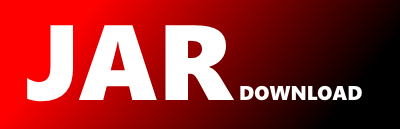
com.google.ads.googleads.v17.services.GenerateReachForecastRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-ads-stubs-v17 Show documentation
Show all versions of google-ads-stubs-v17 Show documentation
Stubs for GAAPI version google-ads-stubs-v17
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/ads/googleads/v17/services/reach_plan_service.proto
// Protobuf Java Version: 3.25.3
package com.google.ads.googleads.v17.services;
/**
*
* Request message for
* [ReachPlanService.GenerateReachForecast][google.ads.googleads.v17.services.ReachPlanService.GenerateReachForecast].
*
*
* Protobuf type {@code google.ads.googleads.v17.services.GenerateReachForecastRequest}
*/
public final class GenerateReachForecastRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.ads.googleads.v17.services.GenerateReachForecastRequest)
GenerateReachForecastRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GenerateReachForecastRequest.newBuilder() to construct.
private GenerateReachForecastRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GenerateReachForecastRequest() {
customerId_ = "";
currencyCode_ = "";
plannedProducts_ = java.util.Collections.emptyList();
customerReachGroup_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GenerateReachForecastRequest();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.services.ReachPlanServiceProto.internal_static_google_ads_googleads_v17_services_GenerateReachForecastRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.services.ReachPlanServiceProto.internal_static_google_ads_googleads_v17_services_GenerateReachForecastRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.services.GenerateReachForecastRequest.class, com.google.ads.googleads.v17.services.GenerateReachForecastRequest.Builder.class);
}
private int bitField0_;
public static final int CUSTOMER_ID_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object customerId_ = "";
/**
*
* Required. The ID of the customer.
*
*
* string customer_id = 1 [(.google.api.field_behavior) = REQUIRED];
* @return The customerId.
*/
@java.lang.Override
public java.lang.String getCustomerId() {
java.lang.Object ref = customerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerId_ = s;
return s;
}
}
/**
*
* Required. The ID of the customer.
*
*
* string customer_id = 1 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for customerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCustomerIdBytes() {
java.lang.Object ref = customerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CURRENCY_CODE_FIELD_NUMBER = 9;
@SuppressWarnings("serial")
private volatile java.lang.Object currencyCode_ = "";
/**
*
* The currency code.
* Three-character ISO 4217 currency code.
*
*
* optional string currency_code = 9;
* @return Whether the currencyCode field is set.
*/
@java.lang.Override
public boolean hasCurrencyCode() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The currency code.
* Three-character ISO 4217 currency code.
*
*
* optional string currency_code = 9;
* @return The currencyCode.
*/
@java.lang.Override
public java.lang.String getCurrencyCode() {
java.lang.Object ref = currencyCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currencyCode_ = s;
return s;
}
}
/**
*
* The currency code.
* Three-character ISO 4217 currency code.
*
*
* optional string currency_code = 9;
* @return The bytes for currencyCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCurrencyCodeBytes() {
java.lang.Object ref = currencyCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CAMPAIGN_DURATION_FIELD_NUMBER = 3;
private com.google.ads.googleads.v17.services.CampaignDuration campaignDuration_;
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
* @return Whether the campaignDuration field is set.
*/
@java.lang.Override
public boolean hasCampaignDuration() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
* @return The campaignDuration.
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.CampaignDuration getCampaignDuration() {
return campaignDuration_ == null ? com.google.ads.googleads.v17.services.CampaignDuration.getDefaultInstance() : campaignDuration_;
}
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.CampaignDurationOrBuilder getCampaignDurationOrBuilder() {
return campaignDuration_ == null ? com.google.ads.googleads.v17.services.CampaignDuration.getDefaultInstance() : campaignDuration_;
}
public static final int COOKIE_FREQUENCY_CAP_FIELD_NUMBER = 10;
private int cookieFrequencyCap_ = 0;
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user.
* If not specified, no cap is applied.
*
* This field is deprecated in v4 and will eventually be removed.
* Use cookie_frequency_cap_setting instead.
*
*
* optional int32 cookie_frequency_cap = 10;
* @return Whether the cookieFrequencyCap field is set.
*/
@java.lang.Override
public boolean hasCookieFrequencyCap() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user.
* If not specified, no cap is applied.
*
* This field is deprecated in v4 and will eventually be removed.
* Use cookie_frequency_cap_setting instead.
*
*
* optional int32 cookie_frequency_cap = 10;
* @return The cookieFrequencyCap.
*/
@java.lang.Override
public int getCookieFrequencyCap() {
return cookieFrequencyCap_;
}
public static final int COOKIE_FREQUENCY_CAP_SETTING_FIELD_NUMBER = 8;
private com.google.ads.googleads.v17.services.FrequencyCap cookieFrequencyCapSetting_;
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
* @return Whether the cookieFrequencyCapSetting field is set.
*/
@java.lang.Override
public boolean hasCookieFrequencyCapSetting() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
* @return The cookieFrequencyCapSetting.
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.FrequencyCap getCookieFrequencyCapSetting() {
return cookieFrequencyCapSetting_ == null ? com.google.ads.googleads.v17.services.FrequencyCap.getDefaultInstance() : cookieFrequencyCapSetting_;
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.FrequencyCapOrBuilder getCookieFrequencyCapSettingOrBuilder() {
return cookieFrequencyCapSetting_ == null ? com.google.ads.googleads.v17.services.FrequencyCap.getDefaultInstance() : cookieFrequencyCapSetting_;
}
public static final int MIN_EFFECTIVE_FREQUENCY_FIELD_NUMBER = 11;
private int minEffectiveFrequency_ = 0;
/**
*
* Chosen minimum effective frequency (the number of times a person was
* exposed to the ad) for the reported reach metrics [1-10].
* This won't affect the targeting, but just the reporting.
* If not specified, a default of 1 is applied.
*
* This field cannot be combined with the effective_frequency_limit field.
*
*
* optional int32 min_effective_frequency = 11;
* @return Whether the minEffectiveFrequency field is set.
*/
@java.lang.Override
public boolean hasMinEffectiveFrequency() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Chosen minimum effective frequency (the number of times a person was
* exposed to the ad) for the reported reach metrics [1-10].
* This won't affect the targeting, but just the reporting.
* If not specified, a default of 1 is applied.
*
* This field cannot be combined with the effective_frequency_limit field.
*
*
* optional int32 min_effective_frequency = 11;
* @return The minEffectiveFrequency.
*/
@java.lang.Override
public int getMinEffectiveFrequency() {
return minEffectiveFrequency_;
}
public static final int EFFECTIVE_FREQUENCY_LIMIT_FIELD_NUMBER = 12;
private com.google.ads.googleads.v17.services.EffectiveFrequencyLimit effectiveFrequencyLimit_;
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
* @return Whether the effectiveFrequencyLimit field is set.
*/
@java.lang.Override
public boolean hasEffectiveFrequencyLimit() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
* @return The effectiveFrequencyLimit.
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.EffectiveFrequencyLimit getEffectiveFrequencyLimit() {
return effectiveFrequencyLimit_ == null ? com.google.ads.googleads.v17.services.EffectiveFrequencyLimit.getDefaultInstance() : effectiveFrequencyLimit_;
}
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.EffectiveFrequencyLimitOrBuilder getEffectiveFrequencyLimitOrBuilder() {
return effectiveFrequencyLimit_ == null ? com.google.ads.googleads.v17.services.EffectiveFrequencyLimit.getDefaultInstance() : effectiveFrequencyLimit_;
}
public static final int TARGETING_FIELD_NUMBER = 6;
private com.google.ads.googleads.v17.services.Targeting targeting_;
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
* @return Whether the targeting field is set.
*/
@java.lang.Override
public boolean hasTargeting() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
* @return The targeting.
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.Targeting getTargeting() {
return targeting_ == null ? com.google.ads.googleads.v17.services.Targeting.getDefaultInstance() : targeting_;
}
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.TargetingOrBuilder getTargetingOrBuilder() {
return targeting_ == null ? com.google.ads.googleads.v17.services.Targeting.getDefaultInstance() : targeting_;
}
public static final int PLANNED_PRODUCTS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private java.util.List plannedProducts_;
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
@java.lang.Override
public java.util.List getPlannedProductsList() {
return plannedProducts_;
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
@java.lang.Override
public java.util.List extends com.google.ads.googleads.v17.services.PlannedProductOrBuilder>
getPlannedProductsOrBuilderList() {
return plannedProducts_;
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
@java.lang.Override
public int getPlannedProductsCount() {
return plannedProducts_.size();
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.PlannedProduct getPlannedProducts(int index) {
return plannedProducts_.get(index);
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.PlannedProductOrBuilder getPlannedProductsOrBuilder(
int index) {
return plannedProducts_.get(index);
}
public static final int FORECAST_METRIC_OPTIONS_FIELD_NUMBER = 13;
private com.google.ads.googleads.v17.services.ForecastMetricOptions forecastMetricOptions_;
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
* @return Whether the forecastMetricOptions field is set.
*/
@java.lang.Override
public boolean hasForecastMetricOptions() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
* @return The forecastMetricOptions.
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.ForecastMetricOptions getForecastMetricOptions() {
return forecastMetricOptions_ == null ? com.google.ads.googleads.v17.services.ForecastMetricOptions.getDefaultInstance() : forecastMetricOptions_;
}
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
*/
@java.lang.Override
public com.google.ads.googleads.v17.services.ForecastMetricOptionsOrBuilder getForecastMetricOptionsOrBuilder() {
return forecastMetricOptions_ == null ? com.google.ads.googleads.v17.services.ForecastMetricOptions.getDefaultInstance() : forecastMetricOptions_;
}
public static final int CUSTOMER_REACH_GROUP_FIELD_NUMBER = 14;
@SuppressWarnings("serial")
private volatile java.lang.Object customerReachGroup_ = "";
/**
*
* The name of the customer being planned for. This is a user-defined value.
*
*
* optional string customer_reach_group = 14;
* @return Whether the customerReachGroup field is set.
*/
@java.lang.Override
public boolean hasCustomerReachGroup() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* The name of the customer being planned for. This is a user-defined value.
*
*
* optional string customer_reach_group = 14;
* @return The customerReachGroup.
*/
@java.lang.Override
public java.lang.String getCustomerReachGroup() {
java.lang.Object ref = customerReachGroup_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerReachGroup_ = s;
return s;
}
}
/**
*
* The name of the customer being planned for. This is a user-defined value.
*
*
* optional string customer_reach_group = 14;
* @return The bytes for customerReachGroup.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCustomerReachGroupBytes() {
java.lang.Object ref = customerReachGroup_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerReachGroup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(customerId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, customerId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(3, getCampaignDuration());
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(6, getTargeting());
}
for (int i = 0; i < plannedProducts_.size(); i++) {
output.writeMessage(7, plannedProducts_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(8, getCookieFrequencyCapSetting());
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, currencyCode_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt32(10, cookieFrequencyCap_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt32(11, minEffectiveFrequency_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(12, getEffectiveFrequencyLimit());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeMessage(13, getForecastMetricOptions());
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, customerReachGroup_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(customerId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, customerId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getCampaignDuration());
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getTargeting());
}
for (int i = 0; i < plannedProducts_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, plannedProducts_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getCookieFrequencyCapSetting());
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, currencyCode_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(10, cookieFrequencyCap_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(11, minEffectiveFrequency_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getEffectiveFrequencyLimit());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getForecastMetricOptions());
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, customerReachGroup_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ads.googleads.v17.services.GenerateReachForecastRequest)) {
return super.equals(obj);
}
com.google.ads.googleads.v17.services.GenerateReachForecastRequest other = (com.google.ads.googleads.v17.services.GenerateReachForecastRequest) obj;
if (!getCustomerId()
.equals(other.getCustomerId())) return false;
if (hasCurrencyCode() != other.hasCurrencyCode()) return false;
if (hasCurrencyCode()) {
if (!getCurrencyCode()
.equals(other.getCurrencyCode())) return false;
}
if (hasCampaignDuration() != other.hasCampaignDuration()) return false;
if (hasCampaignDuration()) {
if (!getCampaignDuration()
.equals(other.getCampaignDuration())) return false;
}
if (hasCookieFrequencyCap() != other.hasCookieFrequencyCap()) return false;
if (hasCookieFrequencyCap()) {
if (getCookieFrequencyCap()
!= other.getCookieFrequencyCap()) return false;
}
if (hasCookieFrequencyCapSetting() != other.hasCookieFrequencyCapSetting()) return false;
if (hasCookieFrequencyCapSetting()) {
if (!getCookieFrequencyCapSetting()
.equals(other.getCookieFrequencyCapSetting())) return false;
}
if (hasMinEffectiveFrequency() != other.hasMinEffectiveFrequency()) return false;
if (hasMinEffectiveFrequency()) {
if (getMinEffectiveFrequency()
!= other.getMinEffectiveFrequency()) return false;
}
if (hasEffectiveFrequencyLimit() != other.hasEffectiveFrequencyLimit()) return false;
if (hasEffectiveFrequencyLimit()) {
if (!getEffectiveFrequencyLimit()
.equals(other.getEffectiveFrequencyLimit())) return false;
}
if (hasTargeting() != other.hasTargeting()) return false;
if (hasTargeting()) {
if (!getTargeting()
.equals(other.getTargeting())) return false;
}
if (!getPlannedProductsList()
.equals(other.getPlannedProductsList())) return false;
if (hasForecastMetricOptions() != other.hasForecastMetricOptions()) return false;
if (hasForecastMetricOptions()) {
if (!getForecastMetricOptions()
.equals(other.getForecastMetricOptions())) return false;
}
if (hasCustomerReachGroup() != other.hasCustomerReachGroup()) return false;
if (hasCustomerReachGroup()) {
if (!getCustomerReachGroup()
.equals(other.getCustomerReachGroup())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CUSTOMER_ID_FIELD_NUMBER;
hash = (53 * hash) + getCustomerId().hashCode();
if (hasCurrencyCode()) {
hash = (37 * hash) + CURRENCY_CODE_FIELD_NUMBER;
hash = (53 * hash) + getCurrencyCode().hashCode();
}
if (hasCampaignDuration()) {
hash = (37 * hash) + CAMPAIGN_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getCampaignDuration().hashCode();
}
if (hasCookieFrequencyCap()) {
hash = (37 * hash) + COOKIE_FREQUENCY_CAP_FIELD_NUMBER;
hash = (53 * hash) + getCookieFrequencyCap();
}
if (hasCookieFrequencyCapSetting()) {
hash = (37 * hash) + COOKIE_FREQUENCY_CAP_SETTING_FIELD_NUMBER;
hash = (53 * hash) + getCookieFrequencyCapSetting().hashCode();
}
if (hasMinEffectiveFrequency()) {
hash = (37 * hash) + MIN_EFFECTIVE_FREQUENCY_FIELD_NUMBER;
hash = (53 * hash) + getMinEffectiveFrequency();
}
if (hasEffectiveFrequencyLimit()) {
hash = (37 * hash) + EFFECTIVE_FREQUENCY_LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getEffectiveFrequencyLimit().hashCode();
}
if (hasTargeting()) {
hash = (37 * hash) + TARGETING_FIELD_NUMBER;
hash = (53 * hash) + getTargeting().hashCode();
}
if (getPlannedProductsCount() > 0) {
hash = (37 * hash) + PLANNED_PRODUCTS_FIELD_NUMBER;
hash = (53 * hash) + getPlannedProductsList().hashCode();
}
if (hasForecastMetricOptions()) {
hash = (37 * hash) + FORECAST_METRIC_OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + getForecastMetricOptions().hashCode();
}
if (hasCustomerReachGroup()) {
hash = (37 * hash) + CUSTOMER_REACH_GROUP_FIELD_NUMBER;
hash = (53 * hash) + getCustomerReachGroup().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ads.googleads.v17.services.GenerateReachForecastRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Request message for
* [ReachPlanService.GenerateReachForecast][google.ads.googleads.v17.services.ReachPlanService.GenerateReachForecast].
*
*
* Protobuf type {@code google.ads.googleads.v17.services.GenerateReachForecastRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.ads.googleads.v17.services.GenerateReachForecastRequest)
com.google.ads.googleads.v17.services.GenerateReachForecastRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ads.googleads.v17.services.ReachPlanServiceProto.internal_static_google_ads_googleads_v17_services_GenerateReachForecastRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ads.googleads.v17.services.ReachPlanServiceProto.internal_static_google_ads_googleads_v17_services_GenerateReachForecastRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ads.googleads.v17.services.GenerateReachForecastRequest.class, com.google.ads.googleads.v17.services.GenerateReachForecastRequest.Builder.class);
}
// Construct using com.google.ads.googleads.v17.services.GenerateReachForecastRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCampaignDurationFieldBuilder();
getCookieFrequencyCapSettingFieldBuilder();
getEffectiveFrequencyLimitFieldBuilder();
getTargetingFieldBuilder();
getPlannedProductsFieldBuilder();
getForecastMetricOptionsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
customerId_ = "";
currencyCode_ = "";
campaignDuration_ = null;
if (campaignDurationBuilder_ != null) {
campaignDurationBuilder_.dispose();
campaignDurationBuilder_ = null;
}
cookieFrequencyCap_ = 0;
cookieFrequencyCapSetting_ = null;
if (cookieFrequencyCapSettingBuilder_ != null) {
cookieFrequencyCapSettingBuilder_.dispose();
cookieFrequencyCapSettingBuilder_ = null;
}
minEffectiveFrequency_ = 0;
effectiveFrequencyLimit_ = null;
if (effectiveFrequencyLimitBuilder_ != null) {
effectiveFrequencyLimitBuilder_.dispose();
effectiveFrequencyLimitBuilder_ = null;
}
targeting_ = null;
if (targetingBuilder_ != null) {
targetingBuilder_.dispose();
targetingBuilder_ = null;
}
if (plannedProductsBuilder_ == null) {
plannedProducts_ = java.util.Collections.emptyList();
} else {
plannedProducts_ = null;
plannedProductsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
forecastMetricOptions_ = null;
if (forecastMetricOptionsBuilder_ != null) {
forecastMetricOptionsBuilder_.dispose();
forecastMetricOptionsBuilder_ = null;
}
customerReachGroup_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ads.googleads.v17.services.ReachPlanServiceProto.internal_static_google_ads_googleads_v17_services_GenerateReachForecastRequest_descriptor;
}
@java.lang.Override
public com.google.ads.googleads.v17.services.GenerateReachForecastRequest getDefaultInstanceForType() {
return com.google.ads.googleads.v17.services.GenerateReachForecastRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.ads.googleads.v17.services.GenerateReachForecastRequest build() {
com.google.ads.googleads.v17.services.GenerateReachForecastRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ads.googleads.v17.services.GenerateReachForecastRequest buildPartial() {
com.google.ads.googleads.v17.services.GenerateReachForecastRequest result = new com.google.ads.googleads.v17.services.GenerateReachForecastRequest(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.ads.googleads.v17.services.GenerateReachForecastRequest result) {
if (plannedProductsBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)) {
plannedProducts_ = java.util.Collections.unmodifiableList(plannedProducts_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.plannedProducts_ = plannedProducts_;
} else {
result.plannedProducts_ = plannedProductsBuilder_.build();
}
}
private void buildPartial0(com.google.ads.googleads.v17.services.GenerateReachForecastRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.customerId_ = customerId_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.currencyCode_ = currencyCode_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.campaignDuration_ = campaignDurationBuilder_ == null
? campaignDuration_
: campaignDurationBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.cookieFrequencyCap_ = cookieFrequencyCap_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.cookieFrequencyCapSetting_ = cookieFrequencyCapSettingBuilder_ == null
? cookieFrequencyCapSetting_
: cookieFrequencyCapSettingBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.minEffectiveFrequency_ = minEffectiveFrequency_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.effectiveFrequencyLimit_ = effectiveFrequencyLimitBuilder_ == null
? effectiveFrequencyLimit_
: effectiveFrequencyLimitBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.targeting_ = targetingBuilder_ == null
? targeting_
: targetingBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.forecastMetricOptions_ = forecastMetricOptionsBuilder_ == null
? forecastMetricOptions_
: forecastMetricOptionsBuilder_.build();
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.customerReachGroup_ = customerReachGroup_;
to_bitField0_ |= 0x00000100;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ads.googleads.v17.services.GenerateReachForecastRequest) {
return mergeFrom((com.google.ads.googleads.v17.services.GenerateReachForecastRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ads.googleads.v17.services.GenerateReachForecastRequest other) {
if (other == com.google.ads.googleads.v17.services.GenerateReachForecastRequest.getDefaultInstance()) return this;
if (!other.getCustomerId().isEmpty()) {
customerId_ = other.customerId_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasCurrencyCode()) {
currencyCode_ = other.currencyCode_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasCampaignDuration()) {
mergeCampaignDuration(other.getCampaignDuration());
}
if (other.hasCookieFrequencyCap()) {
setCookieFrequencyCap(other.getCookieFrequencyCap());
}
if (other.hasCookieFrequencyCapSetting()) {
mergeCookieFrequencyCapSetting(other.getCookieFrequencyCapSetting());
}
if (other.hasMinEffectiveFrequency()) {
setMinEffectiveFrequency(other.getMinEffectiveFrequency());
}
if (other.hasEffectiveFrequencyLimit()) {
mergeEffectiveFrequencyLimit(other.getEffectiveFrequencyLimit());
}
if (other.hasTargeting()) {
mergeTargeting(other.getTargeting());
}
if (plannedProductsBuilder_ == null) {
if (!other.plannedProducts_.isEmpty()) {
if (plannedProducts_.isEmpty()) {
plannedProducts_ = other.plannedProducts_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensurePlannedProductsIsMutable();
plannedProducts_.addAll(other.plannedProducts_);
}
onChanged();
}
} else {
if (!other.plannedProducts_.isEmpty()) {
if (plannedProductsBuilder_.isEmpty()) {
plannedProductsBuilder_.dispose();
plannedProductsBuilder_ = null;
plannedProducts_ = other.plannedProducts_;
bitField0_ = (bitField0_ & ~0x00000100);
plannedProductsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getPlannedProductsFieldBuilder() : null;
} else {
plannedProductsBuilder_.addAllMessages(other.plannedProducts_);
}
}
}
if (other.hasForecastMetricOptions()) {
mergeForecastMetricOptions(other.getForecastMetricOptions());
}
if (other.hasCustomerReachGroup()) {
customerReachGroup_ = other.customerReachGroup_;
bitField0_ |= 0x00000400;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
customerId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 26: {
input.readMessage(
getCampaignDurationFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 50: {
input.readMessage(
getTargetingFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 50
case 58: {
com.google.ads.googleads.v17.services.PlannedProduct m =
input.readMessage(
com.google.ads.googleads.v17.services.PlannedProduct.parser(),
extensionRegistry);
if (plannedProductsBuilder_ == null) {
ensurePlannedProductsIsMutable();
plannedProducts_.add(m);
} else {
plannedProductsBuilder_.addMessage(m);
}
break;
} // case 58
case 66: {
input.readMessage(
getCookieFrequencyCapSettingFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 66
case 74: {
currencyCode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 74
case 80: {
cookieFrequencyCap_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 80
case 88: {
minEffectiveFrequency_ = input.readInt32();
bitField0_ |= 0x00000020;
break;
} // case 88
case 98: {
input.readMessage(
getEffectiveFrequencyLimitFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 98
case 106: {
input.readMessage(
getForecastMetricOptionsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000200;
break;
} // case 106
case 114: {
customerReachGroup_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000400;
break;
} // case 114
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object customerId_ = "";
/**
*
* Required. The ID of the customer.
*
*
* string customer_id = 1 [(.google.api.field_behavior) = REQUIRED];
* @return The customerId.
*/
public java.lang.String getCustomerId() {
java.lang.Object ref = customerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required. The ID of the customer.
*
*
* string customer_id = 1 [(.google.api.field_behavior) = REQUIRED];
* @return The bytes for customerId.
*/
public com.google.protobuf.ByteString
getCustomerIdBytes() {
java.lang.Object ref = customerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required. The ID of the customer.
*
*
* string customer_id = 1 [(.google.api.field_behavior) = REQUIRED];
* @param value The customerId to set.
* @return This builder for chaining.
*/
public Builder setCustomerId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
customerId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Required. The ID of the customer.
*
*
* string customer_id = 1 [(.google.api.field_behavior) = REQUIRED];
* @return This builder for chaining.
*/
public Builder clearCustomerId() {
customerId_ = getDefaultInstance().getCustomerId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Required. The ID of the customer.
*
*
* string customer_id = 1 [(.google.api.field_behavior) = REQUIRED];
* @param value The bytes for customerId to set.
* @return This builder for chaining.
*/
public Builder setCustomerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
customerId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object currencyCode_ = "";
/**
*
* The currency code.
* Three-character ISO 4217 currency code.
*
*
* optional string currency_code = 9;
* @return Whether the currencyCode field is set.
*/
public boolean hasCurrencyCode() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The currency code.
* Three-character ISO 4217 currency code.
*
*
* optional string currency_code = 9;
* @return The currencyCode.
*/
public java.lang.String getCurrencyCode() {
java.lang.Object ref = currencyCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currencyCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The currency code.
* Three-character ISO 4217 currency code.
*
*
* optional string currency_code = 9;
* @return The bytes for currencyCode.
*/
public com.google.protobuf.ByteString
getCurrencyCodeBytes() {
java.lang.Object ref = currencyCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The currency code.
* Three-character ISO 4217 currency code.
*
*
* optional string currency_code = 9;
* @param value The currencyCode to set.
* @return This builder for chaining.
*/
public Builder setCurrencyCode(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
currencyCode_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The currency code.
* Three-character ISO 4217 currency code.
*
*
* optional string currency_code = 9;
* @return This builder for chaining.
*/
public Builder clearCurrencyCode() {
currencyCode_ = getDefaultInstance().getCurrencyCode();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* The currency code.
* Three-character ISO 4217 currency code.
*
*
* optional string currency_code = 9;
* @param value The bytes for currencyCode to set.
* @return This builder for chaining.
*/
public Builder setCurrencyCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
currencyCode_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.ads.googleads.v17.services.CampaignDuration campaignDuration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.CampaignDuration, com.google.ads.googleads.v17.services.CampaignDuration.Builder, com.google.ads.googleads.v17.services.CampaignDurationOrBuilder> campaignDurationBuilder_;
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
* @return Whether the campaignDuration field is set.
*/
public boolean hasCampaignDuration() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
* @return The campaignDuration.
*/
public com.google.ads.googleads.v17.services.CampaignDuration getCampaignDuration() {
if (campaignDurationBuilder_ == null) {
return campaignDuration_ == null ? com.google.ads.googleads.v17.services.CampaignDuration.getDefaultInstance() : campaignDuration_;
} else {
return campaignDurationBuilder_.getMessage();
}
}
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder setCampaignDuration(com.google.ads.googleads.v17.services.CampaignDuration value) {
if (campaignDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
campaignDuration_ = value;
} else {
campaignDurationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder setCampaignDuration(
com.google.ads.googleads.v17.services.CampaignDuration.Builder builderForValue) {
if (campaignDurationBuilder_ == null) {
campaignDuration_ = builderForValue.build();
} else {
campaignDurationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder mergeCampaignDuration(com.google.ads.googleads.v17.services.CampaignDuration value) {
if (campaignDurationBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
campaignDuration_ != null &&
campaignDuration_ != com.google.ads.googleads.v17.services.CampaignDuration.getDefaultInstance()) {
getCampaignDurationBuilder().mergeFrom(value);
} else {
campaignDuration_ = value;
}
} else {
campaignDurationBuilder_.mergeFrom(value);
}
if (campaignDuration_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder clearCampaignDuration() {
bitField0_ = (bitField0_ & ~0x00000004);
campaignDuration_ = null;
if (campaignDurationBuilder_ != null) {
campaignDurationBuilder_.dispose();
campaignDurationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
*/
public com.google.ads.googleads.v17.services.CampaignDuration.Builder getCampaignDurationBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getCampaignDurationFieldBuilder().getBuilder();
}
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
*/
public com.google.ads.googleads.v17.services.CampaignDurationOrBuilder getCampaignDurationOrBuilder() {
if (campaignDurationBuilder_ != null) {
return campaignDurationBuilder_.getMessageOrBuilder();
} else {
return campaignDuration_ == null ?
com.google.ads.googleads.v17.services.CampaignDuration.getDefaultInstance() : campaignDuration_;
}
}
/**
*
* Required. Campaign duration.
*
*
* .google.ads.googleads.v17.services.CampaignDuration campaign_duration = 3 [(.google.api.field_behavior) = REQUIRED];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.CampaignDuration, com.google.ads.googleads.v17.services.CampaignDuration.Builder, com.google.ads.googleads.v17.services.CampaignDurationOrBuilder>
getCampaignDurationFieldBuilder() {
if (campaignDurationBuilder_ == null) {
campaignDurationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.CampaignDuration, com.google.ads.googleads.v17.services.CampaignDuration.Builder, com.google.ads.googleads.v17.services.CampaignDurationOrBuilder>(
getCampaignDuration(),
getParentForChildren(),
isClean());
campaignDuration_ = null;
}
return campaignDurationBuilder_;
}
private int cookieFrequencyCap_ ;
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user.
* If not specified, no cap is applied.
*
* This field is deprecated in v4 and will eventually be removed.
* Use cookie_frequency_cap_setting instead.
*
*
* optional int32 cookie_frequency_cap = 10;
* @return Whether the cookieFrequencyCap field is set.
*/
@java.lang.Override
public boolean hasCookieFrequencyCap() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user.
* If not specified, no cap is applied.
*
* This field is deprecated in v4 and will eventually be removed.
* Use cookie_frequency_cap_setting instead.
*
*
* optional int32 cookie_frequency_cap = 10;
* @return The cookieFrequencyCap.
*/
@java.lang.Override
public int getCookieFrequencyCap() {
return cookieFrequencyCap_;
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user.
* If not specified, no cap is applied.
*
* This field is deprecated in v4 and will eventually be removed.
* Use cookie_frequency_cap_setting instead.
*
*
* optional int32 cookie_frequency_cap = 10;
* @param value The cookieFrequencyCap to set.
* @return This builder for chaining.
*/
public Builder setCookieFrequencyCap(int value) {
cookieFrequencyCap_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user.
* If not specified, no cap is applied.
*
* This field is deprecated in v4 and will eventually be removed.
* Use cookie_frequency_cap_setting instead.
*
*
* optional int32 cookie_frequency_cap = 10;
* @return This builder for chaining.
*/
public Builder clearCookieFrequencyCap() {
bitField0_ = (bitField0_ & ~0x00000008);
cookieFrequencyCap_ = 0;
onChanged();
return this;
}
private com.google.ads.googleads.v17.services.FrequencyCap cookieFrequencyCapSetting_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.FrequencyCap, com.google.ads.googleads.v17.services.FrequencyCap.Builder, com.google.ads.googleads.v17.services.FrequencyCapOrBuilder> cookieFrequencyCapSettingBuilder_;
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
* @return Whether the cookieFrequencyCapSetting field is set.
*/
public boolean hasCookieFrequencyCapSetting() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
* @return The cookieFrequencyCapSetting.
*/
public com.google.ads.googleads.v17.services.FrequencyCap getCookieFrequencyCapSetting() {
if (cookieFrequencyCapSettingBuilder_ == null) {
return cookieFrequencyCapSetting_ == null ? com.google.ads.googleads.v17.services.FrequencyCap.getDefaultInstance() : cookieFrequencyCapSetting_;
} else {
return cookieFrequencyCapSettingBuilder_.getMessage();
}
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
*/
public Builder setCookieFrequencyCapSetting(com.google.ads.googleads.v17.services.FrequencyCap value) {
if (cookieFrequencyCapSettingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cookieFrequencyCapSetting_ = value;
} else {
cookieFrequencyCapSettingBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
*/
public Builder setCookieFrequencyCapSetting(
com.google.ads.googleads.v17.services.FrequencyCap.Builder builderForValue) {
if (cookieFrequencyCapSettingBuilder_ == null) {
cookieFrequencyCapSetting_ = builderForValue.build();
} else {
cookieFrequencyCapSettingBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
*/
public Builder mergeCookieFrequencyCapSetting(com.google.ads.googleads.v17.services.FrequencyCap value) {
if (cookieFrequencyCapSettingBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
cookieFrequencyCapSetting_ != null &&
cookieFrequencyCapSetting_ != com.google.ads.googleads.v17.services.FrequencyCap.getDefaultInstance()) {
getCookieFrequencyCapSettingBuilder().mergeFrom(value);
} else {
cookieFrequencyCapSetting_ = value;
}
} else {
cookieFrequencyCapSettingBuilder_.mergeFrom(value);
}
if (cookieFrequencyCapSetting_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
*/
public Builder clearCookieFrequencyCapSetting() {
bitField0_ = (bitField0_ & ~0x00000010);
cookieFrequencyCapSetting_ = null;
if (cookieFrequencyCapSettingBuilder_ != null) {
cookieFrequencyCapSettingBuilder_.dispose();
cookieFrequencyCapSettingBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
*/
public com.google.ads.googleads.v17.services.FrequencyCap.Builder getCookieFrequencyCapSettingBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getCookieFrequencyCapSettingFieldBuilder().getBuilder();
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
*/
public com.google.ads.googleads.v17.services.FrequencyCapOrBuilder getCookieFrequencyCapSettingOrBuilder() {
if (cookieFrequencyCapSettingBuilder_ != null) {
return cookieFrequencyCapSettingBuilder_.getMessageOrBuilder();
} else {
return cookieFrequencyCapSetting_ == null ?
com.google.ads.googleads.v17.services.FrequencyCap.getDefaultInstance() : cookieFrequencyCapSetting_;
}
}
/**
*
* Chosen cookie frequency cap to be applied to each planned product.
* This is equivalent to the frequency cap exposed in Google Ads when creating
* a campaign, it represents the maximum number of times an ad can be shown to
* the same user during a specified time interval.
* If not specified, a default of 0 (no cap) is applied.
*
* This field replaces the deprecated cookie_frequency_cap field.
*
*
* .google.ads.googleads.v17.services.FrequencyCap cookie_frequency_cap_setting = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.FrequencyCap, com.google.ads.googleads.v17.services.FrequencyCap.Builder, com.google.ads.googleads.v17.services.FrequencyCapOrBuilder>
getCookieFrequencyCapSettingFieldBuilder() {
if (cookieFrequencyCapSettingBuilder_ == null) {
cookieFrequencyCapSettingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.FrequencyCap, com.google.ads.googleads.v17.services.FrequencyCap.Builder, com.google.ads.googleads.v17.services.FrequencyCapOrBuilder>(
getCookieFrequencyCapSetting(),
getParentForChildren(),
isClean());
cookieFrequencyCapSetting_ = null;
}
return cookieFrequencyCapSettingBuilder_;
}
private int minEffectiveFrequency_ ;
/**
*
* Chosen minimum effective frequency (the number of times a person was
* exposed to the ad) for the reported reach metrics [1-10].
* This won't affect the targeting, but just the reporting.
* If not specified, a default of 1 is applied.
*
* This field cannot be combined with the effective_frequency_limit field.
*
*
* optional int32 min_effective_frequency = 11;
* @return Whether the minEffectiveFrequency field is set.
*/
@java.lang.Override
public boolean hasMinEffectiveFrequency() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Chosen minimum effective frequency (the number of times a person was
* exposed to the ad) for the reported reach metrics [1-10].
* This won't affect the targeting, but just the reporting.
* If not specified, a default of 1 is applied.
*
* This field cannot be combined with the effective_frequency_limit field.
*
*
* optional int32 min_effective_frequency = 11;
* @return The minEffectiveFrequency.
*/
@java.lang.Override
public int getMinEffectiveFrequency() {
return minEffectiveFrequency_;
}
/**
*
* Chosen minimum effective frequency (the number of times a person was
* exposed to the ad) for the reported reach metrics [1-10].
* This won't affect the targeting, but just the reporting.
* If not specified, a default of 1 is applied.
*
* This field cannot be combined with the effective_frequency_limit field.
*
*
* optional int32 min_effective_frequency = 11;
* @param value The minEffectiveFrequency to set.
* @return This builder for chaining.
*/
public Builder setMinEffectiveFrequency(int value) {
minEffectiveFrequency_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Chosen minimum effective frequency (the number of times a person was
* exposed to the ad) for the reported reach metrics [1-10].
* This won't affect the targeting, but just the reporting.
* If not specified, a default of 1 is applied.
*
* This field cannot be combined with the effective_frequency_limit field.
*
*
* optional int32 min_effective_frequency = 11;
* @return This builder for chaining.
*/
public Builder clearMinEffectiveFrequency() {
bitField0_ = (bitField0_ & ~0x00000020);
minEffectiveFrequency_ = 0;
onChanged();
return this;
}
private com.google.ads.googleads.v17.services.EffectiveFrequencyLimit effectiveFrequencyLimit_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.EffectiveFrequencyLimit, com.google.ads.googleads.v17.services.EffectiveFrequencyLimit.Builder, com.google.ads.googleads.v17.services.EffectiveFrequencyLimitOrBuilder> effectiveFrequencyLimitBuilder_;
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
* @return Whether the effectiveFrequencyLimit field is set.
*/
public boolean hasEffectiveFrequencyLimit() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
* @return The effectiveFrequencyLimit.
*/
public com.google.ads.googleads.v17.services.EffectiveFrequencyLimit getEffectiveFrequencyLimit() {
if (effectiveFrequencyLimitBuilder_ == null) {
return effectiveFrequencyLimit_ == null ? com.google.ads.googleads.v17.services.EffectiveFrequencyLimit.getDefaultInstance() : effectiveFrequencyLimit_;
} else {
return effectiveFrequencyLimitBuilder_.getMessage();
}
}
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
*/
public Builder setEffectiveFrequencyLimit(com.google.ads.googleads.v17.services.EffectiveFrequencyLimit value) {
if (effectiveFrequencyLimitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
effectiveFrequencyLimit_ = value;
} else {
effectiveFrequencyLimitBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
*/
public Builder setEffectiveFrequencyLimit(
com.google.ads.googleads.v17.services.EffectiveFrequencyLimit.Builder builderForValue) {
if (effectiveFrequencyLimitBuilder_ == null) {
effectiveFrequencyLimit_ = builderForValue.build();
} else {
effectiveFrequencyLimitBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
*/
public Builder mergeEffectiveFrequencyLimit(com.google.ads.googleads.v17.services.EffectiveFrequencyLimit value) {
if (effectiveFrequencyLimitBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0) &&
effectiveFrequencyLimit_ != null &&
effectiveFrequencyLimit_ != com.google.ads.googleads.v17.services.EffectiveFrequencyLimit.getDefaultInstance()) {
getEffectiveFrequencyLimitBuilder().mergeFrom(value);
} else {
effectiveFrequencyLimit_ = value;
}
} else {
effectiveFrequencyLimitBuilder_.mergeFrom(value);
}
if (effectiveFrequencyLimit_ != null) {
bitField0_ |= 0x00000040;
onChanged();
}
return this;
}
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
*/
public Builder clearEffectiveFrequencyLimit() {
bitField0_ = (bitField0_ & ~0x00000040);
effectiveFrequencyLimit_ = null;
if (effectiveFrequencyLimitBuilder_ != null) {
effectiveFrequencyLimitBuilder_.dispose();
effectiveFrequencyLimitBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
*/
public com.google.ads.googleads.v17.services.EffectiveFrequencyLimit.Builder getEffectiveFrequencyLimitBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getEffectiveFrequencyLimitFieldBuilder().getBuilder();
}
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
*/
public com.google.ads.googleads.v17.services.EffectiveFrequencyLimitOrBuilder getEffectiveFrequencyLimitOrBuilder() {
if (effectiveFrequencyLimitBuilder_ != null) {
return effectiveFrequencyLimitBuilder_.getMessageOrBuilder();
} else {
return effectiveFrequencyLimit_ == null ?
com.google.ads.googleads.v17.services.EffectiveFrequencyLimit.getDefaultInstance() : effectiveFrequencyLimit_;
}
}
/**
*
* The highest minimum effective frequency (the number of times a person was
* exposed to the ad) value [1-10] to include in
* Forecast.effective_frequency_breakdowns.
* If not specified, Forecast.effective_frequency_breakdowns will not be
* provided.
*
* The effective frequency value provided here will also be used as the
* minimum effective frequency for the reported reach metrics.
*
* This field cannot be combined with the min_effective_frequency field.
*
*
* optional .google.ads.googleads.v17.services.EffectiveFrequencyLimit effective_frequency_limit = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.EffectiveFrequencyLimit, com.google.ads.googleads.v17.services.EffectiveFrequencyLimit.Builder, com.google.ads.googleads.v17.services.EffectiveFrequencyLimitOrBuilder>
getEffectiveFrequencyLimitFieldBuilder() {
if (effectiveFrequencyLimitBuilder_ == null) {
effectiveFrequencyLimitBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.EffectiveFrequencyLimit, com.google.ads.googleads.v17.services.EffectiveFrequencyLimit.Builder, com.google.ads.googleads.v17.services.EffectiveFrequencyLimitOrBuilder>(
getEffectiveFrequencyLimit(),
getParentForChildren(),
isClean());
effectiveFrequencyLimit_ = null;
}
return effectiveFrequencyLimitBuilder_;
}
private com.google.ads.googleads.v17.services.Targeting targeting_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.Targeting, com.google.ads.googleads.v17.services.Targeting.Builder, com.google.ads.googleads.v17.services.TargetingOrBuilder> targetingBuilder_;
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
* @return Whether the targeting field is set.
*/
public boolean hasTargeting() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
* @return The targeting.
*/
public com.google.ads.googleads.v17.services.Targeting getTargeting() {
if (targetingBuilder_ == null) {
return targeting_ == null ? com.google.ads.googleads.v17.services.Targeting.getDefaultInstance() : targeting_;
} else {
return targetingBuilder_.getMessage();
}
}
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
*/
public Builder setTargeting(com.google.ads.googleads.v17.services.Targeting value) {
if (targetingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
targeting_ = value;
} else {
targetingBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
*/
public Builder setTargeting(
com.google.ads.googleads.v17.services.Targeting.Builder builderForValue) {
if (targetingBuilder_ == null) {
targeting_ = builderForValue.build();
} else {
targetingBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
*/
public Builder mergeTargeting(com.google.ads.googleads.v17.services.Targeting value) {
if (targetingBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0) &&
targeting_ != null &&
targeting_ != com.google.ads.googleads.v17.services.Targeting.getDefaultInstance()) {
getTargetingBuilder().mergeFrom(value);
} else {
targeting_ = value;
}
} else {
targetingBuilder_.mergeFrom(value);
}
if (targeting_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
*/
public Builder clearTargeting() {
bitField0_ = (bitField0_ & ~0x00000080);
targeting_ = null;
if (targetingBuilder_ != null) {
targetingBuilder_.dispose();
targetingBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
*/
public com.google.ads.googleads.v17.services.Targeting.Builder getTargetingBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getTargetingFieldBuilder().getBuilder();
}
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
*/
public com.google.ads.googleads.v17.services.TargetingOrBuilder getTargetingOrBuilder() {
if (targetingBuilder_ != null) {
return targetingBuilder_.getMessageOrBuilder();
} else {
return targeting_ == null ?
com.google.ads.googleads.v17.services.Targeting.getDefaultInstance() : targeting_;
}
}
/**
*
* The targeting to be applied to all products selected in the product mix.
*
* This is planned targeting: execution details might vary based on the
* advertising product, consult an implementation specialist.
*
* See specific metrics for details on how targeting affects them.
*
*
* .google.ads.googleads.v17.services.Targeting targeting = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.Targeting, com.google.ads.googleads.v17.services.Targeting.Builder, com.google.ads.googleads.v17.services.TargetingOrBuilder>
getTargetingFieldBuilder() {
if (targetingBuilder_ == null) {
targetingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.Targeting, com.google.ads.googleads.v17.services.Targeting.Builder, com.google.ads.googleads.v17.services.TargetingOrBuilder>(
getTargeting(),
getParentForChildren(),
isClean());
targeting_ = null;
}
return targetingBuilder_;
}
private java.util.List plannedProducts_ =
java.util.Collections.emptyList();
private void ensurePlannedProductsIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
plannedProducts_ = new java.util.ArrayList(plannedProducts_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.services.PlannedProduct, com.google.ads.googleads.v17.services.PlannedProduct.Builder, com.google.ads.googleads.v17.services.PlannedProductOrBuilder> plannedProductsBuilder_;
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public java.util.List getPlannedProductsList() {
if (plannedProductsBuilder_ == null) {
return java.util.Collections.unmodifiableList(plannedProducts_);
} else {
return plannedProductsBuilder_.getMessageList();
}
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public int getPlannedProductsCount() {
if (plannedProductsBuilder_ == null) {
return plannedProducts_.size();
} else {
return plannedProductsBuilder_.getCount();
}
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public com.google.ads.googleads.v17.services.PlannedProduct getPlannedProducts(int index) {
if (plannedProductsBuilder_ == null) {
return plannedProducts_.get(index);
} else {
return plannedProductsBuilder_.getMessage(index);
}
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder setPlannedProducts(
int index, com.google.ads.googleads.v17.services.PlannedProduct value) {
if (plannedProductsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePlannedProductsIsMutable();
plannedProducts_.set(index, value);
onChanged();
} else {
plannedProductsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder setPlannedProducts(
int index, com.google.ads.googleads.v17.services.PlannedProduct.Builder builderForValue) {
if (plannedProductsBuilder_ == null) {
ensurePlannedProductsIsMutable();
plannedProducts_.set(index, builderForValue.build());
onChanged();
} else {
plannedProductsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder addPlannedProducts(com.google.ads.googleads.v17.services.PlannedProduct value) {
if (plannedProductsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePlannedProductsIsMutable();
plannedProducts_.add(value);
onChanged();
} else {
plannedProductsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder addPlannedProducts(
int index, com.google.ads.googleads.v17.services.PlannedProduct value) {
if (plannedProductsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePlannedProductsIsMutable();
plannedProducts_.add(index, value);
onChanged();
} else {
plannedProductsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder addPlannedProducts(
com.google.ads.googleads.v17.services.PlannedProduct.Builder builderForValue) {
if (plannedProductsBuilder_ == null) {
ensurePlannedProductsIsMutable();
plannedProducts_.add(builderForValue.build());
onChanged();
} else {
plannedProductsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder addPlannedProducts(
int index, com.google.ads.googleads.v17.services.PlannedProduct.Builder builderForValue) {
if (plannedProductsBuilder_ == null) {
ensurePlannedProductsIsMutable();
plannedProducts_.add(index, builderForValue.build());
onChanged();
} else {
plannedProductsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder addAllPlannedProducts(
java.lang.Iterable extends com.google.ads.googleads.v17.services.PlannedProduct> values) {
if (plannedProductsBuilder_ == null) {
ensurePlannedProductsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, plannedProducts_);
onChanged();
} else {
plannedProductsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder clearPlannedProducts() {
if (plannedProductsBuilder_ == null) {
plannedProducts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
plannedProductsBuilder_.clear();
}
return this;
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public Builder removePlannedProducts(int index) {
if (plannedProductsBuilder_ == null) {
ensurePlannedProductsIsMutable();
plannedProducts_.remove(index);
onChanged();
} else {
plannedProductsBuilder_.remove(index);
}
return this;
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public com.google.ads.googleads.v17.services.PlannedProduct.Builder getPlannedProductsBuilder(
int index) {
return getPlannedProductsFieldBuilder().getBuilder(index);
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public com.google.ads.googleads.v17.services.PlannedProductOrBuilder getPlannedProductsOrBuilder(
int index) {
if (plannedProductsBuilder_ == null) {
return plannedProducts_.get(index); } else {
return plannedProductsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public java.util.List extends com.google.ads.googleads.v17.services.PlannedProductOrBuilder>
getPlannedProductsOrBuilderList() {
if (plannedProductsBuilder_ != null) {
return plannedProductsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(plannedProducts_);
}
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public com.google.ads.googleads.v17.services.PlannedProduct.Builder addPlannedProductsBuilder() {
return getPlannedProductsFieldBuilder().addBuilder(
com.google.ads.googleads.v17.services.PlannedProduct.getDefaultInstance());
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public com.google.ads.googleads.v17.services.PlannedProduct.Builder addPlannedProductsBuilder(
int index) {
return getPlannedProductsFieldBuilder().addBuilder(
index, com.google.ads.googleads.v17.services.PlannedProduct.getDefaultInstance());
}
/**
*
* Required. The products to be forecast.
* The max number of allowed planned products is 15.
*
*
* repeated .google.ads.googleads.v17.services.PlannedProduct planned_products = 7 [(.google.api.field_behavior) = REQUIRED];
*/
public java.util.List
getPlannedProductsBuilderList() {
return getPlannedProductsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.services.PlannedProduct, com.google.ads.googleads.v17.services.PlannedProduct.Builder, com.google.ads.googleads.v17.services.PlannedProductOrBuilder>
getPlannedProductsFieldBuilder() {
if (plannedProductsBuilder_ == null) {
plannedProductsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ads.googleads.v17.services.PlannedProduct, com.google.ads.googleads.v17.services.PlannedProduct.Builder, com.google.ads.googleads.v17.services.PlannedProductOrBuilder>(
plannedProducts_,
((bitField0_ & 0x00000100) != 0),
getParentForChildren(),
isClean());
plannedProducts_ = null;
}
return plannedProductsBuilder_;
}
private com.google.ads.googleads.v17.services.ForecastMetricOptions forecastMetricOptions_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.ForecastMetricOptions, com.google.ads.googleads.v17.services.ForecastMetricOptions.Builder, com.google.ads.googleads.v17.services.ForecastMetricOptionsOrBuilder> forecastMetricOptionsBuilder_;
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
* @return Whether the forecastMetricOptions field is set.
*/
public boolean hasForecastMetricOptions() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
* @return The forecastMetricOptions.
*/
public com.google.ads.googleads.v17.services.ForecastMetricOptions getForecastMetricOptions() {
if (forecastMetricOptionsBuilder_ == null) {
return forecastMetricOptions_ == null ? com.google.ads.googleads.v17.services.ForecastMetricOptions.getDefaultInstance() : forecastMetricOptions_;
} else {
return forecastMetricOptionsBuilder_.getMessage();
}
}
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
*/
public Builder setForecastMetricOptions(com.google.ads.googleads.v17.services.ForecastMetricOptions value) {
if (forecastMetricOptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
forecastMetricOptions_ = value;
} else {
forecastMetricOptionsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
*/
public Builder setForecastMetricOptions(
com.google.ads.googleads.v17.services.ForecastMetricOptions.Builder builderForValue) {
if (forecastMetricOptionsBuilder_ == null) {
forecastMetricOptions_ = builderForValue.build();
} else {
forecastMetricOptionsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
*/
public Builder mergeForecastMetricOptions(com.google.ads.googleads.v17.services.ForecastMetricOptions value) {
if (forecastMetricOptionsBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0) &&
forecastMetricOptions_ != null &&
forecastMetricOptions_ != com.google.ads.googleads.v17.services.ForecastMetricOptions.getDefaultInstance()) {
getForecastMetricOptionsBuilder().mergeFrom(value);
} else {
forecastMetricOptions_ = value;
}
} else {
forecastMetricOptionsBuilder_.mergeFrom(value);
}
if (forecastMetricOptions_ != null) {
bitField0_ |= 0x00000200;
onChanged();
}
return this;
}
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
*/
public Builder clearForecastMetricOptions() {
bitField0_ = (bitField0_ & ~0x00000200);
forecastMetricOptions_ = null;
if (forecastMetricOptionsBuilder_ != null) {
forecastMetricOptionsBuilder_.dispose();
forecastMetricOptionsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
*/
public com.google.ads.googleads.v17.services.ForecastMetricOptions.Builder getForecastMetricOptionsBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getForecastMetricOptionsFieldBuilder().getBuilder();
}
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
*/
public com.google.ads.googleads.v17.services.ForecastMetricOptionsOrBuilder getForecastMetricOptionsOrBuilder() {
if (forecastMetricOptionsBuilder_ != null) {
return forecastMetricOptionsBuilder_.getMessageOrBuilder();
} else {
return forecastMetricOptions_ == null ?
com.google.ads.googleads.v17.services.ForecastMetricOptions.getDefaultInstance() : forecastMetricOptions_;
}
}
/**
*
* Controls the forecast metrics returned in the response.
*
*
* .google.ads.googleads.v17.services.ForecastMetricOptions forecast_metric_options = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.ForecastMetricOptions, com.google.ads.googleads.v17.services.ForecastMetricOptions.Builder, com.google.ads.googleads.v17.services.ForecastMetricOptionsOrBuilder>
getForecastMetricOptionsFieldBuilder() {
if (forecastMetricOptionsBuilder_ == null) {
forecastMetricOptionsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ads.googleads.v17.services.ForecastMetricOptions, com.google.ads.googleads.v17.services.ForecastMetricOptions.Builder, com.google.ads.googleads.v17.services.ForecastMetricOptionsOrBuilder>(
getForecastMetricOptions(),
getParentForChildren(),
isClean());
forecastMetricOptions_ = null;
}
return forecastMetricOptionsBuilder_;
}
private java.lang.Object customerReachGroup_ = "";
/**
*
* The name of the customer being planned for. This is a user-defined value.
*
*
* optional string customer_reach_group = 14;
* @return Whether the customerReachGroup field is set.
*/
public boolean hasCustomerReachGroup() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* The name of the customer being planned for. This is a user-defined value.
*
*
* optional string customer_reach_group = 14;
* @return The customerReachGroup.
*/
public java.lang.String getCustomerReachGroup() {
java.lang.Object ref = customerReachGroup_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerReachGroup_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the customer being planned for. This is a user-defined value.
*
*
* optional string customer_reach_group = 14;
* @return The bytes for customerReachGroup.
*/
public com.google.protobuf.ByteString
getCustomerReachGroupBytes() {
java.lang.Object ref = customerReachGroup_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerReachGroup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the customer being planned for. This is a user-defined value.
*
*
* optional string customer_reach_group = 14;
* @param value The customerReachGroup to set.
* @return This builder for chaining.
*/
public Builder setCustomerReachGroup(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
customerReachGroup_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* The name of the customer being planned for. This is a user-defined value.
*
*
* optional string customer_reach_group = 14;
* @return This builder for chaining.
*/
public Builder clearCustomerReachGroup() {
customerReachGroup_ = getDefaultInstance().getCustomerReachGroup();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
return this;
}
/**
*
* The name of the customer being planned for. This is a user-defined value.
*
*
* optional string customer_reach_group = 14;
* @param value The bytes for customerReachGroup to set.
* @return This builder for chaining.
*/
public Builder setCustomerReachGroupBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
customerReachGroup_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.ads.googleads.v17.services.GenerateReachForecastRequest)
}
// @@protoc_insertion_point(class_scope:google.ads.googleads.v17.services.GenerateReachForecastRequest)
private static final com.google.ads.googleads.v17.services.GenerateReachForecastRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ads.googleads.v17.services.GenerateReachForecastRequest();
}
public static com.google.ads.googleads.v17.services.GenerateReachForecastRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GenerateReachForecastRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ads.googleads.v17.services.GenerateReachForecastRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy