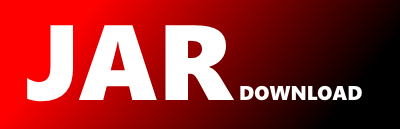
com.google.api.client.http.UrlEncodedParser Maven / Gradle / Ivy
Show all versions of google-api-client Show documentation
/*
* Copyright (c) 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.google.api.client.http;
import com.google.api.client.escape.CharEscapers;
import com.google.api.client.util.ClassInfo;
import com.google.api.client.util.FieldInfo;
import com.google.api.client.util.GenericData;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Map;
import java.util.logging.Level;
/**
* Implements support for HTTP form content encoding parsing of type {@code
* application/x-www-form-urlencoded} as specified in the HTML 4.0 Specification.
*
* The data is parsed using {@link #parse(String, Object)}.
*
*
* Sample usage:
*
*
*
* static void setParser(HttpTransport transport) {
* transport.addParser(new UrlEncodedParser());
* }
*
*
*
*
*
* @since 1.0
* @author Yaniv Inbar
*/
public final class UrlEncodedParser implements HttpParser {
/** {@code "application/x-www-form-urlencoded"} content type. */
public static final String CONTENT_TYPE = "application/x-www-form-urlencoded";
/**
* Whether to disable response content logging (unless {@link Level#ALL} is
* loggable which forces all logging).
*
* Useful for example if content has sensitive data such as an authentication
* token. Defaults to {@code false}.
*/
public boolean disableContentLogging;
/** Content type. Default value is {@link #CONTENT_TYPE}. */
public String contentType = CONTENT_TYPE;
public String getContentType() {
return this.contentType;
}
public T parse(HttpResponse response, Class dataClass)
throws IOException {
if (this.disableContentLogging) {
response.disableContentLogging = true;
}
T newInstance = ClassInfo.newInstance(dataClass);
parse(response.parseAsString(), newInstance);
return newInstance;
}
/**
* Parses the given URL-encoded content into the given data object of data key
* name/value pairs, including support for repeating data key names.
*
* Declared fields of a "primitive" type (as defined by
* {@link FieldInfo#isPrimitive(Class)} are parsed using
* {@link FieldInfo#parsePrimitiveValue(Class, String)} where the
* {@link Class} parameter is the declared field class. Declared fields of
* type {@link Collection} are used to support repeating data key names, so
* each member of the collection is an additional data key value. They are
* parsed the same as "primitive" fields, except that the generic type
* parameter of the collection is used as the {@link Class} parameter. For
* keys not represented by a declared field, the field type is assumed to be
* {@link ArrayList}<String>.
*
* @param content URL-encoded content
* @param data data key name/value pairs
*/
@SuppressWarnings("unchecked")
public static void parse(String content, Object data) {
Class> clazz = data.getClass();
ClassInfo classInfo = ClassInfo.of(clazz);
GenericData genericData =
GenericData.class.isAssignableFrom(clazz) ? (GenericData) data : null;
@SuppressWarnings("unchecked")
Map