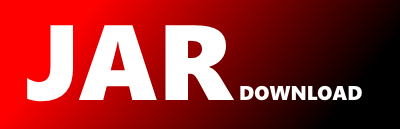
com.google.api.ads.common.lib.soap.SoapClientHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ads-lib Show documentation
Show all versions of ads-lib Show documentation
Client library for Java for accessing ads APIs including DFP.
If you want to use this library, you must also include another Maven
artifact to specify which framework you would like to use it with. For
example, to use DFP with Axis, you should include the "dfp-axis"
artifact.
// Copyright 2011, Google Inc. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package com.google.api.ads.common.lib.soap;
import java.lang.reflect.Method;
import java.util.Arrays;
/**
* Base class for SOAP client handlers. Calling {@link #invoke(SoapCall)} will
* perform the method retrieved from {@link SoapCall#getSoapClientMethod()} on
* the {@link SoapCall#getSoapClient()} with the arguments retrieved from
* {@link SoapCall#getSoapArgs()}.
*
* @param the SOAP client type
*
* @see SoapClientHandlerInterface
*/
public abstract class SoapClientHandler implements SoapClientHandlerInterface {
/**
* Default constructor.
*/
protected SoapClientHandler() {}
/**
* Processes the arguments such that they will be ready to be passed into the
* supplied SOAP client method. This method can be overridden in the case that
* a particular framework must additionally process the arguments.
*
* In the default implementation of processing arguments, if the number of
* arguments is too small, the argument list will be padded with {@code null}.
* If {@code args} is {@code null}, {@code null} will be returned.
*
*
* @param soapClientMethod the SOAP client method that will be called with the
* {@code args}
* @param args the arguments that will be processed
* @return the arguments ready to be passed into the {@code soapClientMethod}.
*/
protected Object[] processSoapArguments(Method soapClientMethod, Object[] args) {
if (args == null) {
return null;
}
int argsCount = Math.max(soapClientMethod.getParameterTypes().length, args.length);
return Arrays.copyOf(args, argsCount);
}
/**
* Gets the method from the SOAP client that matches the supplied method.
*
* In the default implementation, only the method name and return type
* will be matched.
*
*
* @param soapClient the SOAP client within which to search for the method
* @param method the method to match
* @return the SOAP client's matching method
* @throws NoSuchMethodException thrown if the SOAP client does not contain
* the requested method.
*
* TODO(api.arogal): Needs to check parameter types as well as name/return.
*/
@Override
public Method getSoapClientMethod(T soapClient, Method method) throws NoSuchMethodException {
for (Method soapClientMethod : soapClient.getClass().getMethods()) {
if (method.getName().equals(soapClientMethod.getName())
&& method.getReturnType().equals(soapClientMethod.getReturnType())) {
return soapClientMethod;
}
}
throw new NoSuchMethodException("No method named " + method.getName() + " with return type "
+ method.getReturnType() + " found.");
}
/**
* Takes a {@link SoapCall} object and invokes the method by reflection.
*
* @param soapCall contains the SOAP method, SOAP client, and args to be
* called
* @return the response from the SOAP web service
* @throws Exception thrown if calling the operation on the remote service
* fails
*/
protected Object invoke(SoapCall soapCall) throws Exception {
Method soapClientMethod = soapCall.getSoapClientMethod();
Object[] soapArgs = soapCall.getSoapArgs();
soapArgs = processSoapArguments(soapClientMethod, soapArgs);
return soapClientMethod.invoke(soapCall.getSoapClient(), soapArgs);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy