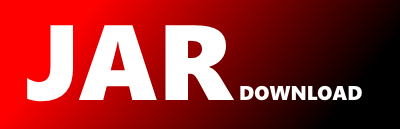
com.google.ads.googleads.v10.resources.CampaignExperiment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v10 Show documentation
Show all versions of google-ads-stubs-v10 Show documentation
Stubs for GAAPI version google-ads-stubs-v10
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v10/resources/campaign_experiment.proto
package com.google.ads.googleads.v10.resources;
/**
*
* An A/B experiment that compares the performance of the base campaign
* (the control) and a variation of that campaign (the experiment).
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.CampaignExperiment}
*/
public final class CampaignExperiment extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v10.resources.CampaignExperiment)
CampaignExperimentOrBuilder {
private static final long serialVersionUID = 0L;
// Use CampaignExperiment.newBuilder() to construct.
private CampaignExperiment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CampaignExperiment() {
resourceName_ = "";
campaignDraft_ = "";
name_ = "";
description_ = "";
trafficSplitType_ = 0;
experimentCampaign_ = "";
status_ = 0;
longRunningOperation_ = "";
startDate_ = "";
endDate_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CampaignExperiment();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.CampaignExperimentProto.internal_static_google_ads_googleads_v10_resources_CampaignExperiment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.CampaignExperimentProto.internal_static_google_ads_googleads_v10_resources_CampaignExperiment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.CampaignExperiment.class, com.google.ads.googleads.v10.resources.CampaignExperiment.Builder.class);
}
private int bitField0_;
public static final int RESOURCE_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object resourceName_;
/**
*
* Immutable. The resource name of the campaign experiment.
* Campaign experiment resource names have the form:
* `customers/{customer_id}/campaignExperiments/{campaign_experiment_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
@java.lang.Override
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
}
}
/**
*
* Immutable. The resource name of the campaign experiment.
* Campaign experiment resource names have the form:
* `customers/{customer_id}/campaignExperiments/{campaign_experiment_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 13;
private long id_;
/**
*
* Output only. The ID of the campaign experiment.
* This field is read-only.
*
*
* optional int64 id = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The ID of the campaign experiment.
* This field is read-only.
*
*
* optional int64 id = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int CAMPAIGN_DRAFT_FIELD_NUMBER = 14;
private volatile java.lang.Object campaignDraft_;
/**
*
* Immutable. The campaign draft with staged changes to the base campaign.
*
*
* optional string campaign_draft = 14 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return Whether the campaignDraft field is set.
*/
@java.lang.Override
public boolean hasCampaignDraft() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Immutable. The campaign draft with staged changes to the base campaign.
*
*
* optional string campaign_draft = 14 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The campaignDraft.
*/
@java.lang.Override
public java.lang.String getCampaignDraft() {
java.lang.Object ref = campaignDraft_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaignDraft_ = s;
return s;
}
}
/**
*
* Immutable. The campaign draft with staged changes to the base campaign.
*
*
* optional string campaign_draft = 14 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for campaignDraft.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCampaignDraftBytes() {
java.lang.Object ref = campaignDraft_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaignDraft_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 15;
private volatile java.lang.Object name_;
/**
*
* The name of the campaign experiment.
* This field is required when creating new campaign experiments
* and must not conflict with the name of another non-removed
* campaign experiment or campaign.
* It must not contain any null (code point 0x0), NL line feed
* (code point 0xA) or carriage return (code point 0xD) characters.
*
*
* optional string name = 15;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The name of the campaign experiment.
* This field is required when creating new campaign experiments
* and must not conflict with the name of another non-removed
* campaign experiment or campaign.
* It must not contain any null (code point 0x0), NL line feed
* (code point 0xA) or carriage return (code point 0xD) characters.
*
*
* optional string name = 15;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* The name of the campaign experiment.
* This field is required when creating new campaign experiments
* and must not conflict with the name of another non-removed
* campaign experiment or campaign.
* It must not contain any null (code point 0x0), NL line feed
* (code point 0xA) or carriage return (code point 0xD) characters.
*
*
* optional string name = 15;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 16;
private volatile java.lang.Object description_;
/**
*
* The description of the experiment.
*
*
* optional string description = 16;
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The description of the experiment.
*
*
* optional string description = 16;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* The description of the experiment.
*
*
* optional string description = 16;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TRAFFIC_SPLIT_PERCENT_FIELD_NUMBER = 17;
private long trafficSplitPercent_;
/**
*
* Immutable. Share of traffic directed to experiment as a percent (must be between 1 and
* 99 inclusive. Base campaign receives the remainder of the traffic
* (100 - traffic_split_percent). Required for create.
*
*
* optional int64 traffic_split_percent = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the trafficSplitPercent field is set.
*/
@java.lang.Override
public boolean hasTrafficSplitPercent() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Immutable. Share of traffic directed to experiment as a percent (must be between 1 and
* 99 inclusive. Base campaign receives the remainder of the traffic
* (100 - traffic_split_percent). Required for create.
*
*
* optional int64 traffic_split_percent = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return The trafficSplitPercent.
*/
@java.lang.Override
public long getTrafficSplitPercent() {
return trafficSplitPercent_;
}
public static final int TRAFFIC_SPLIT_TYPE_FIELD_NUMBER = 7;
private int trafficSplitType_;
/**
*
* Immutable. Determines the behavior of the traffic split.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType traffic_split_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for trafficSplitType.
*/
@java.lang.Override public int getTrafficSplitTypeValue() {
return trafficSplitType_;
}
/**
*
* Immutable. Determines the behavior of the traffic split.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType traffic_split_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return The trafficSplitType.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType getTrafficSplitType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType result = com.google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType.valueOf(trafficSplitType_);
return result == null ? com.google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType.UNRECOGNIZED : result;
}
public static final int EXPERIMENT_CAMPAIGN_FIELD_NUMBER = 18;
private volatile java.lang.Object experimentCampaign_;
/**
*
* Output only. The experiment campaign, as opposed to the base campaign.
*
*
* optional string experiment_campaign = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the experimentCampaign field is set.
*/
@java.lang.Override
public boolean hasExperimentCampaign() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. The experiment campaign, as opposed to the base campaign.
*
*
* optional string experiment_campaign = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The experimentCampaign.
*/
@java.lang.Override
public java.lang.String getExperimentCampaign() {
java.lang.Object ref = experimentCampaign_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
experimentCampaign_ = s;
return s;
}
}
/**
*
* Output only. The experiment campaign, as opposed to the base campaign.
*
*
* optional string experiment_campaign = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for experimentCampaign.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExperimentCampaignBytes() {
java.lang.Object ref = experimentCampaign_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
experimentCampaign_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 9;
private int status_;
/**
*
* Output only. The status of the campaign experiment. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus status = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Output only. The status of the campaign experiment. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus status = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The status.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus getStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus result = com.google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus.valueOf(status_);
return result == null ? com.google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus.UNRECOGNIZED : result;
}
public static final int LONG_RUNNING_OPERATION_FIELD_NUMBER = 19;
private volatile java.lang.Object longRunningOperation_;
/**
*
* Output only. The resource name of the long-running operation that can be used to poll
* for completion of experiment create or promote. The most recent long
* running operation is returned.
*
*
* optional string long_running_operation = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the longRunningOperation field is set.
*/
@java.lang.Override
public boolean hasLongRunningOperation() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. The resource name of the long-running operation that can be used to poll
* for completion of experiment create or promote. The most recent long
* running operation is returned.
*
*
* optional string long_running_operation = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The longRunningOperation.
*/
@java.lang.Override
public java.lang.String getLongRunningOperation() {
java.lang.Object ref = longRunningOperation_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
longRunningOperation_ = s;
return s;
}
}
/**
*
* Output only. The resource name of the long-running operation that can be used to poll
* for completion of experiment create or promote. The most recent long
* running operation is returned.
*
*
* optional string long_running_operation = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for longRunningOperation.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLongRunningOperationBytes() {
java.lang.Object ref = longRunningOperation_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longRunningOperation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int START_DATE_FIELD_NUMBER = 20;
private volatile java.lang.Object startDate_;
/**
*
* Date when the campaign experiment starts. By default, the experiment starts
* now or on the campaign's start date, whichever is later. If this field is
* set, then the experiment starts at the beginning of the specified date in
* the customer's time zone. Cannot be changed once the experiment starts.
* Format: YYYY-MM-DD
* Example: 2019-03-14
*
*
* optional string start_date = 20;
* @return Whether the startDate field is set.
*/
@java.lang.Override
public boolean hasStartDate() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Date when the campaign experiment starts. By default, the experiment starts
* now or on the campaign's start date, whichever is later. If this field is
* set, then the experiment starts at the beginning of the specified date in
* the customer's time zone. Cannot be changed once the experiment starts.
* Format: YYYY-MM-DD
* Example: 2019-03-14
*
*
* optional string start_date = 20;
* @return The startDate.
*/
@java.lang.Override
public java.lang.String getStartDate() {
java.lang.Object ref = startDate_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
startDate_ = s;
return s;
}
}
/**
*
* Date when the campaign experiment starts. By default, the experiment starts
* now or on the campaign's start date, whichever is later. If this field is
* set, then the experiment starts at the beginning of the specified date in
* the customer's time zone. Cannot be changed once the experiment starts.
* Format: YYYY-MM-DD
* Example: 2019-03-14
*
*
* optional string start_date = 20;
* @return The bytes for startDate.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStartDateBytes() {
java.lang.Object ref = startDate_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
startDate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int END_DATE_FIELD_NUMBER = 21;
private volatile java.lang.Object endDate_;
/**
*
* The last day of the campaign experiment. By default, the experiment ends on
* the campaign's end date. If this field is set, then the experiment ends at
* the end of the specified date in the customer's time zone.
* Format: YYYY-MM-DD
* Example: 2019-04-18
*
*
* optional string end_date = 21;
* @return Whether the endDate field is set.
*/
@java.lang.Override
public boolean hasEndDate() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* The last day of the campaign experiment. By default, the experiment ends on
* the campaign's end date. If this field is set, then the experiment ends at
* the end of the specified date in the customer's time zone.
* Format: YYYY-MM-DD
* Example: 2019-04-18
*
*
* optional string end_date = 21;
* @return The endDate.
*/
@java.lang.Override
public java.lang.String getEndDate() {
java.lang.Object ref = endDate_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
endDate_ = s;
return s;
}
}
/**
*
* The last day of the campaign experiment. By default, the experiment ends on
* the campaign's end date. If this field is set, then the experiment ends at
* the end of the specified date in the customer's time zone.
* Format: YYYY-MM-DD
* Example: 2019-04-18
*
*
* optional string end_date = 21;
* @return The bytes for endDate.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getEndDateBytes() {
java.lang.Object ref = endDate_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
endDate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, resourceName_);
}
if (trafficSplitType_ != com.google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType.UNSPECIFIED.getNumber()) {
output.writeEnum(7, trafficSplitType_);
}
if (status_ != com.google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus.UNSPECIFIED.getNumber()) {
output.writeEnum(9, status_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(13, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, campaignDraft_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, name_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, description_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt64(17, trafficSplitPercent_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 18, experimentCampaign_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 19, longRunningOperation_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, startDate_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 21, endDate_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, resourceName_);
}
if (trafficSplitType_ != com.google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, trafficSplitType_);
}
if (status_ != com.google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(9, status_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(13, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, campaignDraft_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, name_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, description_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(17, trafficSplitPercent_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(18, experimentCampaign_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(19, longRunningOperation_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(20, startDate_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(21, endDate_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v10.resources.CampaignExperiment)) {
return super.equals(obj);
}
com.google.ads.googleads.v10.resources.CampaignExperiment other = (com.google.ads.googleads.v10.resources.CampaignExperiment) obj;
if (!getResourceName()
.equals(other.getResourceName())) return false;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId()
!= other.getId()) return false;
}
if (hasCampaignDraft() != other.hasCampaignDraft()) return false;
if (hasCampaignDraft()) {
if (!getCampaignDraft()
.equals(other.getCampaignDraft())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasDescription() != other.hasDescription()) return false;
if (hasDescription()) {
if (!getDescription()
.equals(other.getDescription())) return false;
}
if (hasTrafficSplitPercent() != other.hasTrafficSplitPercent()) return false;
if (hasTrafficSplitPercent()) {
if (getTrafficSplitPercent()
!= other.getTrafficSplitPercent()) return false;
}
if (trafficSplitType_ != other.trafficSplitType_) return false;
if (hasExperimentCampaign() != other.hasExperimentCampaign()) return false;
if (hasExperimentCampaign()) {
if (!getExperimentCampaign()
.equals(other.getExperimentCampaign())) return false;
}
if (status_ != other.status_) return false;
if (hasLongRunningOperation() != other.hasLongRunningOperation()) return false;
if (hasLongRunningOperation()) {
if (!getLongRunningOperation()
.equals(other.getLongRunningOperation())) return false;
}
if (hasStartDate() != other.hasStartDate()) return false;
if (hasStartDate()) {
if (!getStartDate()
.equals(other.getStartDate())) return false;
}
if (hasEndDate() != other.hasEndDate()) return false;
if (hasEndDate()) {
if (!getEndDate()
.equals(other.getEndDate())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RESOURCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getResourceName().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getId());
}
if (hasCampaignDraft()) {
hash = (37 * hash) + CAMPAIGN_DRAFT_FIELD_NUMBER;
hash = (53 * hash) + getCampaignDraft().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
if (hasTrafficSplitPercent()) {
hash = (37 * hash) + TRAFFIC_SPLIT_PERCENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTrafficSplitPercent());
}
hash = (37 * hash) + TRAFFIC_SPLIT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + trafficSplitType_;
if (hasExperimentCampaign()) {
hash = (37 * hash) + EXPERIMENT_CAMPAIGN_FIELD_NUMBER;
hash = (53 * hash) + getExperimentCampaign().hashCode();
}
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (hasLongRunningOperation()) {
hash = (37 * hash) + LONG_RUNNING_OPERATION_FIELD_NUMBER;
hash = (53 * hash) + getLongRunningOperation().hashCode();
}
if (hasStartDate()) {
hash = (37 * hash) + START_DATE_FIELD_NUMBER;
hash = (53 * hash) + getStartDate().hashCode();
}
if (hasEndDate()) {
hash = (37 * hash) + END_DATE_FIELD_NUMBER;
hash = (53 * hash) + getEndDate().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v10.resources.CampaignExperiment prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An A/B experiment that compares the performance of the base campaign
* (the control) and a variation of that campaign (the experiment).
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.CampaignExperiment}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v10.resources.CampaignExperiment)
com.google.ads.googleads.v10.resources.CampaignExperimentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.CampaignExperimentProto.internal_static_google_ads_googleads_v10_resources_CampaignExperiment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.CampaignExperimentProto.internal_static_google_ads_googleads_v10_resources_CampaignExperiment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.CampaignExperiment.class, com.google.ads.googleads.v10.resources.CampaignExperiment.Builder.class);
}
// Construct using com.google.ads.googleads.v10.resources.CampaignExperiment.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
resourceName_ = "";
id_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
campaignDraft_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
description_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
trafficSplitPercent_ = 0L;
bitField0_ = (bitField0_ & ~0x00000010);
trafficSplitType_ = 0;
experimentCampaign_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
status_ = 0;
longRunningOperation_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
startDate_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
endDate_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v10.resources.CampaignExperimentProto.internal_static_google_ads_googleads_v10_resources_CampaignExperiment_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.CampaignExperiment getDefaultInstanceForType() {
return com.google.ads.googleads.v10.resources.CampaignExperiment.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.CampaignExperiment build() {
com.google.ads.googleads.v10.resources.CampaignExperiment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.CampaignExperiment buildPartial() {
com.google.ads.googleads.v10.resources.CampaignExperiment result = new com.google.ads.googleads.v10.resources.CampaignExperiment(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.resourceName_ = resourceName_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.campaignDraft_ = campaignDraft_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.description_ = description_;
if (((from_bitField0_ & 0x00000010) != 0)) {
result.trafficSplitPercent_ = trafficSplitPercent_;
to_bitField0_ |= 0x00000010;
}
result.trafficSplitType_ = trafficSplitType_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.experimentCampaign_ = experimentCampaign_;
result.status_ = status_;
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000040;
}
result.longRunningOperation_ = longRunningOperation_;
if (((from_bitField0_ & 0x00000080) != 0)) {
to_bitField0_ |= 0x00000080;
}
result.startDate_ = startDate_;
if (((from_bitField0_ & 0x00000100) != 0)) {
to_bitField0_ |= 0x00000100;
}
result.endDate_ = endDate_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v10.resources.CampaignExperiment) {
return mergeFrom((com.google.ads.googleads.v10.resources.CampaignExperiment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v10.resources.CampaignExperiment other) {
if (other == com.google.ads.googleads.v10.resources.CampaignExperiment.getDefaultInstance()) return this;
if (!other.getResourceName().isEmpty()) {
resourceName_ = other.resourceName_;
onChanged();
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasCampaignDraft()) {
bitField0_ |= 0x00000002;
campaignDraft_ = other.campaignDraft_;
onChanged();
}
if (other.hasName()) {
bitField0_ |= 0x00000004;
name_ = other.name_;
onChanged();
}
if (other.hasDescription()) {
bitField0_ |= 0x00000008;
description_ = other.description_;
onChanged();
}
if (other.hasTrafficSplitPercent()) {
setTrafficSplitPercent(other.getTrafficSplitPercent());
}
if (other.trafficSplitType_ != 0) {
setTrafficSplitTypeValue(other.getTrafficSplitTypeValue());
}
if (other.hasExperimentCampaign()) {
bitField0_ |= 0x00000020;
experimentCampaign_ = other.experimentCampaign_;
onChanged();
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.hasLongRunningOperation()) {
bitField0_ |= 0x00000040;
longRunningOperation_ = other.longRunningOperation_;
onChanged();
}
if (other.hasStartDate()) {
bitField0_ |= 0x00000080;
startDate_ = other.startDate_;
onChanged();
}
if (other.hasEndDate()) {
bitField0_ |= 0x00000100;
endDate_ = other.endDate_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
resourceName_ = input.readStringRequireUtf8();
break;
} // case 10
case 56: {
trafficSplitType_ = input.readEnum();
break;
} // case 56
case 72: {
status_ = input.readEnum();
break;
} // case 72
case 104: {
id_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 104
case 114: {
campaignDraft_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 114
case 122: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 122
case 130: {
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 130
case 136: {
trafficSplitPercent_ = input.readInt64();
bitField0_ |= 0x00000010;
break;
} // case 136
case 146: {
experimentCampaign_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 146
case 154: {
longRunningOperation_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 154
case 162: {
startDate_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 162
case 170: {
endDate_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case 170
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object resourceName_ = "";
/**
*
* Immutable. The resource name of the campaign experiment.
* Campaign experiment resource names have the form:
* `customers/{customer_id}/campaignExperiments/{campaign_experiment_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The resource name of the campaign experiment.
* Campaign experiment resource names have the form:
* `customers/{customer_id}/campaignExperiments/{campaign_experiment_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The resource name of the campaign experiment.
* Campaign experiment resource names have the form:
* `customers/{customer_id}/campaignExperiments/{campaign_experiment_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resourceName_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the campaign experiment.
* Campaign experiment resource names have the form:
* `customers/{customer_id}/campaignExperiments/{campaign_experiment_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearResourceName() {
resourceName_ = getDefaultInstance().getResourceName();
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the campaign experiment.
* Campaign experiment resource names have the form:
* `customers/{customer_id}/campaignExperiments/{campaign_experiment_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The bytes for resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resourceName_ = value;
onChanged();
return this;
}
private long id_ ;
/**
*
* Output only. The ID of the campaign experiment.
* This field is read-only.
*
*
* optional int64 id = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The ID of the campaign experiment.
* This field is read-only.
*
*
* optional int64 id = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
*
* Output only. The ID of the campaign experiment.
* This field is read-only.
*
*
* optional int64 id = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
*
* Output only. The ID of the campaign experiment.
* This field is read-only.
*
*
* optional int64 id = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object campaignDraft_ = "";
/**
*
* Immutable. The campaign draft with staged changes to the base campaign.
*
*
* optional string campaign_draft = 14 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return Whether the campaignDraft field is set.
*/
public boolean hasCampaignDraft() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Immutable. The campaign draft with staged changes to the base campaign.
*
*
* optional string campaign_draft = 14 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The campaignDraft.
*/
public java.lang.String getCampaignDraft() {
java.lang.Object ref = campaignDraft_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaignDraft_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The campaign draft with staged changes to the base campaign.
*
*
* optional string campaign_draft = 14 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for campaignDraft.
*/
public com.google.protobuf.ByteString
getCampaignDraftBytes() {
java.lang.Object ref = campaignDraft_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaignDraft_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The campaign draft with staged changes to the base campaign.
*
*
* optional string campaign_draft = 14 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The campaignDraft to set.
* @return This builder for chaining.
*/
public Builder setCampaignDraft(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
campaignDraft_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The campaign draft with staged changes to the base campaign.
*
*
* optional string campaign_draft = 14 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearCampaignDraft() {
bitField0_ = (bitField0_ & ~0x00000002);
campaignDraft_ = getDefaultInstance().getCampaignDraft();
onChanged();
return this;
}
/**
*
* Immutable. The campaign draft with staged changes to the base campaign.
*
*
* optional string campaign_draft = 14 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The bytes for campaignDraft to set.
* @return This builder for chaining.
*/
public Builder setCampaignDraftBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000002;
campaignDraft_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* The name of the campaign experiment.
* This field is required when creating new campaign experiments
* and must not conflict with the name of another non-removed
* campaign experiment or campaign.
* It must not contain any null (code point 0x0), NL line feed
* (code point 0xA) or carriage return (code point 0xD) characters.
*
*
* optional string name = 15;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The name of the campaign experiment.
* This field is required when creating new campaign experiments
* and must not conflict with the name of another non-removed
* campaign experiment or campaign.
* It must not contain any null (code point 0x0), NL line feed
* (code point 0xA) or carriage return (code point 0xD) characters.
*
*
* optional string name = 15;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the campaign experiment.
* This field is required when creating new campaign experiments
* and must not conflict with the name of another non-removed
* campaign experiment or campaign.
* It must not contain any null (code point 0x0), NL line feed
* (code point 0xA) or carriage return (code point 0xD) characters.
*
*
* optional string name = 15;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the campaign experiment.
* This field is required when creating new campaign experiments
* and must not conflict with the name of another non-removed
* campaign experiment or campaign.
* It must not contain any null (code point 0x0), NL line feed
* (code point 0xA) or carriage return (code point 0xD) characters.
*
*
* optional string name = 15;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
name_ = value;
onChanged();
return this;
}
/**
*
* The name of the campaign experiment.
* This field is required when creating new campaign experiments
* and must not conflict with the name of another non-removed
* campaign experiment or campaign.
* It must not contain any null (code point 0x0), NL line feed
* (code point 0xA) or carriage return (code point 0xD) characters.
*
*
* optional string name = 15;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000004);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* The name of the campaign experiment.
* This field is required when creating new campaign experiments
* and must not conflict with the name of another non-removed
* campaign experiment or campaign.
* It must not contain any null (code point 0x0), NL line feed
* (code point 0xA) or carriage return (code point 0xD) characters.
*
*
* optional string name = 15;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000004;
name_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* The description of the experiment.
*
*
* optional string description = 16;
* @return Whether the description field is set.
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The description of the experiment.
*
*
* optional string description = 16;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The description of the experiment.
*
*
* optional string description = 16;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The description of the experiment.
*
*
* optional string description = 16;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
description_ = value;
onChanged();
return this;
}
/**
*
* The description of the experiment.
*
*
* optional string description = 16;
* @return This builder for chaining.
*/
public Builder clearDescription() {
bitField0_ = (bitField0_ & ~0x00000008);
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* The description of the experiment.
*
*
* optional string description = 16;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000008;
description_ = value;
onChanged();
return this;
}
private long trafficSplitPercent_ ;
/**
*
* Immutable. Share of traffic directed to experiment as a percent (must be between 1 and
* 99 inclusive. Base campaign receives the remainder of the traffic
* (100 - traffic_split_percent). Required for create.
*
*
* optional int64 traffic_split_percent = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return Whether the trafficSplitPercent field is set.
*/
@java.lang.Override
public boolean hasTrafficSplitPercent() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Immutable. Share of traffic directed to experiment as a percent (must be between 1 and
* 99 inclusive. Base campaign receives the remainder of the traffic
* (100 - traffic_split_percent). Required for create.
*
*
* optional int64 traffic_split_percent = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return The trafficSplitPercent.
*/
@java.lang.Override
public long getTrafficSplitPercent() {
return trafficSplitPercent_;
}
/**
*
* Immutable. Share of traffic directed to experiment as a percent (must be between 1 and
* 99 inclusive. Base campaign receives the remainder of the traffic
* (100 - traffic_split_percent). Required for create.
*
*
* optional int64 traffic_split_percent = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The trafficSplitPercent to set.
* @return This builder for chaining.
*/
public Builder setTrafficSplitPercent(long value) {
bitField0_ |= 0x00000010;
trafficSplitPercent_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Share of traffic directed to experiment as a percent (must be between 1 and
* 99 inclusive. Base campaign receives the remainder of the traffic
* (100 - traffic_split_percent). Required for create.
*
*
* optional int64 traffic_split_percent = 17 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearTrafficSplitPercent() {
bitField0_ = (bitField0_ & ~0x00000010);
trafficSplitPercent_ = 0L;
onChanged();
return this;
}
private int trafficSplitType_ = 0;
/**
*
* Immutable. Determines the behavior of the traffic split.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType traffic_split_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for trafficSplitType.
*/
@java.lang.Override public int getTrafficSplitTypeValue() {
return trafficSplitType_;
}
/**
*
* Immutable. Determines the behavior of the traffic split.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType traffic_split_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for trafficSplitType to set.
* @return This builder for chaining.
*/
public Builder setTrafficSplitTypeValue(int value) {
trafficSplitType_ = value;
onChanged();
return this;
}
/**
*
* Immutable. Determines the behavior of the traffic split.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType traffic_split_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return The trafficSplitType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType getTrafficSplitType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType result = com.google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType.valueOf(trafficSplitType_);
return result == null ? com.google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType.UNRECOGNIZED : result;
}
/**
*
* Immutable. Determines the behavior of the traffic split.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType traffic_split_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The trafficSplitType to set.
* @return This builder for chaining.
*/
public Builder setTrafficSplitType(com.google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType value) {
if (value == null) {
throw new NullPointerException();
}
trafficSplitType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Determines the behavior of the traffic split.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentTrafficSplitTypeEnum.CampaignExperimentTrafficSplitType traffic_split_type = 7 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearTrafficSplitType() {
trafficSplitType_ = 0;
onChanged();
return this;
}
private java.lang.Object experimentCampaign_ = "";
/**
*
* Output only. The experiment campaign, as opposed to the base campaign.
*
*
* optional string experiment_campaign = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the experimentCampaign field is set.
*/
public boolean hasExperimentCampaign() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. The experiment campaign, as opposed to the base campaign.
*
*
* optional string experiment_campaign = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The experimentCampaign.
*/
public java.lang.String getExperimentCampaign() {
java.lang.Object ref = experimentCampaign_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
experimentCampaign_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The experiment campaign, as opposed to the base campaign.
*
*
* optional string experiment_campaign = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for experimentCampaign.
*/
public com.google.protobuf.ByteString
getExperimentCampaignBytes() {
java.lang.Object ref = experimentCampaign_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
experimentCampaign_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The experiment campaign, as opposed to the base campaign.
*
*
* optional string experiment_campaign = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The experimentCampaign to set.
* @return This builder for chaining.
*/
public Builder setExperimentCampaign(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
experimentCampaign_ = value;
onChanged();
return this;
}
/**
*
* Output only. The experiment campaign, as opposed to the base campaign.
*
*
* optional string experiment_campaign = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearExperimentCampaign() {
bitField0_ = (bitField0_ & ~0x00000020);
experimentCampaign_ = getDefaultInstance().getExperimentCampaign();
onChanged();
return this;
}
/**
*
* Output only. The experiment campaign, as opposed to the base campaign.
*
*
* optional string experiment_campaign = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for experimentCampaign to set.
* @return This builder for chaining.
*/
public Builder setExperimentCampaignBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000020;
experimentCampaign_ = value;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Output only. The status of the campaign experiment. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus status = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Output only. The status of the campaign experiment. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus status = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Output only. The status of the campaign experiment. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus status = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The status.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus getStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus result = com.google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus.valueOf(status_);
return result == null ? com.google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus.UNRECOGNIZED : result;
}
/**
*
* Output only. The status of the campaign experiment. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus status = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The status of the campaign experiment. This field is read-only.
*
*
* .google.ads.googleads.v10.enums.CampaignExperimentStatusEnum.CampaignExperimentStatus status = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private java.lang.Object longRunningOperation_ = "";
/**
*
* Output only. The resource name of the long-running operation that can be used to poll
* for completion of experiment create or promote. The most recent long
* running operation is returned.
*
*
* optional string long_running_operation = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the longRunningOperation field is set.
*/
public boolean hasLongRunningOperation() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. The resource name of the long-running operation that can be used to poll
* for completion of experiment create or promote. The most recent long
* running operation is returned.
*
*
* optional string long_running_operation = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The longRunningOperation.
*/
public java.lang.String getLongRunningOperation() {
java.lang.Object ref = longRunningOperation_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
longRunningOperation_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The resource name of the long-running operation that can be used to poll
* for completion of experiment create or promote. The most recent long
* running operation is returned.
*
*
* optional string long_running_operation = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for longRunningOperation.
*/
public com.google.protobuf.ByteString
getLongRunningOperationBytes() {
java.lang.Object ref = longRunningOperation_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longRunningOperation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The resource name of the long-running operation that can be used to poll
* for completion of experiment create or promote. The most recent long
* running operation is returned.
*
*
* optional string long_running_operation = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The longRunningOperation to set.
* @return This builder for chaining.
*/
public Builder setLongRunningOperation(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
longRunningOperation_ = value;
onChanged();
return this;
}
/**
*
* Output only. The resource name of the long-running operation that can be used to poll
* for completion of experiment create or promote. The most recent long
* running operation is returned.
*
*
* optional string long_running_operation = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearLongRunningOperation() {
bitField0_ = (bitField0_ & ~0x00000040);
longRunningOperation_ = getDefaultInstance().getLongRunningOperation();
onChanged();
return this;
}
/**
*
* Output only. The resource name of the long-running operation that can be used to poll
* for completion of experiment create or promote. The most recent long
* running operation is returned.
*
*
* optional string long_running_operation = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for longRunningOperation to set.
* @return This builder for chaining.
*/
public Builder setLongRunningOperationBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000040;
longRunningOperation_ = value;
onChanged();
return this;
}
private java.lang.Object startDate_ = "";
/**
*
* Date when the campaign experiment starts. By default, the experiment starts
* now or on the campaign's start date, whichever is later. If this field is
* set, then the experiment starts at the beginning of the specified date in
* the customer's time zone. Cannot be changed once the experiment starts.
* Format: YYYY-MM-DD
* Example: 2019-03-14
*
*
* optional string start_date = 20;
* @return Whether the startDate field is set.
*/
public boolean hasStartDate() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Date when the campaign experiment starts. By default, the experiment starts
* now or on the campaign's start date, whichever is later. If this field is
* set, then the experiment starts at the beginning of the specified date in
* the customer's time zone. Cannot be changed once the experiment starts.
* Format: YYYY-MM-DD
* Example: 2019-03-14
*
*
* optional string start_date = 20;
* @return The startDate.
*/
public java.lang.String getStartDate() {
java.lang.Object ref = startDate_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
startDate_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Date when the campaign experiment starts. By default, the experiment starts
* now or on the campaign's start date, whichever is later. If this field is
* set, then the experiment starts at the beginning of the specified date in
* the customer's time zone. Cannot be changed once the experiment starts.
* Format: YYYY-MM-DD
* Example: 2019-03-14
*
*
* optional string start_date = 20;
* @return The bytes for startDate.
*/
public com.google.protobuf.ByteString
getStartDateBytes() {
java.lang.Object ref = startDate_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
startDate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Date when the campaign experiment starts. By default, the experiment starts
* now or on the campaign's start date, whichever is later. If this field is
* set, then the experiment starts at the beginning of the specified date in
* the customer's time zone. Cannot be changed once the experiment starts.
* Format: YYYY-MM-DD
* Example: 2019-03-14
*
*
* optional string start_date = 20;
* @param value The startDate to set.
* @return This builder for chaining.
*/
public Builder setStartDate(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
startDate_ = value;
onChanged();
return this;
}
/**
*
* Date when the campaign experiment starts. By default, the experiment starts
* now or on the campaign's start date, whichever is later. If this field is
* set, then the experiment starts at the beginning of the specified date in
* the customer's time zone. Cannot be changed once the experiment starts.
* Format: YYYY-MM-DD
* Example: 2019-03-14
*
*
* optional string start_date = 20;
* @return This builder for chaining.
*/
public Builder clearStartDate() {
bitField0_ = (bitField0_ & ~0x00000080);
startDate_ = getDefaultInstance().getStartDate();
onChanged();
return this;
}
/**
*
* Date when the campaign experiment starts. By default, the experiment starts
* now or on the campaign's start date, whichever is later. If this field is
* set, then the experiment starts at the beginning of the specified date in
* the customer's time zone. Cannot be changed once the experiment starts.
* Format: YYYY-MM-DD
* Example: 2019-03-14
*
*
* optional string start_date = 20;
* @param value The bytes for startDate to set.
* @return This builder for chaining.
*/
public Builder setStartDateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000080;
startDate_ = value;
onChanged();
return this;
}
private java.lang.Object endDate_ = "";
/**
*
* The last day of the campaign experiment. By default, the experiment ends on
* the campaign's end date. If this field is set, then the experiment ends at
* the end of the specified date in the customer's time zone.
* Format: YYYY-MM-DD
* Example: 2019-04-18
*
*
* optional string end_date = 21;
* @return Whether the endDate field is set.
*/
public boolean hasEndDate() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* The last day of the campaign experiment. By default, the experiment ends on
* the campaign's end date. If this field is set, then the experiment ends at
* the end of the specified date in the customer's time zone.
* Format: YYYY-MM-DD
* Example: 2019-04-18
*
*
* optional string end_date = 21;
* @return The endDate.
*/
public java.lang.String getEndDate() {
java.lang.Object ref = endDate_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
endDate_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The last day of the campaign experiment. By default, the experiment ends on
* the campaign's end date. If this field is set, then the experiment ends at
* the end of the specified date in the customer's time zone.
* Format: YYYY-MM-DD
* Example: 2019-04-18
*
*
* optional string end_date = 21;
* @return The bytes for endDate.
*/
public com.google.protobuf.ByteString
getEndDateBytes() {
java.lang.Object ref = endDate_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
endDate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The last day of the campaign experiment. By default, the experiment ends on
* the campaign's end date. If this field is set, then the experiment ends at
* the end of the specified date in the customer's time zone.
* Format: YYYY-MM-DD
* Example: 2019-04-18
*
*
* optional string end_date = 21;
* @param value The endDate to set.
* @return This builder for chaining.
*/
public Builder setEndDate(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
endDate_ = value;
onChanged();
return this;
}
/**
*
* The last day of the campaign experiment. By default, the experiment ends on
* the campaign's end date. If this field is set, then the experiment ends at
* the end of the specified date in the customer's time zone.
* Format: YYYY-MM-DD
* Example: 2019-04-18
*
*
* optional string end_date = 21;
* @return This builder for chaining.
*/
public Builder clearEndDate() {
bitField0_ = (bitField0_ & ~0x00000100);
endDate_ = getDefaultInstance().getEndDate();
onChanged();
return this;
}
/**
*
* The last day of the campaign experiment. By default, the experiment ends on
* the campaign's end date. If this field is set, then the experiment ends at
* the end of the specified date in the customer's time zone.
* Format: YYYY-MM-DD
* Example: 2019-04-18
*
*
* optional string end_date = 21;
* @param value The bytes for endDate to set.
* @return This builder for chaining.
*/
public Builder setEndDateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000100;
endDate_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v10.resources.CampaignExperiment)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v10.resources.CampaignExperiment)
private static final com.google.ads.googleads.v10.resources.CampaignExperiment DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v10.resources.CampaignExperiment();
}
public static com.google.ads.googleads.v10.resources.CampaignExperiment getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CampaignExperiment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.CampaignExperiment getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy