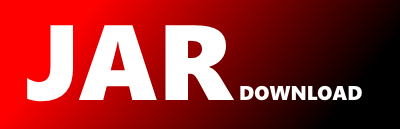
com.google.ads.googleads.v10.resources.ChangeStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v10 Show documentation
Show all versions of google-ads-stubs-v10 Show documentation
Stubs for GAAPI version google-ads-stubs-v10
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v10/resources/change_status.proto
package com.google.ads.googleads.v10.resources;
/**
*
* Describes the status of returned resource. ChangeStatus could have up to 3
* minutes delay to reflect a new change.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.ChangeStatus}
*/
public final class ChangeStatus extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v10.resources.ChangeStatus)
ChangeStatusOrBuilder {
private static final long serialVersionUID = 0L;
// Use ChangeStatus.newBuilder() to construct.
private ChangeStatus(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ChangeStatus() {
resourceName_ = "";
lastChangeDateTime_ = "";
resourceType_ = 0;
campaign_ = "";
adGroup_ = "";
resourceStatus_ = 0;
adGroupAd_ = "";
adGroupCriterion_ = "";
campaignCriterion_ = "";
feed_ = "";
feedItem_ = "";
adGroupFeed_ = "";
campaignFeed_ = "";
adGroupBidModifier_ = "";
sharedSet_ = "";
campaignSharedSet_ = "";
asset_ = "";
customerAsset_ = "";
campaignAsset_ = "";
adGroupAsset_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ChangeStatus();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.ChangeStatusProto.internal_static_google_ads_googleads_v10_resources_ChangeStatus_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.ChangeStatusProto.internal_static_google_ads_googleads_v10_resources_ChangeStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.ChangeStatus.class, com.google.ads.googleads.v10.resources.ChangeStatus.Builder.class);
}
private int bitField0_;
public static final int RESOURCE_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object resourceName_;
/**
*
* Output only. The resource name of the change status.
* Change status resource names have the form:
* `customers/{customer_id}/changeStatus/{change_status_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
@java.lang.Override
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
}
}
/**
*
* Output only. The resource name of the change status.
* Change status resource names have the form:
* `customers/{customer_id}/changeStatus/{change_status_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LAST_CHANGE_DATE_TIME_FIELD_NUMBER = 24;
private volatile java.lang.Object lastChangeDateTime_;
/**
*
* Output only. Time at which the most recent change has occurred on this resource.
*
*
* optional string last_change_date_time = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the lastChangeDateTime field is set.
*/
@java.lang.Override
public boolean hasLastChangeDateTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. Time at which the most recent change has occurred on this resource.
*
*
* optional string last_change_date_time = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The lastChangeDateTime.
*/
@java.lang.Override
public java.lang.String getLastChangeDateTime() {
java.lang.Object ref = lastChangeDateTime_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
lastChangeDateTime_ = s;
return s;
}
}
/**
*
* Output only. Time at which the most recent change has occurred on this resource.
*
*
* optional string last_change_date_time = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for lastChangeDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLastChangeDateTimeBytes() {
java.lang.Object ref = lastChangeDateTime_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
lastChangeDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RESOURCE_TYPE_FIELD_NUMBER = 4;
private int resourceType_;
/**
*
* Output only. Represents the type of the changed resource. This dictates what fields
* will be set. For example, for AD_GROUP, campaign and ad_group fields will
* be set.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType resource_type = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for resourceType.
*/
@java.lang.Override public int getResourceTypeValue() {
return resourceType_;
}
/**
*
* Output only. Represents the type of the changed resource. This dictates what fields
* will be set. For example, for AD_GROUP, campaign and ad_group fields will
* be set.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType resource_type = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The resourceType.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType getResourceType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType result = com.google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType.valueOf(resourceType_);
return result == null ? com.google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType.UNRECOGNIZED : result;
}
public static final int CAMPAIGN_FIELD_NUMBER = 17;
private volatile java.lang.Object campaign_;
/**
*
* Output only. The Campaign affected by this change.
*
*
* optional string campaign = 17 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the campaign field is set.
*/
@java.lang.Override
public boolean hasCampaign() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The Campaign affected by this change.
*
*
* optional string campaign = 17 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The campaign.
*/
@java.lang.Override
public java.lang.String getCampaign() {
java.lang.Object ref = campaign_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaign_ = s;
return s;
}
}
/**
*
* Output only. The Campaign affected by this change.
*
*
* optional string campaign = 17 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for campaign.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCampaignBytes() {
java.lang.Object ref = campaign_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaign_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AD_GROUP_FIELD_NUMBER = 18;
private volatile java.lang.Object adGroup_;
/**
*
* Output only. The AdGroup affected by this change.
*
*
* optional string ad_group = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the adGroup field is set.
*/
@java.lang.Override
public boolean hasAdGroup() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. The AdGroup affected by this change.
*
*
* optional string ad_group = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroup.
*/
@java.lang.Override
public java.lang.String getAdGroup() {
java.lang.Object ref = adGroup_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroup_ = s;
return s;
}
}
/**
*
* Output only. The AdGroup affected by this change.
*
*
* optional string ad_group = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroup.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAdGroupBytes() {
java.lang.Object ref = adGroup_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RESOURCE_STATUS_FIELD_NUMBER = 8;
private int resourceStatus_;
/**
*
* Output only. Represents the status of the changed resource.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation resource_status = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for resourceStatus.
*/
@java.lang.Override public int getResourceStatusValue() {
return resourceStatus_;
}
/**
*
* Output only. Represents the status of the changed resource.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation resource_status = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The resourceStatus.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation getResourceStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation result = com.google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation.valueOf(resourceStatus_);
return result == null ? com.google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation.UNRECOGNIZED : result;
}
public static final int AD_GROUP_AD_FIELD_NUMBER = 25;
private volatile java.lang.Object adGroupAd_;
/**
*
* Output only. The AdGroupAd affected by this change.
*
*
* optional string ad_group_ad = 25 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the adGroupAd field is set.
*/
@java.lang.Override
public boolean hasAdGroupAd() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. The AdGroupAd affected by this change.
*
*
* optional string ad_group_ad = 25 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroupAd.
*/
@java.lang.Override
public java.lang.String getAdGroupAd() {
java.lang.Object ref = adGroupAd_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroupAd_ = s;
return s;
}
}
/**
*
* Output only. The AdGroupAd affected by this change.
*
*
* optional string ad_group_ad = 25 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroupAd.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAdGroupAdBytes() {
java.lang.Object ref = adGroupAd_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroupAd_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AD_GROUP_CRITERION_FIELD_NUMBER = 26;
private volatile java.lang.Object adGroupCriterion_;
/**
*
* Output only. The AdGroupCriterion affected by this change.
*
*
* optional string ad_group_criterion = 26 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the adGroupCriterion field is set.
*/
@java.lang.Override
public boolean hasAdGroupCriterion() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The AdGroupCriterion affected by this change.
*
*
* optional string ad_group_criterion = 26 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroupCriterion.
*/
@java.lang.Override
public java.lang.String getAdGroupCriterion() {
java.lang.Object ref = adGroupCriterion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroupCriterion_ = s;
return s;
}
}
/**
*
* Output only. The AdGroupCriterion affected by this change.
*
*
* optional string ad_group_criterion = 26 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroupCriterion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAdGroupCriterionBytes() {
java.lang.Object ref = adGroupCriterion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroupCriterion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CAMPAIGN_CRITERION_FIELD_NUMBER = 27;
private volatile java.lang.Object campaignCriterion_;
/**
*
* Output only. The CampaignCriterion affected by this change.
*
*
* optional string campaign_criterion = 27 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the campaignCriterion field is set.
*/
@java.lang.Override
public boolean hasCampaignCriterion() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. The CampaignCriterion affected by this change.
*
*
* optional string campaign_criterion = 27 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The campaignCriterion.
*/
@java.lang.Override
public java.lang.String getCampaignCriterion() {
java.lang.Object ref = campaignCriterion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaignCriterion_ = s;
return s;
}
}
/**
*
* Output only. The CampaignCriterion affected by this change.
*
*
* optional string campaign_criterion = 27 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for campaignCriterion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCampaignCriterionBytes() {
java.lang.Object ref = campaignCriterion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaignCriterion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FEED_FIELD_NUMBER = 28;
private volatile java.lang.Object feed_;
/**
*
* Output only. The Feed affected by this change.
*
*
* optional string feed = 28 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the feed field is set.
*/
@java.lang.Override
public boolean hasFeed() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. The Feed affected by this change.
*
*
* optional string feed = 28 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The feed.
*/
@java.lang.Override
public java.lang.String getFeed() {
java.lang.Object ref = feed_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
feed_ = s;
return s;
}
}
/**
*
* Output only. The Feed affected by this change.
*
*
* optional string feed = 28 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for feed.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFeedBytes() {
java.lang.Object ref = feed_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
feed_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FEED_ITEM_FIELD_NUMBER = 29;
private volatile java.lang.Object feedItem_;
/**
*
* Output only. The FeedItem affected by this change.
*
*
* optional string feed_item = 29 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the feedItem field is set.
*/
@java.lang.Override
public boolean hasFeedItem() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Output only. The FeedItem affected by this change.
*
*
* optional string feed_item = 29 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The feedItem.
*/
@java.lang.Override
public java.lang.String getFeedItem() {
java.lang.Object ref = feedItem_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
feedItem_ = s;
return s;
}
}
/**
*
* Output only. The FeedItem affected by this change.
*
*
* optional string feed_item = 29 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for feedItem.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFeedItemBytes() {
java.lang.Object ref = feedItem_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
feedItem_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AD_GROUP_FEED_FIELD_NUMBER = 30;
private volatile java.lang.Object adGroupFeed_;
/**
*
* Output only. The AdGroupFeed affected by this change.
*
*
* optional string ad_group_feed = 30 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the adGroupFeed field is set.
*/
@java.lang.Override
public boolean hasAdGroupFeed() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Output only. The AdGroupFeed affected by this change.
*
*
* optional string ad_group_feed = 30 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroupFeed.
*/
@java.lang.Override
public java.lang.String getAdGroupFeed() {
java.lang.Object ref = adGroupFeed_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroupFeed_ = s;
return s;
}
}
/**
*
* Output only. The AdGroupFeed affected by this change.
*
*
* optional string ad_group_feed = 30 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroupFeed.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAdGroupFeedBytes() {
java.lang.Object ref = adGroupFeed_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroupFeed_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CAMPAIGN_FEED_FIELD_NUMBER = 31;
private volatile java.lang.Object campaignFeed_;
/**
*
* Output only. The CampaignFeed affected by this change.
*
*
* optional string campaign_feed = 31 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the campaignFeed field is set.
*/
@java.lang.Override
public boolean hasCampaignFeed() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Output only. The CampaignFeed affected by this change.
*
*
* optional string campaign_feed = 31 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The campaignFeed.
*/
@java.lang.Override
public java.lang.String getCampaignFeed() {
java.lang.Object ref = campaignFeed_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaignFeed_ = s;
return s;
}
}
/**
*
* Output only. The CampaignFeed affected by this change.
*
*
* optional string campaign_feed = 31 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for campaignFeed.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCampaignFeedBytes() {
java.lang.Object ref = campaignFeed_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaignFeed_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AD_GROUP_BID_MODIFIER_FIELD_NUMBER = 32;
private volatile java.lang.Object adGroupBidModifier_;
/**
*
* Output only. The AdGroupBidModifier affected by this change.
*
*
* optional string ad_group_bid_modifier = 32 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the adGroupBidModifier field is set.
*/
@java.lang.Override
public boolean hasAdGroupBidModifier() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Output only. The AdGroupBidModifier affected by this change.
*
*
* optional string ad_group_bid_modifier = 32 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroupBidModifier.
*/
@java.lang.Override
public java.lang.String getAdGroupBidModifier() {
java.lang.Object ref = adGroupBidModifier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroupBidModifier_ = s;
return s;
}
}
/**
*
* Output only. The AdGroupBidModifier affected by this change.
*
*
* optional string ad_group_bid_modifier = 32 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroupBidModifier.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAdGroupBidModifierBytes() {
java.lang.Object ref = adGroupBidModifier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroupBidModifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SHARED_SET_FIELD_NUMBER = 33;
private volatile java.lang.Object sharedSet_;
/**
*
* Output only. The SharedSet affected by this change.
*
*
* string shared_set = 33 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The sharedSet.
*/
@java.lang.Override
public java.lang.String getSharedSet() {
java.lang.Object ref = sharedSet_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sharedSet_ = s;
return s;
}
}
/**
*
* Output only. The SharedSet affected by this change.
*
*
* string shared_set = 33 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for sharedSet.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSharedSetBytes() {
java.lang.Object ref = sharedSet_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sharedSet_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CAMPAIGN_SHARED_SET_FIELD_NUMBER = 34;
private volatile java.lang.Object campaignSharedSet_;
/**
*
* Output only. The CampaignSharedSet affected by this change.
*
*
* string campaign_shared_set = 34 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The campaignSharedSet.
*/
@java.lang.Override
public java.lang.String getCampaignSharedSet() {
java.lang.Object ref = campaignSharedSet_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaignSharedSet_ = s;
return s;
}
}
/**
*
* Output only. The CampaignSharedSet affected by this change.
*
*
* string campaign_shared_set = 34 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for campaignSharedSet.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCampaignSharedSetBytes() {
java.lang.Object ref = campaignSharedSet_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaignSharedSet_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ASSET_FIELD_NUMBER = 35;
private volatile java.lang.Object asset_;
/**
*
* Output only. The Asset affected by this change.
*
*
* string asset = 35 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The asset.
*/
@java.lang.Override
public java.lang.String getAsset() {
java.lang.Object ref = asset_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
asset_ = s;
return s;
}
}
/**
*
* Output only. The Asset affected by this change.
*
*
* string asset = 35 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for asset.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAssetBytes() {
java.lang.Object ref = asset_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
asset_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CUSTOMER_ASSET_FIELD_NUMBER = 36;
private volatile java.lang.Object customerAsset_;
/**
*
* Output only. The CustomerAsset affected by this change.
*
*
* string customer_asset = 36 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The customerAsset.
*/
@java.lang.Override
public java.lang.String getCustomerAsset() {
java.lang.Object ref = customerAsset_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerAsset_ = s;
return s;
}
}
/**
*
* Output only. The CustomerAsset affected by this change.
*
*
* string customer_asset = 36 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for customerAsset.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCustomerAssetBytes() {
java.lang.Object ref = customerAsset_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerAsset_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CAMPAIGN_ASSET_FIELD_NUMBER = 37;
private volatile java.lang.Object campaignAsset_;
/**
*
* Output only. The CampaignAsset affected by this change.
*
*
* string campaign_asset = 37 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The campaignAsset.
*/
@java.lang.Override
public java.lang.String getCampaignAsset() {
java.lang.Object ref = campaignAsset_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaignAsset_ = s;
return s;
}
}
/**
*
* Output only. The CampaignAsset affected by this change.
*
*
* string campaign_asset = 37 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for campaignAsset.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCampaignAssetBytes() {
java.lang.Object ref = campaignAsset_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaignAsset_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AD_GROUP_ASSET_FIELD_NUMBER = 38;
private volatile java.lang.Object adGroupAsset_;
/**
*
* Output only. The AdGroupAsset affected by this change.
*
*
* string ad_group_asset = 38 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroupAsset.
*/
@java.lang.Override
public java.lang.String getAdGroupAsset() {
java.lang.Object ref = adGroupAsset_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroupAsset_ = s;
return s;
}
}
/**
*
* Output only. The AdGroupAsset affected by this change.
*
*
* string ad_group_asset = 38 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroupAsset.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAdGroupAssetBytes() {
java.lang.Object ref = adGroupAsset_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroupAsset_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, resourceName_);
}
if (resourceType_ != com.google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType.UNSPECIFIED.getNumber()) {
output.writeEnum(4, resourceType_);
}
if (resourceStatus_ != com.google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation.UNSPECIFIED.getNumber()) {
output.writeEnum(8, resourceStatus_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, campaign_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 18, adGroup_);
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 24, lastChangeDateTime_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 25, adGroupAd_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 26, adGroupCriterion_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 27, campaignCriterion_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 28, feed_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 29, feedItem_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30, adGroupFeed_);
}
if (((bitField0_ & 0x00000200) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 31, campaignFeed_);
}
if (((bitField0_ & 0x00000400) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 32, adGroupBidModifier_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sharedSet_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 33, sharedSet_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(campaignSharedSet_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 34, campaignSharedSet_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(asset_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 35, asset_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(customerAsset_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 36, customerAsset_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(campaignAsset_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 37, campaignAsset_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(adGroupAsset_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 38, adGroupAsset_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, resourceName_);
}
if (resourceType_ != com.google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, resourceType_);
}
if (resourceStatus_ != com.google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(8, resourceStatus_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, campaign_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(18, adGroup_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(24, lastChangeDateTime_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(25, adGroupAd_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(26, adGroupCriterion_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(27, campaignCriterion_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(28, feed_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(29, feedItem_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(30, adGroupFeed_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(31, campaignFeed_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(32, adGroupBidModifier_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sharedSet_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(33, sharedSet_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(campaignSharedSet_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(34, campaignSharedSet_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(asset_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(35, asset_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(customerAsset_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(36, customerAsset_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(campaignAsset_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(37, campaignAsset_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(adGroupAsset_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(38, adGroupAsset_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v10.resources.ChangeStatus)) {
return super.equals(obj);
}
com.google.ads.googleads.v10.resources.ChangeStatus other = (com.google.ads.googleads.v10.resources.ChangeStatus) obj;
if (!getResourceName()
.equals(other.getResourceName())) return false;
if (hasLastChangeDateTime() != other.hasLastChangeDateTime()) return false;
if (hasLastChangeDateTime()) {
if (!getLastChangeDateTime()
.equals(other.getLastChangeDateTime())) return false;
}
if (resourceType_ != other.resourceType_) return false;
if (hasCampaign() != other.hasCampaign()) return false;
if (hasCampaign()) {
if (!getCampaign()
.equals(other.getCampaign())) return false;
}
if (hasAdGroup() != other.hasAdGroup()) return false;
if (hasAdGroup()) {
if (!getAdGroup()
.equals(other.getAdGroup())) return false;
}
if (resourceStatus_ != other.resourceStatus_) return false;
if (hasAdGroupAd() != other.hasAdGroupAd()) return false;
if (hasAdGroupAd()) {
if (!getAdGroupAd()
.equals(other.getAdGroupAd())) return false;
}
if (hasAdGroupCriterion() != other.hasAdGroupCriterion()) return false;
if (hasAdGroupCriterion()) {
if (!getAdGroupCriterion()
.equals(other.getAdGroupCriterion())) return false;
}
if (hasCampaignCriterion() != other.hasCampaignCriterion()) return false;
if (hasCampaignCriterion()) {
if (!getCampaignCriterion()
.equals(other.getCampaignCriterion())) return false;
}
if (hasFeed() != other.hasFeed()) return false;
if (hasFeed()) {
if (!getFeed()
.equals(other.getFeed())) return false;
}
if (hasFeedItem() != other.hasFeedItem()) return false;
if (hasFeedItem()) {
if (!getFeedItem()
.equals(other.getFeedItem())) return false;
}
if (hasAdGroupFeed() != other.hasAdGroupFeed()) return false;
if (hasAdGroupFeed()) {
if (!getAdGroupFeed()
.equals(other.getAdGroupFeed())) return false;
}
if (hasCampaignFeed() != other.hasCampaignFeed()) return false;
if (hasCampaignFeed()) {
if (!getCampaignFeed()
.equals(other.getCampaignFeed())) return false;
}
if (hasAdGroupBidModifier() != other.hasAdGroupBidModifier()) return false;
if (hasAdGroupBidModifier()) {
if (!getAdGroupBidModifier()
.equals(other.getAdGroupBidModifier())) return false;
}
if (!getSharedSet()
.equals(other.getSharedSet())) return false;
if (!getCampaignSharedSet()
.equals(other.getCampaignSharedSet())) return false;
if (!getAsset()
.equals(other.getAsset())) return false;
if (!getCustomerAsset()
.equals(other.getCustomerAsset())) return false;
if (!getCampaignAsset()
.equals(other.getCampaignAsset())) return false;
if (!getAdGroupAsset()
.equals(other.getAdGroupAsset())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RESOURCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getResourceName().hashCode();
if (hasLastChangeDateTime()) {
hash = (37 * hash) + LAST_CHANGE_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getLastChangeDateTime().hashCode();
}
hash = (37 * hash) + RESOURCE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + resourceType_;
if (hasCampaign()) {
hash = (37 * hash) + CAMPAIGN_FIELD_NUMBER;
hash = (53 * hash) + getCampaign().hashCode();
}
if (hasAdGroup()) {
hash = (37 * hash) + AD_GROUP_FIELD_NUMBER;
hash = (53 * hash) + getAdGroup().hashCode();
}
hash = (37 * hash) + RESOURCE_STATUS_FIELD_NUMBER;
hash = (53 * hash) + resourceStatus_;
if (hasAdGroupAd()) {
hash = (37 * hash) + AD_GROUP_AD_FIELD_NUMBER;
hash = (53 * hash) + getAdGroupAd().hashCode();
}
if (hasAdGroupCriterion()) {
hash = (37 * hash) + AD_GROUP_CRITERION_FIELD_NUMBER;
hash = (53 * hash) + getAdGroupCriterion().hashCode();
}
if (hasCampaignCriterion()) {
hash = (37 * hash) + CAMPAIGN_CRITERION_FIELD_NUMBER;
hash = (53 * hash) + getCampaignCriterion().hashCode();
}
if (hasFeed()) {
hash = (37 * hash) + FEED_FIELD_NUMBER;
hash = (53 * hash) + getFeed().hashCode();
}
if (hasFeedItem()) {
hash = (37 * hash) + FEED_ITEM_FIELD_NUMBER;
hash = (53 * hash) + getFeedItem().hashCode();
}
if (hasAdGroupFeed()) {
hash = (37 * hash) + AD_GROUP_FEED_FIELD_NUMBER;
hash = (53 * hash) + getAdGroupFeed().hashCode();
}
if (hasCampaignFeed()) {
hash = (37 * hash) + CAMPAIGN_FEED_FIELD_NUMBER;
hash = (53 * hash) + getCampaignFeed().hashCode();
}
if (hasAdGroupBidModifier()) {
hash = (37 * hash) + AD_GROUP_BID_MODIFIER_FIELD_NUMBER;
hash = (53 * hash) + getAdGroupBidModifier().hashCode();
}
hash = (37 * hash) + SHARED_SET_FIELD_NUMBER;
hash = (53 * hash) + getSharedSet().hashCode();
hash = (37 * hash) + CAMPAIGN_SHARED_SET_FIELD_NUMBER;
hash = (53 * hash) + getCampaignSharedSet().hashCode();
hash = (37 * hash) + ASSET_FIELD_NUMBER;
hash = (53 * hash) + getAsset().hashCode();
hash = (37 * hash) + CUSTOMER_ASSET_FIELD_NUMBER;
hash = (53 * hash) + getCustomerAsset().hashCode();
hash = (37 * hash) + CAMPAIGN_ASSET_FIELD_NUMBER;
hash = (53 * hash) + getCampaignAsset().hashCode();
hash = (37 * hash) + AD_GROUP_ASSET_FIELD_NUMBER;
hash = (53 * hash) + getAdGroupAsset().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.ChangeStatus parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v10.resources.ChangeStatus prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes the status of returned resource. ChangeStatus could have up to 3
* minutes delay to reflect a new change.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.ChangeStatus}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v10.resources.ChangeStatus)
com.google.ads.googleads.v10.resources.ChangeStatusOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.ChangeStatusProto.internal_static_google_ads_googleads_v10_resources_ChangeStatus_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.ChangeStatusProto.internal_static_google_ads_googleads_v10_resources_ChangeStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.ChangeStatus.class, com.google.ads.googleads.v10.resources.ChangeStatus.Builder.class);
}
// Construct using com.google.ads.googleads.v10.resources.ChangeStatus.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
resourceName_ = "";
lastChangeDateTime_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
resourceType_ = 0;
campaign_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
adGroup_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
resourceStatus_ = 0;
adGroupAd_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
adGroupCriterion_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
campaignCriterion_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
feed_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
feedItem_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
adGroupFeed_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
campaignFeed_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
adGroupBidModifier_ = "";
bitField0_ = (bitField0_ & ~0x00000400);
sharedSet_ = "";
campaignSharedSet_ = "";
asset_ = "";
customerAsset_ = "";
campaignAsset_ = "";
adGroupAsset_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v10.resources.ChangeStatusProto.internal_static_google_ads_googleads_v10_resources_ChangeStatus_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.ChangeStatus getDefaultInstanceForType() {
return com.google.ads.googleads.v10.resources.ChangeStatus.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.ChangeStatus build() {
com.google.ads.googleads.v10.resources.ChangeStatus result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.ChangeStatus buildPartial() {
com.google.ads.googleads.v10.resources.ChangeStatus result = new com.google.ads.googleads.v10.resources.ChangeStatus(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.resourceName_ = resourceName_;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.lastChangeDateTime_ = lastChangeDateTime_;
result.resourceType_ = resourceType_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.campaign_ = campaign_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.adGroup_ = adGroup_;
result.resourceStatus_ = resourceStatus_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.adGroupAd_ = adGroupAd_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.adGroupCriterion_ = adGroupCriterion_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.campaignCriterion_ = campaignCriterion_;
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000040;
}
result.feed_ = feed_;
if (((from_bitField0_ & 0x00000080) != 0)) {
to_bitField0_ |= 0x00000080;
}
result.feedItem_ = feedItem_;
if (((from_bitField0_ & 0x00000100) != 0)) {
to_bitField0_ |= 0x00000100;
}
result.adGroupFeed_ = adGroupFeed_;
if (((from_bitField0_ & 0x00000200) != 0)) {
to_bitField0_ |= 0x00000200;
}
result.campaignFeed_ = campaignFeed_;
if (((from_bitField0_ & 0x00000400) != 0)) {
to_bitField0_ |= 0x00000400;
}
result.adGroupBidModifier_ = adGroupBidModifier_;
result.sharedSet_ = sharedSet_;
result.campaignSharedSet_ = campaignSharedSet_;
result.asset_ = asset_;
result.customerAsset_ = customerAsset_;
result.campaignAsset_ = campaignAsset_;
result.adGroupAsset_ = adGroupAsset_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v10.resources.ChangeStatus) {
return mergeFrom((com.google.ads.googleads.v10.resources.ChangeStatus)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v10.resources.ChangeStatus other) {
if (other == com.google.ads.googleads.v10.resources.ChangeStatus.getDefaultInstance()) return this;
if (!other.getResourceName().isEmpty()) {
resourceName_ = other.resourceName_;
onChanged();
}
if (other.hasLastChangeDateTime()) {
bitField0_ |= 0x00000001;
lastChangeDateTime_ = other.lastChangeDateTime_;
onChanged();
}
if (other.resourceType_ != 0) {
setResourceTypeValue(other.getResourceTypeValue());
}
if (other.hasCampaign()) {
bitField0_ |= 0x00000002;
campaign_ = other.campaign_;
onChanged();
}
if (other.hasAdGroup()) {
bitField0_ |= 0x00000004;
adGroup_ = other.adGroup_;
onChanged();
}
if (other.resourceStatus_ != 0) {
setResourceStatusValue(other.getResourceStatusValue());
}
if (other.hasAdGroupAd()) {
bitField0_ |= 0x00000008;
adGroupAd_ = other.adGroupAd_;
onChanged();
}
if (other.hasAdGroupCriterion()) {
bitField0_ |= 0x00000010;
adGroupCriterion_ = other.adGroupCriterion_;
onChanged();
}
if (other.hasCampaignCriterion()) {
bitField0_ |= 0x00000020;
campaignCriterion_ = other.campaignCriterion_;
onChanged();
}
if (other.hasFeed()) {
bitField0_ |= 0x00000040;
feed_ = other.feed_;
onChanged();
}
if (other.hasFeedItem()) {
bitField0_ |= 0x00000080;
feedItem_ = other.feedItem_;
onChanged();
}
if (other.hasAdGroupFeed()) {
bitField0_ |= 0x00000100;
adGroupFeed_ = other.adGroupFeed_;
onChanged();
}
if (other.hasCampaignFeed()) {
bitField0_ |= 0x00000200;
campaignFeed_ = other.campaignFeed_;
onChanged();
}
if (other.hasAdGroupBidModifier()) {
bitField0_ |= 0x00000400;
adGroupBidModifier_ = other.adGroupBidModifier_;
onChanged();
}
if (!other.getSharedSet().isEmpty()) {
sharedSet_ = other.sharedSet_;
onChanged();
}
if (!other.getCampaignSharedSet().isEmpty()) {
campaignSharedSet_ = other.campaignSharedSet_;
onChanged();
}
if (!other.getAsset().isEmpty()) {
asset_ = other.asset_;
onChanged();
}
if (!other.getCustomerAsset().isEmpty()) {
customerAsset_ = other.customerAsset_;
onChanged();
}
if (!other.getCampaignAsset().isEmpty()) {
campaignAsset_ = other.campaignAsset_;
onChanged();
}
if (!other.getAdGroupAsset().isEmpty()) {
adGroupAsset_ = other.adGroupAsset_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
resourceName_ = input.readStringRequireUtf8();
break;
} // case 10
case 32: {
resourceType_ = input.readEnum();
break;
} // case 32
case 64: {
resourceStatus_ = input.readEnum();
break;
} // case 64
case 138: {
campaign_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 138
case 146: {
adGroup_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 146
case 194: {
lastChangeDateTime_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 194
case 202: {
adGroupAd_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 202
case 210: {
adGroupCriterion_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 210
case 218: {
campaignCriterion_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 218
case 226: {
feed_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 226
case 234: {
feedItem_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 234
case 242: {
adGroupFeed_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case 242
case 250: {
campaignFeed_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case 250
case 258: {
adGroupBidModifier_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000400;
break;
} // case 258
case 266: {
sharedSet_ = input.readStringRequireUtf8();
break;
} // case 266
case 274: {
campaignSharedSet_ = input.readStringRequireUtf8();
break;
} // case 274
case 282: {
asset_ = input.readStringRequireUtf8();
break;
} // case 282
case 290: {
customerAsset_ = input.readStringRequireUtf8();
break;
} // case 290
case 298: {
campaignAsset_ = input.readStringRequireUtf8();
break;
} // case 298
case 306: {
adGroupAsset_ = input.readStringRequireUtf8();
break;
} // case 306
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object resourceName_ = "";
/**
*
* Output only. The resource name of the change status.
* Change status resource names have the form:
* `customers/{customer_id}/changeStatus/{change_status_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The resource name of the change status.
* Change status resource names have the form:
* `customers/{customer_id}/changeStatus/{change_status_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The resource name of the change status.
* Change status resource names have the form:
* `customers/{customer_id}/changeStatus/{change_status_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resourceName_ = value;
onChanged();
return this;
}
/**
*
* Output only. The resource name of the change status.
* Change status resource names have the form:
* `customers/{customer_id}/changeStatus/{change_status_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearResourceName() {
resourceName_ = getDefaultInstance().getResourceName();
onChanged();
return this;
}
/**
*
* Output only. The resource name of the change status.
* Change status resource names have the form:
* `customers/{customer_id}/changeStatus/{change_status_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resourceName_ = value;
onChanged();
return this;
}
private java.lang.Object lastChangeDateTime_ = "";
/**
*
* Output only. Time at which the most recent change has occurred on this resource.
*
*
* optional string last_change_date_time = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the lastChangeDateTime field is set.
*/
public boolean hasLastChangeDateTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. Time at which the most recent change has occurred on this resource.
*
*
* optional string last_change_date_time = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The lastChangeDateTime.
*/
public java.lang.String getLastChangeDateTime() {
java.lang.Object ref = lastChangeDateTime_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
lastChangeDateTime_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. Time at which the most recent change has occurred on this resource.
*
*
* optional string last_change_date_time = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for lastChangeDateTime.
*/
public com.google.protobuf.ByteString
getLastChangeDateTimeBytes() {
java.lang.Object ref = lastChangeDateTime_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
lastChangeDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. Time at which the most recent change has occurred on this resource.
*
*
* optional string last_change_date_time = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The lastChangeDateTime to set.
* @return This builder for chaining.
*/
public Builder setLastChangeDateTime(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
lastChangeDateTime_ = value;
onChanged();
return this;
}
/**
*
* Output only. Time at which the most recent change has occurred on this resource.
*
*
* optional string last_change_date_time = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearLastChangeDateTime() {
bitField0_ = (bitField0_ & ~0x00000001);
lastChangeDateTime_ = getDefaultInstance().getLastChangeDateTime();
onChanged();
return this;
}
/**
*
* Output only. Time at which the most recent change has occurred on this resource.
*
*
* optional string last_change_date_time = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for lastChangeDateTime to set.
* @return This builder for chaining.
*/
public Builder setLastChangeDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000001;
lastChangeDateTime_ = value;
onChanged();
return this;
}
private int resourceType_ = 0;
/**
*
* Output only. Represents the type of the changed resource. This dictates what fields
* will be set. For example, for AD_GROUP, campaign and ad_group fields will
* be set.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType resource_type = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for resourceType.
*/
@java.lang.Override public int getResourceTypeValue() {
return resourceType_;
}
/**
*
* Output only. Represents the type of the changed resource. This dictates what fields
* will be set. For example, for AD_GROUP, campaign and ad_group fields will
* be set.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType resource_type = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for resourceType to set.
* @return This builder for chaining.
*/
public Builder setResourceTypeValue(int value) {
resourceType_ = value;
onChanged();
return this;
}
/**
*
* Output only. Represents the type of the changed resource. This dictates what fields
* will be set. For example, for AD_GROUP, campaign and ad_group fields will
* be set.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType resource_type = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The resourceType.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType getResourceType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType result = com.google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType.valueOf(resourceType_);
return result == null ? com.google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType.UNRECOGNIZED : result;
}
/**
*
* Output only. Represents the type of the changed resource. This dictates what fields
* will be set. For example, for AD_GROUP, campaign and ad_group fields will
* be set.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType resource_type = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The resourceType to set.
* @return This builder for chaining.
*/
public Builder setResourceType(com.google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType value) {
if (value == null) {
throw new NullPointerException();
}
resourceType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. Represents the type of the changed resource. This dictates what fields
* will be set. For example, for AD_GROUP, campaign and ad_group fields will
* be set.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusResourceTypeEnum.ChangeStatusResourceType resource_type = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearResourceType() {
resourceType_ = 0;
onChanged();
return this;
}
private java.lang.Object campaign_ = "";
/**
*
* Output only. The Campaign affected by this change.
*
*
* optional string campaign = 17 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the campaign field is set.
*/
public boolean hasCampaign() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The Campaign affected by this change.
*
*
* optional string campaign = 17 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The campaign.
*/
public java.lang.String getCampaign() {
java.lang.Object ref = campaign_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaign_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The Campaign affected by this change.
*
*
* optional string campaign = 17 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for campaign.
*/
public com.google.protobuf.ByteString
getCampaignBytes() {
java.lang.Object ref = campaign_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaign_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The Campaign affected by this change.
*
*
* optional string campaign = 17 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The campaign to set.
* @return This builder for chaining.
*/
public Builder setCampaign(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
campaign_ = value;
onChanged();
return this;
}
/**
*
* Output only. The Campaign affected by this change.
*
*
* optional string campaign = 17 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearCampaign() {
bitField0_ = (bitField0_ & ~0x00000002);
campaign_ = getDefaultInstance().getCampaign();
onChanged();
return this;
}
/**
*
* Output only. The Campaign affected by this change.
*
*
* optional string campaign = 17 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for campaign to set.
* @return This builder for chaining.
*/
public Builder setCampaignBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000002;
campaign_ = value;
onChanged();
return this;
}
private java.lang.Object adGroup_ = "";
/**
*
* Output only. The AdGroup affected by this change.
*
*
* optional string ad_group = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the adGroup field is set.
*/
public boolean hasAdGroup() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. The AdGroup affected by this change.
*
*
* optional string ad_group = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroup.
*/
public java.lang.String getAdGroup() {
java.lang.Object ref = adGroup_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroup_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The AdGroup affected by this change.
*
*
* optional string ad_group = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroup.
*/
public com.google.protobuf.ByteString
getAdGroupBytes() {
java.lang.Object ref = adGroup_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The AdGroup affected by this change.
*
*
* optional string ad_group = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The adGroup to set.
* @return This builder for chaining.
*/
public Builder setAdGroup(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
adGroup_ = value;
onChanged();
return this;
}
/**
*
* Output only. The AdGroup affected by this change.
*
*
* optional string ad_group = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearAdGroup() {
bitField0_ = (bitField0_ & ~0x00000004);
adGroup_ = getDefaultInstance().getAdGroup();
onChanged();
return this;
}
/**
*
* Output only. The AdGroup affected by this change.
*
*
* optional string ad_group = 18 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for adGroup to set.
* @return This builder for chaining.
*/
public Builder setAdGroupBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000004;
adGroup_ = value;
onChanged();
return this;
}
private int resourceStatus_ = 0;
/**
*
* Output only. Represents the status of the changed resource.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation resource_status = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for resourceStatus.
*/
@java.lang.Override public int getResourceStatusValue() {
return resourceStatus_;
}
/**
*
* Output only. Represents the status of the changed resource.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation resource_status = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for resourceStatus to set.
* @return This builder for chaining.
*/
public Builder setResourceStatusValue(int value) {
resourceStatus_ = value;
onChanged();
return this;
}
/**
*
* Output only. Represents the status of the changed resource.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation resource_status = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The resourceStatus.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation getResourceStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation result = com.google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation.valueOf(resourceStatus_);
return result == null ? com.google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation.UNRECOGNIZED : result;
}
/**
*
* Output only. Represents the status of the changed resource.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation resource_status = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The resourceStatus to set.
* @return This builder for chaining.
*/
public Builder setResourceStatus(com.google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation value) {
if (value == null) {
throw new NullPointerException();
}
resourceStatus_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. Represents the status of the changed resource.
*
*
* .google.ads.googleads.v10.enums.ChangeStatusOperationEnum.ChangeStatusOperation resource_status = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearResourceStatus() {
resourceStatus_ = 0;
onChanged();
return this;
}
private java.lang.Object adGroupAd_ = "";
/**
*
* Output only. The AdGroupAd affected by this change.
*
*
* optional string ad_group_ad = 25 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the adGroupAd field is set.
*/
public boolean hasAdGroupAd() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. The AdGroupAd affected by this change.
*
*
* optional string ad_group_ad = 25 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroupAd.
*/
public java.lang.String getAdGroupAd() {
java.lang.Object ref = adGroupAd_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroupAd_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The AdGroupAd affected by this change.
*
*
* optional string ad_group_ad = 25 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroupAd.
*/
public com.google.protobuf.ByteString
getAdGroupAdBytes() {
java.lang.Object ref = adGroupAd_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroupAd_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The AdGroupAd affected by this change.
*
*
* optional string ad_group_ad = 25 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The adGroupAd to set.
* @return This builder for chaining.
*/
public Builder setAdGroupAd(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
adGroupAd_ = value;
onChanged();
return this;
}
/**
*
* Output only. The AdGroupAd affected by this change.
*
*
* optional string ad_group_ad = 25 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearAdGroupAd() {
bitField0_ = (bitField0_ & ~0x00000008);
adGroupAd_ = getDefaultInstance().getAdGroupAd();
onChanged();
return this;
}
/**
*
* Output only. The AdGroupAd affected by this change.
*
*
* optional string ad_group_ad = 25 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for adGroupAd to set.
* @return This builder for chaining.
*/
public Builder setAdGroupAdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000008;
adGroupAd_ = value;
onChanged();
return this;
}
private java.lang.Object adGroupCriterion_ = "";
/**
*
* Output only. The AdGroupCriterion affected by this change.
*
*
* optional string ad_group_criterion = 26 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the adGroupCriterion field is set.
*/
public boolean hasAdGroupCriterion() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The AdGroupCriterion affected by this change.
*
*
* optional string ad_group_criterion = 26 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroupCriterion.
*/
public java.lang.String getAdGroupCriterion() {
java.lang.Object ref = adGroupCriterion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroupCriterion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The AdGroupCriterion affected by this change.
*
*
* optional string ad_group_criterion = 26 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroupCriterion.
*/
public com.google.protobuf.ByteString
getAdGroupCriterionBytes() {
java.lang.Object ref = adGroupCriterion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroupCriterion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The AdGroupCriterion affected by this change.
*
*
* optional string ad_group_criterion = 26 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The adGroupCriterion to set.
* @return This builder for chaining.
*/
public Builder setAdGroupCriterion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
adGroupCriterion_ = value;
onChanged();
return this;
}
/**
*
* Output only. The AdGroupCriterion affected by this change.
*
*
* optional string ad_group_criterion = 26 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearAdGroupCriterion() {
bitField0_ = (bitField0_ & ~0x00000010);
adGroupCriterion_ = getDefaultInstance().getAdGroupCriterion();
onChanged();
return this;
}
/**
*
* Output only. The AdGroupCriterion affected by this change.
*
*
* optional string ad_group_criterion = 26 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for adGroupCriterion to set.
* @return This builder for chaining.
*/
public Builder setAdGroupCriterionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000010;
adGroupCriterion_ = value;
onChanged();
return this;
}
private java.lang.Object campaignCriterion_ = "";
/**
*
* Output only. The CampaignCriterion affected by this change.
*
*
* optional string campaign_criterion = 27 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the campaignCriterion field is set.
*/
public boolean hasCampaignCriterion() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. The CampaignCriterion affected by this change.
*
*
* optional string campaign_criterion = 27 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The campaignCriterion.
*/
public java.lang.String getCampaignCriterion() {
java.lang.Object ref = campaignCriterion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaignCriterion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The CampaignCriterion affected by this change.
*
*
* optional string campaign_criterion = 27 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for campaignCriterion.
*/
public com.google.protobuf.ByteString
getCampaignCriterionBytes() {
java.lang.Object ref = campaignCriterion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaignCriterion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The CampaignCriterion affected by this change.
*
*
* optional string campaign_criterion = 27 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The campaignCriterion to set.
* @return This builder for chaining.
*/
public Builder setCampaignCriterion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
campaignCriterion_ = value;
onChanged();
return this;
}
/**
*
* Output only. The CampaignCriterion affected by this change.
*
*
* optional string campaign_criterion = 27 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearCampaignCriterion() {
bitField0_ = (bitField0_ & ~0x00000020);
campaignCriterion_ = getDefaultInstance().getCampaignCriterion();
onChanged();
return this;
}
/**
*
* Output only. The CampaignCriterion affected by this change.
*
*
* optional string campaign_criterion = 27 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for campaignCriterion to set.
* @return This builder for chaining.
*/
public Builder setCampaignCriterionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000020;
campaignCriterion_ = value;
onChanged();
return this;
}
private java.lang.Object feed_ = "";
/**
*
* Output only. The Feed affected by this change.
*
*
* optional string feed = 28 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the feed field is set.
*/
public boolean hasFeed() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. The Feed affected by this change.
*
*
* optional string feed = 28 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The feed.
*/
public java.lang.String getFeed() {
java.lang.Object ref = feed_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
feed_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The Feed affected by this change.
*
*
* optional string feed = 28 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for feed.
*/
public com.google.protobuf.ByteString
getFeedBytes() {
java.lang.Object ref = feed_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
feed_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The Feed affected by this change.
*
*
* optional string feed = 28 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The feed to set.
* @return This builder for chaining.
*/
public Builder setFeed(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
feed_ = value;
onChanged();
return this;
}
/**
*
* Output only. The Feed affected by this change.
*
*
* optional string feed = 28 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearFeed() {
bitField0_ = (bitField0_ & ~0x00000040);
feed_ = getDefaultInstance().getFeed();
onChanged();
return this;
}
/**
*
* Output only. The Feed affected by this change.
*
*
* optional string feed = 28 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for feed to set.
* @return This builder for chaining.
*/
public Builder setFeedBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000040;
feed_ = value;
onChanged();
return this;
}
private java.lang.Object feedItem_ = "";
/**
*
* Output only. The FeedItem affected by this change.
*
*
* optional string feed_item = 29 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the feedItem field is set.
*/
public boolean hasFeedItem() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Output only. The FeedItem affected by this change.
*
*
* optional string feed_item = 29 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The feedItem.
*/
public java.lang.String getFeedItem() {
java.lang.Object ref = feedItem_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
feedItem_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The FeedItem affected by this change.
*
*
* optional string feed_item = 29 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for feedItem.
*/
public com.google.protobuf.ByteString
getFeedItemBytes() {
java.lang.Object ref = feedItem_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
feedItem_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The FeedItem affected by this change.
*
*
* optional string feed_item = 29 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The feedItem to set.
* @return This builder for chaining.
*/
public Builder setFeedItem(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
feedItem_ = value;
onChanged();
return this;
}
/**
*
* Output only. The FeedItem affected by this change.
*
*
* optional string feed_item = 29 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearFeedItem() {
bitField0_ = (bitField0_ & ~0x00000080);
feedItem_ = getDefaultInstance().getFeedItem();
onChanged();
return this;
}
/**
*
* Output only. The FeedItem affected by this change.
*
*
* optional string feed_item = 29 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for feedItem to set.
* @return This builder for chaining.
*/
public Builder setFeedItemBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000080;
feedItem_ = value;
onChanged();
return this;
}
private java.lang.Object adGroupFeed_ = "";
/**
*
* Output only. The AdGroupFeed affected by this change.
*
*
* optional string ad_group_feed = 30 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the adGroupFeed field is set.
*/
public boolean hasAdGroupFeed() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Output only. The AdGroupFeed affected by this change.
*
*
* optional string ad_group_feed = 30 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroupFeed.
*/
public java.lang.String getAdGroupFeed() {
java.lang.Object ref = adGroupFeed_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroupFeed_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The AdGroupFeed affected by this change.
*
*
* optional string ad_group_feed = 30 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroupFeed.
*/
public com.google.protobuf.ByteString
getAdGroupFeedBytes() {
java.lang.Object ref = adGroupFeed_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroupFeed_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The AdGroupFeed affected by this change.
*
*
* optional string ad_group_feed = 30 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The adGroupFeed to set.
* @return This builder for chaining.
*/
public Builder setAdGroupFeed(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
adGroupFeed_ = value;
onChanged();
return this;
}
/**
*
* Output only. The AdGroupFeed affected by this change.
*
*
* optional string ad_group_feed = 30 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearAdGroupFeed() {
bitField0_ = (bitField0_ & ~0x00000100);
adGroupFeed_ = getDefaultInstance().getAdGroupFeed();
onChanged();
return this;
}
/**
*
* Output only. The AdGroupFeed affected by this change.
*
*
* optional string ad_group_feed = 30 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for adGroupFeed to set.
* @return This builder for chaining.
*/
public Builder setAdGroupFeedBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000100;
adGroupFeed_ = value;
onChanged();
return this;
}
private java.lang.Object campaignFeed_ = "";
/**
*
* Output only. The CampaignFeed affected by this change.
*
*
* optional string campaign_feed = 31 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the campaignFeed field is set.
*/
public boolean hasCampaignFeed() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Output only. The CampaignFeed affected by this change.
*
*
* optional string campaign_feed = 31 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The campaignFeed.
*/
public java.lang.String getCampaignFeed() {
java.lang.Object ref = campaignFeed_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaignFeed_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The CampaignFeed affected by this change.
*
*
* optional string campaign_feed = 31 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for campaignFeed.
*/
public com.google.protobuf.ByteString
getCampaignFeedBytes() {
java.lang.Object ref = campaignFeed_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaignFeed_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The CampaignFeed affected by this change.
*
*
* optional string campaign_feed = 31 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The campaignFeed to set.
* @return This builder for chaining.
*/
public Builder setCampaignFeed(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
campaignFeed_ = value;
onChanged();
return this;
}
/**
*
* Output only. The CampaignFeed affected by this change.
*
*
* optional string campaign_feed = 31 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearCampaignFeed() {
bitField0_ = (bitField0_ & ~0x00000200);
campaignFeed_ = getDefaultInstance().getCampaignFeed();
onChanged();
return this;
}
/**
*
* Output only. The CampaignFeed affected by this change.
*
*
* optional string campaign_feed = 31 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for campaignFeed to set.
* @return This builder for chaining.
*/
public Builder setCampaignFeedBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000200;
campaignFeed_ = value;
onChanged();
return this;
}
private java.lang.Object adGroupBidModifier_ = "";
/**
*
* Output only. The AdGroupBidModifier affected by this change.
*
*
* optional string ad_group_bid_modifier = 32 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return Whether the adGroupBidModifier field is set.
*/
public boolean hasAdGroupBidModifier() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Output only. The AdGroupBidModifier affected by this change.
*
*
* optional string ad_group_bid_modifier = 32 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroupBidModifier.
*/
public java.lang.String getAdGroupBidModifier() {
java.lang.Object ref = adGroupBidModifier_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroupBidModifier_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The AdGroupBidModifier affected by this change.
*
*
* optional string ad_group_bid_modifier = 32 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroupBidModifier.
*/
public com.google.protobuf.ByteString
getAdGroupBidModifierBytes() {
java.lang.Object ref = adGroupBidModifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroupBidModifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The AdGroupBidModifier affected by this change.
*
*
* optional string ad_group_bid_modifier = 32 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The adGroupBidModifier to set.
* @return This builder for chaining.
*/
public Builder setAdGroupBidModifier(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
adGroupBidModifier_ = value;
onChanged();
return this;
}
/**
*
* Output only. The AdGroupBidModifier affected by this change.
*
*
* optional string ad_group_bid_modifier = 32 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearAdGroupBidModifier() {
bitField0_ = (bitField0_ & ~0x00000400);
adGroupBidModifier_ = getDefaultInstance().getAdGroupBidModifier();
onChanged();
return this;
}
/**
*
* Output only. The AdGroupBidModifier affected by this change.
*
*
* optional string ad_group_bid_modifier = 32 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for adGroupBidModifier to set.
* @return This builder for chaining.
*/
public Builder setAdGroupBidModifierBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000400;
adGroupBidModifier_ = value;
onChanged();
return this;
}
private java.lang.Object sharedSet_ = "";
/**
*
* Output only. The SharedSet affected by this change.
*
*
* string shared_set = 33 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The sharedSet.
*/
public java.lang.String getSharedSet() {
java.lang.Object ref = sharedSet_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sharedSet_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The SharedSet affected by this change.
*
*
* string shared_set = 33 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for sharedSet.
*/
public com.google.protobuf.ByteString
getSharedSetBytes() {
java.lang.Object ref = sharedSet_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sharedSet_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The SharedSet affected by this change.
*
*
* string shared_set = 33 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The sharedSet to set.
* @return This builder for chaining.
*/
public Builder setSharedSet(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sharedSet_ = value;
onChanged();
return this;
}
/**
*
* Output only. The SharedSet affected by this change.
*
*
* string shared_set = 33 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearSharedSet() {
sharedSet_ = getDefaultInstance().getSharedSet();
onChanged();
return this;
}
/**
*
* Output only. The SharedSet affected by this change.
*
*
* string shared_set = 33 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for sharedSet to set.
* @return This builder for chaining.
*/
public Builder setSharedSetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sharedSet_ = value;
onChanged();
return this;
}
private java.lang.Object campaignSharedSet_ = "";
/**
*
* Output only. The CampaignSharedSet affected by this change.
*
*
* string campaign_shared_set = 34 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The campaignSharedSet.
*/
public java.lang.String getCampaignSharedSet() {
java.lang.Object ref = campaignSharedSet_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaignSharedSet_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The CampaignSharedSet affected by this change.
*
*
* string campaign_shared_set = 34 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for campaignSharedSet.
*/
public com.google.protobuf.ByteString
getCampaignSharedSetBytes() {
java.lang.Object ref = campaignSharedSet_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaignSharedSet_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The CampaignSharedSet affected by this change.
*
*
* string campaign_shared_set = 34 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The campaignSharedSet to set.
* @return This builder for chaining.
*/
public Builder setCampaignSharedSet(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
campaignSharedSet_ = value;
onChanged();
return this;
}
/**
*
* Output only. The CampaignSharedSet affected by this change.
*
*
* string campaign_shared_set = 34 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearCampaignSharedSet() {
campaignSharedSet_ = getDefaultInstance().getCampaignSharedSet();
onChanged();
return this;
}
/**
*
* Output only. The CampaignSharedSet affected by this change.
*
*
* string campaign_shared_set = 34 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for campaignSharedSet to set.
* @return This builder for chaining.
*/
public Builder setCampaignSharedSetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
campaignSharedSet_ = value;
onChanged();
return this;
}
private java.lang.Object asset_ = "";
/**
*
* Output only. The Asset affected by this change.
*
*
* string asset = 35 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The asset.
*/
public java.lang.String getAsset() {
java.lang.Object ref = asset_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
asset_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The Asset affected by this change.
*
*
* string asset = 35 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for asset.
*/
public com.google.protobuf.ByteString
getAssetBytes() {
java.lang.Object ref = asset_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
asset_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The Asset affected by this change.
*
*
* string asset = 35 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The asset to set.
* @return This builder for chaining.
*/
public Builder setAsset(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
asset_ = value;
onChanged();
return this;
}
/**
*
* Output only. The Asset affected by this change.
*
*
* string asset = 35 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearAsset() {
asset_ = getDefaultInstance().getAsset();
onChanged();
return this;
}
/**
*
* Output only. The Asset affected by this change.
*
*
* string asset = 35 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for asset to set.
* @return This builder for chaining.
*/
public Builder setAssetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
asset_ = value;
onChanged();
return this;
}
private java.lang.Object customerAsset_ = "";
/**
*
* Output only. The CustomerAsset affected by this change.
*
*
* string customer_asset = 36 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The customerAsset.
*/
public java.lang.String getCustomerAsset() {
java.lang.Object ref = customerAsset_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerAsset_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The CustomerAsset affected by this change.
*
*
* string customer_asset = 36 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for customerAsset.
*/
public com.google.protobuf.ByteString
getCustomerAssetBytes() {
java.lang.Object ref = customerAsset_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerAsset_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The CustomerAsset affected by this change.
*
*
* string customer_asset = 36 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The customerAsset to set.
* @return This builder for chaining.
*/
public Builder setCustomerAsset(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
customerAsset_ = value;
onChanged();
return this;
}
/**
*
* Output only. The CustomerAsset affected by this change.
*
*
* string customer_asset = 36 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearCustomerAsset() {
customerAsset_ = getDefaultInstance().getCustomerAsset();
onChanged();
return this;
}
/**
*
* Output only. The CustomerAsset affected by this change.
*
*
* string customer_asset = 36 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for customerAsset to set.
* @return This builder for chaining.
*/
public Builder setCustomerAssetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
customerAsset_ = value;
onChanged();
return this;
}
private java.lang.Object campaignAsset_ = "";
/**
*
* Output only. The CampaignAsset affected by this change.
*
*
* string campaign_asset = 37 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The campaignAsset.
*/
public java.lang.String getCampaignAsset() {
java.lang.Object ref = campaignAsset_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
campaignAsset_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The CampaignAsset affected by this change.
*
*
* string campaign_asset = 37 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for campaignAsset.
*/
public com.google.protobuf.ByteString
getCampaignAssetBytes() {
java.lang.Object ref = campaignAsset_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
campaignAsset_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The CampaignAsset affected by this change.
*
*
* string campaign_asset = 37 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The campaignAsset to set.
* @return This builder for chaining.
*/
public Builder setCampaignAsset(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
campaignAsset_ = value;
onChanged();
return this;
}
/**
*
* Output only. The CampaignAsset affected by this change.
*
*
* string campaign_asset = 37 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearCampaignAsset() {
campaignAsset_ = getDefaultInstance().getCampaignAsset();
onChanged();
return this;
}
/**
*
* Output only. The CampaignAsset affected by this change.
*
*
* string campaign_asset = 37 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for campaignAsset to set.
* @return This builder for chaining.
*/
public Builder setCampaignAssetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
campaignAsset_ = value;
onChanged();
return this;
}
private java.lang.Object adGroupAsset_ = "";
/**
*
* Output only. The AdGroupAsset affected by this change.
*
*
* string ad_group_asset = 38 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The adGroupAsset.
*/
public java.lang.String getAdGroupAsset() {
java.lang.Object ref = adGroupAsset_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adGroupAsset_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The AdGroupAsset affected by this change.
*
*
* string ad_group_asset = 38 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for adGroupAsset.
*/
public com.google.protobuf.ByteString
getAdGroupAssetBytes() {
java.lang.Object ref = adGroupAsset_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adGroupAsset_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The AdGroupAsset affected by this change.
*
*
* string ad_group_asset = 38 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The adGroupAsset to set.
* @return This builder for chaining.
*/
public Builder setAdGroupAsset(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
adGroupAsset_ = value;
onChanged();
return this;
}
/**
*
* Output only. The AdGroupAsset affected by this change.
*
*
* string ad_group_asset = 38 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearAdGroupAsset() {
adGroupAsset_ = getDefaultInstance().getAdGroupAsset();
onChanged();
return this;
}
/**
*
* Output only. The AdGroupAsset affected by this change.
*
*
* string ad_group_asset = 38 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for adGroupAsset to set.
* @return This builder for chaining.
*/
public Builder setAdGroupAssetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
adGroupAsset_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v10.resources.ChangeStatus)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v10.resources.ChangeStatus)
private static final com.google.ads.googleads.v10.resources.ChangeStatus DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v10.resources.ChangeStatus();
}
public static com.google.ads.googleads.v10.resources.ChangeStatus getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ChangeStatus parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.ChangeStatus getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy