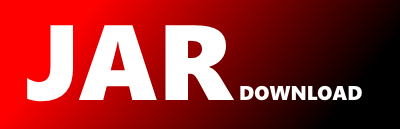
com.google.ads.googleads.v10.resources.AccountBudgetProposal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v10 Show documentation
Show all versions of google-ads-stubs-v10 Show documentation
Stubs for GAAPI version google-ads-stubs-v10
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v10/resources/account_budget_proposal.proto
package com.google.ads.googleads.v10.resources;
/**
*
* An account-level budget proposal.
* All fields prefixed with 'proposed' may not necessarily be applied directly.
* For example, proposed spending limits may be adjusted before their
* application. This is true if the 'proposed' field has an 'approved'
* counterpart, for example, spending limits.
* Note that the proposal type (proposal_type) changes which fields are
* required and which must remain empty.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.AccountBudgetProposal}
*/
public final class AccountBudgetProposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v10.resources.AccountBudgetProposal)
AccountBudgetProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use AccountBudgetProposal.newBuilder() to construct.
private AccountBudgetProposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AccountBudgetProposal() {
resourceName_ = "";
billingSetup_ = "";
accountBudget_ = "";
proposalType_ = 0;
status_ = 0;
proposedName_ = "";
approvedStartDateTime_ = "";
proposedPurchaseOrderNumber_ = "";
proposedNotes_ = "";
creationDateTime_ = "";
approvalDateTime_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AccountBudgetProposal();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.AccountBudgetProposalProto.internal_static_google_ads_googleads_v10_resources_AccountBudgetProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.AccountBudgetProposalProto.internal_static_google_ads_googleads_v10_resources_AccountBudgetProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.AccountBudgetProposal.class, com.google.ads.googleads.v10.resources.AccountBudgetProposal.Builder.class);
}
private int bitField0_;
private int proposedStartTimeCase_ = 0;
private java.lang.Object proposedStartTime_;
public enum ProposedStartTimeCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
PROPOSED_START_DATE_TIME(29),
PROPOSED_START_TIME_TYPE(7),
PROPOSEDSTARTTIME_NOT_SET(0);
private final int value;
private ProposedStartTimeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProposedStartTimeCase valueOf(int value) {
return forNumber(value);
}
public static ProposedStartTimeCase forNumber(int value) {
switch (value) {
case 29: return PROPOSED_START_DATE_TIME;
case 7: return PROPOSED_START_TIME_TYPE;
case 0: return PROPOSEDSTARTTIME_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ProposedStartTimeCase
getProposedStartTimeCase() {
return ProposedStartTimeCase.forNumber(
proposedStartTimeCase_);
}
private int proposedEndTimeCase_ = 0;
private java.lang.Object proposedEndTime_;
public enum ProposedEndTimeCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
PROPOSED_END_DATE_TIME(31),
PROPOSED_END_TIME_TYPE(9),
PROPOSEDENDTIME_NOT_SET(0);
private final int value;
private ProposedEndTimeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProposedEndTimeCase valueOf(int value) {
return forNumber(value);
}
public static ProposedEndTimeCase forNumber(int value) {
switch (value) {
case 31: return PROPOSED_END_DATE_TIME;
case 9: return PROPOSED_END_TIME_TYPE;
case 0: return PROPOSEDENDTIME_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ProposedEndTimeCase
getProposedEndTimeCase() {
return ProposedEndTimeCase.forNumber(
proposedEndTimeCase_);
}
private int approvedEndTimeCase_ = 0;
private java.lang.Object approvedEndTime_;
public enum ApprovedEndTimeCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
APPROVED_END_DATE_TIME(32),
APPROVED_END_TIME_TYPE(22),
APPROVEDENDTIME_NOT_SET(0);
private final int value;
private ApprovedEndTimeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ApprovedEndTimeCase valueOf(int value) {
return forNumber(value);
}
public static ApprovedEndTimeCase forNumber(int value) {
switch (value) {
case 32: return APPROVED_END_DATE_TIME;
case 22: return APPROVED_END_TIME_TYPE;
case 0: return APPROVEDENDTIME_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ApprovedEndTimeCase
getApprovedEndTimeCase() {
return ApprovedEndTimeCase.forNumber(
approvedEndTimeCase_);
}
private int proposedSpendingLimitCase_ = 0;
private java.lang.Object proposedSpendingLimit_;
public enum ProposedSpendingLimitCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
PROPOSED_SPENDING_LIMIT_MICROS(33),
PROPOSED_SPENDING_LIMIT_TYPE(11),
PROPOSEDSPENDINGLIMIT_NOT_SET(0);
private final int value;
private ProposedSpendingLimitCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProposedSpendingLimitCase valueOf(int value) {
return forNumber(value);
}
public static ProposedSpendingLimitCase forNumber(int value) {
switch (value) {
case 33: return PROPOSED_SPENDING_LIMIT_MICROS;
case 11: return PROPOSED_SPENDING_LIMIT_TYPE;
case 0: return PROPOSEDSPENDINGLIMIT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ProposedSpendingLimitCase
getProposedSpendingLimitCase() {
return ProposedSpendingLimitCase.forNumber(
proposedSpendingLimitCase_);
}
private int approvedSpendingLimitCase_ = 0;
private java.lang.Object approvedSpendingLimit_;
public enum ApprovedSpendingLimitCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
APPROVED_SPENDING_LIMIT_MICROS(34),
APPROVED_SPENDING_LIMIT_TYPE(24),
APPROVEDSPENDINGLIMIT_NOT_SET(0);
private final int value;
private ApprovedSpendingLimitCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ApprovedSpendingLimitCase valueOf(int value) {
return forNumber(value);
}
public static ApprovedSpendingLimitCase forNumber(int value) {
switch (value) {
case 34: return APPROVED_SPENDING_LIMIT_MICROS;
case 24: return APPROVED_SPENDING_LIMIT_TYPE;
case 0: return APPROVEDSPENDINGLIMIT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ApprovedSpendingLimitCase
getApprovedSpendingLimitCase() {
return ApprovedSpendingLimitCase.forNumber(
approvedSpendingLimitCase_);
}
public static final int RESOURCE_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object resourceName_;
/**
*
* Immutable. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
@java.lang.Override
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
}
}
/**
*
* Immutable. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 25;
private long id_;
/**
*
* Output only. The ID of the proposal.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The ID of the proposal.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int BILLING_SETUP_FIELD_NUMBER = 26;
private volatile java.lang.Object billingSetup_;
/**
*
* Immutable. The resource name of the billing setup associated with this proposal.
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return Whether the billingSetup field is set.
*/
@java.lang.Override
public boolean hasBillingSetup() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Immutable. The resource name of the billing setup associated with this proposal.
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The billingSetup.
*/
@java.lang.Override
public java.lang.String getBillingSetup() {
java.lang.Object ref = billingSetup_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
billingSetup_ = s;
return s;
}
}
/**
*
* Immutable. The resource name of the billing setup associated with this proposal.
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for billingSetup.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getBillingSetupBytes() {
java.lang.Object ref = billingSetup_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
billingSetup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACCOUNT_BUDGET_FIELD_NUMBER = 27;
private volatile java.lang.Object accountBudget_;
/**
*
* Immutable. The resource name of the account-level budget associated with this
* proposal.
*
*
* optional string account_budget = 27 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return Whether the accountBudget field is set.
*/
@java.lang.Override
public boolean hasAccountBudget() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Immutable. The resource name of the account-level budget associated with this
* proposal.
*
*
* optional string account_budget = 27 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The accountBudget.
*/
@java.lang.Override
public java.lang.String getAccountBudget() {
java.lang.Object ref = accountBudget_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountBudget_ = s;
return s;
}
}
/**
*
* Immutable. The resource name of the account-level budget associated with this
* proposal.
*
*
* optional string account_budget = 27 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for accountBudget.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAccountBudgetBytes() {
java.lang.Object ref = accountBudget_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountBudget_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROPOSAL_TYPE_FIELD_NUMBER = 4;
private int proposalType_;
/**
*
* Immutable. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for proposalType.
*/
@java.lang.Override public int getProposalTypeValue() {
return proposalType_;
}
/**
*
* Immutable. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposalType.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType getProposalType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType result = com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.valueOf(proposalType_);
return result == null ? com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.UNRECOGNIZED : result;
}
public static final int STATUS_FIELD_NUMBER = 15;
private int status_;
/**
*
* Output only. The status of this proposal.
* When a new proposal is created, the status defaults to PENDING.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Output only. The status of this proposal.
* When a new proposal is created, the status defaults to PENDING.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The status.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus getStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus result = com.google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus.valueOf(status_);
return result == null ? com.google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus.UNRECOGNIZED : result;
}
public static final int PROPOSED_NAME_FIELD_NUMBER = 28;
private volatile java.lang.Object proposedName_;
/**
*
* Immutable. The name to assign to the account-level budget.
*
*
* optional string proposed_name = 28 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedName field is set.
*/
@java.lang.Override
public boolean hasProposedName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Immutable. The name to assign to the account-level budget.
*
*
* optional string proposed_name = 28 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedName.
*/
@java.lang.Override
public java.lang.String getProposedName() {
java.lang.Object ref = proposedName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
proposedName_ = s;
return s;
}
}
/**
*
* Immutable. The name to assign to the account-level budget.
*
*
* optional string proposed_name = 28 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for proposedName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProposedNameBytes() {
java.lang.Object ref = proposedName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
proposedName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPROVED_START_DATE_TIME_FIELD_NUMBER = 30;
private volatile java.lang.Object approvedStartDateTime_;
/**
*
* Output only. The approved start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* optional string approved_start_date_time = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedStartDateTime field is set.
*/
@java.lang.Override
public boolean hasApprovedStartDateTime() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The approved start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* optional string approved_start_date_time = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedStartDateTime.
*/
@java.lang.Override
public java.lang.String getApprovedStartDateTime() {
java.lang.Object ref = approvedStartDateTime_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
approvedStartDateTime_ = s;
return s;
}
}
/**
*
* Output only. The approved start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* optional string approved_start_date_time = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for approvedStartDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getApprovedStartDateTimeBytes() {
java.lang.Object ref = approvedStartDateTime_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
approvedStartDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROPOSED_PURCHASE_ORDER_NUMBER_FIELD_NUMBER = 35;
private volatile java.lang.Object proposedPurchaseOrderNumber_;
/**
*
* Immutable. A purchase order number is a value that enables the user to help them
* reference this budget in their monthly invoices.
*
*
* optional string proposed_purchase_order_number = 35 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedPurchaseOrderNumber field is set.
*/
@java.lang.Override
public boolean hasProposedPurchaseOrderNumber() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Immutable. A purchase order number is a value that enables the user to help them
* reference this budget in their monthly invoices.
*
*
* optional string proposed_purchase_order_number = 35 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedPurchaseOrderNumber.
*/
@java.lang.Override
public java.lang.String getProposedPurchaseOrderNumber() {
java.lang.Object ref = proposedPurchaseOrderNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
proposedPurchaseOrderNumber_ = s;
return s;
}
}
/**
*
* Immutable. A purchase order number is a value that enables the user to help them
* reference this budget in their monthly invoices.
*
*
* optional string proposed_purchase_order_number = 35 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for proposedPurchaseOrderNumber.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProposedPurchaseOrderNumberBytes() {
java.lang.Object ref = proposedPurchaseOrderNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
proposedPurchaseOrderNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROPOSED_NOTES_FIELD_NUMBER = 36;
private volatile java.lang.Object proposedNotes_;
/**
*
* Immutable. Notes associated with this budget.
*
*
* optional string proposed_notes = 36 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedNotes field is set.
*/
@java.lang.Override
public boolean hasProposedNotes() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Immutable. Notes associated with this budget.
*
*
* optional string proposed_notes = 36 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedNotes.
*/
@java.lang.Override
public java.lang.String getProposedNotes() {
java.lang.Object ref = proposedNotes_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
proposedNotes_ = s;
return s;
}
}
/**
*
* Immutable. Notes associated with this budget.
*
*
* optional string proposed_notes = 36 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for proposedNotes.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProposedNotesBytes() {
java.lang.Object ref = proposedNotes_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
proposedNotes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CREATION_DATE_TIME_FIELD_NUMBER = 37;
private volatile java.lang.Object creationDateTime_;
/**
*
* Output only. The date time when this account-level budget proposal was created, which is
* not the same as its approval date time, if applicable.
*
*
* optional string creation_date_time = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the creationDateTime field is set.
*/
@java.lang.Override
public boolean hasCreationDateTime() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Output only. The date time when this account-level budget proposal was created, which is
* not the same as its approval date time, if applicable.
*
*
* optional string creation_date_time = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The creationDateTime.
*/
@java.lang.Override
public java.lang.String getCreationDateTime() {
java.lang.Object ref = creationDateTime_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationDateTime_ = s;
return s;
}
}
/**
*
* Output only. The date time when this account-level budget proposal was created, which is
* not the same as its approval date time, if applicable.
*
*
* optional string creation_date_time = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for creationDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCreationDateTimeBytes() {
java.lang.Object ref = creationDateTime_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
creationDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPROVAL_DATE_TIME_FIELD_NUMBER = 38;
private volatile java.lang.Object approvalDateTime_;
/**
*
* Output only. The date time when this account-level budget was approved, if applicable.
*
*
* optional string approval_date_time = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvalDateTime field is set.
*/
@java.lang.Override
public boolean hasApprovalDateTime() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Output only. The date time when this account-level budget was approved, if applicable.
*
*
* optional string approval_date_time = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvalDateTime.
*/
@java.lang.Override
public java.lang.String getApprovalDateTime() {
java.lang.Object ref = approvalDateTime_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
approvalDateTime_ = s;
return s;
}
}
/**
*
* Output only. The date time when this account-level budget was approved, if applicable.
*
*
* optional string approval_date_time = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for approvalDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getApprovalDateTimeBytes() {
java.lang.Object ref = approvalDateTime_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
approvalDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROPOSED_START_DATE_TIME_FIELD_NUMBER = 29;
/**
*
* Immutable. The proposed start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_start_date_time = 29 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedStartDateTime field is set.
*/
public boolean hasProposedStartDateTime() {
return proposedStartTimeCase_ == 29;
}
/**
*
* Immutable. The proposed start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_start_date_time = 29 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedStartDateTime.
*/
public java.lang.String getProposedStartDateTime() {
java.lang.Object ref = "";
if (proposedStartTimeCase_ == 29) {
ref = proposedStartTime_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (proposedStartTimeCase_ == 29) {
proposedStartTime_ = s;
}
return s;
}
}
/**
*
* Immutable. The proposed start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_start_date_time = 29 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for proposedStartDateTime.
*/
public com.google.protobuf.ByteString
getProposedStartDateTimeBytes() {
java.lang.Object ref = "";
if (proposedStartTimeCase_ == 29) {
ref = proposedStartTime_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (proposedStartTimeCase_ == 29) {
proposedStartTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROPOSED_START_TIME_TYPE_FIELD_NUMBER = 7;
/**
*
* Immutable. The proposed start date time as a well-defined type, for example, NOW.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_start_time_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedStartTimeType field is set.
*/
public boolean hasProposedStartTimeType() {
return proposedStartTimeCase_ == 7;
}
/**
*
* Immutable. The proposed start date time as a well-defined type, for example, NOW.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_start_time_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for proposedStartTimeType.
*/
public int getProposedStartTimeTypeValue() {
if (proposedStartTimeCase_ == 7) {
return (java.lang.Integer) proposedStartTime_;
}
return 0;
}
/**
*
* Immutable. The proposed start date time as a well-defined type, for example, NOW.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_start_time_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedStartTimeType.
*/
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getProposedStartTimeType() {
if (proposedStartTimeCase_ == 7) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) proposedStartTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
public static final int PROPOSED_END_DATE_TIME_FIELD_NUMBER = 31;
/**
*
* Immutable. The proposed end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_end_date_time = 31 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedEndDateTime field is set.
*/
public boolean hasProposedEndDateTime() {
return proposedEndTimeCase_ == 31;
}
/**
*
* Immutable. The proposed end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_end_date_time = 31 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedEndDateTime.
*/
public java.lang.String getProposedEndDateTime() {
java.lang.Object ref = "";
if (proposedEndTimeCase_ == 31) {
ref = proposedEndTime_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (proposedEndTimeCase_ == 31) {
proposedEndTime_ = s;
}
return s;
}
}
/**
*
* Immutable. The proposed end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_end_date_time = 31 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for proposedEndDateTime.
*/
public com.google.protobuf.ByteString
getProposedEndDateTimeBytes() {
java.lang.Object ref = "";
if (proposedEndTimeCase_ == 31) {
ref = proposedEndTime_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (proposedEndTimeCase_ == 31) {
proposedEndTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROPOSED_END_TIME_TYPE_FIELD_NUMBER = 9;
/**
*
* Immutable. The proposed end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedEndTimeType field is set.
*/
public boolean hasProposedEndTimeType() {
return proposedEndTimeCase_ == 9;
}
/**
*
* Immutable. The proposed end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for proposedEndTimeType.
*/
public int getProposedEndTimeTypeValue() {
if (proposedEndTimeCase_ == 9) {
return (java.lang.Integer) proposedEndTime_;
}
return 0;
}
/**
*
* Immutable. The proposed end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedEndTimeType.
*/
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getProposedEndTimeType() {
if (proposedEndTimeCase_ == 9) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) proposedEndTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
public static final int APPROVED_END_DATE_TIME_FIELD_NUMBER = 32;
/**
*
* Output only. The approved end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string approved_end_date_time = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedEndDateTime field is set.
*/
public boolean hasApprovedEndDateTime() {
return approvedEndTimeCase_ == 32;
}
/**
*
* Output only. The approved end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string approved_end_date_time = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedEndDateTime.
*/
public java.lang.String getApprovedEndDateTime() {
java.lang.Object ref = "";
if (approvedEndTimeCase_ == 32) {
ref = approvedEndTime_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (approvedEndTimeCase_ == 32) {
approvedEndTime_ = s;
}
return s;
}
}
/**
*
* Output only. The approved end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string approved_end_date_time = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for approvedEndDateTime.
*/
public com.google.protobuf.ByteString
getApprovedEndDateTimeBytes() {
java.lang.Object ref = "";
if (approvedEndTimeCase_ == 32) {
ref = approvedEndTime_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (approvedEndTimeCase_ == 32) {
approvedEndTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPROVED_END_TIME_TYPE_FIELD_NUMBER = 22;
/**
*
* Output only. The approved end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedEndTimeType field is set.
*/
public boolean hasApprovedEndTimeType() {
return approvedEndTimeCase_ == 22;
}
/**
*
* Output only. The approved end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for approvedEndTimeType.
*/
public int getApprovedEndTimeTypeValue() {
if (approvedEndTimeCase_ == 22) {
return (java.lang.Integer) approvedEndTime_;
}
return 0;
}
/**
*
* Output only. The approved end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedEndTimeType.
*/
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getApprovedEndTimeType() {
if (approvedEndTimeCase_ == 22) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) approvedEndTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
public static final int PROPOSED_SPENDING_LIMIT_MICROS_FIELD_NUMBER = 33;
/**
*
* Immutable. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 33 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedSpendingLimitMicros field is set.
*/
@java.lang.Override
public boolean hasProposedSpendingLimitMicros() {
return proposedSpendingLimitCase_ == 33;
}
/**
*
* Immutable. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 33 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedSpendingLimitMicros.
*/
@java.lang.Override
public long getProposedSpendingLimitMicros() {
if (proposedSpendingLimitCase_ == 33) {
return (java.lang.Long) proposedSpendingLimit_;
}
return 0L;
}
public static final int PROPOSED_SPENDING_LIMIT_TYPE_FIELD_NUMBER = 11;
/**
*
* Immutable. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedSpendingLimitType field is set.
*/
public boolean hasProposedSpendingLimitType() {
return proposedSpendingLimitCase_ == 11;
}
/**
*
* Immutable. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for proposedSpendingLimitType.
*/
public int getProposedSpendingLimitTypeValue() {
if (proposedSpendingLimitCase_ == 11) {
return (java.lang.Integer) proposedSpendingLimit_;
}
return 0;
}
/**
*
* Immutable. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedSpendingLimitType.
*/
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getProposedSpendingLimitType() {
if (proposedSpendingLimitCase_ == 11) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) proposedSpendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
public static final int APPROVED_SPENDING_LIMIT_MICROS_FIELD_NUMBER = 34;
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 approved_spending_limit_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedSpendingLimitMicros field is set.
*/
@java.lang.Override
public boolean hasApprovedSpendingLimitMicros() {
return approvedSpendingLimitCase_ == 34;
}
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 approved_spending_limit_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedSpendingLimitMicros.
*/
@java.lang.Override
public long getApprovedSpendingLimitMicros() {
if (approvedSpendingLimitCase_ == 34) {
return (java.lang.Long) approvedSpendingLimit_;
}
return 0L;
}
public static final int APPROVED_SPENDING_LIMIT_TYPE_FIELD_NUMBER = 24;
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedSpendingLimitType field is set.
*/
public boolean hasApprovedSpendingLimitType() {
return approvedSpendingLimitCase_ == 24;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for approvedSpendingLimitType.
*/
public int getApprovedSpendingLimitTypeValue() {
if (approvedSpendingLimitCase_ == 24) {
return (java.lang.Integer) approvedSpendingLimit_;
}
return 0;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedSpendingLimitType.
*/
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getApprovedSpendingLimitType() {
if (approvedSpendingLimitCase_ == 24) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) approvedSpendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, resourceName_);
}
if (proposalType_ != com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.UNSPECIFIED.getNumber()) {
output.writeEnum(4, proposalType_);
}
if (proposedStartTimeCase_ == 7) {
output.writeEnum(7, ((java.lang.Integer) proposedStartTime_));
}
if (proposedEndTimeCase_ == 9) {
output.writeEnum(9, ((java.lang.Integer) proposedEndTime_));
}
if (proposedSpendingLimitCase_ == 11) {
output.writeEnum(11, ((java.lang.Integer) proposedSpendingLimit_));
}
if (status_ != com.google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus.UNSPECIFIED.getNumber()) {
output.writeEnum(15, status_);
}
if (approvedEndTimeCase_ == 22) {
output.writeEnum(22, ((java.lang.Integer) approvedEndTime_));
}
if (approvedSpendingLimitCase_ == 24) {
output.writeEnum(24, ((java.lang.Integer) approvedSpendingLimit_));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(25, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 26, billingSetup_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 27, accountBudget_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 28, proposedName_);
}
if (proposedStartTimeCase_ == 29) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 29, proposedStartTime_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30, approvedStartDateTime_);
}
if (proposedEndTimeCase_ == 31) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 31, proposedEndTime_);
}
if (approvedEndTimeCase_ == 32) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 32, approvedEndTime_);
}
if (proposedSpendingLimitCase_ == 33) {
output.writeInt64(
33, (long)((java.lang.Long) proposedSpendingLimit_));
}
if (approvedSpendingLimitCase_ == 34) {
output.writeInt64(
34, (long)((java.lang.Long) approvedSpendingLimit_));
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 35, proposedPurchaseOrderNumber_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 36, proposedNotes_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 37, creationDateTime_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 38, approvalDateTime_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, resourceName_);
}
if (proposalType_ != com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, proposalType_);
}
if (proposedStartTimeCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, ((java.lang.Integer) proposedStartTime_));
}
if (proposedEndTimeCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(9, ((java.lang.Integer) proposedEndTime_));
}
if (proposedSpendingLimitCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(11, ((java.lang.Integer) proposedSpendingLimit_));
}
if (status_ != com.google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(15, status_);
}
if (approvedEndTimeCase_ == 22) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(22, ((java.lang.Integer) approvedEndTime_));
}
if (approvedSpendingLimitCase_ == 24) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(24, ((java.lang.Integer) approvedSpendingLimit_));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(25, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(26, billingSetup_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(27, accountBudget_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(28, proposedName_);
}
if (proposedStartTimeCase_ == 29) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(29, proposedStartTime_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(30, approvedStartDateTime_);
}
if (proposedEndTimeCase_ == 31) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(31, proposedEndTime_);
}
if (approvedEndTimeCase_ == 32) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(32, approvedEndTime_);
}
if (proposedSpendingLimitCase_ == 33) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
33, (long)((java.lang.Long) proposedSpendingLimit_));
}
if (approvedSpendingLimitCase_ == 34) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
34, (long)((java.lang.Long) approvedSpendingLimit_));
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(35, proposedPurchaseOrderNumber_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(36, proposedNotes_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(37, creationDateTime_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(38, approvalDateTime_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v10.resources.AccountBudgetProposal)) {
return super.equals(obj);
}
com.google.ads.googleads.v10.resources.AccountBudgetProposal other = (com.google.ads.googleads.v10.resources.AccountBudgetProposal) obj;
if (!getResourceName()
.equals(other.getResourceName())) return false;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId()
!= other.getId()) return false;
}
if (hasBillingSetup() != other.hasBillingSetup()) return false;
if (hasBillingSetup()) {
if (!getBillingSetup()
.equals(other.getBillingSetup())) return false;
}
if (hasAccountBudget() != other.hasAccountBudget()) return false;
if (hasAccountBudget()) {
if (!getAccountBudget()
.equals(other.getAccountBudget())) return false;
}
if (proposalType_ != other.proposalType_) return false;
if (status_ != other.status_) return false;
if (hasProposedName() != other.hasProposedName()) return false;
if (hasProposedName()) {
if (!getProposedName()
.equals(other.getProposedName())) return false;
}
if (hasApprovedStartDateTime() != other.hasApprovedStartDateTime()) return false;
if (hasApprovedStartDateTime()) {
if (!getApprovedStartDateTime()
.equals(other.getApprovedStartDateTime())) return false;
}
if (hasProposedPurchaseOrderNumber() != other.hasProposedPurchaseOrderNumber()) return false;
if (hasProposedPurchaseOrderNumber()) {
if (!getProposedPurchaseOrderNumber()
.equals(other.getProposedPurchaseOrderNumber())) return false;
}
if (hasProposedNotes() != other.hasProposedNotes()) return false;
if (hasProposedNotes()) {
if (!getProposedNotes()
.equals(other.getProposedNotes())) return false;
}
if (hasCreationDateTime() != other.hasCreationDateTime()) return false;
if (hasCreationDateTime()) {
if (!getCreationDateTime()
.equals(other.getCreationDateTime())) return false;
}
if (hasApprovalDateTime() != other.hasApprovalDateTime()) return false;
if (hasApprovalDateTime()) {
if (!getApprovalDateTime()
.equals(other.getApprovalDateTime())) return false;
}
if (!getProposedStartTimeCase().equals(other.getProposedStartTimeCase())) return false;
switch (proposedStartTimeCase_) {
case 29:
if (!getProposedStartDateTime()
.equals(other.getProposedStartDateTime())) return false;
break;
case 7:
if (getProposedStartTimeTypeValue()
!= other.getProposedStartTimeTypeValue()) return false;
break;
case 0:
default:
}
if (!getProposedEndTimeCase().equals(other.getProposedEndTimeCase())) return false;
switch (proposedEndTimeCase_) {
case 31:
if (!getProposedEndDateTime()
.equals(other.getProposedEndDateTime())) return false;
break;
case 9:
if (getProposedEndTimeTypeValue()
!= other.getProposedEndTimeTypeValue()) return false;
break;
case 0:
default:
}
if (!getApprovedEndTimeCase().equals(other.getApprovedEndTimeCase())) return false;
switch (approvedEndTimeCase_) {
case 32:
if (!getApprovedEndDateTime()
.equals(other.getApprovedEndDateTime())) return false;
break;
case 22:
if (getApprovedEndTimeTypeValue()
!= other.getApprovedEndTimeTypeValue()) return false;
break;
case 0:
default:
}
if (!getProposedSpendingLimitCase().equals(other.getProposedSpendingLimitCase())) return false;
switch (proposedSpendingLimitCase_) {
case 33:
if (getProposedSpendingLimitMicros()
!= other.getProposedSpendingLimitMicros()) return false;
break;
case 11:
if (getProposedSpendingLimitTypeValue()
!= other.getProposedSpendingLimitTypeValue()) return false;
break;
case 0:
default:
}
if (!getApprovedSpendingLimitCase().equals(other.getApprovedSpendingLimitCase())) return false;
switch (approvedSpendingLimitCase_) {
case 34:
if (getApprovedSpendingLimitMicros()
!= other.getApprovedSpendingLimitMicros()) return false;
break;
case 24:
if (getApprovedSpendingLimitTypeValue()
!= other.getApprovedSpendingLimitTypeValue()) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RESOURCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getResourceName().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getId());
}
if (hasBillingSetup()) {
hash = (37 * hash) + BILLING_SETUP_FIELD_NUMBER;
hash = (53 * hash) + getBillingSetup().hashCode();
}
if (hasAccountBudget()) {
hash = (37 * hash) + ACCOUNT_BUDGET_FIELD_NUMBER;
hash = (53 * hash) + getAccountBudget().hashCode();
}
hash = (37 * hash) + PROPOSAL_TYPE_FIELD_NUMBER;
hash = (53 * hash) + proposalType_;
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (hasProposedName()) {
hash = (37 * hash) + PROPOSED_NAME_FIELD_NUMBER;
hash = (53 * hash) + getProposedName().hashCode();
}
if (hasApprovedStartDateTime()) {
hash = (37 * hash) + APPROVED_START_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getApprovedStartDateTime().hashCode();
}
if (hasProposedPurchaseOrderNumber()) {
hash = (37 * hash) + PROPOSED_PURCHASE_ORDER_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getProposedPurchaseOrderNumber().hashCode();
}
if (hasProposedNotes()) {
hash = (37 * hash) + PROPOSED_NOTES_FIELD_NUMBER;
hash = (53 * hash) + getProposedNotes().hashCode();
}
if (hasCreationDateTime()) {
hash = (37 * hash) + CREATION_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getCreationDateTime().hashCode();
}
if (hasApprovalDateTime()) {
hash = (37 * hash) + APPROVAL_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getApprovalDateTime().hashCode();
}
switch (proposedStartTimeCase_) {
case 29:
hash = (37 * hash) + PROPOSED_START_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getProposedStartDateTime().hashCode();
break;
case 7:
hash = (37 * hash) + PROPOSED_START_TIME_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getProposedStartTimeTypeValue();
break;
case 0:
default:
}
switch (proposedEndTimeCase_) {
case 31:
hash = (37 * hash) + PROPOSED_END_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getProposedEndDateTime().hashCode();
break;
case 9:
hash = (37 * hash) + PROPOSED_END_TIME_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getProposedEndTimeTypeValue();
break;
case 0:
default:
}
switch (approvedEndTimeCase_) {
case 32:
hash = (37 * hash) + APPROVED_END_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getApprovedEndDateTime().hashCode();
break;
case 22:
hash = (37 * hash) + APPROVED_END_TIME_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getApprovedEndTimeTypeValue();
break;
case 0:
default:
}
switch (proposedSpendingLimitCase_) {
case 33:
hash = (37 * hash) + PROPOSED_SPENDING_LIMIT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getProposedSpendingLimitMicros());
break;
case 11:
hash = (37 * hash) + PROPOSED_SPENDING_LIMIT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getProposedSpendingLimitTypeValue();
break;
case 0:
default:
}
switch (approvedSpendingLimitCase_) {
case 34:
hash = (37 * hash) + APPROVED_SPENDING_LIMIT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getApprovedSpendingLimitMicros());
break;
case 24:
hash = (37 * hash) + APPROVED_SPENDING_LIMIT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getApprovedSpendingLimitTypeValue();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v10.resources.AccountBudgetProposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An account-level budget proposal.
* All fields prefixed with 'proposed' may not necessarily be applied directly.
* For example, proposed spending limits may be adjusted before their
* application. This is true if the 'proposed' field has an 'approved'
* counterpart, for example, spending limits.
* Note that the proposal type (proposal_type) changes which fields are
* required and which must remain empty.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.AccountBudgetProposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v10.resources.AccountBudgetProposal)
com.google.ads.googleads.v10.resources.AccountBudgetProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.AccountBudgetProposalProto.internal_static_google_ads_googleads_v10_resources_AccountBudgetProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.AccountBudgetProposalProto.internal_static_google_ads_googleads_v10_resources_AccountBudgetProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.AccountBudgetProposal.class, com.google.ads.googleads.v10.resources.AccountBudgetProposal.Builder.class);
}
// Construct using com.google.ads.googleads.v10.resources.AccountBudgetProposal.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
resourceName_ = "";
id_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
billingSetup_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
accountBudget_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
proposalType_ = 0;
status_ = 0;
proposedName_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
approvedStartDateTime_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
proposedPurchaseOrderNumber_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
proposedNotes_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
creationDateTime_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
approvalDateTime_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
proposedStartTimeCase_ = 0;
proposedStartTime_ = null;
proposedEndTimeCase_ = 0;
proposedEndTime_ = null;
approvedEndTimeCase_ = 0;
approvedEndTime_ = null;
proposedSpendingLimitCase_ = 0;
proposedSpendingLimit_ = null;
approvedSpendingLimitCase_ = 0;
approvedSpendingLimit_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v10.resources.AccountBudgetProposalProto.internal_static_google_ads_googleads_v10_resources_AccountBudgetProposal_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudgetProposal getDefaultInstanceForType() {
return com.google.ads.googleads.v10.resources.AccountBudgetProposal.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudgetProposal build() {
com.google.ads.googleads.v10.resources.AccountBudgetProposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudgetProposal buildPartial() {
com.google.ads.googleads.v10.resources.AccountBudgetProposal result = new com.google.ads.googleads.v10.resources.AccountBudgetProposal(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.resourceName_ = resourceName_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.billingSetup_ = billingSetup_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.accountBudget_ = accountBudget_;
result.proposalType_ = proposalType_;
result.status_ = status_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.proposedName_ = proposedName_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.approvedStartDateTime_ = approvedStartDateTime_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.proposedPurchaseOrderNumber_ = proposedPurchaseOrderNumber_;
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000040;
}
result.proposedNotes_ = proposedNotes_;
if (((from_bitField0_ & 0x00000080) != 0)) {
to_bitField0_ |= 0x00000080;
}
result.creationDateTime_ = creationDateTime_;
if (((from_bitField0_ & 0x00000100) != 0)) {
to_bitField0_ |= 0x00000100;
}
result.approvalDateTime_ = approvalDateTime_;
if (proposedStartTimeCase_ == 29) {
result.proposedStartTime_ = proposedStartTime_;
}
if (proposedStartTimeCase_ == 7) {
result.proposedStartTime_ = proposedStartTime_;
}
if (proposedEndTimeCase_ == 31) {
result.proposedEndTime_ = proposedEndTime_;
}
if (proposedEndTimeCase_ == 9) {
result.proposedEndTime_ = proposedEndTime_;
}
if (approvedEndTimeCase_ == 32) {
result.approvedEndTime_ = approvedEndTime_;
}
if (approvedEndTimeCase_ == 22) {
result.approvedEndTime_ = approvedEndTime_;
}
if (proposedSpendingLimitCase_ == 33) {
result.proposedSpendingLimit_ = proposedSpendingLimit_;
}
if (proposedSpendingLimitCase_ == 11) {
result.proposedSpendingLimit_ = proposedSpendingLimit_;
}
if (approvedSpendingLimitCase_ == 34) {
result.approvedSpendingLimit_ = approvedSpendingLimit_;
}
if (approvedSpendingLimitCase_ == 24) {
result.approvedSpendingLimit_ = approvedSpendingLimit_;
}
result.bitField0_ = to_bitField0_;
result.proposedStartTimeCase_ = proposedStartTimeCase_;
result.proposedEndTimeCase_ = proposedEndTimeCase_;
result.approvedEndTimeCase_ = approvedEndTimeCase_;
result.proposedSpendingLimitCase_ = proposedSpendingLimitCase_;
result.approvedSpendingLimitCase_ = approvedSpendingLimitCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v10.resources.AccountBudgetProposal) {
return mergeFrom((com.google.ads.googleads.v10.resources.AccountBudgetProposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v10.resources.AccountBudgetProposal other) {
if (other == com.google.ads.googleads.v10.resources.AccountBudgetProposal.getDefaultInstance()) return this;
if (!other.getResourceName().isEmpty()) {
resourceName_ = other.resourceName_;
onChanged();
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasBillingSetup()) {
bitField0_ |= 0x00000002;
billingSetup_ = other.billingSetup_;
onChanged();
}
if (other.hasAccountBudget()) {
bitField0_ |= 0x00000004;
accountBudget_ = other.accountBudget_;
onChanged();
}
if (other.proposalType_ != 0) {
setProposalTypeValue(other.getProposalTypeValue());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.hasProposedName()) {
bitField0_ |= 0x00000008;
proposedName_ = other.proposedName_;
onChanged();
}
if (other.hasApprovedStartDateTime()) {
bitField0_ |= 0x00000010;
approvedStartDateTime_ = other.approvedStartDateTime_;
onChanged();
}
if (other.hasProposedPurchaseOrderNumber()) {
bitField0_ |= 0x00000020;
proposedPurchaseOrderNumber_ = other.proposedPurchaseOrderNumber_;
onChanged();
}
if (other.hasProposedNotes()) {
bitField0_ |= 0x00000040;
proposedNotes_ = other.proposedNotes_;
onChanged();
}
if (other.hasCreationDateTime()) {
bitField0_ |= 0x00000080;
creationDateTime_ = other.creationDateTime_;
onChanged();
}
if (other.hasApprovalDateTime()) {
bitField0_ |= 0x00000100;
approvalDateTime_ = other.approvalDateTime_;
onChanged();
}
switch (other.getProposedStartTimeCase()) {
case PROPOSED_START_DATE_TIME: {
proposedStartTimeCase_ = 29;
proposedStartTime_ = other.proposedStartTime_;
onChanged();
break;
}
case PROPOSED_START_TIME_TYPE: {
setProposedStartTimeTypeValue(other.getProposedStartTimeTypeValue());
break;
}
case PROPOSEDSTARTTIME_NOT_SET: {
break;
}
}
switch (other.getProposedEndTimeCase()) {
case PROPOSED_END_DATE_TIME: {
proposedEndTimeCase_ = 31;
proposedEndTime_ = other.proposedEndTime_;
onChanged();
break;
}
case PROPOSED_END_TIME_TYPE: {
setProposedEndTimeTypeValue(other.getProposedEndTimeTypeValue());
break;
}
case PROPOSEDENDTIME_NOT_SET: {
break;
}
}
switch (other.getApprovedEndTimeCase()) {
case APPROVED_END_DATE_TIME: {
approvedEndTimeCase_ = 32;
approvedEndTime_ = other.approvedEndTime_;
onChanged();
break;
}
case APPROVED_END_TIME_TYPE: {
setApprovedEndTimeTypeValue(other.getApprovedEndTimeTypeValue());
break;
}
case APPROVEDENDTIME_NOT_SET: {
break;
}
}
switch (other.getProposedSpendingLimitCase()) {
case PROPOSED_SPENDING_LIMIT_MICROS: {
setProposedSpendingLimitMicros(other.getProposedSpendingLimitMicros());
break;
}
case PROPOSED_SPENDING_LIMIT_TYPE: {
setProposedSpendingLimitTypeValue(other.getProposedSpendingLimitTypeValue());
break;
}
case PROPOSEDSPENDINGLIMIT_NOT_SET: {
break;
}
}
switch (other.getApprovedSpendingLimitCase()) {
case APPROVED_SPENDING_LIMIT_MICROS: {
setApprovedSpendingLimitMicros(other.getApprovedSpendingLimitMicros());
break;
}
case APPROVED_SPENDING_LIMIT_TYPE: {
setApprovedSpendingLimitTypeValue(other.getApprovedSpendingLimitTypeValue());
break;
}
case APPROVEDSPENDINGLIMIT_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
resourceName_ = input.readStringRequireUtf8();
break;
} // case 10
case 32: {
proposalType_ = input.readEnum();
break;
} // case 32
case 56: {
int rawValue = input.readEnum();
proposedStartTimeCase_ = 7;
proposedStartTime_ = rawValue;
break;
} // case 56
case 72: {
int rawValue = input.readEnum();
proposedEndTimeCase_ = 9;
proposedEndTime_ = rawValue;
break;
} // case 72
case 88: {
int rawValue = input.readEnum();
proposedSpendingLimitCase_ = 11;
proposedSpendingLimit_ = rawValue;
break;
} // case 88
case 120: {
status_ = input.readEnum();
break;
} // case 120
case 176: {
int rawValue = input.readEnum();
approvedEndTimeCase_ = 22;
approvedEndTime_ = rawValue;
break;
} // case 176
case 192: {
int rawValue = input.readEnum();
approvedSpendingLimitCase_ = 24;
approvedSpendingLimit_ = rawValue;
break;
} // case 192
case 200: {
id_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 200
case 210: {
billingSetup_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 210
case 218: {
accountBudget_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 218
case 226: {
proposedName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 226
case 234: {
java.lang.String s = input.readStringRequireUtf8();
proposedStartTimeCase_ = 29;
proposedStartTime_ = s;
break;
} // case 234
case 242: {
approvedStartDateTime_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 242
case 250: {
java.lang.String s = input.readStringRequireUtf8();
proposedEndTimeCase_ = 31;
proposedEndTime_ = s;
break;
} // case 250
case 258: {
java.lang.String s = input.readStringRequireUtf8();
approvedEndTimeCase_ = 32;
approvedEndTime_ = s;
break;
} // case 258
case 264: {
proposedSpendingLimit_ = input.readInt64();
proposedSpendingLimitCase_ = 33;
break;
} // case 264
case 272: {
approvedSpendingLimit_ = input.readInt64();
approvedSpendingLimitCase_ = 34;
break;
} // case 272
case 282: {
proposedPurchaseOrderNumber_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 282
case 290: {
proposedNotes_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 290
case 298: {
creationDateTime_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 298
case 306: {
approvalDateTime_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case 306
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int proposedStartTimeCase_ = 0;
private java.lang.Object proposedStartTime_;
public ProposedStartTimeCase
getProposedStartTimeCase() {
return ProposedStartTimeCase.forNumber(
proposedStartTimeCase_);
}
public Builder clearProposedStartTime() {
proposedStartTimeCase_ = 0;
proposedStartTime_ = null;
onChanged();
return this;
}
private int proposedEndTimeCase_ = 0;
private java.lang.Object proposedEndTime_;
public ProposedEndTimeCase
getProposedEndTimeCase() {
return ProposedEndTimeCase.forNumber(
proposedEndTimeCase_);
}
public Builder clearProposedEndTime() {
proposedEndTimeCase_ = 0;
proposedEndTime_ = null;
onChanged();
return this;
}
private int approvedEndTimeCase_ = 0;
private java.lang.Object approvedEndTime_;
public ApprovedEndTimeCase
getApprovedEndTimeCase() {
return ApprovedEndTimeCase.forNumber(
approvedEndTimeCase_);
}
public Builder clearApprovedEndTime() {
approvedEndTimeCase_ = 0;
approvedEndTime_ = null;
onChanged();
return this;
}
private int proposedSpendingLimitCase_ = 0;
private java.lang.Object proposedSpendingLimit_;
public ProposedSpendingLimitCase
getProposedSpendingLimitCase() {
return ProposedSpendingLimitCase.forNumber(
proposedSpendingLimitCase_);
}
public Builder clearProposedSpendingLimit() {
proposedSpendingLimitCase_ = 0;
proposedSpendingLimit_ = null;
onChanged();
return this;
}
private int approvedSpendingLimitCase_ = 0;
private java.lang.Object approvedSpendingLimit_;
public ApprovedSpendingLimitCase
getApprovedSpendingLimitCase() {
return ApprovedSpendingLimitCase.forNumber(
approvedSpendingLimitCase_);
}
public Builder clearApprovedSpendingLimit() {
approvedSpendingLimitCase_ = 0;
approvedSpendingLimit_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object resourceName_ = "";
/**
*
* Immutable. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resourceName_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearResourceName() {
resourceName_ = getDefaultInstance().getResourceName();
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the proposal.
* AccountBudgetProposal resource names have the form:
* `customers/{customer_id}/accountBudgetProposals/{account_budget_proposal_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The bytes for resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resourceName_ = value;
onChanged();
return this;
}
private long id_ ;
/**
*
* Output only. The ID of the proposal.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The ID of the proposal.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
*
* Output only. The ID of the proposal.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
*
* Output only. The ID of the proposal.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object billingSetup_ = "";
/**
*
* Immutable. The resource name of the billing setup associated with this proposal.
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return Whether the billingSetup field is set.
*/
public boolean hasBillingSetup() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Immutable. The resource name of the billing setup associated with this proposal.
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The billingSetup.
*/
public java.lang.String getBillingSetup() {
java.lang.Object ref = billingSetup_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
billingSetup_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The resource name of the billing setup associated with this proposal.
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for billingSetup.
*/
public com.google.protobuf.ByteString
getBillingSetupBytes() {
java.lang.Object ref = billingSetup_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
billingSetup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The resource name of the billing setup associated with this proposal.
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The billingSetup to set.
* @return This builder for chaining.
*/
public Builder setBillingSetup(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
billingSetup_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the billing setup associated with this proposal.
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearBillingSetup() {
bitField0_ = (bitField0_ & ~0x00000002);
billingSetup_ = getDefaultInstance().getBillingSetup();
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the billing setup associated with this proposal.
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The bytes for billingSetup to set.
* @return This builder for chaining.
*/
public Builder setBillingSetupBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000002;
billingSetup_ = value;
onChanged();
return this;
}
private java.lang.Object accountBudget_ = "";
/**
*
* Immutable. The resource name of the account-level budget associated with this
* proposal.
*
*
* optional string account_budget = 27 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return Whether the accountBudget field is set.
*/
public boolean hasAccountBudget() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Immutable. The resource name of the account-level budget associated with this
* proposal.
*
*
* optional string account_budget = 27 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The accountBudget.
*/
public java.lang.String getAccountBudget() {
java.lang.Object ref = accountBudget_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountBudget_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The resource name of the account-level budget associated with this
* proposal.
*
*
* optional string account_budget = 27 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for accountBudget.
*/
public com.google.protobuf.ByteString
getAccountBudgetBytes() {
java.lang.Object ref = accountBudget_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountBudget_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The resource name of the account-level budget associated with this
* proposal.
*
*
* optional string account_budget = 27 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The accountBudget to set.
* @return This builder for chaining.
*/
public Builder setAccountBudget(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
accountBudget_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the account-level budget associated with this
* proposal.
*
*
* optional string account_budget = 27 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearAccountBudget() {
bitField0_ = (bitField0_ & ~0x00000004);
accountBudget_ = getDefaultInstance().getAccountBudget();
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the account-level budget associated with this
* proposal.
*
*
* optional string account_budget = 27 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The bytes for accountBudget to set.
* @return This builder for chaining.
*/
public Builder setAccountBudgetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000004;
accountBudget_ = value;
onChanged();
return this;
}
private int proposalType_ = 0;
/**
*
* Immutable. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for proposalType.
*/
@java.lang.Override public int getProposalTypeValue() {
return proposalType_;
}
/**
*
* Immutable. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for proposalType to set.
* @return This builder for chaining.
*/
public Builder setProposalTypeValue(int value) {
proposalType_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposalType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType getProposalType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType result = com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.valueOf(proposalType_);
return result == null ? com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType.UNRECOGNIZED : result;
}
/**
*
* Immutable. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The proposalType to set.
* @return This builder for chaining.
*/
public Builder setProposalType(com.google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType value) {
if (value == null) {
throw new NullPointerException();
}
proposalType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. The type of this proposal, for example, END to end the budget associated
* with this proposal.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalTypeEnum.AccountBudgetProposalType proposal_type = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearProposalType() {
proposalType_ = 0;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Output only. The status of this proposal.
* When a new proposal is created, the status defaults to PENDING.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Output only. The status of this proposal.
* When a new proposal is created, the status defaults to PENDING.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Output only. The status of this proposal.
* When a new proposal is created, the status defaults to PENDING.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The status.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus getStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus result = com.google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus.valueOf(status_);
return result == null ? com.google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus.UNRECOGNIZED : result;
}
/**
*
* Output only. The status of this proposal.
* When a new proposal is created, the status defaults to PENDING.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The status of this proposal.
* When a new proposal is created, the status defaults to PENDING.
*
*
* .google.ads.googleads.v10.enums.AccountBudgetProposalStatusEnum.AccountBudgetProposalStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private java.lang.Object proposedName_ = "";
/**
*
* Immutable. The name to assign to the account-level budget.
*
*
* optional string proposed_name = 28 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedName field is set.
*/
public boolean hasProposedName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Immutable. The name to assign to the account-level budget.
*
*
* optional string proposed_name = 28 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedName.
*/
public java.lang.String getProposedName() {
java.lang.Object ref = proposedName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
proposedName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The name to assign to the account-level budget.
*
*
* optional string proposed_name = 28 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for proposedName.
*/
public com.google.protobuf.ByteString
getProposedNameBytes() {
java.lang.Object ref = proposedName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
proposedName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The name to assign to the account-level budget.
*
*
* optional string proposed_name = 28 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The proposedName to set.
* @return This builder for chaining.
*/
public Builder setProposedName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
proposedName_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The name to assign to the account-level budget.
*
*
* optional string proposed_name = 28 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearProposedName() {
bitField0_ = (bitField0_ & ~0x00000008);
proposedName_ = getDefaultInstance().getProposedName();
onChanged();
return this;
}
/**
*
* Immutable. The name to assign to the account-level budget.
*
*
* optional string proposed_name = 28 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The bytes for proposedName to set.
* @return This builder for chaining.
*/
public Builder setProposedNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000008;
proposedName_ = value;
onChanged();
return this;
}
private java.lang.Object approvedStartDateTime_ = "";
/**
*
* Output only. The approved start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* optional string approved_start_date_time = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedStartDateTime field is set.
*/
public boolean hasApprovedStartDateTime() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The approved start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* optional string approved_start_date_time = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedStartDateTime.
*/
public java.lang.String getApprovedStartDateTime() {
java.lang.Object ref = approvedStartDateTime_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
approvedStartDateTime_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The approved start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* optional string approved_start_date_time = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for approvedStartDateTime.
*/
public com.google.protobuf.ByteString
getApprovedStartDateTimeBytes() {
java.lang.Object ref = approvedStartDateTime_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
approvedStartDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The approved start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* optional string approved_start_date_time = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The approvedStartDateTime to set.
* @return This builder for chaining.
*/
public Builder setApprovedStartDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
approvedStartDateTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* optional string approved_start_date_time = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearApprovedStartDateTime() {
bitField0_ = (bitField0_ & ~0x00000010);
approvedStartDateTime_ = getDefaultInstance().getApprovedStartDateTime();
onChanged();
return this;
}
/**
*
* Output only. The approved start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* optional string approved_start_date_time = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for approvedStartDateTime to set.
* @return This builder for chaining.
*/
public Builder setApprovedStartDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000010;
approvedStartDateTime_ = value;
onChanged();
return this;
}
private java.lang.Object proposedPurchaseOrderNumber_ = "";
/**
*
* Immutable. A purchase order number is a value that enables the user to help them
* reference this budget in their monthly invoices.
*
*
* optional string proposed_purchase_order_number = 35 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedPurchaseOrderNumber field is set.
*/
public boolean hasProposedPurchaseOrderNumber() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Immutable. A purchase order number is a value that enables the user to help them
* reference this budget in their monthly invoices.
*
*
* optional string proposed_purchase_order_number = 35 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedPurchaseOrderNumber.
*/
public java.lang.String getProposedPurchaseOrderNumber() {
java.lang.Object ref = proposedPurchaseOrderNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
proposedPurchaseOrderNumber_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. A purchase order number is a value that enables the user to help them
* reference this budget in their monthly invoices.
*
*
* optional string proposed_purchase_order_number = 35 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for proposedPurchaseOrderNumber.
*/
public com.google.protobuf.ByteString
getProposedPurchaseOrderNumberBytes() {
java.lang.Object ref = proposedPurchaseOrderNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
proposedPurchaseOrderNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. A purchase order number is a value that enables the user to help them
* reference this budget in their monthly invoices.
*
*
* optional string proposed_purchase_order_number = 35 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The proposedPurchaseOrderNumber to set.
* @return This builder for chaining.
*/
public Builder setProposedPurchaseOrderNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
proposedPurchaseOrderNumber_ = value;
onChanged();
return this;
}
/**
*
* Immutable. A purchase order number is a value that enables the user to help them
* reference this budget in their monthly invoices.
*
*
* optional string proposed_purchase_order_number = 35 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearProposedPurchaseOrderNumber() {
bitField0_ = (bitField0_ & ~0x00000020);
proposedPurchaseOrderNumber_ = getDefaultInstance().getProposedPurchaseOrderNumber();
onChanged();
return this;
}
/**
*
* Immutable. A purchase order number is a value that enables the user to help them
* reference this budget in their monthly invoices.
*
*
* optional string proposed_purchase_order_number = 35 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The bytes for proposedPurchaseOrderNumber to set.
* @return This builder for chaining.
*/
public Builder setProposedPurchaseOrderNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000020;
proposedPurchaseOrderNumber_ = value;
onChanged();
return this;
}
private java.lang.Object proposedNotes_ = "";
/**
*
* Immutable. Notes associated with this budget.
*
*
* optional string proposed_notes = 36 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedNotes field is set.
*/
public boolean hasProposedNotes() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Immutable. Notes associated with this budget.
*
*
* optional string proposed_notes = 36 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedNotes.
*/
public java.lang.String getProposedNotes() {
java.lang.Object ref = proposedNotes_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
proposedNotes_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. Notes associated with this budget.
*
*
* optional string proposed_notes = 36 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for proposedNotes.
*/
public com.google.protobuf.ByteString
getProposedNotesBytes() {
java.lang.Object ref = proposedNotes_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
proposedNotes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. Notes associated with this budget.
*
*
* optional string proposed_notes = 36 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The proposedNotes to set.
* @return This builder for chaining.
*/
public Builder setProposedNotes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
proposedNotes_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Notes associated with this budget.
*
*
* optional string proposed_notes = 36 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearProposedNotes() {
bitField0_ = (bitField0_ & ~0x00000040);
proposedNotes_ = getDefaultInstance().getProposedNotes();
onChanged();
return this;
}
/**
*
* Immutable. Notes associated with this budget.
*
*
* optional string proposed_notes = 36 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The bytes for proposedNotes to set.
* @return This builder for chaining.
*/
public Builder setProposedNotesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000040;
proposedNotes_ = value;
onChanged();
return this;
}
private java.lang.Object creationDateTime_ = "";
/**
*
* Output only. The date time when this account-level budget proposal was created, which is
* not the same as its approval date time, if applicable.
*
*
* optional string creation_date_time = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the creationDateTime field is set.
*/
public boolean hasCreationDateTime() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Output only. The date time when this account-level budget proposal was created, which is
* not the same as its approval date time, if applicable.
*
*
* optional string creation_date_time = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The creationDateTime.
*/
public java.lang.String getCreationDateTime() {
java.lang.Object ref = creationDateTime_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationDateTime_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The date time when this account-level budget proposal was created, which is
* not the same as its approval date time, if applicable.
*
*
* optional string creation_date_time = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for creationDateTime.
*/
public com.google.protobuf.ByteString
getCreationDateTimeBytes() {
java.lang.Object ref = creationDateTime_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
creationDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The date time when this account-level budget proposal was created, which is
* not the same as its approval date time, if applicable.
*
*
* optional string creation_date_time = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The creationDateTime to set.
* @return This builder for chaining.
*/
public Builder setCreationDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
creationDateTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The date time when this account-level budget proposal was created, which is
* not the same as its approval date time, if applicable.
*
*
* optional string creation_date_time = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearCreationDateTime() {
bitField0_ = (bitField0_ & ~0x00000080);
creationDateTime_ = getDefaultInstance().getCreationDateTime();
onChanged();
return this;
}
/**
*
* Output only. The date time when this account-level budget proposal was created, which is
* not the same as its approval date time, if applicable.
*
*
* optional string creation_date_time = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for creationDateTime to set.
* @return This builder for chaining.
*/
public Builder setCreationDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000080;
creationDateTime_ = value;
onChanged();
return this;
}
private java.lang.Object approvalDateTime_ = "";
/**
*
* Output only. The date time when this account-level budget was approved, if applicable.
*
*
* optional string approval_date_time = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvalDateTime field is set.
*/
public boolean hasApprovalDateTime() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Output only. The date time when this account-level budget was approved, if applicable.
*
*
* optional string approval_date_time = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvalDateTime.
*/
public java.lang.String getApprovalDateTime() {
java.lang.Object ref = approvalDateTime_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
approvalDateTime_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The date time when this account-level budget was approved, if applicable.
*
*
* optional string approval_date_time = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for approvalDateTime.
*/
public com.google.protobuf.ByteString
getApprovalDateTimeBytes() {
java.lang.Object ref = approvalDateTime_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
approvalDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The date time when this account-level budget was approved, if applicable.
*
*
* optional string approval_date_time = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The approvalDateTime to set.
* @return This builder for chaining.
*/
public Builder setApprovalDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
approvalDateTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The date time when this account-level budget was approved, if applicable.
*
*
* optional string approval_date_time = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearApprovalDateTime() {
bitField0_ = (bitField0_ & ~0x00000100);
approvalDateTime_ = getDefaultInstance().getApprovalDateTime();
onChanged();
return this;
}
/**
*
* Output only. The date time when this account-level budget was approved, if applicable.
*
*
* optional string approval_date_time = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for approvalDateTime to set.
* @return This builder for chaining.
*/
public Builder setApprovalDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000100;
approvalDateTime_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The proposed start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_start_date_time = 29 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedStartDateTime field is set.
*/
@java.lang.Override
public boolean hasProposedStartDateTime() {
return proposedStartTimeCase_ == 29;
}
/**
*
* Immutable. The proposed start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_start_date_time = 29 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedStartDateTime.
*/
@java.lang.Override
public java.lang.String getProposedStartDateTime() {
java.lang.Object ref = "";
if (proposedStartTimeCase_ == 29) {
ref = proposedStartTime_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (proposedStartTimeCase_ == 29) {
proposedStartTime_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The proposed start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_start_date_time = 29 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for proposedStartDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProposedStartDateTimeBytes() {
java.lang.Object ref = "";
if (proposedStartTimeCase_ == 29) {
ref = proposedStartTime_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (proposedStartTimeCase_ == 29) {
proposedStartTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The proposed start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_start_date_time = 29 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The proposedStartDateTime to set.
* @return This builder for chaining.
*/
public Builder setProposedStartDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
proposedStartTimeCase_ = 29;
proposedStartTime_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The proposed start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_start_date_time = 29 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearProposedStartDateTime() {
if (proposedStartTimeCase_ == 29) {
proposedStartTimeCase_ = 0;
proposedStartTime_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. The proposed start date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_start_date_time = 29 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The bytes for proposedStartDateTime to set.
* @return This builder for chaining.
*/
public Builder setProposedStartDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
proposedStartTimeCase_ = 29;
proposedStartTime_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The proposed start date time as a well-defined type, for example, NOW.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_start_time_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedStartTimeType field is set.
*/
@java.lang.Override
public boolean hasProposedStartTimeType() {
return proposedStartTimeCase_ == 7;
}
/**
*
* Immutable. The proposed start date time as a well-defined type, for example, NOW.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_start_time_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for proposedStartTimeType.
*/
@java.lang.Override
public int getProposedStartTimeTypeValue() {
if (proposedStartTimeCase_ == 7) {
return ((java.lang.Integer) proposedStartTime_).intValue();
}
return 0;
}
/**
*
* Immutable. The proposed start date time as a well-defined type, for example, NOW.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_start_time_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for proposedStartTimeType to set.
* @return This builder for chaining.
*/
public Builder setProposedStartTimeTypeValue(int value) {
proposedStartTimeCase_ = 7;
proposedStartTime_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The proposed start date time as a well-defined type, for example, NOW.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_start_time_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedStartTimeType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getProposedStartTimeType() {
if (proposedStartTimeCase_ == 7) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) proposedStartTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
/**
*
* Immutable. The proposed start date time as a well-defined type, for example, NOW.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_start_time_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The proposedStartTimeType to set.
* @return This builder for chaining.
*/
public Builder setProposedStartTimeType(com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType value) {
if (value == null) {
throw new NullPointerException();
}
proposedStartTimeCase_ = 7;
proposedStartTime_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. The proposed start date time as a well-defined type, for example, NOW.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_start_time_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearProposedStartTimeType() {
if (proposedStartTimeCase_ == 7) {
proposedStartTimeCase_ = 0;
proposedStartTime_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. The proposed end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_end_date_time = 31 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedEndDateTime field is set.
*/
@java.lang.Override
public boolean hasProposedEndDateTime() {
return proposedEndTimeCase_ == 31;
}
/**
*
* Immutable. The proposed end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_end_date_time = 31 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedEndDateTime.
*/
@java.lang.Override
public java.lang.String getProposedEndDateTime() {
java.lang.Object ref = "";
if (proposedEndTimeCase_ == 31) {
ref = proposedEndTime_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (proposedEndTimeCase_ == 31) {
proposedEndTime_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The proposed end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_end_date_time = 31 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for proposedEndDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProposedEndDateTimeBytes() {
java.lang.Object ref = "";
if (proposedEndTimeCase_ == 31) {
ref = proposedEndTime_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (proposedEndTimeCase_ == 31) {
proposedEndTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The proposed end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_end_date_time = 31 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The proposedEndDateTime to set.
* @return This builder for chaining.
*/
public Builder setProposedEndDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
proposedEndTimeCase_ = 31;
proposedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The proposed end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_end_date_time = 31 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearProposedEndDateTime() {
if (proposedEndTimeCase_ == 31) {
proposedEndTimeCase_ = 0;
proposedEndTime_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. The proposed end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string proposed_end_date_time = 31 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The bytes for proposedEndDateTime to set.
* @return This builder for chaining.
*/
public Builder setProposedEndDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
proposedEndTimeCase_ = 31;
proposedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The proposed end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedEndTimeType field is set.
*/
@java.lang.Override
public boolean hasProposedEndTimeType() {
return proposedEndTimeCase_ == 9;
}
/**
*
* Immutable. The proposed end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for proposedEndTimeType.
*/
@java.lang.Override
public int getProposedEndTimeTypeValue() {
if (proposedEndTimeCase_ == 9) {
return ((java.lang.Integer) proposedEndTime_).intValue();
}
return 0;
}
/**
*
* Immutable. The proposed end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for proposedEndTimeType to set.
* @return This builder for chaining.
*/
public Builder setProposedEndTimeTypeValue(int value) {
proposedEndTimeCase_ = 9;
proposedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The proposed end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedEndTimeType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getProposedEndTimeType() {
if (proposedEndTimeCase_ == 9) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) proposedEndTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
/**
*
* Immutable. The proposed end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The proposedEndTimeType to set.
* @return This builder for chaining.
*/
public Builder setProposedEndTimeType(com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType value) {
if (value == null) {
throw new NullPointerException();
}
proposedEndTimeCase_ = 9;
proposedEndTime_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. The proposed end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType proposed_end_time_type = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearProposedEndTimeType() {
if (proposedEndTimeCase_ == 9) {
proposedEndTimeCase_ = 0;
proposedEndTime_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The approved end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string approved_end_date_time = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedEndDateTime field is set.
*/
@java.lang.Override
public boolean hasApprovedEndDateTime() {
return approvedEndTimeCase_ == 32;
}
/**
*
* Output only. The approved end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string approved_end_date_time = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedEndDateTime.
*/
@java.lang.Override
public java.lang.String getApprovedEndDateTime() {
java.lang.Object ref = "";
if (approvedEndTimeCase_ == 32) {
ref = approvedEndTime_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (approvedEndTimeCase_ == 32) {
approvedEndTime_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The approved end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string approved_end_date_time = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for approvedEndDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getApprovedEndDateTimeBytes() {
java.lang.Object ref = "";
if (approvedEndTimeCase_ == 32) {
ref = approvedEndTime_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (approvedEndTimeCase_ == 32) {
approvedEndTime_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The approved end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string approved_end_date_time = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The approvedEndDateTime to set.
* @return This builder for chaining.
*/
public Builder setApprovedEndDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
approvedEndTimeCase_ = 32;
approvedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string approved_end_date_time = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearApprovedEndDateTime() {
if (approvedEndTimeCase_ == 32) {
approvedEndTimeCase_ = 0;
approvedEndTime_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The approved end date time in yyyy-mm-dd hh:mm:ss format.
*
*
* string approved_end_date_time = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for approvedEndDateTime to set.
* @return This builder for chaining.
*/
public Builder setApprovedEndDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
approvedEndTimeCase_ = 32;
approvedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedEndTimeType field is set.
*/
@java.lang.Override
public boolean hasApprovedEndTimeType() {
return approvedEndTimeCase_ == 22;
}
/**
*
* Output only. The approved end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for approvedEndTimeType.
*/
@java.lang.Override
public int getApprovedEndTimeTypeValue() {
if (approvedEndTimeCase_ == 22) {
return ((java.lang.Integer) approvedEndTime_).intValue();
}
return 0;
}
/**
*
* Output only. The approved end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for approvedEndTimeType to set.
* @return This builder for chaining.
*/
public Builder setApprovedEndTimeTypeValue(int value) {
approvedEndTimeCase_ = 22;
approvedEndTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedEndTimeType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType getApprovedEndTimeType() {
if (approvedEndTimeCase_ == 22) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType result = com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.valueOf(
(java.lang.Integer) approvedEndTime_);
return result == null ? com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType.UNSPECIFIED;
}
/**
*
* Output only. The approved end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The approvedEndTimeType to set.
* @return This builder for chaining.
*/
public Builder setApprovedEndTimeType(com.google.ads.googleads.v10.enums.TimeTypeEnum.TimeType value) {
if (value == null) {
throw new NullPointerException();
}
approvedEndTimeCase_ = 22;
approvedEndTime_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The approved end date time as a well-defined type, for example, FOREVER.
*
*
* .google.ads.googleads.v10.enums.TimeTypeEnum.TimeType approved_end_time_type = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearApprovedEndTimeType() {
if (approvedEndTimeCase_ == 22) {
approvedEndTimeCase_ = 0;
approvedEndTime_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 33 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedSpendingLimitMicros field is set.
*/
public boolean hasProposedSpendingLimitMicros() {
return proposedSpendingLimitCase_ == 33;
}
/**
*
* Immutable. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 33 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedSpendingLimitMicros.
*/
public long getProposedSpendingLimitMicros() {
if (proposedSpendingLimitCase_ == 33) {
return (java.lang.Long) proposedSpendingLimit_;
}
return 0L;
}
/**
*
* Immutable. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 33 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The proposedSpendingLimitMicros to set.
* @return This builder for chaining.
*/
public Builder setProposedSpendingLimitMicros(long value) {
proposedSpendingLimitCase_ = 33;
proposedSpendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The proposed spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 proposed_spending_limit_micros = 33 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearProposedSpendingLimitMicros() {
if (proposedSpendingLimitCase_ == 33) {
proposedSpendingLimitCase_ = 0;
proposedSpendingLimit_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the proposedSpendingLimitType field is set.
*/
@java.lang.Override
public boolean hasProposedSpendingLimitType() {
return proposedSpendingLimitCase_ == 11;
}
/**
*
* Immutable. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for proposedSpendingLimitType.
*/
@java.lang.Override
public int getProposedSpendingLimitTypeValue() {
if (proposedSpendingLimitCase_ == 11) {
return ((java.lang.Integer) proposedSpendingLimit_).intValue();
}
return 0;
}
/**
*
* Immutable. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for proposedSpendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setProposedSpendingLimitTypeValue(int value) {
proposedSpendingLimitCase_ = 11;
proposedSpendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return The proposedSpendingLimitType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getProposedSpendingLimitType() {
if (proposedSpendingLimitCase_ == 11) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) proposedSpendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
/**
*
* Immutable. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The proposedSpendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setProposedSpendingLimitType(com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType value) {
if (value == null) {
throw new NullPointerException();
}
proposedSpendingLimitCase_ = 11;
proposedSpendingLimit_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. The proposed spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType proposed_spending_limit_type = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearProposedSpendingLimitType() {
if (proposedSpendingLimitCase_ == 11) {
proposedSpendingLimitCase_ = 0;
proposedSpendingLimit_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 approved_spending_limit_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedSpendingLimitMicros field is set.
*/
public boolean hasApprovedSpendingLimitMicros() {
return approvedSpendingLimitCase_ == 34;
}
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 approved_spending_limit_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedSpendingLimitMicros.
*/
public long getApprovedSpendingLimitMicros() {
if (approvedSpendingLimitCase_ == 34) {
return (java.lang.Long) approvedSpendingLimit_;
}
return 0L;
}
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 approved_spending_limit_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The approvedSpendingLimitMicros to set.
* @return This builder for chaining.
*/
public Builder setApprovedSpendingLimitMicros(long value) {
approvedSpendingLimitCase_ = 34;
approvedSpendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved spending limit in micros. One million is equivalent to
* one unit.
*
*
* int64 approved_spending_limit_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearApprovedSpendingLimitMicros() {
if (approvedSpendingLimitCase_ == 34) {
approvedSpendingLimitCase_ = 0;
approvedSpendingLimit_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the approvedSpendingLimitType field is set.
*/
@java.lang.Override
public boolean hasApprovedSpendingLimitType() {
return approvedSpendingLimitCase_ == 24;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for approvedSpendingLimitType.
*/
@java.lang.Override
public int getApprovedSpendingLimitTypeValue() {
if (approvedSpendingLimitCase_ == 24) {
return ((java.lang.Integer) approvedSpendingLimit_).intValue();
}
return 0;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for approvedSpendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setApprovedSpendingLimitTypeValue(int value) {
approvedSpendingLimitCase_ = 24;
approvedSpendingLimit_ = value;
onChanged();
return this;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The approvedSpendingLimitType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType getApprovedSpendingLimitType() {
if (approvedSpendingLimitCase_ == 24) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType result = com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.valueOf(
(java.lang.Integer) approvedSpendingLimit_);
return result == null ? com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType.UNSPECIFIED;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The approvedSpendingLimitType to set.
* @return This builder for chaining.
*/
public Builder setApprovedSpendingLimitType(com.google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType value) {
if (value == null) {
throw new NullPointerException();
}
approvedSpendingLimitCase_ = 24;
approvedSpendingLimit_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The approved spending limit as a well-defined type, for example,
* INFINITE.
*
*
* .google.ads.googleads.v10.enums.SpendingLimitTypeEnum.SpendingLimitType approved_spending_limit_type = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearApprovedSpendingLimitType() {
if (approvedSpendingLimitCase_ == 24) {
approvedSpendingLimitCase_ = 0;
approvedSpendingLimit_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v10.resources.AccountBudgetProposal)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v10.resources.AccountBudgetProposal)
private static final com.google.ads.googleads.v10.resources.AccountBudgetProposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v10.resources.AccountBudgetProposal();
}
public static com.google.ads.googleads.v10.resources.AccountBudgetProposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AccountBudgetProposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AccountBudgetProposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy