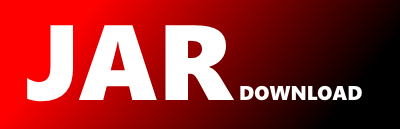
com.google.api.client.auth.oauth2.draft10.AuthorizationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-api-client Show documentation
Show all versions of google-api-client Show documentation
Google API Client Library for Java. Supports Java 5 (or higher) desktop (SE)
and web (EE), Android, and Google App Engine.
/*
* Copyright (c) 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.google.api.client.auth.oauth2.draft10;
import com.google.api.client.http.UrlEncodedParser;
import com.google.api.client.json.GenericJson;
import com.google.api.client.util.Key;
import java.net.URI;
import java.net.URISyntaxException;
/**
* OAuth 2.0 (draft 10) parser for the redirect URL after end user grants or denies authorization as
* specified in Authorization Response.
*
* Check if {@link #error} is {@code null} to check if the end-user granted authorization.
*
*
* Sample usage for a web application:
*
*
public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException {
StringBuffer fullUrlBuf = request.getRequestURL();
if (request.getQueryString() != null) {
fullUrlBuf.append('?').append(request.getQueryString());
}
AuthorizationResponse authResponse = new AuthorizationResponse(fullUrlBuf.toString());
// check for user-denied error
if (authResponse.error != null) {
// authorization denied...
} else {
// request access token using authResponse.code...
}
}
*
*
*
* Sample usage for an installed application:
*
*
static void processRedirectUrl(HttpTransport transport, String redirectUrl) {
AuthorizationResponse response = new AuthorizationResponse(redirectUrl);
if (response.error != null) {
throw new RuntimeException("Authorization denied");
}
AccessProtectedResource.usingAuthorizationHeader(transport, response.accessToken);
}
*
*
*
* @since 1.4
* @author Yaniv Inbar
*/
public class AuthorizationResponse extends GenericJson {
/**
* Error codes listed in Error Codes.
*/
public enum KnownError {
/**
* The request is missing a required parameter, includes an unsupported parameter or parameter
* value, or is otherwise malformed.
*/
INVALID_REQUEST,
/** The client identifier provided is invalid. */
INVALID_CLIENT,
/** The client is not authorized to use the requested response type. */
UNAUTHORIZED_CLIENT,
/** The redirection URI provided does not match a pre-registered value. */
REDIRECT_URI_MISMATCH,
/** The end-user or authorization server denied the request. */
ACCESS_DENIED,
/** The requested response type is not supported by the authorization server. */
UNSUPPORTED_RESPONSE_TYPE,
/** The requested scope is invalid, unknown, or malformed. */
INVALID_SCOPE;
}
/**
* (REQUIRED if the end user grants authorization and the response type is "code" or
* "code_and_token", otherwise MUST NOT be included) The authorization code generated by the
* authorization server. The authorization code SHOULD expire shortly after it is issued. The
* authorization server MUST invalidate the authorization code after a single usage. The
* authorization code is bound to the client identifier and redirection URI.
*/
@Key
public String code;
/**
* (REQUIRED if the end user grants authorization and the response type is "token" or
* "code_and_token", otherwise MUST NOT be included) The access token issued by the authorization
* server.
*/
@Key("access_token")
public String accessToken;
/**
* (OPTIONAL) The duration in seconds of the access token lifetime if an access token is included.
* For example, the value "3600" denotes that the access token will expire in one hour from the
* time the response was generated by the authorization server.
*/
@Key("expires_in")
public Long expiresIn;
/**
* (OPTIONAL) The scope of the access token as a list of space- delimited strings if an access
* token is included. The value of the "scope" parameter is defined by the authorization server.
* If the value contains multiple space-delimited strings, their order does not matter, and each
* string adds an additional access range to the requested scope. The authorization server SHOULD
* include the parameter if the requested scope is different from the one requested by the client.
*/
@Key
public String scope;
/**
* (REQUIRED if the end user denies authorization) A single error code.
*
* @see #getErrorCodeIfKnown()
*/
@Key
public String error;
/**
* (OPTIONAL) A human-readable text providing additional information, used to assist in the
* understanding and resolution of the error occurred.
*/
@Key("error_description")
public String errorDescription;
/**
* (OPTIONAL) A URI identifying a human-readable web page with information about the error, used
* to provide the end-user with additional information about the error.
*/
@Key("error_uri")
public String errorUri;
/**
* (REQUIRED if the "state" parameter was present in the client authorization request) Set to the
* exact value received from the client.
*/
@Key
public String state;
/**
* @param redirectUrl encoded redirect URL
* @throws IllegalArgumentException URI syntax exception
*/
public AuthorizationResponse(String redirectUrl) {
try {
URI uri = new URI(redirectUrl);
// need to check for parameters both in the URL query and the URL fragment
UrlEncodedParser.parse(uri.getRawQuery(), this);
UrlEncodedParser.parse(uri.getRawFragment(), this);
} catch (URISyntaxException e) {
throw new IllegalArgumentException(e);
}
}
/**
* Returns a known error code if {@link #error} is one of the error codes listed in the OAuth 2
* specification or {@code null} if the {@link #error} is {@code null} or not known.
*/
public final KnownError getErrorCodeIfKnown() {
if (error != null) {
try {
return KnownError.valueOf(error.toUpperCase());
} catch (IllegalArgumentException e) {
// ignore; most likely due to an unrecognized error code
}
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy