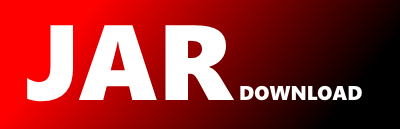
com.google.ads.googleads.v10.resources.UserList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v10 Show documentation
Show all versions of google-ads-stubs-v10 Show documentation
Stubs for GAAPI version google-ads-stubs-v10
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v10/resources/user_list.proto
package com.google.ads.googleads.v10.resources;
/**
*
* A user list. This is a list of users a customer may target.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.UserList}
*/
public final class UserList extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v10.resources.UserList)
UserListOrBuilder {
private static final long serialVersionUID = 0L;
// Use UserList.newBuilder() to construct.
private UserList(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UserList() {
resourceName_ = "";
name_ = "";
description_ = "";
membershipStatus_ = 0;
integrationCode_ = "";
sizeRangeForDisplay_ = 0;
sizeRangeForSearch_ = 0;
type_ = 0;
closingReason_ = 0;
accessReason_ = 0;
accountUserListStatus_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new UserList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.UserListProto.internal_static_google_ads_googleads_v10_resources_UserList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.UserListProto.internal_static_google_ads_googleads_v10_resources_UserList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.UserList.class, com.google.ads.googleads.v10.resources.UserList.Builder.class);
}
private int bitField0_;
private int userListCase_ = 0;
private java.lang.Object userList_;
public enum UserListCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
CRM_BASED_USER_LIST(19),
SIMILAR_USER_LIST(20),
RULE_BASED_USER_LIST(21),
LOGICAL_USER_LIST(22),
BASIC_USER_LIST(23),
USERLIST_NOT_SET(0);
private final int value;
private UserListCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static UserListCase valueOf(int value) {
return forNumber(value);
}
public static UserListCase forNumber(int value) {
switch (value) {
case 19: return CRM_BASED_USER_LIST;
case 20: return SIMILAR_USER_LIST;
case 21: return RULE_BASED_USER_LIST;
case 22: return LOGICAL_USER_LIST;
case 23: return BASIC_USER_LIST;
case 0: return USERLIST_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public UserListCase
getUserListCase() {
return UserListCase.forNumber(
userListCase_);
}
public static final int RESOURCE_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object resourceName_;
/**
*
* Immutable. The resource name of the user list.
* User list resource names have the form:
* `customers/{customer_id}/userLists/{user_list_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
@java.lang.Override
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
}
}
/**
*
* Immutable. The resource name of the user list.
* User list resource names have the form:
* `customers/{customer_id}/userLists/{user_list_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 25;
private long id_;
/**
*
* Output only. Id of the user list.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. Id of the user list.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int READ_ONLY_FIELD_NUMBER = 26;
private boolean readOnly_;
/**
*
* Output only. An option that indicates if a user may edit a list. Depends on the list
* ownership and list type. For example, external remarketing user lists are
* not editable.
* This field is read-only.
*
*
* optional bool read_only = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the readOnly field is set.
*/
@java.lang.Override
public boolean hasReadOnly() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. An option that indicates if a user may edit a list. Depends on the list
* ownership and list type. For example, external remarketing user lists are
* not editable.
* This field is read-only.
*
*
* optional bool read_only = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The readOnly.
*/
@java.lang.Override
public boolean getReadOnly() {
return readOnly_;
}
public static final int NAME_FIELD_NUMBER = 27;
private volatile java.lang.Object name_;
/**
*
* Name of this user list. Depending on its access_reason, the user list name
* may not be unique (for example, if access_reason=SHARED)
*
*
* optional string name = 27;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Name of this user list. Depending on its access_reason, the user list name
* may not be unique (for example, if access_reason=SHARED)
*
*
* optional string name = 27;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* Name of this user list. Depending on its access_reason, the user list name
* may not be unique (for example, if access_reason=SHARED)
*
*
* optional string name = 27;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 28;
private volatile java.lang.Object description_;
/**
*
* Description of this user list.
*
*
* optional string description = 28;
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Description of this user list.
*
*
* optional string description = 28;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* Description of this user list.
*
*
* optional string description = 28;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MEMBERSHIP_STATUS_FIELD_NUMBER = 6;
private int membershipStatus_;
/**
*
* Membership status of this user list. Indicates whether a user list is open
* or active. Only open user lists can accumulate more users and can be
* targeted to.
*
*
* .google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus membership_status = 6;
* @return The enum numeric value on the wire for membershipStatus.
*/
@java.lang.Override public int getMembershipStatusValue() {
return membershipStatus_;
}
/**
*
* Membership status of this user list. Indicates whether a user list is open
* or active. Only open user lists can accumulate more users and can be
* targeted to.
*
*
* .google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus membership_status = 6;
* @return The membershipStatus.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus getMembershipStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus result = com.google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus.valueOf(membershipStatus_);
return result == null ? com.google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus.UNRECOGNIZED : result;
}
public static final int INTEGRATION_CODE_FIELD_NUMBER = 29;
private volatile java.lang.Object integrationCode_;
/**
*
* An ID from external system. It is used by user list sellers to correlate
* IDs on their systems.
*
*
* optional string integration_code = 29;
* @return Whether the integrationCode field is set.
*/
@java.lang.Override
public boolean hasIntegrationCode() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* An ID from external system. It is used by user list sellers to correlate
* IDs on their systems.
*
*
* optional string integration_code = 29;
* @return The integrationCode.
*/
@java.lang.Override
public java.lang.String getIntegrationCode() {
java.lang.Object ref = integrationCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
integrationCode_ = s;
return s;
}
}
/**
*
* An ID from external system. It is used by user list sellers to correlate
* IDs on their systems.
*
*
* optional string integration_code = 29;
* @return The bytes for integrationCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIntegrationCodeBytes() {
java.lang.Object ref = integrationCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
integrationCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MEMBERSHIP_LIFE_SPAN_FIELD_NUMBER = 30;
private long membershipLifeSpan_;
/**
*
* Number of days a user's cookie stays on your list since its most recent
* addition to the list. This field must be between 0 and 540 inclusive.
* However, for CRM based userlists, this field can be set to 10000 which
* means no expiration.
* It'll be ignored for logical_user_list.
*
*
* optional int64 membership_life_span = 30;
* @return Whether the membershipLifeSpan field is set.
*/
@java.lang.Override
public boolean hasMembershipLifeSpan() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Number of days a user's cookie stays on your list since its most recent
* addition to the list. This field must be between 0 and 540 inclusive.
* However, for CRM based userlists, this field can be set to 10000 which
* means no expiration.
* It'll be ignored for logical_user_list.
*
*
* optional int64 membership_life_span = 30;
* @return The membershipLifeSpan.
*/
@java.lang.Override
public long getMembershipLifeSpan() {
return membershipLifeSpan_;
}
public static final int SIZE_FOR_DISPLAY_FIELD_NUMBER = 31;
private long sizeForDisplay_;
/**
*
* Output only. Estimated number of users in this user list, on the Google Display Network.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_display = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the sizeForDisplay field is set.
*/
@java.lang.Override
public boolean hasSizeForDisplay() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. Estimated number of users in this user list, on the Google Display Network.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_display = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The sizeForDisplay.
*/
@java.lang.Override
public long getSizeForDisplay() {
return sizeForDisplay_;
}
public static final int SIZE_RANGE_FOR_DISPLAY_FIELD_NUMBER = 10;
private int sizeRangeForDisplay_;
/**
*
* Output only. Size range in terms of number of users of the UserList, on the Google
* Display Network.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_display = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for sizeRangeForDisplay.
*/
@java.lang.Override public int getSizeRangeForDisplayValue() {
return sizeRangeForDisplay_;
}
/**
*
* Output only. Size range in terms of number of users of the UserList, on the Google
* Display Network.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_display = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The sizeRangeForDisplay.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange getSizeRangeForDisplay() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange result = com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.valueOf(sizeRangeForDisplay_);
return result == null ? com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.UNRECOGNIZED : result;
}
public static final int SIZE_FOR_SEARCH_FIELD_NUMBER = 32;
private long sizeForSearch_;
/**
*
* Output only. Estimated number of users in this user list in the google.com domain.
* These are the users available for targeting in Search campaigns.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_search = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the sizeForSearch field is set.
*/
@java.lang.Override
public boolean hasSizeForSearch() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Output only. Estimated number of users in this user list in the google.com domain.
* These are the users available for targeting in Search campaigns.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_search = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The sizeForSearch.
*/
@java.lang.Override
public long getSizeForSearch() {
return sizeForSearch_;
}
public static final int SIZE_RANGE_FOR_SEARCH_FIELD_NUMBER = 12;
private int sizeRangeForSearch_;
/**
*
* Output only. Size range in terms of number of users of the UserList, for Search ads.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_search = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for sizeRangeForSearch.
*/
@java.lang.Override public int getSizeRangeForSearchValue() {
return sizeRangeForSearch_;
}
/**
*
* Output only. Size range in terms of number of users of the UserList, for Search ads.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_search = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The sizeRangeForSearch.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange getSizeRangeForSearch() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange result = com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.valueOf(sizeRangeForSearch_);
return result == null ? com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.UNRECOGNIZED : result;
}
public static final int TYPE_FIELD_NUMBER = 13;
private int type_;
/**
*
* Output only. Type of this list.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListTypeEnum.UserListType type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
*
* Output only. Type of this list.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListTypeEnum.UserListType type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The type.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.UserListTypeEnum.UserListType getType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListTypeEnum.UserListType result = com.google.ads.googleads.v10.enums.UserListTypeEnum.UserListType.valueOf(type_);
return result == null ? com.google.ads.googleads.v10.enums.UserListTypeEnum.UserListType.UNRECOGNIZED : result;
}
public static final int CLOSING_REASON_FIELD_NUMBER = 14;
private int closingReason_;
/**
*
* Indicating the reason why this user list membership status is closed. It is
* only populated on lists that were automatically closed due to inactivity,
* and will be cleared once the list membership status becomes open.
*
*
* .google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason closing_reason = 14;
* @return The enum numeric value on the wire for closingReason.
*/
@java.lang.Override public int getClosingReasonValue() {
return closingReason_;
}
/**
*
* Indicating the reason why this user list membership status is closed. It is
* only populated on lists that were automatically closed due to inactivity,
* and will be cleared once the list membership status becomes open.
*
*
* .google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason closing_reason = 14;
* @return The closingReason.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason getClosingReason() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason result = com.google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason.valueOf(closingReason_);
return result == null ? com.google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason.UNRECOGNIZED : result;
}
public static final int ACCESS_REASON_FIELD_NUMBER = 15;
private int accessReason_;
/**
*
* Output only. Indicates the reason this account has been granted access to the list.
* The reason can be SHARED, OWNED, LICENSED or SUBSCRIBED.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason access_reason = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for accessReason.
*/
@java.lang.Override public int getAccessReasonValue() {
return accessReason_;
}
/**
*
* Output only. Indicates the reason this account has been granted access to the list.
* The reason can be SHARED, OWNED, LICENSED or SUBSCRIBED.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason access_reason = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The accessReason.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason getAccessReason() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason result = com.google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason.valueOf(accessReason_);
return result == null ? com.google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason.UNRECOGNIZED : result;
}
public static final int ACCOUNT_USER_LIST_STATUS_FIELD_NUMBER = 16;
private int accountUserListStatus_;
/**
*
* Indicates if this share is still enabled. When a UserList is shared with
* the user this field is set to ENABLED. Later the userList owner can decide
* to revoke the share and make it DISABLED.
* The default value of this field is set to ENABLED.
*
*
* .google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus account_user_list_status = 16;
* @return The enum numeric value on the wire for accountUserListStatus.
*/
@java.lang.Override public int getAccountUserListStatusValue() {
return accountUserListStatus_;
}
/**
*
* Indicates if this share is still enabled. When a UserList is shared with
* the user this field is set to ENABLED. Later the userList owner can decide
* to revoke the share and make it DISABLED.
* The default value of this field is set to ENABLED.
*
*
* .google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus account_user_list_status = 16;
* @return The accountUserListStatus.
*/
@java.lang.Override public com.google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus getAccountUserListStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus result = com.google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus.valueOf(accountUserListStatus_);
return result == null ? com.google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus.UNRECOGNIZED : result;
}
public static final int ELIGIBLE_FOR_SEARCH_FIELD_NUMBER = 33;
private boolean eligibleForSearch_;
/**
*
* Indicates if this user list is eligible for Google Search Network.
*
*
* optional bool eligible_for_search = 33;
* @return Whether the eligibleForSearch field is set.
*/
@java.lang.Override
public boolean hasEligibleForSearch() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Indicates if this user list is eligible for Google Search Network.
*
*
* optional bool eligible_for_search = 33;
* @return The eligibleForSearch.
*/
@java.lang.Override
public boolean getEligibleForSearch() {
return eligibleForSearch_;
}
public static final int ELIGIBLE_FOR_DISPLAY_FIELD_NUMBER = 34;
private boolean eligibleForDisplay_;
/**
*
* Output only. Indicates this user list is eligible for Google Display Network.
* This field is read-only.
*
*
* optional bool eligible_for_display = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the eligibleForDisplay field is set.
*/
@java.lang.Override
public boolean hasEligibleForDisplay() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Output only. Indicates this user list is eligible for Google Display Network.
* This field is read-only.
*
*
* optional bool eligible_for_display = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The eligibleForDisplay.
*/
@java.lang.Override
public boolean getEligibleForDisplay() {
return eligibleForDisplay_;
}
public static final int MATCH_RATE_PERCENTAGE_FIELD_NUMBER = 24;
private int matchRatePercentage_;
/**
*
* Output only. Indicates match rate for Customer Match lists. The range of this field is
* [0-100]. This will be null for other list types or when it's not possible
* to calculate the match rate.
* This field is read-only.
*
*
* optional int32 match_rate_percentage = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the matchRatePercentage field is set.
*/
@java.lang.Override
public boolean hasMatchRatePercentage() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Output only. Indicates match rate for Customer Match lists. The range of this field is
* [0-100]. This will be null for other list types or when it's not possible
* to calculate the match rate.
* This field is read-only.
*
*
* optional int32 match_rate_percentage = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The matchRatePercentage.
*/
@java.lang.Override
public int getMatchRatePercentage() {
return matchRatePercentage_;
}
public static final int CRM_BASED_USER_LIST_FIELD_NUMBER = 19;
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
* @return Whether the crmBasedUserList field is set.
*/
@java.lang.Override
public boolean hasCrmBasedUserList() {
return userListCase_ == 19;
}
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
* @return The crmBasedUserList.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.CrmBasedUserListInfo getCrmBasedUserList() {
if (userListCase_ == 19) {
return (com.google.ads.googleads.v10.common.CrmBasedUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.CrmBasedUserListInfo.getDefaultInstance();
}
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.CrmBasedUserListInfoOrBuilder getCrmBasedUserListOrBuilder() {
if (userListCase_ == 19) {
return (com.google.ads.googleads.v10.common.CrmBasedUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.CrmBasedUserListInfo.getDefaultInstance();
}
public static final int SIMILAR_USER_LIST_FIELD_NUMBER = 20;
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the similarUserList field is set.
*/
@java.lang.Override
public boolean hasSimilarUserList() {
return userListCase_ == 20;
}
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The similarUserList.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.SimilarUserListInfo getSimilarUserList() {
if (userListCase_ == 20) {
return (com.google.ads.googleads.v10.common.SimilarUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.SimilarUserListInfo.getDefaultInstance();
}
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.SimilarUserListInfoOrBuilder getSimilarUserListOrBuilder() {
if (userListCase_ == 20) {
return (com.google.ads.googleads.v10.common.SimilarUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.SimilarUserListInfo.getDefaultInstance();
}
public static final int RULE_BASED_USER_LIST_FIELD_NUMBER = 21;
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
* @return Whether the ruleBasedUserList field is set.
*/
@java.lang.Override
public boolean hasRuleBasedUserList() {
return userListCase_ == 21;
}
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
* @return The ruleBasedUserList.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.RuleBasedUserListInfo getRuleBasedUserList() {
if (userListCase_ == 21) {
return (com.google.ads.googleads.v10.common.RuleBasedUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.RuleBasedUserListInfo.getDefaultInstance();
}
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.RuleBasedUserListInfoOrBuilder getRuleBasedUserListOrBuilder() {
if (userListCase_ == 21) {
return (com.google.ads.googleads.v10.common.RuleBasedUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.RuleBasedUserListInfo.getDefaultInstance();
}
public static final int LOGICAL_USER_LIST_FIELD_NUMBER = 22;
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
* @return Whether the logicalUserList field is set.
*/
@java.lang.Override
public boolean hasLogicalUserList() {
return userListCase_ == 22;
}
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
* @return The logicalUserList.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.LogicalUserListInfo getLogicalUserList() {
if (userListCase_ == 22) {
return (com.google.ads.googleads.v10.common.LogicalUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.LogicalUserListInfo.getDefaultInstance();
}
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.LogicalUserListInfoOrBuilder getLogicalUserListOrBuilder() {
if (userListCase_ == 22) {
return (com.google.ads.googleads.v10.common.LogicalUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.LogicalUserListInfo.getDefaultInstance();
}
public static final int BASIC_USER_LIST_FIELD_NUMBER = 23;
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
* @return Whether the basicUserList field is set.
*/
@java.lang.Override
public boolean hasBasicUserList() {
return userListCase_ == 23;
}
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
* @return The basicUserList.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.BasicUserListInfo getBasicUserList() {
if (userListCase_ == 23) {
return (com.google.ads.googleads.v10.common.BasicUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.BasicUserListInfo.getDefaultInstance();
}
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.BasicUserListInfoOrBuilder getBasicUserListOrBuilder() {
if (userListCase_ == 23) {
return (com.google.ads.googleads.v10.common.BasicUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.BasicUserListInfo.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, resourceName_);
}
if (membershipStatus_ != com.google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus.UNSPECIFIED.getNumber()) {
output.writeEnum(6, membershipStatus_);
}
if (sizeRangeForDisplay_ != com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.UNSPECIFIED.getNumber()) {
output.writeEnum(10, sizeRangeForDisplay_);
}
if (sizeRangeForSearch_ != com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.UNSPECIFIED.getNumber()) {
output.writeEnum(12, sizeRangeForSearch_);
}
if (type_ != com.google.ads.googleads.v10.enums.UserListTypeEnum.UserListType.UNSPECIFIED.getNumber()) {
output.writeEnum(13, type_);
}
if (closingReason_ != com.google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason.UNSPECIFIED.getNumber()) {
output.writeEnum(14, closingReason_);
}
if (accessReason_ != com.google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason.UNSPECIFIED.getNumber()) {
output.writeEnum(15, accessReason_);
}
if (accountUserListStatus_ != com.google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus.UNSPECIFIED.getNumber()) {
output.writeEnum(16, accountUserListStatus_);
}
if (userListCase_ == 19) {
output.writeMessage(19, (com.google.ads.googleads.v10.common.CrmBasedUserListInfo) userList_);
}
if (userListCase_ == 20) {
output.writeMessage(20, (com.google.ads.googleads.v10.common.SimilarUserListInfo) userList_);
}
if (userListCase_ == 21) {
output.writeMessage(21, (com.google.ads.googleads.v10.common.RuleBasedUserListInfo) userList_);
}
if (userListCase_ == 22) {
output.writeMessage(22, (com.google.ads.googleads.v10.common.LogicalUserListInfo) userList_);
}
if (userListCase_ == 23) {
output.writeMessage(23, (com.google.ads.googleads.v10.common.BasicUserListInfo) userList_);
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeInt32(24, matchRatePercentage_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(25, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBool(26, readOnly_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 27, name_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 28, description_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 29, integrationCode_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt64(30, membershipLifeSpan_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeInt64(31, sizeForDisplay_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeInt64(32, sizeForSearch_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeBool(33, eligibleForSearch_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeBool(34, eligibleForDisplay_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, resourceName_);
}
if (membershipStatus_ != com.google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, membershipStatus_);
}
if (sizeRangeForDisplay_ != com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, sizeRangeForDisplay_);
}
if (sizeRangeForSearch_ != com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(12, sizeRangeForSearch_);
}
if (type_ != com.google.ads.googleads.v10.enums.UserListTypeEnum.UserListType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(13, type_);
}
if (closingReason_ != com.google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(14, closingReason_);
}
if (accessReason_ != com.google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(15, accessReason_);
}
if (accountUserListStatus_ != com.google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(16, accountUserListStatus_);
}
if (userListCase_ == 19) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(19, (com.google.ads.googleads.v10.common.CrmBasedUserListInfo) userList_);
}
if (userListCase_ == 20) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, (com.google.ads.googleads.v10.common.SimilarUserListInfo) userList_);
}
if (userListCase_ == 21) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, (com.google.ads.googleads.v10.common.RuleBasedUserListInfo) userList_);
}
if (userListCase_ == 22) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(22, (com.google.ads.googleads.v10.common.LogicalUserListInfo) userList_);
}
if (userListCase_ == 23) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(23, (com.google.ads.googleads.v10.common.BasicUserListInfo) userList_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(24, matchRatePercentage_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(25, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(26, readOnly_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(27, name_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(28, description_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(29, integrationCode_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(30, membershipLifeSpan_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(31, sizeForDisplay_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(32, sizeForSearch_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(33, eligibleForSearch_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(34, eligibleForDisplay_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v10.resources.UserList)) {
return super.equals(obj);
}
com.google.ads.googleads.v10.resources.UserList other = (com.google.ads.googleads.v10.resources.UserList) obj;
if (!getResourceName()
.equals(other.getResourceName())) return false;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId()
!= other.getId()) return false;
}
if (hasReadOnly() != other.hasReadOnly()) return false;
if (hasReadOnly()) {
if (getReadOnly()
!= other.getReadOnly()) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasDescription() != other.hasDescription()) return false;
if (hasDescription()) {
if (!getDescription()
.equals(other.getDescription())) return false;
}
if (membershipStatus_ != other.membershipStatus_) return false;
if (hasIntegrationCode() != other.hasIntegrationCode()) return false;
if (hasIntegrationCode()) {
if (!getIntegrationCode()
.equals(other.getIntegrationCode())) return false;
}
if (hasMembershipLifeSpan() != other.hasMembershipLifeSpan()) return false;
if (hasMembershipLifeSpan()) {
if (getMembershipLifeSpan()
!= other.getMembershipLifeSpan()) return false;
}
if (hasSizeForDisplay() != other.hasSizeForDisplay()) return false;
if (hasSizeForDisplay()) {
if (getSizeForDisplay()
!= other.getSizeForDisplay()) return false;
}
if (sizeRangeForDisplay_ != other.sizeRangeForDisplay_) return false;
if (hasSizeForSearch() != other.hasSizeForSearch()) return false;
if (hasSizeForSearch()) {
if (getSizeForSearch()
!= other.getSizeForSearch()) return false;
}
if (sizeRangeForSearch_ != other.sizeRangeForSearch_) return false;
if (type_ != other.type_) return false;
if (closingReason_ != other.closingReason_) return false;
if (accessReason_ != other.accessReason_) return false;
if (accountUserListStatus_ != other.accountUserListStatus_) return false;
if (hasEligibleForSearch() != other.hasEligibleForSearch()) return false;
if (hasEligibleForSearch()) {
if (getEligibleForSearch()
!= other.getEligibleForSearch()) return false;
}
if (hasEligibleForDisplay() != other.hasEligibleForDisplay()) return false;
if (hasEligibleForDisplay()) {
if (getEligibleForDisplay()
!= other.getEligibleForDisplay()) return false;
}
if (hasMatchRatePercentage() != other.hasMatchRatePercentage()) return false;
if (hasMatchRatePercentage()) {
if (getMatchRatePercentage()
!= other.getMatchRatePercentage()) return false;
}
if (!getUserListCase().equals(other.getUserListCase())) return false;
switch (userListCase_) {
case 19:
if (!getCrmBasedUserList()
.equals(other.getCrmBasedUserList())) return false;
break;
case 20:
if (!getSimilarUserList()
.equals(other.getSimilarUserList())) return false;
break;
case 21:
if (!getRuleBasedUserList()
.equals(other.getRuleBasedUserList())) return false;
break;
case 22:
if (!getLogicalUserList()
.equals(other.getLogicalUserList())) return false;
break;
case 23:
if (!getBasicUserList()
.equals(other.getBasicUserList())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RESOURCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getResourceName().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getId());
}
if (hasReadOnly()) {
hash = (37 * hash) + READ_ONLY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getReadOnly());
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
hash = (37 * hash) + MEMBERSHIP_STATUS_FIELD_NUMBER;
hash = (53 * hash) + membershipStatus_;
if (hasIntegrationCode()) {
hash = (37 * hash) + INTEGRATION_CODE_FIELD_NUMBER;
hash = (53 * hash) + getIntegrationCode().hashCode();
}
if (hasMembershipLifeSpan()) {
hash = (37 * hash) + MEMBERSHIP_LIFE_SPAN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMembershipLifeSpan());
}
if (hasSizeForDisplay()) {
hash = (37 * hash) + SIZE_FOR_DISPLAY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSizeForDisplay());
}
hash = (37 * hash) + SIZE_RANGE_FOR_DISPLAY_FIELD_NUMBER;
hash = (53 * hash) + sizeRangeForDisplay_;
if (hasSizeForSearch()) {
hash = (37 * hash) + SIZE_FOR_SEARCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSizeForSearch());
}
hash = (37 * hash) + SIZE_RANGE_FOR_SEARCH_FIELD_NUMBER;
hash = (53 * hash) + sizeRangeForSearch_;
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (37 * hash) + CLOSING_REASON_FIELD_NUMBER;
hash = (53 * hash) + closingReason_;
hash = (37 * hash) + ACCESS_REASON_FIELD_NUMBER;
hash = (53 * hash) + accessReason_;
hash = (37 * hash) + ACCOUNT_USER_LIST_STATUS_FIELD_NUMBER;
hash = (53 * hash) + accountUserListStatus_;
if (hasEligibleForSearch()) {
hash = (37 * hash) + ELIGIBLE_FOR_SEARCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getEligibleForSearch());
}
if (hasEligibleForDisplay()) {
hash = (37 * hash) + ELIGIBLE_FOR_DISPLAY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getEligibleForDisplay());
}
if (hasMatchRatePercentage()) {
hash = (37 * hash) + MATCH_RATE_PERCENTAGE_FIELD_NUMBER;
hash = (53 * hash) + getMatchRatePercentage();
}
switch (userListCase_) {
case 19:
hash = (37 * hash) + CRM_BASED_USER_LIST_FIELD_NUMBER;
hash = (53 * hash) + getCrmBasedUserList().hashCode();
break;
case 20:
hash = (37 * hash) + SIMILAR_USER_LIST_FIELD_NUMBER;
hash = (53 * hash) + getSimilarUserList().hashCode();
break;
case 21:
hash = (37 * hash) + RULE_BASED_USER_LIST_FIELD_NUMBER;
hash = (53 * hash) + getRuleBasedUserList().hashCode();
break;
case 22:
hash = (37 * hash) + LOGICAL_USER_LIST_FIELD_NUMBER;
hash = (53 * hash) + getLogicalUserList().hashCode();
break;
case 23:
hash = (37 * hash) + BASIC_USER_LIST_FIELD_NUMBER;
hash = (53 * hash) + getBasicUserList().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v10.resources.UserList parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.UserList parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.UserList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.UserList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.UserList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v10.resources.UserList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.UserList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.UserList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.UserList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.UserList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v10.resources.UserList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v10.resources.UserList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v10.resources.UserList prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A user list. This is a list of users a customer may target.
*
*
* Protobuf type {@code google.ads.googleads.v10.resources.UserList}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v10.resources.UserList)
com.google.ads.googleads.v10.resources.UserListOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v10.resources.UserListProto.internal_static_google_ads_googleads_v10_resources_UserList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v10.resources.UserListProto.internal_static_google_ads_googleads_v10_resources_UserList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v10.resources.UserList.class, com.google.ads.googleads.v10.resources.UserList.Builder.class);
}
// Construct using com.google.ads.googleads.v10.resources.UserList.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
resourceName_ = "";
id_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
readOnly_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
description_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
membershipStatus_ = 0;
integrationCode_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
membershipLifeSpan_ = 0L;
bitField0_ = (bitField0_ & ~0x00000020);
sizeForDisplay_ = 0L;
bitField0_ = (bitField0_ & ~0x00000040);
sizeRangeForDisplay_ = 0;
sizeForSearch_ = 0L;
bitField0_ = (bitField0_ & ~0x00000080);
sizeRangeForSearch_ = 0;
type_ = 0;
closingReason_ = 0;
accessReason_ = 0;
accountUserListStatus_ = 0;
eligibleForSearch_ = false;
bitField0_ = (bitField0_ & ~0x00000100);
eligibleForDisplay_ = false;
bitField0_ = (bitField0_ & ~0x00000200);
matchRatePercentage_ = 0;
bitField0_ = (bitField0_ & ~0x00000400);
if (crmBasedUserListBuilder_ != null) {
crmBasedUserListBuilder_.clear();
}
if (similarUserListBuilder_ != null) {
similarUserListBuilder_.clear();
}
if (ruleBasedUserListBuilder_ != null) {
ruleBasedUserListBuilder_.clear();
}
if (logicalUserListBuilder_ != null) {
logicalUserListBuilder_.clear();
}
if (basicUserListBuilder_ != null) {
basicUserListBuilder_.clear();
}
userListCase_ = 0;
userList_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v10.resources.UserListProto.internal_static_google_ads_googleads_v10_resources_UserList_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.UserList getDefaultInstanceForType() {
return com.google.ads.googleads.v10.resources.UserList.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.UserList build() {
com.google.ads.googleads.v10.resources.UserList result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.UserList buildPartial() {
com.google.ads.googleads.v10.resources.UserList result = new com.google.ads.googleads.v10.resources.UserList(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.resourceName_ = resourceName_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.readOnly_ = readOnly_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.description_ = description_;
result.membershipStatus_ = membershipStatus_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.integrationCode_ = integrationCode_;
if (((from_bitField0_ & 0x00000020) != 0)) {
result.membershipLifeSpan_ = membershipLifeSpan_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.sizeForDisplay_ = sizeForDisplay_;
to_bitField0_ |= 0x00000040;
}
result.sizeRangeForDisplay_ = sizeRangeForDisplay_;
if (((from_bitField0_ & 0x00000080) != 0)) {
result.sizeForSearch_ = sizeForSearch_;
to_bitField0_ |= 0x00000080;
}
result.sizeRangeForSearch_ = sizeRangeForSearch_;
result.type_ = type_;
result.closingReason_ = closingReason_;
result.accessReason_ = accessReason_;
result.accountUserListStatus_ = accountUserListStatus_;
if (((from_bitField0_ & 0x00000100) != 0)) {
result.eligibleForSearch_ = eligibleForSearch_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.eligibleForDisplay_ = eligibleForDisplay_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.matchRatePercentage_ = matchRatePercentage_;
to_bitField0_ |= 0x00000400;
}
if (userListCase_ == 19) {
if (crmBasedUserListBuilder_ == null) {
result.userList_ = userList_;
} else {
result.userList_ = crmBasedUserListBuilder_.build();
}
}
if (userListCase_ == 20) {
if (similarUserListBuilder_ == null) {
result.userList_ = userList_;
} else {
result.userList_ = similarUserListBuilder_.build();
}
}
if (userListCase_ == 21) {
if (ruleBasedUserListBuilder_ == null) {
result.userList_ = userList_;
} else {
result.userList_ = ruleBasedUserListBuilder_.build();
}
}
if (userListCase_ == 22) {
if (logicalUserListBuilder_ == null) {
result.userList_ = userList_;
} else {
result.userList_ = logicalUserListBuilder_.build();
}
}
if (userListCase_ == 23) {
if (basicUserListBuilder_ == null) {
result.userList_ = userList_;
} else {
result.userList_ = basicUserListBuilder_.build();
}
}
result.bitField0_ = to_bitField0_;
result.userListCase_ = userListCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v10.resources.UserList) {
return mergeFrom((com.google.ads.googleads.v10.resources.UserList)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v10.resources.UserList other) {
if (other == com.google.ads.googleads.v10.resources.UserList.getDefaultInstance()) return this;
if (!other.getResourceName().isEmpty()) {
resourceName_ = other.resourceName_;
onChanged();
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasReadOnly()) {
setReadOnly(other.getReadOnly());
}
if (other.hasName()) {
bitField0_ |= 0x00000004;
name_ = other.name_;
onChanged();
}
if (other.hasDescription()) {
bitField0_ |= 0x00000008;
description_ = other.description_;
onChanged();
}
if (other.membershipStatus_ != 0) {
setMembershipStatusValue(other.getMembershipStatusValue());
}
if (other.hasIntegrationCode()) {
bitField0_ |= 0x00000010;
integrationCode_ = other.integrationCode_;
onChanged();
}
if (other.hasMembershipLifeSpan()) {
setMembershipLifeSpan(other.getMembershipLifeSpan());
}
if (other.hasSizeForDisplay()) {
setSizeForDisplay(other.getSizeForDisplay());
}
if (other.sizeRangeForDisplay_ != 0) {
setSizeRangeForDisplayValue(other.getSizeRangeForDisplayValue());
}
if (other.hasSizeForSearch()) {
setSizeForSearch(other.getSizeForSearch());
}
if (other.sizeRangeForSearch_ != 0) {
setSizeRangeForSearchValue(other.getSizeRangeForSearchValue());
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.closingReason_ != 0) {
setClosingReasonValue(other.getClosingReasonValue());
}
if (other.accessReason_ != 0) {
setAccessReasonValue(other.getAccessReasonValue());
}
if (other.accountUserListStatus_ != 0) {
setAccountUserListStatusValue(other.getAccountUserListStatusValue());
}
if (other.hasEligibleForSearch()) {
setEligibleForSearch(other.getEligibleForSearch());
}
if (other.hasEligibleForDisplay()) {
setEligibleForDisplay(other.getEligibleForDisplay());
}
if (other.hasMatchRatePercentage()) {
setMatchRatePercentage(other.getMatchRatePercentage());
}
switch (other.getUserListCase()) {
case CRM_BASED_USER_LIST: {
mergeCrmBasedUserList(other.getCrmBasedUserList());
break;
}
case SIMILAR_USER_LIST: {
mergeSimilarUserList(other.getSimilarUserList());
break;
}
case RULE_BASED_USER_LIST: {
mergeRuleBasedUserList(other.getRuleBasedUserList());
break;
}
case LOGICAL_USER_LIST: {
mergeLogicalUserList(other.getLogicalUserList());
break;
}
case BASIC_USER_LIST: {
mergeBasicUserList(other.getBasicUserList());
break;
}
case USERLIST_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
resourceName_ = input.readStringRequireUtf8();
break;
} // case 10
case 48: {
membershipStatus_ = input.readEnum();
break;
} // case 48
case 80: {
sizeRangeForDisplay_ = input.readEnum();
break;
} // case 80
case 96: {
sizeRangeForSearch_ = input.readEnum();
break;
} // case 96
case 104: {
type_ = input.readEnum();
break;
} // case 104
case 112: {
closingReason_ = input.readEnum();
break;
} // case 112
case 120: {
accessReason_ = input.readEnum();
break;
} // case 120
case 128: {
accountUserListStatus_ = input.readEnum();
break;
} // case 128
case 154: {
input.readMessage(
getCrmBasedUserListFieldBuilder().getBuilder(),
extensionRegistry);
userListCase_ = 19;
break;
} // case 154
case 162: {
input.readMessage(
getSimilarUserListFieldBuilder().getBuilder(),
extensionRegistry);
userListCase_ = 20;
break;
} // case 162
case 170: {
input.readMessage(
getRuleBasedUserListFieldBuilder().getBuilder(),
extensionRegistry);
userListCase_ = 21;
break;
} // case 170
case 178: {
input.readMessage(
getLogicalUserListFieldBuilder().getBuilder(),
extensionRegistry);
userListCase_ = 22;
break;
} // case 178
case 186: {
input.readMessage(
getBasicUserListFieldBuilder().getBuilder(),
extensionRegistry);
userListCase_ = 23;
break;
} // case 186
case 192: {
matchRatePercentage_ = input.readInt32();
bitField0_ |= 0x00000400;
break;
} // case 192
case 200: {
id_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 200
case 208: {
readOnly_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 208
case 218: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 218
case 226: {
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 226
case 234: {
integrationCode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 234
case 240: {
membershipLifeSpan_ = input.readInt64();
bitField0_ |= 0x00000020;
break;
} // case 240
case 248: {
sizeForDisplay_ = input.readInt64();
bitField0_ |= 0x00000040;
break;
} // case 248
case 256: {
sizeForSearch_ = input.readInt64();
bitField0_ |= 0x00000080;
break;
} // case 256
case 264: {
eligibleForSearch_ = input.readBool();
bitField0_ |= 0x00000100;
break;
} // case 264
case 272: {
eligibleForDisplay_ = input.readBool();
bitField0_ |= 0x00000200;
break;
} // case 272
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int userListCase_ = 0;
private java.lang.Object userList_;
public UserListCase
getUserListCase() {
return UserListCase.forNumber(
userListCase_);
}
public Builder clearUserList() {
userListCase_ = 0;
userList_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object resourceName_ = "";
/**
*
* Immutable. The resource name of the user list.
* User list resource names have the form:
* `customers/{customer_id}/userLists/{user_list_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The resource name of the user list.
* User list resource names have the form:
* `customers/{customer_id}/userLists/{user_list_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The resource name of the user list.
* User list resource names have the form:
* `customers/{customer_id}/userLists/{user_list_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resourceName_ = value;
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the user list.
* User list resource names have the form:
* `customers/{customer_id}/userLists/{user_list_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearResourceName() {
resourceName_ = getDefaultInstance().getResourceName();
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the user list.
* User list resource names have the form:
* `customers/{customer_id}/userLists/{user_list_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The bytes for resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resourceName_ = value;
onChanged();
return this;
}
private long id_ ;
/**
*
* Output only. Id of the user list.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. Id of the user list.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
*
* Output only. Id of the user list.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
*
* Output only. Id of the user list.
*
*
* optional int64 id = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0L;
onChanged();
return this;
}
private boolean readOnly_ ;
/**
*
* Output only. An option that indicates if a user may edit a list. Depends on the list
* ownership and list type. For example, external remarketing user lists are
* not editable.
* This field is read-only.
*
*
* optional bool read_only = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the readOnly field is set.
*/
@java.lang.Override
public boolean hasReadOnly() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. An option that indicates if a user may edit a list. Depends on the list
* ownership and list type. For example, external remarketing user lists are
* not editable.
* This field is read-only.
*
*
* optional bool read_only = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The readOnly.
*/
@java.lang.Override
public boolean getReadOnly() {
return readOnly_;
}
/**
*
* Output only. An option that indicates if a user may edit a list. Depends on the list
* ownership and list type. For example, external remarketing user lists are
* not editable.
* This field is read-only.
*
*
* optional bool read_only = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The readOnly to set.
* @return This builder for chaining.
*/
public Builder setReadOnly(boolean value) {
bitField0_ |= 0x00000002;
readOnly_ = value;
onChanged();
return this;
}
/**
*
* Output only. An option that indicates if a user may edit a list. Depends on the list
* ownership and list type. For example, external remarketing user lists are
* not editable.
* This field is read-only.
*
*
* optional bool read_only = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearReadOnly() {
bitField0_ = (bitField0_ & ~0x00000002);
readOnly_ = false;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* Name of this user list. Depending on its access_reason, the user list name
* may not be unique (for example, if access_reason=SHARED)
*
*
* optional string name = 27;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Name of this user list. Depending on its access_reason, the user list name
* may not be unique (for example, if access_reason=SHARED)
*
*
* optional string name = 27;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of this user list. Depending on its access_reason, the user list name
* may not be unique (for example, if access_reason=SHARED)
*
*
* optional string name = 27;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of this user list. Depending on its access_reason, the user list name
* may not be unique (for example, if access_reason=SHARED)
*
*
* optional string name = 27;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
name_ = value;
onChanged();
return this;
}
/**
*
* Name of this user list. Depending on its access_reason, the user list name
* may not be unique (for example, if access_reason=SHARED)
*
*
* optional string name = 27;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000004);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Name of this user list. Depending on its access_reason, the user list name
* may not be unique (for example, if access_reason=SHARED)
*
*
* optional string name = 27;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000004;
name_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* Description of this user list.
*
*
* optional string description = 28;
* @return Whether the description field is set.
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Description of this user list.
*
*
* optional string description = 28;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Description of this user list.
*
*
* optional string description = 28;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Description of this user list.
*
*
* optional string description = 28;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
description_ = value;
onChanged();
return this;
}
/**
*
* Description of this user list.
*
*
* optional string description = 28;
* @return This builder for chaining.
*/
public Builder clearDescription() {
bitField0_ = (bitField0_ & ~0x00000008);
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* Description of this user list.
*
*
* optional string description = 28;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000008;
description_ = value;
onChanged();
return this;
}
private int membershipStatus_ = 0;
/**
*
* Membership status of this user list. Indicates whether a user list is open
* or active. Only open user lists can accumulate more users and can be
* targeted to.
*
*
* .google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus membership_status = 6;
* @return The enum numeric value on the wire for membershipStatus.
*/
@java.lang.Override public int getMembershipStatusValue() {
return membershipStatus_;
}
/**
*
* Membership status of this user list. Indicates whether a user list is open
* or active. Only open user lists can accumulate more users and can be
* targeted to.
*
*
* .google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus membership_status = 6;
* @param value The enum numeric value on the wire for membershipStatus to set.
* @return This builder for chaining.
*/
public Builder setMembershipStatusValue(int value) {
membershipStatus_ = value;
onChanged();
return this;
}
/**
*
* Membership status of this user list. Indicates whether a user list is open
* or active. Only open user lists can accumulate more users and can be
* targeted to.
*
*
* .google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus membership_status = 6;
* @return The membershipStatus.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus getMembershipStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus result = com.google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus.valueOf(membershipStatus_);
return result == null ? com.google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus.UNRECOGNIZED : result;
}
/**
*
* Membership status of this user list. Indicates whether a user list is open
* or active. Only open user lists can accumulate more users and can be
* targeted to.
*
*
* .google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus membership_status = 6;
* @param value The membershipStatus to set.
* @return This builder for chaining.
*/
public Builder setMembershipStatus(com.google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus value) {
if (value == null) {
throw new NullPointerException();
}
membershipStatus_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Membership status of this user list. Indicates whether a user list is open
* or active. Only open user lists can accumulate more users and can be
* targeted to.
*
*
* .google.ads.googleads.v10.enums.UserListMembershipStatusEnum.UserListMembershipStatus membership_status = 6;
* @return This builder for chaining.
*/
public Builder clearMembershipStatus() {
membershipStatus_ = 0;
onChanged();
return this;
}
private java.lang.Object integrationCode_ = "";
/**
*
* An ID from external system. It is used by user list sellers to correlate
* IDs on their systems.
*
*
* optional string integration_code = 29;
* @return Whether the integrationCode field is set.
*/
public boolean hasIntegrationCode() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* An ID from external system. It is used by user list sellers to correlate
* IDs on their systems.
*
*
* optional string integration_code = 29;
* @return The integrationCode.
*/
public java.lang.String getIntegrationCode() {
java.lang.Object ref = integrationCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
integrationCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* An ID from external system. It is used by user list sellers to correlate
* IDs on their systems.
*
*
* optional string integration_code = 29;
* @return The bytes for integrationCode.
*/
public com.google.protobuf.ByteString
getIntegrationCodeBytes() {
java.lang.Object ref = integrationCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
integrationCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* An ID from external system. It is used by user list sellers to correlate
* IDs on their systems.
*
*
* optional string integration_code = 29;
* @param value The integrationCode to set.
* @return This builder for chaining.
*/
public Builder setIntegrationCode(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
integrationCode_ = value;
onChanged();
return this;
}
/**
*
* An ID from external system. It is used by user list sellers to correlate
* IDs on their systems.
*
*
* optional string integration_code = 29;
* @return This builder for chaining.
*/
public Builder clearIntegrationCode() {
bitField0_ = (bitField0_ & ~0x00000010);
integrationCode_ = getDefaultInstance().getIntegrationCode();
onChanged();
return this;
}
/**
*
* An ID from external system. It is used by user list sellers to correlate
* IDs on their systems.
*
*
* optional string integration_code = 29;
* @param value The bytes for integrationCode to set.
* @return This builder for chaining.
*/
public Builder setIntegrationCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000010;
integrationCode_ = value;
onChanged();
return this;
}
private long membershipLifeSpan_ ;
/**
*
* Number of days a user's cookie stays on your list since its most recent
* addition to the list. This field must be between 0 and 540 inclusive.
* However, for CRM based userlists, this field can be set to 10000 which
* means no expiration.
* It'll be ignored for logical_user_list.
*
*
* optional int64 membership_life_span = 30;
* @return Whether the membershipLifeSpan field is set.
*/
@java.lang.Override
public boolean hasMembershipLifeSpan() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Number of days a user's cookie stays on your list since its most recent
* addition to the list. This field must be between 0 and 540 inclusive.
* However, for CRM based userlists, this field can be set to 10000 which
* means no expiration.
* It'll be ignored for logical_user_list.
*
*
* optional int64 membership_life_span = 30;
* @return The membershipLifeSpan.
*/
@java.lang.Override
public long getMembershipLifeSpan() {
return membershipLifeSpan_;
}
/**
*
* Number of days a user's cookie stays on your list since its most recent
* addition to the list. This field must be between 0 and 540 inclusive.
* However, for CRM based userlists, this field can be set to 10000 which
* means no expiration.
* It'll be ignored for logical_user_list.
*
*
* optional int64 membership_life_span = 30;
* @param value The membershipLifeSpan to set.
* @return This builder for chaining.
*/
public Builder setMembershipLifeSpan(long value) {
bitField0_ |= 0x00000020;
membershipLifeSpan_ = value;
onChanged();
return this;
}
/**
*
* Number of days a user's cookie stays on your list since its most recent
* addition to the list. This field must be between 0 and 540 inclusive.
* However, for CRM based userlists, this field can be set to 10000 which
* means no expiration.
* It'll be ignored for logical_user_list.
*
*
* optional int64 membership_life_span = 30;
* @return This builder for chaining.
*/
public Builder clearMembershipLifeSpan() {
bitField0_ = (bitField0_ & ~0x00000020);
membershipLifeSpan_ = 0L;
onChanged();
return this;
}
private long sizeForDisplay_ ;
/**
*
* Output only. Estimated number of users in this user list, on the Google Display Network.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_display = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the sizeForDisplay field is set.
*/
@java.lang.Override
public boolean hasSizeForDisplay() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. Estimated number of users in this user list, on the Google Display Network.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_display = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The sizeForDisplay.
*/
@java.lang.Override
public long getSizeForDisplay() {
return sizeForDisplay_;
}
/**
*
* Output only. Estimated number of users in this user list, on the Google Display Network.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_display = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The sizeForDisplay to set.
* @return This builder for chaining.
*/
public Builder setSizeForDisplay(long value) {
bitField0_ |= 0x00000040;
sizeForDisplay_ = value;
onChanged();
return this;
}
/**
*
* Output only. Estimated number of users in this user list, on the Google Display Network.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_display = 31 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearSizeForDisplay() {
bitField0_ = (bitField0_ & ~0x00000040);
sizeForDisplay_ = 0L;
onChanged();
return this;
}
private int sizeRangeForDisplay_ = 0;
/**
*
* Output only. Size range in terms of number of users of the UserList, on the Google
* Display Network.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_display = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for sizeRangeForDisplay.
*/
@java.lang.Override public int getSizeRangeForDisplayValue() {
return sizeRangeForDisplay_;
}
/**
*
* Output only. Size range in terms of number of users of the UserList, on the Google
* Display Network.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_display = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for sizeRangeForDisplay to set.
* @return This builder for chaining.
*/
public Builder setSizeRangeForDisplayValue(int value) {
sizeRangeForDisplay_ = value;
onChanged();
return this;
}
/**
*
* Output only. Size range in terms of number of users of the UserList, on the Google
* Display Network.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_display = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The sizeRangeForDisplay.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange getSizeRangeForDisplay() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange result = com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.valueOf(sizeRangeForDisplay_);
return result == null ? com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.UNRECOGNIZED : result;
}
/**
*
* Output only. Size range in terms of number of users of the UserList, on the Google
* Display Network.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_display = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The sizeRangeForDisplay to set.
* @return This builder for chaining.
*/
public Builder setSizeRangeForDisplay(com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange value) {
if (value == null) {
throw new NullPointerException();
}
sizeRangeForDisplay_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. Size range in terms of number of users of the UserList, on the Google
* Display Network.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_display = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearSizeRangeForDisplay() {
sizeRangeForDisplay_ = 0;
onChanged();
return this;
}
private long sizeForSearch_ ;
/**
*
* Output only. Estimated number of users in this user list in the google.com domain.
* These are the users available for targeting in Search campaigns.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_search = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the sizeForSearch field is set.
*/
@java.lang.Override
public boolean hasSizeForSearch() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Output only. Estimated number of users in this user list in the google.com domain.
* These are the users available for targeting in Search campaigns.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_search = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The sizeForSearch.
*/
@java.lang.Override
public long getSizeForSearch() {
return sizeForSearch_;
}
/**
*
* Output only. Estimated number of users in this user list in the google.com domain.
* These are the users available for targeting in Search campaigns.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_search = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The sizeForSearch to set.
* @return This builder for chaining.
*/
public Builder setSizeForSearch(long value) {
bitField0_ |= 0x00000080;
sizeForSearch_ = value;
onChanged();
return this;
}
/**
*
* Output only. Estimated number of users in this user list in the google.com domain.
* These are the users available for targeting in Search campaigns.
* This value is null if the number of users has not yet been determined.
* This field is read-only.
*
*
* optional int64 size_for_search = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearSizeForSearch() {
bitField0_ = (bitField0_ & ~0x00000080);
sizeForSearch_ = 0L;
onChanged();
return this;
}
private int sizeRangeForSearch_ = 0;
/**
*
* Output only. Size range in terms of number of users of the UserList, for Search ads.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_search = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for sizeRangeForSearch.
*/
@java.lang.Override public int getSizeRangeForSearchValue() {
return sizeRangeForSearch_;
}
/**
*
* Output only. Size range in terms of number of users of the UserList, for Search ads.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_search = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for sizeRangeForSearch to set.
* @return This builder for chaining.
*/
public Builder setSizeRangeForSearchValue(int value) {
sizeRangeForSearch_ = value;
onChanged();
return this;
}
/**
*
* Output only. Size range in terms of number of users of the UserList, for Search ads.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_search = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The sizeRangeForSearch.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange getSizeRangeForSearch() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange result = com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.valueOf(sizeRangeForSearch_);
return result == null ? com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange.UNRECOGNIZED : result;
}
/**
*
* Output only. Size range in terms of number of users of the UserList, for Search ads.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_search = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The sizeRangeForSearch to set.
* @return This builder for chaining.
*/
public Builder setSizeRangeForSearch(com.google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange value) {
if (value == null) {
throw new NullPointerException();
}
sizeRangeForSearch_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. Size range in terms of number of users of the UserList, for Search ads.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListSizeRangeEnum.UserListSizeRange size_range_for_search = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearSizeRangeForSearch() {
sizeRangeForSearch_ = 0;
onChanged();
return this;
}
private int type_ = 0;
/**
*
* Output only. Type of this list.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListTypeEnum.UserListType type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
*
* Output only. Type of this list.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListTypeEnum.UserListType type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
* Output only. Type of this list.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListTypeEnum.UserListType type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The type.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.UserListTypeEnum.UserListType getType() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListTypeEnum.UserListType result = com.google.ads.googleads.v10.enums.UserListTypeEnum.UserListType.valueOf(type_);
return result == null ? com.google.ads.googleads.v10.enums.UserListTypeEnum.UserListType.UNRECOGNIZED : result;
}
/**
*
* Output only. Type of this list.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListTypeEnum.UserListType type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(com.google.ads.googleads.v10.enums.UserListTypeEnum.UserListType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. Type of this list.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.UserListTypeEnum.UserListType type = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private int closingReason_ = 0;
/**
*
* Indicating the reason why this user list membership status is closed. It is
* only populated on lists that were automatically closed due to inactivity,
* and will be cleared once the list membership status becomes open.
*
*
* .google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason closing_reason = 14;
* @return The enum numeric value on the wire for closingReason.
*/
@java.lang.Override public int getClosingReasonValue() {
return closingReason_;
}
/**
*
* Indicating the reason why this user list membership status is closed. It is
* only populated on lists that were automatically closed due to inactivity,
* and will be cleared once the list membership status becomes open.
*
*
* .google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason closing_reason = 14;
* @param value The enum numeric value on the wire for closingReason to set.
* @return This builder for chaining.
*/
public Builder setClosingReasonValue(int value) {
closingReason_ = value;
onChanged();
return this;
}
/**
*
* Indicating the reason why this user list membership status is closed. It is
* only populated on lists that were automatically closed due to inactivity,
* and will be cleared once the list membership status becomes open.
*
*
* .google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason closing_reason = 14;
* @return The closingReason.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason getClosingReason() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason result = com.google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason.valueOf(closingReason_);
return result == null ? com.google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason.UNRECOGNIZED : result;
}
/**
*
* Indicating the reason why this user list membership status is closed. It is
* only populated on lists that were automatically closed due to inactivity,
* and will be cleared once the list membership status becomes open.
*
*
* .google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason closing_reason = 14;
* @param value The closingReason to set.
* @return This builder for chaining.
*/
public Builder setClosingReason(com.google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason value) {
if (value == null) {
throw new NullPointerException();
}
closingReason_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Indicating the reason why this user list membership status is closed. It is
* only populated on lists that were automatically closed due to inactivity,
* and will be cleared once the list membership status becomes open.
*
*
* .google.ads.googleads.v10.enums.UserListClosingReasonEnum.UserListClosingReason closing_reason = 14;
* @return This builder for chaining.
*/
public Builder clearClosingReason() {
closingReason_ = 0;
onChanged();
return this;
}
private int accessReason_ = 0;
/**
*
* Output only. Indicates the reason this account has been granted access to the list.
* The reason can be SHARED, OWNED, LICENSED or SUBSCRIBED.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason access_reason = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for accessReason.
*/
@java.lang.Override public int getAccessReasonValue() {
return accessReason_;
}
/**
*
* Output only. Indicates the reason this account has been granted access to the list.
* The reason can be SHARED, OWNED, LICENSED or SUBSCRIBED.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason access_reason = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for accessReason to set.
* @return This builder for chaining.
*/
public Builder setAccessReasonValue(int value) {
accessReason_ = value;
onChanged();
return this;
}
/**
*
* Output only. Indicates the reason this account has been granted access to the list.
* The reason can be SHARED, OWNED, LICENSED or SUBSCRIBED.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason access_reason = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The accessReason.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason getAccessReason() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason result = com.google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason.valueOf(accessReason_);
return result == null ? com.google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason.UNRECOGNIZED : result;
}
/**
*
* Output only. Indicates the reason this account has been granted access to the list.
* The reason can be SHARED, OWNED, LICENSED or SUBSCRIBED.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason access_reason = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The accessReason to set.
* @return This builder for chaining.
*/
public Builder setAccessReason(com.google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason value) {
if (value == null) {
throw new NullPointerException();
}
accessReason_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. Indicates the reason this account has been granted access to the list.
* The reason can be SHARED, OWNED, LICENSED or SUBSCRIBED.
* This field is read-only.
*
*
* .google.ads.googleads.v10.enums.AccessReasonEnum.AccessReason access_reason = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearAccessReason() {
accessReason_ = 0;
onChanged();
return this;
}
private int accountUserListStatus_ = 0;
/**
*
* Indicates if this share is still enabled. When a UserList is shared with
* the user this field is set to ENABLED. Later the userList owner can decide
* to revoke the share and make it DISABLED.
* The default value of this field is set to ENABLED.
*
*
* .google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus account_user_list_status = 16;
* @return The enum numeric value on the wire for accountUserListStatus.
*/
@java.lang.Override public int getAccountUserListStatusValue() {
return accountUserListStatus_;
}
/**
*
* Indicates if this share is still enabled. When a UserList is shared with
* the user this field is set to ENABLED. Later the userList owner can decide
* to revoke the share and make it DISABLED.
* The default value of this field is set to ENABLED.
*
*
* .google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus account_user_list_status = 16;
* @param value The enum numeric value on the wire for accountUserListStatus to set.
* @return This builder for chaining.
*/
public Builder setAccountUserListStatusValue(int value) {
accountUserListStatus_ = value;
onChanged();
return this;
}
/**
*
* Indicates if this share is still enabled. When a UserList is shared with
* the user this field is set to ENABLED. Later the userList owner can decide
* to revoke the share and make it DISABLED.
* The default value of this field is set to ENABLED.
*
*
* .google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus account_user_list_status = 16;
* @return The accountUserListStatus.
*/
@java.lang.Override
public com.google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus getAccountUserListStatus() {
@SuppressWarnings("deprecation")
com.google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus result = com.google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus.valueOf(accountUserListStatus_);
return result == null ? com.google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus.UNRECOGNIZED : result;
}
/**
*
* Indicates if this share is still enabled. When a UserList is shared with
* the user this field is set to ENABLED. Later the userList owner can decide
* to revoke the share and make it DISABLED.
* The default value of this field is set to ENABLED.
*
*
* .google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus account_user_list_status = 16;
* @param value The accountUserListStatus to set.
* @return This builder for chaining.
*/
public Builder setAccountUserListStatus(com.google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus value) {
if (value == null) {
throw new NullPointerException();
}
accountUserListStatus_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Indicates if this share is still enabled. When a UserList is shared with
* the user this field is set to ENABLED. Later the userList owner can decide
* to revoke the share and make it DISABLED.
* The default value of this field is set to ENABLED.
*
*
* .google.ads.googleads.v10.enums.UserListAccessStatusEnum.UserListAccessStatus account_user_list_status = 16;
* @return This builder for chaining.
*/
public Builder clearAccountUserListStatus() {
accountUserListStatus_ = 0;
onChanged();
return this;
}
private boolean eligibleForSearch_ ;
/**
*
* Indicates if this user list is eligible for Google Search Network.
*
*
* optional bool eligible_for_search = 33;
* @return Whether the eligibleForSearch field is set.
*/
@java.lang.Override
public boolean hasEligibleForSearch() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Indicates if this user list is eligible for Google Search Network.
*
*
* optional bool eligible_for_search = 33;
* @return The eligibleForSearch.
*/
@java.lang.Override
public boolean getEligibleForSearch() {
return eligibleForSearch_;
}
/**
*
* Indicates if this user list is eligible for Google Search Network.
*
*
* optional bool eligible_for_search = 33;
* @param value The eligibleForSearch to set.
* @return This builder for chaining.
*/
public Builder setEligibleForSearch(boolean value) {
bitField0_ |= 0x00000100;
eligibleForSearch_ = value;
onChanged();
return this;
}
/**
*
* Indicates if this user list is eligible for Google Search Network.
*
*
* optional bool eligible_for_search = 33;
* @return This builder for chaining.
*/
public Builder clearEligibleForSearch() {
bitField0_ = (bitField0_ & ~0x00000100);
eligibleForSearch_ = false;
onChanged();
return this;
}
private boolean eligibleForDisplay_ ;
/**
*
* Output only. Indicates this user list is eligible for Google Display Network.
* This field is read-only.
*
*
* optional bool eligible_for_display = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the eligibleForDisplay field is set.
*/
@java.lang.Override
public boolean hasEligibleForDisplay() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Output only. Indicates this user list is eligible for Google Display Network.
* This field is read-only.
*
*
* optional bool eligible_for_display = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The eligibleForDisplay.
*/
@java.lang.Override
public boolean getEligibleForDisplay() {
return eligibleForDisplay_;
}
/**
*
* Output only. Indicates this user list is eligible for Google Display Network.
* This field is read-only.
*
*
* optional bool eligible_for_display = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The eligibleForDisplay to set.
* @return This builder for chaining.
*/
public Builder setEligibleForDisplay(boolean value) {
bitField0_ |= 0x00000200;
eligibleForDisplay_ = value;
onChanged();
return this;
}
/**
*
* Output only. Indicates this user list is eligible for Google Display Network.
* This field is read-only.
*
*
* optional bool eligible_for_display = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearEligibleForDisplay() {
bitField0_ = (bitField0_ & ~0x00000200);
eligibleForDisplay_ = false;
onChanged();
return this;
}
private int matchRatePercentage_ ;
/**
*
* Output only. Indicates match rate for Customer Match lists. The range of this field is
* [0-100]. This will be null for other list types or when it's not possible
* to calculate the match rate.
* This field is read-only.
*
*
* optional int32 match_rate_percentage = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the matchRatePercentage field is set.
*/
@java.lang.Override
public boolean hasMatchRatePercentage() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Output only. Indicates match rate for Customer Match lists. The range of this field is
* [0-100]. This will be null for other list types or when it's not possible
* to calculate the match rate.
* This field is read-only.
*
*
* optional int32 match_rate_percentage = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The matchRatePercentage.
*/
@java.lang.Override
public int getMatchRatePercentage() {
return matchRatePercentage_;
}
/**
*
* Output only. Indicates match rate for Customer Match lists. The range of this field is
* [0-100]. This will be null for other list types or when it's not possible
* to calculate the match rate.
* This field is read-only.
*
*
* optional int32 match_rate_percentage = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The matchRatePercentage to set.
* @return This builder for chaining.
*/
public Builder setMatchRatePercentage(int value) {
bitField0_ |= 0x00000400;
matchRatePercentage_ = value;
onChanged();
return this;
}
/**
*
* Output only. Indicates match rate for Customer Match lists. The range of this field is
* [0-100]. This will be null for other list types or when it's not possible
* to calculate the match rate.
* This field is read-only.
*
*
* optional int32 match_rate_percentage = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearMatchRatePercentage() {
bitField0_ = (bitField0_ & ~0x00000400);
matchRatePercentage_ = 0;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.CrmBasedUserListInfo, com.google.ads.googleads.v10.common.CrmBasedUserListInfo.Builder, com.google.ads.googleads.v10.common.CrmBasedUserListInfoOrBuilder> crmBasedUserListBuilder_;
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
* @return Whether the crmBasedUserList field is set.
*/
@java.lang.Override
public boolean hasCrmBasedUserList() {
return userListCase_ == 19;
}
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
* @return The crmBasedUserList.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.CrmBasedUserListInfo getCrmBasedUserList() {
if (crmBasedUserListBuilder_ == null) {
if (userListCase_ == 19) {
return (com.google.ads.googleads.v10.common.CrmBasedUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.CrmBasedUserListInfo.getDefaultInstance();
} else {
if (userListCase_ == 19) {
return crmBasedUserListBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.CrmBasedUserListInfo.getDefaultInstance();
}
}
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
*/
public Builder setCrmBasedUserList(com.google.ads.googleads.v10.common.CrmBasedUserListInfo value) {
if (crmBasedUserListBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
userList_ = value;
onChanged();
} else {
crmBasedUserListBuilder_.setMessage(value);
}
userListCase_ = 19;
return this;
}
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
*/
public Builder setCrmBasedUserList(
com.google.ads.googleads.v10.common.CrmBasedUserListInfo.Builder builderForValue) {
if (crmBasedUserListBuilder_ == null) {
userList_ = builderForValue.build();
onChanged();
} else {
crmBasedUserListBuilder_.setMessage(builderForValue.build());
}
userListCase_ = 19;
return this;
}
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
*/
public Builder mergeCrmBasedUserList(com.google.ads.googleads.v10.common.CrmBasedUserListInfo value) {
if (crmBasedUserListBuilder_ == null) {
if (userListCase_ == 19 &&
userList_ != com.google.ads.googleads.v10.common.CrmBasedUserListInfo.getDefaultInstance()) {
userList_ = com.google.ads.googleads.v10.common.CrmBasedUserListInfo.newBuilder((com.google.ads.googleads.v10.common.CrmBasedUserListInfo) userList_)
.mergeFrom(value).buildPartial();
} else {
userList_ = value;
}
onChanged();
} else {
if (userListCase_ == 19) {
crmBasedUserListBuilder_.mergeFrom(value);
} else {
crmBasedUserListBuilder_.setMessage(value);
}
}
userListCase_ = 19;
return this;
}
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
*/
public Builder clearCrmBasedUserList() {
if (crmBasedUserListBuilder_ == null) {
if (userListCase_ == 19) {
userListCase_ = 0;
userList_ = null;
onChanged();
}
} else {
if (userListCase_ == 19) {
userListCase_ = 0;
userList_ = null;
}
crmBasedUserListBuilder_.clear();
}
return this;
}
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
*/
public com.google.ads.googleads.v10.common.CrmBasedUserListInfo.Builder getCrmBasedUserListBuilder() {
return getCrmBasedUserListFieldBuilder().getBuilder();
}
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.CrmBasedUserListInfoOrBuilder getCrmBasedUserListOrBuilder() {
if ((userListCase_ == 19) && (crmBasedUserListBuilder_ != null)) {
return crmBasedUserListBuilder_.getMessageOrBuilder();
} else {
if (userListCase_ == 19) {
return (com.google.ads.googleads.v10.common.CrmBasedUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.CrmBasedUserListInfo.getDefaultInstance();
}
}
/**
*
* User list of CRM users provided by the advertiser.
*
*
* .google.ads.googleads.v10.common.CrmBasedUserListInfo crm_based_user_list = 19;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.CrmBasedUserListInfo, com.google.ads.googleads.v10.common.CrmBasedUserListInfo.Builder, com.google.ads.googleads.v10.common.CrmBasedUserListInfoOrBuilder>
getCrmBasedUserListFieldBuilder() {
if (crmBasedUserListBuilder_ == null) {
if (!(userListCase_ == 19)) {
userList_ = com.google.ads.googleads.v10.common.CrmBasedUserListInfo.getDefaultInstance();
}
crmBasedUserListBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.CrmBasedUserListInfo, com.google.ads.googleads.v10.common.CrmBasedUserListInfo.Builder, com.google.ads.googleads.v10.common.CrmBasedUserListInfoOrBuilder>(
(com.google.ads.googleads.v10.common.CrmBasedUserListInfo) userList_,
getParentForChildren(),
isClean());
userList_ = null;
}
userListCase_ = 19;
onChanged();;
return crmBasedUserListBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.SimilarUserListInfo, com.google.ads.googleads.v10.common.SimilarUserListInfo.Builder, com.google.ads.googleads.v10.common.SimilarUserListInfoOrBuilder> similarUserListBuilder_;
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the similarUserList field is set.
*/
@java.lang.Override
public boolean hasSimilarUserList() {
return userListCase_ == 20;
}
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The similarUserList.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.SimilarUserListInfo getSimilarUserList() {
if (similarUserListBuilder_ == null) {
if (userListCase_ == 20) {
return (com.google.ads.googleads.v10.common.SimilarUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.SimilarUserListInfo.getDefaultInstance();
} else {
if (userListCase_ == 20) {
return similarUserListBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.SimilarUserListInfo.getDefaultInstance();
}
}
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder setSimilarUserList(com.google.ads.googleads.v10.common.SimilarUserListInfo value) {
if (similarUserListBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
userList_ = value;
onChanged();
} else {
similarUserListBuilder_.setMessage(value);
}
userListCase_ = 20;
return this;
}
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder setSimilarUserList(
com.google.ads.googleads.v10.common.SimilarUserListInfo.Builder builderForValue) {
if (similarUserListBuilder_ == null) {
userList_ = builderForValue.build();
onChanged();
} else {
similarUserListBuilder_.setMessage(builderForValue.build());
}
userListCase_ = 20;
return this;
}
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder mergeSimilarUserList(com.google.ads.googleads.v10.common.SimilarUserListInfo value) {
if (similarUserListBuilder_ == null) {
if (userListCase_ == 20 &&
userList_ != com.google.ads.googleads.v10.common.SimilarUserListInfo.getDefaultInstance()) {
userList_ = com.google.ads.googleads.v10.common.SimilarUserListInfo.newBuilder((com.google.ads.googleads.v10.common.SimilarUserListInfo) userList_)
.mergeFrom(value).buildPartial();
} else {
userList_ = value;
}
onChanged();
} else {
if (userListCase_ == 20) {
similarUserListBuilder_.mergeFrom(value);
} else {
similarUserListBuilder_.setMessage(value);
}
}
userListCase_ = 20;
return this;
}
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public Builder clearSimilarUserList() {
if (similarUserListBuilder_ == null) {
if (userListCase_ == 20) {
userListCase_ = 0;
userList_ = null;
onChanged();
}
} else {
if (userListCase_ == 20) {
userListCase_ = 0;
userList_ = null;
}
similarUserListBuilder_.clear();
}
return this;
}
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
public com.google.ads.googleads.v10.common.SimilarUserListInfo.Builder getSimilarUserListBuilder() {
return getSimilarUserListFieldBuilder().getBuilder();
}
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.SimilarUserListInfoOrBuilder getSimilarUserListOrBuilder() {
if ((userListCase_ == 20) && (similarUserListBuilder_ != null)) {
return similarUserListBuilder_.getMessageOrBuilder();
} else {
if (userListCase_ == 20) {
return (com.google.ads.googleads.v10.common.SimilarUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.SimilarUserListInfo.getDefaultInstance();
}
}
/**
*
* Output only. User list which are similar to users from another UserList.
* These lists are readonly and automatically created by google.
*
*
* .google.ads.googleads.v10.common.SimilarUserListInfo similar_user_list = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.SimilarUserListInfo, com.google.ads.googleads.v10.common.SimilarUserListInfo.Builder, com.google.ads.googleads.v10.common.SimilarUserListInfoOrBuilder>
getSimilarUserListFieldBuilder() {
if (similarUserListBuilder_ == null) {
if (!(userListCase_ == 20)) {
userList_ = com.google.ads.googleads.v10.common.SimilarUserListInfo.getDefaultInstance();
}
similarUserListBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.SimilarUserListInfo, com.google.ads.googleads.v10.common.SimilarUserListInfo.Builder, com.google.ads.googleads.v10.common.SimilarUserListInfoOrBuilder>(
(com.google.ads.googleads.v10.common.SimilarUserListInfo) userList_,
getParentForChildren(),
isClean());
userList_ = null;
}
userListCase_ = 20;
onChanged();;
return similarUserListBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.RuleBasedUserListInfo, com.google.ads.googleads.v10.common.RuleBasedUserListInfo.Builder, com.google.ads.googleads.v10.common.RuleBasedUserListInfoOrBuilder> ruleBasedUserListBuilder_;
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
* @return Whether the ruleBasedUserList field is set.
*/
@java.lang.Override
public boolean hasRuleBasedUserList() {
return userListCase_ == 21;
}
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
* @return The ruleBasedUserList.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.RuleBasedUserListInfo getRuleBasedUserList() {
if (ruleBasedUserListBuilder_ == null) {
if (userListCase_ == 21) {
return (com.google.ads.googleads.v10.common.RuleBasedUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.RuleBasedUserListInfo.getDefaultInstance();
} else {
if (userListCase_ == 21) {
return ruleBasedUserListBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.RuleBasedUserListInfo.getDefaultInstance();
}
}
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
*/
public Builder setRuleBasedUserList(com.google.ads.googleads.v10.common.RuleBasedUserListInfo value) {
if (ruleBasedUserListBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
userList_ = value;
onChanged();
} else {
ruleBasedUserListBuilder_.setMessage(value);
}
userListCase_ = 21;
return this;
}
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
*/
public Builder setRuleBasedUserList(
com.google.ads.googleads.v10.common.RuleBasedUserListInfo.Builder builderForValue) {
if (ruleBasedUserListBuilder_ == null) {
userList_ = builderForValue.build();
onChanged();
} else {
ruleBasedUserListBuilder_.setMessage(builderForValue.build());
}
userListCase_ = 21;
return this;
}
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
*/
public Builder mergeRuleBasedUserList(com.google.ads.googleads.v10.common.RuleBasedUserListInfo value) {
if (ruleBasedUserListBuilder_ == null) {
if (userListCase_ == 21 &&
userList_ != com.google.ads.googleads.v10.common.RuleBasedUserListInfo.getDefaultInstance()) {
userList_ = com.google.ads.googleads.v10.common.RuleBasedUserListInfo.newBuilder((com.google.ads.googleads.v10.common.RuleBasedUserListInfo) userList_)
.mergeFrom(value).buildPartial();
} else {
userList_ = value;
}
onChanged();
} else {
if (userListCase_ == 21) {
ruleBasedUserListBuilder_.mergeFrom(value);
} else {
ruleBasedUserListBuilder_.setMessage(value);
}
}
userListCase_ = 21;
return this;
}
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
*/
public Builder clearRuleBasedUserList() {
if (ruleBasedUserListBuilder_ == null) {
if (userListCase_ == 21) {
userListCase_ = 0;
userList_ = null;
onChanged();
}
} else {
if (userListCase_ == 21) {
userListCase_ = 0;
userList_ = null;
}
ruleBasedUserListBuilder_.clear();
}
return this;
}
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
*/
public com.google.ads.googleads.v10.common.RuleBasedUserListInfo.Builder getRuleBasedUserListBuilder() {
return getRuleBasedUserListFieldBuilder().getBuilder();
}
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.RuleBasedUserListInfoOrBuilder getRuleBasedUserListOrBuilder() {
if ((userListCase_ == 21) && (ruleBasedUserListBuilder_ != null)) {
return ruleBasedUserListBuilder_.getMessageOrBuilder();
} else {
if (userListCase_ == 21) {
return (com.google.ads.googleads.v10.common.RuleBasedUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.RuleBasedUserListInfo.getDefaultInstance();
}
}
/**
*
* User list generated by a rule.
*
*
* .google.ads.googleads.v10.common.RuleBasedUserListInfo rule_based_user_list = 21;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.RuleBasedUserListInfo, com.google.ads.googleads.v10.common.RuleBasedUserListInfo.Builder, com.google.ads.googleads.v10.common.RuleBasedUserListInfoOrBuilder>
getRuleBasedUserListFieldBuilder() {
if (ruleBasedUserListBuilder_ == null) {
if (!(userListCase_ == 21)) {
userList_ = com.google.ads.googleads.v10.common.RuleBasedUserListInfo.getDefaultInstance();
}
ruleBasedUserListBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.RuleBasedUserListInfo, com.google.ads.googleads.v10.common.RuleBasedUserListInfo.Builder, com.google.ads.googleads.v10.common.RuleBasedUserListInfoOrBuilder>(
(com.google.ads.googleads.v10.common.RuleBasedUserListInfo) userList_,
getParentForChildren(),
isClean());
userList_ = null;
}
userListCase_ = 21;
onChanged();;
return ruleBasedUserListBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.LogicalUserListInfo, com.google.ads.googleads.v10.common.LogicalUserListInfo.Builder, com.google.ads.googleads.v10.common.LogicalUserListInfoOrBuilder> logicalUserListBuilder_;
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
* @return Whether the logicalUserList field is set.
*/
@java.lang.Override
public boolean hasLogicalUserList() {
return userListCase_ == 22;
}
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
* @return The logicalUserList.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.LogicalUserListInfo getLogicalUserList() {
if (logicalUserListBuilder_ == null) {
if (userListCase_ == 22) {
return (com.google.ads.googleads.v10.common.LogicalUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.LogicalUserListInfo.getDefaultInstance();
} else {
if (userListCase_ == 22) {
return logicalUserListBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.LogicalUserListInfo.getDefaultInstance();
}
}
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
*/
public Builder setLogicalUserList(com.google.ads.googleads.v10.common.LogicalUserListInfo value) {
if (logicalUserListBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
userList_ = value;
onChanged();
} else {
logicalUserListBuilder_.setMessage(value);
}
userListCase_ = 22;
return this;
}
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
*/
public Builder setLogicalUserList(
com.google.ads.googleads.v10.common.LogicalUserListInfo.Builder builderForValue) {
if (logicalUserListBuilder_ == null) {
userList_ = builderForValue.build();
onChanged();
} else {
logicalUserListBuilder_.setMessage(builderForValue.build());
}
userListCase_ = 22;
return this;
}
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
*/
public Builder mergeLogicalUserList(com.google.ads.googleads.v10.common.LogicalUserListInfo value) {
if (logicalUserListBuilder_ == null) {
if (userListCase_ == 22 &&
userList_ != com.google.ads.googleads.v10.common.LogicalUserListInfo.getDefaultInstance()) {
userList_ = com.google.ads.googleads.v10.common.LogicalUserListInfo.newBuilder((com.google.ads.googleads.v10.common.LogicalUserListInfo) userList_)
.mergeFrom(value).buildPartial();
} else {
userList_ = value;
}
onChanged();
} else {
if (userListCase_ == 22) {
logicalUserListBuilder_.mergeFrom(value);
} else {
logicalUserListBuilder_.setMessage(value);
}
}
userListCase_ = 22;
return this;
}
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
*/
public Builder clearLogicalUserList() {
if (logicalUserListBuilder_ == null) {
if (userListCase_ == 22) {
userListCase_ = 0;
userList_ = null;
onChanged();
}
} else {
if (userListCase_ == 22) {
userListCase_ = 0;
userList_ = null;
}
logicalUserListBuilder_.clear();
}
return this;
}
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
*/
public com.google.ads.googleads.v10.common.LogicalUserListInfo.Builder getLogicalUserListBuilder() {
return getLogicalUserListFieldBuilder().getBuilder();
}
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.LogicalUserListInfoOrBuilder getLogicalUserListOrBuilder() {
if ((userListCase_ == 22) && (logicalUserListBuilder_ != null)) {
return logicalUserListBuilder_.getMessageOrBuilder();
} else {
if (userListCase_ == 22) {
return (com.google.ads.googleads.v10.common.LogicalUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.LogicalUserListInfo.getDefaultInstance();
}
}
/**
*
* User list that is a custom combination of user lists and user interests.
*
*
* .google.ads.googleads.v10.common.LogicalUserListInfo logical_user_list = 22;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.LogicalUserListInfo, com.google.ads.googleads.v10.common.LogicalUserListInfo.Builder, com.google.ads.googleads.v10.common.LogicalUserListInfoOrBuilder>
getLogicalUserListFieldBuilder() {
if (logicalUserListBuilder_ == null) {
if (!(userListCase_ == 22)) {
userList_ = com.google.ads.googleads.v10.common.LogicalUserListInfo.getDefaultInstance();
}
logicalUserListBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.LogicalUserListInfo, com.google.ads.googleads.v10.common.LogicalUserListInfo.Builder, com.google.ads.googleads.v10.common.LogicalUserListInfoOrBuilder>(
(com.google.ads.googleads.v10.common.LogicalUserListInfo) userList_,
getParentForChildren(),
isClean());
userList_ = null;
}
userListCase_ = 22;
onChanged();;
return logicalUserListBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.BasicUserListInfo, com.google.ads.googleads.v10.common.BasicUserListInfo.Builder, com.google.ads.googleads.v10.common.BasicUserListInfoOrBuilder> basicUserListBuilder_;
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
* @return Whether the basicUserList field is set.
*/
@java.lang.Override
public boolean hasBasicUserList() {
return userListCase_ == 23;
}
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
* @return The basicUserList.
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.BasicUserListInfo getBasicUserList() {
if (basicUserListBuilder_ == null) {
if (userListCase_ == 23) {
return (com.google.ads.googleads.v10.common.BasicUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.BasicUserListInfo.getDefaultInstance();
} else {
if (userListCase_ == 23) {
return basicUserListBuilder_.getMessage();
}
return com.google.ads.googleads.v10.common.BasicUserListInfo.getDefaultInstance();
}
}
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
*/
public Builder setBasicUserList(com.google.ads.googleads.v10.common.BasicUserListInfo value) {
if (basicUserListBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
userList_ = value;
onChanged();
} else {
basicUserListBuilder_.setMessage(value);
}
userListCase_ = 23;
return this;
}
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
*/
public Builder setBasicUserList(
com.google.ads.googleads.v10.common.BasicUserListInfo.Builder builderForValue) {
if (basicUserListBuilder_ == null) {
userList_ = builderForValue.build();
onChanged();
} else {
basicUserListBuilder_.setMessage(builderForValue.build());
}
userListCase_ = 23;
return this;
}
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
*/
public Builder mergeBasicUserList(com.google.ads.googleads.v10.common.BasicUserListInfo value) {
if (basicUserListBuilder_ == null) {
if (userListCase_ == 23 &&
userList_ != com.google.ads.googleads.v10.common.BasicUserListInfo.getDefaultInstance()) {
userList_ = com.google.ads.googleads.v10.common.BasicUserListInfo.newBuilder((com.google.ads.googleads.v10.common.BasicUserListInfo) userList_)
.mergeFrom(value).buildPartial();
} else {
userList_ = value;
}
onChanged();
} else {
if (userListCase_ == 23) {
basicUserListBuilder_.mergeFrom(value);
} else {
basicUserListBuilder_.setMessage(value);
}
}
userListCase_ = 23;
return this;
}
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
*/
public Builder clearBasicUserList() {
if (basicUserListBuilder_ == null) {
if (userListCase_ == 23) {
userListCase_ = 0;
userList_ = null;
onChanged();
}
} else {
if (userListCase_ == 23) {
userListCase_ = 0;
userList_ = null;
}
basicUserListBuilder_.clear();
}
return this;
}
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
*/
public com.google.ads.googleads.v10.common.BasicUserListInfo.Builder getBasicUserListBuilder() {
return getBasicUserListFieldBuilder().getBuilder();
}
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
*/
@java.lang.Override
public com.google.ads.googleads.v10.common.BasicUserListInfoOrBuilder getBasicUserListOrBuilder() {
if ((userListCase_ == 23) && (basicUserListBuilder_ != null)) {
return basicUserListBuilder_.getMessageOrBuilder();
} else {
if (userListCase_ == 23) {
return (com.google.ads.googleads.v10.common.BasicUserListInfo) userList_;
}
return com.google.ads.googleads.v10.common.BasicUserListInfo.getDefaultInstance();
}
}
/**
*
* User list targeting as a collection of conversion or remarketing actions.
*
*
* .google.ads.googleads.v10.common.BasicUserListInfo basic_user_list = 23;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.BasicUserListInfo, com.google.ads.googleads.v10.common.BasicUserListInfo.Builder, com.google.ads.googleads.v10.common.BasicUserListInfoOrBuilder>
getBasicUserListFieldBuilder() {
if (basicUserListBuilder_ == null) {
if (!(userListCase_ == 23)) {
userList_ = com.google.ads.googleads.v10.common.BasicUserListInfo.getDefaultInstance();
}
basicUserListBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v10.common.BasicUserListInfo, com.google.ads.googleads.v10.common.BasicUserListInfo.Builder, com.google.ads.googleads.v10.common.BasicUserListInfoOrBuilder>(
(com.google.ads.googleads.v10.common.BasicUserListInfo) userList_,
getParentForChildren(),
isClean());
userList_ = null;
}
userListCase_ = 23;
onChanged();;
return basicUserListBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v10.resources.UserList)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v10.resources.UserList)
private static final com.google.ads.googleads.v10.resources.UserList DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v10.resources.UserList();
}
public static com.google.ads.googleads.v10.resources.UserList getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UserList parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v10.resources.UserList getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy