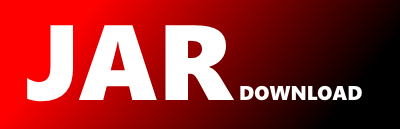
com.google.api.ads.adwords.lib.utils.QueryBuilderInterface Maven / Gradle / Ivy
// Copyright 2018 Google Inc. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package com.google.api.ads.adwords.lib.utils;
/**
* A builder for query objects.
*
* This builder is not thread safe.
*/
public interface QueryBuilderInterface {
/**
* Set the condition of this query as the WHERE clause.
*
* @param field the field of the given entity to be filtered on
* @return the WHERE builder to create a predicate
*/
WhereBuilderInterface where(String field);
/**
* Selects the provided fields for building as a query.
*
* @param fields the provided fields to be selected
* @return this query builder itself for method chaining
*/
QueryBuilderInterface fields(String... fields);
/**
* Selects the provided fields for building a report query.
*
* @param fields the fields to be selected
* @return this builder itself for method chaining
*/
QueryBuilderInterface fields(Iterable fields);
/**
* Builds the query from the attributes of this builder.
*
* @return the built query object
*/
QueryT build();
/**
* A builder for the WHERE clause of queries.
*
* This builder is not thread safe.
*/
interface WhereBuilderInterface {
/**
* Adds the predicate EQUAL_TO to the query for the given value.
*
* @param propertyValue the property value as a String independently of the field type. The
* caller should take care of the formatting if it is necessary
* @return the containing query builder
*/
QueryBuilderInterface equalTo(String propertyValue);
/**
* Adds the predicate EQUAL_TO to the query for the given value.
*
* @param propertyValue the property value as a integer independently of the field type
* @return the containing query builder
*/
QueryBuilderInterface equalTo(int propertyValue);
/**
* Adds the predicate EQUAL_TO to the query for the given value.
*
* @param propertyValue the property value as a long independently of the field type
* @return the containing query builder
*/
QueryBuilderInterface equalTo(long propertyValue);
/**
* Adds the predicate EQUAL_TO to the query for the given value.
*
* @param propertyValue the property value as a boolean independently of the field type
* @return the containing query builder
*/
QueryBuilderInterface equalTo(boolean propertyValue);
/**
* Adds the predicate NOT_EQUAL_TO to the query for the given value.
*
* @param propertyValue the property value as a String independently of the field type. The
* caller should take care of the formatting if it is necessary
* @return the containing query builder
*/
QueryBuilderInterface notEqualTo(String propertyValue);
/**
* Adds the predicate NOT_EQUAL_TO to the query for the given value.
*
* @param propertyValue the property value as a integer independently of the field type
* @return the containing query builder
*/
QueryBuilderInterface notEqualTo(int propertyValue);
/**
* Adds the predicate NOT_EQUAL_TO to the query for the given value.
*
* @param propertyValue the property value as a long independently of the field type
* @return the containing query builder
*/
QueryBuilderInterface notEqualTo(long propertyValue);
/**
* Adds the predicate NOT_EQUAL_TO to the query for the given value.
*
* @param propertyValue the property value as a boolean independently of the field type
* @return the containing query builder
*/
QueryBuilderInterface notEqualTo(boolean propertyValue);
/**
* Adds the predicate CONTAINS to the query for the given value.
*
* @param propertyValue the property value as a String independently of the field type. The
* caller should take care of the formatting if it is necessary
* @return the containing query builder
*/
QueryBuilderInterface contains(String propertyValue);
/**
* Adds the predicate CONTAINS_IGNORE_CASE to the query for the given value.
*
* @param propertyValue the property value as a String independently of the field type. The
* caller should take care of the formatting if it is necessary
* @return the containing query builder
*/
QueryBuilderInterface containsIgnoreCase(String propertyValue);
/**
* Adds the predicate DOES_NOT_CONTAIN to the query for the given value.
*
* @param propertyValue the property value as a String independently of the field type. The
* caller should take care of the formatting if it is necessary
* @return the containing query builder
*/
QueryBuilderInterface doesNotContain(String propertyValue);
/**
* Adds the predicate DOES_NOT_CONTAIN_IGNORE_CASE to the query for the given value.
*
* @param propertyValue the property value as a String independently of the field type. The
* caller should take care of the formatting if it is necessary
* @return the containing query builder
*/
QueryBuilderInterface doesNotContainIgnoreCase(String propertyValue);
/**
* Adds the predicate GREATER_THAN to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface greaterThan(long propertyValue);
/**
* Adds the predicate GREATER_THAN to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface greaterThan(int propertyValue);
/**
* Adds the predicate GREATER_THAN to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface greaterThan(double propertyValue);
/**
* Adds the predicate GREATER_THAN_OR_EQUAL_TO to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface greaterThanOrEqualTo(long propertyValue);
/**
* Adds the predicate GREATER_THAN_OR_EQUAL_TO to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface greaterThanOrEqualTo(int propertyValue);
/**
* Adds the predicate GREATER_THAN_OR_EQUAL_TO to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface greaterThanOrEqualTo(double propertyValue);
/**
* Adds the predicate LESS_THAN to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface lessThan(long propertyValue);
/**
* Adds the predicate LESS_THAN to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface lessThan(int propertyValue);
/**
* Adds the predicate LESS_THAN to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface lessThan(double propertyValue);
/**
* Adds the predicate LESS_THAN_OR_EQUAL_TO to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface lessThanOrEqualTo(long propertyValue);
/**
* Adds the predicate LESS_THAN_OR_EQUAL_TO to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface lessThanOrEqualTo(int propertyValue);
/**
* Adds the predicate LESS_THAN_OR_EQUAL_TO to the query for the given value.
*
* @return the containing query builder
*/
QueryBuilderInterface lessThanOrEqualTo(double propertyValue);
/**
* Adds the predicate STARTS_WITH to the query for the given value.
*
* @param propertyValue the property value as a String independently of the field type. The
* caller should take care of the formatting if it is necessary
* @return the containing query builder
*/
QueryBuilderInterface startsWith(String propertyValue);
/**
* Adds the predicate STARTS_WITH_IGNORE_CASE to the query for the given value.
*
* @param propertyValue the property value as a String independently of the field type. The
* caller should take care of the formatting if it is necessary
* @return the containing query builder
*/
QueryBuilderInterface startsWithIgnoreCase(String propertyValue);
/**
* Adds the predicate IN to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface in(String... values);
/**
* Adds the predicate IN to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface in(int... values);
/**
* Adds the predicate IN to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface in(long... values);
/**
* Adds the predicate IN to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface in(double... values);
/**
* Adds the predicate IN to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface in(boolean... values);
/**
* Adds the predicate NOT_IN to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface notIn(String... values);
/**
* Adds the predicate NOT_IN to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface notIn(int... values);
/**
* Adds the predicate NOT_IN to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface notIn(long... values);
/**
* Adds the predicate NOT_IN to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface notIn(double... values);
/**
* Adds the predicate NOT_IN to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface notIn(boolean... values);
/**
* Adds the predicate CONTAINS_ANY to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsAny(String... values);
/**
* Adds the predicate CONTAINS_ANY to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsAny(int... values);
/**
* Adds the predicate CONTAINS_ANY to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsAny(long... values);
/**
* Adds the predicate CONTAINS_ANY to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsAny(double... values);
/**
* Adds the predicate CONTAINS_ALL to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsAll(String... values);
/**
* Adds the predicate CONTAINS_ALL to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsAll(int... values);
/**
* Adds the predicate CONTAINS_ALL to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsAll(long... values);
/**
* Adds the predicate CONTAINS_ALL to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsAll(double... values);
/**
* Adds the predicate CONTAINS_NONE to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsNone(String... values);
/**
* Adds the predicate CONTAINS_NONE to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsNone(int... values);
/**
* Adds the predicate CONTAINS_NONE to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsNone(long... values);
/**
* Adds the predicate CONTAINS_NONE to the query for the given set of values.
*
* @return the containing query builder
*/
QueryBuilderInterface containsNone(double... values);
}
}