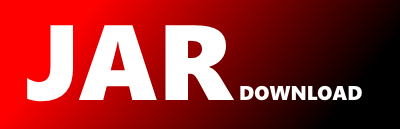
com.google.ads.googleads.v17.services.AudienceInsightsServiceClient Maven / Gradle / Ivy
Show all versions of google-ads-stubs-v17 Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.ads.googleads.v17.services;
import com.google.ads.googleads.v17.common.LocationInfo;
import com.google.ads.googleads.v17.enums.AudienceInsightsDimensionEnum;
import com.google.ads.googleads.v17.services.stub.AudienceInsightsServiceStub;
import com.google.ads.googleads.v17.services.stub.AudienceInsightsServiceStubSettings;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.rpc.UnaryCallable;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Audience Insights Service helps users find information about groups of
* people and how they can be reached with Google Ads. Accessible to allowlisted customers only.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* String customerId = "customerId-1581184615";
* BasicInsightsAudience baselineAudience = BasicInsightsAudience.newBuilder().build();
* BasicInsightsAudience specificAudience = BasicInsightsAudience.newBuilder().build();
* GenerateInsightsFinderReportResponse response =
* audienceInsightsServiceClient.generateInsightsFinderReport(
* customerId, baselineAudience, specificAudience);
* }
* }
*
* Note: close() needs to be called on the AudienceInsightsServiceClient object to clean up
* resources such as threads. In the example above, try-with-resources is used, which automatically
* calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* GenerateInsightsFinderReport
* Creates a saved report that can be viewed in the Insights Finder tool.
*
List of thrown errors: [AuthenticationError]() [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* generateInsightsFinderReport(GenerateInsightsFinderReportRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* generateInsightsFinderReport(String customerId, BasicInsightsAudience baselineAudience, BasicInsightsAudience specificAudience)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* generateInsightsFinderReportCallable()
*
*
*
*
* ListAudienceInsightsAttributes
* Searches for audience attributes that can be used to generate insights.
*
List of thrown errors: [AuthenticationError]() [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listAudienceInsightsAttributes(ListAudienceInsightsAttributesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listAudienceInsightsAttributes(String customerId, List<AudienceInsightsDimensionEnum.AudienceInsightsDimension> dimensions, String queryText)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listAudienceInsightsAttributesCallable()
*
*
*
*
* ListInsightsEligibleDates
* Lists date ranges for which audience insights data can be requested.
*
List of thrown errors: [AuthenticationError]() [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listInsightsEligibleDates(ListInsightsEligibleDatesRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listInsightsEligibleDatesCallable()
*
*
*
*
* GenerateAudienceCompositionInsights
* Returns a collection of attributes that are represented in an audience of interest, with metrics that compare each attribute's share of the audience with its share of a baseline audience.
*
List of thrown errors: [AudienceInsightsError]() [AuthenticationError]() [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* generateAudienceCompositionInsights(GenerateAudienceCompositionInsightsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* generateAudienceCompositionInsights(String customerId, InsightsAudience audience, List<AudienceInsightsDimensionEnum.AudienceInsightsDimension> dimensions)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* generateAudienceCompositionInsightsCallable()
*
*
*
*
* GenerateSuggestedTargetingInsights
* Returns a collection of targeting insights (e.g. targetable audiences) that are relevant to the requested audience.
*
List of thrown errors: [AudienceInsightsError]() [AuthenticationError]() [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* generateSuggestedTargetingInsights(GenerateSuggestedTargetingInsightsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* generateSuggestedTargetingInsights(String customerId, InsightsAudience audience)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* generateSuggestedTargetingInsightsCallable()
*
*
*
*
* GenerateAudienceOverlapInsights
* Returns a collection of audience attributes along with estimates of the overlap between their potential YouTube reach and that of a given input attribute.
*
List of thrown errors: [AudienceInsightsError]() [AuthenticationError]() [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* generateAudienceOverlapInsights(GenerateAudienceOverlapInsightsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* generateAudienceOverlapInsights(String customerId, LocationInfo countryLocation, AudienceInsightsAttribute primaryAttribute, List<AudienceInsightsDimensionEnum.AudienceInsightsDimension> dimensions)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* generateAudienceOverlapInsightsCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of
* AudienceInsightsServiceSettings to create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* AudienceInsightsServiceSettings audienceInsightsServiceSettings =
* AudienceInsightsServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create(audienceInsightsServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* AudienceInsightsServiceSettings audienceInsightsServiceSettings =
* AudienceInsightsServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create(audienceInsightsServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class AudienceInsightsServiceClient implements BackgroundResource {
private final AudienceInsightsServiceSettings settings;
private final AudienceInsightsServiceStub stub;
/** Constructs an instance of AudienceInsightsServiceClient with default settings. */
public static final AudienceInsightsServiceClient create() throws IOException {
return create(AudienceInsightsServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of AudienceInsightsServiceClient, using the given settings. The channels
* are created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final AudienceInsightsServiceClient create(AudienceInsightsServiceSettings settings)
throws IOException {
return new AudienceInsightsServiceClient(settings);
}
/**
* Constructs an instance of AudienceInsightsServiceClient, using the given stub for making calls.
* This is for advanced usage - prefer using create(AudienceInsightsServiceSettings).
*/
public static final AudienceInsightsServiceClient create(AudienceInsightsServiceStub stub) {
return new AudienceInsightsServiceClient(stub);
}
/**
* Constructs an instance of AudienceInsightsServiceClient, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected AudienceInsightsServiceClient(AudienceInsightsServiceSettings settings)
throws IOException {
this.settings = settings;
this.stub = ((AudienceInsightsServiceStubSettings) settings.getStubSettings()).createStub();
}
protected AudienceInsightsServiceClient(AudienceInsightsServiceStub stub) {
this.settings = null;
this.stub = stub;
}
public final AudienceInsightsServiceSettings getSettings() {
return settings;
}
public AudienceInsightsServiceStub getStub() {
return stub;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a saved report that can be viewed in the Insights Finder tool.
*
*
List of thrown errors: [AuthenticationError]() [AuthorizationError]() [FieldError]()
* [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* String customerId = "customerId-1581184615";
* BasicInsightsAudience baselineAudience = BasicInsightsAudience.newBuilder().build();
* BasicInsightsAudience specificAudience = BasicInsightsAudience.newBuilder().build();
* GenerateInsightsFinderReportResponse response =
* audienceInsightsServiceClient.generateInsightsFinderReport(
* customerId, baselineAudience, specificAudience);
* }
* }
*
* @param customerId Required. The ID of the customer.
* @param baselineAudience Required. A baseline audience for this report, typically all people in
* a region.
* @param specificAudience Required. The specific audience of interest for this report. The
* insights in the report will be based on attributes more prevalent in this audience than in
* the report's baseline audience.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateInsightsFinderReportResponse generateInsightsFinderReport(
String customerId,
BasicInsightsAudience baselineAudience,
BasicInsightsAudience specificAudience) {
GenerateInsightsFinderReportRequest request =
GenerateInsightsFinderReportRequest.newBuilder()
.setCustomerId(customerId)
.setBaselineAudience(baselineAudience)
.setSpecificAudience(specificAudience)
.build();
return generateInsightsFinderReport(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a saved report that can be viewed in the Insights Finder tool.
*
* List of thrown errors: [AuthenticationError]() [AuthorizationError]() [FieldError]()
* [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* GenerateInsightsFinderReportRequest request =
* GenerateInsightsFinderReportRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .setBaselineAudience(BasicInsightsAudience.newBuilder().build())
* .setSpecificAudience(BasicInsightsAudience.newBuilder().build())
* .setCustomerInsightsGroup("customerInsightsGroup1092118566")
* .build();
* GenerateInsightsFinderReportResponse response =
* audienceInsightsServiceClient.generateInsightsFinderReport(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateInsightsFinderReportResponse generateInsightsFinderReport(
GenerateInsightsFinderReportRequest request) {
return generateInsightsFinderReportCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a saved report that can be viewed in the Insights Finder tool.
*
* List of thrown errors: [AuthenticationError]() [AuthorizationError]() [FieldError]()
* [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* GenerateInsightsFinderReportRequest request =
* GenerateInsightsFinderReportRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .setBaselineAudience(BasicInsightsAudience.newBuilder().build())
* .setSpecificAudience(BasicInsightsAudience.newBuilder().build())
* .setCustomerInsightsGroup("customerInsightsGroup1092118566")
* .build();
* ApiFuture future =
* audienceInsightsServiceClient.generateInsightsFinderReportCallable().futureCall(request);
* // Do something.
* GenerateInsightsFinderReportResponse response = future.get();
* }
* }
*/
public final UnaryCallable<
GenerateInsightsFinderReportRequest, GenerateInsightsFinderReportResponse>
generateInsightsFinderReportCallable() {
return stub.generateInsightsFinderReportCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches for audience attributes that can be used to generate insights.
*
* List of thrown errors: [AuthenticationError]() [AuthorizationError]() [FieldError]()
* [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List dimensions = new ArrayList<>();
* String queryText = "queryText-1806881259";
* ListAudienceInsightsAttributesResponse response =
* audienceInsightsServiceClient.listAudienceInsightsAttributes(
* customerId, dimensions, queryText);
* }
* }
*
* @param customerId Required. The ID of the customer.
* @param dimensions Required. The types of attributes to be returned.
* @param queryText Required. A free text query. If the requested dimensions include Attributes
* CATEGORY or KNOWLEDGE_GRAPH, then the attributes returned for those dimensions will match
* or be related to this string. For other dimensions, this field is ignored and all available
* attributes are returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAudienceInsightsAttributesResponse listAudienceInsightsAttributes(
String customerId,
List dimensions,
String queryText) {
ListAudienceInsightsAttributesRequest request =
ListAudienceInsightsAttributesRequest.newBuilder()
.setCustomerId(customerId)
.addAllDimensions(dimensions)
.setQueryText(queryText)
.build();
return listAudienceInsightsAttributes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches for audience attributes that can be used to generate insights.
*
* List of thrown errors: [AuthenticationError]() [AuthorizationError]() [FieldError]()
* [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* ListAudienceInsightsAttributesRequest request =
* ListAudienceInsightsAttributesRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .addAllDimensions(
* new ArrayList())
* .setQueryText("queryText-1806881259")
* .setCustomerInsightsGroup("customerInsightsGroup1092118566")
* .addAllLocationCountryFilters(new ArrayList())
* .setYoutubeReachLocation(LocationInfo.newBuilder().build())
* .build();
* ListAudienceInsightsAttributesResponse response =
* audienceInsightsServiceClient.listAudienceInsightsAttributes(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAudienceInsightsAttributesResponse listAudienceInsightsAttributes(
ListAudienceInsightsAttributesRequest request) {
return listAudienceInsightsAttributesCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches for audience attributes that can be used to generate insights.
*
* List of thrown errors: [AuthenticationError]() [AuthorizationError]() [FieldError]()
* [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* ListAudienceInsightsAttributesRequest request =
* ListAudienceInsightsAttributesRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .addAllDimensions(
* new ArrayList())
* .setQueryText("queryText-1806881259")
* .setCustomerInsightsGroup("customerInsightsGroup1092118566")
* .addAllLocationCountryFilters(new ArrayList())
* .setYoutubeReachLocation(LocationInfo.newBuilder().build())
* .build();
* ApiFuture future =
* audienceInsightsServiceClient
* .listAudienceInsightsAttributesCallable()
* .futureCall(request);
* // Do something.
* ListAudienceInsightsAttributesResponse response = future.get();
* }
* }
*/
public final UnaryCallable<
ListAudienceInsightsAttributesRequest, ListAudienceInsightsAttributesResponse>
listAudienceInsightsAttributesCallable() {
return stub.listAudienceInsightsAttributesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists date ranges for which audience insights data can be requested.
*
* List of thrown errors: [AuthenticationError]() [AuthorizationError]() [FieldError]()
* [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* ListInsightsEligibleDatesRequest request =
* ListInsightsEligibleDatesRequest.newBuilder().build();
* ListInsightsEligibleDatesResponse response =
* audienceInsightsServiceClient.listInsightsEligibleDates(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListInsightsEligibleDatesResponse listInsightsEligibleDates(
ListInsightsEligibleDatesRequest request) {
return listInsightsEligibleDatesCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists date ranges for which audience insights data can be requested.
*
* List of thrown errors: [AuthenticationError]() [AuthorizationError]() [FieldError]()
* [HeaderError]() [InternalError]() [QuotaError]() [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* ListInsightsEligibleDatesRequest request =
* ListInsightsEligibleDatesRequest.newBuilder().build();
* ApiFuture future =
* audienceInsightsServiceClient.listInsightsEligibleDatesCallable().futureCall(request);
* // Do something.
* ListInsightsEligibleDatesResponse response = future.get();
* }
* }
*/
public final UnaryCallable
listInsightsEligibleDatesCallable() {
return stub.listInsightsEligibleDatesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a collection of attributes that are represented in an audience of interest, with
* metrics that compare each attribute's share of the audience with its share of a baseline
* audience.
*
* List of thrown errors: [AudienceInsightsError]() [AuthenticationError]()
* [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]()
* [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* String customerId = "customerId-1581184615";
* InsightsAudience audience = InsightsAudience.newBuilder().build();
* List dimensions = new ArrayList<>();
* GenerateAudienceCompositionInsightsResponse response =
* audienceInsightsServiceClient.generateAudienceCompositionInsights(
* customerId, audience, dimensions);
* }
* }
*
* @param customerId Required. The ID of the customer.
* @param audience Required. The audience of interest for which insights are being requested.
* @param dimensions Required. The audience dimensions for which composition insights should be
* returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateAudienceCompositionInsightsResponse generateAudienceCompositionInsights(
String customerId,
InsightsAudience audience,
List dimensions) {
GenerateAudienceCompositionInsightsRequest request =
GenerateAudienceCompositionInsightsRequest.newBuilder()
.setCustomerId(customerId)
.setAudience(audience)
.addAllDimensions(dimensions)
.build();
return generateAudienceCompositionInsights(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a collection of attributes that are represented in an audience of interest, with
* metrics that compare each attribute's share of the audience with its share of a baseline
* audience.
*
* List of thrown errors: [AudienceInsightsError]() [AuthenticationError]()
* [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]()
* [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* GenerateAudienceCompositionInsightsRequest request =
* GenerateAudienceCompositionInsightsRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .setAudience(InsightsAudience.newBuilder().build())
* .setBaselineAudience(InsightsAudience.newBuilder().build())
* .setDataMonth("dataMonth-380142346")
* .addAllDimensions(
* new ArrayList())
* .setCustomerInsightsGroup("customerInsightsGroup1092118566")
* .build();
* GenerateAudienceCompositionInsightsResponse response =
* audienceInsightsServiceClient.generateAudienceCompositionInsights(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateAudienceCompositionInsightsResponse generateAudienceCompositionInsights(
GenerateAudienceCompositionInsightsRequest request) {
return generateAudienceCompositionInsightsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a collection of attributes that are represented in an audience of interest, with
* metrics that compare each attribute's share of the audience with its share of a baseline
* audience.
*
* List of thrown errors: [AudienceInsightsError]() [AuthenticationError]()
* [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]()
* [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* GenerateAudienceCompositionInsightsRequest request =
* GenerateAudienceCompositionInsightsRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .setAudience(InsightsAudience.newBuilder().build())
* .setBaselineAudience(InsightsAudience.newBuilder().build())
* .setDataMonth("dataMonth-380142346")
* .addAllDimensions(
* new ArrayList())
* .setCustomerInsightsGroup("customerInsightsGroup1092118566")
* .build();
* ApiFuture future =
* audienceInsightsServiceClient
* .generateAudienceCompositionInsightsCallable()
* .futureCall(request);
* // Do something.
* GenerateAudienceCompositionInsightsResponse response = future.get();
* }
* }
*/
public final UnaryCallable<
GenerateAudienceCompositionInsightsRequest, GenerateAudienceCompositionInsightsResponse>
generateAudienceCompositionInsightsCallable() {
return stub.generateAudienceCompositionInsightsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a collection of targeting insights (e.g. targetable audiences) that are relevant to the
* requested audience.
*
* List of thrown errors: [AudienceInsightsError]() [AuthenticationError]()
* [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]()
* [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* String customerId = "customerId-1581184615";
* InsightsAudience audience = InsightsAudience.newBuilder().build();
* GenerateSuggestedTargetingInsightsResponse response =
* audienceInsightsServiceClient.generateSuggestedTargetingInsights(customerId, audience);
* }
* }
*
* @param customerId Required. The ID of the customer.
* @param audience Required. The audience of interest for which insights are being requested.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateSuggestedTargetingInsightsResponse generateSuggestedTargetingInsights(
String customerId, InsightsAudience audience) {
GenerateSuggestedTargetingInsightsRequest request =
GenerateSuggestedTargetingInsightsRequest.newBuilder()
.setCustomerId(customerId)
.setAudience(audience)
.build();
return generateSuggestedTargetingInsights(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a collection of targeting insights (e.g. targetable audiences) that are relevant to the
* requested audience.
*
* List of thrown errors: [AudienceInsightsError]() [AuthenticationError]()
* [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]()
* [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* GenerateSuggestedTargetingInsightsRequest request =
* GenerateSuggestedTargetingInsightsRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .setAudience(InsightsAudience.newBuilder().build())
* .setBaselineAudience(InsightsAudience.newBuilder().build())
* .setDataMonth("dataMonth-380142346")
* .setCustomerInsightsGroup("customerInsightsGroup1092118566")
* .build();
* GenerateSuggestedTargetingInsightsResponse response =
* audienceInsightsServiceClient.generateSuggestedTargetingInsights(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateSuggestedTargetingInsightsResponse generateSuggestedTargetingInsights(
GenerateSuggestedTargetingInsightsRequest request) {
return generateSuggestedTargetingInsightsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a collection of targeting insights (e.g. targetable audiences) that are relevant to the
* requested audience.
*
* List of thrown errors: [AudienceInsightsError]() [AuthenticationError]()
* [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]()
* [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* GenerateSuggestedTargetingInsightsRequest request =
* GenerateSuggestedTargetingInsightsRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .setAudience(InsightsAudience.newBuilder().build())
* .setBaselineAudience(InsightsAudience.newBuilder().build())
* .setDataMonth("dataMonth-380142346")
* .setCustomerInsightsGroup("customerInsightsGroup1092118566")
* .build();
* ApiFuture future =
* audienceInsightsServiceClient
* .generateSuggestedTargetingInsightsCallable()
* .futureCall(request);
* // Do something.
* GenerateSuggestedTargetingInsightsResponse response = future.get();
* }
* }
*/
public final UnaryCallable<
GenerateSuggestedTargetingInsightsRequest, GenerateSuggestedTargetingInsightsResponse>
generateSuggestedTargetingInsightsCallable() {
return stub.generateSuggestedTargetingInsightsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a collection of audience attributes along with estimates of the overlap between their
* potential YouTube reach and that of a given input attribute.
*
* List of thrown errors: [AudienceInsightsError]() [AuthenticationError]()
* [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]()
* [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* String customerId = "customerId-1581184615";
* LocationInfo countryLocation = LocationInfo.newBuilder().build();
* AudienceInsightsAttribute primaryAttribute = AudienceInsightsAttribute.newBuilder().build();
* List dimensions = new ArrayList<>();
* GenerateAudienceOverlapInsightsResponse response =
* audienceInsightsServiceClient.generateAudienceOverlapInsights(
* customerId, countryLocation, primaryAttribute, dimensions);
* }
* }
*
* @param customerId Required. The ID of the customer.
* @param countryLocation Required. The country in which to calculate the sizes and overlaps of
* audiences.
* @param primaryAttribute Required. The audience attribute that should be intersected with all
* other eligible audiences. This must be an Affinity or In-Market UserInterest, an AgeRange
* or a Gender.
* @param dimensions Required. The types of attributes of which to calculate the overlap with the
* primary_attribute. The values must be a subset of AFFINITY_USER_INTEREST,
* IN_MARKET_USER_INTEREST, AGE_RANGE and GENDER.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateAudienceOverlapInsightsResponse generateAudienceOverlapInsights(
String customerId,
LocationInfo countryLocation,
AudienceInsightsAttribute primaryAttribute,
List dimensions) {
GenerateAudienceOverlapInsightsRequest request =
GenerateAudienceOverlapInsightsRequest.newBuilder()
.setCustomerId(customerId)
.setCountryLocation(countryLocation)
.setPrimaryAttribute(primaryAttribute)
.addAllDimensions(dimensions)
.build();
return generateAudienceOverlapInsights(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a collection of audience attributes along with estimates of the overlap between their
* potential YouTube reach and that of a given input attribute.
*
* List of thrown errors: [AudienceInsightsError]() [AuthenticationError]()
* [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]()
* [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* GenerateAudienceOverlapInsightsRequest request =
* GenerateAudienceOverlapInsightsRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .setCountryLocation(LocationInfo.newBuilder().build())
* .setPrimaryAttribute(AudienceInsightsAttribute.newBuilder().build())
* .addAllDimensions(
* new ArrayList())
* .setCustomerInsightsGroup("customerInsightsGroup1092118566")
* .build();
* GenerateAudienceOverlapInsightsResponse response =
* audienceInsightsServiceClient.generateAudienceOverlapInsights(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateAudienceOverlapInsightsResponse generateAudienceOverlapInsights(
GenerateAudienceOverlapInsightsRequest request) {
return generateAudienceOverlapInsightsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a collection of audience attributes along with estimates of the overlap between their
* potential YouTube reach and that of a given input attribute.
*
* List of thrown errors: [AudienceInsightsError]() [AuthenticationError]()
* [AuthorizationError]() [FieldError]() [HeaderError]() [InternalError]() [QuotaError]()
* [RangeError]() [RequestError]()
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* GenerateAudienceOverlapInsightsRequest request =
* GenerateAudienceOverlapInsightsRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .setCountryLocation(LocationInfo.newBuilder().build())
* .setPrimaryAttribute(AudienceInsightsAttribute.newBuilder().build())
* .addAllDimensions(
* new ArrayList())
* .setCustomerInsightsGroup("customerInsightsGroup1092118566")
* .build();
* ApiFuture future =
* audienceInsightsServiceClient
* .generateAudienceOverlapInsightsCallable()
* .futureCall(request);
* // Do something.
* GenerateAudienceOverlapInsightsResponse response = future.get();
* }
* }
*/
public final UnaryCallable<
GenerateAudienceOverlapInsightsRequest, GenerateAudienceOverlapInsightsResponse>
generateAudienceOverlapInsightsCallable() {
return stub.generateAudienceOverlapInsightsCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
}