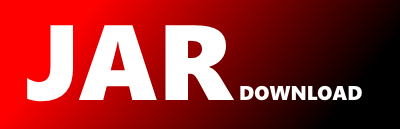
com.google.ads.googleads.v10.resources.AccountBudget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v10 Show documentation
Show all versions of google-ads-stubs-v10 Show documentation
Stubs for GAAPI version google-ads-stubs-v10
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v10/resources/account_budget.proto
package com.google.ads.googleads.v10.resources;
/**
*
* An account-level budget. It contains information about the budget itself,
* as well as the most recently approved changes to the budget and proposed
* changes that are pending approval. The proposed changes that are pending
* approval, if any, are found in 'pending_proposal'. Effective details about
* the budget are found in fields prefixed 'approved_', 'adjusted_' and those
* without a prefix. Since some effective details may differ from what the user
* had originally requested (for example, spending limit), these differences are
* juxtaposed through 'proposed_', 'approved_', and possibly 'adjusted_' fields.
* This resource is mutated using AccountBudgetProposal and cannot be mutated
* directly. A budget may have at most one pending proposal at any given time.
* It is read through pending_proposal.
* Once approved, a budget may be subject to adjustments, such as credit
* adjustments. Adjustments create differences between the 'approved' and
* 'adjusted' fields, which would otherwise be identical.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.AccountBudget}
*/
public final class AccountBudget extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v10.resources.AccountBudget)
AccountBudgetOrBuilder {
private static final long serialVersionUID = 0L;
// Use AccountBudget.newBuilder() to construct.
private AccountBudget(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AccountBudget() {
resourceName_ = "";
billingSetup_ = "";
status_ = 0;
name_ = "";
proposedStartDateTime_ = "";
approvedStartDateTime_ = "";
purchaseOrderNumber_ = "";
notes_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AccountBudget();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.AccountBudgetProto.internal_static_google_ads_googleads_v10_resources_AccountBudget_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.AccountBudgetProto.internal_static_google_ads_googleads_v10_resources_AccountBudget_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.AccountBudget.class, com.google.ads.googleads.v10.resources.AccountBudget.Builder.class);
}
public interface PendingAccountBudgetProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal)
com.google.protobuf.MessageOrBuilder {
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the accountBudgetProposal field is set.
*/
boolean hasAccountBudgetProposal();
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The accountBudgetProposal.
*/
java.lang.String getAccountBudgetProposal();
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for accountBudgetProposal.
*/
com.google.protobuf.ByteString
getAccountBudgetProposalBytes();
/**
*
* Output only. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for proposalType.
*/
int getProposalTypeValue();
/**
*
* Output only. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposalType.
*/
com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType getProposalType();
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The name.
*/
java.lang.String getName();
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the startDateTime field is set.
*/
boolean hasStartDateTime();
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The startDateTime.
*/
java.lang.String getStartDateTime();
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for startDateTime.
*/
com.google.protobuf.ByteString
getStartDateTimeBytes();
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the purchaseOrderNumber field is set.
*/
boolean hasPurchaseOrderNumber();
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The purchaseOrderNumber.
*/
java.lang.String getPurchaseOrderNumber();
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for purchaseOrderNumber.
*/
com.google.protobuf.ByteString
getPurchaseOrderNumberBytes();
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the notes field is set.
*/
boolean hasNotes();
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The notes.
*/
java.lang.String getNotes();
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for notes.
*/
com.google.protobuf.ByteString
getNotesBytes();
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the creationDateTime field is set.
*/
boolean hasCreationDateTime();
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The creationDateTime.
*/
java.lang.String getCreationDateTime();
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for creationDateTime.
*/
com.google.protobuf.ByteString
getCreationDateTimeBytes();
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the endDateTime field is set.
*/
boolean hasEndDateTime();
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The endDateTime.
*/
java.lang.String getEndDateTime();
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for endDateTime.
*/
com.google.protobuf.ByteString
getEndDateTimeBytes();
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the endTimeType field is set.
*/
boolean hasEndTimeType();
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for endTimeType.
*/
int getEndTimeTypeValue();
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The endTimeType.
*/
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getEndTimeType();
/**
*
* Output only. The spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 spending_limit_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the spendingLimitMicros field is set.
*/
boolean hasSpendingLimitMicros();
/**
*
* Output only. The spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 spending_limit_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The spendingLimitMicros.
*/
long getSpendingLimitMicros();
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the spendingLimitType field is set.
*/
boolean hasSpendingLimitType();
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for spendingLimitType.
*/
int getSpendingLimitTypeValue();
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The spendingLimitType.
*/
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getSpendingLimitType();
public com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.EndTimeCase getEndTimeCase();
public com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.SpendingLimitCase getSpendingLimitCase();
}
/**
*
* A pending proposal associated with the enclosing account-level budget,
* if applicable.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal}
*/
public static final class PendingAccountBudgetProposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal)
PendingAccountBudgetProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use PendingAccountBudgetProposal.newBuilder() to construct.
private PendingAccountBudgetProposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PendingAccountBudgetProposal() {
accountBudgetProposal_ = "";
proposalType_ = 0;
name_ = "";
startDateTime_ = "";
purchaseOrderNumber_ = "";
notes_ = "";
creationDateTime_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PendingAccountBudgetProposal();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.AccountBudgetProto.internal_static_google_ads_googleads_v10_resources_AccountBudget_PendingAccountBudgetProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.AccountBudgetProto.internal_static_google_ads_googleads_v10_resources_AccountBudget_PendingAccountBudgetProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.class, com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.Builder.class);
}
private int bitField0_;
private int endTimeCase_ = 0;
private java.lang.Object endTime_;
public enum EndTimeCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
END_DATE_TIME(15),
END_TIME_TYPE(6),
ENDTIME_NOT_SET(0);
private final int value;
private EndTimeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static EndTimeCase valueOf(int value) {
return forNumber(value);
}
public static EndTimeCase forNumber(int value) {
switch (value) {
case 15: return END_DATE_TIME;
case 6: return END_TIME_TYPE;
case 0: return ENDTIME_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public EndTimeCase
getEndTimeCase() {
return EndTimeCase.forNumber(
endTimeCase_);
}
private int spendingLimitCase_ = 0;
private java.lang.Object spendingLimit_;
public enum SpendingLimitCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
SPENDING_LIMIT_MICROS(16),
SPENDING_LIMIT_TYPE(8),
SPENDINGLIMIT_NOT_SET(0);
private final int value;
private SpendingLimitCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SpendingLimitCase valueOf(int value) {
return forNumber(value);
}
public static SpendingLimitCase forNumber(int value) {
switch (value) {
case 16: return SPENDING_LIMIT_MICROS;
case 8: return SPENDING_LIMIT_TYPE;
case 0: return SPENDINGLIMIT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public SpendingLimitCase
getSpendingLimitCase() {
return SpendingLimitCase.forNumber(
spendingLimitCase_);
}
public static final int ACCOUNT_BUDGET_PROPOSAL_FIELD_NUMBER = 12;
private volatile java.lang.Object accountBudgetProposal_;
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the accountBudgetProposal field is set.
*/
@java.lang.Override
public boolean hasAccountBudgetProposal() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The accountBudgetProposal.
*/
@java.lang.Override
public java.lang.String getAccountBudgetProposal() {
java.lang.Object ref = accountBudgetProposal_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountBudgetProposal_ = s;
return s;
}
}
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for accountBudgetProposal.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAccountBudgetProposalBytes() {
java.lang.Object ref = accountBudgetProposal_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountBudgetProposal_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROPOSAL_TYPE_FIELD_NUMBER = 2;
private int proposalType_;
/**
*
* Output only. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for proposalType.
*/
@java.lang.Override public int getProposalTypeValue() {
return proposalType_;
}
/**
*
* Output only. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposalType.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType getProposalType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType result = com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.valueOf(proposalType_);
return result == null ? com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.UNRECOGNIZED : result;
}
public static final int NAME_FIELD_NUMBER = 13;
private volatile java.lang.Object name_;
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int START_DATE_TIME_FIELD_NUMBER = 14;
private volatile java.lang.Object startDateTime_;
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the startDateTime field is set.
*/
@java.lang.Override
public boolean hasStartDateTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The startDateTime.
*/
@java.lang.Override
public java.lang.String getStartDateTime() {
java.lang.Object ref = startDateTime_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
startDateTime_ = s;
return s;
}
}
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for startDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStartDateTimeBytes() {
java.lang.Object ref = startDateTime_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
startDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PURCHASE_ORDER_NUMBER_FIELD_NUMBER = 17;
private volatile java.lang.Object purchaseOrderNumber_;
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the purchaseOrderNumber field is set.
*/
@java.lang.Override
public boolean hasPurchaseOrderNumber() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The purchaseOrderNumber.
*/
@java.lang.Override
public java.lang.String getPurchaseOrderNumber() {
java.lang.Object ref = purchaseOrderNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
purchaseOrderNumber_ = s;
return s;
}
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for purchaseOrderNumber.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPurchaseOrderNumberBytes() {
java.lang.Object ref = purchaseOrderNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
purchaseOrderNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NOTES_FIELD_NUMBER = 18;
private volatile java.lang.Object notes_;
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the notes field is set.
*/
@java.lang.Override
public boolean hasNotes() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The notes.
*/
@java.lang.Override
public java.lang.String getNotes() {
java.lang.Object ref = notes_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
notes_ = s;
return s;
}
}
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for notes.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNotesBytes() {
java.lang.Object ref = notes_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
notes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CREATION_DATE_TIME_FIELD_NUMBER = 19;
private volatile java.lang.Object creationDateTime_;
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the creationDateTime field is set.
*/
@java.lang.Override
public boolean hasCreationDateTime() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The creationDateTime.
*/
@java.lang.Override
public java.lang.String getCreationDateTime() {
java.lang.Object ref = creationDateTime_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationDateTime_ = s;
return s;
}
}
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for creationDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCreationDateTimeBytes() {
java.lang.Object ref = creationDateTime_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
creationDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int END_DATE_TIME_FIELD_NUMBER = 15;
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the endDateTime field is set.
*/
public boolean hasEndDateTime() {
return endTimeCase_ == 15;
}
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The endDateTime.
*/
public java.lang.String getEndDateTime() {
java.lang.Object ref = "";
if (endTimeCase_ == 15) {
ref = endTime_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (endTimeCase_ == 15) {
endTime_ = s;
}
return s;
}
}
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for endDateTime.
*/
public com.google.protobuf.ByteString
getEndDateTimeBytes() {
java.lang.Object ref = "";
if (endTimeCase_ == 15) {
ref = endTime_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (endTimeCase_ == 15) {
endTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int END_TIME_TYPE_FIELD_NUMBER = 6;
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the endTimeType field is set.
*/
public boolean hasEndTimeType() {
return endTimeCase_ == 6;
}
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for endTimeType.
*/
public int getEndTimeTypeValue() {
if (endTimeCase_ == 6) {
return (java.lang.Integer) endTime_;
}
return 0;
}
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The endTimeType.
*/
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getEndTimeType() {
if (endTimeCase_ == 6) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) endTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
public static final int SPENDING_LIMIT_MICROS_FIELD_NUMBER = 16;
/**
*
* Output only. The spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 spending_limit_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the spendingLimitMicros field is set.
*/
@java.lang.Override
public boolean hasSpendingLimitMicros() {
return spendingLimitCase_ == 16;
}
/**
*
* Output only. The spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 spending_limit_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The spendingLimitMicros.
*/
@java.lang.Override
public long getSpendingLimitMicros() {
if (spendingLimitCase_ == 16) {
return (java.lang.Long) spendingLimit_;
}
return 0L;
}
public static final int SPENDING_LIMIT_TYPE_FIELD_NUMBER = 8;
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the spendingLimitType field is set.
*/
public boolean hasSpendingLimitType() {
return spendingLimitCase_ == 8;
}
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for spendingLimitType.
*/
public int getSpendingLimitTypeValue() {
if (spendingLimitCase_ == 8) {
return (java.lang.Integer) spendingLimit_;
}
return 0;
}
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The spendingLimitType.
*/
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getSpendingLimitType() {
if (spendingLimitCase_ == 8) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) spendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (proposalType_ != com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.UNSPECIFIED.getNumber()) {
output.writeEnum(2, proposalType_);
}
if (endTimeCase_ == 6) {
output.writeEnum(6, ((java.lang.Integer) endTime_));
}
if (spendingLimitCase_ == 8) {
output.writeEnum(8, ((java.lang.Integer) spendingLimit_));
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, accountBudgetProposal_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, startDateTime_);
}
if (endTimeCase_ == 15) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, endTime_);
}
if (spendingLimitCase_ == 16) {
output.writeInt64(
16, (long)((java.lang.Long) spendingLimit_));
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, purchaseOrderNumber_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 18, notes_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 19, creationDateTime_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (proposalType_ != com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, proposalType_);
}
if (endTimeCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, ((java.lang.Integer) endTime_));
}
if (spendingLimitCase_ == 8) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(8, ((java.lang.Integer) spendingLimit_));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, accountBudgetProposal_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, startDateTime_);
}
if (endTimeCase_ == 15) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, endTime_);
}
if (spendingLimitCase_ == 16) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
16, (long)((java.lang.Long) spendingLimit_));
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, purchaseOrderNumber_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(18, notes_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(19, creationDateTime_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal)) {
return super.equals(obj);
}
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal other = (com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal) obj;
if (hasAccountBudgetProposal() != other.hasAccountBudgetProposal()) return false;
if (hasAccountBudgetProposal()) {
if (!getAccountBudgetProposal()
.equals(other.getAccountBudgetProposal())) return false;
}
if (proposalType_ != other.proposalType_) return false;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasStartDateTime() != other.hasStartDateTime()) return false;
if (hasStartDateTime()) {
if (!getStartDateTime()
.equals(other.getStartDateTime())) return false;
}
if (hasPurchaseOrderNumber() != other.hasPurchaseOrderNumber()) return false;
if (hasPurchaseOrderNumber()) {
if (!getPurchaseOrderNumber()
.equals(other.getPurchaseOrderNumber())) return false;
}
if (hasNotes() != other.hasNotes()) return false;
if (hasNotes()) {
if (!getNotes()
.equals(other.getNotes())) return false;
}
if (hasCreationDateTime() != other.hasCreationDateTime()) return false;
if (hasCreationDateTime()) {
if (!getCreationDateTime()
.equals(other.getCreationDateTime())) return false;
}
if (!getEndTimeCase().equals(other.getEndTimeCase())) return false;
switch (endTimeCase_) {
case 15:
if (!getEndDateTime()
.equals(other.getEndDateTime())) return false;
break;
case 6:
if (getEndTimeTypeValue()
!= other.getEndTimeTypeValue()) return false;
break;
case 0:
default:
}
if (!getSpendingLimitCase().equals(other.getSpendingLimitCase())) return false;
switch (spendingLimitCase_) {
case 16:
if (getSpendingLimitMicros()
!= other.getSpendingLimitMicros()) return false;
break;
case 8:
if (getSpendingLimitTypeValue()
!= other.getSpendingLimitTypeValue()) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccountBudgetProposal()) {
hash = (37 * hash) + ACCOUNT_BUDGET_PROPOSAL_FIELD_NUMBER;
hash = (53 * hash) + getAccountBudgetProposal().hashCode();
}
hash = (37 * hash) + PROPOSAL_TYPE_FIELD_NUMBER;
hash = (53 * hash) + proposalType_;
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasStartDateTime()) {
hash = (37 * hash) + START_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getStartDateTime().hashCode();
}
if (hasPurchaseOrderNumber()) {
hash = (37 * hash) + PURCHASE_ORDER_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getPurchaseOrderNumber().hashCode();
}
if (hasNotes()) {
hash = (37 * hash) + NOTES_FIELD_NUMBER;
hash = (53 * hash) + getNotes().hashCode();
}
if (hasCreationDateTime()) {
hash = (37 * hash) + CREATION_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getCreationDateTime().hashCode();
}
switch (endTimeCase_) {
case 15:
hash = (37 * hash) + END_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getEndDateTime().hashCode();
break;
case 6:
hash = (37 * hash) + END_TIME_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getEndTimeTypeValue();
break;
case 0:
default:
}
switch (spendingLimitCase_) {
case 16:
hash = (37 * hash) + SPENDING_LIMIT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSpendingLimitMicros());
break;
case 8:
hash = (37 * hash) + SPENDING_LIMIT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getSpendingLimitTypeValue();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A pending proposal associated with the enclosing account-level budget,
* if applicable.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal)
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.AccountBudgetProto.internal_static_google_ads_googleads_v10_resources_AccountBudget_PendingAccountBudgetProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.AccountBudgetProto.internal_static_google_ads_googleads_v10_resources_AccountBudget_PendingAccountBudgetProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.class, com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.Builder.class);
}
// Construct using com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
accountBudgetProposal_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
proposalType_ = 0;
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
startDateTime_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
purchaseOrderNumber_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
notes_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
creationDateTime_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
endTimeCase_ = 0;
endTime_ = null;
spendingLimitCase_ = 0;
spendingLimit_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v10.resources.AccountBudgetProto.internal_static_google_ads_googleads_v10_resources_AccountBudget_PendingAccountBudgetProposal_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal getDefaultInstanceForType() {
return com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal build() {
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal buildPartial() {
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal result = new com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.accountBudgetProposal_ = accountBudgetProposal_;
result.proposalType_ = proposalType_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.startDateTime_ = startDateTime_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.purchaseOrderNumber_ = purchaseOrderNumber_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.notes_ = notes_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.creationDateTime_ = creationDateTime_;
if (endTimeCase_ == 15) {
result.endTime_ = endTime_;
}
if (endTimeCase_ == 6) {
result.endTime_ = endTime_;
}
if (spendingLimitCase_ == 16) {
result.spendingLimit_ = spendingLimit_;
}
if (spendingLimitCase_ == 8) {
result.spendingLimit_ = spendingLimit_;
}
result.bitField0_ = to_bitField0_;
result.endTimeCase_ = endTimeCase_;
result.spendingLimitCase_ = spendingLimitCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal) {
return mergeFrom((com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal other) {
if (other == com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.getDefaultInstance()) return this;
if (other.hasAccountBudgetProposal()) {
bitField0_ |= 0x00000001;
accountBudgetProposal_ = other.accountBudgetProposal_;
onChanged();
}
if (other.proposalType_ != 0) {
setProposalTypeValue(other.getProposalTypeValue());
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
if (other.hasStartDateTime()) {
bitField0_ |= 0x00000004;
startDateTime_ = other.startDateTime_;
onChanged();
}
if (other.hasPurchaseOrderNumber()) {
bitField0_ |= 0x00000008;
purchaseOrderNumber_ = other.purchaseOrderNumber_;
onChanged();
}
if (other.hasNotes()) {
bitField0_ |= 0x00000010;
notes_ = other.notes_;
onChanged();
}
if (other.hasCreationDateTime()) {
bitField0_ |= 0x00000020;
creationDateTime_ = other.creationDateTime_;
onChanged();
}
switch (other.getEndTimeCase()) {
case END_DATE_TIME: {
endTimeCase_ = 15;
endTime_ = other.endTime_;
onChanged();
break;
}
case END_TIME_TYPE: {
setEndTimeTypeValue(other.getEndTimeTypeValue());
break;
}
case ENDTIME_NOT_SET: {
break;
}
}
switch (other.getSpendingLimitCase()) {
case SPENDING_LIMIT_MICROS: {
setSpendingLimitMicros(other.getSpendingLimitMicros());
break;
}
case SPENDING_LIMIT_TYPE: {
setSpendingLimitTypeValue(other.getSpendingLimitTypeValue());
break;
}
case SPENDINGLIMIT_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 16: {
proposalType_ = input.readEnum();
break;
} // case 16
case 48: {
int rawValue = input.readEnum();
endTimeCase_ = 6;
endTime_ = rawValue;
break;
} // case 48
case 64: {
int rawValue = input.readEnum();
spendingLimitCase_ = 8;
spendingLimit_ = rawValue;
break;
} // case 64
case 98: {
accountBudgetProposal_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 98
case 106: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 106
case 114: {
startDateTime_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 114
case 122: {
java.lang.String s = input.readStringRequireUtf8();
endTimeCase_ = 15;
endTime_ = s;
break;
} // case 122
case 128: {
spendingLimit_ = input.readInt64();
spendingLimitCase_ = 16;
break;
} // case 128
case 138: {
purchaseOrderNumber_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 138
case 146: {
notes_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 146
case 154: {
creationDateTime_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 154
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int endTimeCase_ = 0;
private java.lang.Object endTime_;
public EndTimeCase
getEndTimeCase() {
return EndTimeCase.forNumber(
endTimeCase_);
}
public Builder clearEndTime() {
endTimeCase_ = 0;
endTime_ = null;
onChanged();
return this;
}
private int spendingLimitCase_ = 0;
private java.lang.Object spendingLimit_;
public SpendingLimitCase
getSpendingLimitCase() {
return SpendingLimitCase.forNumber(
spendingLimitCase_);
}
public Builder clearSpendingLimit() {
spendingLimitCase_ = 0;
spendingLimit_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object accountBudgetProposal_ = "";
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the accountBudgetProposal field is set.
*/
public boolean hasAccountBudgetProposal() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The accountBudgetProposal.
*/
public java.lang.String getAccountBudgetProposal() {
java.lang.Object ref = accountBudgetProposal_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountBudgetProposal_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for accountBudgetProposal.
*/
public com.google.protobuf.ByteString
getAccountBudgetProposalBytes() {
java.lang.Object ref = accountBudgetProposal_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountBudgetProposal_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The accountBudgetProposal to set.
* @return This builder for chaining.
*/
public Builder setAccountBudgetProposal(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
accountBudgetProposal_ = value;
onChanged();
return this;
}
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearAccountBudgetProposal() {
bitField0_ = (bitField0_ & ~0x00000001);
accountBudgetProposal_ = getDefaultInstance().getAccountBudgetProposal();
onChanged();
return this;
}
/**
*
* Output only. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* optional string account_budget_proposal = 12 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for accountBudgetProposal to set.
* @return This builder for chaining.
*/
public Builder setAccountBudgetProposalBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000001;
accountBudgetProposal_ = value;
onChanged();
return this;
}
private int proposalType_ = 0;
/**
*
* Output only. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for proposalType.
*/
@java.lang.Override public int getProposalTypeValue() {
return proposalType_;
}
/**
*
* Output only. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for proposalType to set.
* @return This builder for chaining.
*/
public Builder setProposalTypeValue(int value) {
proposalType_ = value;
onChanged();
return this;
}
/**
*
* Output only. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposalType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType getProposalType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType result = com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.valueOf(proposalType_);
return result == null ? com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.UNRECOGNIZED : result;
}
/**
*
* Output only. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The proposalType to set.
* @return This builder for chaining.
*/
public Builder setProposalType(com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType value) {
if (value == null) {
throw new NullPointerException();
}
proposalType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearProposalType() {
proposalType_ = 0;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Output only. The name to assign to the account-level budget.
*
*
* optional string name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
private java.lang.Object startDateTime_ = "";
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the startDateTime field is set.
*/
public boolean hasStartDateTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The startDateTime.
*/
public java.lang.String getStartDateTime() {
java.lang.Object ref = startDateTime_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
startDateTime_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for startDateTime.
*/
public com.google.protobuf.ByteString
getStartDateTimeBytes() {
java.lang.Object ref = startDateTime_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
startDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The startDateTime to set.
* @return This builder for chaining.
*/
public Builder setStartDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
startDateTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearStartDateTime() {
bitField0_ = (bitField0_ & ~0x00000004);
startDateTime_ = getDefaultInstance().getStartDateTime();
onChanged();
return this;
}
/**
*
* Output only. The start time in yyyy-MM-dd HH:mm:ss format.
*
*
* optional string start_date_time = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for startDateTime to set.
* @return This builder for chaining.
*/
public Builder setStartDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000004;
startDateTime_ = value;
onChanged();
return this;
}
private java.lang.Object purchaseOrderNumber_ = "";
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the purchaseOrderNumber field is set.
*/
public boolean hasPurchaseOrderNumber() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The purchaseOrderNumber.
*/
public java.lang.String getPurchaseOrderNumber() {
java.lang.Object ref = purchaseOrderNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
purchaseOrderNumber_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for purchaseOrderNumber.
*/
public com.google.protobuf.ByteString
getPurchaseOrderNumberBytes() {
java.lang.Object ref = purchaseOrderNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
purchaseOrderNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The purchaseOrderNumber to set.
* @return This builder for chaining.
*/
public Builder setPurchaseOrderNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
purchaseOrderNumber_ = value;
onChanged();
return this;
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearPurchaseOrderNumber() {
bitField0_ = (bitField0_ & ~0x00000008);
purchaseOrderNumber_ = getDefaultInstance().getPurchaseOrderNumber();
onChanged();
return this;
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for purchaseOrderNumber to set.
* @return This builder for chaining.
*/
public Builder setPurchaseOrderNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000008;
purchaseOrderNumber_ = value;
onChanged();
return this;
}
private java.lang.Object notes_ = "";
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the notes field is set.
*/
public boolean hasNotes() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The notes.
*/
public java.lang.String getNotes() {
java.lang.Object ref = notes_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
notes_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for notes.
*/
public com.google.protobuf.ByteString
getNotesBytes() {
java.lang.Object ref = notes_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
notes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The notes to set.
* @return This builder for chaining.
*/
public Builder setNotes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
notes_ = value;
onChanged();
return this;
}
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearNotes() {
bitField0_ = (bitField0_ & ~0x00000010);
notes_ = getDefaultInstance().getNotes();
onChanged();
return this;
}
/**
*
* Output only. Notes associated with this budget.
*
*
* optional string notes = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for notes to set.
* @return This builder for chaining.
*/
public Builder setNotesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000010;
notes_ = value;
onChanged();
return this;
}
private java.lang.Object creationDateTime_ = "";
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the creationDateTime field is set.
*/
public boolean hasCreationDateTime() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The creationDateTime.
*/
public java.lang.String getCreationDateTime() {
java.lang.Object ref = creationDateTime_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationDateTime_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for creationDateTime.
*/
public com.google.protobuf.ByteString
getCreationDateTimeBytes() {
java.lang.Object ref = creationDateTime_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
creationDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The creationDateTime to set.
* @return This builder for chaining.
*/
public Builder setCreationDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
creationDateTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearCreationDateTime() {
bitField0_ = (bitField0_ & ~0x00000020);
creationDateTime_ = getDefaultInstance().getCreationDateTime();
onChanged();
return this;
}
/**
*
* Output only. The time when this account-level budget proposal was created.
* Formatted as yyyy-MM-dd HH:mm:ss.
*
*
* optional string creation_date_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for creationDateTime to set.
* @return This builder for chaining.
*/
public Builder setCreationDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000020;
creationDateTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the endDateTime field is set.
*/
@java.lang.Override
public boolean hasEndDateTime() {
return endTimeCase_ == 15;
}
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The endDateTime.
*/
@java.lang.Override
public java.lang.String getEndDateTime() {
java.lang.Object ref = "";
if (endTimeCase_ == 15) {
ref = endTime_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (endTimeCase_ == 15) {
endTime_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for endDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getEndDateTimeBytes() {
java.lang.Object ref = "";
if (endTimeCase_ == 15) {
ref = endTime_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (endTimeCase_ == 15) {
endTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The endDateTime to set.
* @return This builder for chaining.
*/
public Builder setEndDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
endTimeCase_ = 15;
endTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearEndDateTime() {
if (endTimeCase_ == 15) {
endTimeCase_ = 0;
endTime_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string end_date_time = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for endDateTime to set.
* @return This builder for chaining.
*/
public Builder setEndDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
endTimeCase_ = 15;
endTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the endTimeType field is set.
*/
@java.lang.Override
public boolean hasEndTimeType() {
return endTimeCase_ == 6;
}
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for endTimeType.
*/
@java.lang.Override
public int getEndTimeTypeValue() {
if (endTimeCase_ == 6) {
return ((java.lang.Integer) endTime_).intValue();
}
return 0;
}
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for endTimeType to set.
* @return This builder for chaining.
*/
public Builder setEndTimeTypeValue(int value) {
endTimeCase_ = 6;
endTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The endTimeType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getEndTimeType() {
if (endTimeCase_ == 6) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) endTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The endTimeType to set.
* @return This builder for chaining.
*/
public Builder setEndTimeType(com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType value) {
if (value == null) {
throw new NullPointerException();
}
endTimeCase_ = 6;
endTime_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType end_time_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearEndTimeType() {
if (endTimeCase_ == 6) {
endTimeCase_ = 0;
endTime_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 spending_limit_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the spendingLimitMicros field is set.
*/
public boolean hasSpendingLimitMicros() {
return spendingLimitCase_ == 16;
}
/**
*
* Output only. The spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 spending_limit_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The spendingLimitMicros.
*/
public long getSpendingLimitMicros() {
if (spendingLimitCase_ == 16) {
return (java.lang.Long) spendingLimit_;
}
return 0L;
}
/**
*
* Output only. The spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 spending_limit_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The spendingLimitMicros to set.
* @return This builder for chaining.
*/
public Builder setSpendingLimitMicros(long value) {
spendingLimitCase_ = 16;
spendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Output only. The spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 spending_limit_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearSpendingLimitMicros() {
if (spendingLimitCase_ == 16) {
spendingLimitCase_ = 0;
spendingLimit_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the spendingLimitType field is set.
*/
@java.lang.Override
public boolean hasSpendingLimitType() {
return spendingLimitCase_ == 8;
}
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for spendingLimitType.
*/
@java.lang.Override
public int getSpendingLimitTypeValue() {
if (spendingLimitCase_ == 8) {
return ((java.lang.Integer) spendingLimit_).intValue();
}
return 0;
}
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for spendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setSpendingLimitTypeValue(int value) {
spendingLimitCase_ = 8;
spendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The spendingLimitType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getSpendingLimitType() {
if (spendingLimitCase_ == 8) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) spendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The spendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setSpendingLimitType(com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType value) {
if (value == null) {
throw new NullPointerException();
}
spendingLimitCase_ = 8;
spendingLimit_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The spending limit as a well-defined type, for example, INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType spending_limit_type = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearSpendingLimitType() {
if (spendingLimitCase_ == 8) {
spendingLimitCase_ = 0;
spendingLimit_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal)
private static final com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal();
}
public static com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PendingAccountBudgetProposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
private int proposedEndTimeCase_ = 0;
private java.lang.Object proposedEndTime_;
public enum ProposedEndTimeCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
PROPOSED_END_DATE_TIME(28),
PROPOSED_END_TIME_TYPE(9),
PROPOSEDENDTIME_NOT_SET(0);
private final int value;
private ProposedEndTimeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProposedEndTimeCase valueOf(int value) {
return forNumber(value);
}
public static ProposedEndTimeCase forNumber(int value) {
switch (value) {
case 28: return PROPOSED_END_DATE_TIME;
case 9: return PROPOSED_END_TIME_TYPE;
case 0: return PROPOSEDENDTIME_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ProposedEndTimeCase
getProposedEndTimeCase() {
return ProposedEndTimeCase.forNumber(
proposedEndTimeCase_);
}
private int approvedEndTimeCase_ = 0;
private java.lang.Object approvedEndTime_;
public enum ApprovedEndTimeCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
APPROVED_END_DATE_TIME(29),
APPROVED_END_TIME_TYPE(11),
APPROVEDENDTIME_NOT_SET(0);
private final int value;
private ApprovedEndTimeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ApprovedEndTimeCase valueOf(int value) {
return forNumber(value);
}
public static ApprovedEndTimeCase forNumber(int value) {
switch (value) {
case 29: return APPROVED_END_DATE_TIME;
case 11: return APPROVED_END_TIME_TYPE;
case 0: return APPROVEDENDTIME_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ApprovedEndTimeCase
getApprovedEndTimeCase() {
return ApprovedEndTimeCase.forNumber(
approvedEndTimeCase_);
}
private int proposedSpendingLimitCase_ = 0;
private java.lang.Object proposedSpendingLimit_;
public enum ProposedSpendingLimitCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
PROPOSED_SPENDING_LIMIT_MICROS(30),
PROPOSED_SPENDING_LIMIT_TYPE(13),
PROPOSEDSPENDINGLIMIT_NOT_SET(0);
private final int value;
private ProposedSpendingLimitCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProposedSpendingLimitCase valueOf(int value) {
return forNumber(value);
}
public static ProposedSpendingLimitCase forNumber(int value) {
switch (value) {
case 30: return PROPOSED_SPENDING_LIMIT_MICROS;
case 13: return PROPOSED_SPENDING_LIMIT_TYPE;
case 0: return PROPOSEDSPENDINGLIMIT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ProposedSpendingLimitCase
getProposedSpendingLimitCase() {
return ProposedSpendingLimitCase.forNumber(
proposedSpendingLimitCase_);
}
private int approvedSpendingLimitCase_ = 0;
private java.lang.Object approvedSpendingLimit_;
public enum ApprovedSpendingLimitCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
APPROVED_SPENDING_LIMIT_MICROS(31),
APPROVED_SPENDING_LIMIT_TYPE(15),
APPROVEDSPENDINGLIMIT_NOT_SET(0);
private final int value;
private ApprovedSpendingLimitCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ApprovedSpendingLimitCase valueOf(int value) {
return forNumber(value);
}
public static ApprovedSpendingLimitCase forNumber(int value) {
switch (value) {
case 31: return APPROVED_SPENDING_LIMIT_MICROS;
case 15: return APPROVED_SPENDING_LIMIT_TYPE;
case 0: return APPROVEDSPENDINGLIMIT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ApprovedSpendingLimitCase
getApprovedSpendingLimitCase() {
return ApprovedSpendingLimitCase.forNumber(
approvedSpendingLimitCase_);
}
private int adjustedSpendingLimitCase_ = 0;
private java.lang.Object adjustedSpendingLimit_;
public enum AdjustedSpendingLimitCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
ADJUSTED_SPENDING_LIMIT_MICROS(32),
ADJUSTED_SPENDING_LIMIT_TYPE(17),
ADJUSTEDSPENDINGLIMIT_NOT_SET(0);
private final int value;
private AdjustedSpendingLimitCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static AdjustedSpendingLimitCase valueOf(int value) {
return forNumber(value);
}
public static AdjustedSpendingLimitCase forNumber(int value) {
switch (value) {
case 32: return ADJUSTED_SPENDING_LIMIT_MICROS;
case 17: return ADJUSTED_SPENDING_LIMIT_TYPE;
case 0: return ADJUSTEDSPENDINGLIMIT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public AdjustedSpendingLimitCase
getAdjustedSpendingLimitCase() {
return AdjustedSpendingLimitCase.forNumber(
adjustedSpendingLimitCase_);
}
public static final int RESOURCE_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object resourceName_;
/**
*
* Output only. The resource name of the account-level budget.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
@java.lang.Override
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
}
}
/**
*
* Output only. The resource name of the account-level budget.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 23;
private long id_;
/**
*
* Output only. The ID of the account-level budget.
*
*
* optional int64 id = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The ID of the account-level budget.
*
*
* optional int64 id = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int BILLING_SETUP_FIELD_NUMBER = 24;
private volatile java.lang.Object billingSetup_;
/**
*
* Output only. The resource name of the billing setup associated with this account-level
* budget. BillingSetup resource names have the form:
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 24 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the billingSetup field is set.
*/
@java.lang.Override
public boolean hasBillingSetup() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The resource name of the billing setup associated with this account-level
* budget. BillingSetup resource names have the form:
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 24 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The billingSetup.
*/
@java.lang.Override
public java.lang.String getBillingSetup() {
java.lang.Object ref = billingSetup_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
billingSetup_ = s;
return s;
}
}
/**
*
* Output only. The resource name of the billing setup associated with this account-level
* budget. BillingSetup resource names have the form:
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 24 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for billingSetup.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getBillingSetupBytes() {
java.lang.Object ref = billingSetup_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
billingSetup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 4;
private int status_;
/**
*
* Output only. The status of this account-level budget.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus status = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Output only. The status of this account-level budget.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus status = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The status.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus getStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus result = com.google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus.valueOf(status_);
return result == null ? com.google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus.UNRECOGNIZED : result;
}
public static final int NAME_FIELD_NUMBER = 25;
private volatile java.lang.Object name_;
/**
*
* Output only. The name of the account-level budget.
*
*
* optional string name = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. The name of the account-level budget.
*
*
* optional string name = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* Output only. The name of the account-level budget.
*
*
* optional string name = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROPOSED_START_DATE_TIME_FIELD_NUMBER = 26;
private volatile java.lang.Object proposedStartDateTime_;
/**
*
* Output only. The proposed start time of the account-level budget in
* yyyy-MM-dd HH:mm:ss format. If a start time type of NOW was proposed,
* this is the time of request.
*
*
* optional string proposed_start_date_time = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the proposedStartDateTime field is set.
*/
@java.lang.Override
public boolean hasProposedStartDateTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. The proposed start time of the account-level budget in
* yyyy-MM-dd HH:mm:ss format. If a start time type of NOW was proposed,
* this is the time of request.
*
*
* optional string proposed_start_date_time = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposedStartDateTime.
*/
@java.lang.Override
public java.lang.String getProposedStartDateTime() {
java.lang.Object ref = proposedStartDateTime_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
proposedStartDateTime_ = s;
return s;
}
}
/**
*
* Output only. The proposed start time of the account-level budget in
* yyyy-MM-dd HH:mm:ss format. If a start time type of NOW was proposed,
* this is the time of request.
*
*
* optional string proposed_start_date_time = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for proposedStartDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProposedStartDateTimeBytes() {
java.lang.Object ref = proposedStartDateTime_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
proposedStartDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPROVED_START_DATE_TIME_FIELD_NUMBER = 27;
private volatile java.lang.Object approvedStartDateTime_;
/**
*
* Output only. The approved start time of the account-level budget in yyyy-MM-dd HH:mm:ss
* format.
* For example, if a new budget is approved after the proposed start time,
* the approved start time is the time of approval.
*
*
* optional string approved_start_date_time = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedStartDateTime field is set.
*/
@java.lang.Override
public boolean hasApprovedStartDateTime() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The approved start time of the account-level budget in yyyy-MM-dd HH:mm:ss
* format.
* For example, if a new budget is approved after the proposed start time,
* the approved start time is the time of approval.
*
*
* optional string approved_start_date_time = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedStartDateTime.
*/
@java.lang.Override
public java.lang.String getApprovedStartDateTime() {
java.lang.Object ref = approvedStartDateTime_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
approvedStartDateTime_ = s;
return s;
}
}
/**
*
* Output only. The approved start time of the account-level budget in yyyy-MM-dd HH:mm:ss
* format.
* For example, if a new budget is approved after the proposed start time,
* the approved start time is the time of approval.
*
*
* optional string approved_start_date_time = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for approvedStartDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getApprovedStartDateTimeBytes() {
java.lang.Object ref = approvedStartDateTime_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
approvedStartDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TOTAL_ADJUSTMENTS_MICROS_FIELD_NUMBER = 33;
private long totalAdjustmentsMicros_;
/**
*
* Output only. The total adjustments amount.
* An example of an adjustment is courtesy credits.
*
*
* int64 total_adjustments_micros = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The totalAdjustmentsMicros.
*/
@java.lang.Override
public long getTotalAdjustmentsMicros() {
return totalAdjustmentsMicros_;
}
public static final int AMOUNT_SERVED_MICROS_FIELD_NUMBER = 34;
private long amountServedMicros_;
/**
*
* Output only. The value of Ads that have been served, in micros.
* This includes overdelivery costs, in which case a credit might be
* automatically applied to the budget (see total_adjustments_micros).
*
*
* int64 amount_served_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The amountServedMicros.
*/
@java.lang.Override
public long getAmountServedMicros() {
return amountServedMicros_;
}
public static final int PURCHASE_ORDER_NUMBER_FIELD_NUMBER = 35;
private volatile java.lang.Object purchaseOrderNumber_;
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the purchaseOrderNumber field is set.
*/
@java.lang.Override
public boolean hasPurchaseOrderNumber() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The purchaseOrderNumber.
*/
@java.lang.Override
public java.lang.String getPurchaseOrderNumber() {
java.lang.Object ref = purchaseOrderNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
purchaseOrderNumber_ = s;
return s;
}
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for purchaseOrderNumber.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPurchaseOrderNumberBytes() {
java.lang.Object ref = purchaseOrderNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
purchaseOrderNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NOTES_FIELD_NUMBER = 36;
private volatile java.lang.Object notes_;
/**
*
* Output only. Notes associated with the budget.
*
*
* optional string notes = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the notes field is set.
*/
@java.lang.Override
public boolean hasNotes() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. Notes associated with the budget.
*
*
* optional string notes = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The notes.
*/
@java.lang.Override
public java.lang.String getNotes() {
java.lang.Object ref = notes_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
notes_ = s;
return s;
}
}
/**
*
* Output only. Notes associated with the budget.
*
*
* optional string notes = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for notes.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNotesBytes() {
java.lang.Object ref = notes_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
notes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PENDING_PROPOSAL_FIELD_NUMBER = 22;
private com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pendingProposal_;
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the pendingProposal field is set.
*/
@java.lang.Override
public boolean hasPendingProposal() {
return pendingProposal_ != null;
}
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The pendingProposal.
*/
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal getPendingProposal() {
return pendingProposal_ == null ? com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.getDefaultInstance() : pendingProposal_;
}
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposalOrBuilder getPendingProposalOrBuilder() {
return getPendingProposal();
}
public static final int PROPOSED_END_DATE_TIME_FIELD_NUMBER = 28;
/**
*
* Output only. The proposed end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string proposed_end_date_time = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the proposedEndDateTime field is set.
*/
public boolean hasProposedEndDateTime() {
return proposedEndTimeCase_ == 28;
}
/**
*
* Output only. The proposed end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string proposed_end_date_time = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposedEndDateTime.
*/
public java.lang.String getProposedEndDateTime() {
java.lang.Object ref = "";
if (proposedEndTimeCase_ == 28) {
ref = proposedEndTime_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (proposedEndTimeCase_ == 28) {
proposedEndTime_ = s;
}
return s;
}
}
/**
*
* Output only. The proposed end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string proposed_end_date_time = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for proposedEndDateTime.
*/
public com.google.protobuf.ByteString
getProposedEndDateTimeBytes() {
java.lang.Object ref = "";
if (proposedEndTimeCase_ == 28) {
ref = proposedEndTime_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (proposedEndTimeCase_ == 28) {
proposedEndTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROPOSED_END_TIME_TYPE_FIELD_NUMBER = 9;
/**
*
* Output only. The proposed end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the proposedEndTimeType field is set.
*/
public boolean hasProposedEndTimeType() {
return proposedEndTimeCase_ == 9;
}
/**
*
* Output only. The proposed end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for proposedEndTimeType.
*/
public int getProposedEndTimeTypeValue() {
if (proposedEndTimeCase_ == 9) {
return (java.lang.Integer) proposedEndTime_;
}
return 0;
}
/**
*
* Output only. The proposed end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposedEndTimeType.
*/
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getProposedEndTimeType() {
if (proposedEndTimeCase_ == 9) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) proposedEndTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
public static final int APPROVED_END_DATE_TIME_FIELD_NUMBER = 29;
/**
*
* Output only. The approved end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string approved_end_date_time = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedEndDateTime field is set.
*/
public boolean hasApprovedEndDateTime() {
return approvedEndTimeCase_ == 29;
}
/**
*
* Output only. The approved end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string approved_end_date_time = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedEndDateTime.
*/
public java.lang.String getApprovedEndDateTime() {
java.lang.Object ref = "";
if (approvedEndTimeCase_ == 29) {
ref = approvedEndTime_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (approvedEndTimeCase_ == 29) {
approvedEndTime_ = s;
}
return s;
}
}
/**
*
* Output only. The approved end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string approved_end_date_time = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for approvedEndDateTime.
*/
public com.google.protobuf.ByteString
getApprovedEndDateTimeBytes() {
java.lang.Object ref = "";
if (approvedEndTimeCase_ == 29) {
ref = approvedEndTime_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (approvedEndTimeCase_ == 29) {
approvedEndTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPROVED_END_TIME_TYPE_FIELD_NUMBER = 11;
/**
*
* Output only. The approved end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedEndTimeType field is set.
*/
public boolean hasApprovedEndTimeType() {
return approvedEndTimeCase_ == 11;
}
/**
*
* Output only. The approved end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for approvedEndTimeType.
*/
public int getApprovedEndTimeTypeValue() {
if (approvedEndTimeCase_ == 11) {
return (java.lang.Integer) approvedEndTime_;
}
return 0;
}
/**
*
* Output only. The approved end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedEndTimeType.
*/
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getApprovedEndTimeType() {
if (approvedEndTimeCase_ == 11) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) approvedEndTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
public static final int PROPOSED_SPENDING_LIMIT_MICROS_FIELD_NUMBER = 30;
/**
*
* Output only. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the proposedSpendingLimitMicros field is set.
*/
@java.lang.Override
public boolean hasProposedSpendingLimitMicros() {
return proposedSpendingLimitCase_ == 30;
}
/**
*
* Output only. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposedSpendingLimitMicros.
*/
@java.lang.Override
public long getProposedSpendingLimitMicros() {
if (proposedSpendingLimitCase_ == 30) {
return (java.lang.Long) proposedSpendingLimit_;
}
return 0L;
}
public static final int PROPOSED_SPENDING_LIMIT_TYPE_FIELD_NUMBER = 13;
/**
*
* Output only. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the proposedSpendingLimitType field is set.
*/
public boolean hasProposedSpendingLimitType() {
return proposedSpendingLimitCase_ == 13;
}
/**
*
* Output only. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for proposedSpendingLimitType.
*/
public int getProposedSpendingLimitTypeValue() {
if (proposedSpendingLimitCase_ == 13) {
return (java.lang.Integer) proposedSpendingLimit_;
}
return 0;
}
/**
*
* Output only. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposedSpendingLimitType.
*/
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getProposedSpendingLimitType() {
if (proposedSpendingLimitCase_ == 13) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) proposedSpendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
public static final int APPROVED_SPENDING_LIMIT_MICROS_FIELD_NUMBER = 31;
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit. This will only be populated if the proposed spending limit
* is finite, and will always be greater than or equal to the
* proposed spending limit.
*
*
* int64 approved_spending_limit_micros = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedSpendingLimitMicros field is set.
*/
@java.lang.Override
public boolean hasApprovedSpendingLimitMicros() {
return approvedSpendingLimitCase_ == 31;
}
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit. This will only be populated if the proposed spending limit
* is finite, and will always be greater than or equal to the
* proposed spending limit.
*
*
* int64 approved_spending_limit_micros = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedSpendingLimitMicros.
*/
@java.lang.Override
public long getApprovedSpendingLimitMicros() {
if (approvedSpendingLimitCase_ == 31) {
return (java.lang.Long) approvedSpendingLimit_;
}
return 0L;
}
public static final int APPROVED_SPENDING_LIMIT_TYPE_FIELD_NUMBER = 15;
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the approved spending limit is
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedSpendingLimitType field is set.
*/
public boolean hasApprovedSpendingLimitType() {
return approvedSpendingLimitCase_ == 15;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the approved spending limit is
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for approvedSpendingLimitType.
*/
public int getApprovedSpendingLimitTypeValue() {
if (approvedSpendingLimitCase_ == 15) {
return (java.lang.Integer) approvedSpendingLimit_;
}
return 0;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the approved spending limit is
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedSpendingLimitType.
*/
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getApprovedSpendingLimitType() {
if (approvedSpendingLimitCase_ == 15) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) approvedSpendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
public static final int ADJUSTED_SPENDING_LIMIT_MICROS_FIELD_NUMBER = 32;
/**
*
* Output only. The adjusted spending limit in micros. One million is equivalent to
* one unit.
* If the approved spending limit is finite, the adjusted
* spending limit may vary depending on the types of adjustments applied
* to this budget, if applicable.
* The different kinds of adjustments are described here:
* https://support.google.com/google-ads/answer/1704323
* For example, a debit adjustment reduces how much the account is
* allowed to spend.
*
*
* int64 adjusted_spending_limit_micros = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the adjustedSpendingLimitMicros field is set.
*/
@java.lang.Override
public boolean hasAdjustedSpendingLimitMicros() {
return adjustedSpendingLimitCase_ == 32;
}
/**
*
* Output only. The adjusted spending limit in micros. One million is equivalent to
* one unit.
* If the approved spending limit is finite, the adjusted
* spending limit may vary depending on the types of adjustments applied
* to this budget, if applicable.
* The different kinds of adjustments are described here:
* https://support.google.com/google-ads/answer/1704323
* For example, a debit adjustment reduces how much the account is
* allowed to spend.
*
*
* int64 adjusted_spending_limit_micros = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The adjustedSpendingLimitMicros.
*/
@java.lang.Override
public long getAdjustedSpendingLimitMicros() {
if (adjustedSpendingLimitCase_ == 32) {
return (java.lang.Long) adjustedSpendingLimit_;
}
return 0L;
}
public static final int ADJUSTED_SPENDING_LIMIT_TYPE_FIELD_NUMBER = 17;
/**
*
* Output only. The adjusted spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the adjusted spending limit is
* INFINITE, which is guaranteed to be true if the approved spending limit
* is INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType adjusted_spending_limit_type = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the adjustedSpendingLimitType field is set.
*/
public boolean hasAdjustedSpendingLimitType() {
return adjustedSpendingLimitCase_ == 17;
}
/**
*
* Output only. The adjusted spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the adjusted spending limit is
* INFINITE, which is guaranteed to be true if the approved spending limit
* is INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType adjusted_spending_limit_type = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for adjustedSpendingLimitType.
*/
public int getAdjustedSpendingLimitTypeValue() {
if (adjustedSpendingLimitCase_ == 17) {
return (java.lang.Integer) adjustedSpendingLimit_;
}
return 0;
}
/**
*
* Output only. The adjusted spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the adjusted spending limit is
* INFINITE, which is guaranteed to be true if the approved spending limit
* is INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType adjusted_spending_limit_type = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The adjustedSpendingLimitType.
*/
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getAdjustedSpendingLimitType() {
if (adjustedSpendingLimitCase_ == 17) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) adjustedSpendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, resourceName_);
}
if (status_ != com.google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus.UNSPECIFIED.getNumber()) {
output.writeEnum(4, status_);
}
if (proposedEndTimeCase_ == 9) {
output.writeEnum(9, ((java.lang.Integer) proposedEndTime_));
}
if (approvedEndTimeCase_ == 11) {
output.writeEnum(11, ((java.lang.Integer) approvedEndTime_));
}
if (proposedSpendingLimitCase_ == 13) {
output.writeEnum(13, ((java.lang.Integer) proposedSpendingLimit_));
}
if (approvedSpendingLimitCase_ == 15) {
output.writeEnum(15, ((java.lang.Integer) approvedSpendingLimit_));
}
if (adjustedSpendingLimitCase_ == 17) {
output.writeEnum(17, ((java.lang.Integer) adjustedSpendingLimit_));
}
if (pendingProposal_ != null) {
output.writeMessage(22, getPendingProposal());
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(23, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 24, billingSetup_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 25, name_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 26, proposedStartDateTime_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 27, approvedStartDateTime_);
}
if (proposedEndTimeCase_ == 28) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 28, proposedEndTime_);
}
if (approvedEndTimeCase_ == 29) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 29, approvedEndTime_);
}
if (proposedSpendingLimitCase_ == 30) {
output.writeInt64(
30, (long)((java.lang.Long) proposedSpendingLimit_));
}
if (approvedSpendingLimitCase_ == 31) {
output.writeInt64(
31, (long)((java.lang.Long) approvedSpendingLimit_));
}
if (adjustedSpendingLimitCase_ == 32) {
output.writeInt64(
32, (long)((java.lang.Long) adjustedSpendingLimit_));
}
if (totalAdjustmentsMicros_ != 0L) {
output.writeInt64(33, totalAdjustmentsMicros_);
}
if (amountServedMicros_ != 0L) {
output.writeInt64(34, amountServedMicros_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 35, purchaseOrderNumber_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 36, notes_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, resourceName_);
}
if (status_ != com.google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, status_);
}
if (proposedEndTimeCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(9, ((java.lang.Integer) proposedEndTime_));
}
if (approvedEndTimeCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(11, ((java.lang.Integer) approvedEndTime_));
}
if (proposedSpendingLimitCase_ == 13) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(13, ((java.lang.Integer) proposedSpendingLimit_));
}
if (approvedSpendingLimitCase_ == 15) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(15, ((java.lang.Integer) approvedSpendingLimit_));
}
if (adjustedSpendingLimitCase_ == 17) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(17, ((java.lang.Integer) adjustedSpendingLimit_));
}
if (pendingProposal_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(22, getPendingProposal());
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(23, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(24, billingSetup_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(25, name_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(26, proposedStartDateTime_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(27, approvedStartDateTime_);
}
if (proposedEndTimeCase_ == 28) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(28, proposedEndTime_);
}
if (approvedEndTimeCase_ == 29) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(29, approvedEndTime_);
}
if (proposedSpendingLimitCase_ == 30) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
30, (long)((java.lang.Long) proposedSpendingLimit_));
}
if (approvedSpendingLimitCase_ == 31) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
31, (long)((java.lang.Long) approvedSpendingLimit_));
}
if (adjustedSpendingLimitCase_ == 32) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
32, (long)((java.lang.Long) adjustedSpendingLimit_));
}
if (totalAdjustmentsMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(33, totalAdjustmentsMicros_);
}
if (amountServedMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(34, amountServedMicros_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(35, purchaseOrderNumber_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(36, notes_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v10.resources.AccountBudget)) {
return super.equals(obj);
}
com.google.ads.googleads.v10.resources.AccountBudget other = (com.google.ads.googleads.v10.resources.AccountBudget) obj;
if (!getResourceName()
.equals(other.getResourceName())) return false;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId()
!= other.getId()) return false;
}
if (hasBillingSetup() != other.hasBillingSetup()) return false;
if (hasBillingSetup()) {
if (!getBillingSetup()
.equals(other.getBillingSetup())) return false;
}
if (status_ != other.status_) return false;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasProposedStartDateTime() != other.hasProposedStartDateTime()) return false;
if (hasProposedStartDateTime()) {
if (!getProposedStartDateTime()
.equals(other.getProposedStartDateTime())) return false;
}
if (hasApprovedStartDateTime() != other.hasApprovedStartDateTime()) return false;
if (hasApprovedStartDateTime()) {
if (!getApprovedStartDateTime()
.equals(other.getApprovedStartDateTime())) return false;
}
if (getTotalAdjustmentsMicros()
!= other.getTotalAdjustmentsMicros()) return false;
if (getAmountServedMicros()
!= other.getAmountServedMicros()) return false;
if (hasPurchaseOrderNumber() != other.hasPurchaseOrderNumber()) return false;
if (hasPurchaseOrderNumber()) {
if (!getPurchaseOrderNumber()
.equals(other.getPurchaseOrderNumber())) return false;
}
if (hasNotes() != other.hasNotes()) return false;
if (hasNotes()) {
if (!getNotes()
.equals(other.getNotes())) return false;
}
if (hasPendingProposal() != other.hasPendingProposal()) return false;
if (hasPendingProposal()) {
if (!getPendingProposal()
.equals(other.getPendingProposal())) return false;
}
if (!getProposedEndTimeCase().equals(other.getProposedEndTimeCase())) return false;
switch (proposedEndTimeCase_) {
case 28:
if (!getProposedEndDateTime()
.equals(other.getProposedEndDateTime())) return false;
break;
case 9:
if (getProposedEndTimeTypeValue()
!= other.getProposedEndTimeTypeValue()) return false;
break;
case 0:
default:
}
if (!getApprovedEndTimeCase().equals(other.getApprovedEndTimeCase())) return false;
switch (approvedEndTimeCase_) {
case 29:
if (!getApprovedEndDateTime()
.equals(other.getApprovedEndDateTime())) return false;
break;
case 11:
if (getApprovedEndTimeTypeValue()
!= other.getApprovedEndTimeTypeValue()) return false;
break;
case 0:
default:
}
if (!getProposedSpendingLimitCase().equals(other.getProposedSpendingLimitCase())) return false;
switch (proposedSpendingLimitCase_) {
case 30:
if (getProposedSpendingLimitMicros()
!= other.getProposedSpendingLimitMicros()) return false;
break;
case 13:
if (getProposedSpendingLimitTypeValue()
!= other.getProposedSpendingLimitTypeValue()) return false;
break;
case 0:
default:
}
if (!getApprovedSpendingLimitCase().equals(other.getApprovedSpendingLimitCase())) return false;
switch (approvedSpendingLimitCase_) {
case 31:
if (getApprovedSpendingLimitMicros()
!= other.getApprovedSpendingLimitMicros()) return false;
break;
case 15:
if (getApprovedSpendingLimitTypeValue()
!= other.getApprovedSpendingLimitTypeValue()) return false;
break;
case 0:
default:
}
if (!getAdjustedSpendingLimitCase().equals(other.getAdjustedSpendingLimitCase())) return false;
switch (adjustedSpendingLimitCase_) {
case 32:
if (getAdjustedSpendingLimitMicros()
!= other.getAdjustedSpendingLimitMicros()) return false;
break;
case 17:
if (getAdjustedSpendingLimitTypeValue()
!= other.getAdjustedSpendingLimitTypeValue()) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RESOURCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getResourceName().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getId());
}
if (hasBillingSetup()) {
hash = (37 * hash) + BILLING_SETUP_FIELD_NUMBER;
hash = (53 * hash) + getBillingSetup().hashCode();
}
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasProposedStartDateTime()) {
hash = (37 * hash) + PROPOSED_START_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getProposedStartDateTime().hashCode();
}
if (hasApprovedStartDateTime()) {
hash = (37 * hash) + APPROVED_START_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getApprovedStartDateTime().hashCode();
}
hash = (37 * hash) + TOTAL_ADJUSTMENTS_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTotalAdjustmentsMicros());
hash = (37 * hash) + AMOUNT_SERVED_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAmountServedMicros());
if (hasPurchaseOrderNumber()) {
hash = (37 * hash) + PURCHASE_ORDER_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getPurchaseOrderNumber().hashCode();
}
if (hasNotes()) {
hash = (37 * hash) + NOTES_FIELD_NUMBER;
hash = (53 * hash) + getNotes().hashCode();
}
if (hasPendingProposal()) {
hash = (37 * hash) + PENDING_PROPOSAL_FIELD_NUMBER;
hash = (53 * hash) + getPendingProposal().hashCode();
}
switch (proposedEndTimeCase_) {
case 28:
hash = (37 * hash) + PROPOSED_END_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getProposedEndDateTime().hashCode();
break;
case 9:
hash = (37 * hash) + PROPOSED_END_TIME_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getProposedEndTimeTypeValue();
break;
case 0:
default:
}
switch (approvedEndTimeCase_) {
case 29:
hash = (37 * hash) + APPROVED_END_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getApprovedEndDateTime().hashCode();
break;
case 11:
hash = (37 * hash) + APPROVED_END_TIME_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getApprovedEndTimeTypeValue();
break;
case 0:
default:
}
switch (proposedSpendingLimitCase_) {
case 30:
hash = (37 * hash) + PROPOSED_SPENDING_LIMIT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getProposedSpendingLimitMicros());
break;
case 13:
hash = (37 * hash) + PROPOSED_SPENDING_LIMIT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getProposedSpendingLimitTypeValue();
break;
case 0:
default:
}
switch (approvedSpendingLimitCase_) {
case 31:
hash = (37 * hash) + APPROVED_SPENDING_LIMIT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getApprovedSpendingLimitMicros());
break;
case 15:
hash = (37 * hash) + APPROVED_SPENDING_LIMIT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getApprovedSpendingLimitTypeValue();
break;
case 0:
default:
}
switch (adjustedSpendingLimitCase_) {
case 32:
hash = (37 * hash) + ADJUSTED_SPENDING_LIMIT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAdjustedSpendingLimitMicros());
break;
case 17:
hash = (37 * hash) + ADJUSTED_SPENDING_LIMIT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getAdjustedSpendingLimitTypeValue();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AccountBudget parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v10.resources.AccountBudget prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An account-level budget. It contains information about the budget itself,
* as well as the most recently approved changes to the budget and proposed
* changes that are pending approval. The proposed changes that are pending
* approval, if any, are found in 'pending_proposal'. Effective details about
* the budget are found in fields prefixed 'approved_', 'adjusted_' and those
* without a prefix. Since some effective details may differ from what the user
* had originally requested (for example, spending limit), these differences are
* juxtaposed through 'proposed_', 'approved_', and possibly 'adjusted_' fields.
* This resource is mutated using AccountBudgetProposal and cannot be mutated
* directly. A budget may have at most one pending proposal at any given time.
* It is read through pending_proposal.
* Once approved, a budget may be subject to adjustments, such as credit
* adjustments. Adjustments create differences between the 'approved' and
* 'adjusted' fields, which would otherwise be identical.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.AccountBudget}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v10.resources.AccountBudget)
com.google.ads.googleads.v10.resources.AccountBudgetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.AccountBudgetProto.internal_static_google_ads_googleads_v10_resources_AccountBudget_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.AccountBudgetProto.internal_static_google_ads_googleads_v10_resources_AccountBudget_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.AccountBudget.class, com.google.ads.googleads.v10.resources.AccountBudget.Builder.class);
}
// Construct using com.google.ads.googleads.v10.resources.AccountBudget.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
resourceName_ = "";
id_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
billingSetup_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
status_ = 0;
name_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
proposedStartDateTime_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
approvedStartDateTime_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
totalAdjustmentsMicros_ = 0L;
amountServedMicros_ = 0L;
purchaseOrderNumber_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
notes_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
if (pendingProposalBuilder_ == null) {
pendingProposal_ = null;
} else {
pendingProposal_ = null;
pendingProposalBuilder_ = null;
}
proposedEndTimeCase_ = 0;
proposedEndTime_ = null;
approvedEndTimeCase_ = 0;
approvedEndTime_ = null;
proposedSpendingLimitCase_ = 0;
proposedSpendingLimit_ = null;
approvedSpendingLimitCase_ = 0;
approvedSpendingLimit_ = null;
adjustedSpendingLimitCase_ = 0;
adjustedSpendingLimit_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v10.resources.AccountBudgetProto.internal_static_google_ads_googleads_v10_resources_AccountBudget_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudget getDefaultInstanceForType() {
return com.google.ads.googleads.v10.resources.AccountBudget.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudget build() {
com.google.ads.googleads.v10.resources.AccountBudget result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudget buildPartial() {
com.google.ads.googleads.v10.resources.AccountBudget result = new com.google.ads.googleads.v10.resources.AccountBudget(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.resourceName_ = resourceName_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.billingSetup_ = billingSetup_;
result.status_ = status_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.proposedStartDateTime_ = proposedStartDateTime_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.approvedStartDateTime_ = approvedStartDateTime_;
result.totalAdjustmentsMicros_ = totalAdjustmentsMicros_;
result.amountServedMicros_ = amountServedMicros_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.purchaseOrderNumber_ = purchaseOrderNumber_;
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000040;
}
result.notes_ = notes_;
if (pendingProposalBuilder_ == null) {
result.pendingProposal_ = pendingProposal_;
} else {
result.pendingProposal_ = pendingProposalBuilder_.build();
}
if (proposedEndTimeCase_ == 28) {
result.proposedEndTime_ = proposedEndTime_;
}
if (proposedEndTimeCase_ == 9) {
result.proposedEndTime_ = proposedEndTime_;
}
if (approvedEndTimeCase_ == 29) {
result.approvedEndTime_ = approvedEndTime_;
}
if (approvedEndTimeCase_ == 11) {
result.approvedEndTime_ = approvedEndTime_;
}
if (proposedSpendingLimitCase_ == 30) {
result.proposedSpendingLimit_ = proposedSpendingLimit_;
}
if (proposedSpendingLimitCase_ == 13) {
result.proposedSpendingLimit_ = proposedSpendingLimit_;
}
if (approvedSpendingLimitCase_ == 31) {
result.approvedSpendingLimit_ = approvedSpendingLimit_;
}
if (approvedSpendingLimitCase_ == 15) {
result.approvedSpendingLimit_ = approvedSpendingLimit_;
}
if (adjustedSpendingLimitCase_ == 32) {
result.adjustedSpendingLimit_ = adjustedSpendingLimit_;
}
if (adjustedSpendingLimitCase_ == 17) {
result.adjustedSpendingLimit_ = adjustedSpendingLimit_;
}
result.bitField0_ = to_bitField0_;
result.proposedEndTimeCase_ = proposedEndTimeCase_;
result.approvedEndTimeCase_ = approvedEndTimeCase_;
result.proposedSpendingLimitCase_ = proposedSpendingLimitCase_;
result.approvedSpendingLimitCase_ = approvedSpendingLimitCase_;
result.adjustedSpendingLimitCase_ = adjustedSpendingLimitCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v10.resources.AccountBudget) {
return mergeFrom((com.google.ads.googleads.v10.resources.AccountBudget)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v10.resources.AccountBudget other) {
if (other == com.google.ads.googleads.v10.resources.AccountBudget.getDefaultInstance()) return this;
if (!other.getResourceName().isEmpty()) {
resourceName_ = other.resourceName_;
onChanged();
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasBillingSetup()) {
bitField0_ |= 0x00000002;
billingSetup_ = other.billingSetup_;
onChanged();
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.hasName()) {
bitField0_ |= 0x00000004;
name_ = other.name_;
onChanged();
}
if (other.hasProposedStartDateTime()) {
bitField0_ |= 0x00000008;
proposedStartDateTime_ = other.proposedStartDateTime_;
onChanged();
}
if (other.hasApprovedStartDateTime()) {
bitField0_ |= 0x00000010;
approvedStartDateTime_ = other.approvedStartDateTime_;
onChanged();
}
if (other.getTotalAdjustmentsMicros() != 0L) {
setTotalAdjustmentsMicros(other.getTotalAdjustmentsMicros());
}
if (other.getAmountServedMicros() != 0L) {
setAmountServedMicros(other.getAmountServedMicros());
}
if (other.hasPurchaseOrderNumber()) {
bitField0_ |= 0x00000020;
purchaseOrderNumber_ = other.purchaseOrderNumber_;
onChanged();
}
if (other.hasNotes()) {
bitField0_ |= 0x00000040;
notes_ = other.notes_;
onChanged();
}
if (other.hasPendingProposal()) {
mergePendingProposal(other.getPendingProposal());
}
switch (other.getProposedEndTimeCase()) {
case PROPOSED_END_DATE_TIME: {
proposedEndTimeCase_ = 28;
proposedEndTime_ = other.proposedEndTime_;
onChanged();
break;
}
case PROPOSED_END_TIME_TYPE: {
setProposedEndTimeTypeValue(other.getProposedEndTimeTypeValue());
break;
}
case PROPOSEDENDTIME_NOT_SET: {
break;
}
}
switch (other.getApprovedEndTimeCase()) {
case APPROVED_END_DATE_TIME: {
approvedEndTimeCase_ = 29;
approvedEndTime_ = other.approvedEndTime_;
onChanged();
break;
}
case APPROVED_END_TIME_TYPE: {
setApprovedEndTimeTypeValue(other.getApprovedEndTimeTypeValue());
break;
}
case APPROVEDENDTIME_NOT_SET: {
break;
}
}
switch (other.getProposedSpendingLimitCase()) {
case PROPOSED_SPENDING_LIMIT_MICROS: {
setProposedSpendingLimitMicros(other.getProposedSpendingLimitMicros());
break;
}
case PROPOSED_SPENDING_LIMIT_TYPE: {
setProposedSpendingLimitTypeValue(other.getProposedSpendingLimitTypeValue());
break;
}
case PROPOSEDSPENDINGLIMIT_NOT_SET: {
break;
}
}
switch (other.getApprovedSpendingLimitCase()) {
case APPROVED_SPENDING_LIMIT_MICROS: {
setApprovedSpendingLimitMicros(other.getApprovedSpendingLimitMicros());
break;
}
case APPROVED_SPENDING_LIMIT_TYPE: {
setApprovedSpendingLimitTypeValue(other.getApprovedSpendingLimitTypeValue());
break;
}
case APPROVEDSPENDINGLIMIT_NOT_SET: {
break;
}
}
switch (other.getAdjustedSpendingLimitCase()) {
case ADJUSTED_SPENDING_LIMIT_MICROS: {
setAdjustedSpendingLimitMicros(other.getAdjustedSpendingLimitMicros());
break;
}
case ADJUSTED_SPENDING_LIMIT_TYPE: {
setAdjustedSpendingLimitTypeValue(other.getAdjustedSpendingLimitTypeValue());
break;
}
case ADJUSTEDSPENDINGLIMIT_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
resourceName_ = input.readStringRequireUtf8();
break;
} // case 10
case 32: {
status_ = input.readEnum();
break;
} // case 32
case 72: {
int rawValue = input.readEnum();
proposedEndTimeCase_ = 9;
proposedEndTime_ = rawValue;
break;
} // case 72
case 88: {
int rawValue = input.readEnum();
approvedEndTimeCase_ = 11;
approvedEndTime_ = rawValue;
break;
} // case 88
case 104: {
int rawValue = input.readEnum();
proposedSpendingLimitCase_ = 13;
proposedSpendingLimit_ = rawValue;
break;
} // case 104
case 120: {
int rawValue = input.readEnum();
approvedSpendingLimitCase_ = 15;
approvedSpendingLimit_ = rawValue;
break;
} // case 120
case 136: {
int rawValue = input.readEnum();
adjustedSpendingLimitCase_ = 17;
adjustedSpendingLimit_ = rawValue;
break;
} // case 136
case 178: {
input.readMessage(
getPendingProposalFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 178
case 184: {
id_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 184
case 194: {
billingSetup_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 194
case 202: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 202
case 210: {
proposedStartDateTime_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 210
case 218: {
approvedStartDateTime_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 218
case 226: {
java.lang.String s = input.readStringRequireUtf8();
proposedEndTimeCase_ = 28;
proposedEndTime_ = s;
break;
} // case 226
case 234: {
java.lang.String s = input.readStringRequireUtf8();
approvedEndTimeCase_ = 29;
approvedEndTime_ = s;
break;
} // case 234
case 240: {
proposedSpendingLimit_ = input.readInt64();
proposedSpendingLimitCase_ = 30;
break;
} // case 240
case 248: {
approvedSpendingLimit_ = input.readInt64();
approvedSpendingLimitCase_ = 31;
break;
} // case 248
case 256: {
adjustedSpendingLimit_ = input.readInt64();
adjustedSpendingLimitCase_ = 32;
break;
} // case 256
case 264: {
totalAdjustmentsMicros_ = input.readInt64();
break;
} // case 264
case 272: {
amountServedMicros_ = input.readInt64();
break;
} // case 272
case 282: {
purchaseOrderNumber_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 282
case 290: {
notes_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 290
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int proposedEndTimeCase_ = 0;
private java.lang.Object proposedEndTime_;
public ProposedEndTimeCase
getProposedEndTimeCase() {
return ProposedEndTimeCase.forNumber(
proposedEndTimeCase_);
}
public Builder clearProposedEndTime() {
proposedEndTimeCase_ = 0;
proposedEndTime_ = null;
onChanged();
return this;
}
private int approvedEndTimeCase_ = 0;
private java.lang.Object approvedEndTime_;
public ApprovedEndTimeCase
getApprovedEndTimeCase() {
return ApprovedEndTimeCase.forNumber(
approvedEndTimeCase_);
}
public Builder clearApprovedEndTime() {
approvedEndTimeCase_ = 0;
approvedEndTime_ = null;
onChanged();
return this;
}
private int proposedSpendingLimitCase_ = 0;
private java.lang.Object proposedSpendingLimit_;
public ProposedSpendingLimitCase
getProposedSpendingLimitCase() {
return ProposedSpendingLimitCase.forNumber(
proposedSpendingLimitCase_);
}
public Builder clearProposedSpendingLimit() {
proposedSpendingLimitCase_ = 0;
proposedSpendingLimit_ = null;
onChanged();
return this;
}
private int approvedSpendingLimitCase_ = 0;
private java.lang.Object approvedSpendingLimit_;
public ApprovedSpendingLimitCase
getApprovedSpendingLimitCase() {
return ApprovedSpendingLimitCase.forNumber(
approvedSpendingLimitCase_);
}
public Builder clearApprovedSpendingLimit() {
approvedSpendingLimitCase_ = 0;
approvedSpendingLimit_ = null;
onChanged();
return this;
}
private int adjustedSpendingLimitCase_ = 0;
private java.lang.Object adjustedSpendingLimit_;
public AdjustedSpendingLimitCase
getAdjustedSpendingLimitCase() {
return AdjustedSpendingLimitCase.forNumber(
adjustedSpendingLimitCase_);
}
public Builder clearAdjustedSpendingLimit() {
adjustedSpendingLimitCase_ = 0;
adjustedSpendingLimit_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object resourceName_ = "";
/**
*
* Output only. The resource name of the account-level budget.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The resource name of the account-level budget.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The resource name of the account-level budget.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resourceName_ = value;
onChanged();
return this;
}
/**
*
* Output only. The resource name of the account-level budget.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearResourceName() {
resourceName_ = getDefaultInstance().getResourceName();
onChanged();
return this;
}
/**
*
* Output only. The resource name of the account-level budget.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resourceName_ = value;
onChanged();
return this;
}
private long id_ ;
/**
*
* Output only. The ID of the account-level budget.
*
*
* optional int64 id = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The ID of the account-level budget.
*
*
* optional int64 id = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
*
* Output only. The ID of the account-level budget.
*
*
* optional int64 id = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
*
* Output only. The ID of the account-level budget.
*
*
* optional int64 id = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object billingSetup_ = "";
/**
*
* Output only. The resource name of the billing setup associated with this account-level
* budget. BillingSetup resource names have the form:
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 24 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the billingSetup field is set.
*/
public boolean hasBillingSetup() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The resource name of the billing setup associated with this account-level
* budget. BillingSetup resource names have the form:
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 24 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The billingSetup.
*/
public java.lang.String getBillingSetup() {
java.lang.Object ref = billingSetup_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
billingSetup_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The resource name of the billing setup associated with this account-level
* budget. BillingSetup resource names have the form:
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 24 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for billingSetup.
*/
public com.google.protobuf.ByteString
getBillingSetupBytes() {
java.lang.Object ref = billingSetup_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
billingSetup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The resource name of the billing setup associated with this account-level
* budget. BillingSetup resource names have the form:
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 24 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The billingSetup to set.
* @return This builder for chaining.
*/
public Builder setBillingSetup(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
billingSetup_ = value;
onChanged();
return this;
}
/**
*
* Output only. The resource name of the billing setup associated with this account-level
* budget. BillingSetup resource names have the form:
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 24 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearBillingSetup() {
bitField0_ = (bitField0_ & ~0x00000002);
billingSetup_ = getDefaultInstance().getBillingSetup();
onChanged();
return this;
}
/**
*
* Output only. The resource name of the billing setup associated with this account-level
* budget. BillingSetup resource names have the form:
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 24 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for billingSetup to set.
* @return This builder for chaining.
*/
public Builder setBillingSetupBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000002;
billingSetup_ = value;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Output only. The status of this account-level budget.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus status = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Output only. The status of this account-level budget.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus status = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Output only. The status of this account-level budget.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus status = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The status.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus getStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus result = com.google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus.valueOf(status_);
return result == null ? com.google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus.UNRECOGNIZED : result;
}
/**
*
* Output only. The status of this account-level budget.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus status = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The status of this account-level budget.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetStatusEnum.AccountBudgetStatus status = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* Output only. The name of the account-level budget.
*
*
* optional string name = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. The name of the account-level budget.
*
*
* optional string name = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The name of the account-level budget.
*
*
* optional string name = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The name of the account-level budget.
*
*
* optional string name = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
name_ = value;
onChanged();
return this;
}
/**
*
* Output only. The name of the account-level budget.
*
*
* optional string name = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000004);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Output only. The name of the account-level budget.
*
*
* optional string name = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000004;
name_ = value;
onChanged();
return this;
}
private java.lang.Object proposedStartDateTime_ = "";
/**
*
* Output only. The proposed start time of the account-level budget in
* yyyy-MM-dd HH:mm:ss format. If a start time type of NOW was proposed,
* this is the time of request.
*
*
* optional string proposed_start_date_time = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the proposedStartDateTime field is set.
*/
public boolean hasProposedStartDateTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. The proposed start time of the account-level budget in
* yyyy-MM-dd HH:mm:ss format. If a start time type of NOW was proposed,
* this is the time of request.
*
*
* optional string proposed_start_date_time = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposedStartDateTime.
*/
public java.lang.String getProposedStartDateTime() {
java.lang.Object ref = proposedStartDateTime_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
proposedStartDateTime_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The proposed start time of the account-level budget in
* yyyy-MM-dd HH:mm:ss format. If a start time type of NOW was proposed,
* this is the time of request.
*
*
* optional string proposed_start_date_time = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for proposedStartDateTime.
*/
public com.google.protobuf.ByteString
getProposedStartDateTimeBytes() {
java.lang.Object ref = proposedStartDateTime_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
proposedStartDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The proposed start time of the account-level budget in
* yyyy-MM-dd HH:mm:ss format. If a start time type of NOW was proposed,
* this is the time of request.
*
*
* optional string proposed_start_date_time = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The proposedStartDateTime to set.
* @return This builder for chaining.
*/
public Builder setProposedStartDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
proposedStartDateTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The proposed start time of the account-level budget in
* yyyy-MM-dd HH:mm:ss format. If a start time type of NOW was proposed,
* this is the time of request.
*
*
* optional string proposed_start_date_time = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearProposedStartDateTime() {
bitField0_ = (bitField0_ & ~0x00000008);
proposedStartDateTime_ = getDefaultInstance().getProposedStartDateTime();
onChanged();
return this;
}
/**
*
* Output only. The proposed start time of the account-level budget in
* yyyy-MM-dd HH:mm:ss format. If a start time type of NOW was proposed,
* this is the time of request.
*
*
* optional string proposed_start_date_time = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for proposedStartDateTime to set.
* @return This builder for chaining.
*/
public Builder setProposedStartDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000008;
proposedStartDateTime_ = value;
onChanged();
return this;
}
private java.lang.Object approvedStartDateTime_ = "";
/**
*
* Output only. The approved start time of the account-level budget in yyyy-MM-dd HH:mm:ss
* format.
* For example, if a new budget is approved after the proposed start time,
* the approved start time is the time of approval.
*
*
* optional string approved_start_date_time = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedStartDateTime field is set.
*/
public boolean hasApprovedStartDateTime() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The approved start time of the account-level budget in yyyy-MM-dd HH:mm:ss
* format.
* For example, if a new budget is approved after the proposed start time,
* the approved start time is the time of approval.
*
*
* optional string approved_start_date_time = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedStartDateTime.
*/
public java.lang.String getApprovedStartDateTime() {
java.lang.Object ref = approvedStartDateTime_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
approvedStartDateTime_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The approved start time of the account-level budget in yyyy-MM-dd HH:mm:ss
* format.
* For example, if a new budget is approved after the proposed start time,
* the approved start time is the time of approval.
*
*
* optional string approved_start_date_time = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for approvedStartDateTime.
*/
public com.google.protobuf.ByteString
getApprovedStartDateTimeBytes() {
java.lang.Object ref = approvedStartDateTime_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
approvedStartDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The approved start time of the account-level budget in yyyy-MM-dd HH:mm:ss
* format.
* For example, if a new budget is approved after the proposed start time,
* the approved start time is the time of approval.
*
*
* optional string approved_start_date_time = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The approvedStartDateTime to set.
* @return This builder for chaining.
*/
public Builder setApprovedStartDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
approvedStartDateTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved start time of the account-level budget in yyyy-MM-dd HH:mm:ss
* format.
* For example, if a new budget is approved after the proposed start time,
* the approved start time is the time of approval.
*
*
* optional string approved_start_date_time = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearApprovedStartDateTime() {
bitField0_ = (bitField0_ & ~0x00000010);
approvedStartDateTime_ = getDefaultInstance().getApprovedStartDateTime();
onChanged();
return this;
}
/**
*
* Output only. The approved start time of the account-level budget in yyyy-MM-dd HH:mm:ss
* format.
* For example, if a new budget is approved after the proposed start time,
* the approved start time is the time of approval.
*
*
* optional string approved_start_date_time = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for approvedStartDateTime to set.
* @return This builder for chaining.
*/
public Builder setApprovedStartDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000010;
approvedStartDateTime_ = value;
onChanged();
return this;
}
private long totalAdjustmentsMicros_ ;
/**
*
* Output only. The total adjustments amount.
* An example of an adjustment is courtesy credits.
*
*
* int64 total_adjustments_micros = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The totalAdjustmentsMicros.
*/
@java.lang.Override
public long getTotalAdjustmentsMicros() {
return totalAdjustmentsMicros_;
}
/**
*
* Output only. The total adjustments amount.
* An example of an adjustment is courtesy credits.
*
*
* int64 total_adjustments_micros = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The totalAdjustmentsMicros to set.
* @return This builder for chaining.
*/
public Builder setTotalAdjustmentsMicros(long value) {
totalAdjustmentsMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The total adjustments amount.
* An example of an adjustment is courtesy credits.
*
*
* int64 total_adjustments_micros = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearTotalAdjustmentsMicros() {
totalAdjustmentsMicros_ = 0L;
onChanged();
return this;
}
private long amountServedMicros_ ;
/**
*
* Output only. The value of Ads that have been served, in micros.
* This includes overdelivery costs, in which case a credit might be
* automatically applied to the budget (see total_adjustments_micros).
*
*
* int64 amount_served_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The amountServedMicros.
*/
@java.lang.Override
public long getAmountServedMicros() {
return amountServedMicros_;
}
/**
*
* Output only. The value of Ads that have been served, in micros.
* This includes overdelivery costs, in which case a credit might be
* automatically applied to the budget (see total_adjustments_micros).
*
*
* int64 amount_served_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The amountServedMicros to set.
* @return This builder for chaining.
*/
public Builder setAmountServedMicros(long value) {
amountServedMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The value of Ads that have been served, in micros.
* This includes overdelivery costs, in which case a credit might be
* automatically applied to the budget (see total_adjustments_micros).
*
*
* int64 amount_served_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearAmountServedMicros() {
amountServedMicros_ = 0L;
onChanged();
return this;
}
private java.lang.Object purchaseOrderNumber_ = "";
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the purchaseOrderNumber field is set.
*/
public boolean hasPurchaseOrderNumber() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The purchaseOrderNumber.
*/
public java.lang.String getPurchaseOrderNumber() {
java.lang.Object ref = purchaseOrderNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
purchaseOrderNumber_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for purchaseOrderNumber.
*/
public com.google.protobuf.ByteString
getPurchaseOrderNumberBytes() {
java.lang.Object ref = purchaseOrderNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
purchaseOrderNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The purchaseOrderNumber to set.
* @return This builder for chaining.
*/
public Builder setPurchaseOrderNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
purchaseOrderNumber_ = value;
onChanged();
return this;
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearPurchaseOrderNumber() {
bitField0_ = (bitField0_ & ~0x00000020);
purchaseOrderNumber_ = getDefaultInstance().getPurchaseOrderNumber();
onChanged();
return this;
}
/**
*
* Output only. A purchase order number is a value that helps users reference this budget
* in their monthly invoices.
*
*
* optional string purchase_order_number = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for purchaseOrderNumber to set.
* @return This builder for chaining.
*/
public Builder setPurchaseOrderNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000020;
purchaseOrderNumber_ = value;
onChanged();
return this;
}
private java.lang.Object notes_ = "";
/**
*
* Output only. Notes associated with the budget.
*
*
* optional string notes = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the notes field is set.
*/
public boolean hasNotes() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. Notes associated with the budget.
*
*
* optional string notes = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The notes.
*/
public java.lang.String getNotes() {
java.lang.Object ref = notes_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
notes_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. Notes associated with the budget.
*
*
* optional string notes = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for notes.
*/
public com.google.protobuf.ByteString
getNotesBytes() {
java.lang.Object ref = notes_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
notes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. Notes associated with the budget.
*
*
* optional string notes = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The notes to set.
* @return This builder for chaining.
*/
public Builder setNotes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
notes_ = value;
onChanged();
return this;
}
/**
*
* Output only. Notes associated with the budget.
*
*
* optional string notes = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearNotes() {
bitField0_ = (bitField0_ & ~0x00000040);
notes_ = getDefaultInstance().getNotes();
onChanged();
return this;
}
/**
*
* Output only. Notes associated with the budget.
*
*
* optional string notes = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for notes to set.
* @return This builder for chaining.
*/
public Builder setNotesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000040;
notes_ = value;
onChanged();
return this;
}
private com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pendingProposal_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal, com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.Builder, com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposalOrBuilder> pendingProposalBuilder_;
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the pendingProposal field is set.
*/
public boolean hasPendingProposal() {
return pendingProposalBuilder_ != null || pendingProposal_ != null;
}
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The pendingProposal.
*/
public com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal getPendingProposal() {
if (pendingProposalBuilder_ == null) {
return pendingProposal_ == null ? com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.getDefaultInstance() : pendingProposal_;
} else {
return pendingProposalBuilder_.getMessage();
}
}
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder setPendingProposal(com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal value) {
if (pendingProposalBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pendingProposal_ = value;
onChanged();
} else {
pendingProposalBuilder_.setMessage(value);
}
return this;
}
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder setPendingProposal(
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.Builder builderForValue) {
if (pendingProposalBuilder_ == null) {
pendingProposal_ = builderForValue.build();
onChanged();
} else {
pendingProposalBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder mergePendingProposal(com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal value) {
if (pendingProposalBuilder_ == null) {
if (pendingProposal_ != null) {
pendingProposal_ =
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.newBuilder(pendingProposal_).mergeFrom(value).buildPartial();
} else {
pendingProposal_ = value;
}
onChanged();
} else {
pendingProposalBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder clearPendingProposal() {
if (pendingProposalBuilder_ == null) {
pendingProposal_ = null;
onChanged();
} else {
pendingProposal_ = null;
pendingProposalBuilder_ = null;
}
return this;
}
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.Builder getPendingProposalBuilder() {
onChanged();
return getPendingProposalFieldBuilder().getBuilder();
}
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposalOrBuilder getPendingProposalOrBuilder() {
if (pendingProposalBuilder_ != null) {
return pendingProposalBuilder_.getMessageOrBuilder();
} else {
return pendingProposal_ == null ?
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.getDefaultInstance() : pendingProposal_;
}
}
/**
*
* Output only. The pending proposal to modify this budget, if applicable.
*
*
* .google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal pending_proposal = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal, com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.Builder, com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposalOrBuilder>
getPendingProposalFieldBuilder() {
if (pendingProposalBuilder_ == null) {
pendingProposalBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal, com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposal.Builder, com.google.ads.googleads.v10.resources.AccountBudget.PendingAccountBudgetProposalOrBuilder>(
getPendingProposal(),
getParentForChildren(),
isClean());
pendingProposal_ = null;
}
return pendingProposalBuilder_;
}
/**
*
* Output only. The proposed end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string proposed_end_date_time = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the proposedEndDateTime field is set.
*/
@java.lang.Override
public boolean hasProposedEndDateTime() {
return proposedEndTimeCase_ == 28;
}
/**
*
* Output only. The proposed end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string proposed_end_date_time = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposedEndDateTime.
*/
@java.lang.Override
public java.lang.String getProposedEndDateTime() {
java.lang.Object ref = "";
if (proposedEndTimeCase_ == 28) {
ref = proposedEndTime_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (proposedEndTimeCase_ == 28) {
proposedEndTime_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The proposed end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string proposed_end_date_time = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for proposedEndDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProposedEndDateTimeBytes() {
java.lang.Object ref = "";
if (proposedEndTimeCase_ == 28) {
ref = proposedEndTime_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (proposedEndTimeCase_ == 28) {
proposedEndTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The proposed end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string proposed_end_date_time = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The proposedEndDateTime to set.
* @return This builder for chaining.
*/
public Builder setProposedEndDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
proposedEndTimeCase_ = 28;
proposedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The proposed end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string proposed_end_date_time = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearProposedEndDateTime() {
if (proposedEndTimeCase_ == 28) {
proposedEndTimeCase_ = 0;
proposedEndTime_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The proposed end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string proposed_end_date_time = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for proposedEndDateTime to set.
* @return This builder for chaining.
*/
public Builder setProposedEndDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
proposedEndTimeCase_ = 28;
proposedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The proposed end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the proposedEndTimeType field is set.
*/
@java.lang.Override
public boolean hasProposedEndTimeType() {
return proposedEndTimeCase_ == 9;
}
/**
*
* Output only. The proposed end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for proposedEndTimeType.
*/
@java.lang.Override
public int getProposedEndTimeTypeValue() {
if (proposedEndTimeCase_ == 9) {
return ((java.lang.Integer) proposedEndTime_).intValue();
}
return 0;
}
/**
*
* Output only. The proposed end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for proposedEndTimeType to set.
* @return This builder for chaining.
*/
public Builder setProposedEndTimeTypeValue(int value) {
proposedEndTimeCase_ = 9;
proposedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The proposed end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposedEndTimeType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getProposedEndTimeType() {
if (proposedEndTimeCase_ == 9) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) proposedEndTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
/**
*
* Output only. The proposed end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The proposedEndTimeType to set.
* @return This builder for chaining.
*/
public Builder setProposedEndTimeType(com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType value) {
if (value == null) {
throw new NullPointerException();
}
proposedEndTimeCase_ = 9;
proposedEndTime_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The proposed end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearProposedEndTimeType() {
if (proposedEndTimeCase_ == 9) {
proposedEndTimeCase_ = 0;
proposedEndTime_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The approved end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string approved_end_date_time = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedEndDateTime field is set.
*/
@java.lang.Override
public boolean hasApprovedEndDateTime() {
return approvedEndTimeCase_ == 29;
}
/**
*
* Output only. The approved end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string approved_end_date_time = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedEndDateTime.
*/
@java.lang.Override
public java.lang.String getApprovedEndDateTime() {
java.lang.Object ref = "";
if (approvedEndTimeCase_ == 29) {
ref = approvedEndTime_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (approvedEndTimeCase_ == 29) {
approvedEndTime_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The approved end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string approved_end_date_time = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for approvedEndDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getApprovedEndDateTimeBytes() {
java.lang.Object ref = "";
if (approvedEndTimeCase_ == 29) {
ref = approvedEndTime_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (approvedEndTimeCase_ == 29) {
approvedEndTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The approved end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string approved_end_date_time = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The approvedEndDateTime to set.
* @return This builder for chaining.
*/
public Builder setApprovedEndDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
approvedEndTimeCase_ = 29;
approvedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string approved_end_date_time = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearApprovedEndDateTime() {
if (approvedEndTimeCase_ == 29) {
approvedEndTimeCase_ = 0;
approvedEndTime_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The approved end time in yyyy-MM-dd HH:mm:ss format.
*
*
* string approved_end_date_time = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for approvedEndDateTime to set.
* @return This builder for chaining.
*/
public Builder setApprovedEndDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
approvedEndTimeCase_ = 29;
approvedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedEndTimeType field is set.
*/
@java.lang.Override
public boolean hasApprovedEndTimeType() {
return approvedEndTimeCase_ == 11;
}
/**
*
* Output only. The approved end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for approvedEndTimeType.
*/
@java.lang.Override
public int getApprovedEndTimeTypeValue() {
if (approvedEndTimeCase_ == 11) {
return ((java.lang.Integer) approvedEndTime_).intValue();
}
return 0;
}
/**
*
* Output only. The approved end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for approvedEndTimeType to set.
* @return This builder for chaining.
*/
public Builder setApprovedEndTimeTypeValue(int value) {
approvedEndTimeCase_ = 11;
approvedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedEndTimeType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getApprovedEndTimeType() {
if (approvedEndTimeCase_ == 11) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) approvedEndTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
/**
*
* Output only. The approved end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The approvedEndTimeType to set.
* @return This builder for chaining.
*/
public Builder setApprovedEndTimeType(com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType value) {
if (value == null) {
throw new NullPointerException();
}
approvedEndTimeCase_ = 11;
approvedEndTime_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The approved end time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearApprovedEndTimeType() {
if (approvedEndTimeCase_ == 11) {
approvedEndTimeCase_ = 0;
approvedEndTime_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the proposedSpendingLimitMicros field is set.
*/
public boolean hasProposedSpendingLimitMicros() {
return proposedSpendingLimitCase_ == 30;
}
/**
*
* Output only. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposedSpendingLimitMicros.
*/
public long getProposedSpendingLimitMicros() {
if (proposedSpendingLimitCase_ == 30) {
return (java.lang.Long) proposedSpendingLimit_;
}
return 0L;
}
/**
*
* Output only. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The proposedSpendingLimitMicros to set.
* @return This builder for chaining.
*/
public Builder setProposedSpendingLimitMicros(long value) {
proposedSpendingLimitCase_ = 30;
proposedSpendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Output only. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearProposedSpendingLimitMicros() {
if (proposedSpendingLimitCase_ == 30) {
proposedSpendingLimitCase_ = 0;
proposedSpendingLimit_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the proposedSpendingLimitType field is set.
*/
@java.lang.Override
public boolean hasProposedSpendingLimitType() {
return proposedSpendingLimitCase_ == 13;
}
/**
*
* Output only. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for proposedSpendingLimitType.
*/
@java.lang.Override
public int getProposedSpendingLimitTypeValue() {
if (proposedSpendingLimitCase_ == 13) {
return ((java.lang.Integer) proposedSpendingLimit_).intValue();
}
return 0;
}
/**
*
* Output only. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for proposedSpendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setProposedSpendingLimitTypeValue(int value) {
proposedSpendingLimitCase_ = 13;
proposedSpendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Output only. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The proposedSpendingLimitType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getProposedSpendingLimitType() {
if (proposedSpendingLimitCase_ == 13) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) proposedSpendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
/**
*
* Output only. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The proposedSpendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setProposedSpendingLimitType(com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType value) {
if (value == null) {
throw new NullPointerException();
}
proposedSpendingLimitCase_ = 13;
proposedSpendingLimit_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearProposedSpendingLimitType() {
if (proposedSpendingLimitCase_ == 13) {
proposedSpendingLimitCase_ = 0;
proposedSpendingLimit_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit. This will only be populated if the proposed spending limit
* is finite, and will always be greater than or equal to the
* proposed spending limit.
*
*
* int64 approved_spending_limit_micros = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedSpendingLimitMicros field is set.
*/
public boolean hasApprovedSpendingLimitMicros() {
return approvedSpendingLimitCase_ == 31;
}
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit. This will only be populated if the proposed spending limit
* is finite, and will always be greater than or equal to the
* proposed spending limit.
*
*
* int64 approved_spending_limit_micros = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedSpendingLimitMicros.
*/
public long getApprovedSpendingLimitMicros() {
if (approvedSpendingLimitCase_ == 31) {
return (java.lang.Long) approvedSpendingLimit_;
}
return 0L;
}
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit. This will only be populated if the proposed spending limit
* is finite, and will always be greater than or equal to the
* proposed spending limit.
*
*
* int64 approved_spending_limit_micros = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The approvedSpendingLimitMicros to set.
* @return This builder for chaining.
*/
public Builder setApprovedSpendingLimitMicros(long value) {
approvedSpendingLimitCase_ = 31;
approvedSpendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit. This will only be populated if the proposed spending limit
* is finite, and will always be greater than or equal to the
* proposed spending limit.
*
*
* int64 approved_spending_limit_micros = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearApprovedSpendingLimitMicros() {
if (approvedSpendingLimitCase_ == 31) {
approvedSpendingLimitCase_ = 0;
approvedSpendingLimit_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the approved spending limit is
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedSpendingLimitType field is set.
*/
@java.lang.Override
public boolean hasApprovedSpendingLimitType() {
return approvedSpendingLimitCase_ == 15;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the approved spending limit is
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for approvedSpendingLimitType.
*/
@java.lang.Override
public int getApprovedSpendingLimitTypeValue() {
if (approvedSpendingLimitCase_ == 15) {
return ((java.lang.Integer) approvedSpendingLimit_).intValue();
}
return 0;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the approved spending limit is
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for approvedSpendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setApprovedSpendingLimitTypeValue(int value) {
approvedSpendingLimitCase_ = 15;
approvedSpendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the approved spending limit is
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedSpendingLimitType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getApprovedSpendingLimitType() {
if (approvedSpendingLimitCase_ == 15) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) approvedSpendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the approved spending limit is
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The approvedSpendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setApprovedSpendingLimitType(com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType value) {
if (value == null) {
throw new NullPointerException();
}
approvedSpendingLimitCase_ = 15;
approvedSpendingLimit_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the approved spending limit is
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearApprovedSpendingLimitType() {
if (approvedSpendingLimitCase_ == 15) {
approvedSpendingLimitCase_ = 0;
approvedSpendingLimit_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The adjusted spending limit in micros. One million is equivalent to
* one unit.
* If the approved spending limit is finite, the adjusted
* spending limit may vary depending on the types of adjustments applied
* to this budget, if applicable.
* The different kinds of adjustments are described here:
* https://support.google.com/google-ads/answer/1704323
* For example, a debit adjustment reduces how much the account is
* allowed to spend.
*
*
* int64 adjusted_spending_limit_micros = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the adjustedSpendingLimitMicros field is set.
*/
public boolean hasAdjustedSpendingLimitMicros() {
return adjustedSpendingLimitCase_ == 32;
}
/**
*
* Output only. The adjusted spending limit in micros. One million is equivalent to
* one unit.
* If the approved spending limit is finite, the adjusted
* spending limit may vary depending on the types of adjustments applied
* to this budget, if applicable.
* The different kinds of adjustments are described here:
* https://support.google.com/google-ads/answer/1704323
* For example, a debit adjustment reduces how much the account is
* allowed to spend.
*
*
* int64 adjusted_spending_limit_micros = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The adjustedSpendingLimitMicros.
*/
public long getAdjustedSpendingLimitMicros() {
if (adjustedSpendingLimitCase_ == 32) {
return (java.lang.Long) adjustedSpendingLimit_;
}
return 0L;
}
/**
*
* Output only. The adjusted spending limit in micros. One million is equivalent to
* one unit.
* If the approved spending limit is finite, the adjusted
* spending limit may vary depending on the types of adjustments applied
* to this budget, if applicable.
* The different kinds of adjustments are described here:
* https://support.google.com/google-ads/answer/1704323
* For example, a debit adjustment reduces how much the account is
* allowed to spend.
*
*
* int64 adjusted_spending_limit_micros = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The adjustedSpendingLimitMicros to set.
* @return This builder for chaining.
*/
public Builder setAdjustedSpendingLimitMicros(long value) {
adjustedSpendingLimitCase_ = 32;
adjustedSpendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Output only. The adjusted spending limit in micros. One million is equivalent to
* one unit.
* If the approved spending limit is finite, the adjusted
* spending limit may vary depending on the types of adjustments applied
* to this budget, if applicable.
* The different kinds of adjustments are described here:
* https://support.google.com/google-ads/answer/1704323
* For example, a debit adjustment reduces how much the account is
* allowed to spend.
*
*
* int64 adjusted_spending_limit_micros = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearAdjustedSpendingLimitMicros() {
if (adjustedSpendingLimitCase_ == 32) {
adjustedSpendingLimitCase_ = 0;
adjustedSpendingLimit_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The adjusted spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the adjusted spending limit is
* INFINITE, which is guaranteed to be true if the approved spending limit
* is INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType adjusted_spending_limit_type = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the adjustedSpendingLimitType field is set.
*/
@java.lang.Override
public boolean hasAdjustedSpendingLimitType() {
return adjustedSpendingLimitCase_ == 17;
}
/**
*
* Output only. The adjusted spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the adjusted spending limit is
* INFINITE, which is guaranteed to be true if the approved spending limit
* is INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType adjusted_spending_limit_type = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for adjustedSpendingLimitType.
*/
@java.lang.Override
public int getAdjustedSpendingLimitTypeValue() {
if (adjustedSpendingLimitCase_ == 17) {
return ((java.lang.Integer) adjustedSpendingLimit_).intValue();
}
return 0;
}
/**
*
* Output only. The adjusted spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the adjusted spending limit is
* INFINITE, which is guaranteed to be true if the approved spending limit
* is INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType adjusted_spending_limit_type = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for adjustedSpendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setAdjustedSpendingLimitTypeValue(int value) {
adjustedSpendingLimitCase_ = 17;
adjustedSpendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Output only. The adjusted spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the adjusted spending limit is
* INFINITE, which is guaranteed to be true if the approved spending limit
* is INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType adjusted_spending_limit_type = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The adjustedSpendingLimitType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getAdjustedSpendingLimitType() {
if (adjustedSpendingLimitCase_ == 17) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) adjustedSpendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
/**
*
* Output only. The adjusted spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the adjusted spending limit is
* INFINITE, which is guaranteed to be true if the approved spending limit
* is INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType adjusted_spending_limit_type = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The adjustedSpendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setAdjustedSpendingLimitType(com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType value) {
if (value == null) {
throw new NullPointerException();
}
adjustedSpendingLimitCase_ = 17;
adjustedSpendingLimit_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The adjusted spending limit as a well-defined type, for example,
* INFINITE. This will only be populated if the adjusted spending limit is
* INFINITE, which is guaranteed to be true if the approved spending limit
* is INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType adjusted_spending_limit_type = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearAdjustedSpendingLimitType() {
if (adjustedSpendingLimitCase_ == 17) {
adjustedSpendingLimitCase_ = 0;
adjustedSpendingLimit_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v10.resources.AccountBudget)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v10.resources.AccountBudget)
private static final com.google.ads.googleads.v10.resources.AccountBudget DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v10.resources.AccountBudget();
}
public static com.google.ads.googleads.v10.resources.AccountBudget getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AccountBudget parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudget getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy