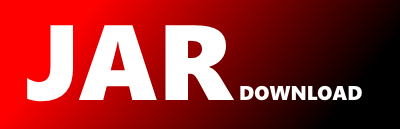
com.google.api.client.http.HttpHeaders Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-api-client Show documentation
Show all versions of google-api-client Show documentation
Google API Client Library for Java. Supports Java 5 (or higher) desktop (SE)
and web (EE), Android, and Google App Engine.
/*
* Copyright (c) 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.google.api.client.http;
import com.google.api.client.util.ClassInfo;
import com.google.api.client.util.GenericData;
import com.google.api.client.util.Key;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
/**
* Stores HTTP headers used in an HTTP request or response, as defined in Header Field
* Definitions.
*
* {@code null} is not allowed as a name or value of a header. Names are
* case-insensitive.
*
* @since 1.0
* @author Yaniv Inbar
*/
public class HttpHeaders extends GenericData {
/** {@code "Accept"} header. */
@Key("Accept")
public String accept;
/**
* {@code "Accept-Encoding"} header. By default, this is {@code "gzip"}.
*
* Warning for users upgrading from version 2.2 of gdata-java-client
* library: the default used to be {@code null} but now it is {@code
* "gzip"}
*
*/
@Key("Accept-Encoding")
public String acceptEncoding = "gzip";
/** {@code "Authorization"} header. */
@Key("Authorization")
public String authorization;
/** {@code "Cache-Control"} header. */
@Key("Cache-Control")
public String cacheControl;
/** {@code "Content-Encoding"} header. */
@Key("Content-Encoding")
public String contentEncoding;
/** {@code "Content-Length"} header. */
@Key("Content-Length")
public String contentLength;
/** {@code "Content-MD5"} header. */
@Key("Content-MD5")
public String contentMD5;
/** {@code "Content-Range"} header. */
@Key("Content-Range")
public String contentRange;
/** {@code "Content-Type"} header. */
@Key("Content-Type")
public String contentType;
/** {@code "Date"} header. */
@Key("Date")
public String date;
/** {@code "ETag"} header. */
@Key("ETag")
public String etag;
/** {@code "Expires"} header. */
@Key("Expires")
public String expires;
/** {@code "If-Modified-Since"} header. */
@Key("If-Modified-Since")
public String ifModifiedSince;
/** {@code "If-Match"} header. */
@Key("If-Match")
public String ifMatch;
/** {@code "If-None-Match"} header. */
@Key("If-None-Match")
public String ifNoneMatch;
/** {@code "If-Unmodified-Since"} header. */
@Key("If-Unmodified-Since")
public String ifUnmodifiedSince;
/** {@code "Last-Modified"} header. */
@Key("Last-Modified")
public String lastModified;
/** {@code "Location"} header. */
@Key("Location")
public String location;
/** {@code "MIME-Version"} header. */
@Key("MIME-Version")
public String mimeVersion;
/** {@code "Range"} header. */
@Key("Range")
public String range;
/** {@code "Retry-After"} header. */
@Key("Retry-After")
public String retryAfter;
/** {@code "User-Agent"} header. */
@Key("User-Agent")
public String userAgent;
/** {@code "WWW-Authenticate"} header. */
@Key("WWW-Authenticate")
public String authenticate;
@Override
public HttpHeaders clone() {
return (HttpHeaders) super.clone();
}
/**
* Computes a canonical map from lower-case header name to its values.
*
* @return canonical map from lower-case header name to its values
*/
public Map> canonicalMap() {
Map> result =
new HashMap>();
for (Map.Entry entry : entrySet()) {
String canonicalName = entry.getKey().toLowerCase();
if (result.containsKey(canonicalName)) {
throw new IllegalArgumentException(
"multiple headers of the same name (headers are case insensitive): "
+ canonicalName);
}
Object value = entry.getValue();
if (value != null) {
if (value instanceof Collection>) {
@SuppressWarnings("unchecked")
Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy