameter, Procedure super T> procedure)
{
boolean changed = false;
for (int i = 0; i < list.size(); i++)
{
T each = list.get(i);
if (predicate.accept(each, parameter))
{
procedure.value(each);
list.remove(i--);
changed = true;
}
}
return changed;
}
/**
* Searches for the first occurrence where the predicate evaluates to true.
*
* @see Iterate#detectIndex(Iterable, Predicate)
*/
public static int detectIndex(List list, Predicate super T> predicate)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
if (predicate.accept(list.get(i)))
{
return i;
}
}
return -1;
}
/**
* Searches for the first occurrence where the predicate evaluates to true.
*
* @see Iterate#detectIndexWith(Iterable, Predicate2, Object)
*/
public static int detectIndexWith(List list, Predicate2 super T, ? super IV> predicate, IV injectedValue)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
if (predicate.accept(list.get(i), injectedValue))
{
return i;
}
}
return -1;
}
public static int detectLastIndex(List list, Predicate super T> predicate)
{
int size = list.size();
for (int i = size - 1; i >= 0; i--)
{
if (predicate.accept(list.get(i)))
{
return i;
}
}
return -1;
}
public static IV injectIntoWith(
IV injectedValue,
List list,
Function3 super IV, ? super T, ? super P, ? extends IV> function,
P parameter)
{
IV result = injectedValue;
int size = list.size();
for (int i = 0; i < size; i++)
{
result = function.value(result, list.get(i), parameter);
}
return result;
}
public static void forEachWith(List list, Procedure2 super T, ? super P> procedure, P parameter)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
procedure.value(list.get(i), parameter);
}
}
public static > R collectWith(
List list,
Function2 super T, ? super P, ? extends A> function,
P parameter,
R targetCollection)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
targetCollection.add(function.value(list.get(i), parameter));
}
return targetCollection;
}
/**
* @deprecated in 7.0.
*/
@Deprecated
public static > R distinct(List list, R targetList)
{
MutableSet seenSoFar = UnifiedSet.newSet();
int size = list.size();
for (int i = 0; i < size; i++)
{
T item = list.get(i);
if (seenSoFar.add(item))
{
targetList.add(item);
}
}
return targetList;
}
/**
* @since 7.0.
*/
public static MutableList distinct(List list)
{
return RandomAccessListIterate.distinct(list, FastList.newList());
}
/**
* @since 7.0.
*/
public static MutableList distinct(List list, HashingStrategy super T> hashingStrategy)
{
MutableSet seenSoFar = UnifiedSetWithHashingStrategy.newSet(hashingStrategy);
FastList result = FastList.newList();
int size = list.size();
for (int i = 0; i < size; i++)
{
T item = list.get(i);
if (seenSoFar.add(item))
{
result.add(item);
}
}
return result;
}
/**
* @see Iterate#take(Iterable, int)
*/
public static MutableList take(List list, int count)
{
if (count < 0)
{
throw new IllegalArgumentException("Count must be greater than zero, but was: " + count);
}
return RandomAccessListIterate.take(list, count, FastList.newList(Math.min(list.size(), count)));
}
/**
* @see Iterate#take(Iterable, int)
*/
public static > R take(List list, int count, R targetList)
{
if (count < 0)
{
throw new IllegalArgumentException("Count must be greater than zero, but was: " + count);
}
int end = Math.min(list.size(), count);
for (int i = 0; i < end; i++)
{
targetList.add(list.get(i));
}
return targetList;
}
/**
* @see Iterate#drop(Iterable, int)
*/
public static MutableList drop(List list, int count)
{
if (count < 0)
{
throw new IllegalArgumentException("Count must be greater than zero, but was: " + count);
}
return RandomAccessListIterate.drop(list, count, FastList.newList(list.size() - Math.min(list.size(), count)));
}
/**
* @see Iterate#drop(Iterable, int)
*/
public static > R drop(List list, int count, R targetList)
{
if (count < 0)
{
throw new IllegalArgumentException("Count must be greater than zero, but was: " + count);
}
if (count >= list.size())
{
return targetList;
}
int start = Math.min(list.size(), count);
targetList.addAll(list.subList(start, list.size()));
return targetList;
}
/**
* @see RichIterable#appendString(Appendable, String, String, String)
*/
public static void appendString(
List list,
Appendable appendable,
String start,
String separator,
String end)
{
try
{
appendable.append(start);
if (Iterate.notEmpty(list))
{
appendable.append(IterableIterate.stringValueOfItem(list, list.get(0)));
int size = list.size();
for (int i = 1; i < size; i++)
{
appendable.append(separator);
appendable.append(IterableIterate.stringValueOfItem(list, list.get(i)));
}
}
appendable.append(end);
}
catch (IOException e)
{
throw new RuntimeException(e);
}
}
/**
* @see Iterate#groupBy(Iterable, Function)
*/
public static FastListMultimap groupBy(
List list,
Function super T, ? extends V> function)
{
return RandomAccessListIterate.groupBy(list, function, FastListMultimap.newMultimap());
}
/**
* @see Iterate#groupBy(Iterable, Function, MutableMultimap)
*/
public static > R groupBy(
List list,
Function super T, ? extends V> function,
R target)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
T item = list.get(i);
target.put(function.valueOf(item), item);
}
return target;
}
public static FastListMultimap groupByEach(
List list,
Function super T, ? extends Iterable> function)
{
return RandomAccessListIterate.groupByEach(list, function, FastListMultimap.newMultimap());
}
public static > R groupByEach(
List list,
Function super T, ? extends Iterable> function,
R target)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
T item = list.get(i);
Iterable iterable = function.valueOf(item);
for (V key : iterable)
{
target.put(key, item);
}
}
return target;
}
public static MutableMap groupByUniqueKey(
List list,
Function super T, ? extends K> function)
{
return RandomAccessListIterate.groupByUniqueKey(list, function, UnifiedMap.newMap());
}
public static > R groupByUniqueKey(
List list,
Function super T, ? extends K> function,
R target)
{
int size = list.size();
for (int i = 0; i < size; i++)
{
T value = list.get(i);
K key = function.valueOf(value);
if (target.put(key, value) != null)
{
throw new IllegalStateException("Key " + key + " already exists in map!");
}
}
return target;
}
public static > T minBy(List list, Function super T, ? extends V> function)
{
if (list.isEmpty())
{
throw new NoSuchElementException();
}
T min = list.get(0);
V minValue = function.valueOf(min);
int size = list.size();
for (int i = 1; i < size; i++)
{
T next = list.get(i);
V nextValue = function.valueOf(next);
if (nextValue.compareTo(minValue) < 0)
{
min = next;
minValue = nextValue;
}
}
return min;
}
public static > T maxBy(List list, Function super T, ? extends V> function)
{
if (list.isEmpty())
{
throw new NoSuchElementException();
}
T max = list.get(0);
V maxValue = function.valueOf(max);
int size = list.size();
for (int i = 1; i < size; i++)
{
T next = list.get(i);
V nextValue = function.valueOf(next);
if (nextValue.compareTo(maxValue) > 0)
{
max = next;
maxValue = nextValue;
}
}
return max;
}
public static T min(List list, Comparator super T> comparator)
{
if (list.isEmpty())
{
throw new NoSuchElementException();
}
T min = list.get(0);
int size = list.size();
for (int i = 1; i < size; i++)
{
T item = list.get(i);
if (comparator.compare(item, min) < 0)
{
min = item;
}
}
return min;
}
public static T max(List list, Comparator super T> comparator)
{
if (list.isEmpty())
{
throw new NoSuchElementException();
}
T max = list.get(0);
int size = list.size();
for (int i = 1; i < size; i++)
{
T item = list.get(i);
if (comparator.compare(item, max) > 0)
{
max = item;
}
}
return max;
}
public static T min(List