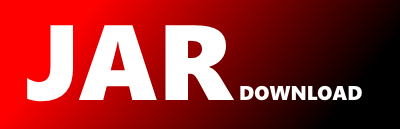
com.google.analytics.data.v1beta.stub.GrpcBetaAnalyticsDataStub Maven / Gradle / Ivy
Show all versions of google-analytics-data Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.analytics.data.v1beta.stub;
import static com.google.analytics.data.v1beta.BetaAnalyticsDataClient.ListAudienceExportsPagedResponse;
import com.google.analytics.data.v1beta.AudienceExport;
import com.google.analytics.data.v1beta.AudienceExportMetadata;
import com.google.analytics.data.v1beta.BatchRunPivotReportsRequest;
import com.google.analytics.data.v1beta.BatchRunPivotReportsResponse;
import com.google.analytics.data.v1beta.BatchRunReportsRequest;
import com.google.analytics.data.v1beta.BatchRunReportsResponse;
import com.google.analytics.data.v1beta.CheckCompatibilityRequest;
import com.google.analytics.data.v1beta.CheckCompatibilityResponse;
import com.google.analytics.data.v1beta.CreateAudienceExportRequest;
import com.google.analytics.data.v1beta.GetAudienceExportRequest;
import com.google.analytics.data.v1beta.GetMetadataRequest;
import com.google.analytics.data.v1beta.ListAudienceExportsRequest;
import com.google.analytics.data.v1beta.ListAudienceExportsResponse;
import com.google.analytics.data.v1beta.Metadata;
import com.google.analytics.data.v1beta.QueryAudienceExportRequest;
import com.google.analytics.data.v1beta.QueryAudienceExportResponse;
import com.google.analytics.data.v1beta.RunPivotReportRequest;
import com.google.analytics.data.v1beta.RunPivotReportResponse;
import com.google.analytics.data.v1beta.RunRealtimeReportRequest;
import com.google.analytics.data.v1beta.RunRealtimeReportResponse;
import com.google.analytics.data.v1beta.RunReportRequest;
import com.google.analytics.data.v1beta.RunReportResponse;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.core.BackgroundResourceAggregation;
import com.google.api.gax.grpc.GrpcCallSettings;
import com.google.api.gax.grpc.GrpcStubCallableFactory;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.RequestParamsBuilder;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.GrpcOperationsStub;
import io.grpc.MethodDescriptor;
import io.grpc.protobuf.ProtoUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* gRPC stub implementation for the BetaAnalyticsData service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@BetaApi
@Generated("by gapic-generator-java")
public class GrpcBetaAnalyticsDataStub extends BetaAnalyticsDataStub {
private static final MethodDescriptor
runReportMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.analytics.data.v1beta.BetaAnalyticsData/RunReport")
.setRequestMarshaller(ProtoUtils.marshaller(RunReportRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(RunReportResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
runPivotReportMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.analytics.data.v1beta.BetaAnalyticsData/RunPivotReport")
.setRequestMarshaller(
ProtoUtils.marshaller(RunPivotReportRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(RunPivotReportResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
batchRunReportsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.analytics.data.v1beta.BetaAnalyticsData/BatchRunReports")
.setRequestMarshaller(
ProtoUtils.marshaller(BatchRunReportsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(BatchRunReportsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
batchRunPivotReportsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1beta.BetaAnalyticsData/BatchRunPivotReports")
.setRequestMarshaller(
ProtoUtils.marshaller(BatchRunPivotReportsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(BatchRunPivotReportsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getMetadataMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.analytics.data.v1beta.BetaAnalyticsData/GetMetadata")
.setRequestMarshaller(ProtoUtils.marshaller(GetMetadataRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Metadata.getDefaultInstance()))
.build();
private static final MethodDescriptor
runRealtimeReportMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.analytics.data.v1beta.BetaAnalyticsData/RunRealtimeReport")
.setRequestMarshaller(
ProtoUtils.marshaller(RunRealtimeReportRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(RunRealtimeReportResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
checkCompatibilityMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1beta.BetaAnalyticsData/CheckCompatibility")
.setRequestMarshaller(
ProtoUtils.marshaller(CheckCompatibilityRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(CheckCompatibilityResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
createAudienceExportMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1beta.BetaAnalyticsData/CreateAudienceExport")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateAudienceExportRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
queryAudienceExportMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1beta.BetaAnalyticsData/QueryAudienceExport")
.setRequestMarshaller(
ProtoUtils.marshaller(QueryAudienceExportRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(QueryAudienceExportResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getAudienceExportMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.analytics.data.v1beta.BetaAnalyticsData/GetAudienceExport")
.setRequestMarshaller(
ProtoUtils.marshaller(GetAudienceExportRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(AudienceExport.getDefaultInstance()))
.build();
private static final MethodDescriptor
listAudienceExportsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.analytics.data.v1beta.BetaAnalyticsData/ListAudienceExports")
.setRequestMarshaller(
ProtoUtils.marshaller(ListAudienceExportsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListAudienceExportsResponse.getDefaultInstance()))
.build();
private final UnaryCallable runReportCallable;
private final UnaryCallable runPivotReportCallable;
private final UnaryCallable
batchRunReportsCallable;
private final UnaryCallable
batchRunPivotReportsCallable;
private final UnaryCallable getMetadataCallable;
private final UnaryCallable
runRealtimeReportCallable;
private final UnaryCallable
checkCompatibilityCallable;
private final UnaryCallable createAudienceExportCallable;
private final OperationCallable<
CreateAudienceExportRequest, AudienceExport, AudienceExportMetadata>
createAudienceExportOperationCallable;
private final UnaryCallable
queryAudienceExportCallable;
private final UnaryCallable getAudienceExportCallable;
private final UnaryCallable
listAudienceExportsCallable;
private final UnaryCallable
listAudienceExportsPagedCallable;
private final BackgroundResource backgroundResources;
private final GrpcOperationsStub operationsStub;
private final GrpcStubCallableFactory callableFactory;
public static final GrpcBetaAnalyticsDataStub create(BetaAnalyticsDataStubSettings settings)
throws IOException {
return new GrpcBetaAnalyticsDataStub(settings, ClientContext.create(settings));
}
public static final GrpcBetaAnalyticsDataStub create(ClientContext clientContext)
throws IOException {
return new GrpcBetaAnalyticsDataStub(
BetaAnalyticsDataStubSettings.newBuilder().build(), clientContext);
}
public static final GrpcBetaAnalyticsDataStub create(
ClientContext clientContext, GrpcStubCallableFactory callableFactory) throws IOException {
return new GrpcBetaAnalyticsDataStub(
BetaAnalyticsDataStubSettings.newBuilder().build(), clientContext, callableFactory);
}
/**
* Constructs an instance of GrpcBetaAnalyticsDataStub, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected GrpcBetaAnalyticsDataStub(
BetaAnalyticsDataStubSettings settings, ClientContext clientContext) throws IOException {
this(settings, clientContext, new GrpcBetaAnalyticsDataCallableFactory());
}
/**
* Constructs an instance of GrpcBetaAnalyticsDataStub, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected GrpcBetaAnalyticsDataStub(
BetaAnalyticsDataStubSettings settings,
ClientContext clientContext,
GrpcStubCallableFactory callableFactory)
throws IOException {
this.callableFactory = callableFactory;
this.operationsStub = GrpcOperationsStub.create(clientContext, callableFactory);
GrpcCallSettings runReportTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(runReportMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("property", String.valueOf(request.getProperty()));
return builder.build();
})
.build();
GrpcCallSettings
runPivotReportTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(runPivotReportMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("property", String.valueOf(request.getProperty()));
return builder.build();
})
.build();
GrpcCallSettings
batchRunReportsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(batchRunReportsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("property", String.valueOf(request.getProperty()));
return builder.build();
})
.build();
GrpcCallSettings
batchRunPivotReportsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(batchRunPivotReportsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("property", String.valueOf(request.getProperty()));
return builder.build();
})
.build();
GrpcCallSettings getMetadataTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getMetadataMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
runRealtimeReportTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(runRealtimeReportMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("property", String.valueOf(request.getProperty()));
return builder.build();
})
.build();
GrpcCallSettings
checkCompatibilityTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(checkCompatibilityMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("property", String.valueOf(request.getProperty()));
return builder.build();
})
.build();
GrpcCallSettings createAudienceExportTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createAudienceExportMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
queryAudienceExportTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(queryAudienceExportMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getAudienceExportTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getAudienceExportMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
listAudienceExportsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listAudienceExportsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
this.runReportCallable =
callableFactory.createUnaryCallable(
runReportTransportSettings, settings.runReportSettings(), clientContext);
this.runPivotReportCallable =
callableFactory.createUnaryCallable(
runPivotReportTransportSettings, settings.runPivotReportSettings(), clientContext);
this.batchRunReportsCallable =
callableFactory.createUnaryCallable(
batchRunReportsTransportSettings, settings.batchRunReportsSettings(), clientContext);
this.batchRunPivotReportsCallable =
callableFactory.createUnaryCallable(
batchRunPivotReportsTransportSettings,
settings.batchRunPivotReportsSettings(),
clientContext);
this.getMetadataCallable =
callableFactory.createUnaryCallable(
getMetadataTransportSettings, settings.getMetadataSettings(), clientContext);
this.runRealtimeReportCallable =
callableFactory.createUnaryCallable(
runRealtimeReportTransportSettings,
settings.runRealtimeReportSettings(),
clientContext);
this.checkCompatibilityCallable =
callableFactory.createUnaryCallable(
checkCompatibilityTransportSettings,
settings.checkCompatibilitySettings(),
clientContext);
this.createAudienceExportCallable =
callableFactory.createUnaryCallable(
createAudienceExportTransportSettings,
settings.createAudienceExportSettings(),
clientContext);
this.createAudienceExportOperationCallable =
callableFactory.createOperationCallable(
createAudienceExportTransportSettings,
settings.createAudienceExportOperationSettings(),
clientContext,
operationsStub);
this.queryAudienceExportCallable =
callableFactory.createUnaryCallable(
queryAudienceExportTransportSettings,
settings.queryAudienceExportSettings(),
clientContext);
this.getAudienceExportCallable =
callableFactory.createUnaryCallable(
getAudienceExportTransportSettings,
settings.getAudienceExportSettings(),
clientContext);
this.listAudienceExportsCallable =
callableFactory.createUnaryCallable(
listAudienceExportsTransportSettings,
settings.listAudienceExportsSettings(),
clientContext);
this.listAudienceExportsPagedCallable =
callableFactory.createPagedCallable(
listAudienceExportsTransportSettings,
settings.listAudienceExportsSettings(),
clientContext);
this.backgroundResources =
new BackgroundResourceAggregation(clientContext.getBackgroundResources());
}
public GrpcOperationsStub getOperationsStub() {
return operationsStub;
}
@Override
public UnaryCallable runReportCallable() {
return runReportCallable;
}
@Override
public UnaryCallable runPivotReportCallable() {
return runPivotReportCallable;
}
@Override
public UnaryCallable batchRunReportsCallable() {
return batchRunReportsCallable;
}
@Override
public UnaryCallable
batchRunPivotReportsCallable() {
return batchRunPivotReportsCallable;
}
@Override
public UnaryCallable getMetadataCallable() {
return getMetadataCallable;
}
@Override
public UnaryCallable
runRealtimeReportCallable() {
return runRealtimeReportCallable;
}
@Override
public UnaryCallable
checkCompatibilityCallable() {
return checkCompatibilityCallable;
}
@Override
public UnaryCallable createAudienceExportCallable() {
return createAudienceExportCallable;
}
@Override
public OperationCallable
createAudienceExportOperationCallable() {
return createAudienceExportOperationCallable;
}
@Override
public UnaryCallable
queryAudienceExportCallable() {
return queryAudienceExportCallable;
}
@Override
public UnaryCallable getAudienceExportCallable() {
return getAudienceExportCallable;
}
@Override
public UnaryCallable
listAudienceExportsCallable() {
return listAudienceExportsCallable;
}
@Override
public UnaryCallable
listAudienceExportsPagedCallable() {
return listAudienceExportsPagedCallable;
}
@Override
public final void close() {
try {
backgroundResources.close();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new IllegalStateException("Failed to close resource", e);
}
}
@Override
public void shutdown() {
backgroundResources.shutdown();
}
@Override
public boolean isShutdown() {
return backgroundResources.isShutdown();
}
@Override
public boolean isTerminated() {
return backgroundResources.isTerminated();
}
@Override
public void shutdownNow() {
backgroundResources.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return backgroundResources.awaitTermination(duration, unit);
}
}