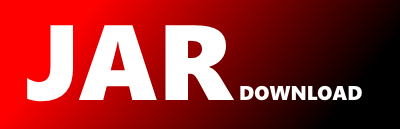
com.google.ads.googleads.v17.resources.FeedItemAttributeValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v17 Show documentation
Show all versions of google-ads-stubs-v17 Show documentation
Stubs for GAAPI version google-ads-stubs-v17
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v17/resources/feed_item.proto
// Protobuf Java Version: 3.25.3
package com.google.ads.googleads.v17.resources;
/**
*
* A feed item attribute value.
*
*
* Protobuf type {@code google.ads.googleads.v17.resources.FeedItemAttributeValue}
*/
public final class FeedItemAttributeValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v17.resources.FeedItemAttributeValue)
FeedItemAttributeValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use FeedItemAttributeValue.newBuilder() to construct.
private FeedItemAttributeValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FeedItemAttributeValue() {
stringValue_ = "";
integerValues_ = emptyLongList();
booleanValues_ = emptyBooleanList();
stringValues_ =
com.google.protobuf.LazyStringArrayList.emptyList();
doubleValues_ = emptyDoubleList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FeedItemAttributeValue();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.resources.FeedItemProto.internal_static_google_ads_googleads_v17_resources_FeedItemAttributeValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.resources.FeedItemProto.internal_static_google_ads_googleads_v17_resources_FeedItemAttributeValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.resources.FeedItemAttributeValue.class, com.google.ads.googleads.v17.resources.FeedItemAttributeValue.Builder.class);
}
private int bitField0_;
public static final int FEED_ATTRIBUTE_ID_FIELD_NUMBER = 11;
private long feedAttributeId_ = 0L;
/**
*
* Id of the feed attribute for which the value is associated with.
*
*
* optional int64 feed_attribute_id = 11;
* @return Whether the feedAttributeId field is set.
*/
@java.lang.Override
public boolean hasFeedAttributeId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Id of the feed attribute for which the value is associated with.
*
*
* optional int64 feed_attribute_id = 11;
* @return The feedAttributeId.
*/
@java.lang.Override
public long getFeedAttributeId() {
return feedAttributeId_;
}
public static final int INTEGER_VALUE_FIELD_NUMBER = 12;
private long integerValue_ = 0L;
/**
*
* Int64 value. Should be set if feed_attribute_id refers to a feed attribute
* of type INT64.
*
*
* optional int64 integer_value = 12;
* @return Whether the integerValue field is set.
*/
@java.lang.Override
public boolean hasIntegerValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Int64 value. Should be set if feed_attribute_id refers to a feed attribute
* of type INT64.
*
*
* optional int64 integer_value = 12;
* @return The integerValue.
*/
@java.lang.Override
public long getIntegerValue() {
return integerValue_;
}
public static final int BOOLEAN_VALUE_FIELD_NUMBER = 13;
private boolean booleanValue_ = false;
/**
*
* Bool value. Should be set if feed_attribute_id refers to a feed attribute
* of type BOOLEAN.
*
*
* optional bool boolean_value = 13;
* @return Whether the booleanValue field is set.
*/
@java.lang.Override
public boolean hasBooleanValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Bool value. Should be set if feed_attribute_id refers to a feed attribute
* of type BOOLEAN.
*
*
* optional bool boolean_value = 13;
* @return The booleanValue.
*/
@java.lang.Override
public boolean getBooleanValue() {
return booleanValue_;
}
public static final int STRING_VALUE_FIELD_NUMBER = 14;
@SuppressWarnings("serial")
private volatile java.lang.Object stringValue_ = "";
/**
*
* String value. Should be set if feed_attribute_id refers to a feed attribute
* of type STRING, URL or DATE_TIME.
* For STRING the maximum length is 1500 characters. For URL the maximum
* length is 2076 characters. For DATE_TIME the string must be in the format
* "YYYYMMDD HHMMSS".
*
*
* optional string string_value = 14;
* @return Whether the stringValue field is set.
*/
@java.lang.Override
public boolean hasStringValue() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* String value. Should be set if feed_attribute_id refers to a feed attribute
* of type STRING, URL or DATE_TIME.
* For STRING the maximum length is 1500 characters. For URL the maximum
* length is 2076 characters. For DATE_TIME the string must be in the format
* "YYYYMMDD HHMMSS".
*
*
* optional string string_value = 14;
* @return The stringValue.
*/
@java.lang.Override
public java.lang.String getStringValue() {
java.lang.Object ref = stringValue_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stringValue_ = s;
return s;
}
}
/**
*
* String value. Should be set if feed_attribute_id refers to a feed attribute
* of type STRING, URL or DATE_TIME.
* For STRING the maximum length is 1500 characters. For URL the maximum
* length is 2076 characters. For DATE_TIME the string must be in the format
* "YYYYMMDD HHMMSS".
*
*
* optional string string_value = 14;
* @return The bytes for stringValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStringValueBytes() {
java.lang.Object ref = stringValue_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stringValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DOUBLE_VALUE_FIELD_NUMBER = 15;
private double doubleValue_ = 0D;
/**
*
* Double value. Should be set if feed_attribute_id refers to a feed attribute
* of type DOUBLE.
*
*
* optional double double_value = 15;
* @return Whether the doubleValue field is set.
*/
@java.lang.Override
public boolean hasDoubleValue() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Double value. Should be set if feed_attribute_id refers to a feed attribute
* of type DOUBLE.
*
*
* optional double double_value = 15;
* @return The doubleValue.
*/
@java.lang.Override
public double getDoubleValue() {
return doubleValue_;
}
public static final int PRICE_VALUE_FIELD_NUMBER = 6;
private com.google.ads.googleads.v17.common.Money priceValue_;
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
* @return Whether the priceValue field is set.
*/
@java.lang.Override
public boolean hasPriceValue() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
* @return The priceValue.
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.Money getPriceValue() {
return priceValue_ == null ? com.google.ads.googleads.v17.common.Money.getDefaultInstance() : priceValue_;
}
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.MoneyOrBuilder getPriceValueOrBuilder() {
return priceValue_ == null ? com.google.ads.googleads.v17.common.Money.getDefaultInstance() : priceValue_;
}
public static final int INTEGER_VALUES_FIELD_NUMBER = 16;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.LongList integerValues_ =
emptyLongList();
/**
*
* Repeated int64 value. Should be set if feed_attribute_id refers to a feed
* attribute of type INT64_LIST.
*
*
* repeated int64 integer_values = 16;
* @return A list containing the integerValues.
*/
@java.lang.Override
public java.util.List
getIntegerValuesList() {
return integerValues_;
}
/**
*
* Repeated int64 value. Should be set if feed_attribute_id refers to a feed
* attribute of type INT64_LIST.
*
*
* repeated int64 integer_values = 16;
* @return The count of integerValues.
*/
public int getIntegerValuesCount() {
return integerValues_.size();
}
/**
*
* Repeated int64 value. Should be set if feed_attribute_id refers to a feed
* attribute of type INT64_LIST.
*
*
* repeated int64 integer_values = 16;
* @param index The index of the element to return.
* @return The integerValues at the given index.
*/
public long getIntegerValues(int index) {
return integerValues_.getLong(index);
}
private int integerValuesMemoizedSerializedSize = -1;
public static final int BOOLEAN_VALUES_FIELD_NUMBER = 17;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.BooleanList booleanValues_ =
emptyBooleanList();
/**
*
* Repeated bool value. Should be set if feed_attribute_id refers to a feed
* attribute of type BOOLEAN_LIST.
*
*
* repeated bool boolean_values = 17;
* @return A list containing the booleanValues.
*/
@java.lang.Override
public java.util.List
getBooleanValuesList() {
return booleanValues_;
}
/**
*
* Repeated bool value. Should be set if feed_attribute_id refers to a feed
* attribute of type BOOLEAN_LIST.
*
*
* repeated bool boolean_values = 17;
* @return The count of booleanValues.
*/
public int getBooleanValuesCount() {
return booleanValues_.size();
}
/**
*
* Repeated bool value. Should be set if feed_attribute_id refers to a feed
* attribute of type BOOLEAN_LIST.
*
*
* repeated bool boolean_values = 17;
* @param index The index of the element to return.
* @return The booleanValues at the given index.
*/
public boolean getBooleanValues(int index) {
return booleanValues_.getBoolean(index);
}
private int booleanValuesMemoizedSerializedSize = -1;
public static final int STRING_VALUES_FIELD_NUMBER = 18;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList stringValues_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @return A list containing the stringValues.
*/
public com.google.protobuf.ProtocolStringList
getStringValuesList() {
return stringValues_;
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @return The count of stringValues.
*/
public int getStringValuesCount() {
return stringValues_.size();
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @param index The index of the element to return.
* @return The stringValues at the given index.
*/
public java.lang.String getStringValues(int index) {
return stringValues_.get(index);
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @param index The index of the value to return.
* @return The bytes of the stringValues at the given index.
*/
public com.google.protobuf.ByteString
getStringValuesBytes(int index) {
return stringValues_.getByteString(index);
}
public static final int DOUBLE_VALUES_FIELD_NUMBER = 19;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.DoubleList doubleValues_ =
emptyDoubleList();
/**
*
* Repeated double value. Should be set if feed_attribute_id refers to a feed
* attribute of type DOUBLE_LIST.
*
*
* repeated double double_values = 19;
* @return A list containing the doubleValues.
*/
@java.lang.Override
public java.util.List
getDoubleValuesList() {
return doubleValues_;
}
/**
*
* Repeated double value. Should be set if feed_attribute_id refers to a feed
* attribute of type DOUBLE_LIST.
*
*
* repeated double double_values = 19;
* @return The count of doubleValues.
*/
public int getDoubleValuesCount() {
return doubleValues_.size();
}
/**
*
* Repeated double value. Should be set if feed_attribute_id refers to a feed
* attribute of type DOUBLE_LIST.
*
*
* repeated double double_values = 19;
* @param index The index of the element to return.
* @return The doubleValues at the given index.
*/
public double getDoubleValues(int index) {
return doubleValues_.getDouble(index);
}
private int doubleValuesMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(6, getPriceValue());
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(11, feedAttributeId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(12, integerValue_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(13, booleanValue_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, stringValue_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeDouble(15, doubleValue_);
}
if (getIntegerValuesList().size() > 0) {
output.writeUInt32NoTag(130);
output.writeUInt32NoTag(integerValuesMemoizedSerializedSize);
}
for (int i = 0; i < integerValues_.size(); i++) {
output.writeInt64NoTag(integerValues_.getLong(i));
}
if (getBooleanValuesList().size() > 0) {
output.writeUInt32NoTag(138);
output.writeUInt32NoTag(booleanValuesMemoizedSerializedSize);
}
for (int i = 0; i < booleanValues_.size(); i++) {
output.writeBoolNoTag(booleanValues_.getBoolean(i));
}
for (int i = 0; i < stringValues_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 18, stringValues_.getRaw(i));
}
if (getDoubleValuesList().size() > 0) {
output.writeUInt32NoTag(154);
output.writeUInt32NoTag(doubleValuesMemoizedSerializedSize);
}
for (int i = 0; i < doubleValues_.size(); i++) {
output.writeDoubleNoTag(doubleValues_.getDouble(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getPriceValue());
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(11, feedAttributeId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(12, integerValue_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, booleanValue_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, stringValue_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(15, doubleValue_);
}
{
int dataSize = 0;
for (int i = 0; i < integerValues_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(integerValues_.getLong(i));
}
size += dataSize;
if (!getIntegerValuesList().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
integerValuesMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
dataSize = 1 * getBooleanValuesList().size();
size += dataSize;
if (!getBooleanValuesList().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
booleanValuesMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < stringValues_.size(); i++) {
dataSize += computeStringSizeNoTag(stringValues_.getRaw(i));
}
size += dataSize;
size += 2 * getStringValuesList().size();
}
{
int dataSize = 0;
dataSize = 8 * getDoubleValuesList().size();
size += dataSize;
if (!getDoubleValuesList().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
doubleValuesMemoizedSerializedSize = dataSize;
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v17.resources.FeedItemAttributeValue)) {
return super.equals(obj);
}
com.google.ads.googleads.v17.resources.FeedItemAttributeValue other = (com.google.ads.googleads.v17.resources.FeedItemAttributeValue) obj;
if (hasFeedAttributeId() != other.hasFeedAttributeId()) return false;
if (hasFeedAttributeId()) {
if (getFeedAttributeId()
!= other.getFeedAttributeId()) return false;
}
if (hasIntegerValue() != other.hasIntegerValue()) return false;
if (hasIntegerValue()) {
if (getIntegerValue()
!= other.getIntegerValue()) return false;
}
if (hasBooleanValue() != other.hasBooleanValue()) return false;
if (hasBooleanValue()) {
if (getBooleanValue()
!= other.getBooleanValue()) return false;
}
if (hasStringValue() != other.hasStringValue()) return false;
if (hasStringValue()) {
if (!getStringValue()
.equals(other.getStringValue())) return false;
}
if (hasDoubleValue() != other.hasDoubleValue()) return false;
if (hasDoubleValue()) {
if (java.lang.Double.doubleToLongBits(getDoubleValue())
!= java.lang.Double.doubleToLongBits(
other.getDoubleValue())) return false;
}
if (hasPriceValue() != other.hasPriceValue()) return false;
if (hasPriceValue()) {
if (!getPriceValue()
.equals(other.getPriceValue())) return false;
}
if (!getIntegerValuesList()
.equals(other.getIntegerValuesList())) return false;
if (!getBooleanValuesList()
.equals(other.getBooleanValuesList())) return false;
if (!getStringValuesList()
.equals(other.getStringValuesList())) return false;
if (!getDoubleValuesList()
.equals(other.getDoubleValuesList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFeedAttributeId()) {
hash = (37 * hash) + FEED_ATTRIBUTE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFeedAttributeId());
}
if (hasIntegerValue()) {
hash = (37 * hash) + INTEGER_VALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getIntegerValue());
}
if (hasBooleanValue()) {
hash = (37 * hash) + BOOLEAN_VALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getBooleanValue());
}
if (hasStringValue()) {
hash = (37 * hash) + STRING_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getStringValue().hashCode();
}
if (hasDoubleValue()) {
hash = (37 * hash) + DOUBLE_VALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getDoubleValue()));
}
if (hasPriceValue()) {
hash = (37 * hash) + PRICE_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getPriceValue().hashCode();
}
if (getIntegerValuesCount() > 0) {
hash = (37 * hash) + INTEGER_VALUES_FIELD_NUMBER;
hash = (53 * hash) + getIntegerValuesList().hashCode();
}
if (getBooleanValuesCount() > 0) {
hash = (37 * hash) + BOOLEAN_VALUES_FIELD_NUMBER;
hash = (53 * hash) + getBooleanValuesList().hashCode();
}
if (getStringValuesCount() > 0) {
hash = (37 * hash) + STRING_VALUES_FIELD_NUMBER;
hash = (53 * hash) + getStringValuesList().hashCode();
}
if (getDoubleValuesCount() > 0) {
hash = (37 * hash) + DOUBLE_VALUES_FIELD_NUMBER;
hash = (53 * hash) + getDoubleValuesList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v17.resources.FeedItemAttributeValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A feed item attribute value.
*
*
* Protobuf type {@code google.ads.googleads.v17.resources.FeedItemAttributeValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v17.resources.FeedItemAttributeValue)
com.google.ads.googleads.v17.resources.FeedItemAttributeValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.resources.FeedItemProto.internal_static_google_ads_googleads_v17_resources_FeedItemAttributeValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.resources.FeedItemProto.internal_static_google_ads_googleads_v17_resources_FeedItemAttributeValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.resources.FeedItemAttributeValue.class, com.google.ads.googleads.v17.resources.FeedItemAttributeValue.Builder.class);
}
// Construct using com.google.ads.googleads.v17.resources.FeedItemAttributeValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getPriceValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
feedAttributeId_ = 0L;
integerValue_ = 0L;
booleanValue_ = false;
stringValue_ = "";
doubleValue_ = 0D;
priceValue_ = null;
if (priceValueBuilder_ != null) {
priceValueBuilder_.dispose();
priceValueBuilder_ = null;
}
integerValues_ = emptyLongList();
booleanValues_ = emptyBooleanList();
stringValues_ =
com.google.protobuf.LazyStringArrayList.emptyList();
doubleValues_ = emptyDoubleList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v17.resources.FeedItemProto.internal_static_google_ads_googleads_v17_resources_FeedItemAttributeValue_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v17.resources.FeedItemAttributeValue getDefaultInstanceForType() {
return com.google.ads.googleads.v17.resources.FeedItemAttributeValue.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v17.resources.FeedItemAttributeValue build() {
com.google.ads.googleads.v17.resources.FeedItemAttributeValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v17.resources.FeedItemAttributeValue buildPartial() {
com.google.ads.googleads.v17.resources.FeedItemAttributeValue result = new com.google.ads.googleads.v17.resources.FeedItemAttributeValue(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.ads.googleads.v17.resources.FeedItemAttributeValue result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.feedAttributeId_ = feedAttributeId_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.integerValue_ = integerValue_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.booleanValue_ = booleanValue_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.stringValue_ = stringValue_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.doubleValue_ = doubleValue_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.priceValue_ = priceValueBuilder_ == null
? priceValue_
: priceValueBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
integerValues_.makeImmutable();
result.integerValues_ = integerValues_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
booleanValues_.makeImmutable();
result.booleanValues_ = booleanValues_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
stringValues_.makeImmutable();
result.stringValues_ = stringValues_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
doubleValues_.makeImmutable();
result.doubleValues_ = doubleValues_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v17.resources.FeedItemAttributeValue) {
return mergeFrom((com.google.ads.googleads.v17.resources.FeedItemAttributeValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v17.resources.FeedItemAttributeValue other) {
if (other == com.google.ads.googleads.v17.resources.FeedItemAttributeValue.getDefaultInstance()) return this;
if (other.hasFeedAttributeId()) {
setFeedAttributeId(other.getFeedAttributeId());
}
if (other.hasIntegerValue()) {
setIntegerValue(other.getIntegerValue());
}
if (other.hasBooleanValue()) {
setBooleanValue(other.getBooleanValue());
}
if (other.hasStringValue()) {
stringValue_ = other.stringValue_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasDoubleValue()) {
setDoubleValue(other.getDoubleValue());
}
if (other.hasPriceValue()) {
mergePriceValue(other.getPriceValue());
}
if (!other.integerValues_.isEmpty()) {
if (integerValues_.isEmpty()) {
integerValues_ = other.integerValues_;
integerValues_.makeImmutable();
bitField0_ |= 0x00000040;
} else {
ensureIntegerValuesIsMutable();
integerValues_.addAll(other.integerValues_);
}
onChanged();
}
if (!other.booleanValues_.isEmpty()) {
if (booleanValues_.isEmpty()) {
booleanValues_ = other.booleanValues_;
booleanValues_.makeImmutable();
bitField0_ |= 0x00000080;
} else {
ensureBooleanValuesIsMutable();
booleanValues_.addAll(other.booleanValues_);
}
onChanged();
}
if (!other.stringValues_.isEmpty()) {
if (stringValues_.isEmpty()) {
stringValues_ = other.stringValues_;
bitField0_ |= 0x00000100;
} else {
ensureStringValuesIsMutable();
stringValues_.addAll(other.stringValues_);
}
onChanged();
}
if (!other.doubleValues_.isEmpty()) {
if (doubleValues_.isEmpty()) {
doubleValues_ = other.doubleValues_;
doubleValues_.makeImmutable();
bitField0_ |= 0x00000200;
} else {
ensureDoubleValuesIsMutable();
doubleValues_.addAll(other.doubleValues_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 50: {
input.readMessage(
getPriceValueFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 50
case 88: {
feedAttributeId_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 88
case 96: {
integerValue_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 96
case 104: {
booleanValue_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case 104
case 114: {
stringValue_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 114
case 121: {
doubleValue_ = input.readDouble();
bitField0_ |= 0x00000010;
break;
} // case 121
case 128: {
long v = input.readInt64();
ensureIntegerValuesIsMutable();
integerValues_.addLong(v);
break;
} // case 128
case 130: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensureIntegerValuesIsMutable();
while (input.getBytesUntilLimit() > 0) {
integerValues_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
} // case 130
case 136: {
boolean v = input.readBool();
ensureBooleanValuesIsMutable();
booleanValues_.addBoolean(v);
break;
} // case 136
case 138: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
int alloc = length > 4096 ? 4096 : length;
ensureBooleanValuesIsMutable(alloc / 1);
while (input.getBytesUntilLimit() > 0) {
booleanValues_.addBoolean(input.readBool());
}
input.popLimit(limit);
break;
} // case 138
case 146: {
java.lang.String s = input.readStringRequireUtf8();
ensureStringValuesIsMutable();
stringValues_.add(s);
break;
} // case 146
case 153: {
double v = input.readDouble();
ensureDoubleValuesIsMutable();
doubleValues_.addDouble(v);
break;
} // case 153
case 154: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
int alloc = length > 4096 ? 4096 : length;
ensureDoubleValuesIsMutable(alloc / 8);
while (input.getBytesUntilLimit() > 0) {
doubleValues_.addDouble(input.readDouble());
}
input.popLimit(limit);
break;
} // case 154
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long feedAttributeId_ ;
/**
*
* Id of the feed attribute for which the value is associated with.
*
*
* optional int64 feed_attribute_id = 11;
* @return Whether the feedAttributeId field is set.
*/
@java.lang.Override
public boolean hasFeedAttributeId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Id of the feed attribute for which the value is associated with.
*
*
* optional int64 feed_attribute_id = 11;
* @return The feedAttributeId.
*/
@java.lang.Override
public long getFeedAttributeId() {
return feedAttributeId_;
}
/**
*
* Id of the feed attribute for which the value is associated with.
*
*
* optional int64 feed_attribute_id = 11;
* @param value The feedAttributeId to set.
* @return This builder for chaining.
*/
public Builder setFeedAttributeId(long value) {
feedAttributeId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Id of the feed attribute for which the value is associated with.
*
*
* optional int64 feed_attribute_id = 11;
* @return This builder for chaining.
*/
public Builder clearFeedAttributeId() {
bitField0_ = (bitField0_ & ~0x00000001);
feedAttributeId_ = 0L;
onChanged();
return this;
}
private long integerValue_ ;
/**
*
* Int64 value. Should be set if feed_attribute_id refers to a feed attribute
* of type INT64.
*
*
* optional int64 integer_value = 12;
* @return Whether the integerValue field is set.
*/
@java.lang.Override
public boolean hasIntegerValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Int64 value. Should be set if feed_attribute_id refers to a feed attribute
* of type INT64.
*
*
* optional int64 integer_value = 12;
* @return The integerValue.
*/
@java.lang.Override
public long getIntegerValue() {
return integerValue_;
}
/**
*
* Int64 value. Should be set if feed_attribute_id refers to a feed attribute
* of type INT64.
*
*
* optional int64 integer_value = 12;
* @param value The integerValue to set.
* @return This builder for chaining.
*/
public Builder setIntegerValue(long value) {
integerValue_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Int64 value. Should be set if feed_attribute_id refers to a feed attribute
* of type INT64.
*
*
* optional int64 integer_value = 12;
* @return This builder for chaining.
*/
public Builder clearIntegerValue() {
bitField0_ = (bitField0_ & ~0x00000002);
integerValue_ = 0L;
onChanged();
return this;
}
private boolean booleanValue_ ;
/**
*
* Bool value. Should be set if feed_attribute_id refers to a feed attribute
* of type BOOLEAN.
*
*
* optional bool boolean_value = 13;
* @return Whether the booleanValue field is set.
*/
@java.lang.Override
public boolean hasBooleanValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Bool value. Should be set if feed_attribute_id refers to a feed attribute
* of type BOOLEAN.
*
*
* optional bool boolean_value = 13;
* @return The booleanValue.
*/
@java.lang.Override
public boolean getBooleanValue() {
return booleanValue_;
}
/**
*
* Bool value. Should be set if feed_attribute_id refers to a feed attribute
* of type BOOLEAN.
*
*
* optional bool boolean_value = 13;
* @param value The booleanValue to set.
* @return This builder for chaining.
*/
public Builder setBooleanValue(boolean value) {
booleanValue_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Bool value. Should be set if feed_attribute_id refers to a feed attribute
* of type BOOLEAN.
*
*
* optional bool boolean_value = 13;
* @return This builder for chaining.
*/
public Builder clearBooleanValue() {
bitField0_ = (bitField0_ & ~0x00000004);
booleanValue_ = false;
onChanged();
return this;
}
private java.lang.Object stringValue_ = "";
/**
*
* String value. Should be set if feed_attribute_id refers to a feed attribute
* of type STRING, URL or DATE_TIME.
* For STRING the maximum length is 1500 characters. For URL the maximum
* length is 2076 characters. For DATE_TIME the string must be in the format
* "YYYYMMDD HHMMSS".
*
*
* optional string string_value = 14;
* @return Whether the stringValue field is set.
*/
public boolean hasStringValue() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* String value. Should be set if feed_attribute_id refers to a feed attribute
* of type STRING, URL or DATE_TIME.
* For STRING the maximum length is 1500 characters. For URL the maximum
* length is 2076 characters. For DATE_TIME the string must be in the format
* "YYYYMMDD HHMMSS".
*
*
* optional string string_value = 14;
* @return The stringValue.
*/
public java.lang.String getStringValue() {
java.lang.Object ref = stringValue_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stringValue_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* String value. Should be set if feed_attribute_id refers to a feed attribute
* of type STRING, URL or DATE_TIME.
* For STRING the maximum length is 1500 characters. For URL the maximum
* length is 2076 characters. For DATE_TIME the string must be in the format
* "YYYYMMDD HHMMSS".
*
*
* optional string string_value = 14;
* @return The bytes for stringValue.
*/
public com.google.protobuf.ByteString
getStringValueBytes() {
java.lang.Object ref = stringValue_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stringValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* String value. Should be set if feed_attribute_id refers to a feed attribute
* of type STRING, URL or DATE_TIME.
* For STRING the maximum length is 1500 characters. For URL the maximum
* length is 2076 characters. For DATE_TIME the string must be in the format
* "YYYYMMDD HHMMSS".
*
*
* optional string string_value = 14;
* @param value The stringValue to set.
* @return This builder for chaining.
*/
public Builder setStringValue(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
stringValue_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* String value. Should be set if feed_attribute_id refers to a feed attribute
* of type STRING, URL or DATE_TIME.
* For STRING the maximum length is 1500 characters. For URL the maximum
* length is 2076 characters. For DATE_TIME the string must be in the format
* "YYYYMMDD HHMMSS".
*
*
* optional string string_value = 14;
* @return This builder for chaining.
*/
public Builder clearStringValue() {
stringValue_ = getDefaultInstance().getStringValue();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
* String value. Should be set if feed_attribute_id refers to a feed attribute
* of type STRING, URL or DATE_TIME.
* For STRING the maximum length is 1500 characters. For URL the maximum
* length is 2076 characters. For DATE_TIME the string must be in the format
* "YYYYMMDD HHMMSS".
*
*
* optional string string_value = 14;
* @param value The bytes for stringValue to set.
* @return This builder for chaining.
*/
public Builder setStringValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
stringValue_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private double doubleValue_ ;
/**
*
* Double value. Should be set if feed_attribute_id refers to a feed attribute
* of type DOUBLE.
*
*
* optional double double_value = 15;
* @return Whether the doubleValue field is set.
*/
@java.lang.Override
public boolean hasDoubleValue() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Double value. Should be set if feed_attribute_id refers to a feed attribute
* of type DOUBLE.
*
*
* optional double double_value = 15;
* @return The doubleValue.
*/
@java.lang.Override
public double getDoubleValue() {
return doubleValue_;
}
/**
*
* Double value. Should be set if feed_attribute_id refers to a feed attribute
* of type DOUBLE.
*
*
* optional double double_value = 15;
* @param value The doubleValue to set.
* @return This builder for chaining.
*/
public Builder setDoubleValue(double value) {
doubleValue_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Double value. Should be set if feed_attribute_id refers to a feed attribute
* of type DOUBLE.
*
*
* optional double double_value = 15;
* @return This builder for chaining.
*/
public Builder clearDoubleValue() {
bitField0_ = (bitField0_ & ~0x00000010);
doubleValue_ = 0D;
onChanged();
return this;
}
private com.google.ads.googleads.v17.common.Money priceValue_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.common.Money, com.google.ads.googleads.v17.common.Money.Builder, com.google.ads.googleads.v17.common.MoneyOrBuilder> priceValueBuilder_;
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
* @return Whether the priceValue field is set.
*/
public boolean hasPriceValue() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
* @return The priceValue.
*/
public com.google.ads.googleads.v17.common.Money getPriceValue() {
if (priceValueBuilder_ == null) {
return priceValue_ == null ? com.google.ads.googleads.v17.common.Money.getDefaultInstance() : priceValue_;
} else {
return priceValueBuilder_.getMessage();
}
}
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
*/
public Builder setPriceValue(com.google.ads.googleads.v17.common.Money value) {
if (priceValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
priceValue_ = value;
} else {
priceValueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
*/
public Builder setPriceValue(
com.google.ads.googleads.v17.common.Money.Builder builderForValue) {
if (priceValueBuilder_ == null) {
priceValue_ = builderForValue.build();
} else {
priceValueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
*/
public Builder mergePriceValue(com.google.ads.googleads.v17.common.Money value) {
if (priceValueBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
priceValue_ != null &&
priceValue_ != com.google.ads.googleads.v17.common.Money.getDefaultInstance()) {
getPriceValueBuilder().mergeFrom(value);
} else {
priceValue_ = value;
}
} else {
priceValueBuilder_.mergeFrom(value);
}
if (priceValue_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
*/
public Builder clearPriceValue() {
bitField0_ = (bitField0_ & ~0x00000020);
priceValue_ = null;
if (priceValueBuilder_ != null) {
priceValueBuilder_.dispose();
priceValueBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
*/
public com.google.ads.googleads.v17.common.Money.Builder getPriceValueBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getPriceValueFieldBuilder().getBuilder();
}
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
*/
public com.google.ads.googleads.v17.common.MoneyOrBuilder getPriceValueOrBuilder() {
if (priceValueBuilder_ != null) {
return priceValueBuilder_.getMessageOrBuilder();
} else {
return priceValue_ == null ?
com.google.ads.googleads.v17.common.Money.getDefaultInstance() : priceValue_;
}
}
/**
*
* Price value. Should be set if feed_attribute_id refers to a feed attribute
* of type PRICE.
*
*
* .google.ads.googleads.v17.common.Money price_value = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.common.Money, com.google.ads.googleads.v17.common.Money.Builder, com.google.ads.googleads.v17.common.MoneyOrBuilder>
getPriceValueFieldBuilder() {
if (priceValueBuilder_ == null) {
priceValueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.common.Money, com.google.ads.googleads.v17.common.Money.Builder, com.google.ads.googleads.v17.common.MoneyOrBuilder>(
getPriceValue(),
getParentForChildren(),
isClean());
priceValue_ = null;
}
return priceValueBuilder_;
}
private com.google.protobuf.Internal.LongList integerValues_ = emptyLongList();
private void ensureIntegerValuesIsMutable() {
if (!integerValues_.isModifiable()) {
integerValues_ = makeMutableCopy(integerValues_);
}
bitField0_ |= 0x00000040;
}
/**
*
* Repeated int64 value. Should be set if feed_attribute_id refers to a feed
* attribute of type INT64_LIST.
*
*
* repeated int64 integer_values = 16;
* @return A list containing the integerValues.
*/
public java.util.List
getIntegerValuesList() {
integerValues_.makeImmutable();
return integerValues_;
}
/**
*
* Repeated int64 value. Should be set if feed_attribute_id refers to a feed
* attribute of type INT64_LIST.
*
*
* repeated int64 integer_values = 16;
* @return The count of integerValues.
*/
public int getIntegerValuesCount() {
return integerValues_.size();
}
/**
*
* Repeated int64 value. Should be set if feed_attribute_id refers to a feed
* attribute of type INT64_LIST.
*
*
* repeated int64 integer_values = 16;
* @param index The index of the element to return.
* @return The integerValues at the given index.
*/
public long getIntegerValues(int index) {
return integerValues_.getLong(index);
}
/**
*
* Repeated int64 value. Should be set if feed_attribute_id refers to a feed
* attribute of type INT64_LIST.
*
*
* repeated int64 integer_values = 16;
* @param index The index to set the value at.
* @param value The integerValues to set.
* @return This builder for chaining.
*/
public Builder setIntegerValues(
int index, long value) {
ensureIntegerValuesIsMutable();
integerValues_.setLong(index, value);
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Repeated int64 value. Should be set if feed_attribute_id refers to a feed
* attribute of type INT64_LIST.
*
*
* repeated int64 integer_values = 16;
* @param value The integerValues to add.
* @return This builder for chaining.
*/
public Builder addIntegerValues(long value) {
ensureIntegerValuesIsMutable();
integerValues_.addLong(value);
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Repeated int64 value. Should be set if feed_attribute_id refers to a feed
* attribute of type INT64_LIST.
*
*
* repeated int64 integer_values = 16;
* @param values The integerValues to add.
* @return This builder for chaining.
*/
public Builder addAllIntegerValues(
java.lang.Iterable extends java.lang.Long> values) {
ensureIntegerValuesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, integerValues_);
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Repeated int64 value. Should be set if feed_attribute_id refers to a feed
* attribute of type INT64_LIST.
*
*
* repeated int64 integer_values = 16;
* @return This builder for chaining.
*/
public Builder clearIntegerValues() {
integerValues_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
private com.google.protobuf.Internal.BooleanList booleanValues_ = emptyBooleanList();
private void ensureBooleanValuesIsMutable() {
if (!booleanValues_.isModifiable()) {
booleanValues_ = makeMutableCopy(booleanValues_);
}
bitField0_ |= 0x00000080;
}
private void ensureBooleanValuesIsMutable(int capacity) {
if (!booleanValues_.isModifiable()) {
booleanValues_ = makeMutableCopy(booleanValues_, capacity);
}
bitField0_ |= 0x00000080;
}
/**
*
* Repeated bool value. Should be set if feed_attribute_id refers to a feed
* attribute of type BOOLEAN_LIST.
*
*
* repeated bool boolean_values = 17;
* @return A list containing the booleanValues.
*/
public java.util.List
getBooleanValuesList() {
booleanValues_.makeImmutable();
return booleanValues_;
}
/**
*
* Repeated bool value. Should be set if feed_attribute_id refers to a feed
* attribute of type BOOLEAN_LIST.
*
*
* repeated bool boolean_values = 17;
* @return The count of booleanValues.
*/
public int getBooleanValuesCount() {
return booleanValues_.size();
}
/**
*
* Repeated bool value. Should be set if feed_attribute_id refers to a feed
* attribute of type BOOLEAN_LIST.
*
*
* repeated bool boolean_values = 17;
* @param index The index of the element to return.
* @return The booleanValues at the given index.
*/
public boolean getBooleanValues(int index) {
return booleanValues_.getBoolean(index);
}
/**
*
* Repeated bool value. Should be set if feed_attribute_id refers to a feed
* attribute of type BOOLEAN_LIST.
*
*
* repeated bool boolean_values = 17;
* @param index The index to set the value at.
* @param value The booleanValues to set.
* @return This builder for chaining.
*/
public Builder setBooleanValues(
int index, boolean value) {
ensureBooleanValuesIsMutable();
booleanValues_.setBoolean(index, value);
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Repeated bool value. Should be set if feed_attribute_id refers to a feed
* attribute of type BOOLEAN_LIST.
*
*
* repeated bool boolean_values = 17;
* @param value The booleanValues to add.
* @return This builder for chaining.
*/
public Builder addBooleanValues(boolean value) {
ensureBooleanValuesIsMutable();
booleanValues_.addBoolean(value);
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Repeated bool value. Should be set if feed_attribute_id refers to a feed
* attribute of type BOOLEAN_LIST.
*
*
* repeated bool boolean_values = 17;
* @param values The booleanValues to add.
* @return This builder for chaining.
*/
public Builder addAllBooleanValues(
java.lang.Iterable extends java.lang.Boolean> values) {
ensureBooleanValuesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, booleanValues_);
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Repeated bool value. Should be set if feed_attribute_id refers to a feed
* attribute of type BOOLEAN_LIST.
*
*
* repeated bool boolean_values = 17;
* @return This builder for chaining.
*/
public Builder clearBooleanValues() {
booleanValues_ = emptyBooleanList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList stringValues_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureStringValuesIsMutable() {
if (!stringValues_.isModifiable()) {
stringValues_ = new com.google.protobuf.LazyStringArrayList(stringValues_);
}
bitField0_ |= 0x00000100;
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @return A list containing the stringValues.
*/
public com.google.protobuf.ProtocolStringList
getStringValuesList() {
stringValues_.makeImmutable();
return stringValues_;
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @return The count of stringValues.
*/
public int getStringValuesCount() {
return stringValues_.size();
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @param index The index of the element to return.
* @return The stringValues at the given index.
*/
public java.lang.String getStringValues(int index) {
return stringValues_.get(index);
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @param index The index of the value to return.
* @return The bytes of the stringValues at the given index.
*/
public com.google.protobuf.ByteString
getStringValuesBytes(int index) {
return stringValues_.getByteString(index);
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @param index The index to set the value at.
* @param value The stringValues to set.
* @return This builder for chaining.
*/
public Builder setStringValues(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureStringValuesIsMutable();
stringValues_.set(index, value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @param value The stringValues to add.
* @return This builder for chaining.
*/
public Builder addStringValues(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureStringValuesIsMutable();
stringValues_.add(value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @param values The stringValues to add.
* @return This builder for chaining.
*/
public Builder addAllStringValues(
java.lang.Iterable values) {
ensureStringValuesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, stringValues_);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @return This builder for chaining.
*/
public Builder clearStringValues() {
stringValues_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);;
onChanged();
return this;
}
/**
*
* Repeated string value. Should be set if feed_attribute_id refers to a feed
* attribute of type STRING_LIST, URL_LIST or DATE_TIME_LIST.
* For STRING_LIST and URL_LIST the total size of the list in bytes may not
* exceed 3000. For DATE_TIME_LIST the number of elements may not exceed 200.
*
* For STRING_LIST the maximum length of each string element is 1500
* characters. For URL_LIST the maximum length is 2076 characters. For
* DATE_TIME the format of the string must be the same as start and end time
* for the feed item.
*
*
* repeated string string_values = 18;
* @param value The bytes of the stringValues to add.
* @return This builder for chaining.
*/
public Builder addStringValuesBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureStringValuesIsMutable();
stringValues_.add(value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private com.google.protobuf.Internal.DoubleList doubleValues_ = emptyDoubleList();
private void ensureDoubleValuesIsMutable() {
if (!doubleValues_.isModifiable()) {
doubleValues_ = makeMutableCopy(doubleValues_);
}
bitField0_ |= 0x00000200;
}
private void ensureDoubleValuesIsMutable(int capacity) {
if (!doubleValues_.isModifiable()) {
doubleValues_ = makeMutableCopy(doubleValues_, capacity);
}
bitField0_ |= 0x00000200;
}
/**
*
* Repeated double value. Should be set if feed_attribute_id refers to a feed
* attribute of type DOUBLE_LIST.
*
*
* repeated double double_values = 19;
* @return A list containing the doubleValues.
*/
public java.util.List
getDoubleValuesList() {
doubleValues_.makeImmutable();
return doubleValues_;
}
/**
*
* Repeated double value. Should be set if feed_attribute_id refers to a feed
* attribute of type DOUBLE_LIST.
*
*
* repeated double double_values = 19;
* @return The count of doubleValues.
*/
public int getDoubleValuesCount() {
return doubleValues_.size();
}
/**
*
* Repeated double value. Should be set if feed_attribute_id refers to a feed
* attribute of type DOUBLE_LIST.
*
*
* repeated double double_values = 19;
* @param index The index of the element to return.
* @return The doubleValues at the given index.
*/
public double getDoubleValues(int index) {
return doubleValues_.getDouble(index);
}
/**
*
* Repeated double value. Should be set if feed_attribute_id refers to a feed
* attribute of type DOUBLE_LIST.
*
*
* repeated double double_values = 19;
* @param index The index to set the value at.
* @param value The doubleValues to set.
* @return This builder for chaining.
*/
public Builder setDoubleValues(
int index, double value) {
ensureDoubleValuesIsMutable();
doubleValues_.setDouble(index, value);
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* Repeated double value. Should be set if feed_attribute_id refers to a feed
* attribute of type DOUBLE_LIST.
*
*
* repeated double double_values = 19;
* @param value The doubleValues to add.
* @return This builder for chaining.
*/
public Builder addDoubleValues(double value) {
ensureDoubleValuesIsMutable();
doubleValues_.addDouble(value);
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* Repeated double value. Should be set if feed_attribute_id refers to a feed
* attribute of type DOUBLE_LIST.
*
*
* repeated double double_values = 19;
* @param values The doubleValues to add.
* @return This builder for chaining.
*/
public Builder addAllDoubleValues(
java.lang.Iterable extends java.lang.Double> values) {
ensureDoubleValuesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, doubleValues_);
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* Repeated double value. Should be set if feed_attribute_id refers to a feed
* attribute of type DOUBLE_LIST.
*
*
* repeated double double_values = 19;
* @return This builder for chaining.
*/
public Builder clearDoubleValues() {
doubleValues_ = emptyDoubleList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v17.resources.FeedItemAttributeValue)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v17.resources.FeedItemAttributeValue)
private static final com.google.ads.googleads.v17.resources.FeedItemAttributeValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v17.resources.FeedItemAttributeValue();
}
public static com.google.ads.googleads.v17.resources.FeedItemAttributeValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FeedItemAttributeValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v17.resources.FeedItemAttributeValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy