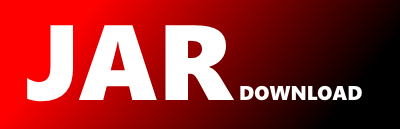
com.gooddata.executeafm.afm.Afm Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2017, GoodData(R) Corporation. All rights reserved.
* This source code is licensed under the BSD-style license found in the
* LICENSE.txt file in the root directory of this source tree.
*/
package com.gooddata.executeafm.afm;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.gooddata.util.GoodDataToStringBuilder;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import static com.gooddata.util.Validate.notNull;
import static java.lang.String.format;
/**
* Attributes Filters and Measures in so called object form (could have MAQL form in future)
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
public class Afm {
private List attributes;
private List filters;
private List measures;
private List nativeTotals;
@JsonCreator
public Afm(@JsonProperty("attributes") final List attributes,
@JsonProperty("filters") final List filters,
@JsonProperty("measures") final List measures,
@JsonProperty("nativeTotals") final List nativeTotals) {
this.attributes = attributes;
this.filters = filters;
this.measures = measures;
this.nativeTotals = nativeTotals;
}
public Afm() {
}
/**
* Find {@link AttributeItem} within attributes by given localIdentifier
* @param localIdentifier identifier used for search
* @return found attribute or throws exception
*/
@JsonIgnore
public AttributeItem getAttribute(final String localIdentifier) {
return getIdentifiable(attributes, localIdentifier);
}
/**
* Find {@link MeasureItem} within measures by given localIdentifier
* @param localIdentifier identifier used for search
* @return found measure or throws exception
*/
@JsonIgnore
public MeasureItem getMeasure(final String localIdentifier) {
return getIdentifiable(measures, localIdentifier);
}
public List getAttributes() {
return attributes;
}
public void setAttributes(final List attributes) {
this.attributes = attributes;
}
public Afm addAttribute(final AttributeItem attribute) {
if (attributes == null) {
setAttributes(new ArrayList<>());
}
attributes.add(notNull(attribute, "attribute"));
return this;
}
public List getFilters() {
return filters;
}
public void setFilters(final List filters) {
this.filters = filters;
}
public Afm addFilter(final CompatibilityFilter filter) {
if (filters == null) {
setFilters(new ArrayList<>());
}
filters.add(notNull(filter, "filter"));
return this;
}
public List getMeasures() {
return measures;
}
public void setMeasures(final List measures) {
this.measures = measures;
}
public Afm addMeasure(final MeasureItem measure) {
if (measures == null) {
setMeasures(new ArrayList<>());
}
measures.add(notNull(measure, "measure"));
return this;
}
public List getNativeTotals() {
return nativeTotals;
}
public void setNativeTotals(final List nativeTotals) {
this.nativeTotals = nativeTotals;
}
public Afm addNativeTotal(final NativeTotalItem total) {
if (nativeTotals == null) {
setNativeTotals(new ArrayList<>());
}
nativeTotals.add(notNull(total, "total"));
return this;
}
@Override
public String toString() {
return GoodDataToStringBuilder.defaultToString(this);
}
private static T getIdentifiable(final List toSearch, final String localIdentifier) {
return Optional.ofNullable(toSearch)
.flatMap(a -> a.stream().filter(i -> Objects.equals(localIdentifier, i.getLocalIdentifier())).findFirst())
.orElseThrow(() -> new IllegalArgumentException(format("Item of localIdentifier=%s not found", localIdentifier)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy