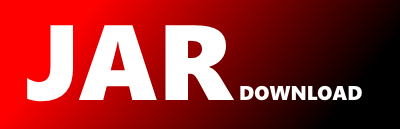
com.gooddata.md.ReportAttachment Maven / Gradle / Ivy
/*
* Copyright (C) 2004-2017, GoodData(R) Corporation. All rights reserved.
* This source code is licensed under the BSD-style license found in the
* LICENSE.txt file in the root directory of this source tree.
*/
package com.gooddata.md;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.gooddata.export.ExportFormat;
import com.gooddata.util.GoodDataToStringBuilder;
import java.util.Arrays;
import java.util.Collection;
import java.util.Map;
/**
* Attachment to {@link ScheduledMail} represents report-related information for the schedule.
*/
public class ReportAttachment extends Attachment {
private final Collection formats;
private final Map exportOptions;
@JsonCreator
protected ReportAttachment(
@JsonProperty("uri") String uri,
@JsonProperty("exportOptions") Map exportOptions,
@JsonProperty("formats") String... formats
) {
super(uri);
this.exportOptions = exportOptions;
this.formats = Arrays.asList(formats);
}
protected ReportAttachment(String uri, Map exportOptions, ExportFormat... formats) {
this(uri, exportOptions, ExportFormat.arrayToStringArray(formats));
}
/**
* Options which modify default export behavior. Due to variety of
* export formats options only work for explicitly listed
* format types.
*
*
* - pageOrientation
*
* - set page orientation
* - default value: 'portrait'
* - supported in: tabular PDF
*
*
*
* - optimalColumnWidth
*
* - set 'yes' to automatically resize all columns to fit cell's content
* - default is: 'no' (do not auto resize)
* - supported in: tabular PDF
*
*
*
* - pageScalePercentage
*
* - down-scaling factor (in percent), 100 means no scale-down
* - may not be combined with scaleToPages, scaleToPagesX and scaleToPagesY
* - default is: 100
* - supported in: tabular PDF
*
*
*
* - scaleToPages
*
* - total number of pages of target PDF file
* - may not be combined with pageScalePercentage, scaleToPagesX and scaleToPagesY
* - default is: unlimited
* - supported in: tabular PDF
*
*
*
* - scaleToPagesX
*
* - number of horizontal pages of target PDF file
* - may not be combined with pageScalePercentage and scaleToPages, but may be combined with scaleToPagesY
* - default is: unlimited
* - supported in: tabular PDF
*
*
*
* - scaleToPagesY
*
* - number of vertical pages of target PDF file
* - may not be combined with pageScalePercentage and scaleToPages, but may be combined with scaleToPagesX
* - default is: unlimited
* - supported in: tabular PDF
*
*
*
*
* @return map of export options
*/
public Map getExportOptions() { return exportOptions; }
public Collection getFormats() { return formats; }
@Override
public boolean equals(Object o) {
if (!super.equals(o)) return false;
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ReportAttachment that = (ReportAttachment) o;
if (formats != null ? !formats.equals(that.formats) : that.formats != null) return false;
if (getUri() != null ? !getUri().equals(that.getUri()) : that.getUri() != null) return false;
return !(exportOptions != null ? !exportOptions.equals(that.exportOptions) : that.exportOptions != null);
}
@Override
public int hashCode() {
int result = formats != null ? formats.hashCode() : 0;
result = 31 * result + (exportOptions != null ? exportOptions.hashCode() : 0);
result = 31 * result + (getUri() != null ? getUri().hashCode() : 0);
return result;
}
@Override
public String toString() {
return new GoodDataToStringBuilder(this).append("uri", getUri()).toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy