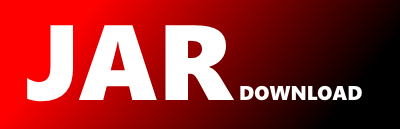
com.google.ads.googleads.v17.services.ConversionAdjustment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v17 Show documentation
Show all versions of google-ads-stubs-v17 Show documentation
Stubs for GAAPI version google-ads-stubs-v17
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v17/services/conversion_adjustment_upload_service.proto
// Protobuf Java Version: 3.25.3
package com.google.ads.googleads.v17.services;
/**
*
* A conversion adjustment.
*
*
* Protobuf type {@code google.ads.googleads.v17.services.ConversionAdjustment}
*/
public final class ConversionAdjustment extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v17.services.ConversionAdjustment)
ConversionAdjustmentOrBuilder {
private static final long serialVersionUID = 0L;
// Use ConversionAdjustment.newBuilder() to construct.
private ConversionAdjustment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConversionAdjustment() {
orderId_ = "";
conversionAction_ = "";
adjustmentDateTime_ = "";
adjustmentType_ = 0;
userIdentifiers_ = java.util.Collections.emptyList();
userAgent_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ConversionAdjustment();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.services.ConversionAdjustmentUploadServiceProto.internal_static_google_ads_googleads_v17_services_ConversionAdjustment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.services.ConversionAdjustmentUploadServiceProto.internal_static_google_ads_googleads_v17_services_ConversionAdjustment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.services.ConversionAdjustment.class, com.google.ads.googleads.v17.services.ConversionAdjustment.Builder.class);
}
private int bitField0_;
public static final int GCLID_DATE_TIME_PAIR_FIELD_NUMBER = 12;
private com.google.ads.googleads.v17.services.GclidDateTimePair gclidDateTimePair_;
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
* @return Whether the gclidDateTimePair field is set.
*/
@java.lang.Override
public boolean hasGclidDateTimePair() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
* @return The gclidDateTimePair.
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.GclidDateTimePair getGclidDateTimePair() {
return gclidDateTimePair_ == null ? com.google.ads.googleads.v17.services.GclidDateTimePair.getDefaultInstance() : gclidDateTimePair_;
}
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.GclidDateTimePairOrBuilder getGclidDateTimePairOrBuilder() {
return gclidDateTimePair_ == null ? com.google.ads.googleads.v17.services.GclidDateTimePair.getDefaultInstance() : gclidDateTimePair_;
}
public static final int ORDER_ID_FIELD_NUMBER = 13;
@SuppressWarnings("serial")
private volatile java.lang.Object orderId_ = "";
/**
*
* The order ID of the conversion to be adjusted. If the conversion was
* reported with an order ID specified, that order ID must be used as the
* identifier here. The order ID is required for enhancements.
*
*
* optional string order_id = 13;
* @return Whether the orderId field is set.
*/
@java.lang.Override
public boolean hasOrderId() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The order ID of the conversion to be adjusted. If the conversion was
* reported with an order ID specified, that order ID must be used as the
* identifier here. The order ID is required for enhancements.
*
*
* optional string order_id = 13;
* @return The orderId.
*/
@java.lang.Override
public java.lang.String getOrderId() {
java.lang.Object ref = orderId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
orderId_ = s;
return s;
}
}
/**
*
* The order ID of the conversion to be adjusted. If the conversion was
* reported with an order ID specified, that order ID must be used as the
* identifier here. The order ID is required for enhancements.
*
*
* optional string order_id = 13;
* @return The bytes for orderId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOrderIdBytes() {
java.lang.Object ref = orderId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
orderId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONVERSION_ACTION_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private volatile java.lang.Object conversionAction_ = "";
/**
*
* Resource name of the conversion action associated with this conversion
* adjustment. Note: Although this resource name consists of a customer id and
* a conversion action id, validation will ignore the customer id and use the
* conversion action id as the sole identifier of the conversion action.
*
*
* optional string conversion_action = 8;
* @return Whether the conversionAction field is set.
*/
@java.lang.Override
public boolean hasConversionAction() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Resource name of the conversion action associated with this conversion
* adjustment. Note: Although this resource name consists of a customer id and
* a conversion action id, validation will ignore the customer id and use the
* conversion action id as the sole identifier of the conversion action.
*
*
* optional string conversion_action = 8;
* @return The conversionAction.
*/
@java.lang.Override
public java.lang.String getConversionAction() {
java.lang.Object ref = conversionAction_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
conversionAction_ = s;
return s;
}
}
/**
*
* Resource name of the conversion action associated with this conversion
* adjustment. Note: Although this resource name consists of a customer id and
* a conversion action id, validation will ignore the customer id and use the
* conversion action id as the sole identifier of the conversion action.
*
*
* optional string conversion_action = 8;
* @return The bytes for conversionAction.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getConversionActionBytes() {
java.lang.Object ref = conversionAction_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
conversionAction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ADJUSTMENT_DATE_TIME_FIELD_NUMBER = 9;
@SuppressWarnings("serial")
private volatile java.lang.Object adjustmentDateTime_ = "";
/**
*
* The date time at which the adjustment occurred. Must be after the
* conversion_date_time. The timezone must be specified. The format is
* "yyyy-mm-dd hh:mm:ss+|-hh:mm", for example, "2019-01-01 12:32:45-08:00".
*
*
* optional string adjustment_date_time = 9;
* @return Whether the adjustmentDateTime field is set.
*/
@java.lang.Override
public boolean hasAdjustmentDateTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The date time at which the adjustment occurred. Must be after the
* conversion_date_time. The timezone must be specified. The format is
* "yyyy-mm-dd hh:mm:ss+|-hh:mm", for example, "2019-01-01 12:32:45-08:00".
*
*
* optional string adjustment_date_time = 9;
* @return The adjustmentDateTime.
*/
@java.lang.Override
public java.lang.String getAdjustmentDateTime() {
java.lang.Object ref = adjustmentDateTime_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adjustmentDateTime_ = s;
return s;
}
}
/**
*
* The date time at which the adjustment occurred. Must be after the
* conversion_date_time. The timezone must be specified. The format is
* "yyyy-mm-dd hh:mm:ss+|-hh:mm", for example, "2019-01-01 12:32:45-08:00".
*
*
* optional string adjustment_date_time = 9;
* @return The bytes for adjustmentDateTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAdjustmentDateTimeBytes() {
java.lang.Object ref = adjustmentDateTime_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adjustmentDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ADJUSTMENT_TYPE_FIELD_NUMBER = 5;
private int adjustmentType_ = 0;
/**
*
* The adjustment type.
*
*
* .google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType adjustment_type = 5;
* @return The enum numeric value on the wire for adjustmentType.
*/
@java.lang.Override public int getAdjustmentTypeValue() {
return adjustmentType_;
}
/**
*
* The adjustment type.
*
*
* .google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType adjustment_type = 5;
* @return The adjustmentType.
*/
@java.lang.Override public com.google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType getAdjustmentType() {
com.google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType result = com.google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType.forNumber(adjustmentType_);
return result == null ? com.google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType.UNRECOGNIZED : result;
}
public static final int RESTATEMENT_VALUE_FIELD_NUMBER = 6;
private com.google.ads.googleads.v17.services.RestatementValue restatementValue_;
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
* @return Whether the restatementValue field is set.
*/
@java.lang.Override
public boolean hasRestatementValue() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
* @return The restatementValue.
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.RestatementValue getRestatementValue() {
return restatementValue_ == null ? com.google.ads.googleads.v17.services.RestatementValue.getDefaultInstance() : restatementValue_;
}
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.RestatementValueOrBuilder getRestatementValueOrBuilder() {
return restatementValue_ == null ? com.google.ads.googleads.v17.services.RestatementValue.getDefaultInstance() : restatementValue_;
}
public static final int USER_IDENTIFIERS_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private java.util.List userIdentifiers_;
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
@java.lang.Override
public java.util.List getUserIdentifiersList() {
return userIdentifiers_;
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
@java.lang.Override
public java.util.List extends com.google.ads.googleads.v17.common.UserIdentifierOrBuilder>
getUserIdentifiersOrBuilderList() {
return userIdentifiers_;
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
@java.lang.Override
public int getUserIdentifiersCount() {
return userIdentifiers_.size();
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.UserIdentifier getUserIdentifiers(int index) {
return userIdentifiers_.get(index);
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
@java.lang.Override
public com.google.ads.googleads.v17.common.UserIdentifierOrBuilder getUserIdentifiersOrBuilder(
int index) {
return userIdentifiers_.get(index);
}
public static final int USER_AGENT_FIELD_NUMBER = 11;
@SuppressWarnings("serial")
private volatile java.lang.Object userAgent_ = "";
/**
*
* The user agent to enhance the original conversion. This can be found in
* your user's HTTP request header when they convert on your web page.
* Example, "Mozilla/5.0 (iPhone; CPU iPhone OS 12_2 like Mac OS X)". User
* agent can only be specified in enhancements with user identifiers. This
* should match the user agent of the request that sent the original
* conversion so the conversion and its enhancement are either both attributed
* as same-device or both attributed as cross-device.
*
*
* optional string user_agent = 11;
* @return Whether the userAgent field is set.
*/
@java.lang.Override
public boolean hasUserAgent() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* The user agent to enhance the original conversion. This can be found in
* your user's HTTP request header when they convert on your web page.
* Example, "Mozilla/5.0 (iPhone; CPU iPhone OS 12_2 like Mac OS X)". User
* agent can only be specified in enhancements with user identifiers. This
* should match the user agent of the request that sent the original
* conversion so the conversion and its enhancement are either both attributed
* as same-device or both attributed as cross-device.
*
*
* optional string user_agent = 11;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
*
* The user agent to enhance the original conversion. This can be found in
* your user's HTTP request header when they convert on your web page.
* Example, "Mozilla/5.0 (iPhone; CPU iPhone OS 12_2 like Mac OS X)". User
* agent can only be specified in enhancements with user identifiers. This
* should match the user agent of the request that sent the original
* conversion so the conversion and its enhancement are either both attributed
* as same-device or both attributed as cross-device.
*
*
* optional string user_agent = 11;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (adjustmentType_ != com.google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType.UNSPECIFIED.getNumber()) {
output.writeEnum(5, adjustmentType_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(6, getRestatementValue());
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, conversionAction_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, adjustmentDateTime_);
}
for (int i = 0; i < userIdentifiers_.size(); i++) {
output.writeMessage(10, userIdentifiers_.get(i));
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, userAgent_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(12, getGclidDateTimePair());
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, orderId_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (adjustmentType_ != com.google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType.UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, adjustmentType_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getRestatementValue());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, conversionAction_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, adjustmentDateTime_);
}
for (int i = 0; i < userIdentifiers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, userIdentifiers_.get(i));
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, userAgent_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getGclidDateTimePair());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, orderId_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v17.services.ConversionAdjustment)) {
return super.equals(obj);
}
com.google.ads.googleads.v17.services.ConversionAdjustment other = (com.google.ads.googleads.v17.services.ConversionAdjustment) obj;
if (hasGclidDateTimePair() != other.hasGclidDateTimePair()) return false;
if (hasGclidDateTimePair()) {
if (!getGclidDateTimePair()
.equals(other.getGclidDateTimePair())) return false;
}
if (hasOrderId() != other.hasOrderId()) return false;
if (hasOrderId()) {
if (!getOrderId()
.equals(other.getOrderId())) return false;
}
if (hasConversionAction() != other.hasConversionAction()) return false;
if (hasConversionAction()) {
if (!getConversionAction()
.equals(other.getConversionAction())) return false;
}
if (hasAdjustmentDateTime() != other.hasAdjustmentDateTime()) return false;
if (hasAdjustmentDateTime()) {
if (!getAdjustmentDateTime()
.equals(other.getAdjustmentDateTime())) return false;
}
if (adjustmentType_ != other.adjustmentType_) return false;
if (hasRestatementValue() != other.hasRestatementValue()) return false;
if (hasRestatementValue()) {
if (!getRestatementValue()
.equals(other.getRestatementValue())) return false;
}
if (!getUserIdentifiersList()
.equals(other.getUserIdentifiersList())) return false;
if (hasUserAgent() != other.hasUserAgent()) return false;
if (hasUserAgent()) {
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasGclidDateTimePair()) {
hash = (37 * hash) + GCLID_DATE_TIME_PAIR_FIELD_NUMBER;
hash = (53 * hash) + getGclidDateTimePair().hashCode();
}
if (hasOrderId()) {
hash = (37 * hash) + ORDER_ID_FIELD_NUMBER;
hash = (53 * hash) + getOrderId().hashCode();
}
if (hasConversionAction()) {
hash = (37 * hash) + CONVERSION_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getConversionAction().hashCode();
}
if (hasAdjustmentDateTime()) {
hash = (37 * hash) + ADJUSTMENT_DATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getAdjustmentDateTime().hashCode();
}
hash = (37 * hash) + ADJUSTMENT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + adjustmentType_;
if (hasRestatementValue()) {
hash = (37 * hash) + RESTATEMENT_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getRestatementValue().hashCode();
}
if (getUserIdentifiersCount() > 0) {
hash = (37 * hash) + USER_IDENTIFIERS_FIELD_NUMBER;
hash = (53 * hash) + getUserIdentifiersList().hashCode();
}
if (hasUserAgent()) {
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v17.services.ConversionAdjustment prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A conversion adjustment.
*
*
* Protobuf type {@code google.ads.googleads.v17.services.ConversionAdjustment}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v17.services.ConversionAdjustment)
com.google.ads.googleads.v17.services.ConversionAdjustmentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.services.ConversionAdjustmentUploadServiceProto.internal_static_google_ads_googleads_v17_services_ConversionAdjustment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.services.ConversionAdjustmentUploadServiceProto.internal_static_google_ads_googleads_v17_services_ConversionAdjustment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.services.ConversionAdjustment.class, com.google.ads.googleads.v17.services.ConversionAdjustment.Builder.class);
}
// Construct using com.google.ads.googleads.v17.services.ConversionAdjustment.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getGclidDateTimePairFieldBuilder();
getRestatementValueFieldBuilder();
getUserIdentifiersFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
gclidDateTimePair_ = null;
if (gclidDateTimePairBuilder_ != null) {
gclidDateTimePairBuilder_.dispose();
gclidDateTimePairBuilder_ = null;
}
orderId_ = "";
conversionAction_ = "";
adjustmentDateTime_ = "";
adjustmentType_ = 0;
restatementValue_ = null;
if (restatementValueBuilder_ != null) {
restatementValueBuilder_.dispose();
restatementValueBuilder_ = null;
}
if (userIdentifiersBuilder_ == null) {
userIdentifiers_ = java.util.Collections.emptyList();
} else {
userIdentifiers_ = null;
userIdentifiersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
userAgent_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v17.services.ConversionAdjustmentUploadServiceProto.internal_static_google_ads_googleads_v17_services_ConversionAdjustment_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v17.services.ConversionAdjustment getDefaultInstanceForType() {
return com.google.ads.googleads.v17.services.ConversionAdjustment.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v17.services.ConversionAdjustment build() {
com.google.ads.googleads.v17.services.ConversionAdjustment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v17.services.ConversionAdjustment buildPartial() {
com.google.ads.googleads.v17.services.ConversionAdjustment result = new com.google.ads.googleads.v17.services.ConversionAdjustment(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.ads.googleads.v17.services.ConversionAdjustment result) {
if (userIdentifiersBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
userIdentifiers_ = java.util.Collections.unmodifiableList(userIdentifiers_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.userIdentifiers_ = userIdentifiers_;
} else {
result.userIdentifiers_ = userIdentifiersBuilder_.build();
}
}
private void buildPartial0(com.google.ads.googleads.v17.services.ConversionAdjustment result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.gclidDateTimePair_ = gclidDateTimePairBuilder_ == null
? gclidDateTimePair_
: gclidDateTimePairBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.orderId_ = orderId_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.conversionAction_ = conversionAction_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.adjustmentDateTime_ = adjustmentDateTime_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.adjustmentType_ = adjustmentType_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.restatementValue_ = restatementValueBuilder_ == null
? restatementValue_
: restatementValueBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.userAgent_ = userAgent_;
to_bitField0_ |= 0x00000020;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v17.services.ConversionAdjustment) {
return mergeFrom((com.google.ads.googleads.v17.services.ConversionAdjustment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v17.services.ConversionAdjustment other) {
if (other == com.google.ads.googleads.v17.services.ConversionAdjustment.getDefaultInstance()) return this;
if (other.hasGclidDateTimePair()) {
mergeGclidDateTimePair(other.getGclidDateTimePair());
}
if (other.hasOrderId()) {
orderId_ = other.orderId_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasConversionAction()) {
conversionAction_ = other.conversionAction_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasAdjustmentDateTime()) {
adjustmentDateTime_ = other.adjustmentDateTime_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.adjustmentType_ != 0) {
setAdjustmentTypeValue(other.getAdjustmentTypeValue());
}
if (other.hasRestatementValue()) {
mergeRestatementValue(other.getRestatementValue());
}
if (userIdentifiersBuilder_ == null) {
if (!other.userIdentifiers_.isEmpty()) {
if (userIdentifiers_.isEmpty()) {
userIdentifiers_ = other.userIdentifiers_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureUserIdentifiersIsMutable();
userIdentifiers_.addAll(other.userIdentifiers_);
}
onChanged();
}
} else {
if (!other.userIdentifiers_.isEmpty()) {
if (userIdentifiersBuilder_.isEmpty()) {
userIdentifiersBuilder_.dispose();
userIdentifiersBuilder_ = null;
userIdentifiers_ = other.userIdentifiers_;
bitField0_ = (bitField0_ & ~0x00000040);
userIdentifiersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getUserIdentifiersFieldBuilder() : null;
} else {
userIdentifiersBuilder_.addAllMessages(other.userIdentifiers_);
}
}
}
if (other.hasUserAgent()) {
userAgent_ = other.userAgent_;
bitField0_ |= 0x00000080;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 40: {
adjustmentType_ = input.readEnum();
bitField0_ |= 0x00000010;
break;
} // case 40
case 50: {
input.readMessage(
getRestatementValueFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 50
case 66: {
conversionAction_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 66
case 74: {
adjustmentDateTime_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 74
case 82: {
com.google.ads.googleads.v17.common.UserIdentifier m =
input.readMessage(
com.google.ads.googleads.v17.common.UserIdentifier.parser(),
extensionRegistry);
if (userIdentifiersBuilder_ == null) {
ensureUserIdentifiersIsMutable();
userIdentifiers_.add(m);
} else {
userIdentifiersBuilder_.addMessage(m);
}
break;
} // case 82
case 90: {
userAgent_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 90
case 98: {
input.readMessage(
getGclidDateTimePairFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 98
case 106: {
orderId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 106
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.ads.googleads.v17.services.GclidDateTimePair gclidDateTimePair_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.GclidDateTimePair, com.google.ads.googleads.v17.services.GclidDateTimePair.Builder, com.google.ads.googleads.v17.services.GclidDateTimePairOrBuilder> gclidDateTimePairBuilder_;
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
* @return Whether the gclidDateTimePair field is set.
*/
public boolean hasGclidDateTimePair() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
* @return The gclidDateTimePair.
*/
public com.google.ads.googleads.v17.services.GclidDateTimePair getGclidDateTimePair() {
if (gclidDateTimePairBuilder_ == null) {
return gclidDateTimePair_ == null ? com.google.ads.googleads.v17.services.GclidDateTimePair.getDefaultInstance() : gclidDateTimePair_;
} else {
return gclidDateTimePairBuilder_.getMessage();
}
}
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
*/
public Builder setGclidDateTimePair(com.google.ads.googleads.v17.services.GclidDateTimePair value) {
if (gclidDateTimePairBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
gclidDateTimePair_ = value;
} else {
gclidDateTimePairBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
*/
public Builder setGclidDateTimePair(
com.google.ads.googleads.v17.services.GclidDateTimePair.Builder builderForValue) {
if (gclidDateTimePairBuilder_ == null) {
gclidDateTimePair_ = builderForValue.build();
} else {
gclidDateTimePairBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
*/
public Builder mergeGclidDateTimePair(com.google.ads.googleads.v17.services.GclidDateTimePair value) {
if (gclidDateTimePairBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
gclidDateTimePair_ != null &&
gclidDateTimePair_ != com.google.ads.googleads.v17.services.GclidDateTimePair.getDefaultInstance()) {
getGclidDateTimePairBuilder().mergeFrom(value);
} else {
gclidDateTimePair_ = value;
}
} else {
gclidDateTimePairBuilder_.mergeFrom(value);
}
if (gclidDateTimePair_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
*/
public Builder clearGclidDateTimePair() {
bitField0_ = (bitField0_ & ~0x00000001);
gclidDateTimePair_ = null;
if (gclidDateTimePairBuilder_ != null) {
gclidDateTimePairBuilder_.dispose();
gclidDateTimePairBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
*/
public com.google.ads.googleads.v17.services.GclidDateTimePair.Builder getGclidDateTimePairBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getGclidDateTimePairFieldBuilder().getBuilder();
}
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
*/
public com.google.ads.googleads.v17.services.GclidDateTimePairOrBuilder getGclidDateTimePairOrBuilder() {
if (gclidDateTimePairBuilder_ != null) {
return gclidDateTimePairBuilder_.getMessageOrBuilder();
} else {
return gclidDateTimePair_ == null ?
com.google.ads.googleads.v17.services.GclidDateTimePair.getDefaultInstance() : gclidDateTimePair_;
}
}
/**
*
* For adjustments, uniquely identifies a conversion that was reported
* without an order ID specified. If the adjustment_type is ENHANCEMENT, this
* value is optional but may be set in addition to the order_id.
*
*
* .google.ads.googleads.v17.services.GclidDateTimePair gclid_date_time_pair = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.GclidDateTimePair, com.google.ads.googleads.v17.services.GclidDateTimePair.Builder, com.google.ads.googleads.v17.services.GclidDateTimePairOrBuilder>
getGclidDateTimePairFieldBuilder() {
if (gclidDateTimePairBuilder_ == null) {
gclidDateTimePairBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.GclidDateTimePair, com.google.ads.googleads.v17.services.GclidDateTimePair.Builder, com.google.ads.googleads.v17.services.GclidDateTimePairOrBuilder>(
getGclidDateTimePair(),
getParentForChildren(),
isClean());
gclidDateTimePair_ = null;
}
return gclidDateTimePairBuilder_;
}
private java.lang.Object orderId_ = "";
/**
*
* The order ID of the conversion to be adjusted. If the conversion was
* reported with an order ID specified, that order ID must be used as the
* identifier here. The order ID is required for enhancements.
*
*
* optional string order_id = 13;
* @return Whether the orderId field is set.
*/
public boolean hasOrderId() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The order ID of the conversion to be adjusted. If the conversion was
* reported with an order ID specified, that order ID must be used as the
* identifier here. The order ID is required for enhancements.
*
*
* optional string order_id = 13;
* @return The orderId.
*/
public java.lang.String getOrderId() {
java.lang.Object ref = orderId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
orderId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The order ID of the conversion to be adjusted. If the conversion was
* reported with an order ID specified, that order ID must be used as the
* identifier here. The order ID is required for enhancements.
*
*
* optional string order_id = 13;
* @return The bytes for orderId.
*/
public com.google.protobuf.ByteString
getOrderIdBytes() {
java.lang.Object ref = orderId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
orderId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The order ID of the conversion to be adjusted. If the conversion was
* reported with an order ID specified, that order ID must be used as the
* identifier here. The order ID is required for enhancements.
*
*
* optional string order_id = 13;
* @param value The orderId to set.
* @return This builder for chaining.
*/
public Builder setOrderId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
orderId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The order ID of the conversion to be adjusted. If the conversion was
* reported with an order ID specified, that order ID must be used as the
* identifier here. The order ID is required for enhancements.
*
*
* optional string order_id = 13;
* @return This builder for chaining.
*/
public Builder clearOrderId() {
orderId_ = getDefaultInstance().getOrderId();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* The order ID of the conversion to be adjusted. If the conversion was
* reported with an order ID specified, that order ID must be used as the
* identifier here. The order ID is required for enhancements.
*
*
* optional string order_id = 13;
* @param value The bytes for orderId to set.
* @return This builder for chaining.
*/
public Builder setOrderIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
orderId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object conversionAction_ = "";
/**
*
* Resource name of the conversion action associated with this conversion
* adjustment. Note: Although this resource name consists of a customer id and
* a conversion action id, validation will ignore the customer id and use the
* conversion action id as the sole identifier of the conversion action.
*
*
* optional string conversion_action = 8;
* @return Whether the conversionAction field is set.
*/
public boolean hasConversionAction() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Resource name of the conversion action associated with this conversion
* adjustment. Note: Although this resource name consists of a customer id and
* a conversion action id, validation will ignore the customer id and use the
* conversion action id as the sole identifier of the conversion action.
*
*
* optional string conversion_action = 8;
* @return The conversionAction.
*/
public java.lang.String getConversionAction() {
java.lang.Object ref = conversionAction_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
conversionAction_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Resource name of the conversion action associated with this conversion
* adjustment. Note: Although this resource name consists of a customer id and
* a conversion action id, validation will ignore the customer id and use the
* conversion action id as the sole identifier of the conversion action.
*
*
* optional string conversion_action = 8;
* @return The bytes for conversionAction.
*/
public com.google.protobuf.ByteString
getConversionActionBytes() {
java.lang.Object ref = conversionAction_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
conversionAction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Resource name of the conversion action associated with this conversion
* adjustment. Note: Although this resource name consists of a customer id and
* a conversion action id, validation will ignore the customer id and use the
* conversion action id as the sole identifier of the conversion action.
*
*
* optional string conversion_action = 8;
* @param value The conversionAction to set.
* @return This builder for chaining.
*/
public Builder setConversionAction(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
conversionAction_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Resource name of the conversion action associated with this conversion
* adjustment. Note: Although this resource name consists of a customer id and
* a conversion action id, validation will ignore the customer id and use the
* conversion action id as the sole identifier of the conversion action.
*
*
* optional string conversion_action = 8;
* @return This builder for chaining.
*/
public Builder clearConversionAction() {
conversionAction_ = getDefaultInstance().getConversionAction();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* Resource name of the conversion action associated with this conversion
* adjustment. Note: Although this resource name consists of a customer id and
* a conversion action id, validation will ignore the customer id and use the
* conversion action id as the sole identifier of the conversion action.
*
*
* optional string conversion_action = 8;
* @param value The bytes for conversionAction to set.
* @return This builder for chaining.
*/
public Builder setConversionActionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
conversionAction_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object adjustmentDateTime_ = "";
/**
*
* The date time at which the adjustment occurred. Must be after the
* conversion_date_time. The timezone must be specified. The format is
* "yyyy-mm-dd hh:mm:ss+|-hh:mm", for example, "2019-01-01 12:32:45-08:00".
*
*
* optional string adjustment_date_time = 9;
* @return Whether the adjustmentDateTime field is set.
*/
public boolean hasAdjustmentDateTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The date time at which the adjustment occurred. Must be after the
* conversion_date_time. The timezone must be specified. The format is
* "yyyy-mm-dd hh:mm:ss+|-hh:mm", for example, "2019-01-01 12:32:45-08:00".
*
*
* optional string adjustment_date_time = 9;
* @return The adjustmentDateTime.
*/
public java.lang.String getAdjustmentDateTime() {
java.lang.Object ref = adjustmentDateTime_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
adjustmentDateTime_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The date time at which the adjustment occurred. Must be after the
* conversion_date_time. The timezone must be specified. The format is
* "yyyy-mm-dd hh:mm:ss+|-hh:mm", for example, "2019-01-01 12:32:45-08:00".
*
*
* optional string adjustment_date_time = 9;
* @return The bytes for adjustmentDateTime.
*/
public com.google.protobuf.ByteString
getAdjustmentDateTimeBytes() {
java.lang.Object ref = adjustmentDateTime_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
adjustmentDateTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The date time at which the adjustment occurred. Must be after the
* conversion_date_time. The timezone must be specified. The format is
* "yyyy-mm-dd hh:mm:ss+|-hh:mm", for example, "2019-01-01 12:32:45-08:00".
*
*
* optional string adjustment_date_time = 9;
* @param value The adjustmentDateTime to set.
* @return This builder for chaining.
*/
public Builder setAdjustmentDateTime(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
adjustmentDateTime_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The date time at which the adjustment occurred. Must be after the
* conversion_date_time. The timezone must be specified. The format is
* "yyyy-mm-dd hh:mm:ss+|-hh:mm", for example, "2019-01-01 12:32:45-08:00".
*
*
* optional string adjustment_date_time = 9;
* @return This builder for chaining.
*/
public Builder clearAdjustmentDateTime() {
adjustmentDateTime_ = getDefaultInstance().getAdjustmentDateTime();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
* The date time at which the adjustment occurred. Must be after the
* conversion_date_time. The timezone must be specified. The format is
* "yyyy-mm-dd hh:mm:ss+|-hh:mm", for example, "2019-01-01 12:32:45-08:00".
*
*
* optional string adjustment_date_time = 9;
* @param value The bytes for adjustmentDateTime to set.
* @return This builder for chaining.
*/
public Builder setAdjustmentDateTimeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
adjustmentDateTime_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private int adjustmentType_ = 0;
/**
*
* The adjustment type.
*
*
* .google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType adjustment_type = 5;
* @return The enum numeric value on the wire for adjustmentType.
*/
@java.lang.Override public int getAdjustmentTypeValue() {
return adjustmentType_;
}
/**
*
* The adjustment type.
*
*
* .google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType adjustment_type = 5;
* @param value The enum numeric value on the wire for adjustmentType to set.
* @return This builder for chaining.
*/
public Builder setAdjustmentTypeValue(int value) {
adjustmentType_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The adjustment type.
*
*
* .google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType adjustment_type = 5;
* @return The adjustmentType.
*/
@java.lang.Override
public com.google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType getAdjustmentType() {
com.google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType result = com.google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType.forNumber(adjustmentType_);
return result == null ? com.google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType.UNRECOGNIZED : result;
}
/**
*
* The adjustment type.
*
*
* .google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType adjustment_type = 5;
* @param value The adjustmentType to set.
* @return This builder for chaining.
*/
public Builder setAdjustmentType(com.google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
adjustmentType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The adjustment type.
*
*
* .google.ads.googleads.v17.enums.ConversionAdjustmentTypeEnum.ConversionAdjustmentType adjustment_type = 5;
* @return This builder for chaining.
*/
public Builder clearAdjustmentType() {
bitField0_ = (bitField0_ & ~0x00000010);
adjustmentType_ = 0;
onChanged();
return this;
}
private com.google.ads.googleads.v17.services.RestatementValue restatementValue_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.RestatementValue, com.google.ads.googleads.v17.services.RestatementValue.Builder, com.google.ads.googleads.v17.services.RestatementValueOrBuilder> restatementValueBuilder_;
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
* @return Whether the restatementValue field is set.
*/
public boolean hasRestatementValue() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
* @return The restatementValue.
*/
public com.google.ads.googleads.v17.services.RestatementValue getRestatementValue() {
if (restatementValueBuilder_ == null) {
return restatementValue_ == null ? com.google.ads.googleads.v17.services.RestatementValue.getDefaultInstance() : restatementValue_;
} else {
return restatementValueBuilder_.getMessage();
}
}
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
*/
public Builder setRestatementValue(com.google.ads.googleads.v17.services.RestatementValue value) {
if (restatementValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
restatementValue_ = value;
} else {
restatementValueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
*/
public Builder setRestatementValue(
com.google.ads.googleads.v17.services.RestatementValue.Builder builderForValue) {
if (restatementValueBuilder_ == null) {
restatementValue_ = builderForValue.build();
} else {
restatementValueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
*/
public Builder mergeRestatementValue(com.google.ads.googleads.v17.services.RestatementValue value) {
if (restatementValueBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
restatementValue_ != null &&
restatementValue_ != com.google.ads.googleads.v17.services.RestatementValue.getDefaultInstance()) {
getRestatementValueBuilder().mergeFrom(value);
} else {
restatementValue_ = value;
}
} else {
restatementValueBuilder_.mergeFrom(value);
}
if (restatementValue_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
*/
public Builder clearRestatementValue() {
bitField0_ = (bitField0_ & ~0x00000020);
restatementValue_ = null;
if (restatementValueBuilder_ != null) {
restatementValueBuilder_.dispose();
restatementValueBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
*/
public com.google.ads.googleads.v17.services.RestatementValue.Builder getRestatementValueBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getRestatementValueFieldBuilder().getBuilder();
}
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
*/
public com.google.ads.googleads.v17.services.RestatementValueOrBuilder getRestatementValueOrBuilder() {
if (restatementValueBuilder_ != null) {
return restatementValueBuilder_.getMessageOrBuilder();
} else {
return restatementValue_ == null ?
com.google.ads.googleads.v17.services.RestatementValue.getDefaultInstance() : restatementValue_;
}
}
/**
*
* Information needed to restate the conversion's value.
* Required for restatements. Should not be supplied for retractions. An error
* will be returned if provided for a retraction.
* NOTE: If you want to upload a second restatement with a different adjusted
* value, it must have a new, more recent, adjustment occurrence time.
* Otherwise, it will be treated as a duplicate of the previous restatement
* and ignored.
*
*
* .google.ads.googleads.v17.services.RestatementValue restatement_value = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.RestatementValue, com.google.ads.googleads.v17.services.RestatementValue.Builder, com.google.ads.googleads.v17.services.RestatementValueOrBuilder>
getRestatementValueFieldBuilder() {
if (restatementValueBuilder_ == null) {
restatementValueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.RestatementValue, com.google.ads.googleads.v17.services.RestatementValue.Builder, com.google.ads.googleads.v17.services.RestatementValueOrBuilder>(
getRestatementValue(),
getParentForChildren(),
isClean());
restatementValue_ = null;
}
return restatementValueBuilder_;
}
private java.util.List userIdentifiers_ =
java.util.Collections.emptyList();
private void ensureUserIdentifiersIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
userIdentifiers_ = new java.util.ArrayList(userIdentifiers_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.UserIdentifier, com.google.ads.googleads.v17.common.UserIdentifier.Builder, com.google.ads.googleads.v17.common.UserIdentifierOrBuilder> userIdentifiersBuilder_;
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public java.util.List getUserIdentifiersList() {
if (userIdentifiersBuilder_ == null) {
return java.util.Collections.unmodifiableList(userIdentifiers_);
} else {
return userIdentifiersBuilder_.getMessageList();
}
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public int getUserIdentifiersCount() {
if (userIdentifiersBuilder_ == null) {
return userIdentifiers_.size();
} else {
return userIdentifiersBuilder_.getCount();
}
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public com.google.ads.googleads.v17.common.UserIdentifier getUserIdentifiers(int index) {
if (userIdentifiersBuilder_ == null) {
return userIdentifiers_.get(index);
} else {
return userIdentifiersBuilder_.getMessage(index);
}
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public Builder setUserIdentifiers(
int index, com.google.ads.googleads.v17.common.UserIdentifier value) {
if (userIdentifiersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserIdentifiersIsMutable();
userIdentifiers_.set(index, value);
onChanged();
} else {
userIdentifiersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public Builder setUserIdentifiers(
int index, com.google.ads.googleads.v17.common.UserIdentifier.Builder builderForValue) {
if (userIdentifiersBuilder_ == null) {
ensureUserIdentifiersIsMutable();
userIdentifiers_.set(index, builderForValue.build());
onChanged();
} else {
userIdentifiersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public Builder addUserIdentifiers(com.google.ads.googleads.v17.common.UserIdentifier value) {
if (userIdentifiersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserIdentifiersIsMutable();
userIdentifiers_.add(value);
onChanged();
} else {
userIdentifiersBuilder_.addMessage(value);
}
return this;
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public Builder addUserIdentifiers(
int index, com.google.ads.googleads.v17.common.UserIdentifier value) {
if (userIdentifiersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserIdentifiersIsMutable();
userIdentifiers_.add(index, value);
onChanged();
} else {
userIdentifiersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public Builder addUserIdentifiers(
com.google.ads.googleads.v17.common.UserIdentifier.Builder builderForValue) {
if (userIdentifiersBuilder_ == null) {
ensureUserIdentifiersIsMutable();
userIdentifiers_.add(builderForValue.build());
onChanged();
} else {
userIdentifiersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public Builder addUserIdentifiers(
int index, com.google.ads.googleads.v17.common.UserIdentifier.Builder builderForValue) {
if (userIdentifiersBuilder_ == null) {
ensureUserIdentifiersIsMutable();
userIdentifiers_.add(index, builderForValue.build());
onChanged();
} else {
userIdentifiersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public Builder addAllUserIdentifiers(
java.lang.Iterable extends com.google.ads.googleads.v17.common.UserIdentifier> values) {
if (userIdentifiersBuilder_ == null) {
ensureUserIdentifiersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, userIdentifiers_);
onChanged();
} else {
userIdentifiersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public Builder clearUserIdentifiers() {
if (userIdentifiersBuilder_ == null) {
userIdentifiers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
userIdentifiersBuilder_.clear();
}
return this;
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public Builder removeUserIdentifiers(int index) {
if (userIdentifiersBuilder_ == null) {
ensureUserIdentifiersIsMutable();
userIdentifiers_.remove(index);
onChanged();
} else {
userIdentifiersBuilder_.remove(index);
}
return this;
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public com.google.ads.googleads.v17.common.UserIdentifier.Builder getUserIdentifiersBuilder(
int index) {
return getUserIdentifiersFieldBuilder().getBuilder(index);
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public com.google.ads.googleads.v17.common.UserIdentifierOrBuilder getUserIdentifiersOrBuilder(
int index) {
if (userIdentifiersBuilder_ == null) {
return userIdentifiers_.get(index); } else {
return userIdentifiersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public java.util.List extends com.google.ads.googleads.v17.common.UserIdentifierOrBuilder>
getUserIdentifiersOrBuilderList() {
if (userIdentifiersBuilder_ != null) {
return userIdentifiersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(userIdentifiers_);
}
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public com.google.ads.googleads.v17.common.UserIdentifier.Builder addUserIdentifiersBuilder() {
return getUserIdentifiersFieldBuilder().addBuilder(
com.google.ads.googleads.v17.common.UserIdentifier.getDefaultInstance());
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public com.google.ads.googleads.v17.common.UserIdentifier.Builder addUserIdentifiersBuilder(
int index) {
return getUserIdentifiersFieldBuilder().addBuilder(
index, com.google.ads.googleads.v17.common.UserIdentifier.getDefaultInstance());
}
/**
*
* The user identifiers to enhance the original conversion.
* ConversionAdjustmentUploadService only accepts user identifiers in
* enhancements. The maximum number of user identifiers for each
* enhancement is 5.
*
*
* repeated .google.ads.googleads.v17.common.UserIdentifier user_identifiers = 10;
*/
public java.util.List
getUserIdentifiersBuilderList() {
return getUserIdentifiersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.UserIdentifier, com.google.ads.googleads.v17.common.UserIdentifier.Builder, com.google.ads.googleads.v17.common.UserIdentifierOrBuilder>
getUserIdentifiersFieldBuilder() {
if (userIdentifiersBuilder_ == null) {
userIdentifiersBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.common.UserIdentifier, com.google.ads.googleads.v17.common.UserIdentifier.Builder, com.google.ads.googleads.v17.common.UserIdentifierOrBuilder>(
userIdentifiers_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
userIdentifiers_ = null;
}
return userIdentifiersBuilder_;
}
private java.lang.Object userAgent_ = "";
/**
*
* The user agent to enhance the original conversion. This can be found in
* your user's HTTP request header when they convert on your web page.
* Example, "Mozilla/5.0 (iPhone; CPU iPhone OS 12_2 like Mac OS X)". User
* agent can only be specified in enhancements with user identifiers. This
* should match the user agent of the request that sent the original
* conversion so the conversion and its enhancement are either both attributed
* as same-device or both attributed as cross-device.
*
*
* optional string user_agent = 11;
* @return Whether the userAgent field is set.
*/
public boolean hasUserAgent() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* The user agent to enhance the original conversion. This can be found in
* your user's HTTP request header when they convert on your web page.
* Example, "Mozilla/5.0 (iPhone; CPU iPhone OS 12_2 like Mac OS X)". User
* agent can only be specified in enhancements with user identifiers. This
* should match the user agent of the request that sent the original
* conversion so the conversion and its enhancement are either both attributed
* as same-device or both attributed as cross-device.
*
*
* optional string user_agent = 11;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The user agent to enhance the original conversion. This can be found in
* your user's HTTP request header when they convert on your web page.
* Example, "Mozilla/5.0 (iPhone; CPU iPhone OS 12_2 like Mac OS X)". User
* agent can only be specified in enhancements with user identifiers. This
* should match the user agent of the request that sent the original
* conversion so the conversion and its enhancement are either both attributed
* as same-device or both attributed as cross-device.
*
*
* optional string user_agent = 11;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The user agent to enhance the original conversion. This can be found in
* your user's HTTP request header when they convert on your web page.
* Example, "Mozilla/5.0 (iPhone; CPU iPhone OS 12_2 like Mac OS X)". User
* agent can only be specified in enhancements with user identifiers. This
* should match the user agent of the request that sent the original
* conversion so the conversion and its enhancement are either both attributed
* as same-device or both attributed as cross-device.
*
*
* optional string user_agent = 11;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
userAgent_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* The user agent to enhance the original conversion. This can be found in
* your user's HTTP request header when they convert on your web page.
* Example, "Mozilla/5.0 (iPhone; CPU iPhone OS 12_2 like Mac OS X)". User
* agent can only be specified in enhancements with user identifiers. This
* should match the user agent of the request that sent the original
* conversion so the conversion and its enhancement are either both attributed
* as same-device or both attributed as cross-device.
*
*
* optional string user_agent = 11;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
* The user agent to enhance the original conversion. This can be found in
* your user's HTTP request header when they convert on your web page.
* Example, "Mozilla/5.0 (iPhone; CPU iPhone OS 12_2 like Mac OS X)". User
* agent can only be specified in enhancements with user identifiers. This
* should match the user agent of the request that sent the original
* conversion so the conversion and its enhancement are either both attributed
* as same-device or both attributed as cross-device.
*
*
* optional string user_agent = 11;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
userAgent_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v17.services.ConversionAdjustment)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v17.services.ConversionAdjustment)
private static final com.google.ads.googleads.v17.services.ConversionAdjustment DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v17.services.ConversionAdjustment();
}
public static com.google.ads.googleads.v17.services.ConversionAdjustment getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ConversionAdjustment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v17.services.ConversionAdjustment getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy