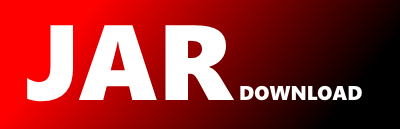
com.google.ads.googleads.v17.resources.CampaignBudget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v17 Show documentation
Show all versions of google-ads-stubs-v17 Show documentation
Stubs for GAAPI version google-ads-stubs-v17
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v17/resources/campaign_budget.proto
// Protobuf Java Version: 3.25.3
package com.google.ads.googleads.v17.resources;
/**
*
* A campaign budget.
*
*
* Protobuf type {@code google.ads.googleads.v17.resources.CampaignBudget}
*/
public final class CampaignBudget extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v17.resources.CampaignBudget)
CampaignBudgetOrBuilder {
private static final long serialVersionUID = 0L;
// Use CampaignBudget.newBuilder() to construct.
private CampaignBudget(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CampaignBudget() {
resourceName_ = "";
name_ = "";
status_ = 0;
deliveryMethod_ = 0;
period_ = 0;
type_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CampaignBudget();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.resources.CampaignBudgetProto.internal_static_google_ads_googleads_v17_resources_CampaignBudget_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.resources.CampaignBudgetProto.internal_static_google_ads_googleads_v17_resources_CampaignBudget_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.resources.CampaignBudget.class, com.google.ads.googleads.v17.resources.CampaignBudget.Builder.class);
}
private int bitField0_;
public static final int RESOURCE_NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object resourceName_ = "";
/**
*
* Immutable. The resource name of the campaign budget.
* Campaign budget resource names have the form:
*
* `customers/{customer_id}/campaignBudgets/{campaign_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
@java.lang.Override
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
}
}
/**
*
* Immutable. The resource name of the campaign budget.
* Campaign budget resource names have the form:
*
* `customers/{customer_id}/campaignBudgets/{campaign_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 19;
private long id_ = 0L;
/**
*
* Output only. The ID of the campaign budget.
*
* A campaign budget is created using the CampaignBudgetService create
* operation and is assigned a budget ID. A budget ID can be shared across
* different campaigns; the system will then allocate the campaign budget
* among different campaigns to get optimum results.
*
*
* optional int64 id = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Output only. The ID of the campaign budget.
*
* A campaign budget is created using the CampaignBudgetService create
* operation and is assigned a budget ID. A budget ID can be shared across
* different campaigns; the system will then allocate the campaign budget
* among different campaigns to get optimum results.
*
*
* optional int64 id = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int NAME_FIELD_NUMBER = 20;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
* The name of the campaign budget.
*
* When creating a campaign budget through CampaignBudgetService, every
* explicitly shared campaign budget must have a non-null, non-empty name.
* Campaign budgets that are not explicitly shared derive their name from the
* attached campaign's name.
*
* The length of this string must be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 20;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The name of the campaign budget.
*
* When creating a campaign budget through CampaignBudgetService, every
* explicitly shared campaign budget must have a non-null, non-empty name.
* Campaign budgets that are not explicitly shared derive their name from the
* attached campaign's name.
*
* The length of this string must be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 20;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* The name of the campaign budget.
*
* When creating a campaign budget through CampaignBudgetService, every
* explicitly shared campaign budget must have a non-null, non-empty name.
* Campaign budgets that are not explicitly shared derive their name from the
* attached campaign's name.
*
* The length of this string must be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 20;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AMOUNT_MICROS_FIELD_NUMBER = 21;
private long amountMicros_ = 0L;
/**
*
* The amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit. Monthly spend is capped at 30.4 times this amount.
*
*
* optional int64 amount_micros = 21;
* @return Whether the amountMicros field is set.
*/
@java.lang.Override
public boolean hasAmountMicros() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit. Monthly spend is capped at 30.4 times this amount.
*
*
* optional int64 amount_micros = 21;
* @return The amountMicros.
*/
@java.lang.Override
public long getAmountMicros() {
return amountMicros_;
}
public static final int TOTAL_AMOUNT_MICROS_FIELD_NUMBER = 22;
private long totalAmountMicros_ = 0L;
/**
*
* The lifetime amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit.
*
*
* optional int64 total_amount_micros = 22;
* @return Whether the totalAmountMicros field is set.
*/
@java.lang.Override
public boolean hasTotalAmountMicros() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The lifetime amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit.
*
*
* optional int64 total_amount_micros = 22;
* @return The totalAmountMicros.
*/
@java.lang.Override
public long getTotalAmountMicros() {
return totalAmountMicros_;
}
public static final int STATUS_FIELD_NUMBER = 6;
private int status_ = 0;
/**
*
* Output only. The status of this campaign budget. This field is read-only.
*
*
* .google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus status = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Output only. The status of this campaign budget. This field is read-only.
*
*
* .google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus status = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The status.
*/
@java.lang.Override public com.google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus getStatus() {
com.google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus result = com.google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus.forNumber(status_);
return result == null ? com.google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus.UNRECOGNIZED : result;
}
public static final int DELIVERY_METHOD_FIELD_NUMBER = 7;
private int deliveryMethod_ = 0;
/**
*
* The delivery method that determines the rate at which the campaign budget
* is spent.
*
* Defaults to STANDARD if unspecified in a create operation.
*
*
* .google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod delivery_method = 7;
* @return The enum numeric value on the wire for deliveryMethod.
*/
@java.lang.Override public int getDeliveryMethodValue() {
return deliveryMethod_;
}
/**
*
* The delivery method that determines the rate at which the campaign budget
* is spent.
*
* Defaults to STANDARD if unspecified in a create operation.
*
*
* .google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod delivery_method = 7;
* @return The deliveryMethod.
*/
@java.lang.Override public com.google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod getDeliveryMethod() {
com.google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod result = com.google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod.forNumber(deliveryMethod_);
return result == null ? com.google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod.UNRECOGNIZED : result;
}
public static final int EXPLICITLY_SHARED_FIELD_NUMBER = 23;
private boolean explicitlyShared_ = false;
/**
*
* Specifies whether the budget is explicitly shared. Defaults to true if
* unspecified in a create operation.
*
* If true, the budget was created with the purpose of sharing
* across one or more campaigns.
*
* If false, the budget was created with the intention of only being used
* with a single campaign. The budget's name and status will stay in sync
* with the campaign's name and status. Attempting to share the budget with a
* second campaign will result in an error.
*
* A non-shared budget can become an explicitly shared. The same operation
* must also assign the budget a name.
*
* A shared campaign budget can never become non-shared.
*
*
* optional bool explicitly_shared = 23;
* @return Whether the explicitlyShared field is set.
*/
@java.lang.Override
public boolean hasExplicitlyShared() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Specifies whether the budget is explicitly shared. Defaults to true if
* unspecified in a create operation.
*
* If true, the budget was created with the purpose of sharing
* across one or more campaigns.
*
* If false, the budget was created with the intention of only being used
* with a single campaign. The budget's name and status will stay in sync
* with the campaign's name and status. Attempting to share the budget with a
* second campaign will result in an error.
*
* A non-shared budget can become an explicitly shared. The same operation
* must also assign the budget a name.
*
* A shared campaign budget can never become non-shared.
*
*
* optional bool explicitly_shared = 23;
* @return The explicitlyShared.
*/
@java.lang.Override
public boolean getExplicitlyShared() {
return explicitlyShared_;
}
public static final int REFERENCE_COUNT_FIELD_NUMBER = 24;
private long referenceCount_ = 0L;
/**
*
* Output only. The number of campaigns actively using the budget.
*
* This field is read-only.
*
*
* optional int64 reference_count = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the referenceCount field is set.
*/
@java.lang.Override
public boolean hasReferenceCount() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Output only. The number of campaigns actively using the budget.
*
* This field is read-only.
*
*
* optional int64 reference_count = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The referenceCount.
*/
@java.lang.Override
public long getReferenceCount() {
return referenceCount_;
}
public static final int HAS_RECOMMENDED_BUDGET_FIELD_NUMBER = 25;
private boolean hasRecommendedBudget_ = false;
/**
*
* Output only. Indicates whether there is a recommended budget for this
* campaign budget.
*
* This field is read-only.
*
*
* optional bool has_recommended_budget = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the hasRecommendedBudget field is set.
*/
@java.lang.Override
public boolean hasHasRecommendedBudget() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Output only. Indicates whether there is a recommended budget for this
* campaign budget.
*
* This field is read-only.
*
*
* optional bool has_recommended_budget = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The hasRecommendedBudget.
*/
@java.lang.Override
public boolean getHasRecommendedBudget() {
return hasRecommendedBudget_;
}
public static final int RECOMMENDED_BUDGET_AMOUNT_MICROS_FIELD_NUMBER = 26;
private long recommendedBudgetAmountMicros_ = 0L;
/**
*
* Output only. The recommended budget amount. If no recommendation is
* available, this will be set to the budget amount. Amount is specified in
* micros, where one million is equivalent to one currency unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_amount_micros = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the recommendedBudgetAmountMicros field is set.
*/
@java.lang.Override
public boolean hasRecommendedBudgetAmountMicros() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Output only. The recommended budget amount. If no recommendation is
* available, this will be set to the budget amount. Amount is specified in
* micros, where one million is equivalent to one currency unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_amount_micros = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The recommendedBudgetAmountMicros.
*/
@java.lang.Override
public long getRecommendedBudgetAmountMicros() {
return recommendedBudgetAmountMicros_;
}
public static final int PERIOD_FIELD_NUMBER = 13;
private int period_ = 0;
/**
*
* Immutable. Period over which to spend the budget. Defaults to DAILY if not
* specified.
*
*
* .google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod period = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for period.
*/
@java.lang.Override public int getPeriodValue() {
return period_;
}
/**
*
* Immutable. Period over which to spend the budget. Defaults to DAILY if not
* specified.
*
*
* .google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod period = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return The period.
*/
@java.lang.Override public com.google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod getPeriod() {
com.google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod result = com.google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod.forNumber(period_);
return result == null ? com.google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod.UNRECOGNIZED : result;
}
public static final int RECOMMENDED_BUDGET_ESTIMATED_CHANGE_WEEKLY_CLICKS_FIELD_NUMBER = 27;
private long recommendedBudgetEstimatedChangeWeeklyClicks_ = 0L;
/**
*
* Output only. The estimated change in weekly clicks if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_clicks = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the recommendedBudgetEstimatedChangeWeeklyClicks field is set.
*/
@java.lang.Override
public boolean hasRecommendedBudgetEstimatedChangeWeeklyClicks() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Output only. The estimated change in weekly clicks if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_clicks = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The recommendedBudgetEstimatedChangeWeeklyClicks.
*/
@java.lang.Override
public long getRecommendedBudgetEstimatedChangeWeeklyClicks() {
return recommendedBudgetEstimatedChangeWeeklyClicks_;
}
public static final int RECOMMENDED_BUDGET_ESTIMATED_CHANGE_WEEKLY_COST_MICROS_FIELD_NUMBER = 28;
private long recommendedBudgetEstimatedChangeWeeklyCostMicros_ = 0L;
/**
*
* Output only. The estimated change in weekly cost in micros if the
* recommended budget is applied. One million is equivalent to one currency
* unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_cost_micros = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the recommendedBudgetEstimatedChangeWeeklyCostMicros field is set.
*/
@java.lang.Override
public boolean hasRecommendedBudgetEstimatedChangeWeeklyCostMicros() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Output only. The estimated change in weekly cost in micros if the
* recommended budget is applied. One million is equivalent to one currency
* unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_cost_micros = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The recommendedBudgetEstimatedChangeWeeklyCostMicros.
*/
@java.lang.Override
public long getRecommendedBudgetEstimatedChangeWeeklyCostMicros() {
return recommendedBudgetEstimatedChangeWeeklyCostMicros_;
}
public static final int RECOMMENDED_BUDGET_ESTIMATED_CHANGE_WEEKLY_INTERACTIONS_FIELD_NUMBER = 29;
private long recommendedBudgetEstimatedChangeWeeklyInteractions_ = 0L;
/**
*
* Output only. The estimated change in weekly interactions if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_interactions = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the recommendedBudgetEstimatedChangeWeeklyInteractions field is set.
*/
@java.lang.Override
public boolean hasRecommendedBudgetEstimatedChangeWeeklyInteractions() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Output only. The estimated change in weekly interactions if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_interactions = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The recommendedBudgetEstimatedChangeWeeklyInteractions.
*/
@java.lang.Override
public long getRecommendedBudgetEstimatedChangeWeeklyInteractions() {
return recommendedBudgetEstimatedChangeWeeklyInteractions_;
}
public static final int RECOMMENDED_BUDGET_ESTIMATED_CHANGE_WEEKLY_VIEWS_FIELD_NUMBER = 30;
private long recommendedBudgetEstimatedChangeWeeklyViews_ = 0L;
/**
*
* Output only. The estimated change in weekly views if the recommended budget
* is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_views = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the recommendedBudgetEstimatedChangeWeeklyViews field is set.
*/
@java.lang.Override
public boolean hasRecommendedBudgetEstimatedChangeWeeklyViews() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* Output only. The estimated change in weekly views if the recommended budget
* is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_views = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The recommendedBudgetEstimatedChangeWeeklyViews.
*/
@java.lang.Override
public long getRecommendedBudgetEstimatedChangeWeeklyViews() {
return recommendedBudgetEstimatedChangeWeeklyViews_;
}
public static final int TYPE_FIELD_NUMBER = 18;
private int type_ = 0;
/**
*
* Immutable. The type of the campaign budget.
*
*
* .google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType type = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
*
* Immutable. The type of the campaign budget.
*
*
* .google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType type = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return The type.
*/
@java.lang.Override public com.google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType getType() {
com.google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType result = com.google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType.forNumber(type_);
return result == null ? com.google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType.UNRECOGNIZED : result;
}
public static final int ALIGNED_BIDDING_STRATEGY_ID_FIELD_NUMBER = 31;
private long alignedBiddingStrategyId_ = 0L;
/**
*
* ID of the portfolio bidding strategy that this shared campaign budget
* is aligned with. When a bidding strategy and a campaign budget are aligned,
* they are attached to the same set of campaigns. After a campaign budget is
* aligned with a bidding strategy, campaigns that are added to the campaign
* budget must also use the aligned bidding strategy.
*
*
* int64 aligned_bidding_strategy_id = 31;
* @return The alignedBiddingStrategyId.
*/
@java.lang.Override
public long getAlignedBiddingStrategyId() {
return alignedBiddingStrategyId_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, resourceName_);
}
if (status_ != com.google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus.UNSPECIFIED.getNumber()) {
output.writeEnum(6, status_);
}
if (deliveryMethod_ != com.google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod.UNSPECIFIED.getNumber()) {
output.writeEnum(7, deliveryMethod_);
}
if (period_ != com.google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod.UNSPECIFIED.getNumber()) {
output.writeEnum(13, period_);
}
if (type_ != com.google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType.UNSPECIFIED.getNumber()) {
output.writeEnum(18, type_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(19, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(21, amountMicros_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt64(22, totalAmountMicros_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeBool(23, explicitlyShared_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt64(24, referenceCount_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeBool(25, hasRecommendedBudget_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeInt64(26, recommendedBudgetAmountMicros_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeInt64(27, recommendedBudgetEstimatedChangeWeeklyClicks_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeInt64(28, recommendedBudgetEstimatedChangeWeeklyCostMicros_);
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeInt64(29, recommendedBudgetEstimatedChangeWeeklyInteractions_);
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeInt64(30, recommendedBudgetEstimatedChangeWeeklyViews_);
}
if (alignedBiddingStrategyId_ != 0L) {
output.writeInt64(31, alignedBiddingStrategyId_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, resourceName_);
}
if (status_ != com.google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, status_);
}
if (deliveryMethod_ != com.google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, deliveryMethod_);
}
if (period_ != com.google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(13, period_);
}
if (type_ != com.google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(18, type_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(19, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(20, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(21, amountMicros_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(22, totalAmountMicros_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(23, explicitlyShared_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(24, referenceCount_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(25, hasRecommendedBudget_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(26, recommendedBudgetAmountMicros_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(27, recommendedBudgetEstimatedChangeWeeklyClicks_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(28, recommendedBudgetEstimatedChangeWeeklyCostMicros_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(29, recommendedBudgetEstimatedChangeWeeklyInteractions_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(30, recommendedBudgetEstimatedChangeWeeklyViews_);
}
if (alignedBiddingStrategyId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(31, alignedBiddingStrategyId_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v17.resources.CampaignBudget)) {
return super.equals(obj);
}
com.google.ads.googleads.v17.resources.CampaignBudget other = (com.google.ads.googleads.v17.resources.CampaignBudget) obj;
if (!getResourceName()
.equals(other.getResourceName())) return false;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId()
!= other.getId()) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasAmountMicros() != other.hasAmountMicros()) return false;
if (hasAmountMicros()) {
if (getAmountMicros()
!= other.getAmountMicros()) return false;
}
if (hasTotalAmountMicros() != other.hasTotalAmountMicros()) return false;
if (hasTotalAmountMicros()) {
if (getTotalAmountMicros()
!= other.getTotalAmountMicros()) return false;
}
if (status_ != other.status_) return false;
if (deliveryMethod_ != other.deliveryMethod_) return false;
if (hasExplicitlyShared() != other.hasExplicitlyShared()) return false;
if (hasExplicitlyShared()) {
if (getExplicitlyShared()
!= other.getExplicitlyShared()) return false;
}
if (hasReferenceCount() != other.hasReferenceCount()) return false;
if (hasReferenceCount()) {
if (getReferenceCount()
!= other.getReferenceCount()) return false;
}
if (hasHasRecommendedBudget() != other.hasHasRecommendedBudget()) return false;
if (hasHasRecommendedBudget()) {
if (getHasRecommendedBudget()
!= other.getHasRecommendedBudget()) return false;
}
if (hasRecommendedBudgetAmountMicros() != other.hasRecommendedBudgetAmountMicros()) return false;
if (hasRecommendedBudgetAmountMicros()) {
if (getRecommendedBudgetAmountMicros()
!= other.getRecommendedBudgetAmountMicros()) return false;
}
if (period_ != other.period_) return false;
if (hasRecommendedBudgetEstimatedChangeWeeklyClicks() != other.hasRecommendedBudgetEstimatedChangeWeeklyClicks()) return false;
if (hasRecommendedBudgetEstimatedChangeWeeklyClicks()) {
if (getRecommendedBudgetEstimatedChangeWeeklyClicks()
!= other.getRecommendedBudgetEstimatedChangeWeeklyClicks()) return false;
}
if (hasRecommendedBudgetEstimatedChangeWeeklyCostMicros() != other.hasRecommendedBudgetEstimatedChangeWeeklyCostMicros()) return false;
if (hasRecommendedBudgetEstimatedChangeWeeklyCostMicros()) {
if (getRecommendedBudgetEstimatedChangeWeeklyCostMicros()
!= other.getRecommendedBudgetEstimatedChangeWeeklyCostMicros()) return false;
}
if (hasRecommendedBudgetEstimatedChangeWeeklyInteractions() != other.hasRecommendedBudgetEstimatedChangeWeeklyInteractions()) return false;
if (hasRecommendedBudgetEstimatedChangeWeeklyInteractions()) {
if (getRecommendedBudgetEstimatedChangeWeeklyInteractions()
!= other.getRecommendedBudgetEstimatedChangeWeeklyInteractions()) return false;
}
if (hasRecommendedBudgetEstimatedChangeWeeklyViews() != other.hasRecommendedBudgetEstimatedChangeWeeklyViews()) return false;
if (hasRecommendedBudgetEstimatedChangeWeeklyViews()) {
if (getRecommendedBudgetEstimatedChangeWeeklyViews()
!= other.getRecommendedBudgetEstimatedChangeWeeklyViews()) return false;
}
if (type_ != other.type_) return false;
if (getAlignedBiddingStrategyId()
!= other.getAlignedBiddingStrategyId()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RESOURCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getResourceName().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getId());
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasAmountMicros()) {
hash = (37 * hash) + AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAmountMicros());
}
if (hasTotalAmountMicros()) {
hash = (37 * hash) + TOTAL_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTotalAmountMicros());
}
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + DELIVERY_METHOD_FIELD_NUMBER;
hash = (53 * hash) + deliveryMethod_;
if (hasExplicitlyShared()) {
hash = (37 * hash) + EXPLICITLY_SHARED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getExplicitlyShared());
}
if (hasReferenceCount()) {
hash = (37 * hash) + REFERENCE_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getReferenceCount());
}
if (hasHasRecommendedBudget()) {
hash = (37 * hash) + HAS_RECOMMENDED_BUDGET_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getHasRecommendedBudget());
}
if (hasRecommendedBudgetAmountMicros()) {
hash = (37 * hash) + RECOMMENDED_BUDGET_AMOUNT_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRecommendedBudgetAmountMicros());
}
hash = (37 * hash) + PERIOD_FIELD_NUMBER;
hash = (53 * hash) + period_;
if (hasRecommendedBudgetEstimatedChangeWeeklyClicks()) {
hash = (37 * hash) + RECOMMENDED_BUDGET_ESTIMATED_CHANGE_WEEKLY_CLICKS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRecommendedBudgetEstimatedChangeWeeklyClicks());
}
if (hasRecommendedBudgetEstimatedChangeWeeklyCostMicros()) {
hash = (37 * hash) + RECOMMENDED_BUDGET_ESTIMATED_CHANGE_WEEKLY_COST_MICROS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRecommendedBudgetEstimatedChangeWeeklyCostMicros());
}
if (hasRecommendedBudgetEstimatedChangeWeeklyInteractions()) {
hash = (37 * hash) + RECOMMENDED_BUDGET_ESTIMATED_CHANGE_WEEKLY_INTERACTIONS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRecommendedBudgetEstimatedChangeWeeklyInteractions());
}
if (hasRecommendedBudgetEstimatedChangeWeeklyViews()) {
hash = (37 * hash) + RECOMMENDED_BUDGET_ESTIMATED_CHANGE_WEEKLY_VIEWS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRecommendedBudgetEstimatedChangeWeeklyViews());
}
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (37 * hash) + ALIGNED_BIDDING_STRATEGY_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAlignedBiddingStrategyId());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.resources.CampaignBudget parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v17.resources.CampaignBudget prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A campaign budget.
*
*
* Protobuf type {@code google.ads.googleads.v17.resources.CampaignBudget}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v17.resources.CampaignBudget)
com.google.ads.googleads.v17.resources.CampaignBudgetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.resources.CampaignBudgetProto.internal_static_google_ads_googleads_v17_resources_CampaignBudget_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.resources.CampaignBudgetProto.internal_static_google_ads_googleads_v17_resources_CampaignBudget_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.resources.CampaignBudget.class, com.google.ads.googleads.v17.resources.CampaignBudget.Builder.class);
}
// Construct using com.google.ads.googleads.v17.resources.CampaignBudget.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
resourceName_ = "";
id_ = 0L;
name_ = "";
amountMicros_ = 0L;
totalAmountMicros_ = 0L;
status_ = 0;
deliveryMethod_ = 0;
explicitlyShared_ = false;
referenceCount_ = 0L;
hasRecommendedBudget_ = false;
recommendedBudgetAmountMicros_ = 0L;
period_ = 0;
recommendedBudgetEstimatedChangeWeeklyClicks_ = 0L;
recommendedBudgetEstimatedChangeWeeklyCostMicros_ = 0L;
recommendedBudgetEstimatedChangeWeeklyInteractions_ = 0L;
recommendedBudgetEstimatedChangeWeeklyViews_ = 0L;
type_ = 0;
alignedBiddingStrategyId_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v17.resources.CampaignBudgetProto.internal_static_google_ads_googleads_v17_resources_CampaignBudget_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v17.resources.CampaignBudget getDefaultInstanceForType() {
return com.google.ads.googleads.v17.resources.CampaignBudget.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v17.resources.CampaignBudget build() {
com.google.ads.googleads.v17.resources.CampaignBudget result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v17.resources.CampaignBudget buildPartial() {
com.google.ads.googleads.v17.resources.CampaignBudget result = new com.google.ads.googleads.v17.resources.CampaignBudget(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.ads.googleads.v17.resources.CampaignBudget result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.resourceName_ = resourceName_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.amountMicros_ = amountMicros_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.totalAmountMicros_ = totalAmountMicros_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.status_ = status_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.deliveryMethod_ = deliveryMethod_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.explicitlyShared_ = explicitlyShared_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.referenceCount_ = referenceCount_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.hasRecommendedBudget_ = hasRecommendedBudget_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.recommendedBudgetAmountMicros_ = recommendedBudgetAmountMicros_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.period_ = period_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.recommendedBudgetEstimatedChangeWeeklyClicks_ = recommendedBudgetEstimatedChangeWeeklyClicks_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.recommendedBudgetEstimatedChangeWeeklyCostMicros_ = recommendedBudgetEstimatedChangeWeeklyCostMicros_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.recommendedBudgetEstimatedChangeWeeklyInteractions_ = recommendedBudgetEstimatedChangeWeeklyInteractions_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.recommendedBudgetEstimatedChangeWeeklyViews_ = recommendedBudgetEstimatedChangeWeeklyViews_;
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.type_ = type_;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.alignedBiddingStrategyId_ = alignedBiddingStrategyId_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v17.resources.CampaignBudget) {
return mergeFrom((com.google.ads.googleads.v17.resources.CampaignBudget)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v17.resources.CampaignBudget other) {
if (other == com.google.ads.googleads.v17.resources.CampaignBudget.getDefaultInstance()) return this;
if (!other.getResourceName().isEmpty()) {
resourceName_ = other.resourceName_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasAmountMicros()) {
setAmountMicros(other.getAmountMicros());
}
if (other.hasTotalAmountMicros()) {
setTotalAmountMicros(other.getTotalAmountMicros());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.deliveryMethod_ != 0) {
setDeliveryMethodValue(other.getDeliveryMethodValue());
}
if (other.hasExplicitlyShared()) {
setExplicitlyShared(other.getExplicitlyShared());
}
if (other.hasReferenceCount()) {
setReferenceCount(other.getReferenceCount());
}
if (other.hasHasRecommendedBudget()) {
setHasRecommendedBudget(other.getHasRecommendedBudget());
}
if (other.hasRecommendedBudgetAmountMicros()) {
setRecommendedBudgetAmountMicros(other.getRecommendedBudgetAmountMicros());
}
if (other.period_ != 0) {
setPeriodValue(other.getPeriodValue());
}
if (other.hasRecommendedBudgetEstimatedChangeWeeklyClicks()) {
setRecommendedBudgetEstimatedChangeWeeklyClicks(other.getRecommendedBudgetEstimatedChangeWeeklyClicks());
}
if (other.hasRecommendedBudgetEstimatedChangeWeeklyCostMicros()) {
setRecommendedBudgetEstimatedChangeWeeklyCostMicros(other.getRecommendedBudgetEstimatedChangeWeeklyCostMicros());
}
if (other.hasRecommendedBudgetEstimatedChangeWeeklyInteractions()) {
setRecommendedBudgetEstimatedChangeWeeklyInteractions(other.getRecommendedBudgetEstimatedChangeWeeklyInteractions());
}
if (other.hasRecommendedBudgetEstimatedChangeWeeklyViews()) {
setRecommendedBudgetEstimatedChangeWeeklyViews(other.getRecommendedBudgetEstimatedChangeWeeklyViews());
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.getAlignedBiddingStrategyId() != 0L) {
setAlignedBiddingStrategyId(other.getAlignedBiddingStrategyId());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
resourceName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 48: {
status_ = input.readEnum();
bitField0_ |= 0x00000020;
break;
} // case 48
case 56: {
deliveryMethod_ = input.readEnum();
bitField0_ |= 0x00000040;
break;
} // case 56
case 104: {
period_ = input.readEnum();
bitField0_ |= 0x00000800;
break;
} // case 104
case 144: {
type_ = input.readEnum();
bitField0_ |= 0x00010000;
break;
} // case 144
case 152: {
id_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 152
case 162: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 162
case 168: {
amountMicros_ = input.readInt64();
bitField0_ |= 0x00000008;
break;
} // case 168
case 176: {
totalAmountMicros_ = input.readInt64();
bitField0_ |= 0x00000010;
break;
} // case 176
case 184: {
explicitlyShared_ = input.readBool();
bitField0_ |= 0x00000080;
break;
} // case 184
case 192: {
referenceCount_ = input.readInt64();
bitField0_ |= 0x00000100;
break;
} // case 192
case 200: {
hasRecommendedBudget_ = input.readBool();
bitField0_ |= 0x00000200;
break;
} // case 200
case 208: {
recommendedBudgetAmountMicros_ = input.readInt64();
bitField0_ |= 0x00000400;
break;
} // case 208
case 216: {
recommendedBudgetEstimatedChangeWeeklyClicks_ = input.readInt64();
bitField0_ |= 0x00001000;
break;
} // case 216
case 224: {
recommendedBudgetEstimatedChangeWeeklyCostMicros_ = input.readInt64();
bitField0_ |= 0x00002000;
break;
} // case 224
case 232: {
recommendedBudgetEstimatedChangeWeeklyInteractions_ = input.readInt64();
bitField0_ |= 0x00004000;
break;
} // case 232
case 240: {
recommendedBudgetEstimatedChangeWeeklyViews_ = input.readInt64();
bitField0_ |= 0x00008000;
break;
} // case 240
case 248: {
alignedBiddingStrategyId_ = input.readInt64();
bitField0_ |= 0x00020000;
break;
} // case 248
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object resourceName_ = "";
/**
*
* Immutable. The resource name of the campaign budget.
* Campaign budget resource names have the form:
*
* `customers/{customer_id}/campaignBudgets/{campaign_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The resourceName.
*/
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Immutable. The resource name of the campaign budget.
* Campaign budget resource names have the form:
*
* `customers/{customer_id}/campaignBudgets/{campaign_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return The bytes for resourceName.
*/
public com.google.protobuf.ByteString
getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Immutable. The resource name of the campaign budget.
* Campaign budget resource names have the form:
*
* `customers/{customer_id}/campaignBudgets/{campaign_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resourceName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the campaign budget.
* Campaign budget resource names have the form:
*
* `customers/{customer_id}/campaignBudgets/{campaign_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @return This builder for chaining.
*/
public Builder clearResourceName() {
resourceName_ = getDefaultInstance().getResourceName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Immutable. The resource name of the campaign budget.
* Campaign budget resource names have the form:
*
* `customers/{customer_id}/campaignBudgets/{campaign_budget_id}`
*
*
* string resource_name = 1 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
* @param value The bytes for resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resourceName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private long id_ ;
/**
*
* Output only. The ID of the campaign budget.
*
* A campaign budget is created using the CampaignBudgetService create
* operation and is assigned a budget ID. A budget ID can be shared across
* different campaigns; the system will then allocate the campaign budget
* among different campaigns to get optimum results.
*
*
* optional int64 id = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Output only. The ID of the campaign budget.
*
* A campaign budget is created using the CampaignBudgetService create
* operation and is assigned a budget ID. A budget ID can be shared across
* different campaigns; the system will then allocate the campaign budget
* among different campaigns to get optimum results.
*
*
* optional int64 id = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
*
* Output only. The ID of the campaign budget.
*
* A campaign budget is created using the CampaignBudgetService create
* operation and is assigned a budget ID. A budget ID can be shared across
* different campaigns; the system will then allocate the campaign budget
* among different campaigns to get optimum results.
*
*
* optional int64 id = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
id_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Output only. The ID of the campaign budget.
*
* A campaign budget is created using the CampaignBudgetService create
* operation and is assigned a budget ID. A budget ID can be shared across
* different campaigns; the system will then allocate the campaign budget
* among different campaigns to get optimum results.
*
*
* optional int64 id = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000002);
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* The name of the campaign budget.
*
* When creating a campaign budget through CampaignBudgetService, every
* explicitly shared campaign budget must have a non-null, non-empty name.
* Campaign budgets that are not explicitly shared derive their name from the
* attached campaign's name.
*
* The length of this string must be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 20;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The name of the campaign budget.
*
* When creating a campaign budget through CampaignBudgetService, every
* explicitly shared campaign budget must have a non-null, non-empty name.
* Campaign budgets that are not explicitly shared derive their name from the
* attached campaign's name.
*
* The length of this string must be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 20;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the campaign budget.
*
* When creating a campaign budget through CampaignBudgetService, every
* explicitly shared campaign budget must have a non-null, non-empty name.
* Campaign budgets that are not explicitly shared derive their name from the
* attached campaign's name.
*
* The length of this string must be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 20;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the campaign budget.
*
* When creating a campaign budget through CampaignBudgetService, every
* explicitly shared campaign budget must have a non-null, non-empty name.
* Campaign budgets that are not explicitly shared derive their name from the
* attached campaign's name.
*
* The length of this string must be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 20;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The name of the campaign budget.
*
* When creating a campaign budget through CampaignBudgetService, every
* explicitly shared campaign budget must have a non-null, non-empty name.
* Campaign budgets that are not explicitly shared derive their name from the
* attached campaign's name.
*
* The length of this string must be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 20;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* The name of the campaign budget.
*
* When creating a campaign budget through CampaignBudgetService, every
* explicitly shared campaign budget must have a non-null, non-empty name.
* Campaign budgets that are not explicitly shared derive their name from the
* attached campaign's name.
*
* The length of this string must be between 1 and 255, inclusive,
* in UTF-8 bytes, (trimmed).
*
*
* optional string name = 20;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private long amountMicros_ ;
/**
*
* The amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit. Monthly spend is capped at 30.4 times this amount.
*
*
* optional int64 amount_micros = 21;
* @return Whether the amountMicros field is set.
*/
@java.lang.Override
public boolean hasAmountMicros() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit. Monthly spend is capped at 30.4 times this amount.
*
*
* optional int64 amount_micros = 21;
* @return The amountMicros.
*/
@java.lang.Override
public long getAmountMicros() {
return amountMicros_;
}
/**
*
* The amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit. Monthly spend is capped at 30.4 times this amount.
*
*
* optional int64 amount_micros = 21;
* @param value The amountMicros to set.
* @return This builder for chaining.
*/
public Builder setAmountMicros(long value) {
amountMicros_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit. Monthly spend is capped at 30.4 times this amount.
*
*
* optional int64 amount_micros = 21;
* @return This builder for chaining.
*/
public Builder clearAmountMicros() {
bitField0_ = (bitField0_ & ~0x00000008);
amountMicros_ = 0L;
onChanged();
return this;
}
private long totalAmountMicros_ ;
/**
*
* The lifetime amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit.
*
*
* optional int64 total_amount_micros = 22;
* @return Whether the totalAmountMicros field is set.
*/
@java.lang.Override
public boolean hasTotalAmountMicros() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The lifetime amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit.
*
*
* optional int64 total_amount_micros = 22;
* @return The totalAmountMicros.
*/
@java.lang.Override
public long getTotalAmountMicros() {
return totalAmountMicros_;
}
/**
*
* The lifetime amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit.
*
*
* optional int64 total_amount_micros = 22;
* @param value The totalAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setTotalAmountMicros(long value) {
totalAmountMicros_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The lifetime amount of the budget, in the local currency for the account.
* Amount is specified in micros, where one million is equivalent to one
* currency unit.
*
*
* optional int64 total_amount_micros = 22;
* @return This builder for chaining.
*/
public Builder clearTotalAmountMicros() {
bitField0_ = (bitField0_ & ~0x00000010);
totalAmountMicros_ = 0L;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Output only. The status of this campaign budget. This field is read-only.
*
*
* .google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus status = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Output only. The status of this campaign budget. This field is read-only.
*
*
* .google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus status = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Output only. The status of this campaign budget. This field is read-only.
*
*
* .google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus status = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The status.
*/
@java.lang.Override
public com.google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus getStatus() {
com.google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus result = com.google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus.forNumber(status_);
return result == null ? com.google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus.UNRECOGNIZED : result;
}
/**
*
* Output only. The status of this campaign budget. This field is read-only.
*
*
* .google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus status = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Output only. The status of this campaign budget. This field is read-only.
*
*
* .google.ads.googleads.v17.enums.BudgetStatusEnum.BudgetStatus status = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000020);
status_ = 0;
onChanged();
return this;
}
private int deliveryMethod_ = 0;
/**
*
* The delivery method that determines the rate at which the campaign budget
* is spent.
*
* Defaults to STANDARD if unspecified in a create operation.
*
*
* .google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod delivery_method = 7;
* @return The enum numeric value on the wire for deliveryMethod.
*/
@java.lang.Override public int getDeliveryMethodValue() {
return deliveryMethod_;
}
/**
*
* The delivery method that determines the rate at which the campaign budget
* is spent.
*
* Defaults to STANDARD if unspecified in a create operation.
*
*
* .google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod delivery_method = 7;
* @param value The enum numeric value on the wire for deliveryMethod to set.
* @return This builder for chaining.
*/
public Builder setDeliveryMethodValue(int value) {
deliveryMethod_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* The delivery method that determines the rate at which the campaign budget
* is spent.
*
* Defaults to STANDARD if unspecified in a create operation.
*
*
* .google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod delivery_method = 7;
* @return The deliveryMethod.
*/
@java.lang.Override
public com.google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod getDeliveryMethod() {
com.google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod result = com.google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod.forNumber(deliveryMethod_);
return result == null ? com.google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod.UNRECOGNIZED : result;
}
/**
*
* The delivery method that determines the rate at which the campaign budget
* is spent.
*
* Defaults to STANDARD if unspecified in a create operation.
*
*
* .google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod delivery_method = 7;
* @param value The deliveryMethod to set.
* @return This builder for chaining.
*/
public Builder setDeliveryMethod(com.google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
deliveryMethod_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The delivery method that determines the rate at which the campaign budget
* is spent.
*
* Defaults to STANDARD if unspecified in a create operation.
*
*
* .google.ads.googleads.v17.enums.BudgetDeliveryMethodEnum.BudgetDeliveryMethod delivery_method = 7;
* @return This builder for chaining.
*/
public Builder clearDeliveryMethod() {
bitField0_ = (bitField0_ & ~0x00000040);
deliveryMethod_ = 0;
onChanged();
return this;
}
private boolean explicitlyShared_ ;
/**
*
* Specifies whether the budget is explicitly shared. Defaults to true if
* unspecified in a create operation.
*
* If true, the budget was created with the purpose of sharing
* across one or more campaigns.
*
* If false, the budget was created with the intention of only being used
* with a single campaign. The budget's name and status will stay in sync
* with the campaign's name and status. Attempting to share the budget with a
* second campaign will result in an error.
*
* A non-shared budget can become an explicitly shared. The same operation
* must also assign the budget a name.
*
* A shared campaign budget can never become non-shared.
*
*
* optional bool explicitly_shared = 23;
* @return Whether the explicitlyShared field is set.
*/
@java.lang.Override
public boolean hasExplicitlyShared() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Specifies whether the budget is explicitly shared. Defaults to true if
* unspecified in a create operation.
*
* If true, the budget was created with the purpose of sharing
* across one or more campaigns.
*
* If false, the budget was created with the intention of only being used
* with a single campaign. The budget's name and status will stay in sync
* with the campaign's name and status. Attempting to share the budget with a
* second campaign will result in an error.
*
* A non-shared budget can become an explicitly shared. The same operation
* must also assign the budget a name.
*
* A shared campaign budget can never become non-shared.
*
*
* optional bool explicitly_shared = 23;
* @return The explicitlyShared.
*/
@java.lang.Override
public boolean getExplicitlyShared() {
return explicitlyShared_;
}
/**
*
* Specifies whether the budget is explicitly shared. Defaults to true if
* unspecified in a create operation.
*
* If true, the budget was created with the purpose of sharing
* across one or more campaigns.
*
* If false, the budget was created with the intention of only being used
* with a single campaign. The budget's name and status will stay in sync
* with the campaign's name and status. Attempting to share the budget with a
* second campaign will result in an error.
*
* A non-shared budget can become an explicitly shared. The same operation
* must also assign the budget a name.
*
* A shared campaign budget can never become non-shared.
*
*
* optional bool explicitly_shared = 23;
* @param value The explicitlyShared to set.
* @return This builder for chaining.
*/
public Builder setExplicitlyShared(boolean value) {
explicitlyShared_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Specifies whether the budget is explicitly shared. Defaults to true if
* unspecified in a create operation.
*
* If true, the budget was created with the purpose of sharing
* across one or more campaigns.
*
* If false, the budget was created with the intention of only being used
* with a single campaign. The budget's name and status will stay in sync
* with the campaign's name and status. Attempting to share the budget with a
* second campaign will result in an error.
*
* A non-shared budget can become an explicitly shared. The same operation
* must also assign the budget a name.
*
* A shared campaign budget can never become non-shared.
*
*
* optional bool explicitly_shared = 23;
* @return This builder for chaining.
*/
public Builder clearExplicitlyShared() {
bitField0_ = (bitField0_ & ~0x00000080);
explicitlyShared_ = false;
onChanged();
return this;
}
private long referenceCount_ ;
/**
*
* Output only. The number of campaigns actively using the budget.
*
* This field is read-only.
*
*
* optional int64 reference_count = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the referenceCount field is set.
*/
@java.lang.Override
public boolean hasReferenceCount() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Output only. The number of campaigns actively using the budget.
*
* This field is read-only.
*
*
* optional int64 reference_count = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The referenceCount.
*/
@java.lang.Override
public long getReferenceCount() {
return referenceCount_;
}
/**
*
* Output only. The number of campaigns actively using the budget.
*
* This field is read-only.
*
*
* optional int64 reference_count = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The referenceCount to set.
* @return This builder for chaining.
*/
public Builder setReferenceCount(long value) {
referenceCount_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* Output only. The number of campaigns actively using the budget.
*
* This field is read-only.
*
*
* optional int64 reference_count = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearReferenceCount() {
bitField0_ = (bitField0_ & ~0x00000100);
referenceCount_ = 0L;
onChanged();
return this;
}
private boolean hasRecommendedBudget_ ;
/**
*
* Output only. Indicates whether there is a recommended budget for this
* campaign budget.
*
* This field is read-only.
*
*
* optional bool has_recommended_budget = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the hasRecommendedBudget field is set.
*/
@java.lang.Override
public boolean hasHasRecommendedBudget() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Output only. Indicates whether there is a recommended budget for this
* campaign budget.
*
* This field is read-only.
*
*
* optional bool has_recommended_budget = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The hasRecommendedBudget.
*/
@java.lang.Override
public boolean getHasRecommendedBudget() {
return hasRecommendedBudget_;
}
/**
*
* Output only. Indicates whether there is a recommended budget for this
* campaign budget.
*
* This field is read-only.
*
*
* optional bool has_recommended_budget = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The hasRecommendedBudget to set.
* @return This builder for chaining.
*/
public Builder setHasRecommendedBudget(boolean value) {
hasRecommendedBudget_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* Output only. Indicates whether there is a recommended budget for this
* campaign budget.
*
* This field is read-only.
*
*
* optional bool has_recommended_budget = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearHasRecommendedBudget() {
bitField0_ = (bitField0_ & ~0x00000200);
hasRecommendedBudget_ = false;
onChanged();
return this;
}
private long recommendedBudgetAmountMicros_ ;
/**
*
* Output only. The recommended budget amount. If no recommendation is
* available, this will be set to the budget amount. Amount is specified in
* micros, where one million is equivalent to one currency unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_amount_micros = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the recommendedBudgetAmountMicros field is set.
*/
@java.lang.Override
public boolean hasRecommendedBudgetAmountMicros() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Output only. The recommended budget amount. If no recommendation is
* available, this will be set to the budget amount. Amount is specified in
* micros, where one million is equivalent to one currency unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_amount_micros = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The recommendedBudgetAmountMicros.
*/
@java.lang.Override
public long getRecommendedBudgetAmountMicros() {
return recommendedBudgetAmountMicros_;
}
/**
*
* Output only. The recommended budget amount. If no recommendation is
* available, this will be set to the budget amount. Amount is specified in
* micros, where one million is equivalent to one currency unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_amount_micros = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The recommendedBudgetAmountMicros to set.
* @return This builder for chaining.
*/
public Builder setRecommendedBudgetAmountMicros(long value) {
recommendedBudgetAmountMicros_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* Output only. The recommended budget amount. If no recommendation is
* available, this will be set to the budget amount. Amount is specified in
* micros, where one million is equivalent to one currency unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_amount_micros = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearRecommendedBudgetAmountMicros() {
bitField0_ = (bitField0_ & ~0x00000400);
recommendedBudgetAmountMicros_ = 0L;
onChanged();
return this;
}
private int period_ = 0;
/**
*
* Immutable. Period over which to spend the budget. Defaults to DAILY if not
* specified.
*
*
* .google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod period = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for period.
*/
@java.lang.Override public int getPeriodValue() {
return period_;
}
/**
*
* Immutable. Period over which to spend the budget. Defaults to DAILY if not
* specified.
*
*
* .google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod period = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for period to set.
* @return This builder for chaining.
*/
public Builder setPeriodValue(int value) {
period_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
* Immutable. Period over which to spend the budget. Defaults to DAILY if not
* specified.
*
*
* .google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod period = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return The period.
*/
@java.lang.Override
public com.google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod getPeriod() {
com.google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod result = com.google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod.forNumber(period_);
return result == null ? com.google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod.UNRECOGNIZED : result;
}
/**
*
* Immutable. Period over which to spend the budget. Defaults to DAILY if not
* specified.
*
*
* .google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod period = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The period to set.
* @return This builder for chaining.
*/
public Builder setPeriod(com.google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
period_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. Period over which to spend the budget. Defaults to DAILY if not
* specified.
*
*
* .google.ads.googleads.v17.enums.BudgetPeriodEnum.BudgetPeriod period = 13 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearPeriod() {
bitField0_ = (bitField0_ & ~0x00000800);
period_ = 0;
onChanged();
return this;
}
private long recommendedBudgetEstimatedChangeWeeklyClicks_ ;
/**
*
* Output only. The estimated change in weekly clicks if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_clicks = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the recommendedBudgetEstimatedChangeWeeklyClicks field is set.
*/
@java.lang.Override
public boolean hasRecommendedBudgetEstimatedChangeWeeklyClicks() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* Output only. The estimated change in weekly clicks if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_clicks = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The recommendedBudgetEstimatedChangeWeeklyClicks.
*/
@java.lang.Override
public long getRecommendedBudgetEstimatedChangeWeeklyClicks() {
return recommendedBudgetEstimatedChangeWeeklyClicks_;
}
/**
*
* Output only. The estimated change in weekly clicks if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_clicks = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The recommendedBudgetEstimatedChangeWeeklyClicks to set.
* @return This builder for chaining.
*/
public Builder setRecommendedBudgetEstimatedChangeWeeklyClicks(long value) {
recommendedBudgetEstimatedChangeWeeklyClicks_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* Output only. The estimated change in weekly clicks if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_clicks = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearRecommendedBudgetEstimatedChangeWeeklyClicks() {
bitField0_ = (bitField0_ & ~0x00001000);
recommendedBudgetEstimatedChangeWeeklyClicks_ = 0L;
onChanged();
return this;
}
private long recommendedBudgetEstimatedChangeWeeklyCostMicros_ ;
/**
*
* Output only. The estimated change in weekly cost in micros if the
* recommended budget is applied. One million is equivalent to one currency
* unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_cost_micros = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the recommendedBudgetEstimatedChangeWeeklyCostMicros field is set.
*/
@java.lang.Override
public boolean hasRecommendedBudgetEstimatedChangeWeeklyCostMicros() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
* Output only. The estimated change in weekly cost in micros if the
* recommended budget is applied. One million is equivalent to one currency
* unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_cost_micros = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The recommendedBudgetEstimatedChangeWeeklyCostMicros.
*/
@java.lang.Override
public long getRecommendedBudgetEstimatedChangeWeeklyCostMicros() {
return recommendedBudgetEstimatedChangeWeeklyCostMicros_;
}
/**
*
* Output only. The estimated change in weekly cost in micros if the
* recommended budget is applied. One million is equivalent to one currency
* unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_cost_micros = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The recommendedBudgetEstimatedChangeWeeklyCostMicros to set.
* @return This builder for chaining.
*/
public Builder setRecommendedBudgetEstimatedChangeWeeklyCostMicros(long value) {
recommendedBudgetEstimatedChangeWeeklyCostMicros_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* Output only. The estimated change in weekly cost in micros if the
* recommended budget is applied. One million is equivalent to one currency
* unit.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_cost_micros = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearRecommendedBudgetEstimatedChangeWeeklyCostMicros() {
bitField0_ = (bitField0_ & ~0x00002000);
recommendedBudgetEstimatedChangeWeeklyCostMicros_ = 0L;
onChanged();
return this;
}
private long recommendedBudgetEstimatedChangeWeeklyInteractions_ ;
/**
*
* Output only. The estimated change in weekly interactions if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_interactions = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the recommendedBudgetEstimatedChangeWeeklyInteractions field is set.
*/
@java.lang.Override
public boolean hasRecommendedBudgetEstimatedChangeWeeklyInteractions() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
* Output only. The estimated change in weekly interactions if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_interactions = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The recommendedBudgetEstimatedChangeWeeklyInteractions.
*/
@java.lang.Override
public long getRecommendedBudgetEstimatedChangeWeeklyInteractions() {
return recommendedBudgetEstimatedChangeWeeklyInteractions_;
}
/**
*
* Output only. The estimated change in weekly interactions if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_interactions = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The recommendedBudgetEstimatedChangeWeeklyInteractions to set.
* @return This builder for chaining.
*/
public Builder setRecommendedBudgetEstimatedChangeWeeklyInteractions(long value) {
recommendedBudgetEstimatedChangeWeeklyInteractions_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
* Output only. The estimated change in weekly interactions if the recommended
* budget is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_interactions = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearRecommendedBudgetEstimatedChangeWeeklyInteractions() {
bitField0_ = (bitField0_ & ~0x00004000);
recommendedBudgetEstimatedChangeWeeklyInteractions_ = 0L;
onChanged();
return this;
}
private long recommendedBudgetEstimatedChangeWeeklyViews_ ;
/**
*
* Output only. The estimated change in weekly views if the recommended budget
* is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_views = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return Whether the recommendedBudgetEstimatedChangeWeeklyViews field is set.
*/
@java.lang.Override
public boolean hasRecommendedBudgetEstimatedChangeWeeklyViews() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
* Output only. The estimated change in weekly views if the recommended budget
* is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_views = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return The recommendedBudgetEstimatedChangeWeeklyViews.
*/
@java.lang.Override
public long getRecommendedBudgetEstimatedChangeWeeklyViews() {
return recommendedBudgetEstimatedChangeWeeklyViews_;
}
/**
*
* Output only. The estimated change in weekly views if the recommended budget
* is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_views = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @param value The recommendedBudgetEstimatedChangeWeeklyViews to set.
* @return This builder for chaining.
*/
public Builder setRecommendedBudgetEstimatedChangeWeeklyViews(long value) {
recommendedBudgetEstimatedChangeWeeklyViews_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
* Output only. The estimated change in weekly views if the recommended budget
* is applied.
*
* This field is read-only.
*
*
* optional int64 recommended_budget_estimated_change_weekly_views = 30 [(.google.api.field_behavior) = OUTPUT_ONLY];
* @return This builder for chaining.
*/
public Builder clearRecommendedBudgetEstimatedChangeWeeklyViews() {
bitField0_ = (bitField0_ & ~0x00008000);
recommendedBudgetEstimatedChangeWeeklyViews_ = 0L;
onChanged();
return this;
}
private int type_ = 0;
/**
*
* Immutable. The type of the campaign budget.
*
*
* .google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType type = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
*
* Immutable. The type of the campaign budget.
*
*
* .google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType type = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
* Immutable. The type of the campaign budget.
*
*
* .google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType type = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return The type.
*/
@java.lang.Override
public com.google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType getType() {
com.google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType result = com.google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType.forNumber(type_);
return result == null ? com.google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType.UNRECOGNIZED : result;
}
/**
*
* Immutable. The type of the campaign budget.
*
*
* .google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType type = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(com.google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Immutable. The type of the campaign budget.
*
*
* .google.ads.googleads.v17.enums.BudgetTypeEnum.BudgetType type = 18 [(.google.api.field_behavior) = IMMUTABLE];
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00010000);
type_ = 0;
onChanged();
return this;
}
private long alignedBiddingStrategyId_ ;
/**
*
* ID of the portfolio bidding strategy that this shared campaign budget
* is aligned with. When a bidding strategy and a campaign budget are aligned,
* they are attached to the same set of campaigns. After a campaign budget is
* aligned with a bidding strategy, campaigns that are added to the campaign
* budget must also use the aligned bidding strategy.
*
*
* int64 aligned_bidding_strategy_id = 31;
* @return The alignedBiddingStrategyId.
*/
@java.lang.Override
public long getAlignedBiddingStrategyId() {
return alignedBiddingStrategyId_;
}
/**
*
* ID of the portfolio bidding strategy that this shared campaign budget
* is aligned with. When a bidding strategy and a campaign budget are aligned,
* they are attached to the same set of campaigns. After a campaign budget is
* aligned with a bidding strategy, campaigns that are added to the campaign
* budget must also use the aligned bidding strategy.
*
*
* int64 aligned_bidding_strategy_id = 31;
* @param value The alignedBiddingStrategyId to set.
* @return This builder for chaining.
*/
public Builder setAlignedBiddingStrategyId(long value) {
alignedBiddingStrategyId_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
* ID of the portfolio bidding strategy that this shared campaign budget
* is aligned with. When a bidding strategy and a campaign budget are aligned,
* they are attached to the same set of campaigns. After a campaign budget is
* aligned with a bidding strategy, campaigns that are added to the campaign
* budget must also use the aligned bidding strategy.
*
*
* int64 aligned_bidding_strategy_id = 31;
* @return This builder for chaining.
*/
public Builder clearAlignedBiddingStrategyId() {
bitField0_ = (bitField0_ & ~0x00020000);
alignedBiddingStrategyId_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v17.resources.CampaignBudget)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v17.resources.CampaignBudget)
private static final com.google.ads.googleads.v17.resources.CampaignBudget DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v17.resources.CampaignBudget();
}
public static com.google.ads.googleads.v17.resources.CampaignBudget getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CampaignBudget parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v17.resources.CampaignBudget getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy