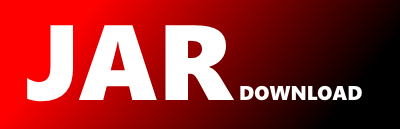
com.google.analytics.data.v1alpha.stub.AlphaAnalyticsDataStub Maven / Gradle / Ivy
Show all versions of google-analytics-data Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.analytics.data.v1alpha.stub;
import static com.google.analytics.data.v1alpha.AlphaAnalyticsDataClient.ListAudienceListsPagedResponse;
import static com.google.analytics.data.v1alpha.AlphaAnalyticsDataClient.ListRecurringAudienceListsPagedResponse;
import com.google.analytics.data.v1alpha.AudienceList;
import com.google.analytics.data.v1alpha.AudienceListMetadata;
import com.google.analytics.data.v1alpha.CreateAudienceListRequest;
import com.google.analytics.data.v1alpha.CreateRecurringAudienceListRequest;
import com.google.analytics.data.v1alpha.GetAudienceListRequest;
import com.google.analytics.data.v1alpha.GetRecurringAudienceListRequest;
import com.google.analytics.data.v1alpha.ListAudienceListsRequest;
import com.google.analytics.data.v1alpha.ListAudienceListsResponse;
import com.google.analytics.data.v1alpha.ListRecurringAudienceListsRequest;
import com.google.analytics.data.v1alpha.ListRecurringAudienceListsResponse;
import com.google.analytics.data.v1alpha.QueryAudienceListRequest;
import com.google.analytics.data.v1alpha.QueryAudienceListResponse;
import com.google.analytics.data.v1alpha.RecurringAudienceList;
import com.google.analytics.data.v1alpha.RunFunnelReportRequest;
import com.google.analytics.data.v1alpha.RunFunnelReportResponse;
import com.google.analytics.data.v1alpha.SheetExportAudienceListRequest;
import com.google.analytics.data.v1alpha.SheetExportAudienceListResponse;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.OperationsStub;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Base stub class for the AlphaAnalyticsData service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@BetaApi
@Generated("by gapic-generator-java")
public abstract class AlphaAnalyticsDataStub implements BackgroundResource {
public OperationsStub getOperationsStub() {
return null;
}
public com.google.api.gax.httpjson.longrunning.stub.OperationsStub getHttpJsonOperationsStub() {
return null;
}
public UnaryCallable runFunnelReportCallable() {
throw new UnsupportedOperationException("Not implemented: runFunnelReportCallable()");
}
public OperationCallable
createAudienceListOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: createAudienceListOperationCallable()");
}
public UnaryCallable createAudienceListCallable() {
throw new UnsupportedOperationException("Not implemented: createAudienceListCallable()");
}
public UnaryCallable
queryAudienceListCallable() {
throw new UnsupportedOperationException("Not implemented: queryAudienceListCallable()");
}
public UnaryCallable
sheetExportAudienceListCallable() {
throw new UnsupportedOperationException("Not implemented: sheetExportAudienceListCallable()");
}
public UnaryCallable getAudienceListCallable() {
throw new UnsupportedOperationException("Not implemented: getAudienceListCallable()");
}
public UnaryCallable
listAudienceListsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listAudienceListsPagedCallable()");
}
public UnaryCallable
listAudienceListsCallable() {
throw new UnsupportedOperationException("Not implemented: listAudienceListsCallable()");
}
public UnaryCallable
createRecurringAudienceListCallable() {
throw new UnsupportedOperationException(
"Not implemented: createRecurringAudienceListCallable()");
}
public UnaryCallable
getRecurringAudienceListCallable() {
throw new UnsupportedOperationException("Not implemented: getRecurringAudienceListCallable()");
}
public UnaryCallable
listRecurringAudienceListsPagedCallable() {
throw new UnsupportedOperationException(
"Not implemented: listRecurringAudienceListsPagedCallable()");
}
public UnaryCallable
listRecurringAudienceListsCallable() {
throw new UnsupportedOperationException(
"Not implemented: listRecurringAudienceListsCallable()");
}
@Override
public abstract void close();
}