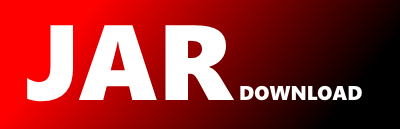
com.google.ads.googleads.v17.common.ImageAdInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v17 Show documentation
Show all versions of google-ads-stubs-v17 Show documentation
Stubs for GAAPI version google-ads-stubs-v17
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v17/common/ad_type_infos.proto
// Protobuf Java Version: 3.25.3
package com.google.ads.googleads.v17.common;
/**
*
* An image ad.
*
*
* Protobuf type {@code google.ads.googleads.v17.common.ImageAdInfo}
*/
public final class ImageAdInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v17.common.ImageAdInfo)
ImageAdInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ImageAdInfo.newBuilder() to construct.
private ImageAdInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ImageAdInfo() {
imageUrl_ = "";
previewImageUrl_ = "";
mimeType_ = 0;
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ImageAdInfo();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.common.AdTypeInfosProto.internal_static_google_ads_googleads_v17_common_ImageAdInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.common.AdTypeInfosProto.internal_static_google_ads_googleads_v17_common_ImageAdInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.common.ImageAdInfo.class, com.google.ads.googleads.v17.common.ImageAdInfo.Builder.class);
}
private int bitField0_;
private int imageCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object image_;
public enum ImageCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
IMAGE_ASSET(22),
DATA(13),
AD_ID_TO_COPY_IMAGE_FROM(14),
IMAGE_NOT_SET(0);
private final int value;
private ImageCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ImageCase valueOf(int value) {
return forNumber(value);
}
public static ImageCase forNumber(int value) {
switch (value) {
case 22: return IMAGE_ASSET;
case 13: return DATA;
case 14: return AD_ID_TO_COPY_IMAGE_FROM;
case 0: return IMAGE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ImageCase
getImageCase() {
return ImageCase.forNumber(
imageCase_);
}
public static final int PIXEL_WIDTH_FIELD_NUMBER = 15;
private long pixelWidth_ = 0L;
/**
*
* Width in pixels of the full size image.
*
*
* optional int64 pixel_width = 15;
* @return Whether the pixelWidth field is set.
*/
@java.lang.Override
public boolean hasPixelWidth() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Width in pixels of the full size image.
*
*
* optional int64 pixel_width = 15;
* @return The pixelWidth.
*/
@java.lang.Override
public long getPixelWidth() {
return pixelWidth_;
}
public static final int PIXEL_HEIGHT_FIELD_NUMBER = 16;
private long pixelHeight_ = 0L;
/**
*
* Height in pixels of the full size image.
*
*
* optional int64 pixel_height = 16;
* @return Whether the pixelHeight field is set.
*/
@java.lang.Override
public boolean hasPixelHeight() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Height in pixels of the full size image.
*
*
* optional int64 pixel_height = 16;
* @return The pixelHeight.
*/
@java.lang.Override
public long getPixelHeight() {
return pixelHeight_;
}
public static final int IMAGE_URL_FIELD_NUMBER = 17;
@SuppressWarnings("serial")
private volatile java.lang.Object imageUrl_ = "";
/**
*
* URL of the full size image.
*
*
* optional string image_url = 17;
* @return Whether the imageUrl field is set.
*/
@java.lang.Override
public boolean hasImageUrl() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* URL of the full size image.
*
*
* optional string image_url = 17;
* @return The imageUrl.
*/
@java.lang.Override
public java.lang.String getImageUrl() {
java.lang.Object ref = imageUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
imageUrl_ = s;
return s;
}
}
/**
*
* URL of the full size image.
*
*
* optional string image_url = 17;
* @return The bytes for imageUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getImageUrlBytes() {
java.lang.Object ref = imageUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
imageUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PREVIEW_PIXEL_WIDTH_FIELD_NUMBER = 18;
private long previewPixelWidth_ = 0L;
/**
*
* Width in pixels of the preview size image.
*
*
* optional int64 preview_pixel_width = 18;
* @return Whether the previewPixelWidth field is set.
*/
@java.lang.Override
public boolean hasPreviewPixelWidth() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Width in pixels of the preview size image.
*
*
* optional int64 preview_pixel_width = 18;
* @return The previewPixelWidth.
*/
@java.lang.Override
public long getPreviewPixelWidth() {
return previewPixelWidth_;
}
public static final int PREVIEW_PIXEL_HEIGHT_FIELD_NUMBER = 19;
private long previewPixelHeight_ = 0L;
/**
*
* Height in pixels of the preview size image.
*
*
* optional int64 preview_pixel_height = 19;
* @return Whether the previewPixelHeight field is set.
*/
@java.lang.Override
public boolean hasPreviewPixelHeight() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Height in pixels of the preview size image.
*
*
* optional int64 preview_pixel_height = 19;
* @return The previewPixelHeight.
*/
@java.lang.Override
public long getPreviewPixelHeight() {
return previewPixelHeight_;
}
public static final int PREVIEW_IMAGE_URL_FIELD_NUMBER = 20;
@SuppressWarnings("serial")
private volatile java.lang.Object previewImageUrl_ = "";
/**
*
* URL of the preview size image.
*
*
* optional string preview_image_url = 20;
* @return Whether the previewImageUrl field is set.
*/
@java.lang.Override
public boolean hasPreviewImageUrl() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* URL of the preview size image.
*
*
* optional string preview_image_url = 20;
* @return The previewImageUrl.
*/
@java.lang.Override
public java.lang.String getPreviewImageUrl() {
java.lang.Object ref = previewImageUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
previewImageUrl_ = s;
return s;
}
}
/**
*
* URL of the preview size image.
*
*
* optional string preview_image_url = 20;
* @return The bytes for previewImageUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPreviewImageUrlBytes() {
java.lang.Object ref = previewImageUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
previewImageUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MIME_TYPE_FIELD_NUMBER = 10;
private int mimeType_ = 0;
/**
*
* The mime type of the image.
*
*
* .google.ads.googleads.v17.enums.MimeTypeEnum.MimeType mime_type = 10;
* @return The enum numeric value on the wire for mimeType.
*/
@java.lang.Override public int getMimeTypeValue() {
return mimeType_;
}
/**
*
* The mime type of the image.
*
*
* .google.ads.googleads.v17.enums.MimeTypeEnum.MimeType mime_type = 10;
* @return The mimeType.
*/
@java.lang.Override public com.google.ads.googleads.v17.enums.MimeTypeEnum.MimeType getMimeType() {
com.google.ads.googleads.v17.enums.MimeTypeEnum.MimeType result = com.google.ads.googleads.v17.enums.MimeTypeEnum.MimeType.forNumber(mimeType_);
return result == null ? com.google.ads.googleads.v17.enums.MimeTypeEnum.MimeType.UNRECOGNIZED : result;
}
public static final int NAME_FIELD_NUMBER = 21;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
* The name of the image. If the image was created from a MediaFile, this is
* the MediaFile's name. If the image was created from bytes, this is empty.
*
*
* optional string name = 21;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* The name of the image. If the image was created from a MediaFile, this is
* the MediaFile's name. If the image was created from bytes, this is empty.
*
*
* optional string name = 21;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* The name of the image. If the image was created from a MediaFile, this is
* the MediaFile's name. If the image was created from bytes, this is empty.
*
*
* optional string name = 21;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IMAGE_ASSET_FIELD_NUMBER = 22;
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
* @return Whether the imageAsset field is set.
*/
@java.lang.Override
public boolean hasImageAsset() {
return imageCase_ == 22;
}
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
* @return The imageAsset.
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.AdImageAsset getImageAsset() {
if (imageCase_ == 22) {
return (com.google.ads.googleads.v17.common.AdImageAsset) image_;
}
return com.google.ads.googleads.v17.common.AdImageAsset.getDefaultInstance();
}
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.AdImageAssetOrBuilder getImageAssetOrBuilder() {
if (imageCase_ == 22) {
return (com.google.ads.googleads.v17.common.AdImageAsset) image_;
}
return com.google.ads.googleads.v17.common.AdImageAsset.getDefaultInstance();
}
public static final int DATA_FIELD_NUMBER = 13;
/**
*
* Raw image data as bytes.
*
*
* bytes data = 13;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return imageCase_ == 13;
}
/**
*
* Raw image data as bytes.
*
*
* bytes data = 13;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
if (imageCase_ == 13) {
return (com.google.protobuf.ByteString) image_;
}
return com.google.protobuf.ByteString.EMPTY;
}
public static final int AD_ID_TO_COPY_IMAGE_FROM_FIELD_NUMBER = 14;
/**
*
* An ad ID to copy the image from.
*
*
* int64 ad_id_to_copy_image_from = 14;
* @return Whether the adIdToCopyImageFrom field is set.
*/
@java.lang.Override
public boolean hasAdIdToCopyImageFrom() {
return imageCase_ == 14;
}
/**
*
* An ad ID to copy the image from.
*
*
* int64 ad_id_to_copy_image_from = 14;
* @return The adIdToCopyImageFrom.
*/
@java.lang.Override
public long getAdIdToCopyImageFrom() {
if (imageCase_ == 14) {
return (java.lang.Long) image_;
}
return 0L;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (mimeType_ != com.google.ads.googleads.v17.enums.MimeTypeEnum.MimeType.UNSPECIFIED.getNumber()) {
output.writeEnum(10, mimeType_);
}
if (imageCase_ == 13) {
output.writeBytes(
13, (com.google.protobuf.ByteString) image_);
}
if (imageCase_ == 14) {
output.writeInt64(
14, (long)((java.lang.Long) image_));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(15, pixelWidth_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(16, pixelHeight_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, imageUrl_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt64(18, previewPixelWidth_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt64(19, previewPixelHeight_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, previewImageUrl_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 21, name_);
}
if (imageCase_ == 22) {
output.writeMessage(22, (com.google.ads.googleads.v17.common.AdImageAsset) image_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (mimeType_ != com.google.ads.googleads.v17.enums.MimeTypeEnum.MimeType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, mimeType_);
}
if (imageCase_ == 13) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(
13, (com.google.protobuf.ByteString) image_);
}
if (imageCase_ == 14) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(
14, (long)((java.lang.Long) image_));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(15, pixelWidth_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(16, pixelHeight_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, imageUrl_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(18, previewPixelWidth_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(19, previewPixelHeight_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(20, previewImageUrl_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(21, name_);
}
if (imageCase_ == 22) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(22, (com.google.ads.googleads.v17.common.AdImageAsset) image_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v17.common.ImageAdInfo)) {
return super.equals(obj);
}
com.google.ads.googleads.v17.common.ImageAdInfo other = (com.google.ads.googleads.v17.common.ImageAdInfo) obj;
if (hasPixelWidth() != other.hasPixelWidth()) return false;
if (hasPixelWidth()) {
if (getPixelWidth()
!= other.getPixelWidth()) return false;
}
if (hasPixelHeight() != other.hasPixelHeight()) return false;
if (hasPixelHeight()) {
if (getPixelHeight()
!= other.getPixelHeight()) return false;
}
if (hasImageUrl() != other.hasImageUrl()) return false;
if (hasImageUrl()) {
if (!getImageUrl()
.equals(other.getImageUrl())) return false;
}
if (hasPreviewPixelWidth() != other.hasPreviewPixelWidth()) return false;
if (hasPreviewPixelWidth()) {
if (getPreviewPixelWidth()
!= other.getPreviewPixelWidth()) return false;
}
if (hasPreviewPixelHeight() != other.hasPreviewPixelHeight()) return false;
if (hasPreviewPixelHeight()) {
if (getPreviewPixelHeight()
!= other.getPreviewPixelHeight()) return false;
}
if (hasPreviewImageUrl() != other.hasPreviewImageUrl()) return false;
if (hasPreviewImageUrl()) {
if (!getPreviewImageUrl()
.equals(other.getPreviewImageUrl())) return false;
}
if (mimeType_ != other.mimeType_) return false;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!getImageCase().equals(other.getImageCase())) return false;
switch (imageCase_) {
case 22:
if (!getImageAsset()
.equals(other.getImageAsset())) return false;
break;
case 13:
if (!getData()
.equals(other.getData())) return false;
break;
case 14:
if (getAdIdToCopyImageFrom()
!= other.getAdIdToCopyImageFrom()) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPixelWidth()) {
hash = (37 * hash) + PIXEL_WIDTH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPixelWidth());
}
if (hasPixelHeight()) {
hash = (37 * hash) + PIXEL_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPixelHeight());
}
if (hasImageUrl()) {
hash = (37 * hash) + IMAGE_URL_FIELD_NUMBER;
hash = (53 * hash) + getImageUrl().hashCode();
}
if (hasPreviewPixelWidth()) {
hash = (37 * hash) + PREVIEW_PIXEL_WIDTH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPreviewPixelWidth());
}
if (hasPreviewPixelHeight()) {
hash = (37 * hash) + PREVIEW_PIXEL_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPreviewPixelHeight());
}
if (hasPreviewImageUrl()) {
hash = (37 * hash) + PREVIEW_IMAGE_URL_FIELD_NUMBER;
hash = (53 * hash) + getPreviewImageUrl().hashCode();
}
hash = (37 * hash) + MIME_TYPE_FIELD_NUMBER;
hash = (53 * hash) + mimeType_;
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
switch (imageCase_) {
case 22:
hash = (37 * hash) + IMAGE_ASSET_FIELD_NUMBER;
hash = (53 * hash) + getImageAsset().hashCode();
break;
case 13:
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
break;
case 14:
hash = (37 * hash) + AD_ID_TO_COPY_IMAGE_FROM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAdIdToCopyImageFrom());
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.common.ImageAdInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v17.common.ImageAdInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An image ad.
*
*
* Protobuf type {@code google.ads.googleads.v17.common.ImageAdInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v17.common.ImageAdInfo)
com.google.ads.googleads.v17.common.ImageAdInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.common.AdTypeInfosProto.internal_static_google_ads_googleads_v17_common_ImageAdInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.common.AdTypeInfosProto.internal_static_google_ads_googleads_v17_common_ImageAdInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.common.ImageAdInfo.class, com.google.ads.googleads.v17.common.ImageAdInfo.Builder.class);
}
// Construct using com.google.ads.googleads.v17.common.ImageAdInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
pixelWidth_ = 0L;
pixelHeight_ = 0L;
imageUrl_ = "";
previewPixelWidth_ = 0L;
previewPixelHeight_ = 0L;
previewImageUrl_ = "";
mimeType_ = 0;
name_ = "";
if (imageAssetBuilder_ != null) {
imageAssetBuilder_.clear();
}
imageCase_ = 0;
image_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v17.common.AdTypeInfosProto.internal_static_google_ads_googleads_v17_common_ImageAdInfo_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v17.common.ImageAdInfo getDefaultInstanceForType() {
return com.google.ads.googleads.v17.common.ImageAdInfo.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v17.common.ImageAdInfo build() {
com.google.ads.googleads.v17.common.ImageAdInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v17.common.ImageAdInfo buildPartial() {
com.google.ads.googleads.v17.common.ImageAdInfo result = new com.google.ads.googleads.v17.common.ImageAdInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.google.ads.googleads.v17.common.ImageAdInfo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.pixelWidth_ = pixelWidth_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.pixelHeight_ = pixelHeight_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.imageUrl_ = imageUrl_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.previewPixelWidth_ = previewPixelWidth_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.previewPixelHeight_ = previewPixelHeight_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.previewImageUrl_ = previewImageUrl_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.mimeType_ = mimeType_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000040;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartialOneofs(com.google.ads.googleads.v17.common.ImageAdInfo result) {
result.imageCase_ = imageCase_;
result.image_ = this.image_;
if (imageCase_ == 22 &&
imageAssetBuilder_ != null) {
result.image_ = imageAssetBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v17.common.ImageAdInfo) {
return mergeFrom((com.google.ads.googleads.v17.common.ImageAdInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v17.common.ImageAdInfo other) {
if (other == com.google.ads.googleads.v17.common.ImageAdInfo.getDefaultInstance()) return this;
if (other.hasPixelWidth()) {
setPixelWidth(other.getPixelWidth());
}
if (other.hasPixelHeight()) {
setPixelHeight(other.getPixelHeight());
}
if (other.hasImageUrl()) {
imageUrl_ = other.imageUrl_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasPreviewPixelWidth()) {
setPreviewPixelWidth(other.getPreviewPixelWidth());
}
if (other.hasPreviewPixelHeight()) {
setPreviewPixelHeight(other.getPreviewPixelHeight());
}
if (other.hasPreviewImageUrl()) {
previewImageUrl_ = other.previewImageUrl_;
bitField0_ |= 0x00000020;
onChanged();
}
if (other.mimeType_ != 0) {
setMimeTypeValue(other.getMimeTypeValue());
}
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000080;
onChanged();
}
switch (other.getImageCase()) {
case IMAGE_ASSET: {
mergeImageAsset(other.getImageAsset());
break;
}
case DATA: {
setData(other.getData());
break;
}
case AD_ID_TO_COPY_IMAGE_FROM: {
setAdIdToCopyImageFrom(other.getAdIdToCopyImageFrom());
break;
}
case IMAGE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 80: {
mimeType_ = input.readEnum();
bitField0_ |= 0x00000040;
break;
} // case 80
case 106: {
image_ = input.readBytes();
imageCase_ = 13;
break;
} // case 106
case 112: {
image_ = input.readInt64();
imageCase_ = 14;
break;
} // case 112
case 120: {
pixelWidth_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 120
case 128: {
pixelHeight_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 128
case 138: {
imageUrl_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 138
case 144: {
previewPixelWidth_ = input.readInt64();
bitField0_ |= 0x00000008;
break;
} // case 144
case 152: {
previewPixelHeight_ = input.readInt64();
bitField0_ |= 0x00000010;
break;
} // case 152
case 162: {
previewImageUrl_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 162
case 170: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 170
case 178: {
input.readMessage(
getImageAssetFieldBuilder().getBuilder(),
extensionRegistry);
imageCase_ = 22;
break;
} // case 178
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int imageCase_ = 0;
private java.lang.Object image_;
public ImageCase
getImageCase() {
return ImageCase.forNumber(
imageCase_);
}
public Builder clearImage() {
imageCase_ = 0;
image_ = null;
onChanged();
return this;
}
private int bitField0_;
private long pixelWidth_ ;
/**
*
* Width in pixels of the full size image.
*
*
* optional int64 pixel_width = 15;
* @return Whether the pixelWidth field is set.
*/
@java.lang.Override
public boolean hasPixelWidth() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Width in pixels of the full size image.
*
*
* optional int64 pixel_width = 15;
* @return The pixelWidth.
*/
@java.lang.Override
public long getPixelWidth() {
return pixelWidth_;
}
/**
*
* Width in pixels of the full size image.
*
*
* optional int64 pixel_width = 15;
* @param value The pixelWidth to set.
* @return This builder for chaining.
*/
public Builder setPixelWidth(long value) {
pixelWidth_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Width in pixels of the full size image.
*
*
* optional int64 pixel_width = 15;
* @return This builder for chaining.
*/
public Builder clearPixelWidth() {
bitField0_ = (bitField0_ & ~0x00000001);
pixelWidth_ = 0L;
onChanged();
return this;
}
private long pixelHeight_ ;
/**
*
* Height in pixels of the full size image.
*
*
* optional int64 pixel_height = 16;
* @return Whether the pixelHeight field is set.
*/
@java.lang.Override
public boolean hasPixelHeight() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Height in pixels of the full size image.
*
*
* optional int64 pixel_height = 16;
* @return The pixelHeight.
*/
@java.lang.Override
public long getPixelHeight() {
return pixelHeight_;
}
/**
*
* Height in pixels of the full size image.
*
*
* optional int64 pixel_height = 16;
* @param value The pixelHeight to set.
* @return This builder for chaining.
*/
public Builder setPixelHeight(long value) {
pixelHeight_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Height in pixels of the full size image.
*
*
* optional int64 pixel_height = 16;
* @return This builder for chaining.
*/
public Builder clearPixelHeight() {
bitField0_ = (bitField0_ & ~0x00000002);
pixelHeight_ = 0L;
onChanged();
return this;
}
private java.lang.Object imageUrl_ = "";
/**
*
* URL of the full size image.
*
*
* optional string image_url = 17;
* @return Whether the imageUrl field is set.
*/
public boolean hasImageUrl() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* URL of the full size image.
*
*
* optional string image_url = 17;
* @return The imageUrl.
*/
public java.lang.String getImageUrl() {
java.lang.Object ref = imageUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
imageUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* URL of the full size image.
*
*
* optional string image_url = 17;
* @return The bytes for imageUrl.
*/
public com.google.protobuf.ByteString
getImageUrlBytes() {
java.lang.Object ref = imageUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
imageUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* URL of the full size image.
*
*
* optional string image_url = 17;
* @param value The imageUrl to set.
* @return This builder for chaining.
*/
public Builder setImageUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
imageUrl_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* URL of the full size image.
*
*
* optional string image_url = 17;
* @return This builder for chaining.
*/
public Builder clearImageUrl() {
imageUrl_ = getDefaultInstance().getImageUrl();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* URL of the full size image.
*
*
* optional string image_url = 17;
* @param value The bytes for imageUrl to set.
* @return This builder for chaining.
*/
public Builder setImageUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
imageUrl_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private long previewPixelWidth_ ;
/**
*
* Width in pixels of the preview size image.
*
*
* optional int64 preview_pixel_width = 18;
* @return Whether the previewPixelWidth field is set.
*/
@java.lang.Override
public boolean hasPreviewPixelWidth() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Width in pixels of the preview size image.
*
*
* optional int64 preview_pixel_width = 18;
* @return The previewPixelWidth.
*/
@java.lang.Override
public long getPreviewPixelWidth() {
return previewPixelWidth_;
}
/**
*
* Width in pixels of the preview size image.
*
*
* optional int64 preview_pixel_width = 18;
* @param value The previewPixelWidth to set.
* @return This builder for chaining.
*/
public Builder setPreviewPixelWidth(long value) {
previewPixelWidth_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Width in pixels of the preview size image.
*
*
* optional int64 preview_pixel_width = 18;
* @return This builder for chaining.
*/
public Builder clearPreviewPixelWidth() {
bitField0_ = (bitField0_ & ~0x00000008);
previewPixelWidth_ = 0L;
onChanged();
return this;
}
private long previewPixelHeight_ ;
/**
*
* Height in pixels of the preview size image.
*
*
* optional int64 preview_pixel_height = 19;
* @return Whether the previewPixelHeight field is set.
*/
@java.lang.Override
public boolean hasPreviewPixelHeight() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Height in pixels of the preview size image.
*
*
* optional int64 preview_pixel_height = 19;
* @return The previewPixelHeight.
*/
@java.lang.Override
public long getPreviewPixelHeight() {
return previewPixelHeight_;
}
/**
*
* Height in pixels of the preview size image.
*
*
* optional int64 preview_pixel_height = 19;
* @param value The previewPixelHeight to set.
* @return This builder for chaining.
*/
public Builder setPreviewPixelHeight(long value) {
previewPixelHeight_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Height in pixels of the preview size image.
*
*
* optional int64 preview_pixel_height = 19;
* @return This builder for chaining.
*/
public Builder clearPreviewPixelHeight() {
bitField0_ = (bitField0_ & ~0x00000010);
previewPixelHeight_ = 0L;
onChanged();
return this;
}
private java.lang.Object previewImageUrl_ = "";
/**
*
* URL of the preview size image.
*
*
* optional string preview_image_url = 20;
* @return Whether the previewImageUrl field is set.
*/
public boolean hasPreviewImageUrl() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* URL of the preview size image.
*
*
* optional string preview_image_url = 20;
* @return The previewImageUrl.
*/
public java.lang.String getPreviewImageUrl() {
java.lang.Object ref = previewImageUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
previewImageUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* URL of the preview size image.
*
*
* optional string preview_image_url = 20;
* @return The bytes for previewImageUrl.
*/
public com.google.protobuf.ByteString
getPreviewImageUrlBytes() {
java.lang.Object ref = previewImageUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
previewImageUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* URL of the preview size image.
*
*
* optional string preview_image_url = 20;
* @param value The previewImageUrl to set.
* @return This builder for chaining.
*/
public Builder setPreviewImageUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
previewImageUrl_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* URL of the preview size image.
*
*
* optional string preview_image_url = 20;
* @return This builder for chaining.
*/
public Builder clearPreviewImageUrl() {
previewImageUrl_ = getDefaultInstance().getPreviewImageUrl();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
* URL of the preview size image.
*
*
* optional string preview_image_url = 20;
* @param value The bytes for previewImageUrl to set.
* @return This builder for chaining.
*/
public Builder setPreviewImageUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
previewImageUrl_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private int mimeType_ = 0;
/**
*
* The mime type of the image.
*
*
* .google.ads.googleads.v17.enums.MimeTypeEnum.MimeType mime_type = 10;
* @return The enum numeric value on the wire for mimeType.
*/
@java.lang.Override public int getMimeTypeValue() {
return mimeType_;
}
/**
*
* The mime type of the image.
*
*
* .google.ads.googleads.v17.enums.MimeTypeEnum.MimeType mime_type = 10;
* @param value The enum numeric value on the wire for mimeType to set.
* @return This builder for chaining.
*/
public Builder setMimeTypeValue(int value) {
mimeType_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* The mime type of the image.
*
*
* .google.ads.googleads.v17.enums.MimeTypeEnum.MimeType mime_type = 10;
* @return The mimeType.
*/
@java.lang.Override
public com.google.ads.googleads.v17.enums.MimeTypeEnum.MimeType getMimeType() {
com.google.ads.googleads.v17.enums.MimeTypeEnum.MimeType result = com.google.ads.googleads.v17.enums.MimeTypeEnum.MimeType.forNumber(mimeType_);
return result == null ? com.google.ads.googleads.v17.enums.MimeTypeEnum.MimeType.UNRECOGNIZED : result;
}
/**
*
* The mime type of the image.
*
*
* .google.ads.googleads.v17.enums.MimeTypeEnum.MimeType mime_type = 10;
* @param value The mimeType to set.
* @return This builder for chaining.
*/
public Builder setMimeType(com.google.ads.googleads.v17.enums.MimeTypeEnum.MimeType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
mimeType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The mime type of the image.
*
*
* .google.ads.googleads.v17.enums.MimeTypeEnum.MimeType mime_type = 10;
* @return This builder for chaining.
*/
public Builder clearMimeType() {
bitField0_ = (bitField0_ & ~0x00000040);
mimeType_ = 0;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* The name of the image. If the image was created from a MediaFile, this is
* the MediaFile's name. If the image was created from bytes, this is empty.
*
*
* optional string name = 21;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* The name of the image. If the image was created from a MediaFile, this is
* the MediaFile's name. If the image was created from bytes, this is empty.
*
*
* optional string name = 21;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the image. If the image was created from a MediaFile, this is
* the MediaFile's name. If the image was created from bytes, this is empty.
*
*
* optional string name = 21;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the image. If the image was created from a MediaFile, this is
* the MediaFile's name. If the image was created from bytes, this is empty.
*
*
* optional string name = 21;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* The name of the image. If the image was created from a MediaFile, this is
* the MediaFile's name. If the image was created from bytes, this is empty.
*
*
* optional string name = 21;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
* The name of the image. If the image was created from a MediaFile, this is
* the MediaFile's name. If the image was created from bytes, this is empty.
*
*
* optional string name = 21;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.common.AdImageAsset, com.google.ads.googleads.v17.common.AdImageAsset.Builder, com.google.ads.googleads.v17.common.AdImageAssetOrBuilder> imageAssetBuilder_;
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
* @return Whether the imageAsset field is set.
*/
@java.lang.Override
public boolean hasImageAsset() {
return imageCase_ == 22;
}
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
* @return The imageAsset.
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.AdImageAsset getImageAsset() {
if (imageAssetBuilder_ == null) {
if (imageCase_ == 22) {
return (com.google.ads.googleads.v17.common.AdImageAsset) image_;
}
return com.google.ads.googleads.v17.common.AdImageAsset.getDefaultInstance();
} else {
if (imageCase_ == 22) {
return imageAssetBuilder_.getMessage();
}
return com.google.ads.googleads.v17.common.AdImageAsset.getDefaultInstance();
}
}
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
*/
public Builder setImageAsset(com.google.ads.googleads.v17.common.AdImageAsset value) {
if (imageAssetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
image_ = value;
onChanged();
} else {
imageAssetBuilder_.setMessage(value);
}
imageCase_ = 22;
return this;
}
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
*/
public Builder setImageAsset(
com.google.ads.googleads.v17.common.AdImageAsset.Builder builderForValue) {
if (imageAssetBuilder_ == null) {
image_ = builderForValue.build();
onChanged();
} else {
imageAssetBuilder_.setMessage(builderForValue.build());
}
imageCase_ = 22;
return this;
}
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
*/
public Builder mergeImageAsset(com.google.ads.googleads.v17.common.AdImageAsset value) {
if (imageAssetBuilder_ == null) {
if (imageCase_ == 22 &&
image_ != com.google.ads.googleads.v17.common.AdImageAsset.getDefaultInstance()) {
image_ = com.google.ads.googleads.v17.common.AdImageAsset.newBuilder((com.google.ads.googleads.v17.common.AdImageAsset) image_)
.mergeFrom(value).buildPartial();
} else {
image_ = value;
}
onChanged();
} else {
if (imageCase_ == 22) {
imageAssetBuilder_.mergeFrom(value);
} else {
imageAssetBuilder_.setMessage(value);
}
}
imageCase_ = 22;
return this;
}
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
*/
public Builder clearImageAsset() {
if (imageAssetBuilder_ == null) {
if (imageCase_ == 22) {
imageCase_ = 0;
image_ = null;
onChanged();
}
} else {
if (imageCase_ == 22) {
imageCase_ = 0;
image_ = null;
}
imageAssetBuilder_.clear();
}
return this;
}
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
*/
public com.google.ads.googleads.v17.common.AdImageAsset.Builder getImageAssetBuilder() {
return getImageAssetFieldBuilder().getBuilder();
}
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.AdImageAssetOrBuilder getImageAssetOrBuilder() {
if ((imageCase_ == 22) && (imageAssetBuilder_ != null)) {
return imageAssetBuilder_.getMessageOrBuilder();
} else {
if (imageCase_ == 22) {
return (com.google.ads.googleads.v17.common.AdImageAsset) image_;
}
return com.google.ads.googleads.v17.common.AdImageAsset.getDefaultInstance();
}
}
/**
*
* The image assets used for the ad.
*
*
* .google.ads.googleads.v17.common.AdImageAsset image_asset = 22;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.common.AdImageAsset, com.google.ads.googleads.v17.common.AdImageAsset.Builder, com.google.ads.googleads.v17.common.AdImageAssetOrBuilder>
getImageAssetFieldBuilder() {
if (imageAssetBuilder_ == null) {
if (!(imageCase_ == 22)) {
image_ = com.google.ads.googleads.v17.common.AdImageAsset.getDefaultInstance();
}
imageAssetBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.common.AdImageAsset, com.google.ads.googleads.v17.common.AdImageAsset.Builder, com.google.ads.googleads.v17.common.AdImageAssetOrBuilder>(
(com.google.ads.googleads.v17.common.AdImageAsset) image_,
getParentForChildren(),
isClean());
image_ = null;
}
imageCase_ = 22;
onChanged();
return imageAssetBuilder_;
}
/**
*
* Raw image data as bytes.
*
*
* bytes data = 13;
* @return Whether the data field is set.
*/
public boolean hasData() {
return imageCase_ == 13;
}
/**
*
* Raw image data as bytes.
*
*
* bytes data = 13;
* @return The data.
*/
public com.google.protobuf.ByteString getData() {
if (imageCase_ == 13) {
return (com.google.protobuf.ByteString) image_;
}
return com.google.protobuf.ByteString.EMPTY;
}
/**
*
* Raw image data as bytes.
*
*
* bytes data = 13;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
imageCase_ = 13;
image_ = value;
onChanged();
return this;
}
/**
*
* Raw image data as bytes.
*
*
* bytes data = 13;
* @return This builder for chaining.
*/
public Builder clearData() {
if (imageCase_ == 13) {
imageCase_ = 0;
image_ = null;
onChanged();
}
return this;
}
/**
*
* An ad ID to copy the image from.
*
*
* int64 ad_id_to_copy_image_from = 14;
* @return Whether the adIdToCopyImageFrom field is set.
*/
public boolean hasAdIdToCopyImageFrom() {
return imageCase_ == 14;
}
/**
*
* An ad ID to copy the image from.
*
*
* int64 ad_id_to_copy_image_from = 14;
* @return The adIdToCopyImageFrom.
*/
public long getAdIdToCopyImageFrom() {
if (imageCase_ == 14) {
return (java.lang.Long) image_;
}
return 0L;
}
/**
*
* An ad ID to copy the image from.
*
*
* int64 ad_id_to_copy_image_from = 14;
* @param value The adIdToCopyImageFrom to set.
* @return This builder for chaining.
*/
public Builder setAdIdToCopyImageFrom(long value) {
imageCase_ = 14;
image_ = value;
onChanged();
return this;
}
/**
*
* An ad ID to copy the image from.
*
*
* int64 ad_id_to_copy_image_from = 14;
* @return This builder for chaining.
*/
public Builder clearAdIdToCopyImageFrom() {
if (imageCase_ == 14) {
imageCase_ = 0;
image_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v17.common.ImageAdInfo)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v17.common.ImageAdInfo)
private static final com.google.ads.googleads.v17.common.ImageAdInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v17.common.ImageAdInfo();
}
public static com.google.ads.googleads.v17.common.ImageAdInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ImageAdInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v17.common.ImageAdInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy