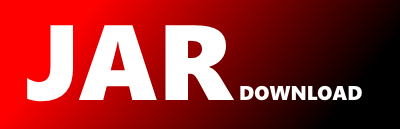
com.google.analytics.data.v1alpha.stub.GrpcAlphaAnalyticsDataStub Maven / Gradle / Ivy
Show all versions of google-analytics-data Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.analytics.data.v1alpha.stub;
import static com.google.analytics.data.v1alpha.AlphaAnalyticsDataClient.ListAudienceListsPagedResponse;
import static com.google.analytics.data.v1alpha.AlphaAnalyticsDataClient.ListRecurringAudienceListsPagedResponse;
import com.google.analytics.data.v1alpha.AudienceList;
import com.google.analytics.data.v1alpha.AudienceListMetadata;
import com.google.analytics.data.v1alpha.CreateAudienceListRequest;
import com.google.analytics.data.v1alpha.CreateRecurringAudienceListRequest;
import com.google.analytics.data.v1alpha.GetAudienceListRequest;
import com.google.analytics.data.v1alpha.GetRecurringAudienceListRequest;
import com.google.analytics.data.v1alpha.ListAudienceListsRequest;
import com.google.analytics.data.v1alpha.ListAudienceListsResponse;
import com.google.analytics.data.v1alpha.ListRecurringAudienceListsRequest;
import com.google.analytics.data.v1alpha.ListRecurringAudienceListsResponse;
import com.google.analytics.data.v1alpha.QueryAudienceListRequest;
import com.google.analytics.data.v1alpha.QueryAudienceListResponse;
import com.google.analytics.data.v1alpha.RecurringAudienceList;
import com.google.analytics.data.v1alpha.RunFunnelReportRequest;
import com.google.analytics.data.v1alpha.RunFunnelReportResponse;
import com.google.analytics.data.v1alpha.SheetExportAudienceListRequest;
import com.google.analytics.data.v1alpha.SheetExportAudienceListResponse;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.core.BackgroundResourceAggregation;
import com.google.api.gax.grpc.GrpcCallSettings;
import com.google.api.gax.grpc.GrpcStubCallableFactory;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.RequestParamsBuilder;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.GrpcOperationsStub;
import io.grpc.MethodDescriptor;
import io.grpc.protobuf.ProtoUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* gRPC stub implementation for the AlphaAnalyticsData service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@BetaApi
@Generated("by gapic-generator-java")
public class GrpcAlphaAnalyticsDataStub extends AlphaAnalyticsDataStub {
private static final MethodDescriptor
runFunnelReportMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.analytics.data.v1alpha.AlphaAnalyticsData/RunFunnelReport")
.setRequestMarshaller(
ProtoUtils.marshaller(RunFunnelReportRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(RunFunnelReportResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
createAudienceListMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1alpha.AlphaAnalyticsData/CreateAudienceList")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateAudienceListRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
queryAudienceListMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1alpha.AlphaAnalyticsData/QueryAudienceList")
.setRequestMarshaller(
ProtoUtils.marshaller(QueryAudienceListRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(QueryAudienceListResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor<
SheetExportAudienceListRequest, SheetExportAudienceListResponse>
sheetExportAudienceListMethodDescriptor =
MethodDescriptor
.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1alpha.AlphaAnalyticsData/SheetExportAudienceList")
.setRequestMarshaller(
ProtoUtils.marshaller(SheetExportAudienceListRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(SheetExportAudienceListResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getAudienceListMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.analytics.data.v1alpha.AlphaAnalyticsData/GetAudienceList")
.setRequestMarshaller(
ProtoUtils.marshaller(GetAudienceListRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(AudienceList.getDefaultInstance()))
.build();
private static final MethodDescriptor
listAudienceListsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1alpha.AlphaAnalyticsData/ListAudienceLists")
.setRequestMarshaller(
ProtoUtils.marshaller(ListAudienceListsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListAudienceListsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
createRecurringAudienceListMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1alpha.AlphaAnalyticsData/CreateRecurringAudienceList")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateRecurringAudienceListRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(RecurringAudienceList.getDefaultInstance()))
.build();
private static final MethodDescriptor
getRecurringAudienceListMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1alpha.AlphaAnalyticsData/GetRecurringAudienceList")
.setRequestMarshaller(
ProtoUtils.marshaller(GetRecurringAudienceListRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(RecurringAudienceList.getDefaultInstance()))
.build();
private static final MethodDescriptor<
ListRecurringAudienceListsRequest, ListRecurringAudienceListsResponse>
listRecurringAudienceListsMethodDescriptor =
MethodDescriptor
.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1alpha.AlphaAnalyticsData/ListRecurringAudienceLists")
.setRequestMarshaller(
ProtoUtils.marshaller(ListRecurringAudienceListsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListRecurringAudienceListsResponse.getDefaultInstance()))
.build();
private final UnaryCallable
runFunnelReportCallable;
private final UnaryCallable createAudienceListCallable;
private final OperationCallable
createAudienceListOperationCallable;
private final UnaryCallable
queryAudienceListCallable;
private final UnaryCallable
sheetExportAudienceListCallable;
private final UnaryCallable getAudienceListCallable;
private final UnaryCallable
listAudienceListsCallable;
private final UnaryCallable
listAudienceListsPagedCallable;
private final UnaryCallable
createRecurringAudienceListCallable;
private final UnaryCallable
getRecurringAudienceListCallable;
private final UnaryCallable
listRecurringAudienceListsCallable;
private final UnaryCallable<
ListRecurringAudienceListsRequest, ListRecurringAudienceListsPagedResponse>
listRecurringAudienceListsPagedCallable;
private final BackgroundResource backgroundResources;
private final GrpcOperationsStub operationsStub;
private final GrpcStubCallableFactory callableFactory;
public static final GrpcAlphaAnalyticsDataStub create(AlphaAnalyticsDataStubSettings settings)
throws IOException {
return new GrpcAlphaAnalyticsDataStub(settings, ClientContext.create(settings));
}
public static final GrpcAlphaAnalyticsDataStub create(ClientContext clientContext)
throws IOException {
return new GrpcAlphaAnalyticsDataStub(
AlphaAnalyticsDataStubSettings.newBuilder().build(), clientContext);
}
public static final GrpcAlphaAnalyticsDataStub create(
ClientContext clientContext, GrpcStubCallableFactory callableFactory) throws IOException {
return new GrpcAlphaAnalyticsDataStub(
AlphaAnalyticsDataStubSettings.newBuilder().build(), clientContext, callableFactory);
}
/**
* Constructs an instance of GrpcAlphaAnalyticsDataStub, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected GrpcAlphaAnalyticsDataStub(
AlphaAnalyticsDataStubSettings settings, ClientContext clientContext) throws IOException {
this(settings, clientContext, new GrpcAlphaAnalyticsDataCallableFactory());
}
/**
* Constructs an instance of GrpcAlphaAnalyticsDataStub, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected GrpcAlphaAnalyticsDataStub(
AlphaAnalyticsDataStubSettings settings,
ClientContext clientContext,
GrpcStubCallableFactory callableFactory)
throws IOException {
this.callableFactory = callableFactory;
this.operationsStub = GrpcOperationsStub.create(clientContext, callableFactory);
GrpcCallSettings
runFunnelReportTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(runFunnelReportMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("property", String.valueOf(request.getProperty()));
return builder.build();
})
.build();
GrpcCallSettings createAudienceListTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createAudienceListMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
queryAudienceListTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(queryAudienceListMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
sheetExportAudienceListTransportSettings =
GrpcCallSettings
.newBuilder()
.setMethodDescriptor(sheetExportAudienceListMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getAudienceListTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getAudienceListMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
listAudienceListsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listAudienceListsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
createRecurringAudienceListTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createRecurringAudienceListMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
getRecurringAudienceListTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getRecurringAudienceListMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
listRecurringAudienceListsTransportSettings =
GrpcCallSettings
.newBuilder()
.setMethodDescriptor(listRecurringAudienceListsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
this.runFunnelReportCallable =
callableFactory.createUnaryCallable(
runFunnelReportTransportSettings, settings.runFunnelReportSettings(), clientContext);
this.createAudienceListCallable =
callableFactory.createUnaryCallable(
createAudienceListTransportSettings,
settings.createAudienceListSettings(),
clientContext);
this.createAudienceListOperationCallable =
callableFactory.createOperationCallable(
createAudienceListTransportSettings,
settings.createAudienceListOperationSettings(),
clientContext,
operationsStub);
this.queryAudienceListCallable =
callableFactory.createUnaryCallable(
queryAudienceListTransportSettings,
settings.queryAudienceListSettings(),
clientContext);
this.sheetExportAudienceListCallable =
callableFactory.createUnaryCallable(
sheetExportAudienceListTransportSettings,
settings.sheetExportAudienceListSettings(),
clientContext);
this.getAudienceListCallable =
callableFactory.createUnaryCallable(
getAudienceListTransportSettings, settings.getAudienceListSettings(), clientContext);
this.listAudienceListsCallable =
callableFactory.createUnaryCallable(
listAudienceListsTransportSettings,
settings.listAudienceListsSettings(),
clientContext);
this.listAudienceListsPagedCallable =
callableFactory.createPagedCallable(
listAudienceListsTransportSettings,
settings.listAudienceListsSettings(),
clientContext);
this.createRecurringAudienceListCallable =
callableFactory.createUnaryCallable(
createRecurringAudienceListTransportSettings,
settings.createRecurringAudienceListSettings(),
clientContext);
this.getRecurringAudienceListCallable =
callableFactory.createUnaryCallable(
getRecurringAudienceListTransportSettings,
settings.getRecurringAudienceListSettings(),
clientContext);
this.listRecurringAudienceListsCallable =
callableFactory.createUnaryCallable(
listRecurringAudienceListsTransportSettings,
settings.listRecurringAudienceListsSettings(),
clientContext);
this.listRecurringAudienceListsPagedCallable =
callableFactory.createPagedCallable(
listRecurringAudienceListsTransportSettings,
settings.listRecurringAudienceListsSettings(),
clientContext);
this.backgroundResources =
new BackgroundResourceAggregation(clientContext.getBackgroundResources());
}
public GrpcOperationsStub getOperationsStub() {
return operationsStub;
}
@Override
public UnaryCallable runFunnelReportCallable() {
return runFunnelReportCallable;
}
@Override
public UnaryCallable createAudienceListCallable() {
return createAudienceListCallable;
}
@Override
public OperationCallable
createAudienceListOperationCallable() {
return createAudienceListOperationCallable;
}
@Override
public UnaryCallable
queryAudienceListCallable() {
return queryAudienceListCallable;
}
@Override
public UnaryCallable
sheetExportAudienceListCallable() {
return sheetExportAudienceListCallable;
}
@Override
public UnaryCallable getAudienceListCallable() {
return getAudienceListCallable;
}
@Override
public UnaryCallable
listAudienceListsCallable() {
return listAudienceListsCallable;
}
@Override
public UnaryCallable
listAudienceListsPagedCallable() {
return listAudienceListsPagedCallable;
}
@Override
public UnaryCallable
createRecurringAudienceListCallable() {
return createRecurringAudienceListCallable;
}
@Override
public UnaryCallable
getRecurringAudienceListCallable() {
return getRecurringAudienceListCallable;
}
@Override
public UnaryCallable
listRecurringAudienceListsCallable() {
return listRecurringAudienceListsCallable;
}
@Override
public UnaryCallable
listRecurringAudienceListsPagedCallable() {
return listRecurringAudienceListsPagedCallable;
}
@Override
public final void close() {
try {
backgroundResources.close();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new IllegalStateException("Failed to close resource", e);
}
}
@Override
public void shutdown() {
backgroundResources.shutdown();
}
@Override
public boolean isShutdown() {
return backgroundResources.isShutdown();
}
@Override
public boolean isTerminated() {
return backgroundResources.isTerminated();
}
@Override
public void shutdownNow() {
backgroundResources.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return backgroundResources.awaitTermination(duration, unit);
}
}