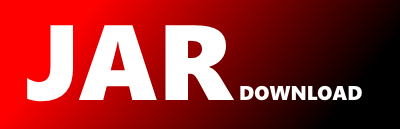
com.google.ads.googleads.v10.resources.Invoice Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v10/resources/invoice.proto
package com.google.ads.googleads.v10.resources;
/**
*
* An invoice. All invoice information is snapshotted to match the PDF invoice.
* For invoices older than the launch of InvoiceService, the snapshotted
* information may not match the PDF invoice.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.Invoice}
*/
public final class Invoice extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v10.resources.Invoice)
InvoiceOrBuilder {
private static final long serialVersionUID = 0L;
// Use Invoice.newBuilder() to construct.
private Invoice(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Invoice() {
resourceName_ = "";
id_ = "";
type_ = 0;
billingSetup_ = "";
paymentsAccountId_ = "";
paymentsProfileId_ = "";
issueDate_ = "";
dueDate_ = "";
currencyCode_ = "";
correctedInvoice_ = "";
replacedInvoices_ = com.google.protobuf.LazyStringArrayList.EMPTY;
pdfUrl_ = "";
accountBudgetSummaries_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Invoice();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.InvoiceProto.internal_static_google_ads_googleads_v10_resources_Invoice_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.InvoiceProto.internal_static_google_ads_googleads_v10_resources_Invoice_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.Invoice.class, com.google.ads.googleads.v10.resources.Invoice.Builder.class);
}
public interface AccountBudgetSummaryOrBuilder extends
// @@protoc_insertion_point(interface_extends:google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary)
com.google.protobuf.MessageOrBuilder {
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the customer field is set.
*/
boolean hasCustomer();
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The customer.
*/
java.lang.String getCustomer();
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for customer.
*/
com.google.protobuf.ByteString
getCustomerBytes();
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the customerDescriptiveName field is set.
*/
boolean hasCustomerDescriptiveName();
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The customerDescriptiveName.
*/
java.lang.String getCustomerDescriptiveName();
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for customerDescriptiveName.
*/
com.google.protobuf.ByteString
getCustomerDescriptiveNameBytes();
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the accountBudget field is set.
*/
boolean hasAccountBudget();
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The accountBudget.
*/
java.lang.String getAccountBudget();
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for accountBudget.
*/
com.google.protobuf.ByteString
getAccountBudgetBytes();
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the accountBudgetName field is set.
*/
boolean hasAccountBudgetName();
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The accountBudgetName.
*/
java.lang.String getAccountBudgetName();
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for accountBudgetName.
*/
com.google.protobuf.ByteString
getAccountBudgetNameBytes();
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the purchaseOrderNumber field is set.
*/
boolean hasPurchaseOrderNumber();
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The purchaseOrderNumber.
*/
java.lang.String getPurchaseOrderNumber();
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for purchaseOrderNumber.
*/
com.google.protobuf.ByteString
getPurchaseOrderNumberBytes();
/**
*
* Output only. The pretax subtotal amount attributable to this budget during the service
* period, in micros.
*
*
* optional int64 subtotal_amount_micros = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the subtotalAmountMicros field is set.
*/
boolean hasSubtotalAmountMicros();
/**
*
* Output only. The pretax subtotal amount attributable to this budget during the service
* period, in micros.
*
*
* optional int64 subtotal_amount_micros = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The subtotalAmountMicros.
*/
long getSubtotalAmountMicros();
/**
*
* Output only. The tax amount attributable to this budget during the service period, in
* micros.
*
*
* optional int64 tax_amount_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the taxAmountMicros field is set.
*/
boolean hasTaxAmountMicros();
/**
*
* Output only. The tax amount attributable to this budget during the service period, in
* micros.
*
*
* optional int64 tax_amount_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The taxAmountMicros.
*/
long getTaxAmountMicros();
/**
*
* Output only. The total amount attributable to this budget during the service period,
* in micros. This equals the sum of the account budget subtotal amount and
* the account budget tax amount.
*
*
* optional int64 total_amount_micros = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the totalAmountMicros field is set.
*/
boolean hasTotalAmountMicros();
/**
*
* Output only. The total amount attributable to this budget during the service period,
* in micros. This equals the sum of the account budget subtotal amount and
* the account budget tax amount.
*
*
* optional int64 total_amount_micros = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The totalAmountMicros.
*/
long getTotalAmountMicros();
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the billableActivityDateRange field is set.
*/
boolean hasBillableActivityDateRange();
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The billableActivityDateRange.
*/
com.google.ads.googleads.v10.common.DateRange getBillableActivityDateRange();
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
com.google.ads.googleads.v10.common.DateRangeOrBuilder getBillableActivityDateRangeOrBuilder();
}
/**
*
* Represents a summarized account budget billable cost.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary}
*/
public static final class AccountBudgetSummary extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary)
AccountBudgetSummaryOrBuilder {
private static final long serialVersionUID = 0L;
// Use AccountBudgetSummary.newBuilder() to construct.
private AccountBudgetSummary(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AccountBudgetSummary() {
customer_ = "";
customerDescriptiveName_ = "";
accountBudget_ = "";
accountBudgetName_ = "";
purchaseOrderNumber_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AccountBudgetSummary();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.InvoiceProto.internal_static_google_ads_googleads_v10_resources_Invoice_AccountBudgetSummary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.InvoiceProto.internal_static_google_ads_googleads_v10_resources_Invoice_AccountBudgetSummary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.class, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.Builder.class);
}
private int bitField0_;
public static final int CUSTOMER_FIELD_NUMBER = 10;
private volatile java.lang.Object customer_;
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the customer field is set.
*/
@java.lang.Override
public boolean hasCustomer() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The customer.
*/
@java.lang.Override
public java.lang.String getCustomer() {
java.lang.Object ref = customer_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customer_ = s;
return s;
}
}
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for customer.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCustomerBytes() {
java.lang.Object ref = customer_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CUSTOMER_DESCRIPTIVE_NAME_FIELD_NUMBER = 11;
private volatile java.lang.Object customerDescriptiveName_;
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the customerDescriptiveName field is set.
*/
@java.lang.Override
public boolean hasCustomerDescriptiveName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The customerDescriptiveName.
*/
@java.lang.Override
public java.lang.String getCustomerDescriptiveName() {
java.lang.Object ref = customerDescriptiveName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerDescriptiveName_ = s;
return s;
}
}
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for customerDescriptiveName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCustomerDescriptiveNameBytes() {
java.lang.Object ref = customerDescriptiveName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerDescriptiveName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACCOUNT_BUDGET_FIELD_NUMBER = 12;
private volatile java.lang.Object accountBudget_;
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the accountBudget field is set.
*/
@java.lang.Override
public boolean hasAccountBudget() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The accountBudget.
*/
@java.lang.Override
public java.lang.String getAccountBudget() {
java.lang.Object ref = accountBudget_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountBudget_ = s;
return s;
}
}
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for accountBudget.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAccountBudgetBytes() {
java.lang.Object ref = accountBudget_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountBudget_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACCOUNT_BUDGET_NAME_FIELD_NUMBER = 13;
private volatile java.lang.Object accountBudgetName_;
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the accountBudgetName field is set.
*/
@java.lang.Override
public boolean hasAccountBudgetName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The accountBudgetName.
*/
@java.lang.Override
public java.lang.String getAccountBudgetName() {
java.lang.Object ref = accountBudgetName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountBudgetName_ = s;
return s;
}
}
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for accountBudgetName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAccountBudgetNameBytes() {
java.lang.Object ref = accountBudgetName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountBudgetName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PURCHASE_ORDER_NUMBER_FIELD_NUMBER = 14;
private volatile java.lang.Object purchaseOrderNumber_;
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the purchaseOrderNumber field is set.
*/
@java.lang.Override
public boolean hasPurchaseOrderNumber() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The purchaseOrderNumber.
*/
@java.lang.Override
public java.lang.String getPurchaseOrderNumber() {
java.lang.Object ref = purchaseOrderNumber_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
purchaseOrderNumber_ = s;
return s;
}
}
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for purchaseOrderNumber.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPurchaseOrderNumberBytes() {
java.lang.Object ref = purchaseOrderNumber_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
purchaseOrderNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBTOTAL_AMOUNT_MICROS_FIELD_NUMBER = 15;
private long subtotalAmountMicros_;
/**
*
* Output only. The pretax subtotal amount attributable to this budget during the service
* period, in micros.
*
*
* optional int64 subtotal_amount_micros = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the subtotalAmountMicros field is set.
*/
@java.lang.Override
public boolean hasSubtotalAmountMicros() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. The pretax subtotal amount attributable to this budget during the service
* period, in micros.
*
*
* optional int64 subtotal_amount_micros = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The subtotalAmountMicros.
*/
@java.lang.Override
public long getSubtotalAmountMicros() {
return subtotalAmountMicros_;
}
public static final int TAX_AMOUNT_MICROS_FIELD_NUMBER = 16;
private long taxAmountMicros_;
/**
*
* Output only. The tax amount attributable to this budget during the service period, in
* micros.
*
*
* optional int64 tax_amount_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the taxAmountMicros field is set.
*/
@java.lang.Override
public boolean hasTaxAmountMicros() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. The tax amount attributable to this budget during the service period, in
* micros.
*
*
* optional int64 tax_amount_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The taxAmountMicros.
*/
@java.lang.Override
public long getTaxAmountMicros() {
return taxAmountMicros_;
}
public static final int TOTAL_AMOUNT_MICROS_FIELD_NUMBER = 17;
private long totalAmountMicros_;
/**
*
* Output only. The total amount attributable to this budget during the service period,
* in micros. This equals the sum of the account budget subtotal amount and
* the account budget tax amount.
*
*
* optional int64 total_amount_micros = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the totalAmountMicros field is set.
*/
@java.lang.Override
public boolean hasTotalAmountMicros() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Output only. The total amount attributable to this budget during the service period,
* in micros. This equals the sum of the account budget subtotal amount and
* the account budget tax amount.
*
*
* optional int64 total_amount_micros = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The totalAmountMicros.
*/
@java.lang.Override
public long getTotalAmountMicros() {
return totalAmountMicros_;
}
public static final int BILLABLE_ACTIVITY_DATE_RANGE_FIELD_NUMBER = 9;
private com.google.ads.googleads.v10.common.DateRange billableActivityDateRange_;
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the billableActivityDateRange field is set.
*/
@java.lang.Override
public boolean hasBillableActivityDateRange() {
return billableActivityDateRange_ != null;
}
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The billableActivityDateRange.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.DateRange getBillableActivityDateRange() {
return billableActivityDateRange_ == null ? com.google.ads.googleads.v10.common.DateRange.getDefaultInstance() : billableActivityDateRange_;
}
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.DateRangeOrBuilder getBillableActivityDateRangeOrBuilder() {
return getBillableActivityDateRange();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (billableActivityDateRange_ != null) {
output.writeMessage(9, getBillableActivityDateRange());
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, customer_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, customerDescriptiveName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, accountBudget_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, accountBudgetName_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, purchaseOrderNumber_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt64(15, subtotalAmountMicros_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeInt64(16, taxAmountMicros_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeInt64(17, totalAmountMicros_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (billableActivityDateRange_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getBillableActivityDateRange());
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, customer_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, customerDescriptiveName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, accountBudget_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, accountBudgetName_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, purchaseOrderNumber_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(15, subtotalAmountMicros_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(16, taxAmountMicros_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(17, totalAmountMicros_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary)) {
return super.equals(obj);
}
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary other = (com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary) obj;
if (hasCustomer() != other.hasCustomer()) return false;
if (hasCustomer()) {
if (!getCustomer()
.equals(other.getCustomer())) return false;
}
if (hasCustomerDescriptiveName() != other.hasCustomerDescriptiveName()) return false;
if (hasCustomerDescriptiveName()) {
if (!getCustomerDescriptiveName()
.equals(other.getCustomerDescriptiveName())) return false;
}
if (hasAccountBudget() != other.hasAccountBudget()) return false;
if (hasAccountBudget()) {
if (!getAccountBudget()
.equals(other.getAccountBudget())) return false;
}
if (hasAccountBudgetName() != other.hasAccountBudgetName()) return false;
if (hasAccountBudgetName()) {
if (!getAccountBudgetName()
.equals(other.getAccountBudgetName())) return false;
}
if (hasPurchaseOrderNumber() != other.hasPurchaseOrderNumber()) return false;
if (hasPurchaseOrderNumber()) {
if (!getPurchaseOrderNumber()
.equals(other.getPurchaseOrderNumber())) return false;
}
if (hasSubtotalAmountMicros() != other.hasSubtotalAmountMicros()) return false;
if (hasSubtotalAmountMicros()) {
if (getSubtotalAmountMicros()
!= other.getSubtotalAmountMicros()) return false;
}
if (hasTaxAmountMicros() != other.hasTaxAmountMicros()) return false;
if (hasTaxAmountMicros()) {
if (getTaxAmountMicros()
!= other.getTaxAmountMicros()) return false;
}
if (hasTotalAmountMicros() != other.hasTotalAmountMicros()) return false;
if (hasTotalAmountMicros()) {
if (getTotalAmountMicros()
!= other.getTotalAmountMicros()) return false;
}
if (hasBillableActivityDateRange() != other.hasBillableActivityDateRange()) return false;
if (hasBillableActivityDateRange()) {
if (!getBillableActivityDateRange()
.equals(other.getBillableActivityDateRange())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCustomer()) {
hash = (37 * hash) + CUSTOMER_FIELD_NUMBER;
hash = (53 * hash) + getCustomer().hashCode();
}
if (hasCustomerDescriptiveName()) {
hash = (37 * hash) + CUSTOMER_DESCRIPTIVE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getCustomerDescriptiveName().hashCode();
}
if (hasAccountBudget()) {
hash = (37 * hash) + ACCOUNT_BUDGET_FIELD_NUMBER;
hash = (53 * hash) + getAccountBudget().hashCode();
}
if (hasAccountBudgetName()) {
hash = (37 * hash) + ACCOUNT_BUDGET_NAME_FIELD_NUMBER;
hash = (53 * hash) + getAccountBudgetName().hashCode();
}
if (hasPurchaseOrderNumber()) {
hash = (37 * hash) + PURCHASE_ORDER_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getPurchaseOrderNumber().hashCode();
}
if (hasSubtotalAmountMicros()) {
hash = (37 * hash) + SUBTOTAL_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSubtotalAmountMicros());
}
if (hasTaxAmountMicros()) {
hash = (37 * hash) + TAX_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTaxAmountMicros());
}
if (hasTotalAmountMicros()) {
hash = (37 * hash) + TOTAL_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTotalAmountMicros());
}
if (hasBillableActivityDateRange()) {
hash = (37 * hash) + BILLABLE_ACTIVITY_DATE_RANGE_FIELD_NUMBER;
hash = (53 * hash) + getBillableActivityDateRange().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Represents a summarized account budget billable cost.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary)
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummaryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.InvoiceProto.internal_static_google_ads_googleads_v10_resources_Invoice_AccountBudgetSummary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.InvoiceProto.internal_static_google_ads_googleads_v10_resources_Invoice_AccountBudgetSummary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.class, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.Builder.class);
}
// Construct using com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
customer_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
customerDescriptiveName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
accountBudget_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
accountBudgetName_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
purchaseOrderNumber_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
subtotalAmountMicros_ = 0L;
bitField0_ = (bitField0_ & ~0x00000020);
taxAmountMicros_ = 0L;
bitField0_ = (bitField0_ & ~0x00000040);
totalAmountMicros_ = 0L;
bitField0_ = (bitField0_ & ~0x00000080);
if (billableActivityDateRangeBuilder_ == null) {
billableActivityDateRange_ = null;
} else {
billableActivityDateRange_ = null;
billableActivityDateRangeBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v10.resources.InvoiceProto.internal_static_google_ads_googleads_v10_resources_Invoice_AccountBudgetSummary_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary getDefaultInstanceForType() {
return com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary build() {
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary buildPartial() {
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary result = new com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.customer_ = customer_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.customerDescriptiveName_ = customerDescriptiveName_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.accountBudget_ = accountBudget_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.accountBudgetName_ = accountBudgetName_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.purchaseOrderNumber_ = purchaseOrderNumber_;
if (((from_bitField0_ & 0x00000020) != 0)) {
result.subtotalAmountMicros_ = subtotalAmountMicros_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.taxAmountMicros_ = taxAmountMicros_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.totalAmountMicros_ = totalAmountMicros_;
to_bitField0_ |= 0x00000080;
}
if (billableActivityDateRangeBuilder_ == null) {
result.billableActivityDateRange_ = billableActivityDateRange_;
} else {
result.billableActivityDateRange_ = billableActivityDateRangeBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary) {
return mergeFrom((com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary other) {
if (other == com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.getDefaultInstance()) return this;
if (other.hasCustomer()) {
bitField0_ |= 0x00000001;
customer_ = other.customer_;
onChanged();
}
if (other.hasCustomerDescriptiveName()) {
bitField0_ |= 0x00000002;
customerDescriptiveName_ = other.customerDescriptiveName_;
onChanged();
}
if (other.hasAccountBudget()) {
bitField0_ |= 0x00000004;
accountBudget_ = other.accountBudget_;
onChanged();
}
if (other.hasAccountBudgetName()) {
bitField0_ |= 0x00000008;
accountBudgetName_ = other.accountBudgetName_;
onChanged();
}
if (other.hasPurchaseOrderNumber()) {
bitField0_ |= 0x00000010;
purchaseOrderNumber_ = other.purchaseOrderNumber_;
onChanged();
}
if (other.hasSubtotalAmountMicros()) {
setSubtotalAmountMicros(other.getSubtotalAmountMicros());
}
if (other.hasTaxAmountMicros()) {
setTaxAmountMicros(other.getTaxAmountMicros());
}
if (other.hasTotalAmountMicros()) {
setTotalAmountMicros(other.getTotalAmountMicros());
}
if (other.hasBillableActivityDateRange()) {
mergeBillableActivityDateRange(other.getBillableActivityDateRange());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 74: {
input.readMessage(
getBillableActivityDateRangeFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 74
case 82: {
customer_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 82
case 90: {
customerDescriptiveName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 90
case 98: {
accountBudget_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 98
case 106: {
accountBudgetName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 106
case 114: {
purchaseOrderNumber_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 114
case 120: {
subtotalAmountMicros_ = input.readInt64();
bitField0_ |= 0x00000020;
break;
} // case 120
case 128: {
taxAmountMicros_ = input.readInt64();
bitField0_ |= 0x00000040;
break;
} // case 128
case 136: {
totalAmountMicros_ = input.readInt64();
bitField0_ |= 0x00000080;
break;
} // case 136
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object customer_ = "";
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the customer field is set.
*/
public boolean hasCustomer() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The customer.
*/
public java.lang.String getCustomer() {
java.lang.Object ref = customer_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customer_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for customer.
*/
public com.google.protobuf.ByteString
getCustomerBytes() {
java.lang.Object ref = customer_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The customer to set.
* @return This builder for chaining.
*/
public Builder setCustomer(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
customer_ = value;
onChanged();
return this;
}
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearCustomer() {
bitField0_ = (bitField0_ & ~0x00000001);
customer_ = getDefaultInstance().getCustomer();
onChanged();
return this;
}
/**
*
* Output only. The resource name of the customer associated with this account budget.
* This contains the customer ID, which appears on the invoice PDF as
* "Account ID".
* Customer resource names have the form:
* `customers/{customer_id}`
*
*
* optional string customer = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for customer to set.
* @return This builder for chaining.
*/
public Builder setCustomerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000001;
customer_ = value;
onChanged();
return this;
}
private java.lang.Object customerDescriptiveName_ = "";
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the customerDescriptiveName field is set.
*/
public boolean hasCustomerDescriptiveName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The customerDescriptiveName.
*/
public java.lang.String getCustomerDescriptiveName() {
java.lang.Object ref = customerDescriptiveName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerDescriptiveName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for customerDescriptiveName.
*/
public com.google.protobuf.ByteString
getCustomerDescriptiveNameBytes() {
java.lang.Object ref = customerDescriptiveName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerDescriptiveName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The customerDescriptiveName to set.
* @return This builder for chaining.
*/
public Builder setCustomerDescriptiveName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
customerDescriptiveName_ = value;
onChanged();
return this;
}
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearCustomerDescriptiveName() {
bitField0_ = (bitField0_ & ~0x00000002);
customerDescriptiveName_ = getDefaultInstance().getCustomerDescriptiveName();
onChanged();
return this;
}
/**
*
* Output only. The descriptive name of the account budget's customer. It appears on the
* invoice PDF as "Account".
*
*
* optional string customer_descriptive_name = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for customerDescriptiveName to set.
* @return This builder for chaining.
*/
public Builder setCustomerDescriptiveNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000002;
customerDescriptiveName_ = value;
onChanged();
return this;
}
private java.lang.Object accountBudget_ = "";
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the accountBudget field is set.
*/
public boolean hasAccountBudget() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The accountBudget.
*/
public java.lang.String getAccountBudget() {
java.lang.Object ref = accountBudget_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountBudget_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for accountBudget.
*/
public com.google.protobuf.ByteString
getAccountBudgetBytes() {
java.lang.Object ref = accountBudget_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountBudget_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The accountBudget to set.
* @return This builder for chaining.
*/
public Builder setAccountBudget(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
accountBudget_ = value;
onChanged();
return this;
}
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearAccountBudget() {
bitField0_ = (bitField0_ & ~0x00000004);
accountBudget_ = getDefaultInstance().getAccountBudget();
onChanged();
return this;
}
/**
*
* Output only. The resource name of the account budget associated with this summarized
* billable cost.
* AccountBudget resource names have the form:
* `customers/{customer_id}/accountBudgets/{account_budget_id}`
*
*
* optional string account_budget = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for accountBudget to set.
* @return This builder for chaining.
*/
public Builder setAccountBudgetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000004;
accountBudget_ = value;
onChanged();
return this;
}
private java.lang.Object accountBudgetName_ = "";
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the accountBudgetName field is set.
*/
public boolean hasAccountBudgetName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The accountBudgetName.
*/
public java.lang.String getAccountBudgetName() {
java.lang.Object ref = accountBudgetName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accountBudgetName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for accountBudgetName.
*/
public com.google.protobuf.ByteString
getAccountBudgetNameBytes() {
java.lang.Object ref = accountBudgetName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accountBudgetName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The accountBudgetName to set.
* @return This builder for chaining.
*/
public Builder setAccountBudgetName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
accountBudgetName_ = value;
onChanged();
return this;
}
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearAccountBudgetName() {
bitField0_ = (bitField0_ & ~0x00000008);
accountBudgetName_ = getDefaultInstance().getAccountBudgetName();
onChanged();
return this;
}
/**
*
* Output only. The name of the account budget. It appears on the invoice PDF as "Account
* budget".
*
*
* optional string account_budget_name = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for accountBudgetName to set.
* @return This builder for chaining.
*/
public Builder setAccountBudgetNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000008;
accountBudgetName_ = value;
onChanged();
return this;
}
private java.lang.Object purchaseOrderNumber_ = "";
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the purchaseOrderNumber field is set.
*/
public boolean hasPurchaseOrderNumber() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The purchaseOrderNumber.
*/
public java.lang.String getPurchaseOrderNumber() {
java.lang.Object ref = purchaseOrderNumber_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
purchaseOrderNumber_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for purchaseOrderNumber.
*/
public com.google.protobuf.ByteString
getPurchaseOrderNumberBytes() {
java.lang.Object ref = purchaseOrderNumber_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
purchaseOrderNumber_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The purchaseOrderNumber to set.
* @return This builder for chaining.
*/
public Builder setPurchaseOrderNumber(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
purchaseOrderNumber_ = value;
onChanged();
return this;
}
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearPurchaseOrderNumber() {
bitField0_ = (bitField0_ & ~0x00000010);
purchaseOrderNumber_ = getDefaultInstance().getPurchaseOrderNumber();
onChanged();
return this;
}
/**
*
* Output only. The purchase order number of the account budget. It appears on the
* invoice PDF as "Purchase order".
*
*
* optional string purchase_order_number = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for purchaseOrderNumber to set.
* @return This builder for chaining.
*/
public Builder setPurchaseOrderNumberBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000010;
purchaseOrderNumber_ = value;
onChanged();
return this;
}
private long subtotalAmountMicros_ ;
/**
*
* Output only. The pretax subtotal amount attributable to this budget during the service
* period, in micros.
*
*
* optional int64 subtotal_amount_micros = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the subtotalAmountMicros field is set.
*/
@java.lang.Override
public boolean hasSubtotalAmountMicros() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. The pretax subtotal amount attributable to this budget during the service
* period, in micros.
*
*
* optional int64 subtotal_amount_micros = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The subtotalAmountMicros.
*/
@java.lang.Override
public long getSubtotalAmountMicros() {
return subtotalAmountMicros_;
}
/**
*
* Output only. The pretax subtotal amount attributable to this budget during the service
* period, in micros.
*
*
* optional int64 subtotal_amount_micros = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The subtotalAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setSubtotalAmountMicros(long value) {
bitField0_ |= 0x00000020;
subtotalAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The pretax subtotal amount attributable to this budget during the service
* period, in micros.
*
*
* optional int64 subtotal_amount_micros = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearSubtotalAmountMicros() {
bitField0_ = (bitField0_ & ~0x00000020);
subtotalAmountMicros_ = 0L;
onChanged();
return this;
}
private long taxAmountMicros_ ;
/**
*
* Output only. The tax amount attributable to this budget during the service period, in
* micros.
*
*
* optional int64 tax_amount_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the taxAmountMicros field is set.
*/
@java.lang.Override
public boolean hasTaxAmountMicros() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. The tax amount attributable to this budget during the service period, in
* micros.
*
*
* optional int64 tax_amount_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The taxAmountMicros.
*/
@java.lang.Override
public long getTaxAmountMicros() {
return taxAmountMicros_;
}
/**
*
* Output only. The tax amount attributable to this budget during the service period, in
* micros.
*
*
* optional int64 tax_amount_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The taxAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setTaxAmountMicros(long value) {
bitField0_ |= 0x00000040;
taxAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The tax amount attributable to this budget during the service period, in
* micros.
*
*
* optional int64 tax_amount_micros = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearTaxAmountMicros() {
bitField0_ = (bitField0_ & ~0x00000040);
taxAmountMicros_ = 0L;
onChanged();
return this;
}
private long totalAmountMicros_ ;
/**
*
* Output only. The total amount attributable to this budget during the service period,
* in micros. This equals the sum of the account budget subtotal amount and
* the account budget tax amount.
*
*
* optional int64 total_amount_micros = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the totalAmountMicros field is set.
*/
@java.lang.Override
public boolean hasTotalAmountMicros() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Output only. The total amount attributable to this budget during the service period,
* in micros. This equals the sum of the account budget subtotal amount and
* the account budget tax amount.
*
*
* optional int64 total_amount_micros = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The totalAmountMicros.
*/
@java.lang.Override
public long getTotalAmountMicros() {
return totalAmountMicros_;
}
/**
*
* Output only. The total amount attributable to this budget during the service period,
* in micros. This equals the sum of the account budget subtotal amount and
* the account budget tax amount.
*
*
* optional int64 total_amount_micros = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The totalAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setTotalAmountMicros(long value) {
bitField0_ |= 0x00000080;
totalAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The total amount attributable to this budget during the service period,
* in micros. This equals the sum of the account budget subtotal amount and
* the account budget tax amount.
*
*
* optional int64 total_amount_micros = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearTotalAmountMicros() {
bitField0_ = (bitField0_ & ~0x00000080);
totalAmountMicros_ = 0L;
onChanged();
return this;
}
private com.google.ads.googleads.v10.common.DateRange billableActivityDateRange_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.DateRange, com.google.ads.googleads.v10.common.DateRange.Builder, com.google.ads.googleads.v10.common.DateRangeOrBuilder> billableActivityDateRangeBuilder_;
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the billableActivityDateRange field is set.
*/
public boolean hasBillableActivityDateRange() {
return billableActivityDateRangeBuilder_ != null || billableActivityDateRange_ != null;
}
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The billableActivityDateRange.
*/
public com.google.ads.googleads.v10.common.DateRange getBillableActivityDateRange() {
if (billableActivityDateRangeBuilder_ == null) {
return billableActivityDateRange_ == null ? com.google.ads.googleads.v10.common.DateRange.getDefaultInstance() : billableActivityDateRange_;
} else {
return billableActivityDateRangeBuilder_.getMessage();
}
}
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder setBillableActivityDateRange(com.google.ads.googleads.v10.common.DateRange value) {
if (billableActivityDateRangeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
billableActivityDateRange_ = value;
onChanged();
} else {
billableActivityDateRangeBuilder_.setMessage(value);
}
return this;
}
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder setBillableActivityDateRange(
com.google.ads.googleads.v10.common.DateRange.Builder builderForValue) {
if (billableActivityDateRangeBuilder_ == null) {
billableActivityDateRange_ = builderForValue.build();
onChanged();
} else {
billableActivityDateRangeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder mergeBillableActivityDateRange(com.google.ads.googleads.v10.common.DateRange value) {
if (billableActivityDateRangeBuilder_ == null) {
if (billableActivityDateRange_ != null) {
billableActivityDateRange_ =
com.google.ads.googleads.v10.common.DateRange.newBuilder(billableActivityDateRange_).mergeFrom(value).buildPartial();
} else {
billableActivityDateRange_ = value;
}
onChanged();
} else {
billableActivityDateRangeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder clearBillableActivityDateRange() {
if (billableActivityDateRangeBuilder_ == null) {
billableActivityDateRange_ = null;
onChanged();
} else {
billableActivityDateRange_ = null;
billableActivityDateRangeBuilder_ = null;
}
return this;
}
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.common.DateRange.Builder getBillableActivityDateRangeBuilder() {
onChanged();
return getBillableActivityDateRangeFieldBuilder().getBuilder();
}
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.common.DateRangeOrBuilder getBillableActivityDateRangeOrBuilder() {
if (billableActivityDateRangeBuilder_ != null) {
return billableActivityDateRangeBuilder_.getMessageOrBuilder();
} else {
return billableActivityDateRange_ == null ?
com.google.ads.googleads.v10.common.DateRange.getDefaultInstance() : billableActivityDateRange_;
}
}
/**
*
* Output only. The billable activity date range of the account budget, within the
* service date range of this invoice. The end date is inclusive. This can
* be different from the account budget's start and end time.
*
*
* .google.ads.googleads.v10.common.DateRange billable_activity_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.DateRange, com.google.ads.googleads.v10.common.DateRange.Builder, com.google.ads.googleads.v10.common.DateRangeOrBuilder>
getBillableActivityDateRangeFieldBuilder() {
if (billableActivityDateRangeBuilder_ == null) {
billableActivityDateRangeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.DateRange, com.google.ads.googleads.v10.common.DateRange.Builder, com.google.ads.googleads.v10.common.DateRangeOrBuilder>(
getBillableActivityDateRange(),
getParentForChildren(),
isClean());
billableActivityDateRange_ = null;
}
return billableActivityDateRangeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary)
private static final com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary();
}
public static com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AccountBudgetSummary parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int RESOURCE_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object resourceName_;
/**
*
* Output only. The resource name of the invoice. Multiple customers can share a given
* invoice, so multiple resource names may point to the same invoice.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
@java.lang.Override
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
}
}
/**
*
* Output only. The resource name of the invoice. Multiple customers can share a given
* invoice, so multiple resource names may point to the same invoice.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 25;
private volatile java.lang.Object id_;
/**
*
* Output only. The ID of the invoice. It appears on the invoice PDF as "Invoice number".
*
*
* optional string id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The ID of the invoice. It appears on the invoice PDF as "Invoice number".
*
*
* optional string id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* Output only. The ID of the invoice. It appears on the invoice PDF as "Invoice number".
*
*
* optional string id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 3;
private int type_;
/**
*
* Output only. The type of invoice.
*
*
* .google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType type = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
*
* Output only. The type of invoice.
*
*
* .google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType type = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The type.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType getType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType result = com.google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType.valueOf(type_);
return result == null ? com.google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType.UNRECOGNIZED : result;
}
public static final int BILLING_SETUP_FIELD_NUMBER = 26;
private volatile java.lang.Object billingSetup_;
/**
*
* Output only. The resource name of this invoice's billing setup.
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the billingSetup field is set.
*/
@java.lang.Override
public boolean hasBillingSetup() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The resource name of this invoice's billing setup.
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The billingSetup.
*/
@java.lang.Override
public java.lang.String getBillingSetup() {
java.lang.Object ref = billingSetup_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
billingSetup_ = s;
return s;
}
}
/**
*
* Output only. The resource name of this invoice's billing setup.
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for billingSetup.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getBillingSetupBytes() {
java.lang.Object ref = billingSetup_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
billingSetup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAYMENTS_ACCOUNT_ID_FIELD_NUMBER = 27;
private volatile java.lang.Object paymentsAccountId_;
/**
*
* Output only. A 16 digit ID used to identify the payments account associated with the
* billing setup, for example, "1234-5678-9012-3456". It appears on the
* invoice PDF as "Billing Account Number".
*
*
* optional string payments_account_id = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the paymentsAccountId field is set.
*/
@java.lang.Override
public boolean hasPaymentsAccountId() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. A 16 digit ID used to identify the payments account associated with the
* billing setup, for example, "1234-5678-9012-3456". It appears on the
* invoice PDF as "Billing Account Number".
*
*
* optional string payments_account_id = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The paymentsAccountId.
*/
@java.lang.Override
public java.lang.String getPaymentsAccountId() {
java.lang.Object ref = paymentsAccountId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
paymentsAccountId_ = s;
return s;
}
}
/**
*
* Output only. A 16 digit ID used to identify the payments account associated with the
* billing setup, for example, "1234-5678-9012-3456". It appears on the
* invoice PDF as "Billing Account Number".
*
*
* optional string payments_account_id = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for paymentsAccountId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPaymentsAccountIdBytes() {
java.lang.Object ref = paymentsAccountId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
paymentsAccountId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAYMENTS_PROFILE_ID_FIELD_NUMBER = 28;
private volatile java.lang.Object paymentsProfileId_;
/**
*
* Output only. A 12 digit ID used to identify the payments profile associated with the
* billing setup, for example, "1234-5678-9012". It appears on the invoice PDF
* as "Billing ID".
*
*
* optional string payments_profile_id = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the paymentsProfileId field is set.
*/
@java.lang.Override
public boolean hasPaymentsProfileId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. A 12 digit ID used to identify the payments profile associated with the
* billing setup, for example, "1234-5678-9012". It appears on the invoice PDF
* as "Billing ID".
*
*
* optional string payments_profile_id = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The paymentsProfileId.
*/
@java.lang.Override
public java.lang.String getPaymentsProfileId() {
java.lang.Object ref = paymentsProfileId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
paymentsProfileId_ = s;
return s;
}
}
/**
*
* Output only. A 12 digit ID used to identify the payments profile associated with the
* billing setup, for example, "1234-5678-9012". It appears on the invoice PDF
* as "Billing ID".
*
*
* optional string payments_profile_id = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for paymentsProfileId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPaymentsProfileIdBytes() {
java.lang.Object ref = paymentsProfileId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
paymentsProfileId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ISSUE_DATE_FIELD_NUMBER = 29;
private volatile java.lang.Object issueDate_;
/**
*
* Output only. The issue date in yyyy-mm-dd format. It appears on the invoice PDF as
* either "Issue date" or "Invoice date".
*
*
* optional string issue_date = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the issueDate field is set.
*/
@java.lang.Override
public boolean hasIssueDate() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The issue date in yyyy-mm-dd format. It appears on the invoice PDF as
* either "Issue date" or "Invoice date".
*
*
* optional string issue_date = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The issueDate.
*/
@java.lang.Override
public java.lang.String getIssueDate() {
java.lang.Object ref = issueDate_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
issueDate_ = s;
return s;
}
}
/**
*
* Output only. The issue date in yyyy-mm-dd format. It appears on the invoice PDF as
* either "Issue date" or "Invoice date".
*
*
* optional string issue_date = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for issueDate.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIssueDateBytes() {
java.lang.Object ref = issueDate_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
issueDate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DUE_DATE_FIELD_NUMBER = 30;
private volatile java.lang.Object dueDate_;
/**
*
* Output only. The due date in yyyy-mm-dd format.
*
*
* optional string due_date = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the dueDate field is set.
*/
@java.lang.Override
public boolean hasDueDate() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. The due date in yyyy-mm-dd format.
*
*
* optional string due_date = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The dueDate.
*/
@java.lang.Override
public java.lang.String getDueDate() {
java.lang.Object ref = dueDate_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
dueDate_ = s;
return s;
}
}
/**
*
* Output only. The due date in yyyy-mm-dd format.
*
*
* optional string due_date = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for dueDate.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDueDateBytes() {
java.lang.Object ref = dueDate_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dueDate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERVICE_DATE_RANGE_FIELD_NUMBER = 9;
private com.google.ads.googleads.v10.common.DateRange serviceDateRange_;
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the serviceDateRange field is set.
*/
@java.lang.Override
public boolean hasServiceDateRange() {
return serviceDateRange_ != null;
}
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The serviceDateRange.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.DateRange getServiceDateRange() {
return serviceDateRange_ == null ? com.google.ads.googleads.v10.common.DateRange.getDefaultInstance() : serviceDateRange_;
}
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.DateRangeOrBuilder getServiceDateRangeOrBuilder() {
return getServiceDateRange();
}
public static final int CURRENCY_CODE_FIELD_NUMBER = 31;
private volatile java.lang.Object currencyCode_;
/**
*
* Output only. The currency code. All costs are returned in this currency. A subset of the
* currency codes derived from the ISO 4217 standard is supported.
*
*
* optional string currency_code = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the currencyCode field is set.
*/
@java.lang.Override
public boolean hasCurrencyCode() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. The currency code. All costs are returned in this currency. A subset of the
* currency codes derived from the ISO 4217 standard is supported.
*
*
* optional string currency_code = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The currencyCode.
*/
@java.lang.Override
public java.lang.String getCurrencyCode() {
java.lang.Object ref = currencyCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currencyCode_ = s;
return s;
}
}
/**
*
* Output only. The currency code. All costs are returned in this currency. A subset of the
* currency codes derived from the ISO 4217 standard is supported.
*
*
* optional string currency_code = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for currencyCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCurrencyCodeBytes() {
java.lang.Object ref = currencyCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ADJUSTMENTS_SUBTOTAL_AMOUNT_MICROS_FIELD_NUMBER = 19;
private long adjustmentsSubtotalAmountMicros_;
/**
*
* Output only. The pretax subtotal amount of invoice level adjustments, in micros.
*
*
* int64 adjustments_subtotal_amount_micros = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The adjustmentsSubtotalAmountMicros.
*/
@java.lang.Override
public long getAdjustmentsSubtotalAmountMicros() {
return adjustmentsSubtotalAmountMicros_;
}
public static final int ADJUSTMENTS_TAX_AMOUNT_MICROS_FIELD_NUMBER = 20;
private long adjustmentsTaxAmountMicros_;
/**
*
* Output only. The sum of taxes on the invoice level adjustments, in micros.
*
*
* int64 adjustments_tax_amount_micros = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The adjustmentsTaxAmountMicros.
*/
@java.lang.Override
public long getAdjustmentsTaxAmountMicros() {
return adjustmentsTaxAmountMicros_;
}
public static final int ADJUSTMENTS_TOTAL_AMOUNT_MICROS_FIELD_NUMBER = 21;
private long adjustmentsTotalAmountMicros_;
/**
*
* Output only. The total amount of invoice level adjustments, in micros.
*
*
* int64 adjustments_total_amount_micros = 21 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The adjustmentsTotalAmountMicros.
*/
@java.lang.Override
public long getAdjustmentsTotalAmountMicros() {
return adjustmentsTotalAmountMicros_;
}
public static final int REGULATORY_COSTS_SUBTOTAL_AMOUNT_MICROS_FIELD_NUMBER = 22;
private long regulatoryCostsSubtotalAmountMicros_;
/**
*
* Output only. The pretax subtotal amount of invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_subtotal_amount_micros = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The regulatoryCostsSubtotalAmountMicros.
*/
@java.lang.Override
public long getRegulatoryCostsSubtotalAmountMicros() {
return regulatoryCostsSubtotalAmountMicros_;
}
public static final int REGULATORY_COSTS_TAX_AMOUNT_MICROS_FIELD_NUMBER = 23;
private long regulatoryCostsTaxAmountMicros_;
/**
*
* Output only. The sum of taxes on the invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_tax_amount_micros = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The regulatoryCostsTaxAmountMicros.
*/
@java.lang.Override
public long getRegulatoryCostsTaxAmountMicros() {
return regulatoryCostsTaxAmountMicros_;
}
public static final int REGULATORY_COSTS_TOTAL_AMOUNT_MICROS_FIELD_NUMBER = 24;
private long regulatoryCostsTotalAmountMicros_;
/**
*
* Output only. The total amount of invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_total_amount_micros = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The regulatoryCostsTotalAmountMicros.
*/
@java.lang.Override
public long getRegulatoryCostsTotalAmountMicros() {
return regulatoryCostsTotalAmountMicros_;
}
public static final int SUBTOTAL_AMOUNT_MICROS_FIELD_NUMBER = 33;
private long subtotalAmountMicros_;
/**
*
* Output only. The pretax subtotal amount, in micros. This equals the
* sum of the AccountBudgetSummary subtotal amounts,
* Invoice.adjustments_subtotal_amount_micros, and
* Invoice.regulatory_costs_subtotal_amount_micros.
* Starting with v6, the Invoice.regulatory_costs_subtotal_amount_micros is no
* longer included.
*
*
* optional int64 subtotal_amount_micros = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the subtotalAmountMicros field is set.
*/
@java.lang.Override
public boolean hasSubtotalAmountMicros() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Output only. The pretax subtotal amount, in micros. This equals the
* sum of the AccountBudgetSummary subtotal amounts,
* Invoice.adjustments_subtotal_amount_micros, and
* Invoice.regulatory_costs_subtotal_amount_micros.
* Starting with v6, the Invoice.regulatory_costs_subtotal_amount_micros is no
* longer included.
*
*
* optional int64 subtotal_amount_micros = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The subtotalAmountMicros.
*/
@java.lang.Override
public long getSubtotalAmountMicros() {
return subtotalAmountMicros_;
}
public static final int TAX_AMOUNT_MICROS_FIELD_NUMBER = 34;
private long taxAmountMicros_;
/**
*
* Output only. The sum of all taxes on the invoice, in micros. This equals the sum of the
* AccountBudgetSummary tax amounts, plus taxes not associated with a specific
* account budget.
*
*
* optional int64 tax_amount_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the taxAmountMicros field is set.
*/
@java.lang.Override
public boolean hasTaxAmountMicros() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Output only. The sum of all taxes on the invoice, in micros. This equals the sum of the
* AccountBudgetSummary tax amounts, plus taxes not associated with a specific
* account budget.
*
*
* optional int64 tax_amount_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The taxAmountMicros.
*/
@java.lang.Override
public long getTaxAmountMicros() {
return taxAmountMicros_;
}
public static final int TOTAL_AMOUNT_MICROS_FIELD_NUMBER = 35;
private long totalAmountMicros_;
/**
*
* Output only. The total amount, in micros. This equals the sum of
* Invoice.subtotal_amount_micros and Invoice.tax_amount_micros.
* Starting with v6, Invoice.regulatory_costs_subtotal_amount_micros is
* also added as it is no longer already included in
* Invoice.tax_amount_micros.
*
*
* optional int64 total_amount_micros = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the totalAmountMicros field is set.
*/
@java.lang.Override
public boolean hasTotalAmountMicros() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Output only. The total amount, in micros. This equals the sum of
* Invoice.subtotal_amount_micros and Invoice.tax_amount_micros.
* Starting with v6, Invoice.regulatory_costs_subtotal_amount_micros is
* also added as it is no longer already included in
* Invoice.tax_amount_micros.
*
*
* optional int64 total_amount_micros = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The totalAmountMicros.
*/
@java.lang.Override
public long getTotalAmountMicros() {
return totalAmountMicros_;
}
public static final int CORRECTED_INVOICE_FIELD_NUMBER = 36;
private volatile java.lang.Object correctedInvoice_;
/**
*
* Output only. The resource name of the original invoice corrected, wrote off, or canceled
* by this invoice, if applicable. If `corrected_invoice` is set,
* `replaced_invoices` will not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* optional string corrected_invoice = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the correctedInvoice field is set.
*/
@java.lang.Override
public boolean hasCorrectedInvoice() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Output only. The resource name of the original invoice corrected, wrote off, or canceled
* by this invoice, if applicable. If `corrected_invoice` is set,
* `replaced_invoices` will not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* optional string corrected_invoice = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The correctedInvoice.
*/
@java.lang.Override
public java.lang.String getCorrectedInvoice() {
java.lang.Object ref = correctedInvoice_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
correctedInvoice_ = s;
return s;
}
}
/**
*
* Output only. The resource name of the original invoice corrected, wrote off, or canceled
* by this invoice, if applicable. If `corrected_invoice` is set,
* `replaced_invoices` will not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* optional string corrected_invoice = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for correctedInvoice.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCorrectedInvoiceBytes() {
java.lang.Object ref = correctedInvoice_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
correctedInvoice_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REPLACED_INVOICES_FIELD_NUMBER = 37;
private com.google.protobuf.LazyStringList replacedInvoices_;
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return A list containing the replacedInvoices.
*/
public com.google.protobuf.ProtocolStringList
getReplacedInvoicesList() {
return replacedInvoices_;
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The count of replacedInvoices.
*/
public int getReplacedInvoicesCount() {
return replacedInvoices_.size();
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param index The index of the element to return.
* @return The replacedInvoices at the given index.
*/
public java.lang.String getReplacedInvoices(int index) {
return replacedInvoices_.get(index);
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param index The index of the value to return.
* @return The bytes of the replacedInvoices at the given index.
*/
public com.google.protobuf.ByteString
getReplacedInvoicesBytes(int index) {
return replacedInvoices_.getByteString(index);
}
public static final int PDF_URL_FIELD_NUMBER = 38;
private volatile java.lang.Object pdfUrl_;
/**
*
* Output only. The URL to a PDF copy of the invoice. Users need to pass in their OAuth
* token to request the PDF with this URL.
*
*
* optional string pdf_url = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the pdfUrl field is set.
*/
@java.lang.Override
public boolean hasPdfUrl() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* Output only. The URL to a PDF copy of the invoice. Users need to pass in their OAuth
* token to request the PDF with this URL.
*
*
* optional string pdf_url = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The pdfUrl.
*/
@java.lang.Override
public java.lang.String getPdfUrl() {
java.lang.Object ref = pdfUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
pdfUrl_ = s;
return s;
}
}
/**
*
* Output only. The URL to a PDF copy of the invoice. Users need to pass in their OAuth
* token to request the PDF with this URL.
*
*
* optional string pdf_url = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for pdfUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPdfUrlBytes() {
java.lang.Object ref = pdfUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pdfUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACCOUNT_BUDGET_SUMMARIES_FIELD_NUMBER = 18;
private java.util.List accountBudgetSummaries_;
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
@java.lang.Override
public java.util.List getAccountBudgetSummariesList() {
return accountBudgetSummaries_;
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
@java.lang.Override
public java.util.List extends com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummaryOrBuilder>
getAccountBudgetSummariesOrBuilderList() {
return accountBudgetSummaries_;
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
@java.lang.Override
public int getAccountBudgetSummariesCount() {
return accountBudgetSummaries_.size();
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
@java.lang.Override
public com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary getAccountBudgetSummaries(int index) {
return accountBudgetSummaries_.get(index);
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
@java.lang.Override
public com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummaryOrBuilder getAccountBudgetSummariesOrBuilder(
int index) {
return accountBudgetSummaries_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, resourceName_);
}
if (type_ != com.google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType.UNSPECIFIED.getNumber()) {
output.writeEnum(3, type_);
}
if (serviceDateRange_ != null) {
output.writeMessage(9, getServiceDateRange());
}
for (int i = 0; i < accountBudgetSummaries_.size(); i++) {
output.writeMessage(18, accountBudgetSummaries_.get(i));
}
if (adjustmentsSubtotalAmountMicros_ != 0L) {
output.writeInt64(19, adjustmentsSubtotalAmountMicros_);
}
if (adjustmentsTaxAmountMicros_ != 0L) {
output.writeInt64(20, adjustmentsTaxAmountMicros_);
}
if (adjustmentsTotalAmountMicros_ != 0L) {
output.writeInt64(21, adjustmentsTotalAmountMicros_);
}
if (regulatoryCostsSubtotalAmountMicros_ != 0L) {
output.writeInt64(22, regulatoryCostsSubtotalAmountMicros_);
}
if (regulatoryCostsTaxAmountMicros_ != 0L) {
output.writeInt64(23, regulatoryCostsTaxAmountMicros_);
}
if (regulatoryCostsTotalAmountMicros_ != 0L) {
output.writeInt64(24, regulatoryCostsTotalAmountMicros_);
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 25, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 26, billingSetup_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 27, paymentsAccountId_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 28, paymentsProfileId_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 29, issueDate_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30, dueDate_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 31, currencyCode_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeInt64(33, subtotalAmountMicros_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeInt64(34, taxAmountMicros_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeInt64(35, totalAmountMicros_);
}
if (((bitField0_ & 0x00000400) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 36, correctedInvoice_);
}
for (int i = 0; i < replacedInvoices_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 37, replacedInvoices_.getRaw(i));
}
if (((bitField0_ & 0x00000800) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 38, pdfUrl_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, resourceName_);
}
if (type_ != com.google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, type_);
}
if (serviceDateRange_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getServiceDateRange());
}
for (int i = 0; i < accountBudgetSummaries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, accountBudgetSummaries_.get(i));
}
if (adjustmentsSubtotalAmountMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(19, adjustmentsSubtotalAmountMicros_);
}
if (adjustmentsTaxAmountMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(20, adjustmentsTaxAmountMicros_);
}
if (adjustmentsTotalAmountMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(21, adjustmentsTotalAmountMicros_);
}
if (regulatoryCostsSubtotalAmountMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(22, regulatoryCostsSubtotalAmountMicros_);
}
if (regulatoryCostsTaxAmountMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(23, regulatoryCostsTaxAmountMicros_);
}
if (regulatoryCostsTotalAmountMicros_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(24, regulatoryCostsTotalAmountMicros_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(25, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(26, billingSetup_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(27, paymentsAccountId_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(28, paymentsProfileId_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(29, issueDate_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(30, dueDate_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(31, currencyCode_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(33, subtotalAmountMicros_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(34, taxAmountMicros_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(35, totalAmountMicros_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(36, correctedInvoice_);
}
{
int dataSize = 0;
for (int i = 0; i < replacedInvoices_.size(); i++) {
dataSize += computeStringSizeNoTag(replacedInvoices_.getRaw(i));
}
size += dataSize;
size += 2 * getReplacedInvoicesList().size();
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(38, pdfUrl_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v10.resources.Invoice)) {
return super.equals(obj);
}
com.google.ads.googleads.v10.resources.Invoice other = (com.google.ads.googleads.v10.resources.Invoice) obj;
if (!getResourceName()
.equals(other.getResourceName())) return false;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (!getId()
.equals(other.getId())) return false;
}
if (type_ != other.type_) return false;
if (hasBillingSetup() != other.hasBillingSetup()) return false;
if (hasBillingSetup()) {
if (!getBillingSetup()
.equals(other.getBillingSetup())) return false;
}
if (hasPaymentsAccountId() != other.hasPaymentsAccountId()) return false;
if (hasPaymentsAccountId()) {
if (!getPaymentsAccountId()
.equals(other.getPaymentsAccountId())) return false;
}
if (hasPaymentsProfileId() != other.hasPaymentsProfileId()) return false;
if (hasPaymentsProfileId()) {
if (!getPaymentsProfileId()
.equals(other.getPaymentsProfileId())) return false;
}
if (hasIssueDate() != other.hasIssueDate()) return false;
if (hasIssueDate()) {
if (!getIssueDate()
.equals(other.getIssueDate())) return false;
}
if (hasDueDate() != other.hasDueDate()) return false;
if (hasDueDate()) {
if (!getDueDate()
.equals(other.getDueDate())) return false;
}
if (hasServiceDateRange() != other.hasServiceDateRange()) return false;
if (hasServiceDateRange()) {
if (!getServiceDateRange()
.equals(other.getServiceDateRange())) return false;
}
if (hasCurrencyCode() != other.hasCurrencyCode()) return false;
if (hasCurrencyCode()) {
if (!getCurrencyCode()
.equals(other.getCurrencyCode())) return false;
}
if (getAdjustmentsSubtotalAmountMicros()
!= other.getAdjustmentsSubtotalAmountMicros()) return false;
if (getAdjustmentsTaxAmountMicros()
!= other.getAdjustmentsTaxAmountMicros()) return false;
if (getAdjustmentsTotalAmountMicros()
!= other.getAdjustmentsTotalAmountMicros()) return false;
if (getRegulatoryCostsSubtotalAmountMicros()
!= other.getRegulatoryCostsSubtotalAmountMicros()) return false;
if (getRegulatoryCostsTaxAmountMicros()
!= other.getRegulatoryCostsTaxAmountMicros()) return false;
if (getRegulatoryCostsTotalAmountMicros()
!= other.getRegulatoryCostsTotalAmountMicros()) return false;
if (hasSubtotalAmountMicros() != other.hasSubtotalAmountMicros()) return false;
if (hasSubtotalAmountMicros()) {
if (getSubtotalAmountMicros()
!= other.getSubtotalAmountMicros()) return false;
}
if (hasTaxAmountMicros() != other.hasTaxAmountMicros()) return false;
if (hasTaxAmountMicros()) {
if (getTaxAmountMicros()
!= other.getTaxAmountMicros()) return false;
}
if (hasTotalAmountMicros() != other.hasTotalAmountMicros()) return false;
if (hasTotalAmountMicros()) {
if (getTotalAmountMicros()
!= other.getTotalAmountMicros()) return false;
}
if (hasCorrectedInvoice() != other.hasCorrectedInvoice()) return false;
if (hasCorrectedInvoice()) {
if (!getCorrectedInvoice()
.equals(other.getCorrectedInvoice())) return false;
}
if (!getReplacedInvoicesList()
.equals(other.getReplacedInvoicesList())) return false;
if (hasPdfUrl() != other.hasPdfUrl()) return false;
if (hasPdfUrl()) {
if (!getPdfUrl()
.equals(other.getPdfUrl())) return false;
}
if (!getAccountBudgetSummariesList()
.equals(other.getAccountBudgetSummariesList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RESOURCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getResourceName().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
if (hasBillingSetup()) {
hash = (37 * hash) + BILLING_SETUP_FIELD_NUMBER;
hash = (53 * hash) + getBillingSetup().hashCode();
}
if (hasPaymentsAccountId()) {
hash = (37 * hash) + PAYMENTS_ACCOUNT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPaymentsAccountId().hashCode();
}
if (hasPaymentsProfileId()) {
hash = (37 * hash) + PAYMENTS_PROFILE_ID_FIELD_NUMBER;
hash = (53 * hash) + getPaymentsProfileId().hashCode();
}
if (hasIssueDate()) {
hash = (37 * hash) + ISSUE_DATE_FIELD_NUMBER;
hash = (53 * hash) + getIssueDate().hashCode();
}
if (hasDueDate()) {
hash = (37 * hash) + DUE_DATE_FIELD_NUMBER;
hash = (53 * hash) + getDueDate().hashCode();
}
if (hasServiceDateRange()) {
hash = (37 * hash) + SERVICE_DATE_RANGE_FIELD_NUMBER;
hash = (53 * hash) + getServiceDateRange().hashCode();
}
if (hasCurrencyCode()) {
hash = (37 * hash) + CURRENCY_CODE_FIELD_NUMBER;
hash = (53 * hash) + getCurrencyCode().hashCode();
}
hash = (37 * hash) + ADJUSTMENTS_SUBTOTAL_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAdjustmentsSubtotalAmountMicros());
hash = (37 * hash) + ADJUSTMENTS_TAX_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAdjustmentsTaxAmountMicros());
hash = (37 * hash) + ADJUSTMENTS_TOTAL_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAdjustmentsTotalAmountMicros());
hash = (37 * hash) + REGULATORY_COSTS_SUBTOTAL_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRegulatoryCostsSubtotalAmountMicros());
hash = (37 * hash) + REGULATORY_COSTS_TAX_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRegulatoryCostsTaxAmountMicros());
hash = (37 * hash) + REGULATORY_COSTS_TOTAL_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRegulatoryCostsTotalAmountMicros());
if (hasSubtotalAmountMicros()) {
hash = (37 * hash) + SUBTOTAL_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSubtotalAmountMicros());
}
if (hasTaxAmountMicros()) {
hash = (37 * hash) + TAX_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTaxAmountMicros());
}
if (hasTotalAmountMicros()) {
hash = (37 * hash) + TOTAL_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTotalAmountMicros());
}
if (hasCorrectedInvoice()) {
hash = (37 * hash) + CORRECTED_INVOICE_FIELD_NUMBER;
hash = (53 * hash) + getCorrectedInvoice().hashCode();
}
if (getReplacedInvoicesCount() > 0) {
hash = (37 * hash) + REPLACED_INVOICES_FIELD_NUMBER;
hash = (53 * hash) + getReplacedInvoicesList().hashCode();
}
if (hasPdfUrl()) {
hash = (37 * hash) + PDF_URL_FIELD_NUMBER;
hash = (53 * hash) + getPdfUrl().hashCode();
}
if (getAccountBudgetSummariesCount() > 0) {
hash = (37 * hash) + ACCOUNT_BUDGET_SUMMARIES_FIELD_NUMBER;
hash = (53 * hash) + getAccountBudgetSummariesList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v10.resources.Invoice parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.Invoice parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.Invoice parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.Invoice parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.Invoice parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.Invoice parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.Invoice parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.Invoice parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.Invoice parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.Invoice parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.Invoice parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.Invoice parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v10.resources.Invoice prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An invoice. All invoice information is snapshotted to match the PDF invoice.
* For invoices older than the launch of InvoiceService, the snapshotted
* information may not match the PDF invoice.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.Invoice}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v10.resources.Invoice)
com.google.ads.googleads.v10.resources.InvoiceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.InvoiceProto.internal_static_google_ads_googleads_v10_resources_Invoice_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.InvoiceProto.internal_static_google_ads_googleads_v10_resources_Invoice_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.Invoice.class, com.google.ads.googleads.v10.resources.Invoice.Builder.class);
}
// Construct using com.google.ads.googleads.v10.resources.Invoice.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
resourceName_ = "";
id_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 0;
billingSetup_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
paymentsAccountId_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
paymentsProfileId_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
issueDate_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
dueDate_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
if (serviceDateRangeBuilder_ == null) {
serviceDateRange_ = null;
} else {
serviceDateRange_ = null;
serviceDateRangeBuilder_ = null;
}
currencyCode_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
adjustmentsSubtotalAmountMicros_ = 0L;
adjustmentsTaxAmountMicros_ = 0L;
adjustmentsTotalAmountMicros_ = 0L;
regulatoryCostsSubtotalAmountMicros_ = 0L;
regulatoryCostsTaxAmountMicros_ = 0L;
regulatoryCostsTotalAmountMicros_ = 0L;
subtotalAmountMicros_ = 0L;
bitField0_ = (bitField0_ & ~0x00000080);
taxAmountMicros_ = 0L;
bitField0_ = (bitField0_ & ~0x00000100);
totalAmountMicros_ = 0L;
bitField0_ = (bitField0_ & ~0x00000200);
correctedInvoice_ = "";
bitField0_ = (bitField0_ & ~0x00000400);
replacedInvoices_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000800);
pdfUrl_ = "";
bitField0_ = (bitField0_ & ~0x00001000);
if (accountBudgetSummariesBuilder_ == null) {
accountBudgetSummaries_ = java.util.Collections.emptyList();
} else {
accountBudgetSummaries_ = null;
accountBudgetSummariesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00002000);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v10.resources.InvoiceProto.internal_static_google_ads_googleads_v10_resources_Invoice_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.Invoice getDefaultInstanceForType() {
return com.google.ads.googleads.v10.resources.Invoice.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.Invoice build() {
com.google.ads.googleads.v10.resources.Invoice result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.Invoice buildPartial() {
com.google.ads.googleads.v10.resources.Invoice result = new com.google.ads.googleads.v10.resources.Invoice(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.resourceName_ = resourceName_;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
result.type_ = type_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.billingSetup_ = billingSetup_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.paymentsAccountId_ = paymentsAccountId_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.paymentsProfileId_ = paymentsProfileId_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.issueDate_ = issueDate_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.dueDate_ = dueDate_;
if (serviceDateRangeBuilder_ == null) {
result.serviceDateRange_ = serviceDateRange_;
} else {
result.serviceDateRange_ = serviceDateRangeBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000040;
}
result.currencyCode_ = currencyCode_;
result.adjustmentsSubtotalAmountMicros_ = adjustmentsSubtotalAmountMicros_;
result.adjustmentsTaxAmountMicros_ = adjustmentsTaxAmountMicros_;
result.adjustmentsTotalAmountMicros_ = adjustmentsTotalAmountMicros_;
result.regulatoryCostsSubtotalAmountMicros_ = regulatoryCostsSubtotalAmountMicros_;
result.regulatoryCostsTaxAmountMicros_ = regulatoryCostsTaxAmountMicros_;
result.regulatoryCostsTotalAmountMicros_ = regulatoryCostsTotalAmountMicros_;
if (((from_bitField0_ & 0x00000080) != 0)) {
result.subtotalAmountMicros_ = subtotalAmountMicros_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.taxAmountMicros_ = taxAmountMicros_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.totalAmountMicros_ = totalAmountMicros_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
to_bitField0_ |= 0x00000400;
}
result.correctedInvoice_ = correctedInvoice_;
if (((bitField0_ & 0x00000800) != 0)) {
replacedInvoices_ = replacedInvoices_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000800);
}
result.replacedInvoices_ = replacedInvoices_;
if (((from_bitField0_ & 0x00001000) != 0)) {
to_bitField0_ |= 0x00000800;
}
result.pdfUrl_ = pdfUrl_;
if (accountBudgetSummariesBuilder_ == null) {
if (((bitField0_ & 0x00002000) != 0)) {
accountBudgetSummaries_ = java.util.Collections.unmodifiableList(accountBudgetSummaries_);
bitField0_ = (bitField0_ & ~0x00002000);
}
result.accountBudgetSummaries_ = accountBudgetSummaries_;
} else {
result.accountBudgetSummaries_ = accountBudgetSummariesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v10.resources.Invoice) {
return mergeFrom((com.google.ads.googleads.v10.resources.Invoice)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v10.resources.Invoice other) {
if (other == com.google.ads.googleads.v10.resources.Invoice.getDefaultInstance()) return this;
if (!other.getResourceName().isEmpty()) {
resourceName_ = other.resourceName_;
onChanged();
}
if (other.hasId()) {
bitField0_ |= 0x00000001;
id_ = other.id_;
onChanged();
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.hasBillingSetup()) {
bitField0_ |= 0x00000002;
billingSetup_ = other.billingSetup_;
onChanged();
}
if (other.hasPaymentsAccountId()) {
bitField0_ |= 0x00000004;
paymentsAccountId_ = other.paymentsAccountId_;
onChanged();
}
if (other.hasPaymentsProfileId()) {
bitField0_ |= 0x00000008;
paymentsProfileId_ = other.paymentsProfileId_;
onChanged();
}
if (other.hasIssueDate()) {
bitField0_ |= 0x00000010;
issueDate_ = other.issueDate_;
onChanged();
}
if (other.hasDueDate()) {
bitField0_ |= 0x00000020;
dueDate_ = other.dueDate_;
onChanged();
}
if (other.hasServiceDateRange()) {
mergeServiceDateRange(other.getServiceDateRange());
}
if (other.hasCurrencyCode()) {
bitField0_ |= 0x00000040;
currencyCode_ = other.currencyCode_;
onChanged();
}
if (other.getAdjustmentsSubtotalAmountMicros() != 0L) {
setAdjustmentsSubtotalAmountMicros(other.getAdjustmentsSubtotalAmountMicros());
}
if (other.getAdjustmentsTaxAmountMicros() != 0L) {
setAdjustmentsTaxAmountMicros(other.getAdjustmentsTaxAmountMicros());
}
if (other.getAdjustmentsTotalAmountMicros() != 0L) {
setAdjustmentsTotalAmountMicros(other.getAdjustmentsTotalAmountMicros());
}
if (other.getRegulatoryCostsSubtotalAmountMicros() != 0L) {
setRegulatoryCostsSubtotalAmountMicros(other.getRegulatoryCostsSubtotalAmountMicros());
}
if (other.getRegulatoryCostsTaxAmountMicros() != 0L) {
setRegulatoryCostsTaxAmountMicros(other.getRegulatoryCostsTaxAmountMicros());
}
if (other.getRegulatoryCostsTotalAmountMicros() != 0L) {
setRegulatoryCostsTotalAmountMicros(other.getRegulatoryCostsTotalAmountMicros());
}
if (other.hasSubtotalAmountMicros()) {
setSubtotalAmountMicros(other.getSubtotalAmountMicros());
}
if (other.hasTaxAmountMicros()) {
setTaxAmountMicros(other.getTaxAmountMicros());
}
if (other.hasTotalAmountMicros()) {
setTotalAmountMicros(other.getTotalAmountMicros());
}
if (other.hasCorrectedInvoice()) {
bitField0_ |= 0x00000400;
correctedInvoice_ = other.correctedInvoice_;
onChanged();
}
if (!other.replacedInvoices_.isEmpty()) {
if (replacedInvoices_.isEmpty()) {
replacedInvoices_ = other.replacedInvoices_;
bitField0_ = (bitField0_ & ~0x00000800);
} else {
ensureReplacedInvoicesIsMutable();
replacedInvoices_.addAll(other.replacedInvoices_);
}
onChanged();
}
if (other.hasPdfUrl()) {
bitField0_ |= 0x00001000;
pdfUrl_ = other.pdfUrl_;
onChanged();
}
if (accountBudgetSummariesBuilder_ == null) {
if (!other.accountBudgetSummaries_.isEmpty()) {
if (accountBudgetSummaries_.isEmpty()) {
accountBudgetSummaries_ = other.accountBudgetSummaries_;
bitField0_ = (bitField0_ & ~0x00002000);
} else {
ensureAccountBudgetSummariesIsMutable();
accountBudgetSummaries_.addAll(other.accountBudgetSummaries_);
}
onChanged();
}
} else {
if (!other.accountBudgetSummaries_.isEmpty()) {
if (accountBudgetSummariesBuilder_.isEmpty()) {
accountBudgetSummariesBuilder_.dispose();
accountBudgetSummariesBuilder_ = null;
accountBudgetSummaries_ = other.accountBudgetSummaries_;
bitField0_ = (bitField0_ & ~0x00002000);
accountBudgetSummariesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAccountBudgetSummariesFieldBuilder() : null;
} else {
accountBudgetSummariesBuilder_.addAllMessages(other.accountBudgetSummaries_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
resourceName_ = input.readStringRequireUtf8();
break;
} // case 10
case 24: {
type_ = input.readEnum();
break;
} // case 24
case 74: {
input.readMessage(
getServiceDateRangeFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 74
case 146: {
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary m =
input.readMessage(
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.parser(),
extensionRegistry);
if (accountBudgetSummariesBuilder_ == null) {
ensureAccountBudgetSummariesIsMutable();
accountBudgetSummaries_.add(m);
} else {
accountBudgetSummariesBuilder_.addMessage(m);
}
break;
} // case 146
case 152: {
adjustmentsSubtotalAmountMicros_ = input.readInt64();
break;
} // case 152
case 160: {
adjustmentsTaxAmountMicros_ = input.readInt64();
break;
} // case 160
case 168: {
adjustmentsTotalAmountMicros_ = input.readInt64();
break;
} // case 168
case 176: {
regulatoryCostsSubtotalAmountMicros_ = input.readInt64();
break;
} // case 176
case 184: {
regulatoryCostsTaxAmountMicros_ = input.readInt64();
break;
} // case 184
case 192: {
regulatoryCostsTotalAmountMicros_ = input.readInt64();
break;
} // case 192
case 202: {
id_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 202
case 210: {
billingSetup_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 210
case 218: {
paymentsAccountId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 218
case 226: {
paymentsProfileId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 226
case 234: {
issueDate_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 234
case 242: {
dueDate_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 242
case 250: {
currencyCode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 250
case 264: {
subtotalAmountMicros_ = input.readInt64();
bitField0_ |= 0x00000080;
break;
} // case 264
case 272: {
taxAmountMicros_ = input.readInt64();
bitField0_ |= 0x00000100;
break;
} // case 272
case 280: {
totalAmountMicros_ = input.readInt64();
bitField0_ |= 0x00000200;
break;
} // case 280
case 290: {
correctedInvoice_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000400;
break;
} // case 290
case 298: {
java.lang.String s = input.readStringRequireUtf8();
ensureReplacedInvoicesIsMutable();
replacedInvoices_.add(s);
break;
} // case 298
case 306: {
pdfUrl_ = input.readStringRequireUtf8();
bitField0_ |= 0x00001000;
break;
} // case 306
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object resourceName_ = "";
/**
*
* Output only. The resource name of the invoice. Multiple customers can share a given
* invoice, so multiple resource names may point to the same invoice.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The resource name of the invoice. Multiple customers can share a given
* invoice, so multiple resource names may point to the same invoice.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The resource name of the invoice. Multiple customers can share a given
* invoice, so multiple resource names may point to the same invoice.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resourceName_ = value;
onChanged();
return this;
}
/**
*
* Output only. The resource name of the invoice. Multiple customers can share a given
* invoice, so multiple resource names may point to the same invoice.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearResourceName() {
resourceName_ = getDefaultInstance().getResourceName();
onChanged();
return this;
}
/**
*
* Output only. The resource name of the invoice. Multiple customers can share a given
* invoice, so multiple resource names may point to the same invoice.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY, (.google.api.resource_reference) = { ... }
* @param value The bytes for resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resourceName_ = value;
onChanged();
return this;
}
private java.lang.Object id_ = "";
/**
*
* Output only. The ID of the invoice. It appears on the invoice PDF as "Invoice number".
*
*
* optional string id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The ID of the invoice. It appears on the invoice PDF as "Invoice number".
*
*
* optional string id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The ID of the invoice. It appears on the invoice PDF as "Invoice number".
*
*
* optional string id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The ID of the invoice. It appears on the invoice PDF as "Invoice number".
*
*
* optional string id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
*
* Output only. The ID of the invoice. It appears on the invoice PDF as "Invoice number".
*
*
* optional string id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* Output only. The ID of the invoice. It appears on the invoice PDF as "Invoice number".
*
*
* optional string id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
private int type_ = 0;
/**
*
* Output only. The type of invoice.
*
*
* .google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType type = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
*
* Output only. The type of invoice.
*
*
* .google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType type = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
* Output only. The type of invoice.
*
*
* .google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType type = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The type.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType getType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType result = com.google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType.valueOf(type_);
return result == null ? com.google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType.UNRECOGNIZED : result;
}
/**
*
* Output only. The type of invoice.
*
*
* .google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType type = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(com.google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The type of invoice.
*
*
* .google.ads.googleads.v10.enums.InvoiceTypeEnum.InvoiceType type = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private java.lang.Object billingSetup_ = "";
/**
*
* Output only. The resource name of this invoice's billing setup.
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the billingSetup field is set.
*/
public boolean hasBillingSetup() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The resource name of this invoice's billing setup.
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The billingSetup.
*/
public java.lang.String getBillingSetup() {
java.lang.Object ref = billingSetup_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
billingSetup_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The resource name of this invoice's billing setup.
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for billingSetup.
*/
public com.google.protobuf.ByteString
getBillingSetupBytes() {
java.lang.Object ref = billingSetup_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
billingSetup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The resource name of this invoice's billing setup.
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The billingSetup to set.
* @return This builder for chaining.
*/
public Builder setBillingSetup(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
billingSetup_ = value;
onChanged();
return this;
}
/**
*
* Output only. The resource name of this invoice's billing setup.
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearBillingSetup() {
bitField0_ = (bitField0_ & ~0x00000002);
billingSetup_ = getDefaultInstance().getBillingSetup();
onChanged();
return this;
}
/**
*
* Output only. The resource name of this invoice's billing setup.
* `customers/{customer_id}/billingSetups/{billing_setup_id}`
*
*
* optional string billing_setup = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for billingSetup to set.
* @return This builder for chaining.
*/
public Builder setBillingSetupBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000002;
billingSetup_ = value;
onChanged();
return this;
}
private java.lang.Object paymentsAccountId_ = "";
/**
*
* Output only. A 16 digit ID used to identify the payments account associated with the
* billing setup, for example, "1234-5678-9012-3456". It appears on the
* invoice PDF as "Billing Account Number".
*
*
* optional string payments_account_id = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the paymentsAccountId field is set.
*/
public boolean hasPaymentsAccountId() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Output only. A 16 digit ID used to identify the payments account associated with the
* billing setup, for example, "1234-5678-9012-3456". It appears on the
* invoice PDF as "Billing Account Number".
*
*
* optional string payments_account_id = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The paymentsAccountId.
*/
public java.lang.String getPaymentsAccountId() {
java.lang.Object ref = paymentsAccountId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
paymentsAccountId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. A 16 digit ID used to identify the payments account associated with the
* billing setup, for example, "1234-5678-9012-3456". It appears on the
* invoice PDF as "Billing Account Number".
*
*
* optional string payments_account_id = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for paymentsAccountId.
*/
public com.google.protobuf.ByteString
getPaymentsAccountIdBytes() {
java.lang.Object ref = paymentsAccountId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
paymentsAccountId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. A 16 digit ID used to identify the payments account associated with the
* billing setup, for example, "1234-5678-9012-3456". It appears on the
* invoice PDF as "Billing Account Number".
*
*
* optional string payments_account_id = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The paymentsAccountId to set.
* @return This builder for chaining.
*/
public Builder setPaymentsAccountId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
paymentsAccountId_ = value;
onChanged();
return this;
}
/**
*
* Output only. A 16 digit ID used to identify the payments account associated with the
* billing setup, for example, "1234-5678-9012-3456". It appears on the
* invoice PDF as "Billing Account Number".
*
*
* optional string payments_account_id = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearPaymentsAccountId() {
bitField0_ = (bitField0_ & ~0x00000004);
paymentsAccountId_ = getDefaultInstance().getPaymentsAccountId();
onChanged();
return this;
}
/**
*
* Output only. A 16 digit ID used to identify the payments account associated with the
* billing setup, for example, "1234-5678-9012-3456". It appears on the
* invoice PDF as "Billing Account Number".
*
*
* optional string payments_account_id = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for paymentsAccountId to set.
* @return This builder for chaining.
*/
public Builder setPaymentsAccountIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000004;
paymentsAccountId_ = value;
onChanged();
return this;
}
private java.lang.Object paymentsProfileId_ = "";
/**
*
* Output only. A 12 digit ID used to identify the payments profile associated with the
* billing setup, for example, "1234-5678-9012". It appears on the invoice PDF
* as "Billing ID".
*
*
* optional string payments_profile_id = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the paymentsProfileId field is set.
*/
public boolean hasPaymentsProfileId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Output only. A 12 digit ID used to identify the payments profile associated with the
* billing setup, for example, "1234-5678-9012". It appears on the invoice PDF
* as "Billing ID".
*
*
* optional string payments_profile_id = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The paymentsProfileId.
*/
public java.lang.String getPaymentsProfileId() {
java.lang.Object ref = paymentsProfileId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
paymentsProfileId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. A 12 digit ID used to identify the payments profile associated with the
* billing setup, for example, "1234-5678-9012". It appears on the invoice PDF
* as "Billing ID".
*
*
* optional string payments_profile_id = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for paymentsProfileId.
*/
public com.google.protobuf.ByteString
getPaymentsProfileIdBytes() {
java.lang.Object ref = paymentsProfileId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
paymentsProfileId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. A 12 digit ID used to identify the payments profile associated with the
* billing setup, for example, "1234-5678-9012". It appears on the invoice PDF
* as "Billing ID".
*
*
* optional string payments_profile_id = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The paymentsProfileId to set.
* @return This builder for chaining.
*/
public Builder setPaymentsProfileId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
paymentsProfileId_ = value;
onChanged();
return this;
}
/**
*
* Output only. A 12 digit ID used to identify the payments profile associated with the
* billing setup, for example, "1234-5678-9012". It appears on the invoice PDF
* as "Billing ID".
*
*
* optional string payments_profile_id = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearPaymentsProfileId() {
bitField0_ = (bitField0_ & ~0x00000008);
paymentsProfileId_ = getDefaultInstance().getPaymentsProfileId();
onChanged();
return this;
}
/**
*
* Output only. A 12 digit ID used to identify the payments profile associated with the
* billing setup, for example, "1234-5678-9012". It appears on the invoice PDF
* as "Billing ID".
*
*
* optional string payments_profile_id = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for paymentsProfileId to set.
* @return This builder for chaining.
*/
public Builder setPaymentsProfileIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000008;
paymentsProfileId_ = value;
onChanged();
return this;
}
private java.lang.Object issueDate_ = "";
/**
*
* Output only. The issue date in yyyy-mm-dd format. It appears on the invoice PDF as
* either "Issue date" or "Invoice date".
*
*
* optional string issue_date = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the issueDate field is set.
*/
public boolean hasIssueDate() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Output only. The issue date in yyyy-mm-dd format. It appears on the invoice PDF as
* either "Issue date" or "Invoice date".
*
*
* optional string issue_date = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The issueDate.
*/
public java.lang.String getIssueDate() {
java.lang.Object ref = issueDate_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
issueDate_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The issue date in yyyy-mm-dd format. It appears on the invoice PDF as
* either "Issue date" or "Invoice date".
*
*
* optional string issue_date = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for issueDate.
*/
public com.google.protobuf.ByteString
getIssueDateBytes() {
java.lang.Object ref = issueDate_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
issueDate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The issue date in yyyy-mm-dd format. It appears on the invoice PDF as
* either "Issue date" or "Invoice date".
*
*
* optional string issue_date = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The issueDate to set.
* @return This builder for chaining.
*/
public Builder setIssueDate(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
issueDate_ = value;
onChanged();
return this;
}
/**
*
* Output only. The issue date in yyyy-mm-dd format. It appears on the invoice PDF as
* either "Issue date" or "Invoice date".
*
*
* optional string issue_date = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearIssueDate() {
bitField0_ = (bitField0_ & ~0x00000010);
issueDate_ = getDefaultInstance().getIssueDate();
onChanged();
return this;
}
/**
*
* Output only. The issue date in yyyy-mm-dd format. It appears on the invoice PDF as
* either "Issue date" or "Invoice date".
*
*
* optional string issue_date = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for issueDate to set.
* @return This builder for chaining.
*/
public Builder setIssueDateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000010;
issueDate_ = value;
onChanged();
return this;
}
private java.lang.Object dueDate_ = "";
/**
*
* Output only. The due date in yyyy-mm-dd format.
*
*
* optional string due_date = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the dueDate field is set.
*/
public boolean hasDueDate() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. The due date in yyyy-mm-dd format.
*
*
* optional string due_date = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The dueDate.
*/
public java.lang.String getDueDate() {
java.lang.Object ref = dueDate_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
dueDate_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The due date in yyyy-mm-dd format.
*
*
* optional string due_date = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for dueDate.
*/
public com.google.protobuf.ByteString
getDueDateBytes() {
java.lang.Object ref = dueDate_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dueDate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The due date in yyyy-mm-dd format.
*
*
* optional string due_date = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The dueDate to set.
* @return This builder for chaining.
*/
public Builder setDueDate(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
dueDate_ = value;
onChanged();
return this;
}
/**
*
* Output only. The due date in yyyy-mm-dd format.
*
*
* optional string due_date = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearDueDate() {
bitField0_ = (bitField0_ & ~0x00000020);
dueDate_ = getDefaultInstance().getDueDate();
onChanged();
return this;
}
/**
*
* Output only. The due date in yyyy-mm-dd format.
*
*
* optional string due_date = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for dueDate to set.
* @return This builder for chaining.
*/
public Builder setDueDateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000020;
dueDate_ = value;
onChanged();
return this;
}
private com.google.ads.googleads.v10.common.DateRange serviceDateRange_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.DateRange, com.google.ads.googleads.v10.common.DateRange.Builder, com.google.ads.googleads.v10.common.DateRangeOrBuilder> serviceDateRangeBuilder_;
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the serviceDateRange field is set.
*/
public boolean hasServiceDateRange() {
return serviceDateRangeBuilder_ != null || serviceDateRange_ != null;
}
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The serviceDateRange.
*/
public com.google.ads.googleads.v10.common.DateRange getServiceDateRange() {
if (serviceDateRangeBuilder_ == null) {
return serviceDateRange_ == null ? com.google.ads.googleads.v10.common.DateRange.getDefaultInstance() : serviceDateRange_;
} else {
return serviceDateRangeBuilder_.getMessage();
}
}
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder setServiceDateRange(com.google.ads.googleads.v10.common.DateRange value) {
if (serviceDateRangeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
serviceDateRange_ = value;
onChanged();
} else {
serviceDateRangeBuilder_.setMessage(value);
}
return this;
}
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder setServiceDateRange(
com.google.ads.googleads.v10.common.DateRange.Builder builderForValue) {
if (serviceDateRangeBuilder_ == null) {
serviceDateRange_ = builderForValue.build();
onChanged();
} else {
serviceDateRangeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder mergeServiceDateRange(com.google.ads.googleads.v10.common.DateRange value) {
if (serviceDateRangeBuilder_ == null) {
if (serviceDateRange_ != null) {
serviceDateRange_ =
com.google.ads.googleads.v10.common.DateRange.newBuilder(serviceDateRange_).mergeFrom(value).buildPartial();
} else {
serviceDateRange_ = value;
}
onChanged();
} else {
serviceDateRangeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder clearServiceDateRange() {
if (serviceDateRangeBuilder_ == null) {
serviceDateRange_ = null;
onChanged();
} else {
serviceDateRange_ = null;
serviceDateRangeBuilder_ = null;
}
return this;
}
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.common.DateRange.Builder getServiceDateRangeBuilder() {
onChanged();
return getServiceDateRangeFieldBuilder().getBuilder();
}
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.common.DateRangeOrBuilder getServiceDateRangeOrBuilder() {
if (serviceDateRangeBuilder_ != null) {
return serviceDateRangeBuilder_.getMessageOrBuilder();
} else {
return serviceDateRange_ == null ?
com.google.ads.googleads.v10.common.DateRange.getDefaultInstance() : serviceDateRange_;
}
}
/**
*
* Output only. The service period date range of this invoice. The end date is inclusive.
*
*
* .google.ads.googleads.v10.common.DateRange service_date_range = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.DateRange, com.google.ads.googleads.v10.common.DateRange.Builder, com.google.ads.googleads.v10.common.DateRangeOrBuilder>
getServiceDateRangeFieldBuilder() {
if (serviceDateRangeBuilder_ == null) {
serviceDateRangeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.DateRange, com.google.ads.googleads.v10.common.DateRange.Builder, com.google.ads.googleads.v10.common.DateRangeOrBuilder>(
getServiceDateRange(),
getParentForChildren(),
isClean());
serviceDateRange_ = null;
}
return serviceDateRangeBuilder_;
}
private java.lang.Object currencyCode_ = "";
/**
*
* Output only. The currency code. All costs are returned in this currency. A subset of the
* currency codes derived from the ISO 4217 standard is supported.
*
*
* optional string currency_code = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the currencyCode field is set.
*/
public boolean hasCurrencyCode() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. The currency code. All costs are returned in this currency. A subset of the
* currency codes derived from the ISO 4217 standard is supported.
*
*
* optional string currency_code = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The currencyCode.
*/
public java.lang.String getCurrencyCode() {
java.lang.Object ref = currencyCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currencyCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The currency code. All costs are returned in this currency. A subset of the
* currency codes derived from the ISO 4217 standard is supported.
*
*
* optional string currency_code = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for currencyCode.
*/
public com.google.protobuf.ByteString
getCurrencyCodeBytes() {
java.lang.Object ref = currencyCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The currency code. All costs are returned in this currency. A subset of the
* currency codes derived from the ISO 4217 standard is supported.
*
*
* optional string currency_code = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The currencyCode to set.
* @return This builder for chaining.
*/
public Builder setCurrencyCode(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
currencyCode_ = value;
onChanged();
return this;
}
/**
*
* Output only. The currency code. All costs are returned in this currency. A subset of the
* currency codes derived from the ISO 4217 standard is supported.
*
*
* optional string currency_code = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearCurrencyCode() {
bitField0_ = (bitField0_ & ~0x00000040);
currencyCode_ = getDefaultInstance().getCurrencyCode();
onChanged();
return this;
}
/**
*
* Output only. The currency code. All costs are returned in this currency. A subset of the
* currency codes derived from the ISO 4217 standard is supported.
*
*
* optional string currency_code = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for currencyCode to set.
* @return This builder for chaining.
*/
public Builder setCurrencyCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000040;
currencyCode_ = value;
onChanged();
return this;
}
private long adjustmentsSubtotalAmountMicros_ ;
/**
*
* Output only. The pretax subtotal amount of invoice level adjustments, in micros.
*
*
* int64 adjustments_subtotal_amount_micros = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The adjustmentsSubtotalAmountMicros.
*/
@java.lang.Override
public long getAdjustmentsSubtotalAmountMicros() {
return adjustmentsSubtotalAmountMicros_;
}
/**
*
* Output only. The pretax subtotal amount of invoice level adjustments, in micros.
*
*
* int64 adjustments_subtotal_amount_micros = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The adjustmentsSubtotalAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setAdjustmentsSubtotalAmountMicros(long value) {
adjustmentsSubtotalAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The pretax subtotal amount of invoice level adjustments, in micros.
*
*
* int64 adjustments_subtotal_amount_micros = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearAdjustmentsSubtotalAmountMicros() {
adjustmentsSubtotalAmountMicros_ = 0L;
onChanged();
return this;
}
private long adjustmentsTaxAmountMicros_ ;
/**
*
* Output only. The sum of taxes on the invoice level adjustments, in micros.
*
*
* int64 adjustments_tax_amount_micros = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The adjustmentsTaxAmountMicros.
*/
@java.lang.Override
public long getAdjustmentsTaxAmountMicros() {
return adjustmentsTaxAmountMicros_;
}
/**
*
* Output only. The sum of taxes on the invoice level adjustments, in micros.
*
*
* int64 adjustments_tax_amount_micros = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The adjustmentsTaxAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setAdjustmentsTaxAmountMicros(long value) {
adjustmentsTaxAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The sum of taxes on the invoice level adjustments, in micros.
*
*
* int64 adjustments_tax_amount_micros = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearAdjustmentsTaxAmountMicros() {
adjustmentsTaxAmountMicros_ = 0L;
onChanged();
return this;
}
private long adjustmentsTotalAmountMicros_ ;
/**
*
* Output only. The total amount of invoice level adjustments, in micros.
*
*
* int64 adjustments_total_amount_micros = 21 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The adjustmentsTotalAmountMicros.
*/
@java.lang.Override
public long getAdjustmentsTotalAmountMicros() {
return adjustmentsTotalAmountMicros_;
}
/**
*
* Output only. The total amount of invoice level adjustments, in micros.
*
*
* int64 adjustments_total_amount_micros = 21 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The adjustmentsTotalAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setAdjustmentsTotalAmountMicros(long value) {
adjustmentsTotalAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The total amount of invoice level adjustments, in micros.
*
*
* int64 adjustments_total_amount_micros = 21 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearAdjustmentsTotalAmountMicros() {
adjustmentsTotalAmountMicros_ = 0L;
onChanged();
return this;
}
private long regulatoryCostsSubtotalAmountMicros_ ;
/**
*
* Output only. The pretax subtotal amount of invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_subtotal_amount_micros = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The regulatoryCostsSubtotalAmountMicros.
*/
@java.lang.Override
public long getRegulatoryCostsSubtotalAmountMicros() {
return regulatoryCostsSubtotalAmountMicros_;
}
/**
*
* Output only. The pretax subtotal amount of invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_subtotal_amount_micros = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The regulatoryCostsSubtotalAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setRegulatoryCostsSubtotalAmountMicros(long value) {
regulatoryCostsSubtotalAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The pretax subtotal amount of invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_subtotal_amount_micros = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearRegulatoryCostsSubtotalAmountMicros() {
regulatoryCostsSubtotalAmountMicros_ = 0L;
onChanged();
return this;
}
private long regulatoryCostsTaxAmountMicros_ ;
/**
*
* Output only. The sum of taxes on the invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_tax_amount_micros = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The regulatoryCostsTaxAmountMicros.
*/
@java.lang.Override
public long getRegulatoryCostsTaxAmountMicros() {
return regulatoryCostsTaxAmountMicros_;
}
/**
*
* Output only. The sum of taxes on the invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_tax_amount_micros = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The regulatoryCostsTaxAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setRegulatoryCostsTaxAmountMicros(long value) {
regulatoryCostsTaxAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The sum of taxes on the invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_tax_amount_micros = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearRegulatoryCostsTaxAmountMicros() {
regulatoryCostsTaxAmountMicros_ = 0L;
onChanged();
return this;
}
private long regulatoryCostsTotalAmountMicros_ ;
/**
*
* Output only. The total amount of invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_total_amount_micros = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The regulatoryCostsTotalAmountMicros.
*/
@java.lang.Override
public long getRegulatoryCostsTotalAmountMicros() {
return regulatoryCostsTotalAmountMicros_;
}
/**
*
* Output only. The total amount of invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_total_amount_micros = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The regulatoryCostsTotalAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setRegulatoryCostsTotalAmountMicros(long value) {
regulatoryCostsTotalAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The total amount of invoice level regulatory costs, in micros.
*
*
* int64 regulatory_costs_total_amount_micros = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearRegulatoryCostsTotalAmountMicros() {
regulatoryCostsTotalAmountMicros_ = 0L;
onChanged();
return this;
}
private long subtotalAmountMicros_ ;
/**
*
* Output only. The pretax subtotal amount, in micros. This equals the
* sum of the AccountBudgetSummary subtotal amounts,
* Invoice.adjustments_subtotal_amount_micros, and
* Invoice.regulatory_costs_subtotal_amount_micros.
* Starting with v6, the Invoice.regulatory_costs_subtotal_amount_micros is no
* longer included.
*
*
* optional int64 subtotal_amount_micros = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the subtotalAmountMicros field is set.
*/
@java.lang.Override
public boolean hasSubtotalAmountMicros() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Output only. The pretax subtotal amount, in micros. This equals the
* sum of the AccountBudgetSummary subtotal amounts,
* Invoice.adjustments_subtotal_amount_micros, and
* Invoice.regulatory_costs_subtotal_amount_micros.
* Starting with v6, the Invoice.regulatory_costs_subtotal_amount_micros is no
* longer included.
*
*
* optional int64 subtotal_amount_micros = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The subtotalAmountMicros.
*/
@java.lang.Override
public long getSubtotalAmountMicros() {
return subtotalAmountMicros_;
}
/**
*
* Output only. The pretax subtotal amount, in micros. This equals the
* sum of the AccountBudgetSummary subtotal amounts,
* Invoice.adjustments_subtotal_amount_micros, and
* Invoice.regulatory_costs_subtotal_amount_micros.
* Starting with v6, the Invoice.regulatory_costs_subtotal_amount_micros is no
* longer included.
*
*
* optional int64 subtotal_amount_micros = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The subtotalAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setSubtotalAmountMicros(long value) {
bitField0_ |= 0x00000080;
subtotalAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The pretax subtotal amount, in micros. This equals the
* sum of the AccountBudgetSummary subtotal amounts,
* Invoice.adjustments_subtotal_amount_micros, and
* Invoice.regulatory_costs_subtotal_amount_micros.
* Starting with v6, the Invoice.regulatory_costs_subtotal_amount_micros is no
* longer included.
*
*
* optional int64 subtotal_amount_micros = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearSubtotalAmountMicros() {
bitField0_ = (bitField0_ & ~0x00000080);
subtotalAmountMicros_ = 0L;
onChanged();
return this;
}
private long taxAmountMicros_ ;
/**
*
* Output only. The sum of all taxes on the invoice, in micros. This equals the sum of the
* AccountBudgetSummary tax amounts, plus taxes not associated with a specific
* account budget.
*
*
* optional int64 tax_amount_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the taxAmountMicros field is set.
*/
@java.lang.Override
public boolean hasTaxAmountMicros() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Output only. The sum of all taxes on the invoice, in micros. This equals the sum of the
* AccountBudgetSummary tax amounts, plus taxes not associated with a specific
* account budget.
*
*
* optional int64 tax_amount_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The taxAmountMicros.
*/
@java.lang.Override
public long getTaxAmountMicros() {
return taxAmountMicros_;
}
/**
*
* Output only. The sum of all taxes on the invoice, in micros. This equals the sum of the
* AccountBudgetSummary tax amounts, plus taxes not associated with a specific
* account budget.
*
*
* optional int64 tax_amount_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The taxAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setTaxAmountMicros(long value) {
bitField0_ |= 0x00000100;
taxAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The sum of all taxes on the invoice, in micros. This equals the sum of the
* AccountBudgetSummary tax amounts, plus taxes not associated with a specific
* account budget.
*
*
* optional int64 tax_amount_micros = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearTaxAmountMicros() {
bitField0_ = (bitField0_ & ~0x00000100);
taxAmountMicros_ = 0L;
onChanged();
return this;
}
private long totalAmountMicros_ ;
/**
*
* Output only. The total amount, in micros. This equals the sum of
* Invoice.subtotal_amount_micros and Invoice.tax_amount_micros.
* Starting with v6, Invoice.regulatory_costs_subtotal_amount_micros is
* also added as it is no longer already included in
* Invoice.tax_amount_micros.
*
*
* optional int64 total_amount_micros = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the totalAmountMicros field is set.
*/
@java.lang.Override
public boolean hasTotalAmountMicros() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Output only. The total amount, in micros. This equals the sum of
* Invoice.subtotal_amount_micros and Invoice.tax_amount_micros.
* Starting with v6, Invoice.regulatory_costs_subtotal_amount_micros is
* also added as it is no longer already included in
* Invoice.tax_amount_micros.
*
*
* optional int64 total_amount_micros = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The totalAmountMicros.
*/
@java.lang.Override
public long getTotalAmountMicros() {
return totalAmountMicros_;
}
/**
*
* Output only. The total amount, in micros. This equals the sum of
* Invoice.subtotal_amount_micros and Invoice.tax_amount_micros.
* Starting with v6, Invoice.regulatory_costs_subtotal_amount_micros is
* also added as it is no longer already included in
* Invoice.tax_amount_micros.
*
*
* optional int64 total_amount_micros = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The totalAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setTotalAmountMicros(long value) {
bitField0_ |= 0x00000200;
totalAmountMicros_ = value;
onChanged();
return this;
}
/**
*
* Output only. The total amount, in micros. This equals the sum of
* Invoice.subtotal_amount_micros and Invoice.tax_amount_micros.
* Starting with v6, Invoice.regulatory_costs_subtotal_amount_micros is
* also added as it is no longer already included in
* Invoice.tax_amount_micros.
*
*
* optional int64 total_amount_micros = 35 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearTotalAmountMicros() {
bitField0_ = (bitField0_ & ~0x00000200);
totalAmountMicros_ = 0L;
onChanged();
return this;
}
private java.lang.Object correctedInvoice_ = "";
/**
*
* Output only. The resource name of the original invoice corrected, wrote off, or canceled
* by this invoice, if applicable. If `corrected_invoice` is set,
* `replaced_invoices` will not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* optional string corrected_invoice = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the correctedInvoice field is set.
*/
public boolean hasCorrectedInvoice() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Output only. The resource name of the original invoice corrected, wrote off, or canceled
* by this invoice, if applicable. If `corrected_invoice` is set,
* `replaced_invoices` will not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* optional string corrected_invoice = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The correctedInvoice.
*/
public java.lang.String getCorrectedInvoice() {
java.lang.Object ref = correctedInvoice_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
correctedInvoice_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The resource name of the original invoice corrected, wrote off, or canceled
* by this invoice, if applicable. If `corrected_invoice` is set,
* `replaced_invoices` will not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* optional string corrected_invoice = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for correctedInvoice.
*/
public com.google.protobuf.ByteString
getCorrectedInvoiceBytes() {
java.lang.Object ref = correctedInvoice_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
correctedInvoice_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The resource name of the original invoice corrected, wrote off, or canceled
* by this invoice, if applicable. If `corrected_invoice` is set,
* `replaced_invoices` will not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* optional string corrected_invoice = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The correctedInvoice to set.
* @return This builder for chaining.
*/
public Builder setCorrectedInvoice(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
correctedInvoice_ = value;
onChanged();
return this;
}
/**
*
* Output only. The resource name of the original invoice corrected, wrote off, or canceled
* by this invoice, if applicable. If `corrected_invoice` is set,
* `replaced_invoices` will not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* optional string corrected_invoice = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearCorrectedInvoice() {
bitField0_ = (bitField0_ & ~0x00000400);
correctedInvoice_ = getDefaultInstance().getCorrectedInvoice();
onChanged();
return this;
}
/**
*
* Output only. The resource name of the original invoice corrected, wrote off, or canceled
* by this invoice, if applicable. If `corrected_invoice` is set,
* `replaced_invoices` will not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* optional string corrected_invoice = 36 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for correctedInvoice to set.
* @return This builder for chaining.
*/
public Builder setCorrectedInvoiceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000400;
correctedInvoice_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList replacedInvoices_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureReplacedInvoicesIsMutable() {
if (!((bitField0_ & 0x00000800) != 0)) {
replacedInvoices_ = new com.google.protobuf.LazyStringArrayList(replacedInvoices_);
bitField0_ |= 0x00000800;
}
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return A list containing the replacedInvoices.
*/
public com.google.protobuf.ProtocolStringList
getReplacedInvoicesList() {
return replacedInvoices_.getUnmodifiableView();
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The count of replacedInvoices.
*/
public int getReplacedInvoicesCount() {
return replacedInvoices_.size();
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param index The index of the element to return.
* @return The replacedInvoices at the given index.
*/
public java.lang.String getReplacedInvoices(int index) {
return replacedInvoices_.get(index);
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param index The index of the value to return.
* @return The bytes of the replacedInvoices at the given index.
*/
public com.google.protobuf.ByteString
getReplacedInvoicesBytes(int index) {
return replacedInvoices_.getByteString(index);
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param index The index to set the value at.
* @param value The replacedInvoices to set.
* @return This builder for chaining.
*/
public Builder setReplacedInvoices(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureReplacedInvoicesIsMutable();
replacedInvoices_.set(index, value);
onChanged();
return this;
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The replacedInvoices to add.
* @return This builder for chaining.
*/
public Builder addReplacedInvoices(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureReplacedInvoicesIsMutable();
replacedInvoices_.add(value);
onChanged();
return this;
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param values The replacedInvoices to add.
* @return This builder for chaining.
*/
public Builder addAllReplacedInvoices(
java.lang.Iterable values) {
ensureReplacedInvoicesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, replacedInvoices_);
onChanged();
return this;
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearReplacedInvoices() {
replacedInvoices_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
/**
*
* Output only. The resource name of the original invoice(s) being rebilled or replaced by
* this invoice, if applicable. There might be multiple replaced invoices due
* to invoice consolidation. The replaced invoices may not belong to the same
* payments account. If `replaced_invoices` is set, `corrected_invoice` will
* not be set.
* Invoice resource names have the form:
* `customers/{customer_id}/invoices/{invoice_id}`
*
*
* repeated string replaced_invoices = 37 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes of the replacedInvoices to add.
* @return This builder for chaining.
*/
public Builder addReplacedInvoicesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureReplacedInvoicesIsMutable();
replacedInvoices_.add(value);
onChanged();
return this;
}
private java.lang.Object pdfUrl_ = "";
/**
*
* Output only. The URL to a PDF copy of the invoice. Users need to pass in their OAuth
* token to request the PDF with this URL.
*
*
* optional string pdf_url = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the pdfUrl field is set.
*/
public boolean hasPdfUrl() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* Output only. The URL to a PDF copy of the invoice. Users need to pass in their OAuth
* token to request the PDF with this URL.
*
*
* optional string pdf_url = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The pdfUrl.
*/
public java.lang.String getPdfUrl() {
java.lang.Object ref = pdfUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
pdfUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Output only. The URL to a PDF copy of the invoice. Users need to pass in their OAuth
* token to request the PDF with this URL.
*
*
* optional string pdf_url = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The bytes for pdfUrl.
*/
public com.google.protobuf.ByteString
getPdfUrlBytes() {
java.lang.Object ref = pdfUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pdfUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Output only. The URL to a PDF copy of the invoice. Users need to pass in their OAuth
* token to request the PDF with this URL.
*
*
* optional string pdf_url = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The pdfUrl to set.
* @return This builder for chaining.
*/
public Builder setPdfUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
pdfUrl_ = value;
onChanged();
return this;
}
/**
*
* Output only. The URL to a PDF copy of the invoice. Users need to pass in their OAuth
* token to request the PDF with this URL.
*
*
* optional string pdf_url = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearPdfUrl() {
bitField0_ = (bitField0_ & ~0x00001000);
pdfUrl_ = getDefaultInstance().getPdfUrl();
onChanged();
return this;
}
/**
*
* Output only. The URL to a PDF copy of the invoice. Users need to pass in their OAuth
* token to request the PDF with this URL.
*
*
* optional string pdf_url = 38 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The bytes for pdfUrl to set.
* @return This builder for chaining.
*/
public Builder setPdfUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00001000;
pdfUrl_ = value;
onChanged();
return this;
}
private java.util.List accountBudgetSummaries_ =
java.util.Collections.emptyList();
private void ensureAccountBudgetSummariesIsMutable() {
if (!((bitField0_ & 0x00002000) != 0)) {
accountBudgetSummaries_ = new java.util.ArrayList(accountBudgetSummaries_);
bitField0_ |= 0x00002000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.Builder, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummaryOrBuilder> accountBudgetSummariesBuilder_;
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public java.util.List getAccountBudgetSummariesList() {
if (accountBudgetSummariesBuilder_ == null) {
return java.util.Collections.unmodifiableList(accountBudgetSummaries_);
} else {
return accountBudgetSummariesBuilder_.getMessageList();
}
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public int getAccountBudgetSummariesCount() {
if (accountBudgetSummariesBuilder_ == null) {
return accountBudgetSummaries_.size();
} else {
return accountBudgetSummariesBuilder_.getCount();
}
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary getAccountBudgetSummaries(int index) {
if (accountBudgetSummariesBuilder_ == null) {
return accountBudgetSummaries_.get(index);
} else {
return accountBudgetSummariesBuilder_.getMessage(index);
}
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder setAccountBudgetSummaries(
int index, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary value) {
if (accountBudgetSummariesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAccountBudgetSummariesIsMutable();
accountBudgetSummaries_.set(index, value);
onChanged();
} else {
accountBudgetSummariesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder setAccountBudgetSummaries(
int index, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.Builder builderForValue) {
if (accountBudgetSummariesBuilder_ == null) {
ensureAccountBudgetSummariesIsMutable();
accountBudgetSummaries_.set(index, builderForValue.build());
onChanged();
} else {
accountBudgetSummariesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder addAccountBudgetSummaries(com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary value) {
if (accountBudgetSummariesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAccountBudgetSummariesIsMutable();
accountBudgetSummaries_.add(value);
onChanged();
} else {
accountBudgetSummariesBuilder_.addMessage(value);
}
return this;
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder addAccountBudgetSummaries(
int index, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary value) {
if (accountBudgetSummariesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAccountBudgetSummariesIsMutable();
accountBudgetSummaries_.add(index, value);
onChanged();
} else {
accountBudgetSummariesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder addAccountBudgetSummaries(
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.Builder builderForValue) {
if (accountBudgetSummariesBuilder_ == null) {
ensureAccountBudgetSummariesIsMutable();
accountBudgetSummaries_.add(builderForValue.build());
onChanged();
} else {
accountBudgetSummariesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder addAccountBudgetSummaries(
int index, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.Builder builderForValue) {
if (accountBudgetSummariesBuilder_ == null) {
ensureAccountBudgetSummariesIsMutable();
accountBudgetSummaries_.add(index, builderForValue.build());
onChanged();
} else {
accountBudgetSummariesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder addAllAccountBudgetSummaries(
java.lang.Iterable extends com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary> values) {
if (accountBudgetSummariesBuilder_ == null) {
ensureAccountBudgetSummariesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, accountBudgetSummaries_);
onChanged();
} else {
accountBudgetSummariesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder clearAccountBudgetSummaries() {
if (accountBudgetSummariesBuilder_ == null) {
accountBudgetSummaries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00002000);
onChanged();
} else {
accountBudgetSummariesBuilder_.clear();
}
return this;
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder removeAccountBudgetSummaries(int index) {
if (accountBudgetSummariesBuilder_ == null) {
ensureAccountBudgetSummariesIsMutable();
accountBudgetSummaries_.remove(index);
onChanged();
} else {
accountBudgetSummariesBuilder_.remove(index);
}
return this;
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.Builder getAccountBudgetSummariesBuilder(
int index) {
return getAccountBudgetSummariesFieldBuilder().getBuilder(index);
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummaryOrBuilder getAccountBudgetSummariesOrBuilder(
int index) {
if (accountBudgetSummariesBuilder_ == null) {
return accountBudgetSummaries_.get(index); } else {
return accountBudgetSummariesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public java.util.List extends com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummaryOrBuilder>
getAccountBudgetSummariesOrBuilderList() {
if (accountBudgetSummariesBuilder_ != null) {
return accountBudgetSummariesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(accountBudgetSummaries_);
}
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.Builder addAccountBudgetSummariesBuilder() {
return getAccountBudgetSummariesFieldBuilder().addBuilder(
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.getDefaultInstance());
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.Builder addAccountBudgetSummariesBuilder(
int index) {
return getAccountBudgetSummariesFieldBuilder().addBuilder(
index, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.getDefaultInstance());
}
/**
*
* Output only. The list of summarized account budget information associated with this
* invoice.
*
*
* repeated .google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary account_budget_summaries = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public java.util.List
getAccountBudgetSummariesBuilderList() {
return getAccountBudgetSummariesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.Builder, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummaryOrBuilder>
getAccountBudgetSummariesFieldBuilder() {
if (accountBudgetSummariesBuilder_ == null) {
accountBudgetSummariesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummary.Builder, com.google.ads.googleads.v10.resources.Invoice.AccountBudgetSummaryOrBuilder>(
accountBudgetSummaries_,
((bitField0_ & 0x00002000) != 0),
getParentForChildren(),
isClean());
accountBudgetSummaries_ = null;
}
return accountBudgetSummariesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v10.resources.Invoice)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v10.resources.Invoice)
private static final com.google.ads.googleads.v10.resources.Invoice DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v10.resources.Invoice();
}
public static com.google.ads.googleads.v10.resources.Invoice getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Invoice parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.Invoice getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy