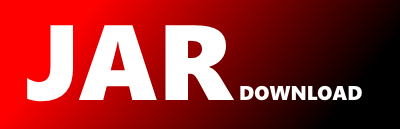
com.google.cloud.retail.v2.CompletionServiceGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grpc-google-cloud-retail-v2 Show documentation
Show all versions of grpc-google-cloud-retail-v2 Show documentation
GRPC library for google-cloud-retail
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.retail.v2;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
*
*
* Autocomplete service for retail.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler",
comments = "Source: google/cloud/retail/v2/completion_service.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class CompletionServiceGrpc {
private CompletionServiceGrpc() {}
public static final java.lang.String SERVICE_NAME = "google.cloud.retail.v2.CompletionService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor<
com.google.cloud.retail.v2.CompleteQueryRequest,
com.google.cloud.retail.v2.CompleteQueryResponse>
getCompleteQueryMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CompleteQuery",
requestType = com.google.cloud.retail.v2.CompleteQueryRequest.class,
responseType = com.google.cloud.retail.v2.CompleteQueryResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor<
com.google.cloud.retail.v2.CompleteQueryRequest,
com.google.cloud.retail.v2.CompleteQueryResponse>
getCompleteQueryMethod() {
io.grpc.MethodDescriptor<
com.google.cloud.retail.v2.CompleteQueryRequest,
com.google.cloud.retail.v2.CompleteQueryResponse>
getCompleteQueryMethod;
if ((getCompleteQueryMethod = CompletionServiceGrpc.getCompleteQueryMethod) == null) {
synchronized (CompletionServiceGrpc.class) {
if ((getCompleteQueryMethod = CompletionServiceGrpc.getCompleteQueryMethod) == null) {
CompletionServiceGrpc.getCompleteQueryMethod =
getCompleteQueryMethod =
io.grpc.MethodDescriptor
.
newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CompleteQuery"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(
io.grpc.protobuf.ProtoUtils.marshaller(
com.google.cloud.retail.v2.CompleteQueryRequest.getDefaultInstance()))
.setResponseMarshaller(
io.grpc.protobuf.ProtoUtils.marshaller(
com.google.cloud.retail.v2.CompleteQueryResponse
.getDefaultInstance()))
.setSchemaDescriptor(
new CompletionServiceMethodDescriptorSupplier("CompleteQuery"))
.build();
}
}
}
return getCompleteQueryMethod;
}
private static volatile io.grpc.MethodDescriptor<
com.google.cloud.retail.v2.ImportCompletionDataRequest, com.google.longrunning.Operation>
getImportCompletionDataMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ImportCompletionData",
requestType = com.google.cloud.retail.v2.ImportCompletionDataRequest.class,
responseType = com.google.longrunning.Operation.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor<
com.google.cloud.retail.v2.ImportCompletionDataRequest, com.google.longrunning.Operation>
getImportCompletionDataMethod() {
io.grpc.MethodDescriptor<
com.google.cloud.retail.v2.ImportCompletionDataRequest,
com.google.longrunning.Operation>
getImportCompletionDataMethod;
if ((getImportCompletionDataMethod = CompletionServiceGrpc.getImportCompletionDataMethod)
== null) {
synchronized (CompletionServiceGrpc.class) {
if ((getImportCompletionDataMethod = CompletionServiceGrpc.getImportCompletionDataMethod)
== null) {
CompletionServiceGrpc.getImportCompletionDataMethod =
getImportCompletionDataMethod =
io.grpc.MethodDescriptor
.
newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
generateFullMethodName(SERVICE_NAME, "ImportCompletionData"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(
io.grpc.protobuf.ProtoUtils.marshaller(
com.google.cloud.retail.v2.ImportCompletionDataRequest
.getDefaultInstance()))
.setResponseMarshaller(
io.grpc.protobuf.ProtoUtils.marshaller(
com.google.longrunning.Operation.getDefaultInstance()))
.setSchemaDescriptor(
new CompletionServiceMethodDescriptorSupplier("ImportCompletionData"))
.build();
}
}
}
return getImportCompletionDataMethod;
}
/** Creates a new async stub that supports all call types for the service */
public static CompletionServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public CompletionServiceStub newStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CompletionServiceStub(channel, callOptions);
}
};
return CompletionServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static CompletionServiceBlockingStub newBlockingStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public CompletionServiceBlockingStub newStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CompletionServiceBlockingStub(channel, callOptions);
}
};
return CompletionServiceBlockingStub.newStub(factory, channel);
}
/** Creates a new ListenableFuture-style stub that supports unary calls on the service */
public static CompletionServiceFutureStub newFutureStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public CompletionServiceFutureStub newStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CompletionServiceFutureStub(channel, callOptions);
}
};
return CompletionServiceFutureStub.newStub(factory, channel);
}
/**
*
*
*
* Autocomplete service for retail.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
public interface AsyncService {
/**
*
*
*
* Completes the specified prefix with keyword suggestions.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
default void completeQuery(
com.google.cloud.retail.v2.CompleteQueryRequest request,
io.grpc.stub.StreamObserver
responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(
getCompleteQueryMethod(), responseObserver);
}
/**
*
*
*
* Bulk import of processed completion dataset.
* Request processing is asynchronous. Partial updating is not supported.
* The operation is successfully finished only after the imported suggestions
* are indexed successfully and ready for serving. The process takes hours.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
default void importCompletionData(
com.google.cloud.retail.v2.ImportCompletionDataRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(
getImportCompletionDataMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service CompletionService.
*
*
* Autocomplete service for retail.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
public abstract static class CompletionServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override
public final io.grpc.ServerServiceDefinition bindService() {
return CompletionServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service CompletionService.
*
*
* Autocomplete service for retail.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
public static final class CompletionServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private CompletionServiceStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected CompletionServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CompletionServiceStub(channel, callOptions);
}
/**
*
*
*
* Completes the specified prefix with keyword suggestions.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
public void completeQuery(
com.google.cloud.retail.v2.CompleteQueryRequest request,
io.grpc.stub.StreamObserver
responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCompleteQueryMethod(), getCallOptions()),
request,
responseObserver);
}
/**
*
*
*
* Bulk import of processed completion dataset.
* Request processing is asynchronous. Partial updating is not supported.
* The operation is successfully finished only after the imported suggestions
* are indexed successfully and ready for serving. The process takes hours.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
public void importCompletionData(
com.google.cloud.retail.v2.ImportCompletionDataRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getImportCompletionDataMethod(), getCallOptions()),
request,
responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service CompletionService.
*
*
* Autocomplete service for retail.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
public static final class CompletionServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private CompletionServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected CompletionServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CompletionServiceBlockingStub(channel, callOptions);
}
/**
*
*
*
* Completes the specified prefix with keyword suggestions.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
public com.google.cloud.retail.v2.CompleteQueryResponse completeQuery(
com.google.cloud.retail.v2.CompleteQueryRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCompleteQueryMethod(), getCallOptions(), request);
}
/**
*
*
*
* Bulk import of processed completion dataset.
* Request processing is asynchronous. Partial updating is not supported.
* The operation is successfully finished only after the imported suggestions
* are indexed successfully and ready for serving. The process takes hours.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
public com.google.longrunning.Operation importCompletionData(
com.google.cloud.retail.v2.ImportCompletionDataRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getImportCompletionDataMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service CompletionService.
*
*
* Autocomplete service for retail.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
public static final class CompletionServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private CompletionServiceFutureStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected CompletionServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new CompletionServiceFutureStub(channel, callOptions);
}
/**
*
*
*
* Completes the specified prefix with keyword suggestions.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
public com.google.common.util.concurrent.ListenableFuture<
com.google.cloud.retail.v2.CompleteQueryResponse>
completeQuery(com.google.cloud.retail.v2.CompleteQueryRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCompleteQueryMethod(), getCallOptions()), request);
}
/**
*
*
*
* Bulk import of processed completion dataset.
* Request processing is asynchronous. Partial updating is not supported.
* The operation is successfully finished only after the imported suggestions
* are indexed successfully and ready for serving. The process takes hours.
* This feature is only available for users who have Retail Search enabled.
* Enable Retail Search on Cloud Console before using this feature.
*
*/
public com.google.common.util.concurrent.ListenableFuture
importCompletionData(com.google.cloud.retail.v2.ImportCompletionDataRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getImportCompletionDataMethod(), getCallOptions()), request);
}
}
private static final int METHODID_COMPLETE_QUERY = 0;
private static final int METHODID_IMPORT_COMPLETION_DATA = 1;
private static final class MethodHandlers
implements io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_COMPLETE_QUERY:
serviceImpl.completeQuery(
(com.google.cloud.retail.v2.CompleteQueryRequest) request,
(io.grpc.stub.StreamObserver)
responseObserver);
break;
case METHODID_IMPORT_COMPLETION_DATA:
serviceImpl.importCompletionData(
(com.google.cloud.retail.v2.ImportCompletionDataRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getCompleteQueryMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.cloud.retail.v2.CompleteQueryRequest,
com.google.cloud.retail.v2.CompleteQueryResponse>(
service, METHODID_COMPLETE_QUERY)))
.addMethod(
getImportCompletionDataMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.google.cloud.retail.v2.ImportCompletionDataRequest,
com.google.longrunning.Operation>(service, METHODID_IMPORT_COMPLETION_DATA)))
.build();
}
private abstract static class CompletionServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier,
io.grpc.protobuf.ProtoServiceDescriptorSupplier {
CompletionServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.google.cloud.retail.v2.CompletionServiceProto.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("CompletionService");
}
}
private static final class CompletionServiceFileDescriptorSupplier
extends CompletionServiceBaseDescriptorSupplier {
CompletionServiceFileDescriptorSupplier() {}
}
private static final class CompletionServiceMethodDescriptorSupplier
extends CompletionServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
CompletionServiceMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (CompletionServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor =
result =
io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new CompletionServiceFileDescriptorSupplier())
.addMethod(getCompleteQueryMethod())
.addMethod(getImportCompletionDataMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy