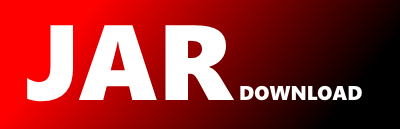
com.google.appengine.v1.AutomaticScalingOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-appengine-admin-v1 Show documentation
Show all versions of proto-google-cloud-appengine-admin-v1 Show documentation
Proto library for google-cloud-appengine-admin
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/appengine/v1/version.proto
// Protobuf Java Version: 3.25.5
package com.google.appengine.v1;
public interface AutomaticScalingOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.appengine.v1.AutomaticScaling)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* The time period that the
* [Autoscaler](https://cloud.google.com/compute/docs/autoscaler/)
* should wait before it starts collecting information from a new instance.
* This prevents the autoscaler from collecting information when the instance
* is initializing, during which the collected usage would not be reliable.
* Only applicable in the App Engine flexible environment.
*
*
* .google.protobuf.Duration cool_down_period = 1;
*
* @return Whether the coolDownPeriod field is set.
*/
boolean hasCoolDownPeriod();
/**
*
*
*
* The time period that the
* [Autoscaler](https://cloud.google.com/compute/docs/autoscaler/)
* should wait before it starts collecting information from a new instance.
* This prevents the autoscaler from collecting information when the instance
* is initializing, during which the collected usage would not be reliable.
* Only applicable in the App Engine flexible environment.
*
*
* .google.protobuf.Duration cool_down_period = 1;
*
* @return The coolDownPeriod.
*/
com.google.protobuf.Duration getCoolDownPeriod();
/**
*
*
*
* The time period that the
* [Autoscaler](https://cloud.google.com/compute/docs/autoscaler/)
* should wait before it starts collecting information from a new instance.
* This prevents the autoscaler from collecting information when the instance
* is initializing, during which the collected usage would not be reliable.
* Only applicable in the App Engine flexible environment.
*
*
* .google.protobuf.Duration cool_down_period = 1;
*/
com.google.protobuf.DurationOrBuilder getCoolDownPeriodOrBuilder();
/**
*
*
*
* Target scaling by CPU usage.
*
*
* .google.appengine.v1.CpuUtilization cpu_utilization = 2;
*
* @return Whether the cpuUtilization field is set.
*/
boolean hasCpuUtilization();
/**
*
*
*
* Target scaling by CPU usage.
*
*
* .google.appengine.v1.CpuUtilization cpu_utilization = 2;
*
* @return The cpuUtilization.
*/
com.google.appengine.v1.CpuUtilization getCpuUtilization();
/**
*
*
*
* Target scaling by CPU usage.
*
*
* .google.appengine.v1.CpuUtilization cpu_utilization = 2;
*/
com.google.appengine.v1.CpuUtilizationOrBuilder getCpuUtilizationOrBuilder();
/**
*
*
*
* Number of concurrent requests an automatic scaling instance can accept
* before the scheduler spawns a new instance.
*
* Defaults to a runtime-specific value.
*
*
* int32 max_concurrent_requests = 3;
*
* @return The maxConcurrentRequests.
*/
int getMaxConcurrentRequests();
/**
*
*
*
* Maximum number of idle instances that should be maintained for this
* version.
*
*
* int32 max_idle_instances = 4;
*
* @return The maxIdleInstances.
*/
int getMaxIdleInstances();
/**
*
*
*
* Maximum number of instances that should be started to handle requests for
* this version.
*
*
* int32 max_total_instances = 5;
*
* @return The maxTotalInstances.
*/
int getMaxTotalInstances();
/**
*
*
*
* Maximum amount of time that a request should wait in the pending queue
* before starting a new instance to handle it.
*
*
* .google.protobuf.Duration max_pending_latency = 6;
*
* @return Whether the maxPendingLatency field is set.
*/
boolean hasMaxPendingLatency();
/**
*
*
*
* Maximum amount of time that a request should wait in the pending queue
* before starting a new instance to handle it.
*
*
* .google.protobuf.Duration max_pending_latency = 6;
*
* @return The maxPendingLatency.
*/
com.google.protobuf.Duration getMaxPendingLatency();
/**
*
*
*
* Maximum amount of time that a request should wait in the pending queue
* before starting a new instance to handle it.
*
*
* .google.protobuf.Duration max_pending_latency = 6;
*/
com.google.protobuf.DurationOrBuilder getMaxPendingLatencyOrBuilder();
/**
*
*
*
* Minimum number of idle instances that should be maintained for
* this version. Only applicable for the default version of a service.
*
*
* int32 min_idle_instances = 7;
*
* @return The minIdleInstances.
*/
int getMinIdleInstances();
/**
*
*
*
* Minimum number of running instances that should be maintained for this
* version.
*
*
* int32 min_total_instances = 8;
*
* @return The minTotalInstances.
*/
int getMinTotalInstances();
/**
*
*
*
* Minimum amount of time a request should wait in the pending queue before
* starting a new instance to handle it.
*
*
* .google.protobuf.Duration min_pending_latency = 9;
*
* @return Whether the minPendingLatency field is set.
*/
boolean hasMinPendingLatency();
/**
*
*
*
* Minimum amount of time a request should wait in the pending queue before
* starting a new instance to handle it.
*
*
* .google.protobuf.Duration min_pending_latency = 9;
*
* @return The minPendingLatency.
*/
com.google.protobuf.Duration getMinPendingLatency();
/**
*
*
*
* Minimum amount of time a request should wait in the pending queue before
* starting a new instance to handle it.
*
*
* .google.protobuf.Duration min_pending_latency = 9;
*/
com.google.protobuf.DurationOrBuilder getMinPendingLatencyOrBuilder();
/**
*
*
*
* Target scaling by request utilization.
*
*
* .google.appengine.v1.RequestUtilization request_utilization = 10;
*
* @return Whether the requestUtilization field is set.
*/
boolean hasRequestUtilization();
/**
*
*
*
* Target scaling by request utilization.
*
*
* .google.appengine.v1.RequestUtilization request_utilization = 10;
*
* @return The requestUtilization.
*/
com.google.appengine.v1.RequestUtilization getRequestUtilization();
/**
*
*
*
* Target scaling by request utilization.
*
*
* .google.appengine.v1.RequestUtilization request_utilization = 10;
*/
com.google.appengine.v1.RequestUtilizationOrBuilder getRequestUtilizationOrBuilder();
/**
*
*
*
* Target scaling by disk usage.
*
*
* .google.appengine.v1.DiskUtilization disk_utilization = 11;
*
* @return Whether the diskUtilization field is set.
*/
boolean hasDiskUtilization();
/**
*
*
*
* Target scaling by disk usage.
*
*
* .google.appengine.v1.DiskUtilization disk_utilization = 11;
*
* @return The diskUtilization.
*/
com.google.appengine.v1.DiskUtilization getDiskUtilization();
/**
*
*
*
* Target scaling by disk usage.
*
*
* .google.appengine.v1.DiskUtilization disk_utilization = 11;
*/
com.google.appengine.v1.DiskUtilizationOrBuilder getDiskUtilizationOrBuilder();
/**
*
*
*
* Target scaling by network usage.
*
*
* .google.appengine.v1.NetworkUtilization network_utilization = 12;
*
* @return Whether the networkUtilization field is set.
*/
boolean hasNetworkUtilization();
/**
*
*
*
* Target scaling by network usage.
*
*
* .google.appengine.v1.NetworkUtilization network_utilization = 12;
*
* @return The networkUtilization.
*/
com.google.appengine.v1.NetworkUtilization getNetworkUtilization();
/**
*
*
*
* Target scaling by network usage.
*
*
* .google.appengine.v1.NetworkUtilization network_utilization = 12;
*/
com.google.appengine.v1.NetworkUtilizationOrBuilder getNetworkUtilizationOrBuilder();
/**
*
*
*
* Scheduler settings for standard environment.
*
*
* .google.appengine.v1.StandardSchedulerSettings standard_scheduler_settings = 20;
*
* @return Whether the standardSchedulerSettings field is set.
*/
boolean hasStandardSchedulerSettings();
/**
*
*
*
* Scheduler settings for standard environment.
*
*
* .google.appengine.v1.StandardSchedulerSettings standard_scheduler_settings = 20;
*
* @return The standardSchedulerSettings.
*/
com.google.appengine.v1.StandardSchedulerSettings getStandardSchedulerSettings();
/**
*
*
*
* Scheduler settings for standard environment.
*
*
* .google.appengine.v1.StandardSchedulerSettings standard_scheduler_settings = 20;
*/
com.google.appengine.v1.StandardSchedulerSettingsOrBuilder
getStandardSchedulerSettingsOrBuilder();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy