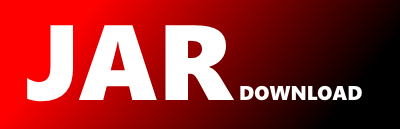
com.google.ads.admanager.v1.package-info Maven / Gradle / Ivy
Show all versions of ad-manager Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* A client to Google Ad Manager API
*
* The interfaces provided are listed below, along with usage samples.
*
*
======================= AdUnitServiceClient =======================
*
*
Service Description: Provides methods for handling AdUnit objects.
*
*
Sample for AdUnitServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdUnitServiceClient adUnitServiceClient = AdUnitServiceClient.create()) {
* AdUnitName name = AdUnitName.of("[NETWORK_CODE]", "[AD_UNIT]");
* AdUnit response = adUnitServiceClient.getAdUnit(name);
* }
* }
*
* ======================= CompanyServiceClient =======================
*
*
Service Description: Provides methods for handling `Company` objects.
*
*
Sample for CompanyServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CompanyServiceClient companyServiceClient = CompanyServiceClient.create()) {
* CompanyName name = CompanyName.of("[NETWORK_CODE]", "[COMPANY]");
* Company response = companyServiceClient.getCompany(name);
* }
* }
*
* ======================= CustomFieldServiceClient =======================
*
*
Service Description: Provides methods for handling `CustomField` objects.
*
*
Sample for CustomFieldServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomFieldServiceClient customFieldServiceClient = CustomFieldServiceClient.create()) {
* CustomFieldName name = CustomFieldName.of("[NETWORK_CODE]", "[CUSTOM_FIELD]");
* CustomField response = customFieldServiceClient.getCustomField(name);
* }
* }
*
* ======================= CustomTargetingKeyServiceClient =======================
*
*
Service Description: Provides methods for handling `CustomTargetingKey` objects.
*
*
Sample for CustomTargetingKeyServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomTargetingKeyServiceClient customTargetingKeyServiceClient =
* CustomTargetingKeyServiceClient.create()) {
* CustomTargetingKeyName name =
* CustomTargetingKeyName.of("[NETWORK_CODE]", "[CUSTOM_TARGETING_KEY]");
* CustomTargetingKey response = customTargetingKeyServiceClient.getCustomTargetingKey(name);
* }
* }
*
* ======================= CustomTargetingValueServiceClient =======================
*
*
Service Description: Provides methods for handling `CustomTargetingValue` objects.
*
*
Sample for CustomTargetingValueServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomTargetingValueServiceClient customTargetingValueServiceClient =
* CustomTargetingValueServiceClient.create()) {
* CustomTargetingValueName name =
* CustomTargetingValueName.of(
* "[NETWORK_CODE]", "[CUSTOM_TARGETING_KEY]", "[CUSTOM_TARGETING_VALUE]");
* CustomTargetingValue response =
* customTargetingValueServiceClient.getCustomTargetingValue(name);
* }
* }
*
* ======================= EntitySignalsMappingServiceClient =======================
*
*
Service Description: Provides methods for handling `EntitySignalsMapping` objects.
*
*
Sample for EntitySignalsMappingServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (EntitySignalsMappingServiceClient entitySignalsMappingServiceClient =
* EntitySignalsMappingServiceClient.create()) {
* EntitySignalsMappingName name =
* EntitySignalsMappingName.of("[NETWORK_CODE]", "[ENTITY_SIGNALS_MAPPING]");
* EntitySignalsMapping response =
* entitySignalsMappingServiceClient.getEntitySignalsMapping(name);
* }
* }
*
* ======================= NetworkServiceClient =======================
*
*
Service Description: Provides methods for handling Network objects.
*
*
Sample for NetworkServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (NetworkServiceClient networkServiceClient = NetworkServiceClient.create()) {
* NetworkName name = NetworkName.of("[NETWORK_CODE]");
* Network response = networkServiceClient.getNetwork(name);
* }
* }
*
* ======================= OrderServiceClient =======================
*
*
Service Description: Provides methods for handling `Order` objects.
*
*
Sample for OrderServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OrderServiceClient orderServiceClient = OrderServiceClient.create()) {
* OrderName name = OrderName.of("[NETWORK_CODE]", "[ORDER]");
* Order response = orderServiceClient.getOrder(name);
* }
* }
*
* ======================= PlacementServiceClient =======================
*
*
Service Description: Provides methods for handling `Placement` objects.
*
*
Sample for PlacementServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PlacementServiceClient placementServiceClient = PlacementServiceClient.create()) {
* PlacementName name = PlacementName.of("[NETWORK_CODE]", "[PLACEMENT]");
* Placement response = placementServiceClient.getPlacement(name);
* }
* }
*
* ======================= ReportServiceClient =======================
*
*
Service Description: Provides methods for interacting with reports.
*
*
Sample for ReportServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ReportServiceClient reportServiceClient = ReportServiceClient.create()) {
* ReportName name = ReportName.of("[NETWORK_CODE]", "[REPORT]");
* Report response = reportServiceClient.getReport(name);
* }
* }
*
* ======================= RoleServiceClient =======================
*
*
Service Description: Provides methods for handling `Role` objects.
*
*
Sample for RoleServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RoleServiceClient roleServiceClient = RoleServiceClient.create()) {
* RoleName name = RoleName.of("[NETWORK_CODE]", "[ROLE]");
* Role response = roleServiceClient.getRole(name);
* }
* }
*
* ======================= TaxonomyCategoryServiceClient =======================
*
*
Service Description: Provides methods for handling `TaxonomyCategory` objects.
*
*
Sample for TaxonomyCategoryServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TaxonomyCategoryServiceClient taxonomyCategoryServiceClient =
* TaxonomyCategoryServiceClient.create()) {
* TaxonomyCategoryName name = TaxonomyCategoryName.of("[NETWORK_CODE]", "[TAXONOMY_CATEGORY]");
* TaxonomyCategory response = taxonomyCategoryServiceClient.getTaxonomyCategory(name);
* }
* }
*
* ======================= UserServiceClient =======================
*
*
Service Description: Provides methods for handling User objects.
*
*
Sample for UserServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (UserServiceClient userServiceClient = UserServiceClient.create()) {
* UserName name = UserName.of("[NETWORK_CODE]", "[USER]");
* User response = userServiceClient.getUser(name);
* }
* }
*/
@Generated("by gapic-generator-java")
package com.google.ads.admanager.v1;
import javax.annotation.Generated;