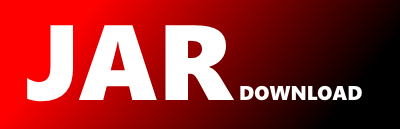
com.google.api.client.http.GenericUrl Maven / Gradle / Ivy
Show all versions of google-api-client Show documentation
/*
* Copyright (c) 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.google.api.client.http;
import com.google.api.client.util.GenericData;
import com.google.api.client.util.Key;
import com.google.api.client.util.escape.CharEscapers;
import com.google.api.client.util.escape.Escaper;
import com.google.api.client.util.escape.PercentEscaper;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* URL builder in which the query parameters are specified as generic data key/value pairs, based on
* the specification RFC 3986: Uniform Resource
* Identifier (URI).
*
* The query parameters are specified with the data key name as the parameter name, and the data
* value as the parameter value. Subclasses can declare fields for known query parameters using the
* {@link Key} annotation. {@code null} parameter names are not allowed, but {@code null} query
* values are allowed.
*
*
* Query parameter values are parsed using {@link UrlEncodedParser#parse(String, Object)}.
*
*
* @since 1.0
* @author Yaniv Inbar
*/
public class GenericUrl extends GenericData {
private static final Escaper URI_FRAGMENT_ESCAPER =
new PercentEscaper("=&-_.!~*'()@:$,;/?:", false);
/** Scheme (lowercase), for example {@code "https"}. */
public String scheme;
/** Host, for example {@code "www.google.com"}. */
public String host;
/** Port number or {@code -1} if undefined, for example {@code 443}. */
public int port = -1;
/**
* Decoded path component by parts with each part separated by a {@code '/'} or {@code null} for
* none, for example {@code "/m8/feeds/contacts/default/full"} is represented by {@code "", "m8",
* "feeds", "contacts", "default", "full"}.
*
* Use {@link #appendRawPath(String)} to append to the path, which ensures that no extra slash is
* added.
*
*/
public List pathParts;
/** Fragment component or {@code null} for none. */
public String fragment;
public GenericUrl() {
}
/**
* Constructs from an encoded URL.
*
* Any known query parameters with pre-defined fields as data keys will be parsed based on their
* data type. Any unrecognized query parameter will always be parsed as a string.
*
* @param encodedUrl encoded URL, including any existing query parameters that should be parsed
* @throws IllegalArgumentException if URL has a syntax error
*/
public GenericUrl(String encodedUrl) {
URI uri;
try {
uri = new URI(encodedUrl);
} catch (URISyntaxException e) {
throw new IllegalArgumentException(e);
}
scheme = uri.getScheme().toLowerCase();
host = uri.getHost();
port = uri.getPort();
pathParts = toPathParts(uri.getRawPath());
fragment = uri.getFragment();
String query = uri.getRawQuery();
if (query != null) {
UrlEncodedParser.parse(query, this);
}
}
@Override
public int hashCode() {
// TODO(yanivi): optimize?
return build().hashCode();
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!super.equals(obj) || !(obj instanceof GenericUrl)) {
return false;
}
GenericUrl other = (GenericUrl) obj;
// TODO(yanivi): optimize?
return build().equals(other.toString());
}
@Override
public String toString() {
return build();
}
@Override
public GenericUrl clone() {
GenericUrl result = (GenericUrl) super.clone();
if (pathParts != null) {
result.pathParts = new ArrayList(pathParts);
}
return result;
}
/**
* Constructs the string representation of the URL, including the path specified by
* {@link #pathParts} and the query parameters specified by this generic URL.
*/
public final String build() {
// scheme, host, port, and path
StringBuilder buf = new StringBuilder();
buf.append(scheme).append("://").append(host);
int port = this.port;
if (port != -1) {
buf.append(':').append(port);
}
if (pathParts != null) {
appendRawPathFromParts(buf);
}
// query parameters (similar to UrlEncodedContent)
boolean first = true;
for (Map.Entry nameValueEntry : entrySet()) {
Object value = nameValueEntry.getValue();
if (value != null) {
String name = CharEscapers.escapeUriQuery(nameValueEntry.getKey());
if (value instanceof Collection>) {
Collection> collectionValue = (Collection>) value;
for (Object repeatedValue : collectionValue) {
first = appendParam(first, buf, name, repeatedValue);
}
} else {
first = appendParam(first, buf, name, value);
}
}
}
// URL fragment
String fragment = this.fragment;
if (fragment != null) {
buf.append('#').append(URI_FRAGMENT_ESCAPER.escape(fragment));
}
return buf.toString();
}
/**
* Returns the first query parameter value for the given query parameter name.
*
* @param name query parameter name
* @return first query parameter value
*/
public Object getFirst(String name) {
Object value = get(name);
if (value instanceof Collection>) {
@SuppressWarnings("unchecked")
Collection