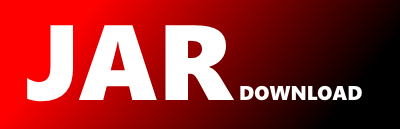
com.google.ads.googleads.v17.services.BatchJobServiceGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v17 Show documentation
Show all versions of google-ads-stubs-v17 Show documentation
Stubs for GAAPI version google-ads-stubs-v17
The newest version!
package com.google.ads.googleads.v17.services;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* Service to manage batch jobs.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler",
comments = "Source: google/ads/googleads/v17/services/batch_job_service.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class BatchJobServiceGrpc {
private BatchJobServiceGrpc() {}
public static final java.lang.String SERVICE_NAME = "google.ads.googleads.v17.services.BatchJobService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getMutateBatchJobMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "MutateBatchJob",
requestType = com.google.ads.googleads.v17.services.MutateBatchJobRequest.class,
responseType = com.google.ads.googleads.v17.services.MutateBatchJobResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getMutateBatchJobMethod() {
io.grpc.MethodDescriptor getMutateBatchJobMethod;
if ((getMutateBatchJobMethod = BatchJobServiceGrpc.getMutateBatchJobMethod) == null) {
synchronized (BatchJobServiceGrpc.class) {
if ((getMutateBatchJobMethod = BatchJobServiceGrpc.getMutateBatchJobMethod) == null) {
BatchJobServiceGrpc.getMutateBatchJobMethod = getMutateBatchJobMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "MutateBatchJob"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.ads.googleads.v17.services.MutateBatchJobRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.ads.googleads.v17.services.MutateBatchJobResponse.getDefaultInstance()))
.setSchemaDescriptor(new BatchJobServiceMethodDescriptorSupplier("MutateBatchJob"))
.build();
}
}
}
return getMutateBatchJobMethod;
}
private static volatile io.grpc.MethodDescriptor getListBatchJobResultsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListBatchJobResults",
requestType = com.google.ads.googleads.v17.services.ListBatchJobResultsRequest.class,
responseType = com.google.ads.googleads.v17.services.ListBatchJobResultsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getListBatchJobResultsMethod() {
io.grpc.MethodDescriptor getListBatchJobResultsMethod;
if ((getListBatchJobResultsMethod = BatchJobServiceGrpc.getListBatchJobResultsMethod) == null) {
synchronized (BatchJobServiceGrpc.class) {
if ((getListBatchJobResultsMethod = BatchJobServiceGrpc.getListBatchJobResultsMethod) == null) {
BatchJobServiceGrpc.getListBatchJobResultsMethod = getListBatchJobResultsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListBatchJobResults"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.ads.googleads.v17.services.ListBatchJobResultsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.ads.googleads.v17.services.ListBatchJobResultsResponse.getDefaultInstance()))
.setSchemaDescriptor(new BatchJobServiceMethodDescriptorSupplier("ListBatchJobResults"))
.build();
}
}
}
return getListBatchJobResultsMethod;
}
private static volatile io.grpc.MethodDescriptor getRunBatchJobMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RunBatchJob",
requestType = com.google.ads.googleads.v17.services.RunBatchJobRequest.class,
responseType = com.google.longrunning.Operation.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRunBatchJobMethod() {
io.grpc.MethodDescriptor getRunBatchJobMethod;
if ((getRunBatchJobMethod = BatchJobServiceGrpc.getRunBatchJobMethod) == null) {
synchronized (BatchJobServiceGrpc.class) {
if ((getRunBatchJobMethod = BatchJobServiceGrpc.getRunBatchJobMethod) == null) {
BatchJobServiceGrpc.getRunBatchJobMethod = getRunBatchJobMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RunBatchJob"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.ads.googleads.v17.services.RunBatchJobRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.longrunning.Operation.getDefaultInstance()))
.setSchemaDescriptor(new BatchJobServiceMethodDescriptorSupplier("RunBatchJob"))
.build();
}
}
}
return getRunBatchJobMethod;
}
private static volatile io.grpc.MethodDescriptor getAddBatchJobOperationsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "AddBatchJobOperations",
requestType = com.google.ads.googleads.v17.services.AddBatchJobOperationsRequest.class,
responseType = com.google.ads.googleads.v17.services.AddBatchJobOperationsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAddBatchJobOperationsMethod() {
io.grpc.MethodDescriptor getAddBatchJobOperationsMethod;
if ((getAddBatchJobOperationsMethod = BatchJobServiceGrpc.getAddBatchJobOperationsMethod) == null) {
synchronized (BatchJobServiceGrpc.class) {
if ((getAddBatchJobOperationsMethod = BatchJobServiceGrpc.getAddBatchJobOperationsMethod) == null) {
BatchJobServiceGrpc.getAddBatchJobOperationsMethod = getAddBatchJobOperationsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "AddBatchJobOperations"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.ads.googleads.v17.services.AddBatchJobOperationsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.ads.googleads.v17.services.AddBatchJobOperationsResponse.getDefaultInstance()))
.setSchemaDescriptor(new BatchJobServiceMethodDescriptorSupplier("AddBatchJobOperations"))
.build();
}
}
}
return getAddBatchJobOperationsMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static BatchJobServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public BatchJobServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BatchJobServiceStub(channel, callOptions);
}
};
return BatchJobServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static BatchJobServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public BatchJobServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BatchJobServiceBlockingStub(channel, callOptions);
}
};
return BatchJobServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static BatchJobServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public BatchJobServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BatchJobServiceFutureStub(channel, callOptions);
}
};
return BatchJobServiceFutureStub.newStub(factory, channel);
}
/**
*
* Service to manage batch jobs.
*
*/
public interface AsyncService {
/**
*
* Mutates a batch job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
* [ResourceCountLimitExceededError]()
*
*/
default void mutateBatchJob(com.google.ads.googleads.v17.services.MutateBatchJobRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getMutateBatchJobMethod(), responseObserver);
}
/**
*
* Returns the results of the batch job. The job must be done.
* Supports standard list paging.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
*
*/
default void listBatchJobResults(com.google.ads.googleads.v17.services.ListBatchJobResultsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListBatchJobResultsMethod(), responseObserver);
}
/**
*
* Runs the batch job.
* The Operation.metadata field type is BatchJobMetadata. When finished, the
* long running operation will not contain errors or a response. Instead, use
* ListBatchJobResults to get the results of the job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
*
*/
default void runBatchJob(com.google.ads.googleads.v17.services.RunBatchJobRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getRunBatchJobMethod(), responseObserver);
}
/**
*
* Add operations to the batch job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
* [ResourceCountLimitExceededError]()
*
*/
default void addBatchJobOperations(com.google.ads.googleads.v17.services.AddBatchJobOperationsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAddBatchJobOperationsMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service BatchJobService.
*
* Service to manage batch jobs.
*
*/
public static abstract class BatchJobServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return BatchJobServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service BatchJobService.
*
* Service to manage batch jobs.
*
*/
public static final class BatchJobServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private BatchJobServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected BatchJobServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BatchJobServiceStub(channel, callOptions);
}
/**
*
* Mutates a batch job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
* [ResourceCountLimitExceededError]()
*
*/
public void mutateBatchJob(com.google.ads.googleads.v17.services.MutateBatchJobRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getMutateBatchJobMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Returns the results of the batch job. The job must be done.
* Supports standard list paging.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
*
*/
public void listBatchJobResults(com.google.ads.googleads.v17.services.ListBatchJobResultsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListBatchJobResultsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Runs the batch job.
* The Operation.metadata field type is BatchJobMetadata. When finished, the
* long running operation will not contain errors or a response. Instead, use
* ListBatchJobResults to get the results of the job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
*
*/
public void runBatchJob(com.google.ads.googleads.v17.services.RunBatchJobRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getRunBatchJobMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Add operations to the batch job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
* [ResourceCountLimitExceededError]()
*
*/
public void addBatchJobOperations(com.google.ads.googleads.v17.services.AddBatchJobOperationsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getAddBatchJobOperationsMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service BatchJobService.
*
* Service to manage batch jobs.
*
*/
public static final class BatchJobServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private BatchJobServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected BatchJobServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BatchJobServiceBlockingStub(channel, callOptions);
}
/**
*
* Mutates a batch job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
* [ResourceCountLimitExceededError]()
*
*/
public com.google.ads.googleads.v17.services.MutateBatchJobResponse mutateBatchJob(com.google.ads.googleads.v17.services.MutateBatchJobRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getMutateBatchJobMethod(), getCallOptions(), request);
}
/**
*
* Returns the results of the batch job. The job must be done.
* Supports standard list paging.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
*
*/
public com.google.ads.googleads.v17.services.ListBatchJobResultsResponse listBatchJobResults(com.google.ads.googleads.v17.services.ListBatchJobResultsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListBatchJobResultsMethod(), getCallOptions(), request);
}
/**
*
* Runs the batch job.
* The Operation.metadata field type is BatchJobMetadata. When finished, the
* long running operation will not contain errors or a response. Instead, use
* ListBatchJobResults to get the results of the job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
*
*/
public com.google.longrunning.Operation runBatchJob(com.google.ads.googleads.v17.services.RunBatchJobRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getRunBatchJobMethod(), getCallOptions(), request);
}
/**
*
* Add operations to the batch job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
* [ResourceCountLimitExceededError]()
*
*/
public com.google.ads.googleads.v17.services.AddBatchJobOperationsResponse addBatchJobOperations(com.google.ads.googleads.v17.services.AddBatchJobOperationsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getAddBatchJobOperationsMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service BatchJobService.
*
* Service to manage batch jobs.
*
*/
public static final class BatchJobServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private BatchJobServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected BatchJobServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BatchJobServiceFutureStub(channel, callOptions);
}
/**
*
* Mutates a batch job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
* [ResourceCountLimitExceededError]()
*
*/
public com.google.common.util.concurrent.ListenableFuture mutateBatchJob(
com.google.ads.googleads.v17.services.MutateBatchJobRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getMutateBatchJobMethod(), getCallOptions()), request);
}
/**
*
* Returns the results of the batch job. The job must be done.
* Supports standard list paging.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
*
*/
public com.google.common.util.concurrent.ListenableFuture listBatchJobResults(
com.google.ads.googleads.v17.services.ListBatchJobResultsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getListBatchJobResultsMethod(), getCallOptions()), request);
}
/**
*
* Runs the batch job.
* The Operation.metadata field type is BatchJobMetadata. When finished, the
* long running operation will not contain errors or a response. Instead, use
* ListBatchJobResults to get the results of the job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
*
*/
public com.google.common.util.concurrent.ListenableFuture runBatchJob(
com.google.ads.googleads.v17.services.RunBatchJobRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getRunBatchJobMethod(), getCallOptions()), request);
}
/**
*
* Add operations to the batch job.
* List of thrown errors:
* [AuthenticationError]()
* [AuthorizationError]()
* [BatchJobError]()
* [HeaderError]()
* [InternalError]()
* [QuotaError]()
* [RequestError]()
* [ResourceCountLimitExceededError]()
*
*/
public com.google.common.util.concurrent.ListenableFuture addBatchJobOperations(
com.google.ads.googleads.v17.services.AddBatchJobOperationsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getAddBatchJobOperationsMethod(), getCallOptions()), request);
}
}
private static final int METHODID_MUTATE_BATCH_JOB = 0;
private static final int METHODID_LIST_BATCH_JOB_RESULTS = 1;
private static final int METHODID_RUN_BATCH_JOB = 2;
private static final int METHODID_ADD_BATCH_JOB_OPERATIONS = 3;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_MUTATE_BATCH_JOB:
serviceImpl.mutateBatchJob((com.google.ads.googleads.v17.services.MutateBatchJobRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_BATCH_JOB_RESULTS:
serviceImpl.listBatchJobResults((com.google.ads.googleads.v17.services.ListBatchJobResultsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_RUN_BATCH_JOB:
serviceImpl.runBatchJob((com.google.ads.googleads.v17.services.RunBatchJobRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_ADD_BATCH_JOB_OPERATIONS:
serviceImpl.addBatchJobOperations((com.google.ads.googleads.v17.services.AddBatchJobOperationsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getMutateBatchJobMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.ads.googleads.v17.services.MutateBatchJobRequest,
com.google.ads.googleads.v17.services.MutateBatchJobResponse>(
service, METHODID_MUTATE_BATCH_JOB)))
.addMethod(
getListBatchJobResultsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.ads.googleads.v17.services.ListBatchJobResultsRequest,
com.google.ads.googleads.v17.services.ListBatchJobResultsResponse>(
service, METHODID_LIST_BATCH_JOB_RESULTS)))
.addMethod(
getRunBatchJobMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.ads.googleads.v17.services.RunBatchJobRequest,
com.google.longrunning.Operation>(
service, METHODID_RUN_BATCH_JOB)))
.addMethod(
getAddBatchJobOperationsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.ads.googleads.v17.services.AddBatchJobOperationsRequest,
com.google.ads.googleads.v17.services.AddBatchJobOperationsResponse>(
service, METHODID_ADD_BATCH_JOB_OPERATIONS)))
.build();
}
private static abstract class BatchJobServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
BatchJobServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.google.ads.googleads.v17.services.BatchJobServiceProto.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("BatchJobService");
}
}
private static final class BatchJobServiceFileDescriptorSupplier
extends BatchJobServiceBaseDescriptorSupplier {
BatchJobServiceFileDescriptorSupplier() {}
}
private static final class BatchJobServiceMethodDescriptorSupplier
extends BatchJobServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
BatchJobServiceMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (BatchJobServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new BatchJobServiceFileDescriptorSupplier())
.addMethod(getMutateBatchJobMethod())
.addMethod(getListBatchJobResultsMethod())
.addMethod(getRunBatchJobMethod())
.addMethod(getAddBatchJobOperationsMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy