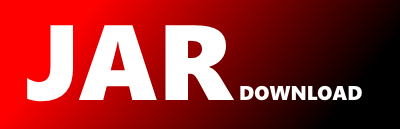
com.google.ads.googleads.v17.services.package-info Maven / Gradle / Ivy
Show all versions of google-ads-stubs-v17 Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* A client to Google Ads API
*
* The interfaces provided are listed below, along with usage samples.
*
*
======================= AccountBudgetProposalServiceClient =======================
*
*
Service Description: A service for managing account-level budgets through proposals.
*
*
A proposal is a request to create a new budget or make changes to an existing one.
*
*
Mutates: The CREATE operation creates a new proposal. UPDATE operations aren't supported. The
* REMOVE operation cancels a pending proposal.
*
*
Sample for AccountBudgetProposalServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AccountBudgetProposalServiceClient accountBudgetProposalServiceClient =
* AccountBudgetProposalServiceClient.create()) {
* String customerId = "customerId-1581184615";
* AccountBudgetProposalOperation operation =
* AccountBudgetProposalOperation.newBuilder().build();
* MutateAccountBudgetProposalResponse response =
* accountBudgetProposalServiceClient.mutateAccountBudgetProposal(customerId, operation);
* }
* }
*
* ======================= AccountLinkServiceClient =======================
*
*
Service Description: This service allows management of links between Google Ads accounts and
* other accounts.
*
*
Sample for AccountLinkServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AccountLinkServiceClient accountLinkServiceClient = AccountLinkServiceClient.create()) {
* String customerId = "customerId-1581184615";
* AccountLink accountLink = AccountLink.newBuilder().build();
* CreateAccountLinkResponse response =
* accountLinkServiceClient.createAccountLink(customerId, accountLink);
* }
* }
*
* ======================= AdGroupAdLabelServiceClient =======================
*
*
Service Description: Service to manage labels on ad group ads.
*
*
Sample for AdGroupAdLabelServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupAdLabelServiceClient adGroupAdLabelServiceClient =
* AdGroupAdLabelServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupAdLabelsResponse response =
* adGroupAdLabelServiceClient.mutateAdGroupAdLabels(customerId, operations);
* }
* }
*
* ======================= AdGroupAdServiceClient =======================
*
*
Service Description: Service to manage ads in an ad group.
*
*
Sample for AdGroupAdServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupAdServiceClient adGroupAdServiceClient = AdGroupAdServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupAdsResponse response =
* adGroupAdServiceClient.mutateAdGroupAds(customerId, operations);
* }
* }
*
* ======================= AdGroupAssetServiceClient =======================
*
*
Service Description: Service to manage ad group assets.
*
*
Sample for AdGroupAssetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupAssetServiceClient adGroupAssetServiceClient = AdGroupAssetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupAssetsResponse response =
* adGroupAssetServiceClient.mutateAdGroupAssets(customerId, operations);
* }
* }
*
* ======================= AdGroupAssetSetServiceClient =======================
*
*
Service Description: Service to manage ad group asset set
*
*
Sample for AdGroupAssetSetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupAssetSetServiceClient adGroupAssetSetServiceClient =
* AdGroupAssetSetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupAssetSetsResponse response =
* adGroupAssetSetServiceClient.mutateAdGroupAssetSets(customerId, operations);
* }
* }
*
* ======================= AdGroupBidModifierServiceClient =======================
*
*
Service Description: Service to manage ad group bid modifiers.
*
*
Sample for AdGroupBidModifierServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupBidModifierServiceClient adGroupBidModifierServiceClient =
* AdGroupBidModifierServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupBidModifiersResponse response =
* adGroupBidModifierServiceClient.mutateAdGroupBidModifiers(customerId, operations);
* }
* }
*
* ======================= AdGroupCriterionCustomizerServiceClient =======================
*
*
Service Description: Service to manage ad group criterion customizer
*
*
Sample for AdGroupCriterionCustomizerServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupCriterionCustomizerServiceClient adGroupCriterionCustomizerServiceClient =
* AdGroupCriterionCustomizerServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupCriterionCustomizersResponse response =
* adGroupCriterionCustomizerServiceClient.mutateAdGroupCriterionCustomizers(
* customerId, operations);
* }
* }
*
* ======================= AdGroupCriterionLabelServiceClient =======================
*
*
Service Description: Service to manage labels on ad group criteria.
*
*
Sample for AdGroupCriterionLabelServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupCriterionLabelServiceClient adGroupCriterionLabelServiceClient =
* AdGroupCriterionLabelServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupCriterionLabelsResponse response =
* adGroupCriterionLabelServiceClient.mutateAdGroupCriterionLabels(customerId, operations);
* }
* }
*
* ======================= AdGroupCriterionServiceClient =======================
*
*
Service Description: Service to manage ad group criteria.
*
*
Sample for AdGroupCriterionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupCriterionServiceClient adGroupCriterionServiceClient =
* AdGroupCriterionServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupCriteriaResponse response =
* adGroupCriterionServiceClient.mutateAdGroupCriteria(customerId, operations);
* }
* }
*
* ======================= AdGroupCustomizerServiceClient =======================
*
*
Service Description: Service to manage ad group customizer
*
*
Sample for AdGroupCustomizerServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupCustomizerServiceClient adGroupCustomizerServiceClient =
* AdGroupCustomizerServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupCustomizersResponse response =
* adGroupCustomizerServiceClient.mutateAdGroupCustomizers(customerId, operations);
* }
* }
*
* ======================= AdGroupExtensionSettingServiceClient =======================
*
*
Service Description: Service to manage ad group extension settings.
*
*
Sample for AdGroupExtensionSettingServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupExtensionSettingServiceClient adGroupExtensionSettingServiceClient =
* AdGroupExtensionSettingServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupExtensionSettingsResponse response =
* adGroupExtensionSettingServiceClient.mutateAdGroupExtensionSettings(
* customerId, operations);
* }
* }
*
* ======================= AdGroupFeedServiceClient =======================
*
*
Service Description: Service to manage ad group feeds.
*
*
Sample for AdGroupFeedServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupFeedServiceClient adGroupFeedServiceClient = AdGroupFeedServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupFeedsResponse response =
* adGroupFeedServiceClient.mutateAdGroupFeeds(customerId, operations);
* }
* }
*
* ======================= AdGroupLabelServiceClient =======================
*
*
Service Description: Service to manage labels on ad groups.
*
*
Sample for AdGroupLabelServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupLabelServiceClient adGroupLabelServiceClient = AdGroupLabelServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupLabelsResponse response =
* adGroupLabelServiceClient.mutateAdGroupLabels(customerId, operations);
* }
* }
*
* ======================= AdGroupServiceClient =======================
*
*
Service Description: Service to manage ad groups.
*
*
Sample for AdGroupServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdGroupServiceClient adGroupServiceClient = AdGroupServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdGroupsResponse response = adGroupServiceClient.mutateAdGroups(customerId, operations);
* }
* }
*
* ======================= AdParameterServiceClient =======================
*
*
Service Description: Service to manage ad parameters.
*
*
Sample for AdParameterServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdParameterServiceClient adParameterServiceClient = AdParameterServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdParametersResponse response =
* adParameterServiceClient.mutateAdParameters(customerId, operations);
* }
* }
*
* ======================= AdServiceClient =======================
*
*
Service Description: Service to manage ads.
*
*
Sample for AdServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AdServiceClient adServiceClient = AdServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAdsResponse response = adServiceClient.mutateAds(customerId, operations);
* }
* }
*
* ======================= AssetGroupAssetServiceClient =======================
*
*
Service Description: Service to manage asset group asset.
*
*
Sample for AssetGroupAssetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AssetGroupAssetServiceClient assetGroupAssetServiceClient =
* AssetGroupAssetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAssetGroupAssetsResponse response =
* assetGroupAssetServiceClient.mutateAssetGroupAssets(customerId, operations);
* }
* }
*
* ======================= AssetGroupListingGroupFilterServiceClient =======================
*
*
Service Description: Service to manage asset group listing group filter.
*
*
Sample for AssetGroupListingGroupFilterServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AssetGroupListingGroupFilterServiceClient assetGroupListingGroupFilterServiceClient =
* AssetGroupListingGroupFilterServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAssetGroupListingGroupFiltersResponse response =
* assetGroupListingGroupFilterServiceClient.mutateAssetGroupListingGroupFilters(
* customerId, operations);
* }
* }
*
* ======================= AssetGroupServiceClient =======================
*
*
Service Description: Service to manage asset group
*
*
Sample for AssetGroupServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AssetGroupServiceClient assetGroupServiceClient = AssetGroupServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAssetGroupsResponse response =
* assetGroupServiceClient.mutateAssetGroups(customerId, operations);
* }
* }
*
* ======================= AssetGroupSignalServiceClient =======================
*
*
Service Description: Service to manage asset group signal.
*
*
Sample for AssetGroupSignalServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AssetGroupSignalServiceClient assetGroupSignalServiceClient =
* AssetGroupSignalServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAssetGroupSignalsResponse response =
* assetGroupSignalServiceClient.mutateAssetGroupSignals(customerId, operations);
* }
* }
*
* ======================= AssetServiceClient =======================
*
*
Service Description: Service to manage assets. Asset types can be created with AssetService
* are YoutubeVideoAsset, MediaBundleAsset and ImageAsset. TextAsset should be created with Ad
* inline.
*
*
Sample for AssetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AssetServiceClient assetServiceClient = AssetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAssetsResponse response = assetServiceClient.mutateAssets(customerId, operations);
* }
* }
*
* ======================= AssetSetAssetServiceClient =======================
*
*
Service Description: Service to manage asset set asset.
*
*
Sample for AssetSetAssetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AssetSetAssetServiceClient assetSetAssetServiceClient =
* AssetSetAssetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAssetSetAssetsResponse response =
* assetSetAssetServiceClient.mutateAssetSetAssets(customerId, operations);
* }
* }
*
* ======================= AssetSetServiceClient =======================
*
*
Service Description: Service to manage asset set
*
*
Sample for AssetSetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AssetSetServiceClient assetSetServiceClient = AssetSetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAssetSetsResponse response =
* assetSetServiceClient.mutateAssetSets(customerId, operations);
* }
* }
*
* ======================= AudienceInsightsServiceClient =======================
*
*
Service Description: Audience Insights Service helps users find information about groups of
* people and how they can be reached with Google Ads. Accessible to allowlisted customers only.
*
*
Sample for AudienceInsightsServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceInsightsServiceClient audienceInsightsServiceClient =
* AudienceInsightsServiceClient.create()) {
* String customerId = "customerId-1581184615";
* BasicInsightsAudience baselineAudience = BasicInsightsAudience.newBuilder().build();
* BasicInsightsAudience specificAudience = BasicInsightsAudience.newBuilder().build();
* GenerateInsightsFinderReportResponse response =
* audienceInsightsServiceClient.generateInsightsFinderReport(
* customerId, baselineAudience, specificAudience);
* }
* }
*
* ======================= AudienceServiceClient =======================
*
*
Service Description: Service to manage audiences.
*
*
Sample for AudienceServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AudienceServiceClient audienceServiceClient = AudienceServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateAudiencesResponse response =
* audienceServiceClient.mutateAudiences(customerId, operations);
* }
* }
*
* ======================= BatchJobServiceClient =======================
*
*
Service Description: Service to manage batch jobs.
*
*
Sample for BatchJobServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BatchJobServiceClient batchJobServiceClient = BatchJobServiceClient.create()) {
* String customerId = "customerId-1581184615";
* BatchJobOperation operation = BatchJobOperation.newBuilder().build();
* MutateBatchJobResponse response = batchJobServiceClient.mutateBatchJob(customerId, operation);
* }
* }
*
* ======================= BiddingDataExclusionServiceClient =======================
*
*
Service Description: Service to manage bidding data exclusions.
*
*
Sample for BiddingDataExclusionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BiddingDataExclusionServiceClient biddingDataExclusionServiceClient =
* BiddingDataExclusionServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateBiddingDataExclusionsResponse response =
* biddingDataExclusionServiceClient.mutateBiddingDataExclusions(customerId, operations);
* }
* }
*
* ======================= BiddingSeasonalityAdjustmentServiceClient =======================
*
*
Service Description: Service to manage bidding seasonality adjustments.
*
*
Sample for BiddingSeasonalityAdjustmentServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BiddingSeasonalityAdjustmentServiceClient biddingSeasonalityAdjustmentServiceClient =
* BiddingSeasonalityAdjustmentServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateBiddingSeasonalityAdjustmentsResponse response =
* biddingSeasonalityAdjustmentServiceClient.mutateBiddingSeasonalityAdjustments(
* customerId, operations);
* }
* }
*
* ======================= BiddingStrategyServiceClient =======================
*
*
Service Description: Service to manage bidding strategies.
*
*
Sample for BiddingStrategyServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BiddingStrategyServiceClient biddingStrategyServiceClient =
* BiddingStrategyServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateBiddingStrategiesResponse response =
* biddingStrategyServiceClient.mutateBiddingStrategies(customerId, operations);
* }
* }
*
* ======================= BillingSetupServiceClient =======================
*
*
Service Description: A service for designating the business entity responsible for accrued
* costs.
*
*
A billing setup is associated with a payments account. Billing-related activity for all
* billing setups associated with a particular payments account will appear on a single invoice
* generated monthly.
*
*
Mutates: The REMOVE operation cancels a pending billing setup. The CREATE operation creates a
* new billing setup.
*
*
Sample for BillingSetupServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BillingSetupServiceClient billingSetupServiceClient = BillingSetupServiceClient.create()) {
* String customerId = "customerId-1581184615";
* BillingSetupOperation operation = BillingSetupOperation.newBuilder().build();
* MutateBillingSetupResponse response =
* billingSetupServiceClient.mutateBillingSetup(customerId, operation);
* }
* }
*
* ======================= BrandSuggestionServiceClient =======================
*
*
Service Description: This service will suggest brands based on a prefix.
*
*
Sample for BrandSuggestionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BrandSuggestionServiceClient brandSuggestionServiceClient =
* BrandSuggestionServiceClient.create()) {
* String customerId = "customerId-1581184615";
* String brandPrefix = "brandPrefix-774313735";
* SuggestBrandsResponse response =
* brandSuggestionServiceClient.suggestBrands(customerId, brandPrefix);
* }
* }
*
* ======================= CampaignAssetServiceClient =======================
*
*
Service Description: Service to manage campaign assets.
*
*
Sample for CampaignAssetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignAssetServiceClient campaignAssetServiceClient =
* CampaignAssetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignAssetsResponse response =
* campaignAssetServiceClient.mutateCampaignAssets(customerId, operations);
* }
* }
*
* ======================= CampaignAssetSetServiceClient =======================
*
*
Service Description: Service to manage campaign asset set
*
*
Sample for CampaignAssetSetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignAssetSetServiceClient campaignAssetSetServiceClient =
* CampaignAssetSetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignAssetSetsResponse response =
* campaignAssetSetServiceClient.mutateCampaignAssetSets(customerId, operations);
* }
* }
*
* ======================= CampaignBidModifierServiceClient =======================
*
*
Service Description: Service to manage campaign bid modifiers.
*
*
Sample for CampaignBidModifierServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignBidModifierServiceClient campaignBidModifierServiceClient =
* CampaignBidModifierServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignBidModifiersResponse response =
* campaignBidModifierServiceClient.mutateCampaignBidModifiers(customerId, operations);
* }
* }
*
* ======================= CampaignBudgetServiceClient =======================
*
*
Service Description: Service to manage campaign budgets.
*
*
Sample for CampaignBudgetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignBudgetServiceClient campaignBudgetServiceClient =
* CampaignBudgetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignBudgetsResponse response =
* campaignBudgetServiceClient.mutateCampaignBudgets(customerId, operations);
* }
* }
*
* ======================= CampaignConversionGoalServiceClient =======================
*
*
Service Description: Service to manage campaign conversion goal.
*
*
Sample for CampaignConversionGoalServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignConversionGoalServiceClient campaignConversionGoalServiceClient =
* CampaignConversionGoalServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignConversionGoalsResponse response =
* campaignConversionGoalServiceClient.mutateCampaignConversionGoals(customerId, operations);
* }
* }
*
* ======================= CampaignCriterionServiceClient =======================
*
*
Service Description: Service to manage campaign criteria.
*
*
Sample for CampaignCriterionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignCriterionServiceClient campaignCriterionServiceClient =
* CampaignCriterionServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignCriteriaResponse response =
* campaignCriterionServiceClient.mutateCampaignCriteria(customerId, operations);
* }
* }
*
* ======================= CampaignCustomizerServiceClient =======================
*
*
Service Description: Service to manage campaign customizer
*
*
Sample for CampaignCustomizerServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignCustomizerServiceClient campaignCustomizerServiceClient =
* CampaignCustomizerServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignCustomizersResponse response =
* campaignCustomizerServiceClient.mutateCampaignCustomizers(customerId, operations);
* }
* }
*
* ======================= CampaignDraftServiceClient =======================
*
*
Service Description: Service to manage campaign drafts.
*
*
Sample for CampaignDraftServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignDraftServiceClient campaignDraftServiceClient =
* CampaignDraftServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignDraftsResponse response =
* campaignDraftServiceClient.mutateCampaignDrafts(customerId, operations);
* }
* }
*
* ======================= CampaignExtensionSettingServiceClient =======================
*
*
Service Description: Service to manage campaign extension settings.
*
*
Sample for CampaignExtensionSettingServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignExtensionSettingServiceClient campaignExtensionSettingServiceClient =
* CampaignExtensionSettingServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignExtensionSettingsResponse response =
* campaignExtensionSettingServiceClient.mutateCampaignExtensionSettings(
* customerId, operations);
* }
* }
*
* ======================= CampaignFeedServiceClient =======================
*
*
Service Description: Service to manage campaign feeds.
*
*
Sample for CampaignFeedServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignFeedServiceClient campaignFeedServiceClient = CampaignFeedServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignFeedsResponse response =
* campaignFeedServiceClient.mutateCampaignFeeds(customerId, operations);
* }
* }
*
* ======================= CampaignGroupServiceClient =======================
*
*
Service Description: Service to manage campaign groups.
*
*
Sample for CampaignGroupServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignGroupServiceClient campaignGroupServiceClient =
* CampaignGroupServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignGroupsResponse response =
* campaignGroupServiceClient.mutateCampaignGroups(customerId, operations);
* }
* }
*
* ======================= CampaignLabelServiceClient =======================
*
*
Service Description: Service to manage labels on campaigns.
*
*
Sample for CampaignLabelServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignLabelServiceClient campaignLabelServiceClient =
* CampaignLabelServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignLabelsResponse response =
* campaignLabelServiceClient.mutateCampaignLabels(customerId, operations);
* }
* }
*
* ======================= CampaignLifecycleGoalServiceClient =======================
*
*
Service Description: Service to configure campaign lifecycle goals.
*
*
Sample for CampaignLifecycleGoalServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignLifecycleGoalServiceClient campaignLifecycleGoalServiceClient =
* CampaignLifecycleGoalServiceClient.create()) {
* String customerId = "customerId-1581184615";
* CampaignLifecycleGoalOperation operation =
* CampaignLifecycleGoalOperation.newBuilder().build();
* ConfigureCampaignLifecycleGoalsResponse response =
* campaignLifecycleGoalServiceClient.configureCampaignLifecycleGoals(customerId, operation);
* }
* }
*
* ======================= CampaignServiceClient =======================
*
*
Service Description: Service to manage campaigns.
*
*
Sample for CampaignServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignServiceClient campaignServiceClient = CampaignServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignsResponse response =
* campaignServiceClient.mutateCampaigns(customerId, operations);
* }
* }
*
* ======================= CampaignSharedSetServiceClient =======================
*
*
Service Description: Service to manage campaign shared sets.
*
*
Sample for CampaignSharedSetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CampaignSharedSetServiceClient campaignSharedSetServiceClient =
* CampaignSharedSetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCampaignSharedSetsResponse response =
* campaignSharedSetServiceClient.mutateCampaignSharedSets(customerId, operations);
* }
* }
*
* ======================= ConversionActionServiceClient =======================
*
*
Service Description: Service to manage conversion actions.
*
*
Sample for ConversionActionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversionActionServiceClient conversionActionServiceClient =
* ConversionActionServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateConversionActionsResponse response =
* conversionActionServiceClient.mutateConversionActions(customerId, operations);
* }
* }
*
* ======================= ConversionAdjustmentUploadServiceClient =======================
*
*
Service Description: Service to upload conversion adjustments.
*
*
Sample for ConversionAdjustmentUploadServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversionAdjustmentUploadServiceClient conversionAdjustmentUploadServiceClient =
* ConversionAdjustmentUploadServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List conversionAdjustments = new ArrayList<>();
* boolean partialFailure = true;
* UploadConversionAdjustmentsResponse response =
* conversionAdjustmentUploadServiceClient.uploadConversionAdjustments(
* customerId, conversionAdjustments, partialFailure);
* }
* }
*
* ======================= ConversionCustomVariableServiceClient =======================
*
*
Service Description: Service to manage conversion custom variables.
*
*
Sample for ConversionCustomVariableServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversionCustomVariableServiceClient conversionCustomVariableServiceClient =
* ConversionCustomVariableServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateConversionCustomVariablesResponse response =
* conversionCustomVariableServiceClient.mutateConversionCustomVariables(
* customerId, operations);
* }
* }
*
* ======================= ConversionGoalCampaignConfigServiceClient =======================
*
*
Service Description: Service to manage conversion goal campaign config.
*
*
Sample for ConversionGoalCampaignConfigServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversionGoalCampaignConfigServiceClient conversionGoalCampaignConfigServiceClient =
* ConversionGoalCampaignConfigServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateConversionGoalCampaignConfigsResponse response =
* conversionGoalCampaignConfigServiceClient.mutateConversionGoalCampaignConfigs(
* customerId, operations);
* }
* }
*
* ======================= ConversionUploadServiceClient =======================
*
*
Service Description: Service to upload conversions.
*
*
Sample for ConversionUploadServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversionUploadServiceClient conversionUploadServiceClient =
* ConversionUploadServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List conversions = new ArrayList<>();
* boolean partialFailure = true;
* UploadClickConversionsResponse response =
* conversionUploadServiceClient.uploadClickConversions(
* customerId, conversions, partialFailure);
* }
* }
*
* ======================= ConversionValueRuleServiceClient =======================
*
*
Service Description: Service to manage conversion value rules.
*
*
Sample for ConversionValueRuleServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversionValueRuleServiceClient conversionValueRuleServiceClient =
* ConversionValueRuleServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateConversionValueRulesResponse response =
* conversionValueRuleServiceClient.mutateConversionValueRules(customerId, operations);
* }
* }
*
* ======================= ConversionValueRuleSetServiceClient =======================
*
*
Service Description: Service to manage conversion value rule sets.
*
*
Sample for ConversionValueRuleSetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversionValueRuleSetServiceClient conversionValueRuleSetServiceClient =
* ConversionValueRuleSetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateConversionValueRuleSetsResponse response =
* conversionValueRuleSetServiceClient.mutateConversionValueRuleSets(customerId, operations);
* }
* }
*
* ======================= CustomAudienceServiceClient =======================
*
*
Service Description: Service to manage custom audiences.
*
*
Sample for CustomAudienceServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomAudienceServiceClient customAudienceServiceClient =
* CustomAudienceServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomAudiencesResponse response =
* customAudienceServiceClient.mutateCustomAudiences(customerId, operations);
* }
* }
*
* ======================= CustomConversionGoalServiceClient =======================
*
*
Service Description: Service to manage custom conversion goal.
*
*
Sample for CustomConversionGoalServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomConversionGoalServiceClient customConversionGoalServiceClient =
* CustomConversionGoalServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomConversionGoalsResponse response =
* customConversionGoalServiceClient.mutateCustomConversionGoals(customerId, operations);
* }
* }
*
* ======================= CustomInterestServiceClient =======================
*
*
Service Description: Service to manage custom interests.
*
*
Sample for CustomInterestServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomInterestServiceClient customInterestServiceClient =
* CustomInterestServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomInterestsResponse response =
* customInterestServiceClient.mutateCustomInterests(customerId, operations);
* }
* }
*
* ======================= CustomerAssetServiceClient =======================
*
*
Service Description: Service to manage customer assets.
*
*
Sample for CustomerAssetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerAssetServiceClient customerAssetServiceClient =
* CustomerAssetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomerAssetsResponse response =
* customerAssetServiceClient.mutateCustomerAssets(customerId, operations);
* }
* }
*
* ======================= CustomerAssetSetServiceClient =======================
*
*
Service Description: Service to manage customer asset set
*
*
Sample for CustomerAssetSetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerAssetSetServiceClient customerAssetSetServiceClient =
* CustomerAssetSetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomerAssetSetsResponse response =
* customerAssetSetServiceClient.mutateCustomerAssetSets(customerId, operations);
* }
* }
*
* ======================= CustomerClientLinkServiceClient =======================
*
*
Service Description: Service to manage customer client links.
*
*
Sample for CustomerClientLinkServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerClientLinkServiceClient customerClientLinkServiceClient =
* CustomerClientLinkServiceClient.create()) {
* String customerId = "customerId-1581184615";
* CustomerClientLinkOperation operation = CustomerClientLinkOperation.newBuilder().build();
* MutateCustomerClientLinkResponse response =
* customerClientLinkServiceClient.mutateCustomerClientLink(customerId, operation);
* }
* }
*
* ======================= CustomerConversionGoalServiceClient =======================
*
*
Service Description: Service to manage customer conversion goal.
*
*
Sample for CustomerConversionGoalServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerConversionGoalServiceClient customerConversionGoalServiceClient =
* CustomerConversionGoalServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomerConversionGoalsResponse response =
* customerConversionGoalServiceClient.mutateCustomerConversionGoals(customerId, operations);
* }
* }
*
* ======================= CustomerCustomizerServiceClient =======================
*
*
Service Description: Service to manage customer customizer
*
*
Sample for CustomerCustomizerServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerCustomizerServiceClient customerCustomizerServiceClient =
* CustomerCustomizerServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomerCustomizersResponse response =
* customerCustomizerServiceClient.mutateCustomerCustomizers(customerId, operations);
* }
* }
*
* ======================= CustomerExtensionSettingServiceClient =======================
*
*
Service Description: Service to manage customer extension settings.
*
*
Sample for CustomerExtensionSettingServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerExtensionSettingServiceClient customerExtensionSettingServiceClient =
* CustomerExtensionSettingServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomerExtensionSettingsResponse response =
* customerExtensionSettingServiceClient.mutateCustomerExtensionSettings(
* customerId, operations);
* }
* }
*
* ======================= CustomerFeedServiceClient =======================
*
*
Service Description: Service to manage customer feeds.
*
*
Sample for CustomerFeedServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerFeedServiceClient customerFeedServiceClient = CustomerFeedServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomerFeedsResponse response =
* customerFeedServiceClient.mutateCustomerFeeds(customerId, operations);
* }
* }
*
* ======================= CustomerLabelServiceClient =======================
*
*
Service Description: Service to manage labels on customers.
*
*
Sample for CustomerLabelServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerLabelServiceClient customerLabelServiceClient =
* CustomerLabelServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomerLabelsResponse response =
* customerLabelServiceClient.mutateCustomerLabels(customerId, operations);
* }
* }
*
* ======================= CustomerLifecycleGoalServiceClient =======================
*
*
Service Description: Service to configure customer lifecycle goals.
*
*
Sample for CustomerLifecycleGoalServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerLifecycleGoalServiceClient customerLifecycleGoalServiceClient =
* CustomerLifecycleGoalServiceClient.create()) {
* String customerId = "customerId-1581184615";
* CustomerLifecycleGoalOperation operation =
* CustomerLifecycleGoalOperation.newBuilder().build();
* ConfigureCustomerLifecycleGoalsResponse response =
* customerLifecycleGoalServiceClient.configureCustomerLifecycleGoals(customerId, operation);
* }
* }
*
* ======================= CustomerManagerLinkServiceClient =======================
*
*
Service Description: Service to manage customer-manager links.
*
*
Sample for CustomerManagerLinkServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerManagerLinkServiceClient customerManagerLinkServiceClient =
* CustomerManagerLinkServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomerManagerLinkResponse response =
* customerManagerLinkServiceClient.mutateCustomerManagerLink(customerId, operations);
* }
* }
*
* ======================= CustomerNegativeCriterionServiceClient =======================
*
*
Service Description: Service to manage customer negative criteria.
*
*
Sample for CustomerNegativeCriterionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerNegativeCriterionServiceClient customerNegativeCriterionServiceClient =
* CustomerNegativeCriterionServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomerNegativeCriteriaResponse response =
* customerNegativeCriterionServiceClient.mutateCustomerNegativeCriteria(
* customerId, operations);
* }
* }
*
* ======================= CustomerServiceClient =======================
*
*
Service Description: Service to manage customers.
*
*
Sample for CustomerServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerServiceClient customerServiceClient = CustomerServiceClient.create()) {
* String customerId = "customerId-1581184615";
* CustomerOperation operation = CustomerOperation.newBuilder().build();
* MutateCustomerResponse response = customerServiceClient.mutateCustomer(customerId, operation);
* }
* }
*
* ======================= CustomerSkAdNetworkConversionValueSchemaServiceClient
* =======================
*
*
Service Description: Service to manage CustomerSkAdNetworkConversionValueSchema.
*
*
Sample for CustomerSkAdNetworkConversionValueSchemaServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerSkAdNetworkConversionValueSchemaServiceClient
* customerSkAdNetworkConversionValueSchemaServiceClient =
* CustomerSkAdNetworkConversionValueSchemaServiceClient.create()) {
* MutateCustomerSkAdNetworkConversionValueSchemaRequest request =
* MutateCustomerSkAdNetworkConversionValueSchemaRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .setOperation(CustomerSkAdNetworkConversionValueSchemaOperation.newBuilder().build())
* .setValidateOnly(true)
* .setEnableWarnings(true)
* .build();
* MutateCustomerSkAdNetworkConversionValueSchemaResponse response =
* customerSkAdNetworkConversionValueSchemaServiceClient
* .mutateCustomerSkAdNetworkConversionValueSchema(request);
* }
* }
*
* ======================= CustomerUserAccessInvitationServiceClient =======================
*
*
Service Description: This service manages the access invitation extended to users for a given
* customer.
*
*
Sample for CustomerUserAccessInvitationServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerUserAccessInvitationServiceClient customerUserAccessInvitationServiceClient =
* CustomerUserAccessInvitationServiceClient.create()) {
* String customerId = "customerId-1581184615";
* CustomerUserAccessInvitationOperation operation =
* CustomerUserAccessInvitationOperation.newBuilder().build();
* MutateCustomerUserAccessInvitationResponse response =
* customerUserAccessInvitationServiceClient.mutateCustomerUserAccessInvitation(
* customerId, operation);
* }
* }
*
* ======================= CustomerUserAccessServiceClient =======================
*
*
Service Description: This service manages the permissions of a user on a given customer.
*
*
Sample for CustomerUserAccessServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomerUserAccessServiceClient customerUserAccessServiceClient =
* CustomerUserAccessServiceClient.create()) {
* String customerId = "customerId-1581184615";
* CustomerUserAccessOperation operation = CustomerUserAccessOperation.newBuilder().build();
* MutateCustomerUserAccessResponse response =
* customerUserAccessServiceClient.mutateCustomerUserAccess(customerId, operation);
* }
* }
*
* ======================= CustomizerAttributeServiceClient =======================
*
*
Service Description: Service to manage customizer attribute
*
*
Sample for CustomizerAttributeServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CustomizerAttributeServiceClient customizerAttributeServiceClient =
* CustomizerAttributeServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateCustomizerAttributesResponse response =
* customizerAttributeServiceClient.mutateCustomizerAttributes(customerId, operations);
* }
* }
*
* ======================= ExperimentArmServiceClient =======================
*
*
Service Description: Service to manage experiment arms.
*
*
Sample for ExperimentArmServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ExperimentArmServiceClient experimentArmServiceClient =
* ExperimentArmServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateExperimentArmsResponse response =
* experimentArmServiceClient.mutateExperimentArms(customerId, operations);
* }
* }
*
* ======================= ExperimentServiceClient =======================
*
*
Service Description: Service to manage experiments.
*
*
Sample for ExperimentServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ExperimentServiceClient experimentServiceClient = ExperimentServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateExperimentsResponse response =
* experimentServiceClient.mutateExperiments(customerId, operations);
* }
* }
*
* ======================= ExtensionFeedItemServiceClient =======================
*
*
Service Description: Service to manage extension feed items.
*
*
Sample for ExtensionFeedItemServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ExtensionFeedItemServiceClient extensionFeedItemServiceClient =
* ExtensionFeedItemServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateExtensionFeedItemsResponse response =
* extensionFeedItemServiceClient.mutateExtensionFeedItems(customerId, operations);
* }
* }
*
* ======================= FeedItemServiceClient =======================
*
*
Service Description: Service to manage feed items.
*
*
Sample for FeedItemServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FeedItemServiceClient feedItemServiceClient = FeedItemServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateFeedItemsResponse response =
* feedItemServiceClient.mutateFeedItems(customerId, operations);
* }
* }
*
* ======================= FeedItemSetLinkServiceClient =======================
*
*
Service Description: Service to manage feed item set links.
*
*
Sample for FeedItemSetLinkServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FeedItemSetLinkServiceClient feedItemSetLinkServiceClient =
* FeedItemSetLinkServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateFeedItemSetLinksResponse response =
* feedItemSetLinkServiceClient.mutateFeedItemSetLinks(customerId, operations);
* }
* }
*
* ======================= FeedItemSetServiceClient =======================
*
*
Service Description: Service to manage feed Item Set
*
*
Sample for FeedItemSetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FeedItemSetServiceClient feedItemSetServiceClient = FeedItemSetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateFeedItemSetsResponse response =
* feedItemSetServiceClient.mutateFeedItemSets(customerId, operations);
* }
* }
*
* ======================= FeedItemTargetServiceClient =======================
*
*
Service Description: Service to manage feed item targets.
*
*
Sample for FeedItemTargetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FeedItemTargetServiceClient feedItemTargetServiceClient =
* FeedItemTargetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateFeedItemTargetsResponse response =
* feedItemTargetServiceClient.mutateFeedItemTargets(customerId, operations);
* }
* }
*
* ======================= FeedMappingServiceClient =======================
*
*
Service Description: Service to manage feed mappings.
*
*
Sample for FeedMappingServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FeedMappingServiceClient feedMappingServiceClient = FeedMappingServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateFeedMappingsResponse response =
* feedMappingServiceClient.mutateFeedMappings(customerId, operations);
* }
* }
*
* ======================= FeedServiceClient =======================
*
*
Service Description: Service to manage feeds.
*
*
Sample for FeedServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FeedServiceClient feedServiceClient = FeedServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateFeedsResponse response = feedServiceClient.mutateFeeds(customerId, operations);
* }
* }
*
* ======================= GeoTargetConstantServiceClient =======================
*
*
Service Description: Service to fetch geo target constants.
*
*
Sample for GeoTargetConstantServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (GeoTargetConstantServiceClient geoTargetConstantServiceClient =
* GeoTargetConstantServiceClient.create()) {
* SuggestGeoTargetConstantsRequest request =
* SuggestGeoTargetConstantsRequest.newBuilder()
* .setLocale("locale-1097462182")
* .setCountryCode("countryCode-1477067101")
* .build();
* SuggestGeoTargetConstantsResponse response =
* geoTargetConstantServiceClient.suggestGeoTargetConstants(request);
* }
* }
*
* ======================= GoogleAdsFieldServiceClient =======================
*
*
Service Description: Service to fetch Google Ads API fields.
*
*
Sample for GoogleAdsFieldServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (GoogleAdsFieldServiceClient googleAdsFieldServiceClient =
* GoogleAdsFieldServiceClient.create()) {
* GoogleAdsFieldName resourceName = GoogleAdsFieldName.of("[GOOGLE_ADS_FIELD]");
* GoogleAdsField response = googleAdsFieldServiceClient.getGoogleAdsField(resourceName);
* }
* }
*
* ======================= GoogleAdsServiceClient =======================
*
*
Service Description: Service to fetch data and metrics across resources.
*
*
Sample for GoogleAdsServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (GoogleAdsServiceClient googleAdsServiceClient = GoogleAdsServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List mutateOperations = new ArrayList<>();
* MutateGoogleAdsResponse response =
* googleAdsServiceClient.mutate(customerId, mutateOperations);
* }
* }
*
* ======================= IdentityVerificationServiceClient =======================
*
*
Service Description: A service for managing Identity Verification Service.
*
*
Sample for IdentityVerificationServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IdentityVerificationServiceClient identityVerificationServiceClient =
* IdentityVerificationServiceClient.create()) {
* String customerId = "customerId-1581184615";
* IdentityVerificationProgramEnum.IdentityVerificationProgram verificationProgram =
* IdentityVerificationProgramEnum.IdentityVerificationProgram.forNumber(0);
* identityVerificationServiceClient.startIdentityVerification(customerId, verificationProgram);
* }
* }
*
* ======================= InvoiceServiceClient =======================
*
*
Service Description: A service to fetch invoices issued for a billing setup during a given
* month.
*
*
Sample for InvoiceServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (InvoiceServiceClient invoiceServiceClient = InvoiceServiceClient.create()) {
* String customerId = "customerId-1581184615";
* String billingSetup = "billingSetup-1435075390";
* String issueYear = "issueYear184914102";
* MonthOfYearEnum.MonthOfYear issueMonth = MonthOfYearEnum.MonthOfYear.forNumber(0);
* ListInvoicesResponse response =
* invoiceServiceClient.listInvoices(customerId, billingSetup, issueYear, issueMonth);
* }
* }
*
* ======================= KeywordPlanAdGroupKeywordServiceClient =======================
*
*
Service Description: Service to manage Keyword Plan ad group keywords. KeywordPlanAdGroup is
* required to add ad group keywords. Positive and negative keywords are supported. A maximum of
* 10,000 positive keywords are allowed per keyword plan. A maximum of 1,000 negative keywords are
* allower per keyword plan. This includes campaign negative keywords and ad group negative
* keywords.
*
*
Sample for KeywordPlanAdGroupKeywordServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (KeywordPlanAdGroupKeywordServiceClient keywordPlanAdGroupKeywordServiceClient =
* KeywordPlanAdGroupKeywordServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateKeywordPlanAdGroupKeywordsResponse response =
* keywordPlanAdGroupKeywordServiceClient.mutateKeywordPlanAdGroupKeywords(
* customerId, operations);
* }
* }
*
* ======================= KeywordPlanAdGroupServiceClient =======================
*
*
Service Description: Service to manage Keyword Plan ad groups.
*
*
Sample for KeywordPlanAdGroupServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (KeywordPlanAdGroupServiceClient keywordPlanAdGroupServiceClient =
* KeywordPlanAdGroupServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateKeywordPlanAdGroupsResponse response =
* keywordPlanAdGroupServiceClient.mutateKeywordPlanAdGroups(customerId, operations);
* }
* }
*
* ======================= KeywordPlanCampaignKeywordServiceClient =======================
*
*
Service Description: Service to manage Keyword Plan campaign keywords. KeywordPlanCampaign is
* required to add the campaign keywords. Only negative keywords are supported. A maximum of 1000
* negative keywords are allowed per plan. This includes both campaign negative keywords and ad
* group negative keywords.
*
*
Sample for KeywordPlanCampaignKeywordServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (KeywordPlanCampaignKeywordServiceClient keywordPlanCampaignKeywordServiceClient =
* KeywordPlanCampaignKeywordServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateKeywordPlanCampaignKeywordsResponse response =
* keywordPlanCampaignKeywordServiceClient.mutateKeywordPlanCampaignKeywords(
* customerId, operations);
* }
* }
*
* ======================= KeywordPlanCampaignServiceClient =======================
*
*
Service Description: Service to manage Keyword Plan campaigns.
*
*
Sample for KeywordPlanCampaignServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (KeywordPlanCampaignServiceClient keywordPlanCampaignServiceClient =
* KeywordPlanCampaignServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateKeywordPlanCampaignsResponse response =
* keywordPlanCampaignServiceClient.mutateKeywordPlanCampaigns(customerId, operations);
* }
* }
*
* ======================= KeywordPlanIdeaServiceClient =======================
*
*
Service Description: Service to generate keyword ideas.
*
*
Sample for KeywordPlanIdeaServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (KeywordPlanIdeaServiceClient keywordPlanIdeaServiceClient =
* KeywordPlanIdeaServiceClient.create()) {
* GenerateKeywordHistoricalMetricsRequest request =
* GenerateKeywordHistoricalMetricsRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .addAllKeywords(new ArrayList())
* .setLanguage("language-1613589672")
* .setIncludeAdultKeywords(true)
* .addAllGeoTargetConstants(new ArrayList())
* .setAggregateMetrics(KeywordPlanAggregateMetrics.newBuilder().build())
* .setHistoricalMetricsOptions(HistoricalMetricsOptions.newBuilder().build())
* .build();
* GenerateKeywordHistoricalMetricsResponse response =
* keywordPlanIdeaServiceClient.generateKeywordHistoricalMetrics(request);
* }
* }
*
* ======================= KeywordPlanServiceClient =======================
*
*
Service Description: Service to manage keyword plans.
*
*
Sample for KeywordPlanServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (KeywordPlanServiceClient keywordPlanServiceClient = KeywordPlanServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateKeywordPlansResponse response =
* keywordPlanServiceClient.mutateKeywordPlans(customerId, operations);
* }
* }
*
* ======================= KeywordThemeConstantServiceClient =======================
*
*
Service Description: Service to fetch Smart Campaign keyword themes.
*
*
Sample for KeywordThemeConstantServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (KeywordThemeConstantServiceClient keywordThemeConstantServiceClient =
* KeywordThemeConstantServiceClient.create()) {
* SuggestKeywordThemeConstantsRequest request =
* SuggestKeywordThemeConstantsRequest.newBuilder()
* .setQueryText("queryText-1806881259")
* .setCountryCode("countryCode-1477067101")
* .setLanguageCode("languageCode-2092349083")
* .build();
* SuggestKeywordThemeConstantsResponse response =
* keywordThemeConstantServiceClient.suggestKeywordThemeConstants(request);
* }
* }
*
* ======================= LabelServiceClient =======================
*
*
Service Description: Service to manage labels.
*
*
Sample for LabelServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LabelServiceClient labelServiceClient = LabelServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateLabelsResponse response = labelServiceClient.mutateLabels(customerId, operations);
* }
* }
*
* ======================= LocalServicesLeadServiceClient =======================
*
*
Service Description: This service allows management of LocalServicesLead resources.
*
*
Sample for LocalServicesLeadServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LocalServicesLeadServiceClient localServicesLeadServiceClient =
* LocalServicesLeadServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List conversations = new ArrayList<>();
* AppendLeadConversationResponse response =
* localServicesLeadServiceClient.appendLeadConversation(customerId, conversations);
* }
* }
*
* ======================= OfflineUserDataJobServiceClient =======================
*
*
Service Description: Service to manage offline user data jobs.
*
*
Sample for OfflineUserDataJobServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OfflineUserDataJobServiceClient offlineUserDataJobServiceClient =
* OfflineUserDataJobServiceClient.create()) {
* String customerId = "customerId-1581184615";
* OfflineUserDataJob job = OfflineUserDataJob.newBuilder().build();
* CreateOfflineUserDataJobResponse response =
* offlineUserDataJobServiceClient.createOfflineUserDataJob(customerId, job);
* }
* }
*
* ======================= PaymentsAccountServiceClient =======================
*
*
Service Description: Service to provide payments accounts that can be used to set up
* consolidated billing.
*
*
Sample for PaymentsAccountServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PaymentsAccountServiceClient paymentsAccountServiceClient =
* PaymentsAccountServiceClient.create()) {
* String customerId = "customerId-1581184615";
* ListPaymentsAccountsResponse response =
* paymentsAccountServiceClient.listPaymentsAccounts(customerId);
* }
* }
*
* ======================= ProductLinkInvitationServiceClient =======================
*
*
Service Description: This service allows management of product link invitations from Google
* Ads accounts to other accounts.
*
*
Sample for ProductLinkInvitationServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ProductLinkInvitationServiceClient productLinkInvitationServiceClient =
* ProductLinkInvitationServiceClient.create()) {
* String customerId = "customerId-1581184615";
* ProductLinkInvitation productLinkInvitation = ProductLinkInvitation.newBuilder().build();
* CreateProductLinkInvitationResponse response =
* productLinkInvitationServiceClient.createProductLinkInvitation(
* customerId, productLinkInvitation);
* }
* }
*
* ======================= ProductLinkServiceClient =======================
*
*
Service Description: This service allows management of links between a Google Ads customer and
* another product.
*
*
Sample for ProductLinkServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ProductLinkServiceClient productLinkServiceClient = ProductLinkServiceClient.create()) {
* String customerId = "customerId-1581184615";
* ProductLink productLink = ProductLink.newBuilder().build();
* CreateProductLinkResponse response =
* productLinkServiceClient.createProductLink(customerId, productLink);
* }
* }
*
* ======================= ReachPlanServiceClient =======================
*
*
Service Description: Reach Plan Service gives users information about audience size that can
* be reached through advertisement on YouTube. In particular, GenerateReachForecast provides
* estimated number of people of specified demographics that can be reached by an ad in a given
* market by a campaign of certain duration with a defined budget.
*
*
Sample for ReachPlanServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ReachPlanServiceClient reachPlanServiceClient = ReachPlanServiceClient.create()) {
* ListPlannableLocationsRequest request = ListPlannableLocationsRequest.newBuilder().build();
* ListPlannableLocationsResponse response =
* reachPlanServiceClient.listPlannableLocations(request);
* }
* }
*
* ======================= RecommendationServiceClient =======================
*
*
Service Description: Service to manage recommendations.
*
*
Sample for RecommendationServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RecommendationServiceClient recommendationServiceClient =
* RecommendationServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* ApplyRecommendationResponse response =
* recommendationServiceClient.applyRecommendation(customerId, operations);
* }
* }
*
* ======================= RecommendationSubscriptionServiceClient =======================
*
*
Service Description: Service to manage recommendation subscriptions.
*
*
Sample for RecommendationSubscriptionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RecommendationSubscriptionServiceClient recommendationSubscriptionServiceClient =
* RecommendationSubscriptionServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateRecommendationSubscriptionResponse response =
* recommendationSubscriptionServiceClient.mutateRecommendationSubscription(
* customerId, operations);
* }
* }
*
* ======================= RemarketingActionServiceClient =======================
*
*
Service Description: Service to manage remarketing actions.
*
*
Sample for RemarketingActionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RemarketingActionServiceClient remarketingActionServiceClient =
* RemarketingActionServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateRemarketingActionsResponse response =
* remarketingActionServiceClient.mutateRemarketingActions(customerId, operations);
* }
* }
*
* ======================= ShareablePreviewServiceClient =======================
*
*
Service Description: Service to generate Shareable Previews.
*
*
Sample for ShareablePreviewServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ShareablePreviewServiceClient shareablePreviewServiceClient =
* ShareablePreviewServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List shareablePreviews = new ArrayList<>();
* GenerateShareablePreviewsResponse response =
* shareablePreviewServiceClient.generateShareablePreviews(customerId, shareablePreviews);
* }
* }
*
* ======================= SharedCriterionServiceClient =======================
*
*
Service Description: Service to manage shared criteria.
*
*
Sample for SharedCriterionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (SharedCriterionServiceClient sharedCriterionServiceClient =
* SharedCriterionServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateSharedCriteriaResponse response =
* sharedCriterionServiceClient.mutateSharedCriteria(customerId, operations);
* }
* }
*
* ======================= SharedSetServiceClient =======================
*
*
Service Description: Service to manage shared sets.
*
*
Sample for SharedSetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (SharedSetServiceClient sharedSetServiceClient = SharedSetServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateSharedSetsResponse response =
* sharedSetServiceClient.mutateSharedSets(customerId, operations);
* }
* }
*
* ======================= SmartCampaignSettingServiceClient =======================
*
*
Service Description: Service to manage Smart campaign settings.
*
*
Sample for SmartCampaignSettingServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (SmartCampaignSettingServiceClient smartCampaignSettingServiceClient =
* SmartCampaignSettingServiceClient.create()) {
* SmartCampaignSettingName resourceName =
* SmartCampaignSettingName.of("[CUSTOMER_ID]", "[CAMPAIGN_ID]");
* GetSmartCampaignStatusResponse response =
* smartCampaignSettingServiceClient.getSmartCampaignStatus(resourceName);
* }
* }
*
* ======================= SmartCampaignSuggestServiceClient =======================
*
*
Service Description: Service to get suggestions for Smart Campaigns.
*
*
Sample for SmartCampaignSuggestServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (SmartCampaignSuggestServiceClient smartCampaignSuggestServiceClient =
* SmartCampaignSuggestServiceClient.create()) {
* SuggestSmartCampaignBudgetOptionsRequest request =
* SuggestSmartCampaignBudgetOptionsRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .build();
* SuggestSmartCampaignBudgetOptionsResponse response =
* smartCampaignSuggestServiceClient.suggestSmartCampaignBudgetOptions(request);
* }
* }
*
* ======================= ThirdPartyAppAnalyticsLinkServiceClient =======================
*
*
Service Description: This service allows management of links between Google Ads and third
* party app analytics.
*
*
Sample for ThirdPartyAppAnalyticsLinkServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ThirdPartyAppAnalyticsLinkServiceClient thirdPartyAppAnalyticsLinkServiceClient =
* ThirdPartyAppAnalyticsLinkServiceClient.create()) {
* RegenerateShareableLinkIdRequest request =
* RegenerateShareableLinkIdRequest.newBuilder()
* .setResourceName(
* ThirdPartyAppAnalyticsLinkName.of("[CUSTOMER_ID]", "[CUSTOMER_LINK_ID]")
* .toString())
* .build();
* RegenerateShareableLinkIdResponse response =
* thirdPartyAppAnalyticsLinkServiceClient.regenerateShareableLinkId(request);
* }
* }
*
* ======================= TravelAssetSuggestionServiceClient =======================
*
*
Service Description: Service to retrieve Travel asset suggestions.
*
*
Sample for TravelAssetSuggestionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TravelAssetSuggestionServiceClient travelAssetSuggestionServiceClient =
* TravelAssetSuggestionServiceClient.create()) {
* String customerId = "customerId-1581184615";
* String languageOption = "languageOption-357816851";
* SuggestTravelAssetsResponse response =
* travelAssetSuggestionServiceClient.suggestTravelAssets(customerId, languageOption);
* }
* }
*
* ======================= UserDataServiceClient =======================
*
*
Service Description: Service to manage user data uploads. Any uploads made to a Customer Match
* list through this service will be eligible for matching as per the customer matching process. See
* https://support.google.com/google-ads/answer/7474263. However, the uploads made through this
* service will not be visible under the 'Segment members' section for the Customer Match List in
* the Google Ads UI.
*
*
Sample for UserDataServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (UserDataServiceClient userDataServiceClient = UserDataServiceClient.create()) {
* UploadUserDataRequest request =
* UploadUserDataRequest.newBuilder()
* .setCustomerId("customerId-1581184615")
* .addAllOperations(new ArrayList())
* .build();
* UploadUserDataResponse response = userDataServiceClient.uploadUserData(request);
* }
* }
*
* ======================= UserListCustomerTypeServiceClient =======================
*
*
Service Description: Service to manage user list customer types.
*
*
Sample for UserListCustomerTypeServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (UserListCustomerTypeServiceClient userListCustomerTypeServiceClient =
* UserListCustomerTypeServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateUserListCustomerTypesResponse response =
* userListCustomerTypeServiceClient.mutateUserListCustomerTypes(customerId, operations);
* }
* }
*
* ======================= UserListServiceClient =======================
*
*
Service Description: Service to manage user lists.
*
*
Sample for UserListServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (UserListServiceClient userListServiceClient = UserListServiceClient.create()) {
* String customerId = "customerId-1581184615";
* List operations = new ArrayList<>();
* MutateUserListsResponse response =
* userListServiceClient.mutateUserLists(customerId, operations);
* }
* }
*/
@Generated("by gapic-generator-java")
package com.google.ads.googleads.v17.services;
import javax.annotation.Generated;