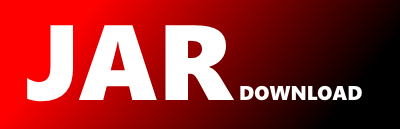
com.google.ads.googleads.v10.common.ListingDimensionInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v10 Show documentation
Show all versions of google-ads-stubs-v10 Show documentation
Stubs for GAAPI version google-ads-stubs-v10
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v10/common/criteria.proto
package com.google.ads.googleads.v10.common;
/**
*
* Listing dimensions for listing group criterion.
*
*
* Protobuf type {@code google.ads.googleads.v10.common.ListingDimensionInfo}
*/
public final class ListingDimensionInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v10.common.ListingDimensionInfo)
ListingDimensionInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ListingDimensionInfo.newBuilder() to construct.
private ListingDimensionInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListingDimensionInfo() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ListingDimensionInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.common.CriteriaProto.internal_static_google_ads_googleads_v10_common_ListingDimensionInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.common.CriteriaProto.internal_static_google_ads_googleads_v10_common_ListingDimensionInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.common.ListingDimensionInfo.class, com.google.ads.googleads.v10.common.ListingDimensionInfo.Builder.class);
}
private int dimensionCase_ = 0;
private java.lang.Object dimension_;
public enum DimensionCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
HOTEL_ID(2),
HOTEL_CLASS(3),
HOTEL_COUNTRY_REGION(4),
HOTEL_STATE(5),
HOTEL_CITY(6),
PRODUCT_BIDDING_CATEGORY(13),
PRODUCT_BRAND(15),
PRODUCT_CHANNEL(8),
PRODUCT_CHANNEL_EXCLUSIVITY(9),
PRODUCT_CONDITION(10),
PRODUCT_CUSTOM_ATTRIBUTE(16),
PRODUCT_ITEM_ID(11),
PRODUCT_TYPE(12),
PRODUCT_GROUPING(17),
PRODUCT_LABELS(18),
PRODUCT_LEGACY_CONDITION(19),
PRODUCT_TYPE_FULL(20),
UNKNOWN_LISTING_DIMENSION(14),
DIMENSION_NOT_SET(0);
private final int value;
private DimensionCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DimensionCase valueOf(int value) {
return forNumber(value);
}
public static DimensionCase forNumber(int value) {
switch (value) {
case 2: return HOTEL_ID;
case 3: return HOTEL_CLASS;
case 4: return HOTEL_COUNTRY_REGION;
case 5: return HOTEL_STATE;
case 6: return HOTEL_CITY;
case 13: return PRODUCT_BIDDING_CATEGORY;
case 15: return PRODUCT_BRAND;
case 8: return PRODUCT_CHANNEL;
case 9: return PRODUCT_CHANNEL_EXCLUSIVITY;
case 10: return PRODUCT_CONDITION;
case 16: return PRODUCT_CUSTOM_ATTRIBUTE;
case 11: return PRODUCT_ITEM_ID;
case 12: return PRODUCT_TYPE;
case 17: return PRODUCT_GROUPING;
case 18: return PRODUCT_LABELS;
case 19: return PRODUCT_LEGACY_CONDITION;
case 20: return PRODUCT_TYPE_FULL;
case 14: return UNKNOWN_LISTING_DIMENSION;
case 0: return DIMENSION_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public DimensionCase
getDimensionCase() {
return DimensionCase.forNumber(
dimensionCase_);
}
public static final int HOTEL_ID_FIELD_NUMBER = 2;
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
* @return Whether the hotelId field is set.
*/
@java.lang.Override
public boolean hasHotelId() {
return dimensionCase_ == 2;
}
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
* @return The hotelId.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelIdInfo getHotelId() {
if (dimensionCase_ == 2) {
return (com.google.ads.googleads.v10.common.HotelIdInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelIdInfo.getDefaultInstance();
}
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelIdInfoOrBuilder getHotelIdOrBuilder() {
if (dimensionCase_ == 2) {
return (com.google.ads.googleads.v10.common.HotelIdInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelIdInfo.getDefaultInstance();
}
public static final int HOTEL_CLASS_FIELD_NUMBER = 3;
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
* @return Whether the hotelClass field is set.
*/
@java.lang.Override
public boolean hasHotelClass() {
return dimensionCase_ == 3;
}
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
* @return The hotelClass.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelClassInfo getHotelClass() {
if (dimensionCase_ == 3) {
return (com.google.ads.googleads.v10.common.HotelClassInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelClassInfo.getDefaultInstance();
}
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelClassInfoOrBuilder getHotelClassOrBuilder() {
if (dimensionCase_ == 3) {
return (com.google.ads.googleads.v10.common.HotelClassInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelClassInfo.getDefaultInstance();
}
public static final int HOTEL_COUNTRY_REGION_FIELD_NUMBER = 4;
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
* @return Whether the hotelCountryRegion field is set.
*/
@java.lang.Override
public boolean hasHotelCountryRegion() {
return dimensionCase_ == 4;
}
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
* @return The hotelCountryRegion.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelCountryRegionInfo getHotelCountryRegion() {
if (dimensionCase_ == 4) {
return (com.google.ads.googleads.v10.common.HotelCountryRegionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelCountryRegionInfo.getDefaultInstance();
}
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelCountryRegionInfoOrBuilder getHotelCountryRegionOrBuilder() {
if (dimensionCase_ == 4) {
return (com.google.ads.googleads.v10.common.HotelCountryRegionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelCountryRegionInfo.getDefaultInstance();
}
public static final int HOTEL_STATE_FIELD_NUMBER = 5;
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
* @return Whether the hotelState field is set.
*/
@java.lang.Override
public boolean hasHotelState() {
return dimensionCase_ == 5;
}
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
* @return The hotelState.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelStateInfo getHotelState() {
if (dimensionCase_ == 5) {
return (com.google.ads.googleads.v10.common.HotelStateInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelStateInfo.getDefaultInstance();
}
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelStateInfoOrBuilder getHotelStateOrBuilder() {
if (dimensionCase_ == 5) {
return (com.google.ads.googleads.v10.common.HotelStateInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelStateInfo.getDefaultInstance();
}
public static final int HOTEL_CITY_FIELD_NUMBER = 6;
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
* @return Whether the hotelCity field is set.
*/
@java.lang.Override
public boolean hasHotelCity() {
return dimensionCase_ == 6;
}
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
* @return The hotelCity.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelCityInfo getHotelCity() {
if (dimensionCase_ == 6) {
return (com.google.ads.googleads.v10.common.HotelCityInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelCityInfo.getDefaultInstance();
}
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelCityInfoOrBuilder getHotelCityOrBuilder() {
if (dimensionCase_ == 6) {
return (com.google.ads.googleads.v10.common.HotelCityInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelCityInfo.getDefaultInstance();
}
public static final int PRODUCT_BIDDING_CATEGORY_FIELD_NUMBER = 13;
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
* @return Whether the productBiddingCategory field is set.
*/
@java.lang.Override
public boolean hasProductBiddingCategory() {
return dimensionCase_ == 13;
}
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
* @return The productBiddingCategory.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo getProductBiddingCategory() {
if (dimensionCase_ == 13) {
return (com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.getDefaultInstance();
}
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductBiddingCategoryInfoOrBuilder getProductBiddingCategoryOrBuilder() {
if (dimensionCase_ == 13) {
return (com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.getDefaultInstance();
}
public static final int PRODUCT_BRAND_FIELD_NUMBER = 15;
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
* @return Whether the productBrand field is set.
*/
@java.lang.Override
public boolean hasProductBrand() {
return dimensionCase_ == 15;
}
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
* @return The productBrand.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductBrandInfo getProductBrand() {
if (dimensionCase_ == 15) {
return (com.google.ads.googleads.v10.common.ProductBrandInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductBrandInfo.getDefaultInstance();
}
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductBrandInfoOrBuilder getProductBrandOrBuilder() {
if (dimensionCase_ == 15) {
return (com.google.ads.googleads.v10.common.ProductBrandInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductBrandInfo.getDefaultInstance();
}
public static final int PRODUCT_CHANNEL_FIELD_NUMBER = 8;
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
* @return Whether the productChannel field is set.
*/
@java.lang.Override
public boolean hasProductChannel() {
return dimensionCase_ == 8;
}
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
* @return The productChannel.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductChannelInfo getProductChannel() {
if (dimensionCase_ == 8) {
return (com.google.ads.googleads.v10.common.ProductChannelInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductChannelInfo.getDefaultInstance();
}
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductChannelInfoOrBuilder getProductChannelOrBuilder() {
if (dimensionCase_ == 8) {
return (com.google.ads.googleads.v10.common.ProductChannelInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductChannelInfo.getDefaultInstance();
}
public static final int PRODUCT_CHANNEL_EXCLUSIVITY_FIELD_NUMBER = 9;
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
* @return Whether the productChannelExclusivity field is set.
*/
@java.lang.Override
public boolean hasProductChannelExclusivity() {
return dimensionCase_ == 9;
}
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
* @return The productChannelExclusivity.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo getProductChannelExclusivity() {
if (dimensionCase_ == 9) {
return (com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.getDefaultInstance();
}
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductChannelExclusivityInfoOrBuilder getProductChannelExclusivityOrBuilder() {
if (dimensionCase_ == 9) {
return (com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.getDefaultInstance();
}
public static final int PRODUCT_CONDITION_FIELD_NUMBER = 10;
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
* @return Whether the productCondition field is set.
*/
@java.lang.Override
public boolean hasProductCondition() {
return dimensionCase_ == 10;
}
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
* @return The productCondition.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductConditionInfo getProductCondition() {
if (dimensionCase_ == 10) {
return (com.google.ads.googleads.v10.common.ProductConditionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductConditionInfo.getDefaultInstance();
}
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductConditionInfoOrBuilder getProductConditionOrBuilder() {
if (dimensionCase_ == 10) {
return (com.google.ads.googleads.v10.common.ProductConditionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductConditionInfo.getDefaultInstance();
}
public static final int PRODUCT_CUSTOM_ATTRIBUTE_FIELD_NUMBER = 16;
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
* @return Whether the productCustomAttribute field is set.
*/
@java.lang.Override
public boolean hasProductCustomAttribute() {
return dimensionCase_ == 16;
}
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
* @return The productCustomAttribute.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductCustomAttributeInfo getProductCustomAttribute() {
if (dimensionCase_ == 16) {
return (com.google.ads.googleads.v10.common.ProductCustomAttributeInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.getDefaultInstance();
}
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductCustomAttributeInfoOrBuilder getProductCustomAttributeOrBuilder() {
if (dimensionCase_ == 16) {
return (com.google.ads.googleads.v10.common.ProductCustomAttributeInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.getDefaultInstance();
}
public static final int PRODUCT_ITEM_ID_FIELD_NUMBER = 11;
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
* @return Whether the productItemId field is set.
*/
@java.lang.Override
public boolean hasProductItemId() {
return dimensionCase_ == 11;
}
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
* @return The productItemId.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductItemIdInfo getProductItemId() {
if (dimensionCase_ == 11) {
return (com.google.ads.googleads.v10.common.ProductItemIdInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductItemIdInfo.getDefaultInstance();
}
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductItemIdInfoOrBuilder getProductItemIdOrBuilder() {
if (dimensionCase_ == 11) {
return (com.google.ads.googleads.v10.common.ProductItemIdInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductItemIdInfo.getDefaultInstance();
}
public static final int PRODUCT_TYPE_FIELD_NUMBER = 12;
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
* @return Whether the productType field is set.
*/
@java.lang.Override
public boolean hasProductType() {
return dimensionCase_ == 12;
}
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
* @return The productType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductTypeInfo getProductType() {
if (dimensionCase_ == 12) {
return (com.google.ads.googleads.v10.common.ProductTypeInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductTypeInfo.getDefaultInstance();
}
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductTypeInfoOrBuilder getProductTypeOrBuilder() {
if (dimensionCase_ == 12) {
return (com.google.ads.googleads.v10.common.ProductTypeInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductTypeInfo.getDefaultInstance();
}
public static final int PRODUCT_GROUPING_FIELD_NUMBER = 17;
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
* @return Whether the productGrouping field is set.
*/
@java.lang.Override
public boolean hasProductGrouping() {
return dimensionCase_ == 17;
}
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
* @return The productGrouping.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductGroupingInfo getProductGrouping() {
if (dimensionCase_ == 17) {
return (com.google.ads.googleads.v10.common.ProductGroupingInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductGroupingInfo.getDefaultInstance();
}
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductGroupingInfoOrBuilder getProductGroupingOrBuilder() {
if (dimensionCase_ == 17) {
return (com.google.ads.googleads.v10.common.ProductGroupingInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductGroupingInfo.getDefaultInstance();
}
public static final int PRODUCT_LABELS_FIELD_NUMBER = 18;
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
* @return Whether the productLabels field is set.
*/
@java.lang.Override
public boolean hasProductLabels() {
return dimensionCase_ == 18;
}
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
* @return The productLabels.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductLabelsInfo getProductLabels() {
if (dimensionCase_ == 18) {
return (com.google.ads.googleads.v10.common.ProductLabelsInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductLabelsInfo.getDefaultInstance();
}
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductLabelsInfoOrBuilder getProductLabelsOrBuilder() {
if (dimensionCase_ == 18) {
return (com.google.ads.googleads.v10.common.ProductLabelsInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductLabelsInfo.getDefaultInstance();
}
public static final int PRODUCT_LEGACY_CONDITION_FIELD_NUMBER = 19;
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
* @return Whether the productLegacyCondition field is set.
*/
@java.lang.Override
public boolean hasProductLegacyCondition() {
return dimensionCase_ == 19;
}
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
* @return The productLegacyCondition.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductLegacyConditionInfo getProductLegacyCondition() {
if (dimensionCase_ == 19) {
return (com.google.ads.googleads.v10.common.ProductLegacyConditionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.getDefaultInstance();
}
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductLegacyConditionInfoOrBuilder getProductLegacyConditionOrBuilder() {
if (dimensionCase_ == 19) {
return (com.google.ads.googleads.v10.common.ProductLegacyConditionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.getDefaultInstance();
}
public static final int PRODUCT_TYPE_FULL_FIELD_NUMBER = 20;
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
* @return Whether the productTypeFull field is set.
*/
@java.lang.Override
public boolean hasProductTypeFull() {
return dimensionCase_ == 20;
}
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
* @return The productTypeFull.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductTypeFullInfo getProductTypeFull() {
if (dimensionCase_ == 20) {
return (com.google.ads.googleads.v10.common.ProductTypeFullInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductTypeFullInfo.getDefaultInstance();
}
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductTypeFullInfoOrBuilder getProductTypeFullOrBuilder() {
if (dimensionCase_ == 20) {
return (com.google.ads.googleads.v10.common.ProductTypeFullInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductTypeFullInfo.getDefaultInstance();
}
public static final int UNKNOWN_LISTING_DIMENSION_FIELD_NUMBER = 14;
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
* @return Whether the unknownListingDimension field is set.
*/
@java.lang.Override
public boolean hasUnknownListingDimension() {
return dimensionCase_ == 14;
}
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
* @return The unknownListingDimension.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.UnknownListingDimensionInfo getUnknownListingDimension() {
if (dimensionCase_ == 14) {
return (com.google.ads.googleads.v10.common.UnknownListingDimensionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.getDefaultInstance();
}
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.UnknownListingDimensionInfoOrBuilder getUnknownListingDimensionOrBuilder() {
if (dimensionCase_ == 14) {
return (com.google.ads.googleads.v10.common.UnknownListingDimensionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (dimensionCase_ == 2) {
output.writeMessage(2, (com.google.ads.googleads.v10.common.HotelIdInfo) dimension_);
}
if (dimensionCase_ == 3) {
output.writeMessage(3, (com.google.ads.googleads.v10.common.HotelClassInfo) dimension_);
}
if (dimensionCase_ == 4) {
output.writeMessage(4, (com.google.ads.googleads.v10.common.HotelCountryRegionInfo) dimension_);
}
if (dimensionCase_ == 5) {
output.writeMessage(5, (com.google.ads.googleads.v10.common.HotelStateInfo) dimension_);
}
if (dimensionCase_ == 6) {
output.writeMessage(6, (com.google.ads.googleads.v10.common.HotelCityInfo) dimension_);
}
if (dimensionCase_ == 8) {
output.writeMessage(8, (com.google.ads.googleads.v10.common.ProductChannelInfo) dimension_);
}
if (dimensionCase_ == 9) {
output.writeMessage(9, (com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo) dimension_);
}
if (dimensionCase_ == 10) {
output.writeMessage(10, (com.google.ads.googleads.v10.common.ProductConditionInfo) dimension_);
}
if (dimensionCase_ == 11) {
output.writeMessage(11, (com.google.ads.googleads.v10.common.ProductItemIdInfo) dimension_);
}
if (dimensionCase_ == 12) {
output.writeMessage(12, (com.google.ads.googleads.v10.common.ProductTypeInfo) dimension_);
}
if (dimensionCase_ == 13) {
output.writeMessage(13, (com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo) dimension_);
}
if (dimensionCase_ == 14) {
output.writeMessage(14, (com.google.ads.googleads.v10.common.UnknownListingDimensionInfo) dimension_);
}
if (dimensionCase_ == 15) {
output.writeMessage(15, (com.google.ads.googleads.v10.common.ProductBrandInfo) dimension_);
}
if (dimensionCase_ == 16) {
output.writeMessage(16, (com.google.ads.googleads.v10.common.ProductCustomAttributeInfo) dimension_);
}
if (dimensionCase_ == 17) {
output.writeMessage(17, (com.google.ads.googleads.v10.common.ProductGroupingInfo) dimension_);
}
if (dimensionCase_ == 18) {
output.writeMessage(18, (com.google.ads.googleads.v10.common.ProductLabelsInfo) dimension_);
}
if (dimensionCase_ == 19) {
output.writeMessage(19, (com.google.ads.googleads.v10.common.ProductLegacyConditionInfo) dimension_);
}
if (dimensionCase_ == 20) {
output.writeMessage(20, (com.google.ads.googleads.v10.common.ProductTypeFullInfo) dimension_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (dimensionCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (com.google.ads.googleads.v10.common.HotelIdInfo) dimension_);
}
if (dimensionCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (com.google.ads.googleads.v10.common.HotelClassInfo) dimension_);
}
if (dimensionCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (com.google.ads.googleads.v10.common.HotelCountryRegionInfo) dimension_);
}
if (dimensionCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (com.google.ads.googleads.v10.common.HotelStateInfo) dimension_);
}
if (dimensionCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, (com.google.ads.googleads.v10.common.HotelCityInfo) dimension_);
}
if (dimensionCase_ == 8) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, (com.google.ads.googleads.v10.common.ProductChannelInfo) dimension_);
}
if (dimensionCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, (com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo) dimension_);
}
if (dimensionCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (com.google.ads.googleads.v10.common.ProductConditionInfo) dimension_);
}
if (dimensionCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, (com.google.ads.googleads.v10.common.ProductItemIdInfo) dimension_);
}
if (dimensionCase_ == 12) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, (com.google.ads.googleads.v10.common.ProductTypeInfo) dimension_);
}
if (dimensionCase_ == 13) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, (com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo) dimension_);
}
if (dimensionCase_ == 14) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, (com.google.ads.googleads.v10.common.UnknownListingDimensionInfo) dimension_);
}
if (dimensionCase_ == 15) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, (com.google.ads.googleads.v10.common.ProductBrandInfo) dimension_);
}
if (dimensionCase_ == 16) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, (com.google.ads.googleads.v10.common.ProductCustomAttributeInfo) dimension_);
}
if (dimensionCase_ == 17) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(17, (com.google.ads.googleads.v10.common.ProductGroupingInfo) dimension_);
}
if (dimensionCase_ == 18) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, (com.google.ads.googleads.v10.common.ProductLabelsInfo) dimension_);
}
if (dimensionCase_ == 19) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(19, (com.google.ads.googleads.v10.common.ProductLegacyConditionInfo) dimension_);
}
if (dimensionCase_ == 20) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, (com.google.ads.googleads.v10.common.ProductTypeFullInfo) dimension_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v10.common.ListingDimensionInfo)) {
return super.equals(obj);
}
com.google.ads.googleads.v10.common.ListingDimensionInfo other = (com.google.ads.googleads.v10.common.ListingDimensionInfo) obj;
if (!getDimensionCase().equals(other.getDimensionCase())) return false;
switch (dimensionCase_) {
case 2:
if (!getHotelId()
.equals(other.getHotelId())) return false;
break;
case 3:
if (!getHotelClass()
.equals(other.getHotelClass())) return false;
break;
case 4:
if (!getHotelCountryRegion()
.equals(other.getHotelCountryRegion())) return false;
break;
case 5:
if (!getHotelState()
.equals(other.getHotelState())) return false;
break;
case 6:
if (!getHotelCity()
.equals(other.getHotelCity())) return false;
break;
case 13:
if (!getProductBiddingCategory()
.equals(other.getProductBiddingCategory())) return false;
break;
case 15:
if (!getProductBrand()
.equals(other.getProductBrand())) return false;
break;
case 8:
if (!getProductChannel()
.equals(other.getProductChannel())) return false;
break;
case 9:
if (!getProductChannelExclusivity()
.equals(other.getProductChannelExclusivity())) return false;
break;
case 10:
if (!getProductCondition()
.equals(other.getProductCondition())) return false;
break;
case 16:
if (!getProductCustomAttribute()
.equals(other.getProductCustomAttribute())) return false;
break;
case 11:
if (!getProductItemId()
.equals(other.getProductItemId())) return false;
break;
case 12:
if (!getProductType()
.equals(other.getProductType())) return false;
break;
case 17:
if (!getProductGrouping()
.equals(other.getProductGrouping())) return false;
break;
case 18:
if (!getProductLabels()
.equals(other.getProductLabels())) return false;
break;
case 19:
if (!getProductLegacyCondition()
.equals(other.getProductLegacyCondition())) return false;
break;
case 20:
if (!getProductTypeFull()
.equals(other.getProductTypeFull())) return false;
break;
case 14:
if (!getUnknownListingDimension()
.equals(other.getUnknownListingDimension())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (dimensionCase_) {
case 2:
hash = (37 * hash) + HOTEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getHotelId().hashCode();
break;
case 3:
hash = (37 * hash) + HOTEL_CLASS_FIELD_NUMBER;
hash = (53 * hash) + getHotelClass().hashCode();
break;
case 4:
hash = (37 * hash) + HOTEL_COUNTRY_REGION_FIELD_NUMBER;
hash = (53 * hash) + getHotelCountryRegion().hashCode();
break;
case 5:
hash = (37 * hash) + HOTEL_STATE_FIELD_NUMBER;
hash = (53 * hash) + getHotelState().hashCode();
break;
case 6:
hash = (37 * hash) + HOTEL_CITY_FIELD_NUMBER;
hash = (53 * hash) + getHotelCity().hashCode();
break;
case 13:
hash = (37 * hash) + PRODUCT_BIDDING_CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + getProductBiddingCategory().hashCode();
break;
case 15:
hash = (37 * hash) + PRODUCT_BRAND_FIELD_NUMBER;
hash = (53 * hash) + getProductBrand().hashCode();
break;
case 8:
hash = (37 * hash) + PRODUCT_CHANNEL_FIELD_NUMBER;
hash = (53 * hash) + getProductChannel().hashCode();
break;
case 9:
hash = (37 * hash) + PRODUCT_CHANNEL_EXCLUSIVITY_FIELD_NUMBER;
hash = (53 * hash) + getProductChannelExclusivity().hashCode();
break;
case 10:
hash = (37 * hash) + PRODUCT_CONDITION_FIELD_NUMBER;
hash = (53 * hash) + getProductCondition().hashCode();
break;
case 16:
hash = (37 * hash) + PRODUCT_CUSTOM_ATTRIBUTE_FIELD_NUMBER;
hash = (53 * hash) + getProductCustomAttribute().hashCode();
break;
case 11:
hash = (37 * hash) + PRODUCT_ITEM_ID_FIELD_NUMBER;
hash = (53 * hash) + getProductItemId().hashCode();
break;
case 12:
hash = (37 * hash) + PRODUCT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getProductType().hashCode();
break;
case 17:
hash = (37 * hash) + PRODUCT_GROUPING_FIELD_NUMBER;
hash = (53 * hash) + getProductGrouping().hashCode();
break;
case 18:
hash = (37 * hash) + PRODUCT_LABELS_FIELD_NUMBER;
hash = (53 * hash) + getProductLabels().hashCode();
break;
case 19:
hash = (37 * hash) + PRODUCT_LEGACY_CONDITION_FIELD_NUMBER;
hash = (53 * hash) + getProductLegacyCondition().hashCode();
break;
case 20:
hash = (37 * hash) + PRODUCT_TYPE_FULL_FIELD_NUMBER;
hash = (53 * hash) + getProductTypeFull().hashCode();
break;
case 14:
hash = (37 * hash) + UNKNOWN_LISTING_DIMENSION_FIELD_NUMBER;
hash = (53 * hash) + getUnknownListingDimension().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v10.common.ListingDimensionInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Listing dimensions for listing group criterion.
*
*
* Protobuf type {@code google.ads.googleads.v10.common.ListingDimensionInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v10.common.ListingDimensionInfo)
com.google.ads.googleads.v10.common.ListingDimensionInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.common.CriteriaProto.internal_static_google_ads_googleads_v10_common_ListingDimensionInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.common.CriteriaProto.internal_static_google_ads_googleads_v10_common_ListingDimensionInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.common.ListingDimensionInfo.class, com.google.ads.googleads.v10.common.ListingDimensionInfo.Builder.class);
}
// Construct using com.google.ads.googleads.v10.common.ListingDimensionInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
if (hotelIdBuilder_ != null) {
hotelIdBuilder_.clear();
}
if (hotelClassBuilder_ != null) {
hotelClassBuilder_.clear();
}
if (hotelCountryRegionBuilder_ != null) {
hotelCountryRegionBuilder_.clear();
}
if (hotelStateBuilder_ != null) {
hotelStateBuilder_.clear();
}
if (hotelCityBuilder_ != null) {
hotelCityBuilder_.clear();
}
if (productBiddingCategoryBuilder_ != null) {
productBiddingCategoryBuilder_.clear();
}
if (productBrandBuilder_ != null) {
productBrandBuilder_.clear();
}
if (productChannelBuilder_ != null) {
productChannelBuilder_.clear();
}
if (productChannelExclusivityBuilder_ != null) {
productChannelExclusivityBuilder_.clear();
}
if (productConditionBuilder_ != null) {
productConditionBuilder_.clear();
}
if (productCustomAttributeBuilder_ != null) {
productCustomAttributeBuilder_.clear();
}
if (productItemIdBuilder_ != null) {
productItemIdBuilder_.clear();
}
if (productTypeBuilder_ != null) {
productTypeBuilder_.clear();
}
if (productGroupingBuilder_ != null) {
productGroupingBuilder_.clear();
}
if (productLabelsBuilder_ != null) {
productLabelsBuilder_.clear();
}
if (productLegacyConditionBuilder_ != null) {
productLegacyConditionBuilder_.clear();
}
if (productTypeFullBuilder_ != null) {
productTypeFullBuilder_.clear();
}
if (unknownListingDimensionBuilder_ != null) {
unknownListingDimensionBuilder_.clear();
}
dimensionCase_ = 0;
dimension_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v10.common.CriteriaProto.internal_static_google_ads_googleads_v10_common_ListingDimensionInfo_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v10.common.ListingDimensionInfo getDefaultInstanceForType() {
return com.google.ads.googleads.v10.common.ListingDimensionInfo.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v10.common.ListingDimensionInfo build() {
com.google.ads.googleads.v10.common.ListingDimensionInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v10.common.ListingDimensionInfo buildPartial() {
com.google.ads.googleads.v10.common.ListingDimensionInfo result = new com.google.ads.googleads.v10.common.ListingDimensionInfo(this);
if (dimensionCase_ == 2) {
if (hotelIdBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = hotelIdBuilder_.build();
}
}
if (dimensionCase_ == 3) {
if (hotelClassBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = hotelClassBuilder_.build();
}
}
if (dimensionCase_ == 4) {
if (hotelCountryRegionBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = hotelCountryRegionBuilder_.build();
}
}
if (dimensionCase_ == 5) {
if (hotelStateBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = hotelStateBuilder_.build();
}
}
if (dimensionCase_ == 6) {
if (hotelCityBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = hotelCityBuilder_.build();
}
}
if (dimensionCase_ == 13) {
if (productBiddingCategoryBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productBiddingCategoryBuilder_.build();
}
}
if (dimensionCase_ == 15) {
if (productBrandBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productBrandBuilder_.build();
}
}
if (dimensionCase_ == 8) {
if (productChannelBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productChannelBuilder_.build();
}
}
if (dimensionCase_ == 9) {
if (productChannelExclusivityBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productChannelExclusivityBuilder_.build();
}
}
if (dimensionCase_ == 10) {
if (productConditionBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productConditionBuilder_.build();
}
}
if (dimensionCase_ == 16) {
if (productCustomAttributeBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productCustomAttributeBuilder_.build();
}
}
if (dimensionCase_ == 11) {
if (productItemIdBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productItemIdBuilder_.build();
}
}
if (dimensionCase_ == 12) {
if (productTypeBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productTypeBuilder_.build();
}
}
if (dimensionCase_ == 17) {
if (productGroupingBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productGroupingBuilder_.build();
}
}
if (dimensionCase_ == 18) {
if (productLabelsBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productLabelsBuilder_.build();
}
}
if (dimensionCase_ == 19) {
if (productLegacyConditionBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productLegacyConditionBuilder_.build();
}
}
if (dimensionCase_ == 20) {
if (productTypeFullBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = productTypeFullBuilder_.build();
}
}
if (dimensionCase_ == 14) {
if (unknownListingDimensionBuilder_ == null) {
result.dimension_ = dimension_;
} else {
result.dimension_ = unknownListingDimensionBuilder_.build();
}
}
result.dimensionCase_ = dimensionCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v10.common.ListingDimensionInfo) {
return mergeFrom((com.google.ads.googleads.v10.common.ListingDimensionInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v10.common.ListingDimensionInfo other) {
if (other == com.google.ads.googleads.v10.common.ListingDimensionInfo.getDefaultInstance()) return this;
switch (other.getDimensionCase()) {
case HOTEL_ID: {
mergeHotelId(other.getHotelId());
break;
}
case HOTEL_CLASS: {
mergeHotelClass(other.getHotelClass());
break;
}
case HOTEL_COUNTRY_REGION: {
mergeHotelCountryRegion(other.getHotelCountryRegion());
break;
}
case HOTEL_STATE: {
mergeHotelState(other.getHotelState());
break;
}
case HOTEL_CITY: {
mergeHotelCity(other.getHotelCity());
break;
}
case PRODUCT_BIDDING_CATEGORY: {
mergeProductBiddingCategory(other.getProductBiddingCategory());
break;
}
case PRODUCT_BRAND: {
mergeProductBrand(other.getProductBrand());
break;
}
case PRODUCT_CHANNEL: {
mergeProductChannel(other.getProductChannel());
break;
}
case PRODUCT_CHANNEL_EXCLUSIVITY: {
mergeProductChannelExclusivity(other.getProductChannelExclusivity());
break;
}
case PRODUCT_CONDITION: {
mergeProductCondition(other.getProductCondition());
break;
}
case PRODUCT_CUSTOM_ATTRIBUTE: {
mergeProductCustomAttribute(other.getProductCustomAttribute());
break;
}
case PRODUCT_ITEM_ID: {
mergeProductItemId(other.getProductItemId());
break;
}
case PRODUCT_TYPE: {
mergeProductType(other.getProductType());
break;
}
case PRODUCT_GROUPING: {
mergeProductGrouping(other.getProductGrouping());
break;
}
case PRODUCT_LABELS: {
mergeProductLabels(other.getProductLabels());
break;
}
case PRODUCT_LEGACY_CONDITION: {
mergeProductLegacyCondition(other.getProductLegacyCondition());
break;
}
case PRODUCT_TYPE_FULL: {
mergeProductTypeFull(other.getProductTypeFull());
break;
}
case UNKNOWN_LISTING_DIMENSION: {
mergeUnknownListingDimension(other.getUnknownListingDimension());
break;
}
case DIMENSION_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18: {
input.readMessage(
getHotelIdFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 2;
break;
} // case 18
case 26: {
input.readMessage(
getHotelClassFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 3;
break;
} // case 26
case 34: {
input.readMessage(
getHotelCountryRegionFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 4;
break;
} // case 34
case 42: {
input.readMessage(
getHotelStateFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 5;
break;
} // case 42
case 50: {
input.readMessage(
getHotelCityFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 6;
break;
} // case 50
case 66: {
input.readMessage(
getProductChannelFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 8;
break;
} // case 66
case 74: {
input.readMessage(
getProductChannelExclusivityFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 9;
break;
} // case 74
case 82: {
input.readMessage(
getProductConditionFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 10;
break;
} // case 82
case 90: {
input.readMessage(
getProductItemIdFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 11;
break;
} // case 90
case 98: {
input.readMessage(
getProductTypeFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 12;
break;
} // case 98
case 106: {
input.readMessage(
getProductBiddingCategoryFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 13;
break;
} // case 106
case 114: {
input.readMessage(
getUnknownListingDimensionFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 14;
break;
} // case 114
case 122: {
input.readMessage(
getProductBrandFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 15;
break;
} // case 122
case 130: {
input.readMessage(
getProductCustomAttributeFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 16;
break;
} // case 130
case 138: {
input.readMessage(
getProductGroupingFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 17;
break;
} // case 138
case 146: {
input.readMessage(
getProductLabelsFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 18;
break;
} // case 146
case 154: {
input.readMessage(
getProductLegacyConditionFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 19;
break;
} // case 154
case 162: {
input.readMessage(
getProductTypeFullFieldBuilder().getBuilder(),
extensionRegistry);
dimensionCase_ = 20;
break;
} // case 162
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int dimensionCase_ = 0;
private java.lang.Object dimension_;
public DimensionCase
getDimensionCase() {
return DimensionCase.forNumber(
dimensionCase_);
}
public Builder clearDimension() {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelIdInfo, com.google.ads.googleads.v10.common.HotelIdInfo.Builder, com.google.ads.googleads.v10.common.HotelIdInfoOrBuilder> hotelIdBuilder_;
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
* @return Whether the hotelId field is set.
*/
@java.lang.Override
public boolean hasHotelId() {
return dimensionCase_ == 2;
}
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
* @return The hotelId.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelIdInfo getHotelId() {
if (hotelIdBuilder_ == null) {
if (dimensionCase_ == 2) {
return (com.google.ads.googleads.v10.common.HotelIdInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelIdInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 2) {
return hotelIdBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.HotelIdInfo.getDefaultInstance();
}
}
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
*/
public Builder setHotelId(com.google.ads.googleads.v10.common.HotelIdInfo value) {
if (hotelIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
hotelIdBuilder_.setMessage(value);
}
dimensionCase_ = 2;
return this;
}
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
*/
public Builder setHotelId(
com.google.ads.googleads.v10.common.HotelIdInfo.Builder builderForValue) {
if (hotelIdBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
hotelIdBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 2;
return this;
}
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
*/
public Builder mergeHotelId(com.google.ads.googleads.v10.common.HotelIdInfo value) {
if (hotelIdBuilder_ == null) {
if (dimensionCase_ == 2 &&
dimension_ != com.google.ads.googleads.v10.common.HotelIdInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.HotelIdInfo.newBuilder((com.google.ads.googleads.v10.common.HotelIdInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 2) {
hotelIdBuilder_.mergeFrom(value);
} else {
hotelIdBuilder_.setMessage(value);
}
}
dimensionCase_ = 2;
return this;
}
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
*/
public Builder clearHotelId() {
if (hotelIdBuilder_ == null) {
if (dimensionCase_ == 2) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 2) {
dimensionCase_ = 0;
dimension_ = null;
}
hotelIdBuilder_.clear();
}
return this;
}
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
*/
public com.google.ads.googleads.v10.common.HotelIdInfo.Builder getHotelIdBuilder() {
return getHotelIdFieldBuilder().getBuilder();
}
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelIdInfoOrBuilder getHotelIdOrBuilder() {
if ((dimensionCase_ == 2) && (hotelIdBuilder_ != null)) {
return hotelIdBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 2) {
return (com.google.ads.googleads.v10.common.HotelIdInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelIdInfo.getDefaultInstance();
}
}
/**
*
* Advertiser-specific hotel ID.
*
*
* .google.ads.googleads.v10.common.HotelIdInfo hotel_id = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelIdInfo, com.google.ads.googleads.v10.common.HotelIdInfo.Builder, com.google.ads.googleads.v10.common.HotelIdInfoOrBuilder>
getHotelIdFieldBuilder() {
if (hotelIdBuilder_ == null) {
if (!(dimensionCase_ == 2)) {
dimension_ = com.google.ads.googleads.v10.common.HotelIdInfo.getDefaultInstance();
}
hotelIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelIdInfo, com.google.ads.googleads.v10.common.HotelIdInfo.Builder, com.google.ads.googleads.v10.common.HotelIdInfoOrBuilder>(
(com.google.ads.googleads.v10.common.HotelIdInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 2;
onChanged();;
return hotelIdBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelClassInfo, com.google.ads.googleads.v10.common.HotelClassInfo.Builder, com.google.ads.googleads.v10.common.HotelClassInfoOrBuilder> hotelClassBuilder_;
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
* @return Whether the hotelClass field is set.
*/
@java.lang.Override
public boolean hasHotelClass() {
return dimensionCase_ == 3;
}
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
* @return The hotelClass.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelClassInfo getHotelClass() {
if (hotelClassBuilder_ == null) {
if (dimensionCase_ == 3) {
return (com.google.ads.googleads.v10.common.HotelClassInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelClassInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 3) {
return hotelClassBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.HotelClassInfo.getDefaultInstance();
}
}
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
*/
public Builder setHotelClass(com.google.ads.googleads.v10.common.HotelClassInfo value) {
if (hotelClassBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
hotelClassBuilder_.setMessage(value);
}
dimensionCase_ = 3;
return this;
}
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
*/
public Builder setHotelClass(
com.google.ads.googleads.v10.common.HotelClassInfo.Builder builderForValue) {
if (hotelClassBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
hotelClassBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 3;
return this;
}
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
*/
public Builder mergeHotelClass(com.google.ads.googleads.v10.common.HotelClassInfo value) {
if (hotelClassBuilder_ == null) {
if (dimensionCase_ == 3 &&
dimension_ != com.google.ads.googleads.v10.common.HotelClassInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.HotelClassInfo.newBuilder((com.google.ads.googleads.v10.common.HotelClassInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 3) {
hotelClassBuilder_.mergeFrom(value);
} else {
hotelClassBuilder_.setMessage(value);
}
}
dimensionCase_ = 3;
return this;
}
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
*/
public Builder clearHotelClass() {
if (hotelClassBuilder_ == null) {
if (dimensionCase_ == 3) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 3) {
dimensionCase_ = 0;
dimension_ = null;
}
hotelClassBuilder_.clear();
}
return this;
}
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
*/
public com.google.ads.googleads.v10.common.HotelClassInfo.Builder getHotelClassBuilder() {
return getHotelClassFieldBuilder().getBuilder();
}
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelClassInfoOrBuilder getHotelClassOrBuilder() {
if ((dimensionCase_ == 3) && (hotelClassBuilder_ != null)) {
return hotelClassBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 3) {
return (com.google.ads.googleads.v10.common.HotelClassInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelClassInfo.getDefaultInstance();
}
}
/**
*
* Class of the hotel as a number of stars 1 to 5.
*
*
* .google.ads.googleads.v10.common.HotelClassInfo hotel_class = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelClassInfo, com.google.ads.googleads.v10.common.HotelClassInfo.Builder, com.google.ads.googleads.v10.common.HotelClassInfoOrBuilder>
getHotelClassFieldBuilder() {
if (hotelClassBuilder_ == null) {
if (!(dimensionCase_ == 3)) {
dimension_ = com.google.ads.googleads.v10.common.HotelClassInfo.getDefaultInstance();
}
hotelClassBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelClassInfo, com.google.ads.googleads.v10.common.HotelClassInfo.Builder, com.google.ads.googleads.v10.common.HotelClassInfoOrBuilder>(
(com.google.ads.googleads.v10.common.HotelClassInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 3;
onChanged();;
return hotelClassBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelCountryRegionInfo, com.google.ads.googleads.v10.common.HotelCountryRegionInfo.Builder, com.google.ads.googleads.v10.common.HotelCountryRegionInfoOrBuilder> hotelCountryRegionBuilder_;
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
* @return Whether the hotelCountryRegion field is set.
*/
@java.lang.Override
public boolean hasHotelCountryRegion() {
return dimensionCase_ == 4;
}
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
* @return The hotelCountryRegion.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelCountryRegionInfo getHotelCountryRegion() {
if (hotelCountryRegionBuilder_ == null) {
if (dimensionCase_ == 4) {
return (com.google.ads.googleads.v10.common.HotelCountryRegionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelCountryRegionInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 4) {
return hotelCountryRegionBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.HotelCountryRegionInfo.getDefaultInstance();
}
}
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
*/
public Builder setHotelCountryRegion(com.google.ads.googleads.v10.common.HotelCountryRegionInfo value) {
if (hotelCountryRegionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
hotelCountryRegionBuilder_.setMessage(value);
}
dimensionCase_ = 4;
return this;
}
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
*/
public Builder setHotelCountryRegion(
com.google.ads.googleads.v10.common.HotelCountryRegionInfo.Builder builderForValue) {
if (hotelCountryRegionBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
hotelCountryRegionBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 4;
return this;
}
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
*/
public Builder mergeHotelCountryRegion(com.google.ads.googleads.v10.common.HotelCountryRegionInfo value) {
if (hotelCountryRegionBuilder_ == null) {
if (dimensionCase_ == 4 &&
dimension_ != com.google.ads.googleads.v10.common.HotelCountryRegionInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.HotelCountryRegionInfo.newBuilder((com.google.ads.googleads.v10.common.HotelCountryRegionInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 4) {
hotelCountryRegionBuilder_.mergeFrom(value);
} else {
hotelCountryRegionBuilder_.setMessage(value);
}
}
dimensionCase_ = 4;
return this;
}
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
*/
public Builder clearHotelCountryRegion() {
if (hotelCountryRegionBuilder_ == null) {
if (dimensionCase_ == 4) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 4) {
dimensionCase_ = 0;
dimension_ = null;
}
hotelCountryRegionBuilder_.clear();
}
return this;
}
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
*/
public com.google.ads.googleads.v10.common.HotelCountryRegionInfo.Builder getHotelCountryRegionBuilder() {
return getHotelCountryRegionFieldBuilder().getBuilder();
}
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelCountryRegionInfoOrBuilder getHotelCountryRegionOrBuilder() {
if ((dimensionCase_ == 4) && (hotelCountryRegionBuilder_ != null)) {
return hotelCountryRegionBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 4) {
return (com.google.ads.googleads.v10.common.HotelCountryRegionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelCountryRegionInfo.getDefaultInstance();
}
}
/**
*
* Country or Region the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCountryRegionInfo hotel_country_region = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelCountryRegionInfo, com.google.ads.googleads.v10.common.HotelCountryRegionInfo.Builder, com.google.ads.googleads.v10.common.HotelCountryRegionInfoOrBuilder>
getHotelCountryRegionFieldBuilder() {
if (hotelCountryRegionBuilder_ == null) {
if (!(dimensionCase_ == 4)) {
dimension_ = com.google.ads.googleads.v10.common.HotelCountryRegionInfo.getDefaultInstance();
}
hotelCountryRegionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelCountryRegionInfo, com.google.ads.googleads.v10.common.HotelCountryRegionInfo.Builder, com.google.ads.googleads.v10.common.HotelCountryRegionInfoOrBuilder>(
(com.google.ads.googleads.v10.common.HotelCountryRegionInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 4;
onChanged();;
return hotelCountryRegionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelStateInfo, com.google.ads.googleads.v10.common.HotelStateInfo.Builder, com.google.ads.googleads.v10.common.HotelStateInfoOrBuilder> hotelStateBuilder_;
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
* @return Whether the hotelState field is set.
*/
@java.lang.Override
public boolean hasHotelState() {
return dimensionCase_ == 5;
}
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
* @return The hotelState.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelStateInfo getHotelState() {
if (hotelStateBuilder_ == null) {
if (dimensionCase_ == 5) {
return (com.google.ads.googleads.v10.common.HotelStateInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelStateInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 5) {
return hotelStateBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.HotelStateInfo.getDefaultInstance();
}
}
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
*/
public Builder setHotelState(com.google.ads.googleads.v10.common.HotelStateInfo value) {
if (hotelStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
hotelStateBuilder_.setMessage(value);
}
dimensionCase_ = 5;
return this;
}
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
*/
public Builder setHotelState(
com.google.ads.googleads.v10.common.HotelStateInfo.Builder builderForValue) {
if (hotelStateBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
hotelStateBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 5;
return this;
}
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
*/
public Builder mergeHotelState(com.google.ads.googleads.v10.common.HotelStateInfo value) {
if (hotelStateBuilder_ == null) {
if (dimensionCase_ == 5 &&
dimension_ != com.google.ads.googleads.v10.common.HotelStateInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.HotelStateInfo.newBuilder((com.google.ads.googleads.v10.common.HotelStateInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 5) {
hotelStateBuilder_.mergeFrom(value);
} else {
hotelStateBuilder_.setMessage(value);
}
}
dimensionCase_ = 5;
return this;
}
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
*/
public Builder clearHotelState() {
if (hotelStateBuilder_ == null) {
if (dimensionCase_ == 5) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 5) {
dimensionCase_ = 0;
dimension_ = null;
}
hotelStateBuilder_.clear();
}
return this;
}
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
*/
public com.google.ads.googleads.v10.common.HotelStateInfo.Builder getHotelStateBuilder() {
return getHotelStateFieldBuilder().getBuilder();
}
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelStateInfoOrBuilder getHotelStateOrBuilder() {
if ((dimensionCase_ == 5) && (hotelStateBuilder_ != null)) {
return hotelStateBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 5) {
return (com.google.ads.googleads.v10.common.HotelStateInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelStateInfo.getDefaultInstance();
}
}
/**
*
* State the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelStateInfo hotel_state = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelStateInfo, com.google.ads.googleads.v10.common.HotelStateInfo.Builder, com.google.ads.googleads.v10.common.HotelStateInfoOrBuilder>
getHotelStateFieldBuilder() {
if (hotelStateBuilder_ == null) {
if (!(dimensionCase_ == 5)) {
dimension_ = com.google.ads.googleads.v10.common.HotelStateInfo.getDefaultInstance();
}
hotelStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelStateInfo, com.google.ads.googleads.v10.common.HotelStateInfo.Builder, com.google.ads.googleads.v10.common.HotelStateInfoOrBuilder>(
(com.google.ads.googleads.v10.common.HotelStateInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 5;
onChanged();;
return hotelStateBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelCityInfo, com.google.ads.googleads.v10.common.HotelCityInfo.Builder, com.google.ads.googleads.v10.common.HotelCityInfoOrBuilder> hotelCityBuilder_;
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
* @return Whether the hotelCity field is set.
*/
@java.lang.Override
public boolean hasHotelCity() {
return dimensionCase_ == 6;
}
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
* @return The hotelCity.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelCityInfo getHotelCity() {
if (hotelCityBuilder_ == null) {
if (dimensionCase_ == 6) {
return (com.google.ads.googleads.v10.common.HotelCityInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelCityInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 6) {
return hotelCityBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.HotelCityInfo.getDefaultInstance();
}
}
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
*/
public Builder setHotelCity(com.google.ads.googleads.v10.common.HotelCityInfo value) {
if (hotelCityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
hotelCityBuilder_.setMessage(value);
}
dimensionCase_ = 6;
return this;
}
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
*/
public Builder setHotelCity(
com.google.ads.googleads.v10.common.HotelCityInfo.Builder builderForValue) {
if (hotelCityBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
hotelCityBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 6;
return this;
}
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
*/
public Builder mergeHotelCity(com.google.ads.googleads.v10.common.HotelCityInfo value) {
if (hotelCityBuilder_ == null) {
if (dimensionCase_ == 6 &&
dimension_ != com.google.ads.googleads.v10.common.HotelCityInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.HotelCityInfo.newBuilder((com.google.ads.googleads.v10.common.HotelCityInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 6) {
hotelCityBuilder_.mergeFrom(value);
} else {
hotelCityBuilder_.setMessage(value);
}
}
dimensionCase_ = 6;
return this;
}
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
*/
public Builder clearHotelCity() {
if (hotelCityBuilder_ == null) {
if (dimensionCase_ == 6) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 6) {
dimensionCase_ = 0;
dimension_ = null;
}
hotelCityBuilder_.clear();
}
return this;
}
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
*/
public com.google.ads.googleads.v10.common.HotelCityInfo.Builder getHotelCityBuilder() {
return getHotelCityFieldBuilder().getBuilder();
}
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.HotelCityInfoOrBuilder getHotelCityOrBuilder() {
if ((dimensionCase_ == 6) && (hotelCityBuilder_ != null)) {
return hotelCityBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 6) {
return (com.google.ads.googleads.v10.common.HotelCityInfo) dimension_;
}
return com.google.ads.googleads.v10.common.HotelCityInfo.getDefaultInstance();
}
}
/**
*
* City the hotel is located in.
*
*
* .google.ads.googleads.v10.common.HotelCityInfo hotel_city = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelCityInfo, com.google.ads.googleads.v10.common.HotelCityInfo.Builder, com.google.ads.googleads.v10.common.HotelCityInfoOrBuilder>
getHotelCityFieldBuilder() {
if (hotelCityBuilder_ == null) {
if (!(dimensionCase_ == 6)) {
dimension_ = com.google.ads.googleads.v10.common.HotelCityInfo.getDefaultInstance();
}
hotelCityBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.HotelCityInfo, com.google.ads.googleads.v10.common.HotelCityInfo.Builder, com.google.ads.googleads.v10.common.HotelCityInfoOrBuilder>(
(com.google.ads.googleads.v10.common.HotelCityInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 6;
onChanged();;
return hotelCityBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo, com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.Builder, com.google.ads.googleads.v10.common.ProductBiddingCategoryInfoOrBuilder> productBiddingCategoryBuilder_;
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
* @return Whether the productBiddingCategory field is set.
*/
@java.lang.Override
public boolean hasProductBiddingCategory() {
return dimensionCase_ == 13;
}
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
* @return The productBiddingCategory.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo getProductBiddingCategory() {
if (productBiddingCategoryBuilder_ == null) {
if (dimensionCase_ == 13) {
return (com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 13) {
return productBiddingCategoryBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.getDefaultInstance();
}
}
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
*/
public Builder setProductBiddingCategory(com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo value) {
if (productBiddingCategoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productBiddingCategoryBuilder_.setMessage(value);
}
dimensionCase_ = 13;
return this;
}
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
*/
public Builder setProductBiddingCategory(
com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.Builder builderForValue) {
if (productBiddingCategoryBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productBiddingCategoryBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 13;
return this;
}
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
*/
public Builder mergeProductBiddingCategory(com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo value) {
if (productBiddingCategoryBuilder_ == null) {
if (dimensionCase_ == 13 &&
dimension_ != com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.newBuilder((com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 13) {
productBiddingCategoryBuilder_.mergeFrom(value);
} else {
productBiddingCategoryBuilder_.setMessage(value);
}
}
dimensionCase_ = 13;
return this;
}
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
*/
public Builder clearProductBiddingCategory() {
if (productBiddingCategoryBuilder_ == null) {
if (dimensionCase_ == 13) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 13) {
dimensionCase_ = 0;
dimension_ = null;
}
productBiddingCategoryBuilder_.clear();
}
return this;
}
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
*/
public com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.Builder getProductBiddingCategoryBuilder() {
return getProductBiddingCategoryFieldBuilder().getBuilder();
}
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductBiddingCategoryInfoOrBuilder getProductBiddingCategoryOrBuilder() {
if ((dimensionCase_ == 13) && (productBiddingCategoryBuilder_ != null)) {
return productBiddingCategoryBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 13) {
return (com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.getDefaultInstance();
}
}
/**
*
* Bidding category of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBiddingCategoryInfo product_bidding_category = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo, com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.Builder, com.google.ads.googleads.v10.common.ProductBiddingCategoryInfoOrBuilder>
getProductBiddingCategoryFieldBuilder() {
if (productBiddingCategoryBuilder_ == null) {
if (!(dimensionCase_ == 13)) {
dimension_ = com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.getDefaultInstance();
}
productBiddingCategoryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo, com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo.Builder, com.google.ads.googleads.v10.common.ProductBiddingCategoryInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductBiddingCategoryInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 13;
onChanged();;
return productBiddingCategoryBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductBrandInfo, com.google.ads.googleads.v10.common.ProductBrandInfo.Builder, com.google.ads.googleads.v10.common.ProductBrandInfoOrBuilder> productBrandBuilder_;
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
* @return Whether the productBrand field is set.
*/
@java.lang.Override
public boolean hasProductBrand() {
return dimensionCase_ == 15;
}
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
* @return The productBrand.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductBrandInfo getProductBrand() {
if (productBrandBuilder_ == null) {
if (dimensionCase_ == 15) {
return (com.google.ads.googleads.v10.common.ProductBrandInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductBrandInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 15) {
return productBrandBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductBrandInfo.getDefaultInstance();
}
}
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
*/
public Builder setProductBrand(com.google.ads.googleads.v10.common.ProductBrandInfo value) {
if (productBrandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productBrandBuilder_.setMessage(value);
}
dimensionCase_ = 15;
return this;
}
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
*/
public Builder setProductBrand(
com.google.ads.googleads.v10.common.ProductBrandInfo.Builder builderForValue) {
if (productBrandBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productBrandBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 15;
return this;
}
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
*/
public Builder mergeProductBrand(com.google.ads.googleads.v10.common.ProductBrandInfo value) {
if (productBrandBuilder_ == null) {
if (dimensionCase_ == 15 &&
dimension_ != com.google.ads.googleads.v10.common.ProductBrandInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductBrandInfo.newBuilder((com.google.ads.googleads.v10.common.ProductBrandInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 15) {
productBrandBuilder_.mergeFrom(value);
} else {
productBrandBuilder_.setMessage(value);
}
}
dimensionCase_ = 15;
return this;
}
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
*/
public Builder clearProductBrand() {
if (productBrandBuilder_ == null) {
if (dimensionCase_ == 15) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 15) {
dimensionCase_ = 0;
dimension_ = null;
}
productBrandBuilder_.clear();
}
return this;
}
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
*/
public com.google.ads.googleads.v10.common.ProductBrandInfo.Builder getProductBrandBuilder() {
return getProductBrandFieldBuilder().getBuilder();
}
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductBrandInfoOrBuilder getProductBrandOrBuilder() {
if ((dimensionCase_ == 15) && (productBrandBuilder_ != null)) {
return productBrandBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 15) {
return (com.google.ads.googleads.v10.common.ProductBrandInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductBrandInfo.getDefaultInstance();
}
}
/**
*
* Brand of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductBrandInfo product_brand = 15;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductBrandInfo, com.google.ads.googleads.v10.common.ProductBrandInfo.Builder, com.google.ads.googleads.v10.common.ProductBrandInfoOrBuilder>
getProductBrandFieldBuilder() {
if (productBrandBuilder_ == null) {
if (!(dimensionCase_ == 15)) {
dimension_ = com.google.ads.googleads.v10.common.ProductBrandInfo.getDefaultInstance();
}
productBrandBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductBrandInfo, com.google.ads.googleads.v10.common.ProductBrandInfo.Builder, com.google.ads.googleads.v10.common.ProductBrandInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductBrandInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 15;
onChanged();;
return productBrandBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductChannelInfo, com.google.ads.googleads.v10.common.ProductChannelInfo.Builder, com.google.ads.googleads.v10.common.ProductChannelInfoOrBuilder> productChannelBuilder_;
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
* @return Whether the productChannel field is set.
*/
@java.lang.Override
public boolean hasProductChannel() {
return dimensionCase_ == 8;
}
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
* @return The productChannel.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductChannelInfo getProductChannel() {
if (productChannelBuilder_ == null) {
if (dimensionCase_ == 8) {
return (com.google.ads.googleads.v10.common.ProductChannelInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductChannelInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 8) {
return productChannelBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductChannelInfo.getDefaultInstance();
}
}
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
*/
public Builder setProductChannel(com.google.ads.googleads.v10.common.ProductChannelInfo value) {
if (productChannelBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productChannelBuilder_.setMessage(value);
}
dimensionCase_ = 8;
return this;
}
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
*/
public Builder setProductChannel(
com.google.ads.googleads.v10.common.ProductChannelInfo.Builder builderForValue) {
if (productChannelBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productChannelBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 8;
return this;
}
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
*/
public Builder mergeProductChannel(com.google.ads.googleads.v10.common.ProductChannelInfo value) {
if (productChannelBuilder_ == null) {
if (dimensionCase_ == 8 &&
dimension_ != com.google.ads.googleads.v10.common.ProductChannelInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductChannelInfo.newBuilder((com.google.ads.googleads.v10.common.ProductChannelInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 8) {
productChannelBuilder_.mergeFrom(value);
} else {
productChannelBuilder_.setMessage(value);
}
}
dimensionCase_ = 8;
return this;
}
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
*/
public Builder clearProductChannel() {
if (productChannelBuilder_ == null) {
if (dimensionCase_ == 8) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 8) {
dimensionCase_ = 0;
dimension_ = null;
}
productChannelBuilder_.clear();
}
return this;
}
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
*/
public com.google.ads.googleads.v10.common.ProductChannelInfo.Builder getProductChannelBuilder() {
return getProductChannelFieldBuilder().getBuilder();
}
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductChannelInfoOrBuilder getProductChannelOrBuilder() {
if ((dimensionCase_ == 8) && (productChannelBuilder_ != null)) {
return productChannelBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 8) {
return (com.google.ads.googleads.v10.common.ProductChannelInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductChannelInfo.getDefaultInstance();
}
}
/**
*
* Locality of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelInfo product_channel = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductChannelInfo, com.google.ads.googleads.v10.common.ProductChannelInfo.Builder, com.google.ads.googleads.v10.common.ProductChannelInfoOrBuilder>
getProductChannelFieldBuilder() {
if (productChannelBuilder_ == null) {
if (!(dimensionCase_ == 8)) {
dimension_ = com.google.ads.googleads.v10.common.ProductChannelInfo.getDefaultInstance();
}
productChannelBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductChannelInfo, com.google.ads.googleads.v10.common.ProductChannelInfo.Builder, com.google.ads.googleads.v10.common.ProductChannelInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductChannelInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 8;
onChanged();;
return productChannelBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo, com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.Builder, com.google.ads.googleads.v10.common.ProductChannelExclusivityInfoOrBuilder> productChannelExclusivityBuilder_;
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
* @return Whether the productChannelExclusivity field is set.
*/
@java.lang.Override
public boolean hasProductChannelExclusivity() {
return dimensionCase_ == 9;
}
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
* @return The productChannelExclusivity.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo getProductChannelExclusivity() {
if (productChannelExclusivityBuilder_ == null) {
if (dimensionCase_ == 9) {
return (com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 9) {
return productChannelExclusivityBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.getDefaultInstance();
}
}
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
*/
public Builder setProductChannelExclusivity(com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo value) {
if (productChannelExclusivityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productChannelExclusivityBuilder_.setMessage(value);
}
dimensionCase_ = 9;
return this;
}
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
*/
public Builder setProductChannelExclusivity(
com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.Builder builderForValue) {
if (productChannelExclusivityBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productChannelExclusivityBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 9;
return this;
}
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
*/
public Builder mergeProductChannelExclusivity(com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo value) {
if (productChannelExclusivityBuilder_ == null) {
if (dimensionCase_ == 9 &&
dimension_ != com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.newBuilder((com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 9) {
productChannelExclusivityBuilder_.mergeFrom(value);
} else {
productChannelExclusivityBuilder_.setMessage(value);
}
}
dimensionCase_ = 9;
return this;
}
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
*/
public Builder clearProductChannelExclusivity() {
if (productChannelExclusivityBuilder_ == null) {
if (dimensionCase_ == 9) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 9) {
dimensionCase_ = 0;
dimension_ = null;
}
productChannelExclusivityBuilder_.clear();
}
return this;
}
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
*/
public com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.Builder getProductChannelExclusivityBuilder() {
return getProductChannelExclusivityFieldBuilder().getBuilder();
}
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductChannelExclusivityInfoOrBuilder getProductChannelExclusivityOrBuilder() {
if ((dimensionCase_ == 9) && (productChannelExclusivityBuilder_ != null)) {
return productChannelExclusivityBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 9) {
return (com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.getDefaultInstance();
}
}
/**
*
* Availability of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductChannelExclusivityInfo product_channel_exclusivity = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo, com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.Builder, com.google.ads.googleads.v10.common.ProductChannelExclusivityInfoOrBuilder>
getProductChannelExclusivityFieldBuilder() {
if (productChannelExclusivityBuilder_ == null) {
if (!(dimensionCase_ == 9)) {
dimension_ = com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.getDefaultInstance();
}
productChannelExclusivityBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo, com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo.Builder, com.google.ads.googleads.v10.common.ProductChannelExclusivityInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductChannelExclusivityInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 9;
onChanged();;
return productChannelExclusivityBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductConditionInfo, com.google.ads.googleads.v10.common.ProductConditionInfo.Builder, com.google.ads.googleads.v10.common.ProductConditionInfoOrBuilder> productConditionBuilder_;
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
* @return Whether the productCondition field is set.
*/
@java.lang.Override
public boolean hasProductCondition() {
return dimensionCase_ == 10;
}
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
* @return The productCondition.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductConditionInfo getProductCondition() {
if (productConditionBuilder_ == null) {
if (dimensionCase_ == 10) {
return (com.google.ads.googleads.v10.common.ProductConditionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductConditionInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 10) {
return productConditionBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductConditionInfo.getDefaultInstance();
}
}
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
*/
public Builder setProductCondition(com.google.ads.googleads.v10.common.ProductConditionInfo value) {
if (productConditionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productConditionBuilder_.setMessage(value);
}
dimensionCase_ = 10;
return this;
}
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
*/
public Builder setProductCondition(
com.google.ads.googleads.v10.common.ProductConditionInfo.Builder builderForValue) {
if (productConditionBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productConditionBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 10;
return this;
}
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
*/
public Builder mergeProductCondition(com.google.ads.googleads.v10.common.ProductConditionInfo value) {
if (productConditionBuilder_ == null) {
if (dimensionCase_ == 10 &&
dimension_ != com.google.ads.googleads.v10.common.ProductConditionInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductConditionInfo.newBuilder((com.google.ads.googleads.v10.common.ProductConditionInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 10) {
productConditionBuilder_.mergeFrom(value);
} else {
productConditionBuilder_.setMessage(value);
}
}
dimensionCase_ = 10;
return this;
}
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
*/
public Builder clearProductCondition() {
if (productConditionBuilder_ == null) {
if (dimensionCase_ == 10) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 10) {
dimensionCase_ = 0;
dimension_ = null;
}
productConditionBuilder_.clear();
}
return this;
}
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
*/
public com.google.ads.googleads.v10.common.ProductConditionInfo.Builder getProductConditionBuilder() {
return getProductConditionFieldBuilder().getBuilder();
}
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductConditionInfoOrBuilder getProductConditionOrBuilder() {
if ((dimensionCase_ == 10) && (productConditionBuilder_ != null)) {
return productConditionBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 10) {
return (com.google.ads.googleads.v10.common.ProductConditionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductConditionInfo.getDefaultInstance();
}
}
/**
*
* Condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductConditionInfo product_condition = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductConditionInfo, com.google.ads.googleads.v10.common.ProductConditionInfo.Builder, com.google.ads.googleads.v10.common.ProductConditionInfoOrBuilder>
getProductConditionFieldBuilder() {
if (productConditionBuilder_ == null) {
if (!(dimensionCase_ == 10)) {
dimension_ = com.google.ads.googleads.v10.common.ProductConditionInfo.getDefaultInstance();
}
productConditionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductConditionInfo, com.google.ads.googleads.v10.common.ProductConditionInfo.Builder, com.google.ads.googleads.v10.common.ProductConditionInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductConditionInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 10;
onChanged();;
return productConditionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductCustomAttributeInfo, com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.Builder, com.google.ads.googleads.v10.common.ProductCustomAttributeInfoOrBuilder> productCustomAttributeBuilder_;
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
* @return Whether the productCustomAttribute field is set.
*/
@java.lang.Override
public boolean hasProductCustomAttribute() {
return dimensionCase_ == 16;
}
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
* @return The productCustomAttribute.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductCustomAttributeInfo getProductCustomAttribute() {
if (productCustomAttributeBuilder_ == null) {
if (dimensionCase_ == 16) {
return (com.google.ads.googleads.v10.common.ProductCustomAttributeInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 16) {
return productCustomAttributeBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.getDefaultInstance();
}
}
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
*/
public Builder setProductCustomAttribute(com.google.ads.googleads.v10.common.ProductCustomAttributeInfo value) {
if (productCustomAttributeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productCustomAttributeBuilder_.setMessage(value);
}
dimensionCase_ = 16;
return this;
}
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
*/
public Builder setProductCustomAttribute(
com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.Builder builderForValue) {
if (productCustomAttributeBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productCustomAttributeBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 16;
return this;
}
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
*/
public Builder mergeProductCustomAttribute(com.google.ads.googleads.v10.common.ProductCustomAttributeInfo value) {
if (productCustomAttributeBuilder_ == null) {
if (dimensionCase_ == 16 &&
dimension_ != com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.newBuilder((com.google.ads.googleads.v10.common.ProductCustomAttributeInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 16) {
productCustomAttributeBuilder_.mergeFrom(value);
} else {
productCustomAttributeBuilder_.setMessage(value);
}
}
dimensionCase_ = 16;
return this;
}
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
*/
public Builder clearProductCustomAttribute() {
if (productCustomAttributeBuilder_ == null) {
if (dimensionCase_ == 16) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 16) {
dimensionCase_ = 0;
dimension_ = null;
}
productCustomAttributeBuilder_.clear();
}
return this;
}
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
*/
public com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.Builder getProductCustomAttributeBuilder() {
return getProductCustomAttributeFieldBuilder().getBuilder();
}
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductCustomAttributeInfoOrBuilder getProductCustomAttributeOrBuilder() {
if ((dimensionCase_ == 16) && (productCustomAttributeBuilder_ != null)) {
return productCustomAttributeBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 16) {
return (com.google.ads.googleads.v10.common.ProductCustomAttributeInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.getDefaultInstance();
}
}
/**
*
* Custom attribute of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductCustomAttributeInfo product_custom_attribute = 16;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductCustomAttributeInfo, com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.Builder, com.google.ads.googleads.v10.common.ProductCustomAttributeInfoOrBuilder>
getProductCustomAttributeFieldBuilder() {
if (productCustomAttributeBuilder_ == null) {
if (!(dimensionCase_ == 16)) {
dimension_ = com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.getDefaultInstance();
}
productCustomAttributeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductCustomAttributeInfo, com.google.ads.googleads.v10.common.ProductCustomAttributeInfo.Builder, com.google.ads.googleads.v10.common.ProductCustomAttributeInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductCustomAttributeInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 16;
onChanged();;
return productCustomAttributeBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductItemIdInfo, com.google.ads.googleads.v10.common.ProductItemIdInfo.Builder, com.google.ads.googleads.v10.common.ProductItemIdInfoOrBuilder> productItemIdBuilder_;
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
* @return Whether the productItemId field is set.
*/
@java.lang.Override
public boolean hasProductItemId() {
return dimensionCase_ == 11;
}
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
* @return The productItemId.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductItemIdInfo getProductItemId() {
if (productItemIdBuilder_ == null) {
if (dimensionCase_ == 11) {
return (com.google.ads.googleads.v10.common.ProductItemIdInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductItemIdInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 11) {
return productItemIdBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductItemIdInfo.getDefaultInstance();
}
}
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
*/
public Builder setProductItemId(com.google.ads.googleads.v10.common.ProductItemIdInfo value) {
if (productItemIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productItemIdBuilder_.setMessage(value);
}
dimensionCase_ = 11;
return this;
}
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
*/
public Builder setProductItemId(
com.google.ads.googleads.v10.common.ProductItemIdInfo.Builder builderForValue) {
if (productItemIdBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productItemIdBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 11;
return this;
}
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
*/
public Builder mergeProductItemId(com.google.ads.googleads.v10.common.ProductItemIdInfo value) {
if (productItemIdBuilder_ == null) {
if (dimensionCase_ == 11 &&
dimension_ != com.google.ads.googleads.v10.common.ProductItemIdInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductItemIdInfo.newBuilder((com.google.ads.googleads.v10.common.ProductItemIdInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 11) {
productItemIdBuilder_.mergeFrom(value);
} else {
productItemIdBuilder_.setMessage(value);
}
}
dimensionCase_ = 11;
return this;
}
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
*/
public Builder clearProductItemId() {
if (productItemIdBuilder_ == null) {
if (dimensionCase_ == 11) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 11) {
dimensionCase_ = 0;
dimension_ = null;
}
productItemIdBuilder_.clear();
}
return this;
}
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
*/
public com.google.ads.googleads.v10.common.ProductItemIdInfo.Builder getProductItemIdBuilder() {
return getProductItemIdFieldBuilder().getBuilder();
}
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductItemIdInfoOrBuilder getProductItemIdOrBuilder() {
if ((dimensionCase_ == 11) && (productItemIdBuilder_ != null)) {
return productItemIdBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 11) {
return (com.google.ads.googleads.v10.common.ProductItemIdInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductItemIdInfo.getDefaultInstance();
}
}
/**
*
* Item id of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductItemIdInfo product_item_id = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductItemIdInfo, com.google.ads.googleads.v10.common.ProductItemIdInfo.Builder, com.google.ads.googleads.v10.common.ProductItemIdInfoOrBuilder>
getProductItemIdFieldBuilder() {
if (productItemIdBuilder_ == null) {
if (!(dimensionCase_ == 11)) {
dimension_ = com.google.ads.googleads.v10.common.ProductItemIdInfo.getDefaultInstance();
}
productItemIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductItemIdInfo, com.google.ads.googleads.v10.common.ProductItemIdInfo.Builder, com.google.ads.googleads.v10.common.ProductItemIdInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductItemIdInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 11;
onChanged();;
return productItemIdBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductTypeInfo, com.google.ads.googleads.v10.common.ProductTypeInfo.Builder, com.google.ads.googleads.v10.common.ProductTypeInfoOrBuilder> productTypeBuilder_;
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
* @return Whether the productType field is set.
*/
@java.lang.Override
public boolean hasProductType() {
return dimensionCase_ == 12;
}
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
* @return The productType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductTypeInfo getProductType() {
if (productTypeBuilder_ == null) {
if (dimensionCase_ == 12) {
return (com.google.ads.googleads.v10.common.ProductTypeInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductTypeInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 12) {
return productTypeBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductTypeInfo.getDefaultInstance();
}
}
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
*/
public Builder setProductType(com.google.ads.googleads.v10.common.ProductTypeInfo value) {
if (productTypeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productTypeBuilder_.setMessage(value);
}
dimensionCase_ = 12;
return this;
}
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
*/
public Builder setProductType(
com.google.ads.googleads.v10.common.ProductTypeInfo.Builder builderForValue) {
if (productTypeBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productTypeBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 12;
return this;
}
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
*/
public Builder mergeProductType(com.google.ads.googleads.v10.common.ProductTypeInfo value) {
if (productTypeBuilder_ == null) {
if (dimensionCase_ == 12 &&
dimension_ != com.google.ads.googleads.v10.common.ProductTypeInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductTypeInfo.newBuilder((com.google.ads.googleads.v10.common.ProductTypeInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 12) {
productTypeBuilder_.mergeFrom(value);
} else {
productTypeBuilder_.setMessage(value);
}
}
dimensionCase_ = 12;
return this;
}
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
*/
public Builder clearProductType() {
if (productTypeBuilder_ == null) {
if (dimensionCase_ == 12) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 12) {
dimensionCase_ = 0;
dimension_ = null;
}
productTypeBuilder_.clear();
}
return this;
}
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
*/
public com.google.ads.googleads.v10.common.ProductTypeInfo.Builder getProductTypeBuilder() {
return getProductTypeFieldBuilder().getBuilder();
}
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductTypeInfoOrBuilder getProductTypeOrBuilder() {
if ((dimensionCase_ == 12) && (productTypeBuilder_ != null)) {
return productTypeBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 12) {
return (com.google.ads.googleads.v10.common.ProductTypeInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductTypeInfo.getDefaultInstance();
}
}
/**
*
* Type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeInfo product_type = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductTypeInfo, com.google.ads.googleads.v10.common.ProductTypeInfo.Builder, com.google.ads.googleads.v10.common.ProductTypeInfoOrBuilder>
getProductTypeFieldBuilder() {
if (productTypeBuilder_ == null) {
if (!(dimensionCase_ == 12)) {
dimension_ = com.google.ads.googleads.v10.common.ProductTypeInfo.getDefaultInstance();
}
productTypeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductTypeInfo, com.google.ads.googleads.v10.common.ProductTypeInfo.Builder, com.google.ads.googleads.v10.common.ProductTypeInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductTypeInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 12;
onChanged();;
return productTypeBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductGroupingInfo, com.google.ads.googleads.v10.common.ProductGroupingInfo.Builder, com.google.ads.googleads.v10.common.ProductGroupingInfoOrBuilder> productGroupingBuilder_;
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
* @return Whether the productGrouping field is set.
*/
@java.lang.Override
public boolean hasProductGrouping() {
return dimensionCase_ == 17;
}
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
* @return The productGrouping.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductGroupingInfo getProductGrouping() {
if (productGroupingBuilder_ == null) {
if (dimensionCase_ == 17) {
return (com.google.ads.googleads.v10.common.ProductGroupingInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductGroupingInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 17) {
return productGroupingBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductGroupingInfo.getDefaultInstance();
}
}
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
*/
public Builder setProductGrouping(com.google.ads.googleads.v10.common.ProductGroupingInfo value) {
if (productGroupingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productGroupingBuilder_.setMessage(value);
}
dimensionCase_ = 17;
return this;
}
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
*/
public Builder setProductGrouping(
com.google.ads.googleads.v10.common.ProductGroupingInfo.Builder builderForValue) {
if (productGroupingBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productGroupingBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 17;
return this;
}
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
*/
public Builder mergeProductGrouping(com.google.ads.googleads.v10.common.ProductGroupingInfo value) {
if (productGroupingBuilder_ == null) {
if (dimensionCase_ == 17 &&
dimension_ != com.google.ads.googleads.v10.common.ProductGroupingInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductGroupingInfo.newBuilder((com.google.ads.googleads.v10.common.ProductGroupingInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 17) {
productGroupingBuilder_.mergeFrom(value);
} else {
productGroupingBuilder_.setMessage(value);
}
}
dimensionCase_ = 17;
return this;
}
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
*/
public Builder clearProductGrouping() {
if (productGroupingBuilder_ == null) {
if (dimensionCase_ == 17) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 17) {
dimensionCase_ = 0;
dimension_ = null;
}
productGroupingBuilder_.clear();
}
return this;
}
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
*/
public com.google.ads.googleads.v10.common.ProductGroupingInfo.Builder getProductGroupingBuilder() {
return getProductGroupingFieldBuilder().getBuilder();
}
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductGroupingInfoOrBuilder getProductGroupingOrBuilder() {
if ((dimensionCase_ == 17) && (productGroupingBuilder_ != null)) {
return productGroupingBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 17) {
return (com.google.ads.googleads.v10.common.ProductGroupingInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductGroupingInfo.getDefaultInstance();
}
}
/**
*
* Grouping of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductGroupingInfo product_grouping = 17;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductGroupingInfo, com.google.ads.googleads.v10.common.ProductGroupingInfo.Builder, com.google.ads.googleads.v10.common.ProductGroupingInfoOrBuilder>
getProductGroupingFieldBuilder() {
if (productGroupingBuilder_ == null) {
if (!(dimensionCase_ == 17)) {
dimension_ = com.google.ads.googleads.v10.common.ProductGroupingInfo.getDefaultInstance();
}
productGroupingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductGroupingInfo, com.google.ads.googleads.v10.common.ProductGroupingInfo.Builder, com.google.ads.googleads.v10.common.ProductGroupingInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductGroupingInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 17;
onChanged();;
return productGroupingBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductLabelsInfo, com.google.ads.googleads.v10.common.ProductLabelsInfo.Builder, com.google.ads.googleads.v10.common.ProductLabelsInfoOrBuilder> productLabelsBuilder_;
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
* @return Whether the productLabels field is set.
*/
@java.lang.Override
public boolean hasProductLabels() {
return dimensionCase_ == 18;
}
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
* @return The productLabels.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductLabelsInfo getProductLabels() {
if (productLabelsBuilder_ == null) {
if (dimensionCase_ == 18) {
return (com.google.ads.googleads.v10.common.ProductLabelsInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductLabelsInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 18) {
return productLabelsBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductLabelsInfo.getDefaultInstance();
}
}
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
*/
public Builder setProductLabels(com.google.ads.googleads.v10.common.ProductLabelsInfo value) {
if (productLabelsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productLabelsBuilder_.setMessage(value);
}
dimensionCase_ = 18;
return this;
}
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
*/
public Builder setProductLabels(
com.google.ads.googleads.v10.common.ProductLabelsInfo.Builder builderForValue) {
if (productLabelsBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productLabelsBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 18;
return this;
}
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
*/
public Builder mergeProductLabels(com.google.ads.googleads.v10.common.ProductLabelsInfo value) {
if (productLabelsBuilder_ == null) {
if (dimensionCase_ == 18 &&
dimension_ != com.google.ads.googleads.v10.common.ProductLabelsInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductLabelsInfo.newBuilder((com.google.ads.googleads.v10.common.ProductLabelsInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 18) {
productLabelsBuilder_.mergeFrom(value);
} else {
productLabelsBuilder_.setMessage(value);
}
}
dimensionCase_ = 18;
return this;
}
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
*/
public Builder clearProductLabels() {
if (productLabelsBuilder_ == null) {
if (dimensionCase_ == 18) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 18) {
dimensionCase_ = 0;
dimension_ = null;
}
productLabelsBuilder_.clear();
}
return this;
}
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
*/
public com.google.ads.googleads.v10.common.ProductLabelsInfo.Builder getProductLabelsBuilder() {
return getProductLabelsFieldBuilder().getBuilder();
}
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductLabelsInfoOrBuilder getProductLabelsOrBuilder() {
if ((dimensionCase_ == 18) && (productLabelsBuilder_ != null)) {
return productLabelsBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 18) {
return (com.google.ads.googleads.v10.common.ProductLabelsInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductLabelsInfo.getDefaultInstance();
}
}
/**
*
* Labels of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLabelsInfo product_labels = 18;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductLabelsInfo, com.google.ads.googleads.v10.common.ProductLabelsInfo.Builder, com.google.ads.googleads.v10.common.ProductLabelsInfoOrBuilder>
getProductLabelsFieldBuilder() {
if (productLabelsBuilder_ == null) {
if (!(dimensionCase_ == 18)) {
dimension_ = com.google.ads.googleads.v10.common.ProductLabelsInfo.getDefaultInstance();
}
productLabelsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductLabelsInfo, com.google.ads.googleads.v10.common.ProductLabelsInfo.Builder, com.google.ads.googleads.v10.common.ProductLabelsInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductLabelsInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 18;
onChanged();;
return productLabelsBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductLegacyConditionInfo, com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.Builder, com.google.ads.googleads.v10.common.ProductLegacyConditionInfoOrBuilder> productLegacyConditionBuilder_;
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
* @return Whether the productLegacyCondition field is set.
*/
@java.lang.Override
public boolean hasProductLegacyCondition() {
return dimensionCase_ == 19;
}
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
* @return The productLegacyCondition.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductLegacyConditionInfo getProductLegacyCondition() {
if (productLegacyConditionBuilder_ == null) {
if (dimensionCase_ == 19) {
return (com.google.ads.googleads.v10.common.ProductLegacyConditionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 19) {
return productLegacyConditionBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.getDefaultInstance();
}
}
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
*/
public Builder setProductLegacyCondition(com.google.ads.googleads.v10.common.ProductLegacyConditionInfo value) {
if (productLegacyConditionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productLegacyConditionBuilder_.setMessage(value);
}
dimensionCase_ = 19;
return this;
}
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
*/
public Builder setProductLegacyCondition(
com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.Builder builderForValue) {
if (productLegacyConditionBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productLegacyConditionBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 19;
return this;
}
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
*/
public Builder mergeProductLegacyCondition(com.google.ads.googleads.v10.common.ProductLegacyConditionInfo value) {
if (productLegacyConditionBuilder_ == null) {
if (dimensionCase_ == 19 &&
dimension_ != com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.newBuilder((com.google.ads.googleads.v10.common.ProductLegacyConditionInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 19) {
productLegacyConditionBuilder_.mergeFrom(value);
} else {
productLegacyConditionBuilder_.setMessage(value);
}
}
dimensionCase_ = 19;
return this;
}
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
*/
public Builder clearProductLegacyCondition() {
if (productLegacyConditionBuilder_ == null) {
if (dimensionCase_ == 19) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 19) {
dimensionCase_ = 0;
dimension_ = null;
}
productLegacyConditionBuilder_.clear();
}
return this;
}
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
*/
public com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.Builder getProductLegacyConditionBuilder() {
return getProductLegacyConditionFieldBuilder().getBuilder();
}
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductLegacyConditionInfoOrBuilder getProductLegacyConditionOrBuilder() {
if ((dimensionCase_ == 19) && (productLegacyConditionBuilder_ != null)) {
return productLegacyConditionBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 19) {
return (com.google.ads.googleads.v10.common.ProductLegacyConditionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.getDefaultInstance();
}
}
/**
*
* Legacy condition of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductLegacyConditionInfo product_legacy_condition = 19;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductLegacyConditionInfo, com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.Builder, com.google.ads.googleads.v10.common.ProductLegacyConditionInfoOrBuilder>
getProductLegacyConditionFieldBuilder() {
if (productLegacyConditionBuilder_ == null) {
if (!(dimensionCase_ == 19)) {
dimension_ = com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.getDefaultInstance();
}
productLegacyConditionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductLegacyConditionInfo, com.google.ads.googleads.v10.common.ProductLegacyConditionInfo.Builder, com.google.ads.googleads.v10.common.ProductLegacyConditionInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductLegacyConditionInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 19;
onChanged();;
return productLegacyConditionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductTypeFullInfo, com.google.ads.googleads.v10.common.ProductTypeFullInfo.Builder, com.google.ads.googleads.v10.common.ProductTypeFullInfoOrBuilder> productTypeFullBuilder_;
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
* @return Whether the productTypeFull field is set.
*/
@java.lang.Override
public boolean hasProductTypeFull() {
return dimensionCase_ == 20;
}
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
* @return The productTypeFull.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductTypeFullInfo getProductTypeFull() {
if (productTypeFullBuilder_ == null) {
if (dimensionCase_ == 20) {
return (com.google.ads.googleads.v10.common.ProductTypeFullInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductTypeFullInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 20) {
return productTypeFullBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.ProductTypeFullInfo.getDefaultInstance();
}
}
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
*/
public Builder setProductTypeFull(com.google.ads.googleads.v10.common.ProductTypeFullInfo value) {
if (productTypeFullBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
productTypeFullBuilder_.setMessage(value);
}
dimensionCase_ = 20;
return this;
}
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
*/
public Builder setProductTypeFull(
com.google.ads.googleads.v10.common.ProductTypeFullInfo.Builder builderForValue) {
if (productTypeFullBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
productTypeFullBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 20;
return this;
}
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
*/
public Builder mergeProductTypeFull(com.google.ads.googleads.v10.common.ProductTypeFullInfo value) {
if (productTypeFullBuilder_ == null) {
if (dimensionCase_ == 20 &&
dimension_ != com.google.ads.googleads.v10.common.ProductTypeFullInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.ProductTypeFullInfo.newBuilder((com.google.ads.googleads.v10.common.ProductTypeFullInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 20) {
productTypeFullBuilder_.mergeFrom(value);
} else {
productTypeFullBuilder_.setMessage(value);
}
}
dimensionCase_ = 20;
return this;
}
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
*/
public Builder clearProductTypeFull() {
if (productTypeFullBuilder_ == null) {
if (dimensionCase_ == 20) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 20) {
dimensionCase_ = 0;
dimension_ = null;
}
productTypeFullBuilder_.clear();
}
return this;
}
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
*/
public com.google.ads.googleads.v10.common.ProductTypeFullInfo.Builder getProductTypeFullBuilder() {
return getProductTypeFullFieldBuilder().getBuilder();
}
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.ProductTypeFullInfoOrBuilder getProductTypeFullOrBuilder() {
if ((dimensionCase_ == 20) && (productTypeFullBuilder_ != null)) {
return productTypeFullBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 20) {
return (com.google.ads.googleads.v10.common.ProductTypeFullInfo) dimension_;
}
return com.google.ads.googleads.v10.common.ProductTypeFullInfo.getDefaultInstance();
}
}
/**
*
* Full type of a product offer.
*
*
* .google.ads.googleads.v10.common.ProductTypeFullInfo product_type_full = 20;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductTypeFullInfo, com.google.ads.googleads.v10.common.ProductTypeFullInfo.Builder, com.google.ads.googleads.v10.common.ProductTypeFullInfoOrBuilder>
getProductTypeFullFieldBuilder() {
if (productTypeFullBuilder_ == null) {
if (!(dimensionCase_ == 20)) {
dimension_ = com.google.ads.googleads.v10.common.ProductTypeFullInfo.getDefaultInstance();
}
productTypeFullBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.ProductTypeFullInfo, com.google.ads.googleads.v10.common.ProductTypeFullInfo.Builder, com.google.ads.googleads.v10.common.ProductTypeFullInfoOrBuilder>(
(com.google.ads.googleads.v10.common.ProductTypeFullInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 20;
onChanged();;
return productTypeFullBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.UnknownListingDimensionInfo, com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.Builder, com.google.ads.googleads.v10.common.UnknownListingDimensionInfoOrBuilder> unknownListingDimensionBuilder_;
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
* @return Whether the unknownListingDimension field is set.
*/
@java.lang.Override
public boolean hasUnknownListingDimension() {
return dimensionCase_ == 14;
}
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
* @return The unknownListingDimension.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.UnknownListingDimensionInfo getUnknownListingDimension() {
if (unknownListingDimensionBuilder_ == null) {
if (dimensionCase_ == 14) {
return (com.google.ads.googleads.v10.common.UnknownListingDimensionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.getDefaultInstance();
} else {
if (dimensionCase_ == 14) {
return unknownListingDimensionBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.getDefaultInstance();
}
}
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
*/
public Builder setUnknownListingDimension(com.google.ads.googleads.v10.common.UnknownListingDimensionInfo value) {
if (unknownListingDimensionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dimension_ = value;
onChanged();
} else {
unknownListingDimensionBuilder_.setMessage(value);
}
dimensionCase_ = 14;
return this;
}
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
*/
public Builder setUnknownListingDimension(
com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.Builder builderForValue) {
if (unknownListingDimensionBuilder_ == null) {
dimension_ = builderForValue.build();
onChanged();
} else {
unknownListingDimensionBuilder_.setMessage(builderForValue.build());
}
dimensionCase_ = 14;
return this;
}
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
*/
public Builder mergeUnknownListingDimension(com.google.ads.googleads.v10.common.UnknownListingDimensionInfo value) {
if (unknownListingDimensionBuilder_ == null) {
if (dimensionCase_ == 14 &&
dimension_ != com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.getDefaultInstance()) {
dimension_ = com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.newBuilder((com.google.ads.googleads.v10.common.UnknownListingDimensionInfo) dimension_)
.mergeFrom(value).buildPartial();
} else {
dimension_ = value;
}
onChanged();
} else {
if (dimensionCase_ == 14) {
unknownListingDimensionBuilder_.mergeFrom(value);
} else {
unknownListingDimensionBuilder_.setMessage(value);
}
}
dimensionCase_ = 14;
return this;
}
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
*/
public Builder clearUnknownListingDimension() {
if (unknownListingDimensionBuilder_ == null) {
if (dimensionCase_ == 14) {
dimensionCase_ = 0;
dimension_ = null;
onChanged();
}
} else {
if (dimensionCase_ == 14) {
dimensionCase_ = 0;
dimension_ = null;
}
unknownListingDimensionBuilder_.clear();
}
return this;
}
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
*/
public com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.Builder getUnknownListingDimensionBuilder() {
return getUnknownListingDimensionFieldBuilder().getBuilder();
}
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.UnknownListingDimensionInfoOrBuilder getUnknownListingDimensionOrBuilder() {
if ((dimensionCase_ == 14) && (unknownListingDimensionBuilder_ != null)) {
return unknownListingDimensionBuilder_.getMessageOrBuilder();
} else {
if (dimensionCase_ == 14) {
return (com.google.ads.googleads.v10.common.UnknownListingDimensionInfo) dimension_;
}
return com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.getDefaultInstance();
}
}
/**
*
* Unknown dimension. Set when no other listing dimension is set.
*
*
* .google.ads.googleads.v10.common.UnknownListingDimensionInfo unknown_listing_dimension = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.UnknownListingDimensionInfo, com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.Builder, com.google.ads.googleads.v10.common.UnknownListingDimensionInfoOrBuilder>
getUnknownListingDimensionFieldBuilder() {
if (unknownListingDimensionBuilder_ == null) {
if (!(dimensionCase_ == 14)) {
dimension_ = com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.getDefaultInstance();
}
unknownListingDimensionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.UnknownListingDimensionInfo, com.google.ads.googleads.v10.common.UnknownListingDimensionInfo.Builder, com.google.ads.googleads.v10.common.UnknownListingDimensionInfoOrBuilder>(
(com.google.ads.googleads.v10.common.UnknownListingDimensionInfo) dimension_,
getParentForChildren(),
isClean());
dimension_ = null;
}
dimensionCase_ = 14;
onChanged();;
return unknownListingDimensionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v10.common.ListingDimensionInfo)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v10.common.ListingDimensionInfo)
private static final com.google.ads.googleads.v10.common.ListingDimensionInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v10.common.ListingDimensionInfo();
}
public static com.google.ads.googleads.v10.common.ListingDimensionInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ListingDimensionInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v10.common.ListingDimensionInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy