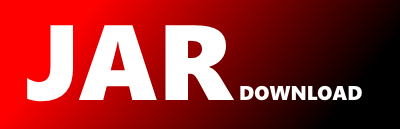
com.google.ads.googleads.v10.resources.BiddingStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v10 Show documentation
Show all versions of google-ads-stubs-v10 Show documentation
Stubs for GAAPI version google-ads-stubs-v10
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v10/resources/bidding_strategy.proto
package com.google.ads.googleads.v10.resources;
/**
*
* A bidding strategy.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.BiddingStrategy}
*/
public final class BiddingStrategy extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v10.resources.BiddingStrategy)
BiddingStrategyOrBuilder {
private static final long serialVersionUID = 0L;
// Use BiddingStrategy.newBuilder() to construct.
private BiddingStrategy(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BiddingStrategy() {
resourceName_ = "";
name_ = "";
status_ = 0;
type_ = 0;
currencyCode_ = "";
effectiveCurrencyCode_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new BiddingStrategy();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.BiddingStrategyProto.internal_static_google_ads_googleads_v10_resources_BiddingStrategy_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.BiddingStrategyProto.internal_static_google_ads_googleads_v10_resources_BiddingStrategy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.BiddingStrategy.class, com.google.ads.googleads.v10.resources.BiddingStrategy.Builder.class);
}
private int bitField0_;
private int schemeCase_ = 0;
private java.lang.Object scheme_;
public enum SchemeCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
ENHANCED_CPC(7),
MAXIMIZE_CONVERSION_VALUE(21),
MAXIMIZE_CONVERSIONS(22),
TARGET_CPA(9),
TARGET_IMPRESSION_SHARE(48),
TARGET_ROAS(11),
TARGET_SPEND(12),
SCHEME_NOT_SET(0);
private final int value;
private SchemeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SchemeCase valueOf(int value) {
return forNumber(value);
}
public static SchemeCase forNumber(int value) {
switch (value) {
case 7: return ENHANCED_CPC;
case 21: return MAXIMIZE_CONVERSION_VALUE;
case 22: return MAXIMIZE_CONVERSIONS;
case 9: return TARGET_CPA;
case 48: return TARGET_IMPRESSION_SHARE;
case 11: return TARGET_ROAS;
case 12: return TARGET_SPEND;
case 0: return SCHEME_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public SchemeCase
getSchemeCase() {
return SchemeCase.forNumber(
schemeCase_);
}
public static final int RESOURCE_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object resourceName_;
/**
*
* Immutable. The resource name of the bidding strategy.
* Bidding strategy resource names have the form:
* `customers/{customer_id}/biddingStrategies/{bidding_strategy_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
@java.lang.Override
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
}
}
/**
*
* Immutable. The resource name of the bidding strategy.
* Bidding strategy resource names have the form:
* `customers/{customer_id}/biddingStrategies/{bidding_strategy_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 16;
private long id_;
/**
*
* Output only. The ID of the bidding strategy.
*
*
* optional int64 id = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The ID of the bidding strategy.
*
*
* optional int64 id = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int NAME_FIELD_NUMBER = 17;
private volatile java.lang.Object name_;
/**
*
* The name of the bidding strategy.
* All bidding strategies within an account must be named distinctly.
* The length of this string should be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 17;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The name of the bidding strategy.
* All bidding strategies within an account must be named distinctly.
* The length of this string should be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 17;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* The name of the bidding strategy.
* All bidding strategies within an account must be named distinctly.
* The length of this string should be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 17;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 15;
private int status_;
/**
*
* Output only. The status of the bidding strategy.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Output only. The status of the bidding strategy.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The status.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus getStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus result = com.google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus.valueOf(status_);
return result == null ? com.google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus.UNRECOGNIZED : result;
}
public static final int TYPE_FIELD_NUMBER = 5;
private int type_;
/**
*
* Output only. The type of the bidding strategy.
* Create a bidding strategy by setting the bidding scheme.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType type = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
*
* Output only. The type of the bidding strategy.
* Create a bidding strategy by setting the bidding scheme.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType type = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The type.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType getType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType result = com.google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType.valueOf(type_);
return result == null ? com.google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType.UNRECOGNIZED : result;
}
public static final int CURRENCY_CODE_FIELD_NUMBER = 23;
private volatile java.lang.Object currencyCode_;
/**
*
* Immutable. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this currency can be set on
* creation and defaults to the manager customer's currency. For serving
* customers, this field cannot be set; all strategies in a serving customer
* implicitly use the serving customer's currency. In all cases the
* effective_currency_code field returns the currency used by the strategy.
*
*
* string currency_code = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return The currencyCode.
*/
@java.lang.Override
public java.lang.String getCurrencyCode() {
java.lang.Object ref = currencyCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currencyCode_ = s;
return s;
}
}
/**
*
* Immutable. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this currency can be set on
* creation and defaults to the manager customer's currency. For serving
* customers, this field cannot be set; all strategies in a serving customer
* implicitly use the serving customer's currency. In all cases the
* effective_currency_code field returns the currency used by the strategy.
*
*
* string currency_code = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for currencyCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCurrencyCodeBytes() {
java.lang.Object ref = currencyCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EFFECTIVE_CURRENCY_CODE_FIELD_NUMBER = 20;
private volatile java.lang.Object effectiveCurrencyCode_;
/**
*
* Output only. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this is the currency set by
* the advertiser when creating the strategy. For serving customers, this is
* the customer's currency_code.
* Bidding strategy metrics are reported in this currency.
* This field is read-only.
*
*
* optional string effective_currency_code = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the effectiveCurrencyCode field is set.
*/
@java.lang.Override
public boolean hasEffectiveCurrencyCode() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this is the currency set by
* the advertiser when creating the strategy. For serving customers, this is
* the customer's currency_code.
* Bidding strategy metrics are reported in this currency.
* This field is read-only.
*
*
* optional string effective_currency_code = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The effectiveCurrencyCode.
*/
@java.lang.Override
public java.lang.String getEffectiveCurrencyCode() {
java.lang.Object ref = effectiveCurrencyCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
effectiveCurrencyCode_ = s;
return s;
}
}
/**
*
* Output only. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this is the currency set by
* the advertiser when creating the strategy. For serving customers, this is
* the customer's currency_code.
* Bidding strategy metrics are reported in this currency.
* This field is read-only.
*
*
* optional string effective_currency_code = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for effectiveCurrencyCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getEffectiveCurrencyCodeBytes() {
java.lang.Object ref = effectiveCurrencyCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
effectiveCurrencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CAMPAIGN_COUNT_FIELD_NUMBER = 18;
private long campaignCount_;
/**
*
* Output only. The number of campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 campaign_count = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the campaignCount field is set.
*/
@java.lang.Override
public boolean hasCampaignCount() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. The number of campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 campaign_count = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The campaignCount.
*/
@java.lang.Override
public long getCampaignCount() {
return campaignCount_;
}
public static final int NON_REMOVED_CAMPAIGN_COUNT_FIELD_NUMBER = 19;
private long nonRemovedCampaignCount_;
/**
*
* Output only. The number of non-removed campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 non_removed_campaign_count = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the nonRemovedCampaignCount field is set.
*/
@java.lang.Override
public boolean hasNonRemovedCampaignCount() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The number of non-removed campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 non_removed_campaign_count = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The nonRemovedCampaignCount.
*/
@java.lang.Override
public long getNonRemovedCampaignCount() {
return nonRemovedCampaignCount_;
}
public static final int ENHANCED_CPC_FIELD_NUMBER = 7;
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
* @return Whether the enhancedCpc field is set.
*/
@java.lang.Override
public boolean hasEnhancedCpc() {
return schemeCase_ == 7;
}
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
* @return The enhancedCpc.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.EnhancedCpc getEnhancedCpc() {
if (schemeCase_ == 7) {
return (com.google.ads.googleads.v10.common.EnhancedCpc) scheme_;
}
return com.google.ads.googleads.v10.common.EnhancedCpc.getDefaultInstance();
}
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.EnhancedCpcOrBuilder getEnhancedCpcOrBuilder() {
if (schemeCase_ == 7) {
return (com.google.ads.googleads.v10.common.EnhancedCpc) scheme_;
}
return com.google.ads.googleads.v10.common.EnhancedCpc.getDefaultInstance();
}
public static final int MAXIMIZE_CONVERSION_VALUE_FIELD_NUMBER = 21;
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
* @return Whether the maximizeConversionValue field is set.
*/
@java.lang.Override
public boolean hasMaximizeConversionValue() {
return schemeCase_ == 21;
}
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
* @return The maximizeConversionValue.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.MaximizeConversionValue getMaximizeConversionValue() {
if (schemeCase_ == 21) {
return (com.google.ads.googleads.v10.common.MaximizeConversionValue) scheme_;
}
return com.google.ads.googleads.v10.common.MaximizeConversionValue.getDefaultInstance();
}
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.MaximizeConversionValueOrBuilder getMaximizeConversionValueOrBuilder() {
if (schemeCase_ == 21) {
return (com.google.ads.googleads.v10.common.MaximizeConversionValue) scheme_;
}
return com.google.ads.googleads.v10.common.MaximizeConversionValue.getDefaultInstance();
}
public static final int MAXIMIZE_CONVERSIONS_FIELD_NUMBER = 22;
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
* @return Whether the maximizeConversions field is set.
*/
@java.lang.Override
public boolean hasMaximizeConversions() {
return schemeCase_ == 22;
}
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
* @return The maximizeConversions.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.MaximizeConversions getMaximizeConversions() {
if (schemeCase_ == 22) {
return (com.google.ads.googleads.v10.common.MaximizeConversions) scheme_;
}
return com.google.ads.googleads.v10.common.MaximizeConversions.getDefaultInstance();
}
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.MaximizeConversionsOrBuilder getMaximizeConversionsOrBuilder() {
if (schemeCase_ == 22) {
return (com.google.ads.googleads.v10.common.MaximizeConversions) scheme_;
}
return com.google.ads.googleads.v10.common.MaximizeConversions.getDefaultInstance();
}
public static final int TARGET_CPA_FIELD_NUMBER = 9;
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
* @return Whether the targetCpa field is set.
*/
@java.lang.Override
public boolean hasTargetCpa() {
return schemeCase_ == 9;
}
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
* @return The targetCpa.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetCpa getTargetCpa() {
if (schemeCase_ == 9) {
return (com.google.ads.googleads.v10.common.TargetCpa) scheme_;
}
return com.google.ads.googleads.v10.common.TargetCpa.getDefaultInstance();
}
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetCpaOrBuilder getTargetCpaOrBuilder() {
if (schemeCase_ == 9) {
return (com.google.ads.googleads.v10.common.TargetCpa) scheme_;
}
return com.google.ads.googleads.v10.common.TargetCpa.getDefaultInstance();
}
public static final int TARGET_IMPRESSION_SHARE_FIELD_NUMBER = 48;
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
* @return Whether the targetImpressionShare field is set.
*/
@java.lang.Override
public boolean hasTargetImpressionShare() {
return schemeCase_ == 48;
}
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
* @return The targetImpressionShare.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetImpressionShare getTargetImpressionShare() {
if (schemeCase_ == 48) {
return (com.google.ads.googleads.v10.common.TargetImpressionShare) scheme_;
}
return com.google.ads.googleads.v10.common.TargetImpressionShare.getDefaultInstance();
}
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetImpressionShareOrBuilder getTargetImpressionShareOrBuilder() {
if (schemeCase_ == 48) {
return (com.google.ads.googleads.v10.common.TargetImpressionShare) scheme_;
}
return com.google.ads.googleads.v10.common.TargetImpressionShare.getDefaultInstance();
}
public static final int TARGET_ROAS_FIELD_NUMBER = 11;
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
* @return Whether the targetRoas field is set.
*/
@java.lang.Override
public boolean hasTargetRoas() {
return schemeCase_ == 11;
}
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
* @return The targetRoas.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetRoas getTargetRoas() {
if (schemeCase_ == 11) {
return (com.google.ads.googleads.v10.common.TargetRoas) scheme_;
}
return com.google.ads.googleads.v10.common.TargetRoas.getDefaultInstance();
}
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetRoasOrBuilder getTargetRoasOrBuilder() {
if (schemeCase_ == 11) {
return (com.google.ads.googleads.v10.common.TargetRoas) scheme_;
}
return com.google.ads.googleads.v10.common.TargetRoas.getDefaultInstance();
}
public static final int TARGET_SPEND_FIELD_NUMBER = 12;
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
* @return Whether the targetSpend field is set.
*/
@java.lang.Override
public boolean hasTargetSpend() {
return schemeCase_ == 12;
}
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
* @return The targetSpend.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetSpend getTargetSpend() {
if (schemeCase_ == 12) {
return (com.google.ads.googleads.v10.common.TargetSpend) scheme_;
}
return com.google.ads.googleads.v10.common.TargetSpend.getDefaultInstance();
}
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetSpendOrBuilder getTargetSpendOrBuilder() {
if (schemeCase_ == 12) {
return (com.google.ads.googleads.v10.common.TargetSpend) scheme_;
}
return com.google.ads.googleads.v10.common.TargetSpend.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, resourceName_);
}
if (type_ != com.google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType.UNSPECIFIED.getNumber()) {
output.writeEnum(5, type_);
}
if (schemeCase_ == 7) {
output.writeMessage(7, (com.google.ads.googleads.v10.common.EnhancedCpc) scheme_);
}
if (schemeCase_ == 9) {
output.writeMessage(9, (com.google.ads.googleads.v10.common.TargetCpa) scheme_);
}
if (schemeCase_ == 11) {
output.writeMessage(11, (com.google.ads.googleads.v10.common.TargetRoas) scheme_);
}
if (schemeCase_ == 12) {
output.writeMessage(12, (com.google.ads.googleads.v10.common.TargetSpend) scheme_);
}
if (status_ != com.google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus.UNSPECIFIED.getNumber()) {
output.writeEnum(15, status_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(16, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, name_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt64(18, campaignCount_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt64(19, nonRemovedCampaignCount_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, effectiveCurrencyCode_);
}
if (schemeCase_ == 21) {
output.writeMessage(21, (com.google.ads.googleads.v10.common.MaximizeConversionValue) scheme_);
}
if (schemeCase_ == 22) {
output.writeMessage(22, (com.google.ads.googleads.v10.common.MaximizeConversions) scheme_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(currencyCode_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 23, currencyCode_);
}
if (schemeCase_ == 48) {
output.writeMessage(48, (com.google.ads.googleads.v10.common.TargetImpressionShare) scheme_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, resourceName_);
}
if (type_ != com.google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, type_);
}
if (schemeCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, (com.google.ads.googleads.v10.common.EnhancedCpc) scheme_);
}
if (schemeCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, (com.google.ads.googleads.v10.common.TargetCpa) scheme_);
}
if (schemeCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, (com.google.ads.googleads.v10.common.TargetRoas) scheme_);
}
if (schemeCase_ == 12) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, (com.google.ads.googleads.v10.common.TargetSpend) scheme_);
}
if (status_ != com.google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(15, status_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(16, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, name_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(18, campaignCount_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(19, nonRemovedCampaignCount_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(20, effectiveCurrencyCode_);
}
if (schemeCase_ == 21) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, (com.google.ads.googleads.v10.common.MaximizeConversionValue) scheme_);
}
if (schemeCase_ == 22) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(22, (com.google.ads.googleads.v10.common.MaximizeConversions) scheme_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(currencyCode_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(23, currencyCode_);
}
if (schemeCase_ == 48) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(48, (com.google.ads.googleads.v10.common.TargetImpressionShare) scheme_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v10.resources.BiddingStrategy)) {
return super.equals(obj);
}
com.google.ads.googleads.v10.resources.BiddingStrategy other = (com.google.ads.googleads.v10.resources.BiddingStrategy) obj;
if (!getResourceName()
.equals(other.getResourceName())) return false;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId()
!= other.getId()) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (status_ != other.status_) return false;
if (type_ != other.type_) return false;
if (!getCurrencyCode()
.equals(other.getCurrencyCode())) return false;
if (hasEffectiveCurrencyCode() != other.hasEffectiveCurrencyCode()) return false;
if (hasEffectiveCurrencyCode()) {
if (!getEffectiveCurrencyCode()
.equals(other.getEffectiveCurrencyCode())) return false;
}
if (hasCampaignCount() != other.hasCampaignCount()) return false;
if (hasCampaignCount()) {
if (getCampaignCount()
!= other.getCampaignCount()) return false;
}
if (hasNonRemovedCampaignCount() != other.hasNonRemovedCampaignCount()) return false;
if (hasNonRemovedCampaignCount()) {
if (getNonRemovedCampaignCount()
!= other.getNonRemovedCampaignCount()) return false;
}
if (!getSchemeCase().equals(other.getSchemeCase())) return false;
switch (schemeCase_) {
case 7:
if (!getEnhancedCpc()
.equals(other.getEnhancedCpc())) return false;
break;
case 21:
if (!getMaximizeConversionValue()
.equals(other.getMaximizeConversionValue())) return false;
break;
case 22:
if (!getMaximizeConversions()
.equals(other.getMaximizeConversions())) return false;
break;
case 9:
if (!getTargetCpa()
.equals(other.getTargetCpa())) return false;
break;
case 48:
if (!getTargetImpressionShare()
.equals(other.getTargetImpressionShare())) return false;
break;
case 11:
if (!getTargetRoas()
.equals(other.getTargetRoas())) return false;
break;
case 12:
if (!getTargetSpend()
.equals(other.getTargetSpend())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RESOURCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getResourceName().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getId());
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (37 * hash) + CURRENCY_CODE_FIELD_NUMBER;
hash = (53 * hash) + getCurrencyCode().hashCode();
if (hasEffectiveCurrencyCode()) {
hash = (37 * hash) + EFFECTIVE_CURRENCY_CODE_FIELD_NUMBER;
hash = (53 * hash) + getEffectiveCurrencyCode().hashCode();
}
if (hasCampaignCount()) {
hash = (37 * hash) + CAMPAIGN_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCampaignCount());
}
if (hasNonRemovedCampaignCount()) {
hash = (37 * hash) + NON_REMOVED_CAMPAIGN_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNonRemovedCampaignCount());
}
switch (schemeCase_) {
case 7:
hash = (37 * hash) + ENHANCED_CPC_FIELD_NUMBER;
hash = (53 * hash) + getEnhancedCpc().hashCode();
break;
case 21:
hash = (37 * hash) + MAXIMIZE_CONVERSION_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getMaximizeConversionValue().hashCode();
break;
case 22:
hash = (37 * hash) + MAXIMIZE_CONVERSIONS_FIELD_NUMBER;
hash = (53 * hash) + getMaximizeConversions().hashCode();
break;
case 9:
hash = (37 * hash) + TARGET_CPA_FIELD_NUMBER;
hash = (53 * hash) + getTargetCpa().hashCode();
break;
case 48:
hash = (37 * hash) + TARGET_IMPRESSION_SHARE_FIELD_NUMBER;
hash = (53 * hash) + getTargetImpressionShare().hashCode();
break;
case 11:
hash = (37 * hash) + TARGET_ROAS_FIELD_NUMBER;
hash = (53 * hash) + getTargetRoas().hashCode();
break;
case 12:
hash = (37 * hash) + TARGET_SPEND_FIELD_NUMBER;
hash = (53 * hash) + getTargetSpend().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v10.resources.BiddingStrategy prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A bidding strategy.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.BiddingStrategy}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v10.resources.BiddingStrategy)
com.google.ads.googleads.v10.resources.BiddingStrategyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.BiddingStrategyProto.internal_static_google_ads_googleads_v10_resources_BiddingStrategy_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.BiddingStrategyProto.internal_static_google_ads_googleads_v10_resources_BiddingStrategy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.BiddingStrategy.class, com.google.ads.googleads.v10.resources.BiddingStrategy.Builder.class);
}
// Construct using com.google.ads.googleads.v10.resources.BiddingStrategy.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
resourceName_ = "";
id_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
status_ = 0;
type_ = 0;
currencyCode_ = "";
effectiveCurrencyCode_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
campaignCount_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
nonRemovedCampaignCount_ = 0L;
bitField0_ = (bitField0_ & ~0x00000010);
if (enhancedCpcBuilder_ != null) {
enhancedCpcBuilder_.clear();
}
if (maximizeConversionValueBuilder_ != null) {
maximizeConversionValueBuilder_.clear();
}
if (maximizeConversionsBuilder_ != null) {
maximizeConversionsBuilder_.clear();
}
if (targetCpaBuilder_ != null) {
targetCpaBuilder_.clear();
}
if (targetImpressionShareBuilder_ != null) {
targetImpressionShareBuilder_.clear();
}
if (targetRoasBuilder_ != null) {
targetRoasBuilder_.clear();
}
if (targetSpendBuilder_ != null) {
targetSpendBuilder_.clear();
}
schemeCase_ = 0;
scheme_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v10.resources.BiddingStrategyProto.internal_static_google_ads_googleads_v10_resources_BiddingStrategy_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.BiddingStrategy getDefaultInstanceForType() {
return com.google.ads.googleads.v10.resources.BiddingStrategy.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.BiddingStrategy build() {
com.google.ads.googleads.v10.resources.BiddingStrategy result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.BiddingStrategy buildPartial() {
com.google.ads.googleads.v10.resources.BiddingStrategy result = new com.google.ads.googleads.v10.resources.BiddingStrategy(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.resourceName_ = resourceName_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
result.status_ = status_;
result.type_ = type_;
result.currencyCode_ = currencyCode_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.effectiveCurrencyCode_ = effectiveCurrencyCode_;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.campaignCount_ = campaignCount_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.nonRemovedCampaignCount_ = nonRemovedCampaignCount_;
to_bitField0_ |= 0x00000010;
}
if (schemeCase_ == 7) {
if (enhancedCpcBuilder_ == null) {
result.scheme_ = scheme_;
} else {
result.scheme_ = enhancedCpcBuilder_.build();
}
}
if (schemeCase_ == 21) {
if (maximizeConversionValueBuilder_ == null) {
result.scheme_ = scheme_;
} else {
result.scheme_ = maximizeConversionValueBuilder_.build();
}
}
if (schemeCase_ == 22) {
if (maximizeConversionsBuilder_ == null) {
result.scheme_ = scheme_;
} else {
result.scheme_ = maximizeConversionsBuilder_.build();
}
}
if (schemeCase_ == 9) {
if (targetCpaBuilder_ == null) {
result.scheme_ = scheme_;
} else {
result.scheme_ = targetCpaBuilder_.build();
}
}
if (schemeCase_ == 48) {
if (targetImpressionShareBuilder_ == null) {
result.scheme_ = scheme_;
} else {
result.scheme_ = targetImpressionShareBuilder_.build();
}
}
if (schemeCase_ == 11) {
if (targetRoasBuilder_ == null) {
result.scheme_ = scheme_;
} else {
result.scheme_ = targetRoasBuilder_.build();
}
}
if (schemeCase_ == 12) {
if (targetSpendBuilder_ == null) {
result.scheme_ = scheme_;
} else {
result.scheme_ = targetSpendBuilder_.build();
}
}
result.bitField0_ = to_bitField0_;
result.schemeCase_ = schemeCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v10.resources.BiddingStrategy) {
return mergeFrom((com.google.ads.googleads.v10.resources.BiddingStrategy)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v10.resources.BiddingStrategy other) {
if (other == com.google.ads.googleads.v10.resources.BiddingStrategy.getDefaultInstance()) return this;
if (!other.getResourceName().isEmpty()) {
resourceName_ = other.resourceName_;
onChanged();
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (!other.getCurrencyCode().isEmpty()) {
currencyCode_ = other.currencyCode_;
onChanged();
}
if (other.hasEffectiveCurrencyCode()) {
bitField0_ |= 0x00000004;
effectiveCurrencyCode_ = other.effectiveCurrencyCode_;
onChanged();
}
if (other.hasCampaignCount()) {
setCampaignCount(other.getCampaignCount());
}
if (other.hasNonRemovedCampaignCount()) {
setNonRemovedCampaignCount(other.getNonRemovedCampaignCount());
}
switch (other.getSchemeCase()) {
case ENHANCED_CPC: {
mergeEnhancedCpc(other.getEnhancedCpc());
break;
}
case MAXIMIZE_CONVERSION_VALUE: {
mergeMaximizeConversionValue(other.getMaximizeConversionValue());
break;
}
case MAXIMIZE_CONVERSIONS: {
mergeMaximizeConversions(other.getMaximizeConversions());
break;
}
case TARGET_CPA: {
mergeTargetCpa(other.getTargetCpa());
break;
}
case TARGET_IMPRESSION_SHARE: {
mergeTargetImpressionShare(other.getTargetImpressionShare());
break;
}
case TARGET_ROAS: {
mergeTargetRoas(other.getTargetRoas());
break;
}
case TARGET_SPEND: {
mergeTargetSpend(other.getTargetSpend());
break;
}
case SCHEME_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
resourceName_ = input.readStringRequireUtf8();
break;
} // case 10
case 40: {
type_ = input.readEnum();
break;
} // case 40
case 58: {
input.readMessage(
getEnhancedCpcFieldBuilder().getBuilder(),
extensionRegistry);
schemeCase_ = 7;
break;
} // case 58
case 74: {
input.readMessage(
getTargetCpaFieldBuilder().getBuilder(),
extensionRegistry);
schemeCase_ = 9;
break;
} // case 74
case 90: {
input.readMessage(
getTargetRoasFieldBuilder().getBuilder(),
extensionRegistry);
schemeCase_ = 11;
break;
} // case 90
case 98: {
input.readMessage(
getTargetSpendFieldBuilder().getBuilder(),
extensionRegistry);
schemeCase_ = 12;
break;
} // case 98
case 120: {
status_ = input.readEnum();
break;
} // case 120
case 128: {
id_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 128
case 138: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 138
case 144: {
campaignCount_ = input.readInt64();
bitField0_ |= 0x00000008;
break;
} // case 144
case 152: {
nonRemovedCampaignCount_ = input.readInt64();
bitField0_ |= 0x00000010;
break;
} // case 152
case 162: {
effectiveCurrencyCode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 162
case 170: {
input.readMessage(
getMaximizeConversionValueFieldBuilder().getBuilder(),
extensionRegistry);
schemeCase_ = 21;
break;
} // case 170
case 178: {
input.readMessage(
getMaximizeConversionsFieldBuilder().getBuilder(),
extensionRegistry);
schemeCase_ = 22;
break;
} // case 178
case 186: {
currencyCode_ = input.readStringRequireUtf8();
break;
} // case 186
case 386: {
input.readMessage(
getTargetImpressionShareFieldBuilder().getBuilder(),
extensionRegistry);
schemeCase_ = 48;
break;
} // case 386
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int schemeCase_ = 0;
private java.lang.Object scheme_;
public SchemeCase
getSchemeCase() {
return SchemeCase.forNumber(
schemeCase_);
}
public Builder clearScheme() {
schemeCase_ = 0;
scheme_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object resourceName_ = "";
/**
*
* Immutable. The resource name of the bidding strategy.
* Bidding strategy resource names have the form:
* `customers/{customer_id}/biddingStrategies/{bidding_strategy_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The resource name of the bidding strategy.
* Bidding strategy resource names have the form:
* `customers/{customer_id}/biddingStrategies/{bidding_strategy_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The resource name of the bidding strategy.
* Bidding strategy resource names have the form:
* `customers/{customer_id}/biddingStrategies/{bidding_strategy_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resourceName_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the bidding strategy.
* Bidding strategy resource names have the form:
* `customers/{customer_id}/biddingStrategies/{bidding_strategy_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearResourceName() {
resourceName_ = getDefaultInstance().getResourceName();
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the bidding strategy.
* Bidding strategy resource names have the form:
* `customers/{customer_id}/biddingStrategies/{bidding_strategy_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The bytes for resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resourceName_ = value;
onChanged();
return this;
}
private long id_ ;
/**
*
* Output only. The ID of the bidding strategy.
*
*
* optional int64 id = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The ID of the bidding strategy.
*
*
* optional int64 id = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
*
* Output only. The ID of the bidding strategy.
*
*
* optional int64 id = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
*
* Output only. The ID of the bidding strategy.
*
*
* optional int64 id = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* The name of the bidding strategy.
* All bidding strategies within an account must be named distinctly.
* The length of this string should be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 17;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The name of the bidding strategy.
* All bidding strategies within an account must be named distinctly.
* The length of this string should be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 17;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the bidding strategy.
* All bidding strategies within an account must be named distinctly.
* The length of this string should be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 17;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the bidding strategy.
* All bidding strategies within an account must be named distinctly.
* The length of this string should be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 17;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
*
* The name of the bidding strategy.
* All bidding strategies within an account must be named distinctly.
* The length of this string should be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 17;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* The name of the bidding strategy.
* All bidding strategies within an account must be named distinctly.
* The length of this string should be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 17;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Output only. The status of the bidding strategy.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Output only. The status of the bidding strategy.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Output only. The status of the bidding strategy.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The status.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus getStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus result = com.google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus.valueOf(status_);
return result == null ? com.google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus.UNRECOGNIZED : result;
}
/**
*
* Output only. The status of the bidding strategy.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The status of the bidding strategy.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyStatusEnum.BiddingStrategyStatus status = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private int type_ = 0;
/**
*
* Output only. The type of the bidding strategy.
* Create a bidding strategy by setting the bidding scheme.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType type = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
*
* Output only. The type of the bidding strategy.
* Create a bidding strategy by setting the bidding scheme.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType type = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
* Output only. The type of the bidding strategy.
* Create a bidding strategy by setting the bidding scheme.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType type = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The type.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType getType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType result = com.google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType.valueOf(type_);
return result == null ? com.google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType.UNRECOGNIZED : result;
}
/**
*
* Output only. The type of the bidding strategy.
* Create a bidding strategy by setting the bidding scheme.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType type = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(com.google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The type of the bidding strategy.
* Create a bidding strategy by setting the bidding scheme.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.BiddingStrategyTypeEnum.BiddingStrategyType type = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private java.lang.Object currencyCode_ = "";
/**
*
* Immutable. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this currency can be set on
* creation and defaults to the manager customer's currency. For serving
* customers, this field cannot be set; all strategies in a serving customer
* implicitly use the serving customer's currency. In all cases the
* effective_currency_code field returns the currency used by the strategy.
*
*
* string currency_code = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return The currencyCode.
*/
public java.lang.String getCurrencyCode() {
java.lang.Object ref = currencyCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currencyCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this currency can be set on
* creation and defaults to the manager customer's currency. For serving
* customers, this field cannot be set; all strategies in a serving customer
* implicitly use the serving customer's currency. In all cases the
* effective_currency_code field returns the currency used by the strategy.
*
*
* string currency_code = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return The bytes for currencyCode.
*/
public com.google.protobuf.ByteString
getCurrencyCodeBytes() {
java.lang.Object ref = currencyCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this currency can be set on
* creation and defaults to the manager customer's currency. For serving
* customers, this field cannot be set; all strategies in a serving customer
* implicitly use the serving customer's currency. In all cases the
* effective_currency_code field returns the currency used by the strategy.
*
*
* string currency_code = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The currencyCode to set.
* @return This builder for chaining.
*/
public Builder setCurrencyCode(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
currencyCode_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this currency can be set on
* creation and defaults to the manager customer's currency. For serving
* customers, this field cannot be set; all strategies in a serving customer
* implicitly use the serving customer's currency. In all cases the
* effective_currency_code field returns the currency used by the strategy.
*
*
* string currency_code = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearCurrencyCode() {
currencyCode_ = getDefaultInstance().getCurrencyCode();
onChanged();
return this;
}
/**
*
* Immutable. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this currency can be set on
* creation and defaults to the manager customer's currency. For serving
* customers, this field cannot be set; all strategies in a serving customer
* implicitly use the serving customer's currency. In all cases the
* effective_currency_code field returns the currency used by the strategy.
*
*
* string currency_code = 23 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The bytes for currencyCode to set.
* @return This builder for chaining.
*/
public Builder setCurrencyCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
currencyCode_ = value;
onChanged();
return this;
}
private java.lang.Object effectiveCurrencyCode_ = "";
/**
*
* Output only. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this is the currency set by
* the advertiser when creating the strategy. For serving customers, this is
* the customer's currency_code.
* Bidding strategy metrics are reported in this currency.
* This field is read-only.
*
*
* optional string effective_currency_code = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the effectiveCurrencyCode field is set.
*/
public boolean hasEffectiveCurrencyCode() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this is the currency set by
* the advertiser when creating the strategy. For serving customers, this is
* the customer's currency_code.
* Bidding strategy metrics are reported in this currency.
* This field is read-only.
*
*
* optional string effective_currency_code = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The effectiveCurrencyCode.
*/
public java.lang.String getEffectiveCurrencyCode() {
java.lang.Object ref = effectiveCurrencyCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
effectiveCurrencyCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this is the currency set by
* the advertiser when creating the strategy. For serving customers, this is
* the customer's currency_code.
* Bidding strategy metrics are reported in this currency.
* This field is read-only.
*
*
* optional string effective_currency_code = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for effectiveCurrencyCode.
*/
public com.google.protobuf.ByteString
getEffectiveCurrencyCodeBytes() {
java.lang.Object ref = effectiveCurrencyCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
effectiveCurrencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this is the currency set by
* the advertiser when creating the strategy. For serving customers, this is
* the customer's currency_code.
* Bidding strategy metrics are reported in this currency.
* This field is read-only.
*
*
* optional string effective_currency_code = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The effectiveCurrencyCode to set.
* @return This builder for chaining.
*/
public Builder setEffectiveCurrencyCode(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
effectiveCurrencyCode_ = value;
onChanged();
return this;
}
/**
*
* Output only. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this is the currency set by
* the advertiser when creating the strategy. For serving customers, this is
* the customer's currency_code.
* Bidding strategy metrics are reported in this currency.
* This field is read-only.
*
*
* optional string effective_currency_code = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearEffectiveCurrencyCode() {
bitField0_ = (bitField0_ & ~0x00000004);
effectiveCurrencyCode_ = getDefaultInstance().getEffectiveCurrencyCode();
onChanged();
return this;
}
/**
*
* Output only. The currency used by the bidding strategy (ISO 4217 three-letter code).
* For bidding strategies in manager customers, this is the currency set by
* the advertiser when creating the strategy. For serving customers, this is
* the customer's currency_code.
* Bidding strategy metrics are reported in this currency.
* This field is read-only.
*
*
* optional string effective_currency_code = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for effectiveCurrencyCode to set.
* @return This builder for chaining.
*/
public Builder setEffectiveCurrencyCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000004;
effectiveCurrencyCode_ = value;
onChanged();
return this;
}
private long campaignCount_ ;
/**
*
* Output only. The number of campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 campaign_count = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the campaignCount field is set.
*/
@java.lang.Override
public boolean hasCampaignCount() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. The number of campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 campaign_count = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The campaignCount.
*/
@java.lang.Override
public long getCampaignCount() {
return campaignCount_;
}
/**
*
* Output only. The number of campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 campaign_count = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The campaignCount to set.
* @return This builder for chaining.
*/
public Builder setCampaignCount(long value) {
bitField0_ |= 0x00000008;
campaignCount_ = value;
onChanged();
return this;
}
/**
*
* Output only. The number of campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 campaign_count = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearCampaignCount() {
bitField0_ = (bitField0_ & ~0x00000008);
campaignCount_ = 0L;
onChanged();
return this;
}
private long nonRemovedCampaignCount_ ;
/**
*
* Output only. The number of non-removed campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 non_removed_campaign_count = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the nonRemovedCampaignCount field is set.
*/
@java.lang.Override
public boolean hasNonRemovedCampaignCount() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The number of non-removed campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 non_removed_campaign_count = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The nonRemovedCampaignCount.
*/
@java.lang.Override
public long getNonRemovedCampaignCount() {
return nonRemovedCampaignCount_;
}
/**
*
* Output only. The number of non-removed campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 non_removed_campaign_count = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The nonRemovedCampaignCount to set.
* @return This builder for chaining.
*/
public Builder setNonRemovedCampaignCount(long value) {
bitField0_ |= 0x00000010;
nonRemovedCampaignCount_ = value;
onChanged();
return this;
}
/**
*
* Output only. The number of non-removed campaigns attached to this bidding strategy.
* This field is read-only.
*
*
* optional int64 non_removed_campaign_count = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearNonRemovedCampaignCount() {
bitField0_ = (bitField0_ & ~0x00000010);
nonRemovedCampaignCount_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.EnhancedCpc, com.google.ads.googleads.v10.common.EnhancedCpc.Builder, com.google.ads.googleads.v10.common.EnhancedCpcOrBuilder> enhancedCpcBuilder_;
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
* @return Whether the enhancedCpc field is set.
*/
@java.lang.Override
public boolean hasEnhancedCpc() {
return schemeCase_ == 7;
}
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
* @return The enhancedCpc.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.EnhancedCpc getEnhancedCpc() {
if (enhancedCpcBuilder_ == null) {
if (schemeCase_ == 7) {
return (com.google.ads.googleads.v10.common.EnhancedCpc) scheme_;
}
return com.google.ads.googleads.v10.common.EnhancedCpc.getDefaultInstance();
} else {
if (schemeCase_ == 7) {
return enhancedCpcBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.EnhancedCpc.getDefaultInstance();
}
}
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
*/
public Builder setEnhancedCpc(com.google.ads.googleads.v10.common.EnhancedCpc value) {
if (enhancedCpcBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scheme_ = value;
onChanged();
} else {
enhancedCpcBuilder_.setMessage(value);
}
schemeCase_ = 7;
return this;
}
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
*/
public Builder setEnhancedCpc(
com.google.ads.googleads.v10.common.EnhancedCpc.Builder builderForValue) {
if (enhancedCpcBuilder_ == null) {
scheme_ = builderForValue.build();
onChanged();
} else {
enhancedCpcBuilder_.setMessage(builderForValue.build());
}
schemeCase_ = 7;
return this;
}
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
*/
public Builder mergeEnhancedCpc(com.google.ads.googleads.v10.common.EnhancedCpc value) {
if (enhancedCpcBuilder_ == null) {
if (schemeCase_ == 7 &&
scheme_ != com.google.ads.googleads.v10.common.EnhancedCpc.getDefaultInstance()) {
scheme_ = com.google.ads.googleads.v10.common.EnhancedCpc.newBuilder((com.google.ads.googleads.v10.common.EnhancedCpc) scheme_)
.mergeFrom(value).buildPartial();
} else {
scheme_ = value;
}
onChanged();
} else {
if (schemeCase_ == 7) {
enhancedCpcBuilder_.mergeFrom(value);
} else {
enhancedCpcBuilder_.setMessage(value);
}
}
schemeCase_ = 7;
return this;
}
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
*/
public Builder clearEnhancedCpc() {
if (enhancedCpcBuilder_ == null) {
if (schemeCase_ == 7) {
schemeCase_ = 0;
scheme_ = null;
onChanged();
}
} else {
if (schemeCase_ == 7) {
schemeCase_ = 0;
scheme_ = null;
}
enhancedCpcBuilder_.clear();
}
return this;
}
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
*/
public com.google.ads.googleads.v10.common.EnhancedCpc.Builder getEnhancedCpcBuilder() {
return getEnhancedCpcFieldBuilder().getBuilder();
}
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.EnhancedCpcOrBuilder getEnhancedCpcOrBuilder() {
if ((schemeCase_ == 7) && (enhancedCpcBuilder_ != null)) {
return enhancedCpcBuilder_.getMessageOrBuilder();
} else {
if (schemeCase_ == 7) {
return (com.google.ads.googleads.v10.common.EnhancedCpc) scheme_;
}
return com.google.ads.googleads.v10.common.EnhancedCpc.getDefaultInstance();
}
}
/**
*
* A bidding strategy that raises bids for clicks that seem more likely to
* lead to a conversion and lowers them for clicks where they seem less
* likely.
*
*
* .google.ads.googleads.v10.common.EnhancedCpc enhanced_cpc = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.EnhancedCpc, com.google.ads.googleads.v10.common.EnhancedCpc.Builder, com.google.ads.googleads.v10.common.EnhancedCpcOrBuilder>
getEnhancedCpcFieldBuilder() {
if (enhancedCpcBuilder_ == null) {
if (!(schemeCase_ == 7)) {
scheme_ = com.google.ads.googleads.v10.common.EnhancedCpc.getDefaultInstance();
}
enhancedCpcBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.EnhancedCpc, com.google.ads.googleads.v10.common.EnhancedCpc.Builder, com.google.ads.googleads.v10.common.EnhancedCpcOrBuilder>(
(com.google.ads.googleads.v10.common.EnhancedCpc) scheme_,
getParentForChildren(),
isClean());
scheme_ = null;
}
schemeCase_ = 7;
onChanged();;
return enhancedCpcBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.MaximizeConversionValue, com.google.ads.googleads.v10.common.MaximizeConversionValue.Builder, com.google.ads.googleads.v10.common.MaximizeConversionValueOrBuilder> maximizeConversionValueBuilder_;
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
* @return Whether the maximizeConversionValue field is set.
*/
@java.lang.Override
public boolean hasMaximizeConversionValue() {
return schemeCase_ == 21;
}
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
* @return The maximizeConversionValue.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.MaximizeConversionValue getMaximizeConversionValue() {
if (maximizeConversionValueBuilder_ == null) {
if (schemeCase_ == 21) {
return (com.google.ads.googleads.v10.common.MaximizeConversionValue) scheme_;
}
return com.google.ads.googleads.v10.common.MaximizeConversionValue.getDefaultInstance();
} else {
if (schemeCase_ == 21) {
return maximizeConversionValueBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.MaximizeConversionValue.getDefaultInstance();
}
}
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
*/
public Builder setMaximizeConversionValue(com.google.ads.googleads.v10.common.MaximizeConversionValue value) {
if (maximizeConversionValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scheme_ = value;
onChanged();
} else {
maximizeConversionValueBuilder_.setMessage(value);
}
schemeCase_ = 21;
return this;
}
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
*/
public Builder setMaximizeConversionValue(
com.google.ads.googleads.v10.common.MaximizeConversionValue.Builder builderForValue) {
if (maximizeConversionValueBuilder_ == null) {
scheme_ = builderForValue.build();
onChanged();
} else {
maximizeConversionValueBuilder_.setMessage(builderForValue.build());
}
schemeCase_ = 21;
return this;
}
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
*/
public Builder mergeMaximizeConversionValue(com.google.ads.googleads.v10.common.MaximizeConversionValue value) {
if (maximizeConversionValueBuilder_ == null) {
if (schemeCase_ == 21 &&
scheme_ != com.google.ads.googleads.v10.common.MaximizeConversionValue.getDefaultInstance()) {
scheme_ = com.google.ads.googleads.v10.common.MaximizeConversionValue.newBuilder((com.google.ads.googleads.v10.common.MaximizeConversionValue) scheme_)
.mergeFrom(value).buildPartial();
} else {
scheme_ = value;
}
onChanged();
} else {
if (schemeCase_ == 21) {
maximizeConversionValueBuilder_.mergeFrom(value);
} else {
maximizeConversionValueBuilder_.setMessage(value);
}
}
schemeCase_ = 21;
return this;
}
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
*/
public Builder clearMaximizeConversionValue() {
if (maximizeConversionValueBuilder_ == null) {
if (schemeCase_ == 21) {
schemeCase_ = 0;
scheme_ = null;
onChanged();
}
} else {
if (schemeCase_ == 21) {
schemeCase_ = 0;
scheme_ = null;
}
maximizeConversionValueBuilder_.clear();
}
return this;
}
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
*/
public com.google.ads.googleads.v10.common.MaximizeConversionValue.Builder getMaximizeConversionValueBuilder() {
return getMaximizeConversionValueFieldBuilder().getBuilder();
}
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.MaximizeConversionValueOrBuilder getMaximizeConversionValueOrBuilder() {
if ((schemeCase_ == 21) && (maximizeConversionValueBuilder_ != null)) {
return maximizeConversionValueBuilder_.getMessageOrBuilder();
} else {
if (schemeCase_ == 21) {
return (com.google.ads.googleads.v10.common.MaximizeConversionValue) scheme_;
}
return com.google.ads.googleads.v10.common.MaximizeConversionValue.getDefaultInstance();
}
}
/**
*
* An automated bidding strategy to help get the most conversion value for
* your campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversionValue maximize_conversion_value = 21;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.MaximizeConversionValue, com.google.ads.googleads.v10.common.MaximizeConversionValue.Builder, com.google.ads.googleads.v10.common.MaximizeConversionValueOrBuilder>
getMaximizeConversionValueFieldBuilder() {
if (maximizeConversionValueBuilder_ == null) {
if (!(schemeCase_ == 21)) {
scheme_ = com.google.ads.googleads.v10.common.MaximizeConversionValue.getDefaultInstance();
}
maximizeConversionValueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.MaximizeConversionValue, com.google.ads.googleads.v10.common.MaximizeConversionValue.Builder, com.google.ads.googleads.v10.common.MaximizeConversionValueOrBuilder>(
(com.google.ads.googleads.v10.common.MaximizeConversionValue) scheme_,
getParentForChildren(),
isClean());
scheme_ = null;
}
schemeCase_ = 21;
onChanged();;
return maximizeConversionValueBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.MaximizeConversions, com.google.ads.googleads.v10.common.MaximizeConversions.Builder, com.google.ads.googleads.v10.common.MaximizeConversionsOrBuilder> maximizeConversionsBuilder_;
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
* @return Whether the maximizeConversions field is set.
*/
@java.lang.Override
public boolean hasMaximizeConversions() {
return schemeCase_ == 22;
}
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
* @return The maximizeConversions.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.MaximizeConversions getMaximizeConversions() {
if (maximizeConversionsBuilder_ == null) {
if (schemeCase_ == 22) {
return (com.google.ads.googleads.v10.common.MaximizeConversions) scheme_;
}
return com.google.ads.googleads.v10.common.MaximizeConversions.getDefaultInstance();
} else {
if (schemeCase_ == 22) {
return maximizeConversionsBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.MaximizeConversions.getDefaultInstance();
}
}
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
*/
public Builder setMaximizeConversions(com.google.ads.googleads.v10.common.MaximizeConversions value) {
if (maximizeConversionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scheme_ = value;
onChanged();
} else {
maximizeConversionsBuilder_.setMessage(value);
}
schemeCase_ = 22;
return this;
}
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
*/
public Builder setMaximizeConversions(
com.google.ads.googleads.v10.common.MaximizeConversions.Builder builderForValue) {
if (maximizeConversionsBuilder_ == null) {
scheme_ = builderForValue.build();
onChanged();
} else {
maximizeConversionsBuilder_.setMessage(builderForValue.build());
}
schemeCase_ = 22;
return this;
}
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
*/
public Builder mergeMaximizeConversions(com.google.ads.googleads.v10.common.MaximizeConversions value) {
if (maximizeConversionsBuilder_ == null) {
if (schemeCase_ == 22 &&
scheme_ != com.google.ads.googleads.v10.common.MaximizeConversions.getDefaultInstance()) {
scheme_ = com.google.ads.googleads.v10.common.MaximizeConversions.newBuilder((com.google.ads.googleads.v10.common.MaximizeConversions) scheme_)
.mergeFrom(value).buildPartial();
} else {
scheme_ = value;
}
onChanged();
} else {
if (schemeCase_ == 22) {
maximizeConversionsBuilder_.mergeFrom(value);
} else {
maximizeConversionsBuilder_.setMessage(value);
}
}
schemeCase_ = 22;
return this;
}
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
*/
public Builder clearMaximizeConversions() {
if (maximizeConversionsBuilder_ == null) {
if (schemeCase_ == 22) {
schemeCase_ = 0;
scheme_ = null;
onChanged();
}
} else {
if (schemeCase_ == 22) {
schemeCase_ = 0;
scheme_ = null;
}
maximizeConversionsBuilder_.clear();
}
return this;
}
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
*/
public com.google.ads.googleads.v10.common.MaximizeConversions.Builder getMaximizeConversionsBuilder() {
return getMaximizeConversionsFieldBuilder().getBuilder();
}
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.MaximizeConversionsOrBuilder getMaximizeConversionsOrBuilder() {
if ((schemeCase_ == 22) && (maximizeConversionsBuilder_ != null)) {
return maximizeConversionsBuilder_.getMessageOrBuilder();
} else {
if (schemeCase_ == 22) {
return (com.google.ads.googleads.v10.common.MaximizeConversions) scheme_;
}
return com.google.ads.googleads.v10.common.MaximizeConversions.getDefaultInstance();
}
}
/**
*
* An automated bidding strategy to help get the most conversions for your
* campaigns while spending your budget.
*
*
* .google.ads.googleads.v10.common.MaximizeConversions maximize_conversions = 22;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.MaximizeConversions, com.google.ads.googleads.v10.common.MaximizeConversions.Builder, com.google.ads.googleads.v10.common.MaximizeConversionsOrBuilder>
getMaximizeConversionsFieldBuilder() {
if (maximizeConversionsBuilder_ == null) {
if (!(schemeCase_ == 22)) {
scheme_ = com.google.ads.googleads.v10.common.MaximizeConversions.getDefaultInstance();
}
maximizeConversionsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.MaximizeConversions, com.google.ads.googleads.v10.common.MaximizeConversions.Builder, com.google.ads.googleads.v10.common.MaximizeConversionsOrBuilder>(
(com.google.ads.googleads.v10.common.MaximizeConversions) scheme_,
getParentForChildren(),
isClean());
scheme_ = null;
}
schemeCase_ = 22;
onChanged();;
return maximizeConversionsBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetCpa, com.google.ads.googleads.v10.common.TargetCpa.Builder, com.google.ads.googleads.v10.common.TargetCpaOrBuilder> targetCpaBuilder_;
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
* @return Whether the targetCpa field is set.
*/
@java.lang.Override
public boolean hasTargetCpa() {
return schemeCase_ == 9;
}
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
* @return The targetCpa.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetCpa getTargetCpa() {
if (targetCpaBuilder_ == null) {
if (schemeCase_ == 9) {
return (com.google.ads.googleads.v10.common.TargetCpa) scheme_;
}
return com.google.ads.googleads.v10.common.TargetCpa.getDefaultInstance();
} else {
if (schemeCase_ == 9) {
return targetCpaBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.TargetCpa.getDefaultInstance();
}
}
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
*/
public Builder setTargetCpa(com.google.ads.googleads.v10.common.TargetCpa value) {
if (targetCpaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scheme_ = value;
onChanged();
} else {
targetCpaBuilder_.setMessage(value);
}
schemeCase_ = 9;
return this;
}
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
*/
public Builder setTargetCpa(
com.google.ads.googleads.v10.common.TargetCpa.Builder builderForValue) {
if (targetCpaBuilder_ == null) {
scheme_ = builderForValue.build();
onChanged();
} else {
targetCpaBuilder_.setMessage(builderForValue.build());
}
schemeCase_ = 9;
return this;
}
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
*/
public Builder mergeTargetCpa(com.google.ads.googleads.v10.common.TargetCpa value) {
if (targetCpaBuilder_ == null) {
if (schemeCase_ == 9 &&
scheme_ != com.google.ads.googleads.v10.common.TargetCpa.getDefaultInstance()) {
scheme_ = com.google.ads.googleads.v10.common.TargetCpa.newBuilder((com.google.ads.googleads.v10.common.TargetCpa) scheme_)
.mergeFrom(value).buildPartial();
} else {
scheme_ = value;
}
onChanged();
} else {
if (schemeCase_ == 9) {
targetCpaBuilder_.mergeFrom(value);
} else {
targetCpaBuilder_.setMessage(value);
}
}
schemeCase_ = 9;
return this;
}
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
*/
public Builder clearTargetCpa() {
if (targetCpaBuilder_ == null) {
if (schemeCase_ == 9) {
schemeCase_ = 0;
scheme_ = null;
onChanged();
}
} else {
if (schemeCase_ == 9) {
schemeCase_ = 0;
scheme_ = null;
}
targetCpaBuilder_.clear();
}
return this;
}
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
*/
public com.google.ads.googleads.v10.common.TargetCpa.Builder getTargetCpaBuilder() {
return getTargetCpaFieldBuilder().getBuilder();
}
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetCpaOrBuilder getTargetCpaOrBuilder() {
if ((schemeCase_ == 9) && (targetCpaBuilder_ != null)) {
return targetCpaBuilder_.getMessageOrBuilder();
} else {
if (schemeCase_ == 9) {
return (com.google.ads.googleads.v10.common.TargetCpa) scheme_;
}
return com.google.ads.googleads.v10.common.TargetCpa.getDefaultInstance();
}
}
/**
*
* A bidding strategy that sets bids to help get as many conversions as
* possible at the target cost-per-acquisition (CPA) you set.
*
*
* .google.ads.googleads.v10.common.TargetCpa target_cpa = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetCpa, com.google.ads.googleads.v10.common.TargetCpa.Builder, com.google.ads.googleads.v10.common.TargetCpaOrBuilder>
getTargetCpaFieldBuilder() {
if (targetCpaBuilder_ == null) {
if (!(schemeCase_ == 9)) {
scheme_ = com.google.ads.googleads.v10.common.TargetCpa.getDefaultInstance();
}
targetCpaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetCpa, com.google.ads.googleads.v10.common.TargetCpa.Builder, com.google.ads.googleads.v10.common.TargetCpaOrBuilder>(
(com.google.ads.googleads.v10.common.TargetCpa) scheme_,
getParentForChildren(),
isClean());
scheme_ = null;
}
schemeCase_ = 9;
onChanged();;
return targetCpaBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetImpressionShare, com.google.ads.googleads.v10.common.TargetImpressionShare.Builder, com.google.ads.googleads.v10.common.TargetImpressionShareOrBuilder> targetImpressionShareBuilder_;
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
* @return Whether the targetImpressionShare field is set.
*/
@java.lang.Override
public boolean hasTargetImpressionShare() {
return schemeCase_ == 48;
}
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
* @return The targetImpressionShare.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetImpressionShare getTargetImpressionShare() {
if (targetImpressionShareBuilder_ == null) {
if (schemeCase_ == 48) {
return (com.google.ads.googleads.v10.common.TargetImpressionShare) scheme_;
}
return com.google.ads.googleads.v10.common.TargetImpressionShare.getDefaultInstance();
} else {
if (schemeCase_ == 48) {
return targetImpressionShareBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.TargetImpressionShare.getDefaultInstance();
}
}
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
*/
public Builder setTargetImpressionShare(com.google.ads.googleads.v10.common.TargetImpressionShare value) {
if (targetImpressionShareBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scheme_ = value;
onChanged();
} else {
targetImpressionShareBuilder_.setMessage(value);
}
schemeCase_ = 48;
return this;
}
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
*/
public Builder setTargetImpressionShare(
com.google.ads.googleads.v10.common.TargetImpressionShare.Builder builderForValue) {
if (targetImpressionShareBuilder_ == null) {
scheme_ = builderForValue.build();
onChanged();
} else {
targetImpressionShareBuilder_.setMessage(builderForValue.build());
}
schemeCase_ = 48;
return this;
}
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
*/
public Builder mergeTargetImpressionShare(com.google.ads.googleads.v10.common.TargetImpressionShare value) {
if (targetImpressionShareBuilder_ == null) {
if (schemeCase_ == 48 &&
scheme_ != com.google.ads.googleads.v10.common.TargetImpressionShare.getDefaultInstance()) {
scheme_ = com.google.ads.googleads.v10.common.TargetImpressionShare.newBuilder((com.google.ads.googleads.v10.common.TargetImpressionShare) scheme_)
.mergeFrom(value).buildPartial();
} else {
scheme_ = value;
}
onChanged();
} else {
if (schemeCase_ == 48) {
targetImpressionShareBuilder_.mergeFrom(value);
} else {
targetImpressionShareBuilder_.setMessage(value);
}
}
schemeCase_ = 48;
return this;
}
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
*/
public Builder clearTargetImpressionShare() {
if (targetImpressionShareBuilder_ == null) {
if (schemeCase_ == 48) {
schemeCase_ = 0;
scheme_ = null;
onChanged();
}
} else {
if (schemeCase_ == 48) {
schemeCase_ = 0;
scheme_ = null;
}
targetImpressionShareBuilder_.clear();
}
return this;
}
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
*/
public com.google.ads.googleads.v10.common.TargetImpressionShare.Builder getTargetImpressionShareBuilder() {
return getTargetImpressionShareFieldBuilder().getBuilder();
}
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetImpressionShareOrBuilder getTargetImpressionShareOrBuilder() {
if ((schemeCase_ == 48) && (targetImpressionShareBuilder_ != null)) {
return targetImpressionShareBuilder_.getMessageOrBuilder();
} else {
if (schemeCase_ == 48) {
return (com.google.ads.googleads.v10.common.TargetImpressionShare) scheme_;
}
return com.google.ads.googleads.v10.common.TargetImpressionShare.getDefaultInstance();
}
}
/**
*
* A bidding strategy that automatically optimizes towards a chosen
* percentage of impressions.
*
*
* .google.ads.googleads.v10.common.TargetImpressionShare target_impression_share = 48;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetImpressionShare, com.google.ads.googleads.v10.common.TargetImpressionShare.Builder, com.google.ads.googleads.v10.common.TargetImpressionShareOrBuilder>
getTargetImpressionShareFieldBuilder() {
if (targetImpressionShareBuilder_ == null) {
if (!(schemeCase_ == 48)) {
scheme_ = com.google.ads.googleads.v10.common.TargetImpressionShare.getDefaultInstance();
}
targetImpressionShareBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetImpressionShare, com.google.ads.googleads.v10.common.TargetImpressionShare.Builder, com.google.ads.googleads.v10.common.TargetImpressionShareOrBuilder>(
(com.google.ads.googleads.v10.common.TargetImpressionShare) scheme_,
getParentForChildren(),
isClean());
scheme_ = null;
}
schemeCase_ = 48;
onChanged();;
return targetImpressionShareBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetRoas, com.google.ads.googleads.v10.common.TargetRoas.Builder, com.google.ads.googleads.v10.common.TargetRoasOrBuilder> targetRoasBuilder_;
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
* @return Whether the targetRoas field is set.
*/
@java.lang.Override
public boolean hasTargetRoas() {
return schemeCase_ == 11;
}
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
* @return The targetRoas.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetRoas getTargetRoas() {
if (targetRoasBuilder_ == null) {
if (schemeCase_ == 11) {
return (com.google.ads.googleads.v10.common.TargetRoas) scheme_;
}
return com.google.ads.googleads.v10.common.TargetRoas.getDefaultInstance();
} else {
if (schemeCase_ == 11) {
return targetRoasBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.TargetRoas.getDefaultInstance();
}
}
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
*/
public Builder setTargetRoas(com.google.ads.googleads.v10.common.TargetRoas value) {
if (targetRoasBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scheme_ = value;
onChanged();
} else {
targetRoasBuilder_.setMessage(value);
}
schemeCase_ = 11;
return this;
}
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
*/
public Builder setTargetRoas(
com.google.ads.googleads.v10.common.TargetRoas.Builder builderForValue) {
if (targetRoasBuilder_ == null) {
scheme_ = builderForValue.build();
onChanged();
} else {
targetRoasBuilder_.setMessage(builderForValue.build());
}
schemeCase_ = 11;
return this;
}
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
*/
public Builder mergeTargetRoas(com.google.ads.googleads.v10.common.TargetRoas value) {
if (targetRoasBuilder_ == null) {
if (schemeCase_ == 11 &&
scheme_ != com.google.ads.googleads.v10.common.TargetRoas.getDefaultInstance()) {
scheme_ = com.google.ads.googleads.v10.common.TargetRoas.newBuilder((com.google.ads.googleads.v10.common.TargetRoas) scheme_)
.mergeFrom(value).buildPartial();
} else {
scheme_ = value;
}
onChanged();
} else {
if (schemeCase_ == 11) {
targetRoasBuilder_.mergeFrom(value);
} else {
targetRoasBuilder_.setMessage(value);
}
}
schemeCase_ = 11;
return this;
}
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
*/
public Builder clearTargetRoas() {
if (targetRoasBuilder_ == null) {
if (schemeCase_ == 11) {
schemeCase_ = 0;
scheme_ = null;
onChanged();
}
} else {
if (schemeCase_ == 11) {
schemeCase_ = 0;
scheme_ = null;
}
targetRoasBuilder_.clear();
}
return this;
}
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
*/
public com.google.ads.googleads.v10.common.TargetRoas.Builder getTargetRoasBuilder() {
return getTargetRoasFieldBuilder().getBuilder();
}
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetRoasOrBuilder getTargetRoasOrBuilder() {
if ((schemeCase_ == 11) && (targetRoasBuilder_ != null)) {
return targetRoasBuilder_.getMessageOrBuilder();
} else {
if (schemeCase_ == 11) {
return (com.google.ads.googleads.v10.common.TargetRoas) scheme_;
}
return com.google.ads.googleads.v10.common.TargetRoas.getDefaultInstance();
}
}
/**
*
* A bidding strategy that helps you maximize revenue while averaging a
* specific target Return On Ad Spend (ROAS).
*
*
* .google.ads.googleads.v10.common.TargetRoas target_roas = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetRoas, com.google.ads.googleads.v10.common.TargetRoas.Builder, com.google.ads.googleads.v10.common.TargetRoasOrBuilder>
getTargetRoasFieldBuilder() {
if (targetRoasBuilder_ == null) {
if (!(schemeCase_ == 11)) {
scheme_ = com.google.ads.googleads.v10.common.TargetRoas.getDefaultInstance();
}
targetRoasBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetRoas, com.google.ads.googleads.v10.common.TargetRoas.Builder, com.google.ads.googleads.v10.common.TargetRoasOrBuilder>(
(com.google.ads.googleads.v10.common.TargetRoas) scheme_,
getParentForChildren(),
isClean());
scheme_ = null;
}
schemeCase_ = 11;
onChanged();;
return targetRoasBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetSpend, com.google.ads.googleads.v10.common.TargetSpend.Builder, com.google.ads.googleads.v10.common.TargetSpendOrBuilder> targetSpendBuilder_;
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
* @return Whether the targetSpend field is set.
*/
@java.lang.Override
public boolean hasTargetSpend() {
return schemeCase_ == 12;
}
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
* @return The targetSpend.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetSpend getTargetSpend() {
if (targetSpendBuilder_ == null) {
if (schemeCase_ == 12) {
return (com.google.ads.googleads.v10.common.TargetSpend) scheme_;
}
return com.google.ads.googleads.v10.common.TargetSpend.getDefaultInstance();
} else {
if (schemeCase_ == 12) {
return targetSpendBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.TargetSpend.getDefaultInstance();
}
}
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
*/
public Builder setTargetSpend(com.google.ads.googleads.v10.common.TargetSpend value) {
if (targetSpendBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scheme_ = value;
onChanged();
} else {
targetSpendBuilder_.setMessage(value);
}
schemeCase_ = 12;
return this;
}
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
*/
public Builder setTargetSpend(
com.google.ads.googleads.v10.common.TargetSpend.Builder builderForValue) {
if (targetSpendBuilder_ == null) {
scheme_ = builderForValue.build();
onChanged();
} else {
targetSpendBuilder_.setMessage(builderForValue.build());
}
schemeCase_ = 12;
return this;
}
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
*/
public Builder mergeTargetSpend(com.google.ads.googleads.v10.common.TargetSpend value) {
if (targetSpendBuilder_ == null) {
if (schemeCase_ == 12 &&
scheme_ != com.google.ads.googleads.v10.common.TargetSpend.getDefaultInstance()) {
scheme_ = com.google.ads.googleads.v10.common.TargetSpend.newBuilder((com.google.ads.googleads.v10.common.TargetSpend) scheme_)
.mergeFrom(value).buildPartial();
} else {
scheme_ = value;
}
onChanged();
} else {
if (schemeCase_ == 12) {
targetSpendBuilder_.mergeFrom(value);
} else {
targetSpendBuilder_.setMessage(value);
}
}
schemeCase_ = 12;
return this;
}
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
*/
public Builder clearTargetSpend() {
if (targetSpendBuilder_ == null) {
if (schemeCase_ == 12) {
schemeCase_ = 0;
scheme_ = null;
onChanged();
}
} else {
if (schemeCase_ == 12) {
schemeCase_ = 0;
scheme_ = null;
}
targetSpendBuilder_.clear();
}
return this;
}
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
*/
public com.google.ads.googleads.v10.common.TargetSpend.Builder getTargetSpendBuilder() {
return getTargetSpendFieldBuilder().getBuilder();
}
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.TargetSpendOrBuilder getTargetSpendOrBuilder() {
if ((schemeCase_ == 12) && (targetSpendBuilder_ != null)) {
return targetSpendBuilder_.getMessageOrBuilder();
} else {
if (schemeCase_ == 12) {
return (com.google.ads.googleads.v10.common.TargetSpend) scheme_;
}
return com.google.ads.googleads.v10.common.TargetSpend.getDefaultInstance();
}
}
/**
*
* A bid strategy that sets your bids to help get as many clicks as
* possible within your budget.
*
*
* .google.ads.googleads.v10.common.TargetSpend target_spend = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetSpend, com.google.ads.googleads.v10.common.TargetSpend.Builder, com.google.ads.googleads.v10.common.TargetSpendOrBuilder>
getTargetSpendFieldBuilder() {
if (targetSpendBuilder_ == null) {
if (!(schemeCase_ == 12)) {
scheme_ = com.google.ads.googleads.v10.common.TargetSpend.getDefaultInstance();
}
targetSpendBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.TargetSpend, com.google.ads.googleads.v10.common.TargetSpend.Builder, com.google.ads.googleads.v10.common.TargetSpendOrBuilder>(
(com.google.ads.googleads.v10.common.TargetSpend) scheme_,
getParentForChildren(),
isClean());
scheme_ = null;
}
schemeCase_ = 12;
onChanged();;
return targetSpendBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v10.resources.BiddingStrategy)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v10.resources.BiddingStrategy)
private static final com.google.ads.googleads.v10.resources.BiddingStrategy DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v10.resources.BiddingStrategy();
}
public static com.google.ads.googleads.v10.resources.BiddingStrategy getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BiddingStrategy parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.BiddingStrategy getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy