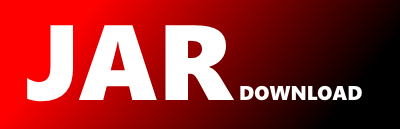
com.google.appengine.v1.AuthorizedCertificateOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-appengine-admin-v1 Show documentation
Show all versions of proto-google-cloud-appengine-admin-v1 Show documentation
Proto library for google-cloud-appengine-admin
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/appengine/v1/certificate.proto
// Protobuf Java Version: 3.25.5
package com.google.appengine.v1;
public interface AuthorizedCertificateOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.appengine.v1.AuthorizedCertificate)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Full path to the `AuthorizedCertificate` resource in the API. Example:
* `apps/myapp/authorizedCertificates/12345`.
*
* @OutputOnly
*
*
* string name = 1;
*
* @return The name.
*/
java.lang.String getName();
/**
*
*
*
* Full path to the `AuthorizedCertificate` resource in the API. Example:
* `apps/myapp/authorizedCertificates/12345`.
*
* @OutputOnly
*
*
* string name = 1;
*
* @return The bytes for name.
*/
com.google.protobuf.ByteString getNameBytes();
/**
*
*
*
* Relative name of the certificate. This is a unique value autogenerated
* on `AuthorizedCertificate` resource creation. Example: `12345`.
*
* @OutputOnly
*
*
* string id = 2;
*
* @return The id.
*/
java.lang.String getId();
/**
*
*
*
* Relative name of the certificate. This is a unique value autogenerated
* on `AuthorizedCertificate` resource creation. Example: `12345`.
*
* @OutputOnly
*
*
* string id = 2;
*
* @return The bytes for id.
*/
com.google.protobuf.ByteString getIdBytes();
/**
*
*
*
* The user-specified display name of the certificate. This is not
* guaranteed to be unique. Example: `My Certificate`.
*
*
* string display_name = 3;
*
* @return The displayName.
*/
java.lang.String getDisplayName();
/**
*
*
*
* The user-specified display name of the certificate. This is not
* guaranteed to be unique. Example: `My Certificate`.
*
*
* string display_name = 3;
*
* @return The bytes for displayName.
*/
com.google.protobuf.ByteString getDisplayNameBytes();
/**
*
*
*
* Topmost applicable domains of this certificate. This certificate
* applies to these domains and their subdomains. Example: `example.com`.
*
* @OutputOnly
*
*
* repeated string domain_names = 4;
*
* @return A list containing the domainNames.
*/
java.util.List getDomainNamesList();
/**
*
*
*
* Topmost applicable domains of this certificate. This certificate
* applies to these domains and their subdomains. Example: `example.com`.
*
* @OutputOnly
*
*
* repeated string domain_names = 4;
*
* @return The count of domainNames.
*/
int getDomainNamesCount();
/**
*
*
*
* Topmost applicable domains of this certificate. This certificate
* applies to these domains and their subdomains. Example: `example.com`.
*
* @OutputOnly
*
*
* repeated string domain_names = 4;
*
* @param index The index of the element to return.
* @return The domainNames at the given index.
*/
java.lang.String getDomainNames(int index);
/**
*
*
*
* Topmost applicable domains of this certificate. This certificate
* applies to these domains and their subdomains. Example: `example.com`.
*
* @OutputOnly
*
*
* repeated string domain_names = 4;
*
* @param index The index of the value to return.
* @return The bytes of the domainNames at the given index.
*/
com.google.protobuf.ByteString getDomainNamesBytes(int index);
/**
*
*
*
* The time when this certificate expires. To update the renewal time on this
* certificate, upload an SSL certificate with a different expiration time
* using [`AuthorizedCertificates.UpdateAuthorizedCertificate`]().
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp expire_time = 5;
*
* @return Whether the expireTime field is set.
*/
boolean hasExpireTime();
/**
*
*
*
* The time when this certificate expires. To update the renewal time on this
* certificate, upload an SSL certificate with a different expiration time
* using [`AuthorizedCertificates.UpdateAuthorizedCertificate`]().
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp expire_time = 5;
*
* @return The expireTime.
*/
com.google.protobuf.Timestamp getExpireTime();
/**
*
*
*
* The time when this certificate expires. To update the renewal time on this
* certificate, upload an SSL certificate with a different expiration time
* using [`AuthorizedCertificates.UpdateAuthorizedCertificate`]().
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp expire_time = 5;
*/
com.google.protobuf.TimestampOrBuilder getExpireTimeOrBuilder();
/**
*
*
*
* The SSL certificate serving the `AuthorizedCertificate` resource. This
* must be obtained independently from a certificate authority.
*
*
* .google.appengine.v1.CertificateRawData certificate_raw_data = 6;
*
* @return Whether the certificateRawData field is set.
*/
boolean hasCertificateRawData();
/**
*
*
*
* The SSL certificate serving the `AuthorizedCertificate` resource. This
* must be obtained independently from a certificate authority.
*
*
* .google.appengine.v1.CertificateRawData certificate_raw_data = 6;
*
* @return The certificateRawData.
*/
com.google.appengine.v1.CertificateRawData getCertificateRawData();
/**
*
*
*
* The SSL certificate serving the `AuthorizedCertificate` resource. This
* must be obtained independently from a certificate authority.
*
*
* .google.appengine.v1.CertificateRawData certificate_raw_data = 6;
*/
com.google.appengine.v1.CertificateRawDataOrBuilder getCertificateRawDataOrBuilder();
/**
*
*
*
* Only applicable if this certificate is managed by App Engine. Managed
* certificates are tied to the lifecycle of a `DomainMapping` and cannot be
* updated or deleted via the `AuthorizedCertificates` API. If this
* certificate is manually administered by the user, this field will be empty.
*
* @OutputOnly
*
*
* .google.appengine.v1.ManagedCertificate managed_certificate = 7;
*
* @return Whether the managedCertificate field is set.
*/
boolean hasManagedCertificate();
/**
*
*
*
* Only applicable if this certificate is managed by App Engine. Managed
* certificates are tied to the lifecycle of a `DomainMapping` and cannot be
* updated or deleted via the `AuthorizedCertificates` API. If this
* certificate is manually administered by the user, this field will be empty.
*
* @OutputOnly
*
*
* .google.appengine.v1.ManagedCertificate managed_certificate = 7;
*
* @return The managedCertificate.
*/
com.google.appengine.v1.ManagedCertificate getManagedCertificate();
/**
*
*
*
* Only applicable if this certificate is managed by App Engine. Managed
* certificates are tied to the lifecycle of a `DomainMapping` and cannot be
* updated or deleted via the `AuthorizedCertificates` API. If this
* certificate is manually administered by the user, this field will be empty.
*
* @OutputOnly
*
*
* .google.appengine.v1.ManagedCertificate managed_certificate = 7;
*/
com.google.appengine.v1.ManagedCertificateOrBuilder getManagedCertificateOrBuilder();
/**
*
*
*
* The full paths to user visible Domain Mapping resources that have this
* certificate mapped. Example: `apps/myapp/domainMappings/example.com`.
*
* This may not represent the full list of mapped domain mappings if the user
* does not have `VIEWER` permissions on all of the applications that have
* this certificate mapped. See `domain_mappings_count` for a complete count.
*
* Only returned by `GET` or `LIST` requests when specifically requested by
* the `view=FULL_CERTIFICATE` option.
*
* @OutputOnly
*
*
* repeated string visible_domain_mappings = 8;
*
* @return A list containing the visibleDomainMappings.
*/
java.util.List getVisibleDomainMappingsList();
/**
*
*
*
* The full paths to user visible Domain Mapping resources that have this
* certificate mapped. Example: `apps/myapp/domainMappings/example.com`.
*
* This may not represent the full list of mapped domain mappings if the user
* does not have `VIEWER` permissions on all of the applications that have
* this certificate mapped. See `domain_mappings_count` for a complete count.
*
* Only returned by `GET` or `LIST` requests when specifically requested by
* the `view=FULL_CERTIFICATE` option.
*
* @OutputOnly
*
*
* repeated string visible_domain_mappings = 8;
*
* @return The count of visibleDomainMappings.
*/
int getVisibleDomainMappingsCount();
/**
*
*
*
* The full paths to user visible Domain Mapping resources that have this
* certificate mapped. Example: `apps/myapp/domainMappings/example.com`.
*
* This may not represent the full list of mapped domain mappings if the user
* does not have `VIEWER` permissions on all of the applications that have
* this certificate mapped. See `domain_mappings_count` for a complete count.
*
* Only returned by `GET` or `LIST` requests when specifically requested by
* the `view=FULL_CERTIFICATE` option.
*
* @OutputOnly
*
*
* repeated string visible_domain_mappings = 8;
*
* @param index The index of the element to return.
* @return The visibleDomainMappings at the given index.
*/
java.lang.String getVisibleDomainMappings(int index);
/**
*
*
*
* The full paths to user visible Domain Mapping resources that have this
* certificate mapped. Example: `apps/myapp/domainMappings/example.com`.
*
* This may not represent the full list of mapped domain mappings if the user
* does not have `VIEWER` permissions on all of the applications that have
* this certificate mapped. See `domain_mappings_count` for a complete count.
*
* Only returned by `GET` or `LIST` requests when specifically requested by
* the `view=FULL_CERTIFICATE` option.
*
* @OutputOnly
*
*
* repeated string visible_domain_mappings = 8;
*
* @param index The index of the value to return.
* @return The bytes of the visibleDomainMappings at the given index.
*/
com.google.protobuf.ByteString getVisibleDomainMappingsBytes(int index);
/**
*
*
*
* Aggregate count of the domain mappings with this certificate mapped. This
* count includes domain mappings on applications for which the user does not
* have `VIEWER` permissions.
*
* Only returned by `GET` or `LIST` requests when specifically requested by
* the `view=FULL_CERTIFICATE` option.
*
* @OutputOnly
*
*
* int32 domain_mappings_count = 9;
*
* @return The domainMappingsCount.
*/
int getDomainMappingsCount();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy