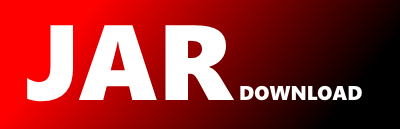
com.google.ads.googleads.v17.common.LeadFormAsset Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v17 Show documentation
Show all versions of google-ads-stubs-v17 Show documentation
Stubs for GAAPI version google-ads-stubs-v17
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v17/common/asset_types.proto
// Protobuf Java Version: 3.25.3
package com.google.ads.googleads.v17.common;
/**
*
* A Lead Form asset.
*
*
* Protobuf type {@code google.ads.googleads.v17.common.LeadFormAsset}
*/
public final class LeadFormAsset extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v17.common.LeadFormAsset)
LeadFormAssetOrBuilder {
private static final long serialVersionUID = 0L;
// Use LeadFormAsset.newBuilder() to construct.
private LeadFormAsset(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LeadFormAsset() {
businessName_ = "";
callToActionType_ = 0;
callToActionDescription_ = "";
headline_ = "";
description_ = "";
privacyPolicyUrl_ = "";
postSubmitHeadline_ = "";
postSubmitDescription_ = "";
fields_ = java.util.Collections.emptyList();
customQuestionFields_ = java.util.Collections.emptyList();
deliveryMethods_ = java.util.Collections.emptyList();
postSubmitCallToActionType_ = 0;
backgroundImageAsset_ = "";
desiredIntent_ = 0;
customDisclosure_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LeadFormAsset();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.common.AssetTypesProto.internal_static_google_ads_googleads_v17_common_LeadFormAsset_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.common.AssetTypesProto.internal_static_google_ads_googleads_v17_common_LeadFormAsset_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.common.LeadFormAsset.class, com.google.ads.googleads.v17.common.LeadFormAsset.Builder.class);
}
private int bitField0_;
public static final int BUSINESS_NAME_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private volatile java.lang.Object businessName_ = "";
/**
*
* Required. The name of the business being advertised.
*
*
* string business_name = 10 [(.google.api.field_behavior) = REQUIRED];
* @return The businessName.
*/
@java.lang.Override
public java.lang.String getBusinessName() {
java.lang.Object ref = businessName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
businessName_ = s;
return s;
}
}
/**
*
* Required. The name of the business being advertised.
*
*
* string business_name = 10 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for businessName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getBusinessNameBytes() {
java.lang.Object ref = businessName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
businessName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CALL_TO_ACTION_TYPE_FIELD_NUMBER = 17;
private int callToActionType_ = 0;
/**
*
* Required. Pre-defined display text that encourages user to expand the form.
*
*
* .google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType call_to_action_type = 17 [(.google.api.field_behavior) = REQUIRED];
* @return The enum numeric value on the wire for callToActionType.
*/
@java.lang.Override public int getCallToActionTypeValue() {
return callToActionType_;
}
/**
*
* Required. Pre-defined display text that encourages user to expand the form.
*
*
* .google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType call_to_action_type = 17 [(.google.api.field_behavior) = REQUIRED];
* @return The callToActionType.
*/
@java.lang.Override public com.google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType getCallToActionType() {
com.google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType result = com.google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType.forNumber(callToActionType_);
return result == null ? com.google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType.UNRECOGNIZED : result;
}
public static final int CALL_TO_ACTION_DESCRIPTION_FIELD_NUMBER = 18;
@SuppressWarnings("serial")
private volatile java.lang.Object callToActionDescription_ = "";
/**
*
* Required. Text giving a clear value proposition of what users expect once
* they expand the form.
*
*
* string call_to_action_description = 18 [(.google.api.field_behavior) = REQUIRED];
* @return The callToActionDescription.
*/
@java.lang.Override
public java.lang.String getCallToActionDescription() {
java.lang.Object ref = callToActionDescription_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
callToActionDescription_ = s;
return s;
}
}
/**
*
* Required. Text giving a clear value proposition of what users expect once
* they expand the form.
*
*
* string call_to_action_description = 18 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for callToActionDescription.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCallToActionDescriptionBytes() {
java.lang.Object ref = callToActionDescription_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
callToActionDescription_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HEADLINE_FIELD_NUMBER = 12;
@SuppressWarnings("serial")
private volatile java.lang.Object headline_ = "";
/**
*
* Required. Headline of the expanded form to describe what the form is asking
* for or facilitating.
*
*
* string headline = 12 [(.google.api.field_behavior) = REQUIRED];
* @return The headline.
*/
@java.lang.Override
public java.lang.String getHeadline() {
java.lang.Object ref = headline_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
headline_ = s;
return s;
}
}
/**
*
* Required. Headline of the expanded form to describe what the form is asking
* for or facilitating.
*
*
* string headline = 12 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for headline.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getHeadlineBytes() {
java.lang.Object ref = headline_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
headline_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 13;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
*
* Required. Detailed description of the expanded form to describe what the
* form is asking for or facilitating.
*
*
* string description = 13 [(.google.api.field_behavior) = REQUIRED];
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* Required. Detailed description of the expanded form to describe what the
* form is asking for or facilitating.
*
*
* string description = 13 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PRIVACY_POLICY_URL_FIELD_NUMBER = 14;
@SuppressWarnings("serial")
private volatile java.lang.Object privacyPolicyUrl_ = "";
/**
*
* Required. Link to a page describing the policy on how the collected data is
* handled by the advertiser/business.
*
*
* string privacy_policy_url = 14 [(.google.api.field_behavior) = REQUIRED];
* @return The privacyPolicyUrl.
*/
@java.lang.Override
public java.lang.String getPrivacyPolicyUrl() {
java.lang.Object ref = privacyPolicyUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
privacyPolicyUrl_ = s;
return s;
}
}
/**
*
* Required. Link to a page describing the policy on how the collected data is
* handled by the advertiser/business.
*
*
* string privacy_policy_url = 14 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for privacyPolicyUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPrivacyPolicyUrlBytes() {
java.lang.Object ref = privacyPolicyUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
privacyPolicyUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int POST_SUBMIT_HEADLINE_FIELD_NUMBER = 15;
@SuppressWarnings("serial")
private volatile java.lang.Object postSubmitHeadline_ = "";
/**
*
* Headline of text shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_headline = 15;
* @return Whether the postSubmitHeadline field is set.
*/
@java.lang.Override
public boolean hasPostSubmitHeadline() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Headline of text shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_headline = 15;
* @return The postSubmitHeadline.
*/
@java.lang.Override
public java.lang.String getPostSubmitHeadline() {
java.lang.Object ref = postSubmitHeadline_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
postSubmitHeadline_ = s;
return s;
}
}
/**
*
* Headline of text shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_headline = 15;
* @return The bytes for postSubmitHeadline.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPostSubmitHeadlineBytes() {
java.lang.Object ref = postSubmitHeadline_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
postSubmitHeadline_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int POST_SUBMIT_DESCRIPTION_FIELD_NUMBER = 16;
@SuppressWarnings("serial")
private volatile java.lang.Object postSubmitDescription_ = "";
/**
*
* Detailed description shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_description = 16;
* @return Whether the postSubmitDescription field is set.
*/
@java.lang.Override
public boolean hasPostSubmitDescription() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Detailed description shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_description = 16;
* @return The postSubmitDescription.
*/
@java.lang.Override
public java.lang.String getPostSubmitDescription() {
java.lang.Object ref = postSubmitDescription_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
postSubmitDescription_ = s;
return s;
}
}
/**
*
* Detailed description shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_description = 16;
* @return The bytes for postSubmitDescription.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPostSubmitDescriptionBytes() {
java.lang.Object ref = postSubmitDescription_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
postSubmitDescription_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FIELDS_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private java.util.List fields_;
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
@java.lang.Override
public java.util.List getFieldsList() {
return fields_;
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
@java.lang.Override
public java.util.List extends com.google.ads.googleads.v17.common.LeadFormFieldOrBuilder>
getFieldsOrBuilderList() {
return fields_;
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
@java.lang.Override
public int getFieldsCount() {
return fields_.size();
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.LeadFormField getFields(int index) {
return fields_.get(index);
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.LeadFormFieldOrBuilder getFieldsOrBuilder(
int index) {
return fields_.get(index);
}
public static final int CUSTOM_QUESTION_FIELDS_FIELD_NUMBER = 23;
@SuppressWarnings("serial")
private java.util.List customQuestionFields_;
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
@java.lang.Override
public java.util.List getCustomQuestionFieldsList() {
return customQuestionFields_;
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
@java.lang.Override
public java.util.List extends com.google.ads.googleads.v17.common.LeadFormCustomQuestionFieldOrBuilder>
getCustomQuestionFieldsOrBuilderList() {
return customQuestionFields_;
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
@java.lang.Override
public int getCustomQuestionFieldsCount() {
return customQuestionFields_.size();
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.LeadFormCustomQuestionField getCustomQuestionFields(int index) {
return customQuestionFields_.get(index);
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.LeadFormCustomQuestionFieldOrBuilder getCustomQuestionFieldsOrBuilder(
int index) {
return customQuestionFields_.get(index);
}
public static final int DELIVERY_METHODS_FIELD_NUMBER = 9;
@SuppressWarnings("serial")
private java.util.List deliveryMethods_;
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
@java.lang.Override
public java.util.List getDeliveryMethodsList() {
return deliveryMethods_;
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
@java.lang.Override
public java.util.List extends com.google.ads.googleads.v17.common.LeadFormDeliveryMethodOrBuilder>
getDeliveryMethodsOrBuilderList() {
return deliveryMethods_;
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
@java.lang.Override
public int getDeliveryMethodsCount() {
return deliveryMethods_.size();
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.LeadFormDeliveryMethod getDeliveryMethods(int index) {
return deliveryMethods_.get(index);
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.LeadFormDeliveryMethodOrBuilder getDeliveryMethodsOrBuilder(
int index) {
return deliveryMethods_.get(index);
}
public static final int POST_SUBMIT_CALL_TO_ACTION_TYPE_FIELD_NUMBER = 19;
private int postSubmitCallToActionType_ = 0;
/**
*
* Pre-defined display text that encourages user action after the form is
* submitted.
*
*
* .google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType post_submit_call_to_action_type = 19;
* @return The enum numeric value on the wire for postSubmitCallToActionType.
*/
@java.lang.Override public int getPostSubmitCallToActionTypeValue() {
return postSubmitCallToActionType_;
}
/**
*
* Pre-defined display text that encourages user action after the form is
* submitted.
*
*
* .google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType post_submit_call_to_action_type = 19;
* @return The postSubmitCallToActionType.
*/
@java.lang.Override public com.google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType getPostSubmitCallToActionType() {
com.google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType result = com.google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType.forNumber(postSubmitCallToActionType_);
return result == null ? com.google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType.UNRECOGNIZED : result;
}
public static final int BACKGROUND_IMAGE_ASSET_FIELD_NUMBER = 20;
@SuppressWarnings("serial")
private volatile java.lang.Object backgroundImageAsset_ = "";
/**
*
* Asset resource name of the background image.
* The image dimensions must be exactly 1200x628.
*
*
* optional string background_image_asset = 20;
* @return Whether the backgroundImageAsset field is set.
*/
@java.lang.Override
public boolean hasBackgroundImageAsset() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Asset resource name of the background image.
* The image dimensions must be exactly 1200x628.
*
*
* optional string background_image_asset = 20;
* @return The backgroundImageAsset.
*/
@java.lang.Override
public java.lang.String getBackgroundImageAsset() {
java.lang.Object ref = backgroundImageAsset_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
backgroundImageAsset_ = s;
return s;
}
}
/**
*
* Asset resource name of the background image.
* The image dimensions must be exactly 1200x628.
*
*
* optional string background_image_asset = 20;
* @return The bytes for backgroundImageAsset.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getBackgroundImageAssetBytes() {
java.lang.Object ref = backgroundImageAsset_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
backgroundImageAsset_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESIRED_INTENT_FIELD_NUMBER = 21;
private int desiredIntent_ = 0;
/**
*
* Chosen intent for the lead form, for example, more volume or more
* qualified.
*
*
* .google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent desired_intent = 21;
* @return The enum numeric value on the wire for desiredIntent.
*/
@java.lang.Override public int getDesiredIntentValue() {
return desiredIntent_;
}
/**
*
* Chosen intent for the lead form, for example, more volume or more
* qualified.
*
*
* .google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent desired_intent = 21;
* @return The desiredIntent.
*/
@java.lang.Override public com.google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent getDesiredIntent() {
com.google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent result = com.google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent.forNumber(desiredIntent_);
return result == null ? com.google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent.UNRECOGNIZED : result;
}
public static final int CUSTOM_DISCLOSURE_FIELD_NUMBER = 22;
@SuppressWarnings("serial")
private volatile java.lang.Object customDisclosure_ = "";
/**
*
* Custom disclosure shown along with Google disclaimer on the lead form.
* Accessible to allowed customers only.
*
*
* optional string custom_disclosure = 22;
* @return Whether the customDisclosure field is set.
*/
@java.lang.Override
public boolean hasCustomDisclosure() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Custom disclosure shown along with Google disclaimer on the lead form.
* Accessible to allowed customers only.
*
*
* optional string custom_disclosure = 22;
* @return The customDisclosure.
*/
@java.lang.Override
public java.lang.String getCustomDisclosure() {
java.lang.Object ref = customDisclosure_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customDisclosure_ = s;
return s;
}
}
/**
*
* Custom disclosure shown along with Google disclaimer on the lead form.
* Accessible to allowed customers only.
*
*
* optional string custom_disclosure = 22;
* @return The bytes for customDisclosure.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCustomDisclosureBytes() {
java.lang.Object ref = customDisclosure_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customDisclosure_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < fields_.size(); i++) {
output.writeMessage(8, fields_.get(i));
}
for (int i = 0; i < deliveryMethods_.size(); i++) {
output.writeMessage(9, deliveryMethods_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(businessName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, businessName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(headline_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, headline_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, description_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(privacyPolicyUrl_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, privacyPolicyUrl_);
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, postSubmitHeadline_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, postSubmitDescription_);
}
if (callToActionType_ != com.google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType.UNSPECIFIED.getNumber()) {
output.writeEnum(17, callToActionType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(callToActionDescription_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 18, callToActionDescription_);
}
if (postSubmitCallToActionType_ != com.google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType.UNSPECIFIED.getNumber()) {
output.writeEnum(19, postSubmitCallToActionType_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, backgroundImageAsset_);
}
if (desiredIntent_ != com.google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent.UNSPECIFIED.getNumber()) {
output.writeEnum(21, desiredIntent_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 22, customDisclosure_);
}
for (int i = 0; i < customQuestionFields_.size(); i++) {
output.writeMessage(23, customQuestionFields_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < fields_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, fields_.get(i));
}
for (int i = 0; i < deliveryMethods_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, deliveryMethods_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(businessName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, businessName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(headline_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, headline_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, description_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(privacyPolicyUrl_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, privacyPolicyUrl_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, postSubmitHeadline_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, postSubmitDescription_);
}
if (callToActionType_ != com.google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(17, callToActionType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(callToActionDescription_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(18, callToActionDescription_);
}
if (postSubmitCallToActionType_ != com.google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(19, postSubmitCallToActionType_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(20, backgroundImageAsset_);
}
if (desiredIntent_ != com.google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(21, desiredIntent_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(22, customDisclosure_);
}
for (int i = 0; i < customQuestionFields_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(23, customQuestionFields_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v17.common.LeadFormAsset)) {
return super.equals(obj);
}
com.google.ads.googleads.v17.common.LeadFormAsset other = (com.google.ads.googleads.v17.common.LeadFormAsset) obj;
if (!getBusinessName()
.equals(other.getBusinessName())) return false;
if (callToActionType_ != other.callToActionType_) return false;
if (!getCallToActionDescription()
.equals(other.getCallToActionDescription())) return false;
if (!getHeadline()
.equals(other.getHeadline())) return false;
if (!getDescription()
.equals(other.getDescription())) return false;
if (!getPrivacyPolicyUrl()
.equals(other.getPrivacyPolicyUrl())) return false;
if (hasPostSubmitHeadline() != other.hasPostSubmitHeadline()) return false;
if (hasPostSubmitHeadline()) {
if (!getPostSubmitHeadline()
.equals(other.getPostSubmitHeadline())) return false;
}
if (hasPostSubmitDescription() != other.hasPostSubmitDescription()) return false;
if (hasPostSubmitDescription()) {
if (!getPostSubmitDescription()
.equals(other.getPostSubmitDescription())) return false;
}
if (!getFieldsList()
.equals(other.getFieldsList())) return false;
if (!getCustomQuestionFieldsList()
.equals(other.getCustomQuestionFieldsList())) return false;
if (!getDeliveryMethodsList()
.equals(other.getDeliveryMethodsList())) return false;
if (postSubmitCallToActionType_ != other.postSubmitCallToActionType_) return false;
if (hasBackgroundImageAsset() != other.hasBackgroundImageAsset()) return false;
if (hasBackgroundImageAsset()) {
if (!getBackgroundImageAsset()
.equals(other.getBackgroundImageAsset())) return false;
}
if (desiredIntent_ != other.desiredIntent_) return false;
if (hasCustomDisclosure() != other.hasCustomDisclosure()) return false;
if (hasCustomDisclosure()) {
if (!getCustomDisclosure()
.equals(other.getCustomDisclosure())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + BUSINESS_NAME_FIELD_NUMBER;
hash = (53 * hash) + getBusinessName().hashCode();
hash = (37 * hash) + CALL_TO_ACTION_TYPE_FIELD_NUMBER;
hash = (53 * hash) + callToActionType_;
hash = (37 * hash) + CALL_TO_ACTION_DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getCallToActionDescription().hashCode();
hash = (37 * hash) + HEADLINE_FIELD_NUMBER;
hash = (53 * hash) + getHeadline().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + PRIVACY_POLICY_URL_FIELD_NUMBER;
hash = (53 * hash) + getPrivacyPolicyUrl().hashCode();
if (hasPostSubmitHeadline()) {
hash = (37 * hash) + POST_SUBMIT_HEADLINE_FIELD_NUMBER;
hash = (53 * hash) + getPostSubmitHeadline().hashCode();
}
if (hasPostSubmitDescription()) {
hash = (37 * hash) + POST_SUBMIT_DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getPostSubmitDescription().hashCode();
}
if (getFieldsCount() > 0) {
hash = (37 * hash) + FIELDS_FIELD_NUMBER;
hash = (53 * hash) + getFieldsList().hashCode();
}
if (getCustomQuestionFieldsCount() > 0) {
hash = (37 * hash) + CUSTOM_QUESTION_FIELDS_FIELD_NUMBER;
hash = (53 * hash) + getCustomQuestionFieldsList().hashCode();
}
if (getDeliveryMethodsCount() > 0) {
hash = (37 * hash) + DELIVERY_METHODS_FIELD_NUMBER;
hash = (53 * hash) + getDeliveryMethodsList().hashCode();
}
hash = (37 * hash) + POST_SUBMIT_CALL_TO_ACTION_TYPE_FIELD_NUMBER;
hash = (53 * hash) + postSubmitCallToActionType_;
if (hasBackgroundImageAsset()) {
hash = (37 * hash) + BACKGROUND_IMAGE_ASSET_FIELD_NUMBER;
hash = (53 * hash) + getBackgroundImageAsset().hashCode();
}
hash = (37 * hash) + DESIRED_INTENT_FIELD_NUMBER;
hash = (53 * hash) + desiredIntent_;
if (hasCustomDisclosure()) {
hash = (37 * hash) + CUSTOM_DISCLOSURE_FIELD_NUMBER;
hash = (53 * hash) + getCustomDisclosure().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.common.LeadFormAsset parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v17.common.LeadFormAsset prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A Lead Form asset.
*
*
* Protobuf type {@code google.ads.googleads.v17.common.LeadFormAsset}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v17.common.LeadFormAsset)
com.google.ads.googleads.v17.common.LeadFormAssetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.common.AssetTypesProto.internal_static_google_ads_googleads_v17_common_LeadFormAsset_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.common.AssetTypesProto.internal_static_google_ads_googleads_v17_common_LeadFormAsset_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.common.LeadFormAsset.class, com.google.ads.googleads.v17.common.LeadFormAsset.Builder.class);
}
// Construct using com.google.ads.googleads.v17.common.LeadFormAsset.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
businessName_ = "";
callToActionType_ = 0;
callToActionDescription_ = "";
headline_ = "";
description_ = "";
privacyPolicyUrl_ = "";
postSubmitHeadline_ = "";
postSubmitDescription_ = "";
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
} else {
fields_ = null;
fieldsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
if (customQuestionFieldsBuilder_ == null) {
customQuestionFields_ = java.util.Collections.emptyList();
} else {
customQuestionFields_ = null;
customQuestionFieldsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
if (deliveryMethodsBuilder_ == null) {
deliveryMethods_ = java.util.Collections.emptyList();
} else {
deliveryMethods_ = null;
deliveryMethodsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
postSubmitCallToActionType_ = 0;
backgroundImageAsset_ = "";
desiredIntent_ = 0;
customDisclosure_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v17.common.AssetTypesProto.internal_static_google_ads_googleads_v17_common_LeadFormAsset_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v17.common.LeadFormAsset getDefaultInstanceForType() {
return com.google.ads.googleads.v17.common.LeadFormAsset.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v17.common.LeadFormAsset build() {
com.google.ads.googleads.v17.common.LeadFormAsset result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v17.common.LeadFormAsset buildPartial() {
com.google.ads.googleads.v17.common.LeadFormAsset result = new com.google.ads.googleads.v17.common.LeadFormAsset(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.ads.googleads.v17.common.LeadFormAsset result) {
if (fieldsBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)) {
fields_ = java.util.Collections.unmodifiableList(fields_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.fields_ = fields_;
} else {
result.fields_ = fieldsBuilder_.build();
}
if (customQuestionFieldsBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)) {
customQuestionFields_ = java.util.Collections.unmodifiableList(customQuestionFields_);
bitField0_ = (bitField0_ & ~0x00000200);
}
result.customQuestionFields_ = customQuestionFields_;
} else {
result.customQuestionFields_ = customQuestionFieldsBuilder_.build();
}
if (deliveryMethodsBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0)) {
deliveryMethods_ = java.util.Collections.unmodifiableList(deliveryMethods_);
bitField0_ = (bitField0_ & ~0x00000400);
}
result.deliveryMethods_ = deliveryMethods_;
} else {
result.deliveryMethods_ = deliveryMethodsBuilder_.build();
}
}
private void buildPartial0(com.google.ads.googleads.v17.common.LeadFormAsset result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.businessName_ = businessName_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.callToActionType_ = callToActionType_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.callToActionDescription_ = callToActionDescription_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.headline_ = headline_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.description_ = description_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.privacyPolicyUrl_ = privacyPolicyUrl_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000040) != 0)) {
result.postSubmitHeadline_ = postSubmitHeadline_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.postSubmitDescription_ = postSubmitDescription_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.postSubmitCallToActionType_ = postSubmitCallToActionType_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.backgroundImageAsset_ = backgroundImageAsset_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.desiredIntent_ = desiredIntent_;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.customDisclosure_ = customDisclosure_;
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v17.common.LeadFormAsset) {
return mergeFrom((com.google.ads.googleads.v17.common.LeadFormAsset)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v17.common.LeadFormAsset other) {
if (other == com.google.ads.googleads.v17.common.LeadFormAsset.getDefaultInstance()) return this;
if (!other.getBusinessName().isEmpty()) {
businessName_ = other.businessName_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.callToActionType_ != 0) {
setCallToActionTypeValue(other.getCallToActionTypeValue());
}
if (!other.getCallToActionDescription().isEmpty()) {
callToActionDescription_ = other.callToActionDescription_;
bitField0_ |= 0x00000004;
onChanged();
}
if (!other.getHeadline().isEmpty()) {
headline_ = other.headline_;
bitField0_ |= 0x00000008;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
bitField0_ |= 0x00000010;
onChanged();
}
if (!other.getPrivacyPolicyUrl().isEmpty()) {
privacyPolicyUrl_ = other.privacyPolicyUrl_;
bitField0_ |= 0x00000020;
onChanged();
}
if (other.hasPostSubmitHeadline()) {
postSubmitHeadline_ = other.postSubmitHeadline_;
bitField0_ |= 0x00000040;
onChanged();
}
if (other.hasPostSubmitDescription()) {
postSubmitDescription_ = other.postSubmitDescription_;
bitField0_ |= 0x00000080;
onChanged();
}
if (fieldsBuilder_ == null) {
if (!other.fields_.isEmpty()) {
if (fields_.isEmpty()) {
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureFieldsIsMutable();
fields_.addAll(other.fields_);
}
onChanged();
}
} else {
if (!other.fields_.isEmpty()) {
if (fieldsBuilder_.isEmpty()) {
fieldsBuilder_.dispose();
fieldsBuilder_ = null;
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000100);
fieldsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFieldsFieldBuilder() : null;
} else {
fieldsBuilder_.addAllMessages(other.fields_);
}
}
}
if (customQuestionFieldsBuilder_ == null) {
if (!other.customQuestionFields_.isEmpty()) {
if (customQuestionFields_.isEmpty()) {
customQuestionFields_ = other.customQuestionFields_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureCustomQuestionFieldsIsMutable();
customQuestionFields_.addAll(other.customQuestionFields_);
}
onChanged();
}
} else {
if (!other.customQuestionFields_.isEmpty()) {
if (customQuestionFieldsBuilder_.isEmpty()) {
customQuestionFieldsBuilder_.dispose();
customQuestionFieldsBuilder_ = null;
customQuestionFields_ = other.customQuestionFields_;
bitField0_ = (bitField0_ & ~0x00000200);
customQuestionFieldsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCustomQuestionFieldsFieldBuilder() : null;
} else {
customQuestionFieldsBuilder_.addAllMessages(other.customQuestionFields_);
}
}
}
if (deliveryMethodsBuilder_ == null) {
if (!other.deliveryMethods_.isEmpty()) {
if (deliveryMethods_.isEmpty()) {
deliveryMethods_ = other.deliveryMethods_;
bitField0_ = (bitField0_ & ~0x00000400);
} else {
ensureDeliveryMethodsIsMutable();
deliveryMethods_.addAll(other.deliveryMethods_);
}
onChanged();
}
} else {
if (!other.deliveryMethods_.isEmpty()) {
if (deliveryMethodsBuilder_.isEmpty()) {
deliveryMethodsBuilder_.dispose();
deliveryMethodsBuilder_ = null;
deliveryMethods_ = other.deliveryMethods_;
bitField0_ = (bitField0_ & ~0x00000400);
deliveryMethodsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getDeliveryMethodsFieldBuilder() : null;
} else {
deliveryMethodsBuilder_.addAllMessages(other.deliveryMethods_);
}
}
}
if (other.postSubmitCallToActionType_ != 0) {
setPostSubmitCallToActionTypeValue(other.getPostSubmitCallToActionTypeValue());
}
if (other.hasBackgroundImageAsset()) {
backgroundImageAsset_ = other.backgroundImageAsset_;
bitField0_ |= 0x00001000;
onChanged();
}
if (other.desiredIntent_ != 0) {
setDesiredIntentValue(other.getDesiredIntentValue());
}
if (other.hasCustomDisclosure()) {
customDisclosure_ = other.customDisclosure_;
bitField0_ |= 0x00004000;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 66: {
com.google.ads.googleads.v17.common.LeadFormField m =
input.readMessage(
com.google.ads.googleads.v17.common.LeadFormField.parser(),
extensionRegistry);
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(m);
} else {
fieldsBuilder_.addMessage(m);
}
break;
} // case 66
case 74: {
com.google.ads.googleads.v17.common.LeadFormDeliveryMethod m =
input.readMessage(
com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.parser(),
extensionRegistry);
if (deliveryMethodsBuilder_ == null) {
ensureDeliveryMethodsIsMutable();
deliveryMethods_.add(m);
} else {
deliveryMethodsBuilder_.addMessage(m);
}
break;
} // case 74
case 82: {
businessName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 82
case 98: {
headline_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 98
case 106: {
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 106
case 114: {
privacyPolicyUrl_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 114
case 122: {
postSubmitHeadline_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 122
case 130: {
postSubmitDescription_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 130
case 136: {
callToActionType_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 136
case 146: {
callToActionDescription_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 146
case 152: {
postSubmitCallToActionType_ = input.readEnum();
bitField0_ |= 0x00000800;
break;
} // case 152
case 162: {
backgroundImageAsset_ = input.readStringRequireUtf8();
bitField0_ |= 0x00001000;
break;
} // case 162
case 168: {
desiredIntent_ = input.readEnum();
bitField0_ |= 0x00002000;
break;
} // case 168
case 178: {
customDisclosure_ = input.readStringRequireUtf8();
bitField0_ |= 0x00004000;
break;
} // case 178
case 186: {
com.google.ads.googleads.v17.common.LeadFormCustomQuestionField m =
input.readMessage(
com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.parser(),
extensionRegistry);
if (customQuestionFieldsBuilder_ == null) {
ensureCustomQuestionFieldsIsMutable();
customQuestionFields_.add(m);
} else {
customQuestionFieldsBuilder_.addMessage(m);
}
break;
} // case 186
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object businessName_ = "";
/**
*
* Required. The name of the business being advertised.
*
*
* string business_name = 10 [(.google.api.field_behavior) = REQUIRED];
* @return The businessName.
*/
public java.lang.String getBusinessName() {
java.lang.Object ref = businessName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
businessName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required. The name of the business being advertised.
*
*
* string business_name = 10 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for businessName.
*/
public com.google.protobuf.ByteString
getBusinessNameBytes() {
java.lang.Object ref = businessName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
businessName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required. The name of the business being advertised.
*
*
* string business_name = 10 [(.google.api.field_behavior) = REQUIRED];
* @param value The businessName to set.
* @return This builder for chaining.
*/
public Builder setBusinessName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
businessName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Required. The name of the business being advertised.
*
*
* string business_name = 10 [(.google.api.field_behavior) = REQUIRED];
* @return This builder for chaining.
*/
public Builder clearBusinessName() {
businessName_ = getDefaultInstance().getBusinessName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Required. The name of the business being advertised.
*
*
* string business_name = 10 [(.google.api.field_behavior) = REQUIRED];
* @param value The bytes for businessName to set.
* @return This builder for chaining.
*/
public Builder setBusinessNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
businessName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int callToActionType_ = 0;
/**
*
* Required. Pre-defined display text that encourages user to expand the form.
*
*
* .google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType call_to_action_type = 17 [(.google.api.field_behavior) = REQUIRED];
* @return The enum numeric value on the wire for callToActionType.
*/
@java.lang.Override public int getCallToActionTypeValue() {
return callToActionType_;
}
/**
*
* Required. Pre-defined display text that encourages user to expand the form.
*
*
* .google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType call_to_action_type = 17 [(.google.api.field_behavior) = REQUIRED];
* @param value The enum numeric value on the wire for callToActionType to set.
* @return This builder for chaining.
*/
public Builder setCallToActionTypeValue(int value) {
callToActionType_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Required. Pre-defined display text that encourages user to expand the form.
*
*
* .google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType call_to_action_type = 17 [(.google.api.field_behavior) = REQUIRED];
* @return The callToActionType.
*/
@java.lang.Override
public com.google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType getCallToActionType() {
com.google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType result = com.google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType.forNumber(callToActionType_);
return result == null ? com.google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType.UNRECOGNIZED : result;
}
/**
*
* Required. Pre-defined display text that encourages user to expand the form.
*
*
* .google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType call_to_action_type = 17 [(.google.api.field_behavior) = REQUIRED];
* @param value The callToActionType to set.
* @return This builder for chaining.
*/
public Builder setCallToActionType(com.google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
callToActionType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Required. Pre-defined display text that encourages user to expand the form.
*
*
* .google.ads.googleads.v17.enums.LeadFormCallToActionTypeEnum.LeadFormCallToActionType call_to_action_type = 17 [(.google.api.field_behavior) = REQUIRED];
* @return This builder for chaining.
*/
public Builder clearCallToActionType() {
bitField0_ = (bitField0_ & ~0x00000002);
callToActionType_ = 0;
onChanged();
return this;
}
private java.lang.Object callToActionDescription_ = "";
/**
*
* Required. Text giving a clear value proposition of what users expect once
* they expand the form.
*
*
* string call_to_action_description = 18 [(.google.api.field_behavior) = REQUIRED];
* @return The callToActionDescription.
*/
public java.lang.String getCallToActionDescription() {
java.lang.Object ref = callToActionDescription_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
callToActionDescription_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required. Text giving a clear value proposition of what users expect once
* they expand the form.
*
*
* string call_to_action_description = 18 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for callToActionDescription.
*/
public com.google.protobuf.ByteString
getCallToActionDescriptionBytes() {
java.lang.Object ref = callToActionDescription_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
callToActionDescription_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required. Text giving a clear value proposition of what users expect once
* they expand the form.
*
*
* string call_to_action_description = 18 [(.google.api.field_behavior) = REQUIRED];
* @param value The callToActionDescription to set.
* @return This builder for chaining.
*/
public Builder setCallToActionDescription(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
callToActionDescription_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Required. Text giving a clear value proposition of what users expect once
* they expand the form.
*
*
* string call_to_action_description = 18 [(.google.api.field_behavior) = REQUIRED];
* @return This builder for chaining.
*/
public Builder clearCallToActionDescription() {
callToActionDescription_ = getDefaultInstance().getCallToActionDescription();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* Required. Text giving a clear value proposition of what users expect once
* they expand the form.
*
*
* string call_to_action_description = 18 [(.google.api.field_behavior) = REQUIRED];
* @param value The bytes for callToActionDescription to set.
* @return This builder for chaining.
*/
public Builder setCallToActionDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
callToActionDescription_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object headline_ = "";
/**
*
* Required. Headline of the expanded form to describe what the form is asking
* for or facilitating.
*
*
* string headline = 12 [(.google.api.field_behavior) = REQUIRED];
* @return The headline.
*/
public java.lang.String getHeadline() {
java.lang.Object ref = headline_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
headline_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required. Headline of the expanded form to describe what the form is asking
* for or facilitating.
*
*
* string headline = 12 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for headline.
*/
public com.google.protobuf.ByteString
getHeadlineBytes() {
java.lang.Object ref = headline_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
headline_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required. Headline of the expanded form to describe what the form is asking
* for or facilitating.
*
*
* string headline = 12 [(.google.api.field_behavior) = REQUIRED];
* @param value The headline to set.
* @return This builder for chaining.
*/
public Builder setHeadline(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
headline_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Required. Headline of the expanded form to describe what the form is asking
* for or facilitating.
*
*
* string headline = 12 [(.google.api.field_behavior) = REQUIRED];
* @return This builder for chaining.
*/
public Builder clearHeadline() {
headline_ = getDefaultInstance().getHeadline();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
* Required. Headline of the expanded form to describe what the form is asking
* for or facilitating.
*
*
* string headline = 12 [(.google.api.field_behavior) = REQUIRED];
* @param value The bytes for headline to set.
* @return This builder for chaining.
*/
public Builder setHeadlineBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
headline_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* Required. Detailed description of the expanded form to describe what the
* form is asking for or facilitating.
*
*
* string description = 13 [(.google.api.field_behavior) = REQUIRED];
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required. Detailed description of the expanded form to describe what the
* form is asking for or facilitating.
*
*
* string description = 13 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required. Detailed description of the expanded form to describe what the
* form is asking for or facilitating.
*
*
* string description = 13 [(.google.api.field_behavior) = REQUIRED];
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
description_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Required. Detailed description of the expanded form to describe what the
* form is asking for or facilitating.
*
*
* string description = 13 [(.google.api.field_behavior) = REQUIRED];
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
* Required. Detailed description of the expanded form to describe what the
* form is asking for or facilitating.
*
*
* string description = 13 [(.google.api.field_behavior) = REQUIRED];
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
description_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private java.lang.Object privacyPolicyUrl_ = "";
/**
*
* Required. Link to a page describing the policy on how the collected data is
* handled by the advertiser/business.
*
*
* string privacy_policy_url = 14 [(.google.api.field_behavior) = REQUIRED];
* @return The privacyPolicyUrl.
*/
public java.lang.String getPrivacyPolicyUrl() {
java.lang.Object ref = privacyPolicyUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
privacyPolicyUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required. Link to a page describing the policy on how the collected data is
* handled by the advertiser/business.
*
*
* string privacy_policy_url = 14 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for privacyPolicyUrl.
*/
public com.google.protobuf.ByteString
getPrivacyPolicyUrlBytes() {
java.lang.Object ref = privacyPolicyUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
privacyPolicyUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required. Link to a page describing the policy on how the collected data is
* handled by the advertiser/business.
*
*
* string privacy_policy_url = 14 [(.google.api.field_behavior) = REQUIRED];
* @param value The privacyPolicyUrl to set.
* @return This builder for chaining.
*/
public Builder setPrivacyPolicyUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
privacyPolicyUrl_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Required. Link to a page describing the policy on how the collected data is
* handled by the advertiser/business.
*
*
* string privacy_policy_url = 14 [(.google.api.field_behavior) = REQUIRED];
* @return This builder for chaining.
*/
public Builder clearPrivacyPolicyUrl() {
privacyPolicyUrl_ = getDefaultInstance().getPrivacyPolicyUrl();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
* Required. Link to a page describing the policy on how the collected data is
* handled by the advertiser/business.
*
*
* string privacy_policy_url = 14 [(.google.api.field_behavior) = REQUIRED];
* @param value The bytes for privacyPolicyUrl to set.
* @return This builder for chaining.
*/
public Builder setPrivacyPolicyUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
privacyPolicyUrl_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.lang.Object postSubmitHeadline_ = "";
/**
*
* Headline of text shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_headline = 15;
* @return Whether the postSubmitHeadline field is set.
*/
public boolean hasPostSubmitHeadline() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Headline of text shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_headline = 15;
* @return The postSubmitHeadline.
*/
public java.lang.String getPostSubmitHeadline() {
java.lang.Object ref = postSubmitHeadline_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
postSubmitHeadline_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Headline of text shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_headline = 15;
* @return The bytes for postSubmitHeadline.
*/
public com.google.protobuf.ByteString
getPostSubmitHeadlineBytes() {
java.lang.Object ref = postSubmitHeadline_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
postSubmitHeadline_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Headline of text shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_headline = 15;
* @param value The postSubmitHeadline to set.
* @return This builder for chaining.
*/
public Builder setPostSubmitHeadline(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
postSubmitHeadline_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Headline of text shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_headline = 15;
* @return This builder for chaining.
*/
public Builder clearPostSubmitHeadline() {
postSubmitHeadline_ = getDefaultInstance().getPostSubmitHeadline();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
* Headline of text shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_headline = 15;
* @param value The bytes for postSubmitHeadline to set.
* @return This builder for chaining.
*/
public Builder setPostSubmitHeadlineBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
postSubmitHeadline_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object postSubmitDescription_ = "";
/**
*
* Detailed description shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_description = 16;
* @return Whether the postSubmitDescription field is set.
*/
public boolean hasPostSubmitDescription() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Detailed description shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_description = 16;
* @return The postSubmitDescription.
*/
public java.lang.String getPostSubmitDescription() {
java.lang.Object ref = postSubmitDescription_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
postSubmitDescription_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Detailed description shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_description = 16;
* @return The bytes for postSubmitDescription.
*/
public com.google.protobuf.ByteString
getPostSubmitDescriptionBytes() {
java.lang.Object ref = postSubmitDescription_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
postSubmitDescription_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Detailed description shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_description = 16;
* @param value The postSubmitDescription to set.
* @return This builder for chaining.
*/
public Builder setPostSubmitDescription(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
postSubmitDescription_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Detailed description shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_description = 16;
* @return This builder for chaining.
*/
public Builder clearPostSubmitDescription() {
postSubmitDescription_ = getDefaultInstance().getPostSubmitDescription();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
* Detailed description shown after form submission that describes how the
* advertiser will follow up with the user.
*
*
* optional string post_submit_description = 16;
* @param value The bytes for postSubmitDescription to set.
* @return This builder for chaining.
*/
public Builder setPostSubmitDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
postSubmitDescription_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private java.util.List fields_ =
java.util.Collections.emptyList();
private void ensureFieldsIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
fields_ = new java.util.ArrayList(fields_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.LeadFormField, com.google.ads.googleads.v17.common.LeadFormField.Builder, com.google.ads.googleads.v17.common.LeadFormFieldOrBuilder> fieldsBuilder_;
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public java.util.List getFieldsList() {
if (fieldsBuilder_ == null) {
return java.util.Collections.unmodifiableList(fields_);
} else {
return fieldsBuilder_.getMessageList();
}
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public int getFieldsCount() {
if (fieldsBuilder_ == null) {
return fields_.size();
} else {
return fieldsBuilder_.getCount();
}
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public com.google.ads.googleads.v17.common.LeadFormField getFields(int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index);
} else {
return fieldsBuilder_.getMessage(index);
}
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public Builder setFields(
int index, com.google.ads.googleads.v17.common.LeadFormField value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.set(index, value);
onChanged();
} else {
fieldsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public Builder setFields(
int index, com.google.ads.googleads.v17.common.LeadFormField.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.set(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public Builder addFields(com.google.ads.googleads.v17.common.LeadFormField value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(value);
onChanged();
} else {
fieldsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public Builder addFields(
int index, com.google.ads.googleads.v17.common.LeadFormField value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(index, value);
onChanged();
} else {
fieldsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public Builder addFields(
com.google.ads.googleads.v17.common.LeadFormField.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public Builder addFields(
int index, com.google.ads.googleads.v17.common.LeadFormField.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public Builder addAllFields(
java.lang.Iterable extends com.google.ads.googleads.v17.common.LeadFormField> values) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fields_);
onChanged();
} else {
fieldsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public Builder clearFields() {
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
fieldsBuilder_.clear();
}
return this;
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public Builder removeFields(int index) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.remove(index);
onChanged();
} else {
fieldsBuilder_.remove(index);
}
return this;
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public com.google.ads.googleads.v17.common.LeadFormField.Builder getFieldsBuilder(
int index) {
return getFieldsFieldBuilder().getBuilder(index);
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public com.google.ads.googleads.v17.common.LeadFormFieldOrBuilder getFieldsOrBuilder(
int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index); } else {
return fieldsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public java.util.List extends com.google.ads.googleads.v17.common.LeadFormFieldOrBuilder>
getFieldsOrBuilderList() {
if (fieldsBuilder_ != null) {
return fieldsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(fields_);
}
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public com.google.ads.googleads.v17.common.LeadFormField.Builder addFieldsBuilder() {
return getFieldsFieldBuilder().addBuilder(
com.google.ads.googleads.v17.common.LeadFormField.getDefaultInstance());
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public com.google.ads.googleads.v17.common.LeadFormField.Builder addFieldsBuilder(
int index) {
return getFieldsFieldBuilder().addBuilder(
index, com.google.ads.googleads.v17.common.LeadFormField.getDefaultInstance());
}
/**
*
* Ordered list of input fields. This field can be updated by reordering
* questions, but not by adding or removing questions.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormField fields = 8;
*/
public java.util.List
getFieldsBuilderList() {
return getFieldsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.LeadFormField, com.google.ads.googleads.v17.common.LeadFormField.Builder, com.google.ads.googleads.v17.common.LeadFormFieldOrBuilder>
getFieldsFieldBuilder() {
if (fieldsBuilder_ == null) {
fieldsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.LeadFormField, com.google.ads.googleads.v17.common.LeadFormField.Builder, com.google.ads.googleads.v17.common.LeadFormFieldOrBuilder>(
fields_,
((bitField0_ & 0x00000100) != 0),
getParentForChildren(),
isClean());
fields_ = null;
}
return fieldsBuilder_;
}
private java.util.List customQuestionFields_ =
java.util.Collections.emptyList();
private void ensureCustomQuestionFieldsIsMutable() {
if (!((bitField0_ & 0x00000200) != 0)) {
customQuestionFields_ = new java.util.ArrayList(customQuestionFields_);
bitField0_ |= 0x00000200;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.LeadFormCustomQuestionField, com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.Builder, com.google.ads.googleads.v17.common.LeadFormCustomQuestionFieldOrBuilder> customQuestionFieldsBuilder_;
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public java.util.List getCustomQuestionFieldsList() {
if (customQuestionFieldsBuilder_ == null) {
return java.util.Collections.unmodifiableList(customQuestionFields_);
} else {
return customQuestionFieldsBuilder_.getMessageList();
}
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public int getCustomQuestionFieldsCount() {
if (customQuestionFieldsBuilder_ == null) {
return customQuestionFields_.size();
} else {
return customQuestionFieldsBuilder_.getCount();
}
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public com.google.ads.googleads.v17.common.LeadFormCustomQuestionField getCustomQuestionFields(int index) {
if (customQuestionFieldsBuilder_ == null) {
return customQuestionFields_.get(index);
} else {
return customQuestionFieldsBuilder_.getMessage(index);
}
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public Builder setCustomQuestionFields(
int index, com.google.ads.googleads.v17.common.LeadFormCustomQuestionField value) {
if (customQuestionFieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCustomQuestionFieldsIsMutable();
customQuestionFields_.set(index, value);
onChanged();
} else {
customQuestionFieldsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public Builder setCustomQuestionFields(
int index, com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.Builder builderForValue) {
if (customQuestionFieldsBuilder_ == null) {
ensureCustomQuestionFieldsIsMutable();
customQuestionFields_.set(index, builderForValue.build());
onChanged();
} else {
customQuestionFieldsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public Builder addCustomQuestionFields(com.google.ads.googleads.v17.common.LeadFormCustomQuestionField value) {
if (customQuestionFieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCustomQuestionFieldsIsMutable();
customQuestionFields_.add(value);
onChanged();
} else {
customQuestionFieldsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public Builder addCustomQuestionFields(
int index, com.google.ads.googleads.v17.common.LeadFormCustomQuestionField value) {
if (customQuestionFieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCustomQuestionFieldsIsMutable();
customQuestionFields_.add(index, value);
onChanged();
} else {
customQuestionFieldsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public Builder addCustomQuestionFields(
com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.Builder builderForValue) {
if (customQuestionFieldsBuilder_ == null) {
ensureCustomQuestionFieldsIsMutable();
customQuestionFields_.add(builderForValue.build());
onChanged();
} else {
customQuestionFieldsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public Builder addCustomQuestionFields(
int index, com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.Builder builderForValue) {
if (customQuestionFieldsBuilder_ == null) {
ensureCustomQuestionFieldsIsMutable();
customQuestionFields_.add(index, builderForValue.build());
onChanged();
} else {
customQuestionFieldsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public Builder addAllCustomQuestionFields(
java.lang.Iterable extends com.google.ads.googleads.v17.common.LeadFormCustomQuestionField> values) {
if (customQuestionFieldsBuilder_ == null) {
ensureCustomQuestionFieldsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, customQuestionFields_);
onChanged();
} else {
customQuestionFieldsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public Builder clearCustomQuestionFields() {
if (customQuestionFieldsBuilder_ == null) {
customQuestionFields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
} else {
customQuestionFieldsBuilder_.clear();
}
return this;
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public Builder removeCustomQuestionFields(int index) {
if (customQuestionFieldsBuilder_ == null) {
ensureCustomQuestionFieldsIsMutable();
customQuestionFields_.remove(index);
onChanged();
} else {
customQuestionFieldsBuilder_.remove(index);
}
return this;
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.Builder getCustomQuestionFieldsBuilder(
int index) {
return getCustomQuestionFieldsFieldBuilder().getBuilder(index);
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public com.google.ads.googleads.v17.common.LeadFormCustomQuestionFieldOrBuilder getCustomQuestionFieldsOrBuilder(
int index) {
if (customQuestionFieldsBuilder_ == null) {
return customQuestionFields_.get(index); } else {
return customQuestionFieldsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public java.util.List extends com.google.ads.googleads.v17.common.LeadFormCustomQuestionFieldOrBuilder>
getCustomQuestionFieldsOrBuilderList() {
if (customQuestionFieldsBuilder_ != null) {
return customQuestionFieldsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(customQuestionFields_);
}
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.Builder addCustomQuestionFieldsBuilder() {
return getCustomQuestionFieldsFieldBuilder().addBuilder(
com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.getDefaultInstance());
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.Builder addCustomQuestionFieldsBuilder(
int index) {
return getCustomQuestionFieldsFieldBuilder().addBuilder(
index, com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.getDefaultInstance());
}
/**
*
* Ordered list of custom question fields. This field is subject to a limit of
* 5 qualifying questions per form.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormCustomQuestionField custom_question_fields = 23;
*/
public java.util.List
getCustomQuestionFieldsBuilderList() {
return getCustomQuestionFieldsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.LeadFormCustomQuestionField, com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.Builder, com.google.ads.googleads.v17.common.LeadFormCustomQuestionFieldOrBuilder>
getCustomQuestionFieldsFieldBuilder() {
if (customQuestionFieldsBuilder_ == null) {
customQuestionFieldsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.LeadFormCustomQuestionField, com.google.ads.googleads.v17.common.LeadFormCustomQuestionField.Builder, com.google.ads.googleads.v17.common.LeadFormCustomQuestionFieldOrBuilder>(
customQuestionFields_,
((bitField0_ & 0x00000200) != 0),
getParentForChildren(),
isClean());
customQuestionFields_ = null;
}
return customQuestionFieldsBuilder_;
}
private java.util.List deliveryMethods_ =
java.util.Collections.emptyList();
private void ensureDeliveryMethodsIsMutable() {
if (!((bitField0_ & 0x00000400) != 0)) {
deliveryMethods_ = new java.util.ArrayList(deliveryMethods_);
bitField0_ |= 0x00000400;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.LeadFormDeliveryMethod, com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.Builder, com.google.ads.googleads.v17.common.LeadFormDeliveryMethodOrBuilder> deliveryMethodsBuilder_;
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public java.util.List getDeliveryMethodsList() {
if (deliveryMethodsBuilder_ == null) {
return java.util.Collections.unmodifiableList(deliveryMethods_);
} else {
return deliveryMethodsBuilder_.getMessageList();
}
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public int getDeliveryMethodsCount() {
if (deliveryMethodsBuilder_ == null) {
return deliveryMethods_.size();
} else {
return deliveryMethodsBuilder_.getCount();
}
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public com.google.ads.googleads.v17.common.LeadFormDeliveryMethod getDeliveryMethods(int index) {
if (deliveryMethodsBuilder_ == null) {
return deliveryMethods_.get(index);
} else {
return deliveryMethodsBuilder_.getMessage(index);
}
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public Builder setDeliveryMethods(
int index, com.google.ads.googleads.v17.common.LeadFormDeliveryMethod value) {
if (deliveryMethodsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDeliveryMethodsIsMutable();
deliveryMethods_.set(index, value);
onChanged();
} else {
deliveryMethodsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public Builder setDeliveryMethods(
int index, com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.Builder builderForValue) {
if (deliveryMethodsBuilder_ == null) {
ensureDeliveryMethodsIsMutable();
deliveryMethods_.set(index, builderForValue.build());
onChanged();
} else {
deliveryMethodsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public Builder addDeliveryMethods(com.google.ads.googleads.v17.common.LeadFormDeliveryMethod value) {
if (deliveryMethodsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDeliveryMethodsIsMutable();
deliveryMethods_.add(value);
onChanged();
} else {
deliveryMethodsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public Builder addDeliveryMethods(
int index, com.google.ads.googleads.v17.common.LeadFormDeliveryMethod value) {
if (deliveryMethodsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDeliveryMethodsIsMutable();
deliveryMethods_.add(index, value);
onChanged();
} else {
deliveryMethodsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public Builder addDeliveryMethods(
com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.Builder builderForValue) {
if (deliveryMethodsBuilder_ == null) {
ensureDeliveryMethodsIsMutable();
deliveryMethods_.add(builderForValue.build());
onChanged();
} else {
deliveryMethodsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public Builder addDeliveryMethods(
int index, com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.Builder builderForValue) {
if (deliveryMethodsBuilder_ == null) {
ensureDeliveryMethodsIsMutable();
deliveryMethods_.add(index, builderForValue.build());
onChanged();
} else {
deliveryMethodsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public Builder addAllDeliveryMethods(
java.lang.Iterable extends com.google.ads.googleads.v17.common.LeadFormDeliveryMethod> values) {
if (deliveryMethodsBuilder_ == null) {
ensureDeliveryMethodsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, deliveryMethods_);
onChanged();
} else {
deliveryMethodsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public Builder clearDeliveryMethods() {
if (deliveryMethodsBuilder_ == null) {
deliveryMethods_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
} else {
deliveryMethodsBuilder_.clear();
}
return this;
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public Builder removeDeliveryMethods(int index) {
if (deliveryMethodsBuilder_ == null) {
ensureDeliveryMethodsIsMutable();
deliveryMethods_.remove(index);
onChanged();
} else {
deliveryMethodsBuilder_.remove(index);
}
return this;
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.Builder getDeliveryMethodsBuilder(
int index) {
return getDeliveryMethodsFieldBuilder().getBuilder(index);
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public com.google.ads.googleads.v17.common.LeadFormDeliveryMethodOrBuilder getDeliveryMethodsOrBuilder(
int index) {
if (deliveryMethodsBuilder_ == null) {
return deliveryMethods_.get(index); } else {
return deliveryMethodsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public java.util.List extends com.google.ads.googleads.v17.common.LeadFormDeliveryMethodOrBuilder>
getDeliveryMethodsOrBuilderList() {
if (deliveryMethodsBuilder_ != null) {
return deliveryMethodsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(deliveryMethods_);
}
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.Builder addDeliveryMethodsBuilder() {
return getDeliveryMethodsFieldBuilder().addBuilder(
com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.getDefaultInstance());
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.Builder addDeliveryMethodsBuilder(
int index) {
return getDeliveryMethodsFieldBuilder().addBuilder(
index, com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.getDefaultInstance());
}
/**
*
* Configured methods for collected lead data to be delivered to advertiser.
* Only one method typed as WebhookDelivery can be configured.
*
*
* repeated .google.ads.googleads.v17.common.LeadFormDeliveryMethod delivery_methods = 9;
*/
public java.util.List
getDeliveryMethodsBuilderList() {
return getDeliveryMethodsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.LeadFormDeliveryMethod, com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.Builder, com.google.ads.googleads.v17.common.LeadFormDeliveryMethodOrBuilder>
getDeliveryMethodsFieldBuilder() {
if (deliveryMethodsBuilder_ == null) {
deliveryMethodsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.LeadFormDeliveryMethod, com.google.ads.googleads.v17.common.LeadFormDeliveryMethod.Builder, com.google.ads.googleads.v17.common.LeadFormDeliveryMethodOrBuilder>(
deliveryMethods_,
((bitField0_ & 0x00000400) != 0),
getParentForChildren(),
isClean());
deliveryMethods_ = null;
}
return deliveryMethodsBuilder_;
}
private int postSubmitCallToActionType_ = 0;
/**
*
* Pre-defined display text that encourages user action after the form is
* submitted.
*
*
* .google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType post_submit_call_to_action_type = 19;
* @return The enum numeric value on the wire for postSubmitCallToActionType.
*/
@java.lang.Override public int getPostSubmitCallToActionTypeValue() {
return postSubmitCallToActionType_;
}
/**
*
* Pre-defined display text that encourages user action after the form is
* submitted.
*
*
* .google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType post_submit_call_to_action_type = 19;
* @param value The enum numeric value on the wire for postSubmitCallToActionType to set.
* @return This builder for chaining.
*/
public Builder setPostSubmitCallToActionTypeValue(int value) {
postSubmitCallToActionType_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
* Pre-defined display text that encourages user action after the form is
* submitted.
*
*
* .google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType post_submit_call_to_action_type = 19;
* @return The postSubmitCallToActionType.
*/
@java.lang.Override
public com.google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType getPostSubmitCallToActionType() {
com.google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType result = com.google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType.forNumber(postSubmitCallToActionType_);
return result == null ? com.google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType.UNRECOGNIZED : result;
}
/**
*
* Pre-defined display text that encourages user action after the form is
* submitted.
*
*
* .google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType post_submit_call_to_action_type = 19;
* @param value The postSubmitCallToActionType to set.
* @return This builder for chaining.
*/
public Builder setPostSubmitCallToActionType(com.google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
postSubmitCallToActionType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Pre-defined display text that encourages user action after the form is
* submitted.
*
*
* .google.ads.googleads.v17.enums.LeadFormPostSubmitCallToActionTypeEnum.LeadFormPostSubmitCallToActionType post_submit_call_to_action_type = 19;
* @return This builder for chaining.
*/
public Builder clearPostSubmitCallToActionType() {
bitField0_ = (bitField0_ & ~0x00000800);
postSubmitCallToActionType_ = 0;
onChanged();
return this;
}
private java.lang.Object backgroundImageAsset_ = "";
/**
*
* Asset resource name of the background image.
* The image dimensions must be exactly 1200x628.
*
*
* optional string background_image_asset = 20;
* @return Whether the backgroundImageAsset field is set.
*/
public boolean hasBackgroundImageAsset() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* Asset resource name of the background image.
* The image dimensions must be exactly 1200x628.
*
*
* optional string background_image_asset = 20;
* @return The backgroundImageAsset.
*/
public java.lang.String getBackgroundImageAsset() {
java.lang.Object ref = backgroundImageAsset_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
backgroundImageAsset_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Asset resource name of the background image.
* The image dimensions must be exactly 1200x628.
*
*
* optional string background_image_asset = 20;
* @return The bytes for backgroundImageAsset.
*/
public com.google.protobuf.ByteString
getBackgroundImageAssetBytes() {
java.lang.Object ref = backgroundImageAsset_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
backgroundImageAsset_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Asset resource name of the background image.
* The image dimensions must be exactly 1200x628.
*
*
* optional string background_image_asset = 20;
* @param value The backgroundImageAsset to set.
* @return This builder for chaining.
*/
public Builder setBackgroundImageAsset(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
backgroundImageAsset_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* Asset resource name of the background image.
* The image dimensions must be exactly 1200x628.
*
*
* optional string background_image_asset = 20;
* @return This builder for chaining.
*/
public Builder clearBackgroundImageAsset() {
backgroundImageAsset_ = getDefaultInstance().getBackgroundImageAsset();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
return this;
}
/**
*
* Asset resource name of the background image.
* The image dimensions must be exactly 1200x628.
*
*
* optional string background_image_asset = 20;
* @param value The bytes for backgroundImageAsset to set.
* @return This builder for chaining.
*/
public Builder setBackgroundImageAssetBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
backgroundImageAsset_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
private int desiredIntent_ = 0;
/**
*
* Chosen intent for the lead form, for example, more volume or more
* qualified.
*
*
* .google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent desired_intent = 21;
* @return The enum numeric value on the wire for desiredIntent.
*/
@java.lang.Override public int getDesiredIntentValue() {
return desiredIntent_;
}
/**
*
* Chosen intent for the lead form, for example, more volume or more
* qualified.
*
*
* .google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent desired_intent = 21;
* @param value The enum numeric value on the wire for desiredIntent to set.
* @return This builder for chaining.
*/
public Builder setDesiredIntentValue(int value) {
desiredIntent_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* Chosen intent for the lead form, for example, more volume or more
* qualified.
*
*
* .google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent desired_intent = 21;
* @return The desiredIntent.
*/
@java.lang.Override
public com.google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent getDesiredIntent() {
com.google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent result = com.google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent.forNumber(desiredIntent_);
return result == null ? com.google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent.UNRECOGNIZED : result;
}
/**
*
* Chosen intent for the lead form, for example, more volume or more
* qualified.
*
*
* .google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent desired_intent = 21;
* @param value The desiredIntent to set.
* @return This builder for chaining.
*/
public Builder setDesiredIntent(com.google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
desiredIntent_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Chosen intent for the lead form, for example, more volume or more
* qualified.
*
*
* .google.ads.googleads.v17.enums.LeadFormDesiredIntentEnum.LeadFormDesiredIntent desired_intent = 21;
* @return This builder for chaining.
*/
public Builder clearDesiredIntent() {
bitField0_ = (bitField0_ & ~0x00002000);
desiredIntent_ = 0;
onChanged();
return this;
}
private java.lang.Object customDisclosure_ = "";
/**
*
* Custom disclosure shown along with Google disclaimer on the lead form.
* Accessible to allowed customers only.
*
*
* optional string custom_disclosure = 22;
* @return Whether the customDisclosure field is set.
*/
public boolean hasCustomDisclosure() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
* Custom disclosure shown along with Google disclaimer on the lead form.
* Accessible to allowed customers only.
*
*
* optional string custom_disclosure = 22;
* @return The customDisclosure.
*/
public java.lang.String getCustomDisclosure() {
java.lang.Object ref = customDisclosure_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customDisclosure_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Custom disclosure shown along with Google disclaimer on the lead form.
* Accessible to allowed customers only.
*
*
* optional string custom_disclosure = 22;
* @return The bytes for customDisclosure.
*/
public com.google.protobuf.ByteString
getCustomDisclosureBytes() {
java.lang.Object ref = customDisclosure_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customDisclosure_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Custom disclosure shown along with Google disclaimer on the lead form.
* Accessible to allowed customers only.
*
*
* optional string custom_disclosure = 22;
* @param value The customDisclosure to set.
* @return This builder for chaining.
*/
public Builder setCustomDisclosure(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
customDisclosure_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
* Custom disclosure shown along with Google disclaimer on the lead form.
* Accessible to allowed customers only.
*
*
* optional string custom_disclosure = 22;
* @return This builder for chaining.
*/
public Builder clearCustomDisclosure() {
customDisclosure_ = getDefaultInstance().getCustomDisclosure();
bitField0_ = (bitField0_ & ~0x00004000);
onChanged();
return this;
}
/**
*
* Custom disclosure shown along with Google disclaimer on the lead form.
* Accessible to allowed customers only.
*
*
* optional string custom_disclosure = 22;
* @param value The bytes for customDisclosure to set.
* @return This builder for chaining.
*/
public Builder setCustomDisclosureBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
customDisclosure_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v17.common.LeadFormAsset)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v17.common.LeadFormAsset)
private static final com.google.ads.googleads.v17.common.LeadFormAsset DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v17.common.LeadFormAsset();
}
public static com.google.ads.googleads.v17.common.LeadFormAsset getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LeadFormAsset parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v17.common.LeadFormAsset getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy