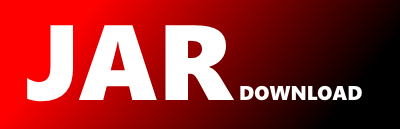
com.google.ads.googleads.v10.resources.AttributeFieldMapping Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v10 Show documentation
Show all versions of google-ads-stubs-v10 Show documentation
Stubs for GAAPI version google-ads-stubs-v10
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v10/resources/feed_mapping.proto
package com.google.ads.googleads.v10.resources;
/**
*
* Maps from feed attribute id to a placeholder or criterion field id.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.AttributeFieldMapping}
*/
public final class AttributeFieldMapping extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v10.resources.AttributeFieldMapping)
AttributeFieldMappingOrBuilder {
private static final long serialVersionUID = 0L;
// Use AttributeFieldMapping.newBuilder() to construct.
private AttributeFieldMapping(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AttributeFieldMapping() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AttributeFieldMapping();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.FeedMappingProto.internal_static_google_ads_googleads_v10_resources_AttributeFieldMapping_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.FeedMappingProto.internal_static_google_ads_googleads_v10_resources_AttributeFieldMapping_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.AttributeFieldMapping.class, com.google.ads.googleads.v10.resources.AttributeFieldMapping.Builder.class);
}
private int bitField0_;
private int fieldCase_ = 0;
private java.lang.Object field_;
public enum FieldCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
SITELINK_FIELD(3),
CALL_FIELD(4),
APP_FIELD(5),
LOCATION_FIELD(6),
AFFILIATE_LOCATION_FIELD(7),
CALLOUT_FIELD(8),
STRUCTURED_SNIPPET_FIELD(9),
MESSAGE_FIELD(10),
PRICE_FIELD(11),
PROMOTION_FIELD(12),
AD_CUSTOMIZER_FIELD(13),
DSA_PAGE_FEED_FIELD(14),
LOCATION_EXTENSION_TARGETING_FIELD(15),
EDUCATION_FIELD(16),
FLIGHT_FIELD(17),
CUSTOM_FIELD(18),
HOTEL_FIELD(19),
REAL_ESTATE_FIELD(20),
TRAVEL_FIELD(21),
LOCAL_FIELD(22),
JOB_FIELD(23),
IMAGE_FIELD(26),
FIELD_NOT_SET(0);
private final int value;
private FieldCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static FieldCase valueOf(int value) {
return forNumber(value);
}
public static FieldCase forNumber(int value) {
switch (value) {
case 3: return SITELINK_FIELD;
case 4: return CALL_FIELD;
case 5: return APP_FIELD;
case 6: return LOCATION_FIELD;
case 7: return AFFILIATE_LOCATION_FIELD;
case 8: return CALLOUT_FIELD;
case 9: return STRUCTURED_SNIPPET_FIELD;
case 10: return MESSAGE_FIELD;
case 11: return PRICE_FIELD;
case 12: return PROMOTION_FIELD;
case 13: return AD_CUSTOMIZER_FIELD;
case 14: return DSA_PAGE_FEED_FIELD;
case 15: return LOCATION_EXTENSION_TARGETING_FIELD;
case 16: return EDUCATION_FIELD;
case 17: return FLIGHT_FIELD;
case 18: return CUSTOM_FIELD;
case 19: return HOTEL_FIELD;
case 20: return REAL_ESTATE_FIELD;
case 21: return TRAVEL_FIELD;
case 22: return LOCAL_FIELD;
case 23: return JOB_FIELD;
case 26: return IMAGE_FIELD;
case 0: return FIELD_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public FieldCase
getFieldCase() {
return FieldCase.forNumber(
fieldCase_);
}
public static final int FEED_ATTRIBUTE_ID_FIELD_NUMBER = 24;
private long feedAttributeId_;
/**
*
* Immutable. Feed attribute from which to map.
*
*
* optional int64 feed_attribute_id = 24 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the feedAttributeId field is set.
*/
@java.lang.Override
public boolean hasFeedAttributeId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Immutable. Feed attribute from which to map.
*
*
* optional int64 feed_attribute_id = 24 [(.google.api.field_behavior) = IMMUTABLE];
* @return The feedAttributeId.
*/
@java.lang.Override
public long getFeedAttributeId() {
return feedAttributeId_;
}
public static final int FIELD_ID_FIELD_NUMBER = 25;
private long fieldId_;
/**
*
* Output only. The placeholder field ID. If a placeholder field enum is not published in
* the current API version, then this field will be populated and the field
* oneof will be empty.
* This field is read-only.
*
*
* optional int64 field_id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the fieldId field is set.
*/
@java.lang.Override
public boolean hasFieldId() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The placeholder field ID. If a placeholder field enum is not published in
* the current API version, then this field will be populated and the field
* oneof will be empty.
* This field is read-only.
*
*
* optional int64 field_id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The fieldId.
*/
@java.lang.Override
public long getFieldId() {
return fieldId_;
}
public static final int SITELINK_FIELD_FIELD_NUMBER = 3;
/**
*
* Immutable. Sitelink Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField sitelink_field = 3 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the sitelinkField field is set.
*/
public boolean hasSitelinkField() {
return fieldCase_ == 3;
}
/**
*
* Immutable. Sitelink Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField sitelink_field = 3 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for sitelinkField.
*/
public int getSitelinkFieldValue() {
if (fieldCase_ == 3) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Sitelink Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField sitelink_field = 3 [(.google.api.field_behavior) = IMMUTABLE];
* @return The sitelinkField.
*/
public com.google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField getSitelinkField() {
if (fieldCase_ == 3) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField result = com.google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField.UNSPECIFIED;
}
public static final int CALL_FIELD_FIELD_NUMBER = 4;
/**
*
* Immutable. Call Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField call_field = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the callField field is set.
*/
public boolean hasCallField() {
return fieldCase_ == 4;
}
/**
*
* Immutable. Call Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField call_field = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for callField.
*/
public int getCallFieldValue() {
if (fieldCase_ == 4) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Call Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField call_field = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return The callField.
*/
public com.google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField getCallField() {
if (fieldCase_ == 4) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField result = com.google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField.UNSPECIFIED;
}
public static final int APP_FIELD_FIELD_NUMBER = 5;
/**
*
* Immutable. App Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField app_field = 5 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the appField field is set.
*/
public boolean hasAppField() {
return fieldCase_ == 5;
}
/**
*
* Immutable. App Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField app_field = 5 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for appField.
*/
public int getAppFieldValue() {
if (fieldCase_ == 5) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. App Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField app_field = 5 [(.google.api.field_behavior) = IMMUTABLE];
* @return The appField.
*/
public com.google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField getAppField() {
if (fieldCase_ == 5) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField result = com.google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField.UNSPECIFIED;
}
public static final int LOCATION_FIELD_FIELD_NUMBER = 6;
/**
*
* Output only. Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField location_field = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the locationField field is set.
*/
public boolean hasLocationField() {
return fieldCase_ == 6;
}
/**
*
* Output only. Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField location_field = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for locationField.
*/
public int getLocationFieldValue() {
if (fieldCase_ == 6) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Output only. Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField location_field = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The locationField.
*/
public com.google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField getLocationField() {
if (fieldCase_ == 6) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField result = com.google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField.UNSPECIFIED;
}
public static final int AFFILIATE_LOCATION_FIELD_FIELD_NUMBER = 7;
/**
*
* Output only. Affiliate Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField affiliate_location_field = 7 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the affiliateLocationField field is set.
*/
public boolean hasAffiliateLocationField() {
return fieldCase_ == 7;
}
/**
*
* Output only. Affiliate Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField affiliate_location_field = 7 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for affiliateLocationField.
*/
public int getAffiliateLocationFieldValue() {
if (fieldCase_ == 7) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Output only. Affiliate Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField affiliate_location_field = 7 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The affiliateLocationField.
*/
public com.google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField getAffiliateLocationField() {
if (fieldCase_ == 7) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField result = com.google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField.UNSPECIFIED;
}
public static final int CALLOUT_FIELD_FIELD_NUMBER = 8;
/**
*
* Immutable. Callout Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField callout_field = 8 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the calloutField field is set.
*/
public boolean hasCalloutField() {
return fieldCase_ == 8;
}
/**
*
* Immutable. Callout Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField callout_field = 8 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for calloutField.
*/
public int getCalloutFieldValue() {
if (fieldCase_ == 8) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Callout Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField callout_field = 8 [(.google.api.field_behavior) = IMMUTABLE];
* @return The calloutField.
*/
public com.google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField getCalloutField() {
if (fieldCase_ == 8) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField result = com.google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField.UNSPECIFIED;
}
public static final int STRUCTURED_SNIPPET_FIELD_FIELD_NUMBER = 9;
/**
*
* Immutable. Structured Snippet Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField structured_snippet_field = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the structuredSnippetField field is set.
*/
public boolean hasStructuredSnippetField() {
return fieldCase_ == 9;
}
/**
*
* Immutable. Structured Snippet Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField structured_snippet_field = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for structuredSnippetField.
*/
public int getStructuredSnippetFieldValue() {
if (fieldCase_ == 9) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Structured Snippet Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField structured_snippet_field = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return The structuredSnippetField.
*/
public com.google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField getStructuredSnippetField() {
if (fieldCase_ == 9) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField result = com.google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField.UNSPECIFIED;
}
public static final int MESSAGE_FIELD_FIELD_NUMBER = 10;
/**
*
* Immutable. Message Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField message_field = 10 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the messageField field is set.
*/
public boolean hasMessageField() {
return fieldCase_ == 10;
}
/**
*
* Immutable. Message Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField message_field = 10 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for messageField.
*/
public int getMessageFieldValue() {
if (fieldCase_ == 10) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Message Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField message_field = 10 [(.google.api.field_behavior) = IMMUTABLE];
* @return The messageField.
*/
public com.google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField getMessageField() {
if (fieldCase_ == 10) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField result = com.google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField.UNSPECIFIED;
}
public static final int PRICE_FIELD_FIELD_NUMBER = 11;
/**
*
* Immutable. Price Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField price_field = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the priceField field is set.
*/
public boolean hasPriceField() {
return fieldCase_ == 11;
}
/**
*
* Immutable. Price Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField price_field = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for priceField.
*/
public int getPriceFieldValue() {
if (fieldCase_ == 11) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Price Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField price_field = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return The priceField.
*/
public com.google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField getPriceField() {
if (fieldCase_ == 11) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField result = com.google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField.UNSPECIFIED;
}
public static final int PROMOTION_FIELD_FIELD_NUMBER = 12;
/**
*
* Immutable. Promotion Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField promotion_field = 12 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the promotionField field is set.
*/
public boolean hasPromotionField() {
return fieldCase_ == 12;
}
/**
*
* Immutable. Promotion Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField promotion_field = 12 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for promotionField.
*/
public int getPromotionFieldValue() {
if (fieldCase_ == 12) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Promotion Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField promotion_field = 12 [(.google.api.field_behavior) = IMMUTABLE];
* @return The promotionField.
*/
public com.google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField getPromotionField() {
if (fieldCase_ == 12) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField result = com.google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField.UNSPECIFIED;
}
public static final int AD_CUSTOMIZER_FIELD_FIELD_NUMBER = 13;
/**
*
* Immutable. Ad Customizer Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField ad_customizer_field = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the adCustomizerField field is set.
*/
public boolean hasAdCustomizerField() {
return fieldCase_ == 13;
}
/**
*
* Immutable. Ad Customizer Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField ad_customizer_field = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for adCustomizerField.
*/
public int getAdCustomizerFieldValue() {
if (fieldCase_ == 13) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Ad Customizer Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField ad_customizer_field = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return The adCustomizerField.
*/
public com.google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField getAdCustomizerField() {
if (fieldCase_ == 13) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField result = com.google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField.UNSPECIFIED;
}
public static final int DSA_PAGE_FEED_FIELD_FIELD_NUMBER = 14;
/**
*
* Immutable. Dynamic Search Ad Page Feed Fields.
*
*
* .google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField dsa_page_feed_field = 14 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the dsaPageFeedField field is set.
*/
public boolean hasDsaPageFeedField() {
return fieldCase_ == 14;
}
/**
*
* Immutable. Dynamic Search Ad Page Feed Fields.
*
*
* .google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField dsa_page_feed_field = 14 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for dsaPageFeedField.
*/
public int getDsaPageFeedFieldValue() {
if (fieldCase_ == 14) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Dynamic Search Ad Page Feed Fields.
*
*
* .google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField dsa_page_feed_field = 14 [(.google.api.field_behavior) = IMMUTABLE];
* @return The dsaPageFeedField.
*/
public com.google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField getDsaPageFeedField() {
if (fieldCase_ == 14) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField result = com.google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField.UNSPECIFIED;
}
public static final int LOCATION_EXTENSION_TARGETING_FIELD_FIELD_NUMBER = 15;
/**
*
* Immutable. Location Target Fields.
*
*
* .google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField location_extension_targeting_field = 15 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the locationExtensionTargetingField field is set.
*/
public boolean hasLocationExtensionTargetingField() {
return fieldCase_ == 15;
}
/**
*
* Immutable. Location Target Fields.
*
*
* .google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField location_extension_targeting_field = 15 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for locationExtensionTargetingField.
*/
public int getLocationExtensionTargetingFieldValue() {
if (fieldCase_ == 15) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Location Target Fields.
*
*
* .google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField location_extension_targeting_field = 15 [(.google.api.field_behavior) = IMMUTABLE];
* @return The locationExtensionTargetingField.
*/
public com.google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField getLocationExtensionTargetingField() {
if (fieldCase_ == 15) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField result = com.google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField.UNSPECIFIED;
}
public static final int EDUCATION_FIELD_FIELD_NUMBER = 16;
/**
*
* Immutable. Education Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField education_field = 16 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the educationField field is set.
*/
public boolean hasEducationField() {
return fieldCase_ == 16;
}
/**
*
* Immutable. Education Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField education_field = 16 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for educationField.
*/
public int getEducationFieldValue() {
if (fieldCase_ == 16) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Education Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField education_field = 16 [(.google.api.field_behavior) = IMMUTABLE];
* @return The educationField.
*/
public com.google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField getEducationField() {
if (fieldCase_ == 16) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField result = com.google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField.UNSPECIFIED;
}
public static final int FLIGHT_FIELD_FIELD_NUMBER = 17;
/**
*
* Immutable. Flight Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField flight_field = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the flightField field is set.
*/
public boolean hasFlightField() {
return fieldCase_ == 17;
}
/**
*
* Immutable. Flight Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField flight_field = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for flightField.
*/
public int getFlightFieldValue() {
if (fieldCase_ == 17) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Flight Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField flight_field = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return The flightField.
*/
public com.google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField getFlightField() {
if (fieldCase_ == 17) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField result = com.google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField.UNSPECIFIED;
}
public static final int CUSTOM_FIELD_FIELD_NUMBER = 18;
/**
*
* Immutable. Custom Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField custom_field = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the customField field is set.
*/
public boolean hasCustomField() {
return fieldCase_ == 18;
}
/**
*
* Immutable. Custom Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField custom_field = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for customField.
*/
public int getCustomFieldValue() {
if (fieldCase_ == 18) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Custom Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField custom_field = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return The customField.
*/
public com.google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField getCustomField() {
if (fieldCase_ == 18) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField result = com.google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField.UNSPECIFIED;
}
public static final int HOTEL_FIELD_FIELD_NUMBER = 19;
/**
*
* Immutable. Hotel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField hotel_field = 19 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the hotelField field is set.
*/
public boolean hasHotelField() {
return fieldCase_ == 19;
}
/**
*
* Immutable. Hotel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField hotel_field = 19 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for hotelField.
*/
public int getHotelFieldValue() {
if (fieldCase_ == 19) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Hotel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField hotel_field = 19 [(.google.api.field_behavior) = IMMUTABLE];
* @return The hotelField.
*/
public com.google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField getHotelField() {
if (fieldCase_ == 19) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField result = com.google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField.UNSPECIFIED;
}
public static final int REAL_ESTATE_FIELD_FIELD_NUMBER = 20;
/**
*
* Immutable. Real Estate Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField real_estate_field = 20 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the realEstateField field is set.
*/
public boolean hasRealEstateField() {
return fieldCase_ == 20;
}
/**
*
* Immutable. Real Estate Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField real_estate_field = 20 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for realEstateField.
*/
public int getRealEstateFieldValue() {
if (fieldCase_ == 20) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Real Estate Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField real_estate_field = 20 [(.google.api.field_behavior) = IMMUTABLE];
* @return The realEstateField.
*/
public com.google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField getRealEstateField() {
if (fieldCase_ == 20) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField result = com.google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField.UNSPECIFIED;
}
public static final int TRAVEL_FIELD_FIELD_NUMBER = 21;
/**
*
* Immutable. Travel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField travel_field = 21 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the travelField field is set.
*/
public boolean hasTravelField() {
return fieldCase_ == 21;
}
/**
*
* Immutable. Travel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField travel_field = 21 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for travelField.
*/
public int getTravelFieldValue() {
if (fieldCase_ == 21) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Travel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField travel_field = 21 [(.google.api.field_behavior) = IMMUTABLE];
* @return The travelField.
*/
public com.google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField getTravelField() {
if (fieldCase_ == 21) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField result = com.google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField.UNSPECIFIED;
}
public static final int LOCAL_FIELD_FIELD_NUMBER = 22;
/**
*
* Immutable. Local Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField local_field = 22 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the localField field is set.
*/
public boolean hasLocalField() {
return fieldCase_ == 22;
}
/**
*
* Immutable. Local Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField local_field = 22 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for localField.
*/
public int getLocalFieldValue() {
if (fieldCase_ == 22) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Local Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField local_field = 22 [(.google.api.field_behavior) = IMMUTABLE];
* @return The localField.
*/
public com.google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField getLocalField() {
if (fieldCase_ == 22) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField result = com.google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField.UNSPECIFIED;
}
public static final int JOB_FIELD_FIELD_NUMBER = 23;
/**
*
* Immutable. Job Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField job_field = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the jobField field is set.
*/
public boolean hasJobField() {
return fieldCase_ == 23;
}
/**
*
* Immutable. Job Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField job_field = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for jobField.
*/
public int getJobFieldValue() {
if (fieldCase_ == 23) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Job Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField job_field = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return The jobField.
*/
public com.google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField getJobField() {
if (fieldCase_ == 23) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField result = com.google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField.UNSPECIFIED;
}
public static final int IMAGE_FIELD_FIELD_NUMBER = 26;
/**
*
* Immutable. Image Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField image_field = 26 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the imageField field is set.
*/
public boolean hasImageField() {
return fieldCase_ == 26;
}
/**
*
* Immutable. Image Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField image_field = 26 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for imageField.
*/
public int getImageFieldValue() {
if (fieldCase_ == 26) {
return (java.lang.Integer) field_;
}
return 0;
}
/**
*
* Immutable. Image Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField image_field = 26 [(.google.api.field_behavior) = IMMUTABLE];
* @return The imageField.
*/
public com.google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField getImageField() {
if (fieldCase_ == 26) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField result = com.google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField.UNSPECIFIED;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (fieldCase_ == 3) {
output.writeEnum(3, ((java.lang.Integer) field_));
}
if (fieldCase_ == 4) {
output.writeEnum(4, ((java.lang.Integer) field_));
}
if (fieldCase_ == 5) {
output.writeEnum(5, ((java.lang.Integer) field_));
}
if (fieldCase_ == 6) {
output.writeEnum(6, ((java.lang.Integer) field_));
}
if (fieldCase_ == 7) {
output.writeEnum(7, ((java.lang.Integer) field_));
}
if (fieldCase_ == 8) {
output.writeEnum(8, ((java.lang.Integer) field_));
}
if (fieldCase_ == 9) {
output.writeEnum(9, ((java.lang.Integer) field_));
}
if (fieldCase_ == 10) {
output.writeEnum(10, ((java.lang.Integer) field_));
}
if (fieldCase_ == 11) {
output.writeEnum(11, ((java.lang.Integer) field_));
}
if (fieldCase_ == 12) {
output.writeEnum(12, ((java.lang.Integer) field_));
}
if (fieldCase_ == 13) {
output.writeEnum(13, ((java.lang.Integer) field_));
}
if (fieldCase_ == 14) {
output.writeEnum(14, ((java.lang.Integer) field_));
}
if (fieldCase_ == 15) {
output.writeEnum(15, ((java.lang.Integer) field_));
}
if (fieldCase_ == 16) {
output.writeEnum(16, ((java.lang.Integer) field_));
}
if (fieldCase_ == 17) {
output.writeEnum(17, ((java.lang.Integer) field_));
}
if (fieldCase_ == 18) {
output.writeEnum(18, ((java.lang.Integer) field_));
}
if (fieldCase_ == 19) {
output.writeEnum(19, ((java.lang.Integer) field_));
}
if (fieldCase_ == 20) {
output.writeEnum(20, ((java.lang.Integer) field_));
}
if (fieldCase_ == 21) {
output.writeEnum(21, ((java.lang.Integer) field_));
}
if (fieldCase_ == 22) {
output.writeEnum(22, ((java.lang.Integer) field_));
}
if (fieldCase_ == 23) {
output.writeEnum(23, ((java.lang.Integer) field_));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(24, feedAttributeId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(25, fieldId_);
}
if (fieldCase_ == 26) {
output.writeEnum(26, ((java.lang.Integer) field_));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (fieldCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, ((java.lang.Integer) field_));
}
if (fieldCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, ((java.lang.Integer) field_));
}
if (fieldCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, ((java.lang.Integer) field_));
}
if (fieldCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, ((java.lang.Integer) field_));
}
if (fieldCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, ((java.lang.Integer) field_));
}
if (fieldCase_ == 8) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(8, ((java.lang.Integer) field_));
}
if (fieldCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(9, ((java.lang.Integer) field_));
}
if (fieldCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, ((java.lang.Integer) field_));
}
if (fieldCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(11, ((java.lang.Integer) field_));
}
if (fieldCase_ == 12) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(12, ((java.lang.Integer) field_));
}
if (fieldCase_ == 13) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(13, ((java.lang.Integer) field_));
}
if (fieldCase_ == 14) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(14, ((java.lang.Integer) field_));
}
if (fieldCase_ == 15) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(15, ((java.lang.Integer) field_));
}
if (fieldCase_ == 16) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(16, ((java.lang.Integer) field_));
}
if (fieldCase_ == 17) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(17, ((java.lang.Integer) field_));
}
if (fieldCase_ == 18) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(18, ((java.lang.Integer) field_));
}
if (fieldCase_ == 19) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(19, ((java.lang.Integer) field_));
}
if (fieldCase_ == 20) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(20, ((java.lang.Integer) field_));
}
if (fieldCase_ == 21) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(21, ((java.lang.Integer) field_));
}
if (fieldCase_ == 22) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(22, ((java.lang.Integer) field_));
}
if (fieldCase_ == 23) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(23, ((java.lang.Integer) field_));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(24, feedAttributeId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(25, fieldId_);
}
if (fieldCase_ == 26) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(26, ((java.lang.Integer) field_));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v10.resources.AttributeFieldMapping)) {
return super.equals(obj);
}
com.google.ads.googleads.v10.resources.AttributeFieldMapping other = (com.google.ads.googleads.v10.resources.AttributeFieldMapping) obj;
if (hasFeedAttributeId() != other.hasFeedAttributeId()) return false;
if (hasFeedAttributeId()) {
if (getFeedAttributeId()
!= other.getFeedAttributeId()) return false;
}
if (hasFieldId() != other.hasFieldId()) return false;
if (hasFieldId()) {
if (getFieldId()
!= other.getFieldId()) return false;
}
if (!getFieldCase().equals(other.getFieldCase())) return false;
switch (fieldCase_) {
case 3:
if (getSitelinkFieldValue()
!= other.getSitelinkFieldValue()) return false;
break;
case 4:
if (getCallFieldValue()
!= other.getCallFieldValue()) return false;
break;
case 5:
if (getAppFieldValue()
!= other.getAppFieldValue()) return false;
break;
case 6:
if (getLocationFieldValue()
!= other.getLocationFieldValue()) return false;
break;
case 7:
if (getAffiliateLocationFieldValue()
!= other.getAffiliateLocationFieldValue()) return false;
break;
case 8:
if (getCalloutFieldValue()
!= other.getCalloutFieldValue()) return false;
break;
case 9:
if (getStructuredSnippetFieldValue()
!= other.getStructuredSnippetFieldValue()) return false;
break;
case 10:
if (getMessageFieldValue()
!= other.getMessageFieldValue()) return false;
break;
case 11:
if (getPriceFieldValue()
!= other.getPriceFieldValue()) return false;
break;
case 12:
if (getPromotionFieldValue()
!= other.getPromotionFieldValue()) return false;
break;
case 13:
if (getAdCustomizerFieldValue()
!= other.getAdCustomizerFieldValue()) return false;
break;
case 14:
if (getDsaPageFeedFieldValue()
!= other.getDsaPageFeedFieldValue()) return false;
break;
case 15:
if (getLocationExtensionTargetingFieldValue()
!= other.getLocationExtensionTargetingFieldValue()) return false;
break;
case 16:
if (getEducationFieldValue()
!= other.getEducationFieldValue()) return false;
break;
case 17:
if (getFlightFieldValue()
!= other.getFlightFieldValue()) return false;
break;
case 18:
if (getCustomFieldValue()
!= other.getCustomFieldValue()) return false;
break;
case 19:
if (getHotelFieldValue()
!= other.getHotelFieldValue()) return false;
break;
case 20:
if (getRealEstateFieldValue()
!= other.getRealEstateFieldValue()) return false;
break;
case 21:
if (getTravelFieldValue()
!= other.getTravelFieldValue()) return false;
break;
case 22:
if (getLocalFieldValue()
!= other.getLocalFieldValue()) return false;
break;
case 23:
if (getJobFieldValue()
!= other.getJobFieldValue()) return false;
break;
case 26:
if (getImageFieldValue()
!= other.getImageFieldValue()) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFeedAttributeId()) {
hash = (37 * hash) + FEED_ATTRIBUTE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFeedAttributeId());
}
if (hasFieldId()) {
hash = (37 * hash) + FIELD_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFieldId());
}
switch (fieldCase_) {
case 3:
hash = (37 * hash) + SITELINK_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getSitelinkFieldValue();
break;
case 4:
hash = (37 * hash) + CALL_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getCallFieldValue();
break;
case 5:
hash = (37 * hash) + APP_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getAppFieldValue();
break;
case 6:
hash = (37 * hash) + LOCATION_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getLocationFieldValue();
break;
case 7:
hash = (37 * hash) + AFFILIATE_LOCATION_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getAffiliateLocationFieldValue();
break;
case 8:
hash = (37 * hash) + CALLOUT_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getCalloutFieldValue();
break;
case 9:
hash = (37 * hash) + STRUCTURED_SNIPPET_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getStructuredSnippetFieldValue();
break;
case 10:
hash = (37 * hash) + MESSAGE_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getMessageFieldValue();
break;
case 11:
hash = (37 * hash) + PRICE_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getPriceFieldValue();
break;
case 12:
hash = (37 * hash) + PROMOTION_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getPromotionFieldValue();
break;
case 13:
hash = (37 * hash) + AD_CUSTOMIZER_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getAdCustomizerFieldValue();
break;
case 14:
hash = (37 * hash) + DSA_PAGE_FEED_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getDsaPageFeedFieldValue();
break;
case 15:
hash = (37 * hash) + LOCATION_EXTENSION_TARGETING_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getLocationExtensionTargetingFieldValue();
break;
case 16:
hash = (37 * hash) + EDUCATION_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getEducationFieldValue();
break;
case 17:
hash = (37 * hash) + FLIGHT_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getFlightFieldValue();
break;
case 18:
hash = (37 * hash) + CUSTOM_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getCustomFieldValue();
break;
case 19:
hash = (37 * hash) + HOTEL_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getHotelFieldValue();
break;
case 20:
hash = (37 * hash) + REAL_ESTATE_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getRealEstateFieldValue();
break;
case 21:
hash = (37 * hash) + TRAVEL_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getTravelFieldValue();
break;
case 22:
hash = (37 * hash) + LOCAL_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getLocalFieldValue();
break;
case 23:
hash = (37 * hash) + JOB_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getJobFieldValue();
break;
case 26:
hash = (37 * hash) + IMAGE_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getImageFieldValue();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v10.resources.AttributeFieldMapping prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Maps from feed attribute id to a placeholder or criterion field id.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.AttributeFieldMapping}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v10.resources.AttributeFieldMapping)
com.google.ads.googleads.v10.resources.AttributeFieldMappingOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.FeedMappingProto.internal_static_google_ads_googleads_v10_resources_AttributeFieldMapping_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.FeedMappingProto.internal_static_google_ads_googleads_v10_resources_AttributeFieldMapping_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.AttributeFieldMapping.class, com.google.ads.googleads.v10.resources.AttributeFieldMapping.Builder.class);
}
// Construct using com.google.ads.googleads.v10.resources.AttributeFieldMapping.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
feedAttributeId_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
fieldId_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
fieldCase_ = 0;
field_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v10.resources.FeedMappingProto.internal_static_google_ads_googleads_v10_resources_AttributeFieldMapping_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AttributeFieldMapping getDefaultInstanceForType() {
return com.google.ads.googleads.v10.resources.AttributeFieldMapping.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AttributeFieldMapping build() {
com.google.ads.googleads.v10.resources.AttributeFieldMapping result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AttributeFieldMapping buildPartial() {
com.google.ads.googleads.v10.resources.AttributeFieldMapping result = new com.google.ads.googleads.v10.resources.AttributeFieldMapping(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.feedAttributeId_ = feedAttributeId_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.fieldId_ = fieldId_;
to_bitField0_ |= 0x00000002;
}
if (fieldCase_ == 3) {
result.field_ = field_;
}
if (fieldCase_ == 4) {
result.field_ = field_;
}
if (fieldCase_ == 5) {
result.field_ = field_;
}
if (fieldCase_ == 6) {
result.field_ = field_;
}
if (fieldCase_ == 7) {
result.field_ = field_;
}
if (fieldCase_ == 8) {
result.field_ = field_;
}
if (fieldCase_ == 9) {
result.field_ = field_;
}
if (fieldCase_ == 10) {
result.field_ = field_;
}
if (fieldCase_ == 11) {
result.field_ = field_;
}
if (fieldCase_ == 12) {
result.field_ = field_;
}
if (fieldCase_ == 13) {
result.field_ = field_;
}
if (fieldCase_ == 14) {
result.field_ = field_;
}
if (fieldCase_ == 15) {
result.field_ = field_;
}
if (fieldCase_ == 16) {
result.field_ = field_;
}
if (fieldCase_ == 17) {
result.field_ = field_;
}
if (fieldCase_ == 18) {
result.field_ = field_;
}
if (fieldCase_ == 19) {
result.field_ = field_;
}
if (fieldCase_ == 20) {
result.field_ = field_;
}
if (fieldCase_ == 21) {
result.field_ = field_;
}
if (fieldCase_ == 22) {
result.field_ = field_;
}
if (fieldCase_ == 23) {
result.field_ = field_;
}
if (fieldCase_ == 26) {
result.field_ = field_;
}
result.bitField0_ = to_bitField0_;
result.fieldCase_ = fieldCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v10.resources.AttributeFieldMapping) {
return mergeFrom((com.google.ads.googleads.v10.resources.AttributeFieldMapping)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v10.resources.AttributeFieldMapping other) {
if (other == com.google.ads.googleads.v10.resources.AttributeFieldMapping.getDefaultInstance()) return this;
if (other.hasFeedAttributeId()) {
setFeedAttributeId(other.getFeedAttributeId());
}
if (other.hasFieldId()) {
setFieldId(other.getFieldId());
}
switch (other.getFieldCase()) {
case SITELINK_FIELD: {
setSitelinkFieldValue(other.getSitelinkFieldValue());
break;
}
case CALL_FIELD: {
setCallFieldValue(other.getCallFieldValue());
break;
}
case APP_FIELD: {
setAppFieldValue(other.getAppFieldValue());
break;
}
case LOCATION_FIELD: {
setLocationFieldValue(other.getLocationFieldValue());
break;
}
case AFFILIATE_LOCATION_FIELD: {
setAffiliateLocationFieldValue(other.getAffiliateLocationFieldValue());
break;
}
case CALLOUT_FIELD: {
setCalloutFieldValue(other.getCalloutFieldValue());
break;
}
case STRUCTURED_SNIPPET_FIELD: {
setStructuredSnippetFieldValue(other.getStructuredSnippetFieldValue());
break;
}
case MESSAGE_FIELD: {
setMessageFieldValue(other.getMessageFieldValue());
break;
}
case PRICE_FIELD: {
setPriceFieldValue(other.getPriceFieldValue());
break;
}
case PROMOTION_FIELD: {
setPromotionFieldValue(other.getPromotionFieldValue());
break;
}
case AD_CUSTOMIZER_FIELD: {
setAdCustomizerFieldValue(other.getAdCustomizerFieldValue());
break;
}
case DSA_PAGE_FEED_FIELD: {
setDsaPageFeedFieldValue(other.getDsaPageFeedFieldValue());
break;
}
case LOCATION_EXTENSION_TARGETING_FIELD: {
setLocationExtensionTargetingFieldValue(other.getLocationExtensionTargetingFieldValue());
break;
}
case EDUCATION_FIELD: {
setEducationFieldValue(other.getEducationFieldValue());
break;
}
case FLIGHT_FIELD: {
setFlightFieldValue(other.getFlightFieldValue());
break;
}
case CUSTOM_FIELD: {
setCustomFieldValue(other.getCustomFieldValue());
break;
}
case HOTEL_FIELD: {
setHotelFieldValue(other.getHotelFieldValue());
break;
}
case REAL_ESTATE_FIELD: {
setRealEstateFieldValue(other.getRealEstateFieldValue());
break;
}
case TRAVEL_FIELD: {
setTravelFieldValue(other.getTravelFieldValue());
break;
}
case LOCAL_FIELD: {
setLocalFieldValue(other.getLocalFieldValue());
break;
}
case JOB_FIELD: {
setJobFieldValue(other.getJobFieldValue());
break;
}
case IMAGE_FIELD: {
setImageFieldValue(other.getImageFieldValue());
break;
}
case FIELD_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 24: {
int rawValue = input.readEnum();
fieldCase_ = 3;
field_ = rawValue;
break;
} // case 24
case 32: {
int rawValue = input.readEnum();
fieldCase_ = 4;
field_ = rawValue;
break;
} // case 32
case 40: {
int rawValue = input.readEnum();
fieldCase_ = 5;
field_ = rawValue;
break;
} // case 40
case 48: {
int rawValue = input.readEnum();
fieldCase_ = 6;
field_ = rawValue;
break;
} // case 48
case 56: {
int rawValue = input.readEnum();
fieldCase_ = 7;
field_ = rawValue;
break;
} // case 56
case 64: {
int rawValue = input.readEnum();
fieldCase_ = 8;
field_ = rawValue;
break;
} // case 64
case 72: {
int rawValue = input.readEnum();
fieldCase_ = 9;
field_ = rawValue;
break;
} // case 72
case 80: {
int rawValue = input.readEnum();
fieldCase_ = 10;
field_ = rawValue;
break;
} // case 80
case 88: {
int rawValue = input.readEnum();
fieldCase_ = 11;
field_ = rawValue;
break;
} // case 88
case 96: {
int rawValue = input.readEnum();
fieldCase_ = 12;
field_ = rawValue;
break;
} // case 96
case 104: {
int rawValue = input.readEnum();
fieldCase_ = 13;
field_ = rawValue;
break;
} // case 104
case 112: {
int rawValue = input.readEnum();
fieldCase_ = 14;
field_ = rawValue;
break;
} // case 112
case 120: {
int rawValue = input.readEnum();
fieldCase_ = 15;
field_ = rawValue;
break;
} // case 120
case 128: {
int rawValue = input.readEnum();
fieldCase_ = 16;
field_ = rawValue;
break;
} // case 128
case 136: {
int rawValue = input.readEnum();
fieldCase_ = 17;
field_ = rawValue;
break;
} // case 136
case 144: {
int rawValue = input.readEnum();
fieldCase_ = 18;
field_ = rawValue;
break;
} // case 144
case 152: {
int rawValue = input.readEnum();
fieldCase_ = 19;
field_ = rawValue;
break;
} // case 152
case 160: {
int rawValue = input.readEnum();
fieldCase_ = 20;
field_ = rawValue;
break;
} // case 160
case 168: {
int rawValue = input.readEnum();
fieldCase_ = 21;
field_ = rawValue;
break;
} // case 168
case 176: {
int rawValue = input.readEnum();
fieldCase_ = 22;
field_ = rawValue;
break;
} // case 176
case 184: {
int rawValue = input.readEnum();
fieldCase_ = 23;
field_ = rawValue;
break;
} // case 184
case 192: {
feedAttributeId_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 192
case 200: {
fieldId_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 200
case 208: {
int rawValue = input.readEnum();
fieldCase_ = 26;
field_ = rawValue;
break;
} // case 208
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int fieldCase_ = 0;
private java.lang.Object field_;
public FieldCase
getFieldCase() {
return FieldCase.forNumber(
fieldCase_);
}
public Builder clearField() {
fieldCase_ = 0;
field_ = null;
onChanged();
return this;
}
private int bitField0_;
private long feedAttributeId_ ;
/**
*
* Immutable. Feed attribute from which to map.
*
*
* optional int64 feed_attribute_id = 24 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the feedAttributeId field is set.
*/
@java.lang.Override
public boolean hasFeedAttributeId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Immutable. Feed attribute from which to map.
*
*
* optional int64 feed_attribute_id = 24 [(.google.api.field_behavior) = IMMUTABLE];
* @return The feedAttributeId.
*/
@java.lang.Override
public long getFeedAttributeId() {
return feedAttributeId_;
}
/**
*
* Immutable. Feed attribute from which to map.
*
*
* optional int64 feed_attribute_id = 24 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The feedAttributeId to set.
* @return This builder for chaining.
*/
public Builder setFeedAttributeId(long value) {
bitField0_ |= 0x00000001;
feedAttributeId_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Feed attribute from which to map.
*
*
* optional int64 feed_attribute_id = 24 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearFeedAttributeId() {
bitField0_ = (bitField0_ & ~0x00000001);
feedAttributeId_ = 0L;
onChanged();
return this;
}
private long fieldId_ ;
/**
*
* Output only. The placeholder field ID. If a placeholder field enum is not published in
* the current API version, then this field will be populated and the field
* oneof will be empty.
* This field is read-only.
*
*
* optional int64 field_id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the fieldId field is set.
*/
@java.lang.Override
public boolean hasFieldId() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The placeholder field ID. If a placeholder field enum is not published in
* the current API version, then this field will be populated and the field
* oneof will be empty.
* This field is read-only.
*
*
* optional int64 field_id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The fieldId.
*/
@java.lang.Override
public long getFieldId() {
return fieldId_;
}
/**
*
* Output only. The placeholder field ID. If a placeholder field enum is not published in
* the current API version, then this field will be populated and the field
* oneof will be empty.
* This field is read-only.
*
*
* optional int64 field_id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The fieldId to set.
* @return This builder for chaining.
*/
public Builder setFieldId(long value) {
bitField0_ |= 0x00000002;
fieldId_ = value;
onChanged();
return this;
}
/**
*
* Output only. The placeholder field ID. If a placeholder field enum is not published in
* the current API version, then this field will be populated and the field
* oneof will be empty.
* This field is read-only.
*
*
* optional int64 field_id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearFieldId() {
bitField0_ = (bitField0_ & ~0x00000002);
fieldId_ = 0L;
onChanged();
return this;
}
/**
*
* Immutable. Sitelink Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField sitelink_field = 3 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the sitelinkField field is set.
*/
@java.lang.Override
public boolean hasSitelinkField() {
return fieldCase_ == 3;
}
/**
*
* Immutable. Sitelink Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField sitelink_field = 3 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for sitelinkField.
*/
@java.lang.Override
public int getSitelinkFieldValue() {
if (fieldCase_ == 3) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Sitelink Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField sitelink_field = 3 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for sitelinkField to set.
* @return This builder for chaining.
*/
public Builder setSitelinkFieldValue(int value) {
fieldCase_ = 3;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Sitelink Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField sitelink_field = 3 [(.google.api.field_behavior) = IMMUTABLE];
* @return The sitelinkField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField getSitelinkField() {
if (fieldCase_ == 3) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField result = com.google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Sitelink Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField sitelink_field = 3 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The sitelinkField to set.
* @return This builder for chaining.
*/
public Builder setSitelinkField(com.google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 3;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Sitelink Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.SitelinkPlaceholderFieldEnum.SitelinkPlaceholderField sitelink_field = 3 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearSitelinkField() {
if (fieldCase_ == 3) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Call Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField call_field = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the callField field is set.
*/
@java.lang.Override
public boolean hasCallField() {
return fieldCase_ == 4;
}
/**
*
* Immutable. Call Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField call_field = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for callField.
*/
@java.lang.Override
public int getCallFieldValue() {
if (fieldCase_ == 4) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Call Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField call_field = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for callField to set.
* @return This builder for chaining.
*/
public Builder setCallFieldValue(int value) {
fieldCase_ = 4;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Call Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField call_field = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return The callField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField getCallField() {
if (fieldCase_ == 4) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField result = com.google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Call Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField call_field = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The callField to set.
* @return This builder for chaining.
*/
public Builder setCallField(com.google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 4;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Call Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CallPlaceholderFieldEnum.CallPlaceholderField call_field = 4 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearCallField() {
if (fieldCase_ == 4) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. App Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField app_field = 5 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the appField field is set.
*/
@java.lang.Override
public boolean hasAppField() {
return fieldCase_ == 5;
}
/**
*
* Immutable. App Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField app_field = 5 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for appField.
*/
@java.lang.Override
public int getAppFieldValue() {
if (fieldCase_ == 5) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. App Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField app_field = 5 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for appField to set.
* @return This builder for chaining.
*/
public Builder setAppFieldValue(int value) {
fieldCase_ = 5;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. App Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField app_field = 5 [(.google.api.field_behavior) = IMMUTABLE];
* @return The appField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField getAppField() {
if (fieldCase_ == 5) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField result = com.google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. App Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField app_field = 5 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The appField to set.
* @return This builder for chaining.
*/
public Builder setAppField(com.google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 5;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. App Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.AppPlaceholderFieldEnum.AppPlaceholderField app_field = 5 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearAppField() {
if (fieldCase_ == 5) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField location_field = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the locationField field is set.
*/
@java.lang.Override
public boolean hasLocationField() {
return fieldCase_ == 6;
}
/**
*
* Output only. Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField location_field = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for locationField.
*/
@java.lang.Override
public int getLocationFieldValue() {
if (fieldCase_ == 6) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Output only. Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField location_field = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for locationField to set.
* @return This builder for chaining.
*/
public Builder setLocationFieldValue(int value) {
fieldCase_ = 6;
field_ = value;
onChanged();
return this;
}
/**
*
* Output only. Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField location_field = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The locationField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField getLocationField() {
if (fieldCase_ == 6) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField result = com.google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField.UNSPECIFIED;
}
/**
*
* Output only. Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField location_field = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The locationField to set.
* @return This builder for chaining.
*/
public Builder setLocationField(com.google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 6;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.LocationPlaceholderFieldEnum.LocationPlaceholderField location_field = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearLocationField() {
if (fieldCase_ == 6) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Output only. Affiliate Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField affiliate_location_field = 7 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the affiliateLocationField field is set.
*/
@java.lang.Override
public boolean hasAffiliateLocationField() {
return fieldCase_ == 7;
}
/**
*
* Output only. Affiliate Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField affiliate_location_field = 7 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for affiliateLocationField.
*/
@java.lang.Override
public int getAffiliateLocationFieldValue() {
if (fieldCase_ == 7) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Output only. Affiliate Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField affiliate_location_field = 7 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for affiliateLocationField to set.
* @return This builder for chaining.
*/
public Builder setAffiliateLocationFieldValue(int value) {
fieldCase_ = 7;
field_ = value;
onChanged();
return this;
}
/**
*
* Output only. Affiliate Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField affiliate_location_field = 7 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The affiliateLocationField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField getAffiliateLocationField() {
if (fieldCase_ == 7) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField result = com.google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField.UNSPECIFIED;
}
/**
*
* Output only. Affiliate Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField affiliate_location_field = 7 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The affiliateLocationField to set.
* @return This builder for chaining.
*/
public Builder setAffiliateLocationField(com.google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 7;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. Affiliate Location Placeholder Fields. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AffiliateLocationPlaceholderFieldEnum.AffiliateLocationPlaceholderField affiliate_location_field = 7 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearAffiliateLocationField() {
if (fieldCase_ == 7) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Callout Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField callout_field = 8 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the calloutField field is set.
*/
@java.lang.Override
public boolean hasCalloutField() {
return fieldCase_ == 8;
}
/**
*
* Immutable. Callout Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField callout_field = 8 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for calloutField.
*/
@java.lang.Override
public int getCalloutFieldValue() {
if (fieldCase_ == 8) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Callout Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField callout_field = 8 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for calloutField to set.
* @return This builder for chaining.
*/
public Builder setCalloutFieldValue(int value) {
fieldCase_ = 8;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Callout Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField callout_field = 8 [(.google.api.field_behavior) = IMMUTABLE];
* @return The calloutField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField getCalloutField() {
if (fieldCase_ == 8) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField result = com.google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Callout Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField callout_field = 8 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The calloutField to set.
* @return This builder for chaining.
*/
public Builder setCalloutField(com.google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 8;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Callout Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.CalloutPlaceholderFieldEnum.CalloutPlaceholderField callout_field = 8 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearCalloutField() {
if (fieldCase_ == 8) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Structured Snippet Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField structured_snippet_field = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the structuredSnippetField field is set.
*/
@java.lang.Override
public boolean hasStructuredSnippetField() {
return fieldCase_ == 9;
}
/**
*
* Immutable. Structured Snippet Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField structured_snippet_field = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for structuredSnippetField.
*/
@java.lang.Override
public int getStructuredSnippetFieldValue() {
if (fieldCase_ == 9) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Structured Snippet Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField structured_snippet_field = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for structuredSnippetField to set.
* @return This builder for chaining.
*/
public Builder setStructuredSnippetFieldValue(int value) {
fieldCase_ = 9;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Structured Snippet Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField structured_snippet_field = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return The structuredSnippetField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField getStructuredSnippetField() {
if (fieldCase_ == 9) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField result = com.google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Structured Snippet Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField structured_snippet_field = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The structuredSnippetField to set.
* @return This builder for chaining.
*/
public Builder setStructuredSnippetField(com.google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 9;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Structured Snippet Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.StructuredSnippetPlaceholderFieldEnum.StructuredSnippetPlaceholderField structured_snippet_field = 9 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearStructuredSnippetField() {
if (fieldCase_ == 9) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Message Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField message_field = 10 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the messageField field is set.
*/
@java.lang.Override
public boolean hasMessageField() {
return fieldCase_ == 10;
}
/**
*
* Immutable. Message Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField message_field = 10 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for messageField.
*/
@java.lang.Override
public int getMessageFieldValue() {
if (fieldCase_ == 10) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Message Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField message_field = 10 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for messageField to set.
* @return This builder for chaining.
*/
public Builder setMessageFieldValue(int value) {
fieldCase_ = 10;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Message Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField message_field = 10 [(.google.api.field_behavior) = IMMUTABLE];
* @return The messageField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField getMessageField() {
if (fieldCase_ == 10) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField result = com.google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Message Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField message_field = 10 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The messageField to set.
* @return This builder for chaining.
*/
public Builder setMessageField(com.google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 10;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Message Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.MessagePlaceholderFieldEnum.MessagePlaceholderField message_field = 10 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearMessageField() {
if (fieldCase_ == 10) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Price Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField price_field = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the priceField field is set.
*/
@java.lang.Override
public boolean hasPriceField() {
return fieldCase_ == 11;
}
/**
*
* Immutable. Price Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField price_field = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for priceField.
*/
@java.lang.Override
public int getPriceFieldValue() {
if (fieldCase_ == 11) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Price Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField price_field = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for priceField to set.
* @return This builder for chaining.
*/
public Builder setPriceFieldValue(int value) {
fieldCase_ = 11;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Price Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField price_field = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return The priceField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField getPriceField() {
if (fieldCase_ == 11) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField result = com.google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Price Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField price_field = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The priceField to set.
* @return This builder for chaining.
*/
public Builder setPriceField(com.google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 11;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Price Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PricePlaceholderFieldEnum.PricePlaceholderField price_field = 11 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearPriceField() {
if (fieldCase_ == 11) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Promotion Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField promotion_field = 12 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the promotionField field is set.
*/
@java.lang.Override
public boolean hasPromotionField() {
return fieldCase_ == 12;
}
/**
*
* Immutable. Promotion Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField promotion_field = 12 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for promotionField.
*/
@java.lang.Override
public int getPromotionFieldValue() {
if (fieldCase_ == 12) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Promotion Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField promotion_field = 12 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for promotionField to set.
* @return This builder for chaining.
*/
public Builder setPromotionFieldValue(int value) {
fieldCase_ = 12;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Promotion Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField promotion_field = 12 [(.google.api.field_behavior) = IMMUTABLE];
* @return The promotionField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField getPromotionField() {
if (fieldCase_ == 12) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField result = com.google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Promotion Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField promotion_field = 12 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The promotionField to set.
* @return This builder for chaining.
*/
public Builder setPromotionField(com.google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 12;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Promotion Placeholder Fields.
*
*
* .google.ads.googleads.v10.enums.PromotionPlaceholderFieldEnum.PromotionPlaceholderField promotion_field = 12 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearPromotionField() {
if (fieldCase_ == 12) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Ad Customizer Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField ad_customizer_field = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the adCustomizerField field is set.
*/
@java.lang.Override
public boolean hasAdCustomizerField() {
return fieldCase_ == 13;
}
/**
*
* Immutable. Ad Customizer Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField ad_customizer_field = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for adCustomizerField.
*/
@java.lang.Override
public int getAdCustomizerFieldValue() {
if (fieldCase_ == 13) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Ad Customizer Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField ad_customizer_field = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for adCustomizerField to set.
* @return This builder for chaining.
*/
public Builder setAdCustomizerFieldValue(int value) {
fieldCase_ = 13;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Ad Customizer Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField ad_customizer_field = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return The adCustomizerField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField getAdCustomizerField() {
if (fieldCase_ == 13) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField result = com.google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Ad Customizer Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField ad_customizer_field = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The adCustomizerField to set.
* @return This builder for chaining.
*/
public Builder setAdCustomizerField(com.google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 13;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Ad Customizer Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.AdCustomizerPlaceholderFieldEnum.AdCustomizerPlaceholderField ad_customizer_field = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearAdCustomizerField() {
if (fieldCase_ == 13) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Dynamic Search Ad Page Feed Fields.
*
*
* .google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField dsa_page_feed_field = 14 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the dsaPageFeedField field is set.
*/
@java.lang.Override
public boolean hasDsaPageFeedField() {
return fieldCase_ == 14;
}
/**
*
* Immutable. Dynamic Search Ad Page Feed Fields.
*
*
* .google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField dsa_page_feed_field = 14 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for dsaPageFeedField.
*/
@java.lang.Override
public int getDsaPageFeedFieldValue() {
if (fieldCase_ == 14) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Dynamic Search Ad Page Feed Fields.
*
*
* .google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField dsa_page_feed_field = 14 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for dsaPageFeedField to set.
* @return This builder for chaining.
*/
public Builder setDsaPageFeedFieldValue(int value) {
fieldCase_ = 14;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Dynamic Search Ad Page Feed Fields.
*
*
* .google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField dsa_page_feed_field = 14 [(.google.api.field_behavior) = IMMUTABLE];
* @return The dsaPageFeedField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField getDsaPageFeedField() {
if (fieldCase_ == 14) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField result = com.google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField.UNSPECIFIED;
}
/**
*
* Immutable. Dynamic Search Ad Page Feed Fields.
*
*
* .google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField dsa_page_feed_field = 14 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The dsaPageFeedField to set.
* @return This builder for chaining.
*/
public Builder setDsaPageFeedField(com.google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 14;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Dynamic Search Ad Page Feed Fields.
*
*
* .google.ads.googleads.v10.enums.DsaPageFeedCriterionFieldEnum.DsaPageFeedCriterionField dsa_page_feed_field = 14 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearDsaPageFeedField() {
if (fieldCase_ == 14) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Location Target Fields.
*
*
* .google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField location_extension_targeting_field = 15 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the locationExtensionTargetingField field is set.
*/
@java.lang.Override
public boolean hasLocationExtensionTargetingField() {
return fieldCase_ == 15;
}
/**
*
* Immutable. Location Target Fields.
*
*
* .google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField location_extension_targeting_field = 15 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for locationExtensionTargetingField.
*/
@java.lang.Override
public int getLocationExtensionTargetingFieldValue() {
if (fieldCase_ == 15) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Location Target Fields.
*
*
* .google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField location_extension_targeting_field = 15 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for locationExtensionTargetingField to set.
* @return This builder for chaining.
*/
public Builder setLocationExtensionTargetingFieldValue(int value) {
fieldCase_ = 15;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Location Target Fields.
*
*
* .google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField location_extension_targeting_field = 15 [(.google.api.field_behavior) = IMMUTABLE];
* @return The locationExtensionTargetingField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField getLocationExtensionTargetingField() {
if (fieldCase_ == 15) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField result = com.google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField.UNSPECIFIED;
}
/**
*
* Immutable. Location Target Fields.
*
*
* .google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField location_extension_targeting_field = 15 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The locationExtensionTargetingField to set.
* @return This builder for chaining.
*/
public Builder setLocationExtensionTargetingField(com.google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 15;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Location Target Fields.
*
*
* .google.ads.googleads.v10.enums.LocationExtensionTargetingCriterionFieldEnum.LocationExtensionTargetingCriterionField location_extension_targeting_field = 15 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearLocationExtensionTargetingField() {
if (fieldCase_ == 15) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Education Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField education_field = 16 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the educationField field is set.
*/
@java.lang.Override
public boolean hasEducationField() {
return fieldCase_ == 16;
}
/**
*
* Immutable. Education Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField education_field = 16 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for educationField.
*/
@java.lang.Override
public int getEducationFieldValue() {
if (fieldCase_ == 16) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Education Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField education_field = 16 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for educationField to set.
* @return This builder for chaining.
*/
public Builder setEducationFieldValue(int value) {
fieldCase_ = 16;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Education Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField education_field = 16 [(.google.api.field_behavior) = IMMUTABLE];
* @return The educationField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField getEducationField() {
if (fieldCase_ == 16) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField result = com.google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Education Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField education_field = 16 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The educationField to set.
* @return This builder for chaining.
*/
public Builder setEducationField(com.google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 16;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Education Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.EducationPlaceholderFieldEnum.EducationPlaceholderField education_field = 16 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearEducationField() {
if (fieldCase_ == 16) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Flight Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField flight_field = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the flightField field is set.
*/
@java.lang.Override
public boolean hasFlightField() {
return fieldCase_ == 17;
}
/**
*
* Immutable. Flight Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField flight_field = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for flightField.
*/
@java.lang.Override
public int getFlightFieldValue() {
if (fieldCase_ == 17) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Flight Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField flight_field = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for flightField to set.
* @return This builder for chaining.
*/
public Builder setFlightFieldValue(int value) {
fieldCase_ = 17;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Flight Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField flight_field = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return The flightField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField getFlightField() {
if (fieldCase_ == 17) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField result = com.google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Flight Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField flight_field = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The flightField to set.
* @return This builder for chaining.
*/
public Builder setFlightField(com.google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 17;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Flight Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.FlightPlaceholderFieldEnum.FlightPlaceholderField flight_field = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearFlightField() {
if (fieldCase_ == 17) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Custom Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField custom_field = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the customField field is set.
*/
@java.lang.Override
public boolean hasCustomField() {
return fieldCase_ == 18;
}
/**
*
* Immutable. Custom Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField custom_field = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for customField.
*/
@java.lang.Override
public int getCustomFieldValue() {
if (fieldCase_ == 18) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Custom Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField custom_field = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for customField to set.
* @return This builder for chaining.
*/
public Builder setCustomFieldValue(int value) {
fieldCase_ = 18;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Custom Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField custom_field = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return The customField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField getCustomField() {
if (fieldCase_ == 18) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField result = com.google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Custom Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField custom_field = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The customField to set.
* @return This builder for chaining.
*/
public Builder setCustomField(com.google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 18;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Custom Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.CustomPlaceholderFieldEnum.CustomPlaceholderField custom_field = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearCustomField() {
if (fieldCase_ == 18) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Hotel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField hotel_field = 19 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the hotelField field is set.
*/
@java.lang.Override
public boolean hasHotelField() {
return fieldCase_ == 19;
}
/**
*
* Immutable. Hotel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField hotel_field = 19 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for hotelField.
*/
@java.lang.Override
public int getHotelFieldValue() {
if (fieldCase_ == 19) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Hotel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField hotel_field = 19 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for hotelField to set.
* @return This builder for chaining.
*/
public Builder setHotelFieldValue(int value) {
fieldCase_ = 19;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Hotel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField hotel_field = 19 [(.google.api.field_behavior) = IMMUTABLE];
* @return The hotelField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField getHotelField() {
if (fieldCase_ == 19) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField result = com.google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Hotel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField hotel_field = 19 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The hotelField to set.
* @return This builder for chaining.
*/
public Builder setHotelField(com.google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 19;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Hotel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.HotelPlaceholderFieldEnum.HotelPlaceholderField hotel_field = 19 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearHotelField() {
if (fieldCase_ == 19) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Real Estate Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField real_estate_field = 20 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the realEstateField field is set.
*/
@java.lang.Override
public boolean hasRealEstateField() {
return fieldCase_ == 20;
}
/**
*
* Immutable. Real Estate Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField real_estate_field = 20 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for realEstateField.
*/
@java.lang.Override
public int getRealEstateFieldValue() {
if (fieldCase_ == 20) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Real Estate Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField real_estate_field = 20 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for realEstateField to set.
* @return This builder for chaining.
*/
public Builder setRealEstateFieldValue(int value) {
fieldCase_ = 20;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Real Estate Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField real_estate_field = 20 [(.google.api.field_behavior) = IMMUTABLE];
* @return The realEstateField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField getRealEstateField() {
if (fieldCase_ == 20) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField result = com.google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Real Estate Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField real_estate_field = 20 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The realEstateField to set.
* @return This builder for chaining.
*/
public Builder setRealEstateField(com.google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 20;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Real Estate Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.RealEstatePlaceholderFieldEnum.RealEstatePlaceholderField real_estate_field = 20 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearRealEstateField() {
if (fieldCase_ == 20) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Travel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField travel_field = 21 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the travelField field is set.
*/
@java.lang.Override
public boolean hasTravelField() {
return fieldCase_ == 21;
}
/**
*
* Immutable. Travel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField travel_field = 21 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for travelField.
*/
@java.lang.Override
public int getTravelFieldValue() {
if (fieldCase_ == 21) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Travel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField travel_field = 21 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for travelField to set.
* @return This builder for chaining.
*/
public Builder setTravelFieldValue(int value) {
fieldCase_ = 21;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Travel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField travel_field = 21 [(.google.api.field_behavior) = IMMUTABLE];
* @return The travelField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField getTravelField() {
if (fieldCase_ == 21) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField result = com.google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Travel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField travel_field = 21 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The travelField to set.
* @return This builder for chaining.
*/
public Builder setTravelField(com.google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 21;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Travel Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.TravelPlaceholderFieldEnum.TravelPlaceholderField travel_field = 21 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearTravelField() {
if (fieldCase_ == 21) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Local Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField local_field = 22 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the localField field is set.
*/
@java.lang.Override
public boolean hasLocalField() {
return fieldCase_ == 22;
}
/**
*
* Immutable. Local Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField local_field = 22 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for localField.
*/
@java.lang.Override
public int getLocalFieldValue() {
if (fieldCase_ == 22) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Local Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField local_field = 22 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for localField to set.
* @return This builder for chaining.
*/
public Builder setLocalFieldValue(int value) {
fieldCase_ = 22;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Local Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField local_field = 22 [(.google.api.field_behavior) = IMMUTABLE];
* @return The localField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField getLocalField() {
if (fieldCase_ == 22) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField result = com.google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Local Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField local_field = 22 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The localField to set.
* @return This builder for chaining.
*/
public Builder setLocalField(com.google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 22;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Local Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.LocalPlaceholderFieldEnum.LocalPlaceholderField local_field = 22 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearLocalField() {
if (fieldCase_ == 22) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Job Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField job_field = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the jobField field is set.
*/
@java.lang.Override
public boolean hasJobField() {
return fieldCase_ == 23;
}
/**
*
* Immutable. Job Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField job_field = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for jobField.
*/
@java.lang.Override
public int getJobFieldValue() {
if (fieldCase_ == 23) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Job Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField job_field = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for jobField to set.
* @return This builder for chaining.
*/
public Builder setJobFieldValue(int value) {
fieldCase_ = 23;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Job Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField job_field = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return The jobField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField getJobField() {
if (fieldCase_ == 23) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField result = com.google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Job Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField job_field = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The jobField to set.
* @return This builder for chaining.
*/
public Builder setJobField(com.google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 23;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Job Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.JobPlaceholderFieldEnum.JobPlaceholderField job_field = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearJobField() {
if (fieldCase_ == 23) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
/**
*
* Immutable. Image Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField image_field = 26 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the imageField field is set.
*/
@java.lang.Override
public boolean hasImageField() {
return fieldCase_ == 26;
}
/**
*
* Immutable. Image Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField image_field = 26 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for imageField.
*/
@java.lang.Override
public int getImageFieldValue() {
if (fieldCase_ == 26) {
return ((java.lang.Integer) field_).intValue();
}
return 0;
}
/**
*
* Immutable. Image Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField image_field = 26 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for imageField to set.
* @return This builder for chaining.
*/
public Builder setImageFieldValue(int value) {
fieldCase_ = 26;
field_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Image Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField image_field = 26 [(.google.api.field_behavior) = IMMUTABLE];
* @return The imageField.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField getImageField() {
if (fieldCase_ == 26) {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField result = com.google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField.valueOf(
(java.lang.Integer) field_);
return result == null ? com.google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField.UNRECOGNIZED : result;
}
return com.google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField.UNSPECIFIED;
}
/**
*
* Immutable. Image Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField image_field = 26 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The imageField to set.
* @return This builder for chaining.
*/
public Builder setImageField(com.google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField value) {
if (value == null) {
throw new NullPointerException();
}
fieldCase_ = 26;
field_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Image Placeholder Fields
*
*
* .google.ads.googleads.v10.enums.ImagePlaceholderFieldEnum.ImagePlaceholderField image_field = 26 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearImageField() {
if (fieldCase_ == 26) {
fieldCase_ = 0;
field_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v10.resources.AttributeFieldMapping)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v10.resources.AttributeFieldMapping)
private static final com.google.ads.googleads.v10.resources.AttributeFieldMapping DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v10.resources.AttributeFieldMapping();
}
public static com.google.ads.googleads.v10.resources.AttributeFieldMapping getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AttributeFieldMapping parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.AttributeFieldMapping getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy