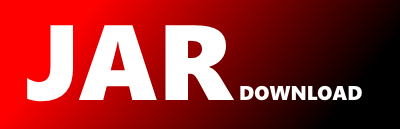
com.google.appengine.v1.Version Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-appengine-admin-v1 Show documentation
Show all versions of proto-google-cloud-appengine-admin-v1 Show documentation
Proto library for google-cloud-appengine-admin
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/appengine/v1/version.proto
// Protobuf Java Version: 3.25.5
package com.google.appengine.v1;
/**
*
*
*
* A Version resource is a specific set of source code and configuration files
* that are deployed into a service.
*
*
* Protobuf type {@code google.appengine.v1.Version}
*/
public final class Version extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.appengine.v1.Version)
VersionOrBuilder {
private static final long serialVersionUID = 0L;
// Use Version.newBuilder() to construct.
private Version(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Version() {
name_ = "";
id_ = "";
inboundServices_ = java.util.Collections.emptyList();
instanceClass_ = "";
zones_ = com.google.protobuf.LazyStringArrayList.emptyList();
runtime_ = "";
runtimeChannel_ = "";
env_ = "";
servingStatus_ = 0;
createdBy_ = "";
runtimeApiVersion_ = "";
runtimeMainExecutablePath_ = "";
serviceAccount_ = "";
handlers_ = java.util.Collections.emptyList();
errorHandlers_ = java.util.Collections.emptyList();
libraries_ = java.util.Collections.emptyList();
nobuildFilesRegex_ = "";
versionUrl_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Version();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.appengine.v1.VersionProto
.internal_static_google_appengine_v1_Version_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 13:
return internalGetBetaSettings();
case 104:
return internalGetEnvVariables();
case 125:
return internalGetBuildEnvVariables();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.v1.VersionProto
.internal_static_google_appengine_v1_Version_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.v1.Version.class, com.google.appengine.v1.Version.Builder.class);
}
private int bitField0_;
private int scalingCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object scaling_;
public enum ScalingCase
implements
com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
AUTOMATIC_SCALING(3),
BASIC_SCALING(4),
MANUAL_SCALING(5),
SCALING_NOT_SET(0);
private final int value;
private ScalingCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ScalingCase valueOf(int value) {
return forNumber(value);
}
public static ScalingCase forNumber(int value) {
switch (value) {
case 3:
return AUTOMATIC_SCALING;
case 4:
return BASIC_SCALING;
case 5:
return MANUAL_SCALING;
case 0:
return SCALING_NOT_SET;
default:
return null;
}
}
public int getNumber() {
return this.value;
}
};
public ScalingCase getScalingCase() {
return ScalingCase.forNumber(scalingCase_);
}
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* Full path to the Version resource in the API. Example:
* `apps/myapp/services/default/versions/v1`.
*
* @OutputOnly
*
*
* string name = 1;
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* Full path to the Version resource in the API. Example:
* `apps/myapp/services/default/versions/v1`.
*
* @OutputOnly
*
*
* string name = 1;
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object id_ = "";
/**
*
*
*
* Relative name of the version within the service. Example: `v1`.
* Version names can contain only lowercase letters, numbers, or hyphens.
* Reserved names: "default", "latest", and any name with the prefix "ah-".
*
*
* string id = 2;
*
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
*
*
* Relative name of the version within the service. Example: `v1`.
* Version names can contain only lowercase letters, numbers, or hyphens.
* Reserved names: "default", "latest", and any name with the prefix "ah-".
*
*
* string id = 2;
*
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AUTOMATIC_SCALING_FIELD_NUMBER = 3;
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*
* @return Whether the automaticScaling field is set.
*/
@java.lang.Override
public boolean hasAutomaticScaling() {
return scalingCase_ == 3;
}
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*
* @return The automaticScaling.
*/
@java.lang.Override
public com.google.appengine.v1.AutomaticScaling getAutomaticScaling() {
if (scalingCase_ == 3) {
return (com.google.appengine.v1.AutomaticScaling) scaling_;
}
return com.google.appengine.v1.AutomaticScaling.getDefaultInstance();
}
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*/
@java.lang.Override
public com.google.appengine.v1.AutomaticScalingOrBuilder getAutomaticScalingOrBuilder() {
if (scalingCase_ == 3) {
return (com.google.appengine.v1.AutomaticScaling) scaling_;
}
return com.google.appengine.v1.AutomaticScaling.getDefaultInstance();
}
public static final int BASIC_SCALING_FIELD_NUMBER = 4;
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*
* @return Whether the basicScaling field is set.
*/
@java.lang.Override
public boolean hasBasicScaling() {
return scalingCase_ == 4;
}
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*
* @return The basicScaling.
*/
@java.lang.Override
public com.google.appengine.v1.BasicScaling getBasicScaling() {
if (scalingCase_ == 4) {
return (com.google.appengine.v1.BasicScaling) scaling_;
}
return com.google.appengine.v1.BasicScaling.getDefaultInstance();
}
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*/
@java.lang.Override
public com.google.appengine.v1.BasicScalingOrBuilder getBasicScalingOrBuilder() {
if (scalingCase_ == 4) {
return (com.google.appengine.v1.BasicScaling) scaling_;
}
return com.google.appengine.v1.BasicScaling.getDefaultInstance();
}
public static final int MANUAL_SCALING_FIELD_NUMBER = 5;
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*
* @return Whether the manualScaling field is set.
*/
@java.lang.Override
public boolean hasManualScaling() {
return scalingCase_ == 5;
}
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*
* @return The manualScaling.
*/
@java.lang.Override
public com.google.appengine.v1.ManualScaling getManualScaling() {
if (scalingCase_ == 5) {
return (com.google.appengine.v1.ManualScaling) scaling_;
}
return com.google.appengine.v1.ManualScaling.getDefaultInstance();
}
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*/
@java.lang.Override
public com.google.appengine.v1.ManualScalingOrBuilder getManualScalingOrBuilder() {
if (scalingCase_ == 5) {
return (com.google.appengine.v1.ManualScaling) scaling_;
}
return com.google.appengine.v1.ManualScaling.getDefaultInstance();
}
public static final int INBOUND_SERVICES_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private java.util.List inboundServices_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.appengine.v1.InboundServiceType>
inboundServices_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.appengine.v1.InboundServiceType>() {
public com.google.appengine.v1.InboundServiceType convert(java.lang.Integer from) {
com.google.appengine.v1.InboundServiceType result =
com.google.appengine.v1.InboundServiceType.forNumber(from);
return result == null
? com.google.appengine.v1.InboundServiceType.UNRECOGNIZED
: result;
}
};
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @return A list containing the inboundServices.
*/
@java.lang.Override
public java.util.List getInboundServicesList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.appengine.v1.InboundServiceType>(
inboundServices_, inboundServices_converter_);
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @return The count of inboundServices.
*/
@java.lang.Override
public int getInboundServicesCount() {
return inboundServices_.size();
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @param index The index of the element to return.
* @return The inboundServices at the given index.
*/
@java.lang.Override
public com.google.appengine.v1.InboundServiceType getInboundServices(int index) {
return inboundServices_converter_.convert(inboundServices_.get(index));
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @return A list containing the enum numeric values on the wire for inboundServices.
*/
@java.lang.Override
public java.util.List getInboundServicesValueList() {
return inboundServices_;
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @param index The index of the value to return.
* @return The enum numeric value on the wire of inboundServices at the given index.
*/
@java.lang.Override
public int getInboundServicesValue(int index) {
return inboundServices_.get(index);
}
private int inboundServicesMemoizedSerializedSize;
public static final int INSTANCE_CLASS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object instanceClass_ = "";
/**
*
*
*
* Instance class that is used to run this version. Valid values are:
*
* * AutomaticScaling: `F1`, `F2`, `F4`, `F4_1G`
* * ManualScaling or BasicScaling: `B1`, `B2`, `B4`, `B8`, `B4_1G`
*
* Defaults to `F1` for AutomaticScaling and `B1` for ManualScaling or
* BasicScaling.
*
*
* string instance_class = 7;
*
* @return The instanceClass.
*/
@java.lang.Override
public java.lang.String getInstanceClass() {
java.lang.Object ref = instanceClass_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
instanceClass_ = s;
return s;
}
}
/**
*
*
*
* Instance class that is used to run this version. Valid values are:
*
* * AutomaticScaling: `F1`, `F2`, `F4`, `F4_1G`
* * ManualScaling or BasicScaling: `B1`, `B2`, `B4`, `B8`, `B4_1G`
*
* Defaults to `F1` for AutomaticScaling and `B1` for ManualScaling or
* BasicScaling.
*
*
* string instance_class = 7;
*
* @return The bytes for instanceClass.
*/
@java.lang.Override
public com.google.protobuf.ByteString getInstanceClassBytes() {
java.lang.Object ref = instanceClass_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
instanceClass_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NETWORK_FIELD_NUMBER = 8;
private com.google.appengine.v1.Network network_;
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*
* @return Whether the network field is set.
*/
@java.lang.Override
public boolean hasNetwork() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*
* @return The network.
*/
@java.lang.Override
public com.google.appengine.v1.Network getNetwork() {
return network_ == null ? com.google.appengine.v1.Network.getDefaultInstance() : network_;
}
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*/
@java.lang.Override
public com.google.appengine.v1.NetworkOrBuilder getNetworkOrBuilder() {
return network_ == null ? com.google.appengine.v1.Network.getDefaultInstance() : network_;
}
public static final int ZONES_FIELD_NUMBER = 118;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList zones_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @return A list containing the zones.
*/
public com.google.protobuf.ProtocolStringList getZonesList() {
return zones_;
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @return The count of zones.
*/
public int getZonesCount() {
return zones_.size();
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @param index The index of the element to return.
* @return The zones at the given index.
*/
public java.lang.String getZones(int index) {
return zones_.get(index);
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @param index The index of the value to return.
* @return The bytes of the zones at the given index.
*/
public com.google.protobuf.ByteString getZonesBytes(int index) {
return zones_.getByteString(index);
}
public static final int RESOURCES_FIELD_NUMBER = 9;
private com.google.appengine.v1.Resources resources_;
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*
* @return Whether the resources field is set.
*/
@java.lang.Override
public boolean hasResources() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*
* @return The resources.
*/
@java.lang.Override
public com.google.appengine.v1.Resources getResources() {
return resources_ == null ? com.google.appengine.v1.Resources.getDefaultInstance() : resources_;
}
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*/
@java.lang.Override
public com.google.appengine.v1.ResourcesOrBuilder getResourcesOrBuilder() {
return resources_ == null ? com.google.appengine.v1.Resources.getDefaultInstance() : resources_;
}
public static final int RUNTIME_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private volatile java.lang.Object runtime_ = "";
/**
*
*
*
* Desired runtime. Example: `python27`.
*
*
* string runtime = 10;
*
* @return The runtime.
*/
@java.lang.Override
public java.lang.String getRuntime() {
java.lang.Object ref = runtime_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runtime_ = s;
return s;
}
}
/**
*
*
*
* Desired runtime. Example: `python27`.
*
*
* string runtime = 10;
*
* @return The bytes for runtime.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRuntimeBytes() {
java.lang.Object ref = runtime_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
runtime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RUNTIME_CHANNEL_FIELD_NUMBER = 117;
@SuppressWarnings("serial")
private volatile java.lang.Object runtimeChannel_ = "";
/**
*
*
*
* The channel of the runtime to use. Only available for some
* runtimes. Defaults to the `default` channel.
*
*
* string runtime_channel = 117;
*
* @return The runtimeChannel.
*/
@java.lang.Override
public java.lang.String getRuntimeChannel() {
java.lang.Object ref = runtimeChannel_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runtimeChannel_ = s;
return s;
}
}
/**
*
*
*
* The channel of the runtime to use. Only available for some
* runtimes. Defaults to the `default` channel.
*
*
* string runtime_channel = 117;
*
* @return The bytes for runtimeChannel.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRuntimeChannelBytes() {
java.lang.Object ref = runtimeChannel_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
runtimeChannel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int THREADSAFE_FIELD_NUMBER = 11;
private boolean threadsafe_ = false;
/**
*
*
*
* Whether multiple requests can be dispatched to this version at once.
*
*
* bool threadsafe = 11;
*
* @return The threadsafe.
*/
@java.lang.Override
public boolean getThreadsafe() {
return threadsafe_;
}
public static final int VM_FIELD_NUMBER = 12;
private boolean vm_ = false;
/**
*
*
*
* Whether to deploy this version in a container on a virtual machine.
*
*
* bool vm = 12;
*
* @return The vm.
*/
@java.lang.Override
public boolean getVm() {
return vm_;
}
public static final int APP_ENGINE_APIS_FIELD_NUMBER = 128;
private boolean appEngineApis_ = false;
/**
*
*
*
* Allows App Engine second generation runtimes to access the legacy bundled
* services.
*
*
* bool app_engine_apis = 128;
*
* @return The appEngineApis.
*/
@java.lang.Override
public boolean getAppEngineApis() {
return appEngineApis_;
}
public static final int BETA_SETTINGS_FIELD_NUMBER = 13;
private static final class BetaSettingsDefaultEntryHolder {
static final com.google.protobuf.MapEntry defaultEntry =
com.google.protobuf.MapEntry.newDefaultInstance(
com.google.appengine.v1.VersionProto
.internal_static_google_appengine_v1_Version_BetaSettingsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField betaSettings_;
private com.google.protobuf.MapField
internalGetBetaSettings() {
if (betaSettings_ == null) {
return com.google.protobuf.MapField.emptyMapField(
BetaSettingsDefaultEntryHolder.defaultEntry);
}
return betaSettings_;
}
public int getBetaSettingsCount() {
return internalGetBetaSettings().getMap().size();
}
/**
*
*
*
* Metadata settings that are supplied to this version to enable
* beta runtime features.
*
*
* map<string, string> beta_settings = 13;
*/
@java.lang.Override
public boolean containsBetaSettings(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetBetaSettings().getMap().containsKey(key);
}
/** Use {@link #getBetaSettingsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getBetaSettings() {
return getBetaSettingsMap();
}
/**
*
*
*
* Metadata settings that are supplied to this version to enable
* beta runtime features.
*
*
* map<string, string> beta_settings = 13;
*/
@java.lang.Override
public java.util.Map getBetaSettingsMap() {
return internalGetBetaSettings().getMap();
}
/**
*
*
*
* Metadata settings that are supplied to this version to enable
* beta runtime features.
*
*
* map<string, string> beta_settings = 13;
*/
@java.lang.Override
public /* nullable */ java.lang.String getBetaSettingsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetBetaSettings().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Metadata settings that are supplied to this version to enable
* beta runtime features.
*
*
* map<string, string> beta_settings = 13;
*/
@java.lang.Override
public java.lang.String getBetaSettingsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetBetaSettings().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int ENV_FIELD_NUMBER = 14;
@SuppressWarnings("serial")
private volatile java.lang.Object env_ = "";
/**
*
*
*
* App Engine execution environment for this version.
*
* Defaults to `standard`.
*
*
* string env = 14;
*
* @return The env.
*/
@java.lang.Override
public java.lang.String getEnv() {
java.lang.Object ref = env_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
env_ = s;
return s;
}
}
/**
*
*
*
* App Engine execution environment for this version.
*
* Defaults to `standard`.
*
*
* string env = 14;
*
* @return The bytes for env.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEnvBytes() {
java.lang.Object ref = env_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
env_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERVING_STATUS_FIELD_NUMBER = 15;
private int servingStatus_ = 0;
/**
*
*
*
* Current serving status of this version. Only the versions with a
* `SERVING` status create instances and can be billed.
*
* `SERVING_STATUS_UNSPECIFIED` is an invalid value. Defaults to `SERVING`.
*
*
* .google.appengine.v1.ServingStatus serving_status = 15;
*
* @return The enum numeric value on the wire for servingStatus.
*/
@java.lang.Override
public int getServingStatusValue() {
return servingStatus_;
}
/**
*
*
*
* Current serving status of this version. Only the versions with a
* `SERVING` status create instances and can be billed.
*
* `SERVING_STATUS_UNSPECIFIED` is an invalid value. Defaults to `SERVING`.
*
*
* .google.appengine.v1.ServingStatus serving_status = 15;
*
* @return The servingStatus.
*/
@java.lang.Override
public com.google.appengine.v1.ServingStatus getServingStatus() {
com.google.appengine.v1.ServingStatus result =
com.google.appengine.v1.ServingStatus.forNumber(servingStatus_);
return result == null ? com.google.appengine.v1.ServingStatus.UNRECOGNIZED : result;
}
public static final int CREATED_BY_FIELD_NUMBER = 16;
@SuppressWarnings("serial")
private volatile java.lang.Object createdBy_ = "";
/**
*
*
*
* Email address of the user who created this version.
*
* @OutputOnly
*
*
* string created_by = 16;
*
* @return The createdBy.
*/
@java.lang.Override
public java.lang.String getCreatedBy() {
java.lang.Object ref = createdBy_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
createdBy_ = s;
return s;
}
}
/**
*
*
*
* Email address of the user who created this version.
*
* @OutputOnly
*
*
* string created_by = 16;
*
* @return The bytes for createdBy.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCreatedByBytes() {
java.lang.Object ref = createdBy_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
createdBy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CREATE_TIME_FIELD_NUMBER = 17;
private com.google.protobuf.Timestamp createTime_;
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*
* @return Whether the createTime field is set.
*/
@java.lang.Override
public boolean hasCreateTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*
* @return The createTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getCreateTime() {
return createTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : createTime_;
}
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder() {
return createTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : createTime_;
}
public static final int DISK_USAGE_BYTES_FIELD_NUMBER = 18;
private long diskUsageBytes_ = 0L;
/**
*
*
*
* Total size in bytes of all the files that are included in this version
* and currently hosted on the App Engine disk.
*
* @OutputOnly
*
*
* int64 disk_usage_bytes = 18;
*
* @return The diskUsageBytes.
*/
@java.lang.Override
public long getDiskUsageBytes() {
return diskUsageBytes_;
}
public static final int RUNTIME_API_VERSION_FIELD_NUMBER = 21;
@SuppressWarnings("serial")
private volatile java.lang.Object runtimeApiVersion_ = "";
/**
*
*
*
* The version of the API in the given runtime environment. Please see the
* app.yaml reference for valid values at
* https://cloud.google.com/appengine/docs/standard/<language>/config/appref
*
*
* string runtime_api_version = 21;
*
* @return The runtimeApiVersion.
*/
@java.lang.Override
public java.lang.String getRuntimeApiVersion() {
java.lang.Object ref = runtimeApiVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runtimeApiVersion_ = s;
return s;
}
}
/**
*
*
*
* The version of the API in the given runtime environment. Please see the
* app.yaml reference for valid values at
* https://cloud.google.com/appengine/docs/standard/<language>/config/appref
*
*
* string runtime_api_version = 21;
*
* @return The bytes for runtimeApiVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRuntimeApiVersionBytes() {
java.lang.Object ref = runtimeApiVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
runtimeApiVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RUNTIME_MAIN_EXECUTABLE_PATH_FIELD_NUMBER = 22;
@SuppressWarnings("serial")
private volatile java.lang.Object runtimeMainExecutablePath_ = "";
/**
*
*
*
* The path or name of the app's main executable.
*
*
* string runtime_main_executable_path = 22;
*
* @return The runtimeMainExecutablePath.
*/
@java.lang.Override
public java.lang.String getRuntimeMainExecutablePath() {
java.lang.Object ref = runtimeMainExecutablePath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runtimeMainExecutablePath_ = s;
return s;
}
}
/**
*
*
*
* The path or name of the app's main executable.
*
*
* string runtime_main_executable_path = 22;
*
* @return The bytes for runtimeMainExecutablePath.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRuntimeMainExecutablePathBytes() {
java.lang.Object ref = runtimeMainExecutablePath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
runtimeMainExecutablePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERVICE_ACCOUNT_FIELD_NUMBER = 127;
@SuppressWarnings("serial")
private volatile java.lang.Object serviceAccount_ = "";
/**
*
*
*
* The identity that the deployed version will run as.
* Admin API will use the App Engine Appspot service account as default if
* this field is neither provided in app.yaml file nor through CLI flag.
*
*
* string service_account = 127;
*
* @return The serviceAccount.
*/
@java.lang.Override
public java.lang.String getServiceAccount() {
java.lang.Object ref = serviceAccount_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serviceAccount_ = s;
return s;
}
}
/**
*
*
*
* The identity that the deployed version will run as.
* Admin API will use the App Engine Appspot service account as default if
* this field is neither provided in app.yaml file nor through CLI flag.
*
*
* string service_account = 127;
*
* @return The bytes for serviceAccount.
*/
@java.lang.Override
public com.google.protobuf.ByteString getServiceAccountBytes() {
java.lang.Object ref = serviceAccount_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
serviceAccount_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HANDLERS_FIELD_NUMBER = 100;
@SuppressWarnings("serial")
private java.util.List handlers_;
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
@java.lang.Override
public java.util.List getHandlersList() {
return handlers_;
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
@java.lang.Override
public java.util.List extends com.google.appengine.v1.UrlMapOrBuilder>
getHandlersOrBuilderList() {
return handlers_;
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
@java.lang.Override
public int getHandlersCount() {
return handlers_.size();
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
@java.lang.Override
public com.google.appengine.v1.UrlMap getHandlers(int index) {
return handlers_.get(index);
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
@java.lang.Override
public com.google.appengine.v1.UrlMapOrBuilder getHandlersOrBuilder(int index) {
return handlers_.get(index);
}
public static final int ERROR_HANDLERS_FIELD_NUMBER = 101;
@SuppressWarnings("serial")
private java.util.List errorHandlers_;
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
@java.lang.Override
public java.util.List getErrorHandlersList() {
return errorHandlers_;
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
@java.lang.Override
public java.util.List extends com.google.appengine.v1.ErrorHandlerOrBuilder>
getErrorHandlersOrBuilderList() {
return errorHandlers_;
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
@java.lang.Override
public int getErrorHandlersCount() {
return errorHandlers_.size();
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
@java.lang.Override
public com.google.appengine.v1.ErrorHandler getErrorHandlers(int index) {
return errorHandlers_.get(index);
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
@java.lang.Override
public com.google.appengine.v1.ErrorHandlerOrBuilder getErrorHandlersOrBuilder(int index) {
return errorHandlers_.get(index);
}
public static final int LIBRARIES_FIELD_NUMBER = 102;
@SuppressWarnings("serial")
private java.util.List libraries_;
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
@java.lang.Override
public java.util.List getLibrariesList() {
return libraries_;
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
@java.lang.Override
public java.util.List extends com.google.appengine.v1.LibraryOrBuilder>
getLibrariesOrBuilderList() {
return libraries_;
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
@java.lang.Override
public int getLibrariesCount() {
return libraries_.size();
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
@java.lang.Override
public com.google.appengine.v1.Library getLibraries(int index) {
return libraries_.get(index);
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
@java.lang.Override
public com.google.appengine.v1.LibraryOrBuilder getLibrariesOrBuilder(int index) {
return libraries_.get(index);
}
public static final int API_CONFIG_FIELD_NUMBER = 103;
private com.google.appengine.v1.ApiConfigHandler apiConfig_;
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*
* @return Whether the apiConfig field is set.
*/
@java.lang.Override
public boolean hasApiConfig() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*
* @return The apiConfig.
*/
@java.lang.Override
public com.google.appengine.v1.ApiConfigHandler getApiConfig() {
return apiConfig_ == null
? com.google.appengine.v1.ApiConfigHandler.getDefaultInstance()
: apiConfig_;
}
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*/
@java.lang.Override
public com.google.appengine.v1.ApiConfigHandlerOrBuilder getApiConfigOrBuilder() {
return apiConfig_ == null
? com.google.appengine.v1.ApiConfigHandler.getDefaultInstance()
: apiConfig_;
}
public static final int ENV_VARIABLES_FIELD_NUMBER = 104;
private static final class EnvVariablesDefaultEntryHolder {
static final com.google.protobuf.MapEntry defaultEntry =
com.google.protobuf.MapEntry.newDefaultInstance(
com.google.appengine.v1.VersionProto
.internal_static_google_appengine_v1_Version_EnvVariablesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField envVariables_;
private com.google.protobuf.MapField
internalGetEnvVariables() {
if (envVariables_ == null) {
return com.google.protobuf.MapField.emptyMapField(
EnvVariablesDefaultEntryHolder.defaultEntry);
}
return envVariables_;
}
public int getEnvVariablesCount() {
return internalGetEnvVariables().getMap().size();
}
/**
*
*
*
* Environment variables available to the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> env_variables = 104;
*/
@java.lang.Override
public boolean containsEnvVariables(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetEnvVariables().getMap().containsKey(key);
}
/** Use {@link #getEnvVariablesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getEnvVariables() {
return getEnvVariablesMap();
}
/**
*
*
*
* Environment variables available to the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> env_variables = 104;
*/
@java.lang.Override
public java.util.Map getEnvVariablesMap() {
return internalGetEnvVariables().getMap();
}
/**
*
*
*
* Environment variables available to the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> env_variables = 104;
*/
@java.lang.Override
public /* nullable */ java.lang.String getEnvVariablesOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetEnvVariables().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Environment variables available to the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> env_variables = 104;
*/
@java.lang.Override
public java.lang.String getEnvVariablesOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetEnvVariables().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int BUILD_ENV_VARIABLES_FIELD_NUMBER = 125;
private static final class BuildEnvVariablesDefaultEntryHolder {
static final com.google.protobuf.MapEntry defaultEntry =
com.google.protobuf.MapEntry.newDefaultInstance(
com.google.appengine.v1.VersionProto
.internal_static_google_appengine_v1_Version_BuildEnvVariablesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField buildEnvVariables_;
private com.google.protobuf.MapField
internalGetBuildEnvVariables() {
if (buildEnvVariables_ == null) {
return com.google.protobuf.MapField.emptyMapField(
BuildEnvVariablesDefaultEntryHolder.defaultEntry);
}
return buildEnvVariables_;
}
public int getBuildEnvVariablesCount() {
return internalGetBuildEnvVariables().getMap().size();
}
/**
*
*
*
* Environment variables available to the build environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> build_env_variables = 125;
*/
@java.lang.Override
public boolean containsBuildEnvVariables(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetBuildEnvVariables().getMap().containsKey(key);
}
/** Use {@link #getBuildEnvVariablesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getBuildEnvVariables() {
return getBuildEnvVariablesMap();
}
/**
*
*
*
* Environment variables available to the build environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> build_env_variables = 125;
*/
@java.lang.Override
public java.util.Map getBuildEnvVariablesMap() {
return internalGetBuildEnvVariables().getMap();
}
/**
*
*
*
* Environment variables available to the build environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> build_env_variables = 125;
*/
@java.lang.Override
public /* nullable */ java.lang.String getBuildEnvVariablesOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetBuildEnvVariables().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Environment variables available to the build environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> build_env_variables = 125;
*/
@java.lang.Override
public java.lang.String getBuildEnvVariablesOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetBuildEnvVariables().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int DEFAULT_EXPIRATION_FIELD_NUMBER = 105;
private com.google.protobuf.Duration defaultExpiration_;
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*
* @return Whether the defaultExpiration field is set.
*/
@java.lang.Override
public boolean hasDefaultExpiration() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*
* @return The defaultExpiration.
*/
@java.lang.Override
public com.google.protobuf.Duration getDefaultExpiration() {
return defaultExpiration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: defaultExpiration_;
}
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getDefaultExpirationOrBuilder() {
return defaultExpiration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: defaultExpiration_;
}
public static final int HEALTH_CHECK_FIELD_NUMBER = 106;
private com.google.appengine.v1.HealthCheck healthCheck_;
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*
* @return Whether the healthCheck field is set.
*/
@java.lang.Override
public boolean hasHealthCheck() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*
* @return The healthCheck.
*/
@java.lang.Override
public com.google.appengine.v1.HealthCheck getHealthCheck() {
return healthCheck_ == null
? com.google.appengine.v1.HealthCheck.getDefaultInstance()
: healthCheck_;
}
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*/
@java.lang.Override
public com.google.appengine.v1.HealthCheckOrBuilder getHealthCheckOrBuilder() {
return healthCheck_ == null
? com.google.appengine.v1.HealthCheck.getDefaultInstance()
: healthCheck_;
}
public static final int READINESS_CHECK_FIELD_NUMBER = 112;
private com.google.appengine.v1.ReadinessCheck readinessCheck_;
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*
* @return Whether the readinessCheck field is set.
*/
@java.lang.Override
public boolean hasReadinessCheck() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*
* @return The readinessCheck.
*/
@java.lang.Override
public com.google.appengine.v1.ReadinessCheck getReadinessCheck() {
return readinessCheck_ == null
? com.google.appengine.v1.ReadinessCheck.getDefaultInstance()
: readinessCheck_;
}
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*/
@java.lang.Override
public com.google.appengine.v1.ReadinessCheckOrBuilder getReadinessCheckOrBuilder() {
return readinessCheck_ == null
? com.google.appengine.v1.ReadinessCheck.getDefaultInstance()
: readinessCheck_;
}
public static final int LIVENESS_CHECK_FIELD_NUMBER = 113;
private com.google.appengine.v1.LivenessCheck livenessCheck_;
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*
* @return Whether the livenessCheck field is set.
*/
@java.lang.Override
public boolean hasLivenessCheck() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*
* @return The livenessCheck.
*/
@java.lang.Override
public com.google.appengine.v1.LivenessCheck getLivenessCheck() {
return livenessCheck_ == null
? com.google.appengine.v1.LivenessCheck.getDefaultInstance()
: livenessCheck_;
}
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*/
@java.lang.Override
public com.google.appengine.v1.LivenessCheckOrBuilder getLivenessCheckOrBuilder() {
return livenessCheck_ == null
? com.google.appengine.v1.LivenessCheck.getDefaultInstance()
: livenessCheck_;
}
public static final int NOBUILD_FILES_REGEX_FIELD_NUMBER = 107;
@SuppressWarnings("serial")
private volatile java.lang.Object nobuildFilesRegex_ = "";
/**
*
*
*
* Files that match this pattern will not be built into this version.
* Only applicable for Go runtimes.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* string nobuild_files_regex = 107;
*
* @return The nobuildFilesRegex.
*/
@java.lang.Override
public java.lang.String getNobuildFilesRegex() {
java.lang.Object ref = nobuildFilesRegex_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nobuildFilesRegex_ = s;
return s;
}
}
/**
*
*
*
* Files that match this pattern will not be built into this version.
* Only applicable for Go runtimes.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* string nobuild_files_regex = 107;
*
* @return The bytes for nobuildFilesRegex.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNobuildFilesRegexBytes() {
java.lang.Object ref = nobuildFilesRegex_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
nobuildFilesRegex_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEPLOYMENT_FIELD_NUMBER = 108;
private com.google.appengine.v1.Deployment deployment_;
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*
* @return Whether the deployment field is set.
*/
@java.lang.Override
public boolean hasDeployment() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*
* @return The deployment.
*/
@java.lang.Override
public com.google.appengine.v1.Deployment getDeployment() {
return deployment_ == null
? com.google.appengine.v1.Deployment.getDefaultInstance()
: deployment_;
}
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*/
@java.lang.Override
public com.google.appengine.v1.DeploymentOrBuilder getDeploymentOrBuilder() {
return deployment_ == null
? com.google.appengine.v1.Deployment.getDefaultInstance()
: deployment_;
}
public static final int VERSION_URL_FIELD_NUMBER = 109;
@SuppressWarnings("serial")
private volatile java.lang.Object versionUrl_ = "";
/**
*
*
*
* Serving URL for this version. Example:
* "https://myversion-dot-myservice-dot-myapp.appspot.com"
*
* @OutputOnly
*
*
* string version_url = 109;
*
* @return The versionUrl.
*/
@java.lang.Override
public java.lang.String getVersionUrl() {
java.lang.Object ref = versionUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
versionUrl_ = s;
return s;
}
}
/**
*
*
*
* Serving URL for this version. Example:
* "https://myversion-dot-myservice-dot-myapp.appspot.com"
*
* @OutputOnly
*
*
* string version_url = 109;
*
* @return The bytes for versionUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString getVersionUrlBytes() {
java.lang.Object ref = versionUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
versionUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ENDPOINTS_API_SERVICE_FIELD_NUMBER = 110;
private com.google.appengine.v1.EndpointsApiService endpointsApiService_;
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*
* @return Whether the endpointsApiService field is set.
*/
@java.lang.Override
public boolean hasEndpointsApiService() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*
* @return The endpointsApiService.
*/
@java.lang.Override
public com.google.appengine.v1.EndpointsApiService getEndpointsApiService() {
return endpointsApiService_ == null
? com.google.appengine.v1.EndpointsApiService.getDefaultInstance()
: endpointsApiService_;
}
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*/
@java.lang.Override
public com.google.appengine.v1.EndpointsApiServiceOrBuilder getEndpointsApiServiceOrBuilder() {
return endpointsApiService_ == null
? com.google.appengine.v1.EndpointsApiService.getDefaultInstance()
: endpointsApiService_;
}
public static final int ENTRYPOINT_FIELD_NUMBER = 122;
private com.google.appengine.v1.Entrypoint entrypoint_;
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*
* @return Whether the entrypoint field is set.
*/
@java.lang.Override
public boolean hasEntrypoint() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*
* @return The entrypoint.
*/
@java.lang.Override
public com.google.appengine.v1.Entrypoint getEntrypoint() {
return entrypoint_ == null
? com.google.appengine.v1.Entrypoint.getDefaultInstance()
: entrypoint_;
}
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*/
@java.lang.Override
public com.google.appengine.v1.EntrypointOrBuilder getEntrypointOrBuilder() {
return entrypoint_ == null
? com.google.appengine.v1.Entrypoint.getDefaultInstance()
: entrypoint_;
}
public static final int VPC_ACCESS_CONNECTOR_FIELD_NUMBER = 121;
private com.google.appengine.v1.VpcAccessConnector vpcAccessConnector_;
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*
* @return Whether the vpcAccessConnector field is set.
*/
@java.lang.Override
public boolean hasVpcAccessConnector() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*
* @return The vpcAccessConnector.
*/
@java.lang.Override
public com.google.appengine.v1.VpcAccessConnector getVpcAccessConnector() {
return vpcAccessConnector_ == null
? com.google.appengine.v1.VpcAccessConnector.getDefaultInstance()
: vpcAccessConnector_;
}
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*/
@java.lang.Override
public com.google.appengine.v1.VpcAccessConnectorOrBuilder getVpcAccessConnectorOrBuilder() {
return vpcAccessConnector_ == null
? com.google.appengine.v1.VpcAccessConnector.getDefaultInstance()
: vpcAccessConnector_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
getSerializedSize();
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, id_);
}
if (scalingCase_ == 3) {
output.writeMessage(3, (com.google.appengine.v1.AutomaticScaling) scaling_);
}
if (scalingCase_ == 4) {
output.writeMessage(4, (com.google.appengine.v1.BasicScaling) scaling_);
}
if (scalingCase_ == 5) {
output.writeMessage(5, (com.google.appengine.v1.ManualScaling) scaling_);
}
if (getInboundServicesList().size() > 0) {
output.writeUInt32NoTag(50);
output.writeUInt32NoTag(inboundServicesMemoizedSerializedSize);
}
for (int i = 0; i < inboundServices_.size(); i++) {
output.writeEnumNoTag(inboundServices_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(instanceClass_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, instanceClass_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(8, getNetwork());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(9, getResources());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(runtime_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, runtime_);
}
if (threadsafe_ != false) {
output.writeBool(11, threadsafe_);
}
if (vm_ != false) {
output.writeBool(12, vm_);
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetBetaSettings(), BetaSettingsDefaultEntryHolder.defaultEntry, 13);
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(env_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, env_);
}
if (servingStatus_
!= com.google.appengine.v1.ServingStatus.SERVING_STATUS_UNSPECIFIED.getNumber()) {
output.writeEnum(15, servingStatus_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(createdBy_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, createdBy_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(17, getCreateTime());
}
if (diskUsageBytes_ != 0L) {
output.writeInt64(18, diskUsageBytes_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(runtimeApiVersion_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 21, runtimeApiVersion_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(runtimeMainExecutablePath_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 22, runtimeMainExecutablePath_);
}
for (int i = 0; i < handlers_.size(); i++) {
output.writeMessage(100, handlers_.get(i));
}
for (int i = 0; i < errorHandlers_.size(); i++) {
output.writeMessage(101, errorHandlers_.get(i));
}
for (int i = 0; i < libraries_.size(); i++) {
output.writeMessage(102, libraries_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(103, getApiConfig());
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetEnvVariables(), EnvVariablesDefaultEntryHolder.defaultEntry, 104);
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(105, getDefaultExpiration());
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(106, getHealthCheck());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(nobuildFilesRegex_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 107, nobuildFilesRegex_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeMessage(108, getDeployment());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(versionUrl_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 109, versionUrl_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeMessage(110, getEndpointsApiService());
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(112, getReadinessCheck());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeMessage(113, getLivenessCheck());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(runtimeChannel_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 117, runtimeChannel_);
}
for (int i = 0; i < zones_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 118, zones_.getRaw(i));
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeMessage(121, getVpcAccessConnector());
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeMessage(122, getEntrypoint());
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output,
internalGetBuildEnvVariables(),
BuildEnvVariablesDefaultEntryHolder.defaultEntry,
125);
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(serviceAccount_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 127, serviceAccount_);
}
if (appEngineApis_ != false) {
output.writeBool(128, appEngineApis_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, id_);
}
if (scalingCase_ == 3) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
3, (com.google.appengine.v1.AutomaticScaling) scaling_);
}
if (scalingCase_ == 4) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
4, (com.google.appengine.v1.BasicScaling) scaling_);
}
if (scalingCase_ == 5) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
5, (com.google.appengine.v1.ManualScaling) scaling_);
}
{
int dataSize = 0;
for (int i = 0; i < inboundServices_.size(); i++) {
dataSize +=
com.google.protobuf.CodedOutputStream.computeEnumSizeNoTag(inboundServices_.get(i));
}
size += dataSize;
if (!getInboundServicesList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(dataSize);
}
inboundServicesMemoizedSerializedSize = dataSize;
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(instanceClass_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, instanceClass_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(8, getNetwork());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(9, getResources());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(runtime_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, runtime_);
}
if (threadsafe_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(11, threadsafe_);
}
if (vm_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(12, vm_);
}
for (java.util.Map.Entry entry :
internalGetBetaSettings().getMap().entrySet()) {
com.google.protobuf.MapEntry betaSettings__ =
BetaSettingsDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(13, betaSettings__);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(env_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, env_);
}
if (servingStatus_
!= com.google.appengine.v1.ServingStatus.SERVING_STATUS_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(15, servingStatus_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(createdBy_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, createdBy_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(17, getCreateTime());
}
if (diskUsageBytes_ != 0L) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(18, diskUsageBytes_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(runtimeApiVersion_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(21, runtimeApiVersion_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(runtimeMainExecutablePath_)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(22, runtimeMainExecutablePath_);
}
for (int i = 0; i < handlers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(100, handlers_.get(i));
}
for (int i = 0; i < errorHandlers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(101, errorHandlers_.get(i));
}
for (int i = 0; i < libraries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(102, libraries_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(103, getApiConfig());
}
for (java.util.Map.Entry entry :
internalGetEnvVariables().getMap().entrySet()) {
com.google.protobuf.MapEntry envVariables__ =
EnvVariablesDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(104, envVariables__);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(105, getDefaultExpiration());
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(106, getHealthCheck());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(nobuildFilesRegex_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(107, nobuildFilesRegex_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(108, getDeployment());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(versionUrl_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(109, versionUrl_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(110, getEndpointsApiService());
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(112, getReadinessCheck());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(113, getLivenessCheck());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(runtimeChannel_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(117, runtimeChannel_);
}
{
int dataSize = 0;
for (int i = 0; i < zones_.size(); i++) {
dataSize += computeStringSizeNoTag(zones_.getRaw(i));
}
size += dataSize;
size += 2 * getZonesList().size();
}
if (((bitField0_ & 0x00000800) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(121, getVpcAccessConnector());
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(122, getEntrypoint());
}
for (java.util.Map.Entry entry :
internalGetBuildEnvVariables().getMap().entrySet()) {
com.google.protobuf.MapEntry buildEnvVariables__ =
BuildEnvVariablesDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(125, buildEnvVariables__);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(serviceAccount_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(127, serviceAccount_);
}
if (appEngineApis_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(128, appEngineApis_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.v1.Version)) {
return super.equals(obj);
}
com.google.appengine.v1.Version other = (com.google.appengine.v1.Version) obj;
if (!getName().equals(other.getName())) return false;
if (!getId().equals(other.getId())) return false;
if (!inboundServices_.equals(other.inboundServices_)) return false;
if (!getInstanceClass().equals(other.getInstanceClass())) return false;
if (hasNetwork() != other.hasNetwork()) return false;
if (hasNetwork()) {
if (!getNetwork().equals(other.getNetwork())) return false;
}
if (!getZonesList().equals(other.getZonesList())) return false;
if (hasResources() != other.hasResources()) return false;
if (hasResources()) {
if (!getResources().equals(other.getResources())) return false;
}
if (!getRuntime().equals(other.getRuntime())) return false;
if (!getRuntimeChannel().equals(other.getRuntimeChannel())) return false;
if (getThreadsafe() != other.getThreadsafe()) return false;
if (getVm() != other.getVm()) return false;
if (getAppEngineApis() != other.getAppEngineApis()) return false;
if (!internalGetBetaSettings().equals(other.internalGetBetaSettings())) return false;
if (!getEnv().equals(other.getEnv())) return false;
if (servingStatus_ != other.servingStatus_) return false;
if (!getCreatedBy().equals(other.getCreatedBy())) return false;
if (hasCreateTime() != other.hasCreateTime()) return false;
if (hasCreateTime()) {
if (!getCreateTime().equals(other.getCreateTime())) return false;
}
if (getDiskUsageBytes() != other.getDiskUsageBytes()) return false;
if (!getRuntimeApiVersion().equals(other.getRuntimeApiVersion())) return false;
if (!getRuntimeMainExecutablePath().equals(other.getRuntimeMainExecutablePath())) return false;
if (!getServiceAccount().equals(other.getServiceAccount())) return false;
if (!getHandlersList().equals(other.getHandlersList())) return false;
if (!getErrorHandlersList().equals(other.getErrorHandlersList())) return false;
if (!getLibrariesList().equals(other.getLibrariesList())) return false;
if (hasApiConfig() != other.hasApiConfig()) return false;
if (hasApiConfig()) {
if (!getApiConfig().equals(other.getApiConfig())) return false;
}
if (!internalGetEnvVariables().equals(other.internalGetEnvVariables())) return false;
if (!internalGetBuildEnvVariables().equals(other.internalGetBuildEnvVariables())) return false;
if (hasDefaultExpiration() != other.hasDefaultExpiration()) return false;
if (hasDefaultExpiration()) {
if (!getDefaultExpiration().equals(other.getDefaultExpiration())) return false;
}
if (hasHealthCheck() != other.hasHealthCheck()) return false;
if (hasHealthCheck()) {
if (!getHealthCheck().equals(other.getHealthCheck())) return false;
}
if (hasReadinessCheck() != other.hasReadinessCheck()) return false;
if (hasReadinessCheck()) {
if (!getReadinessCheck().equals(other.getReadinessCheck())) return false;
}
if (hasLivenessCheck() != other.hasLivenessCheck()) return false;
if (hasLivenessCheck()) {
if (!getLivenessCheck().equals(other.getLivenessCheck())) return false;
}
if (!getNobuildFilesRegex().equals(other.getNobuildFilesRegex())) return false;
if (hasDeployment() != other.hasDeployment()) return false;
if (hasDeployment()) {
if (!getDeployment().equals(other.getDeployment())) return false;
}
if (!getVersionUrl().equals(other.getVersionUrl())) return false;
if (hasEndpointsApiService() != other.hasEndpointsApiService()) return false;
if (hasEndpointsApiService()) {
if (!getEndpointsApiService().equals(other.getEndpointsApiService())) return false;
}
if (hasEntrypoint() != other.hasEntrypoint()) return false;
if (hasEntrypoint()) {
if (!getEntrypoint().equals(other.getEntrypoint())) return false;
}
if (hasVpcAccessConnector() != other.hasVpcAccessConnector()) return false;
if (hasVpcAccessConnector()) {
if (!getVpcAccessConnector().equals(other.getVpcAccessConnector())) return false;
}
if (!getScalingCase().equals(other.getScalingCase())) return false;
switch (scalingCase_) {
case 3:
if (!getAutomaticScaling().equals(other.getAutomaticScaling())) return false;
break;
case 4:
if (!getBasicScaling().equals(other.getBasicScaling())) return false;
break;
case 5:
if (!getManualScaling().equals(other.getManualScaling())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
if (getInboundServicesCount() > 0) {
hash = (37 * hash) + INBOUND_SERVICES_FIELD_NUMBER;
hash = (53 * hash) + inboundServices_.hashCode();
}
hash = (37 * hash) + INSTANCE_CLASS_FIELD_NUMBER;
hash = (53 * hash) + getInstanceClass().hashCode();
if (hasNetwork()) {
hash = (37 * hash) + NETWORK_FIELD_NUMBER;
hash = (53 * hash) + getNetwork().hashCode();
}
if (getZonesCount() > 0) {
hash = (37 * hash) + ZONES_FIELD_NUMBER;
hash = (53 * hash) + getZonesList().hashCode();
}
if (hasResources()) {
hash = (37 * hash) + RESOURCES_FIELD_NUMBER;
hash = (53 * hash) + getResources().hashCode();
}
hash = (37 * hash) + RUNTIME_FIELD_NUMBER;
hash = (53 * hash) + getRuntime().hashCode();
hash = (37 * hash) + RUNTIME_CHANNEL_FIELD_NUMBER;
hash = (53 * hash) + getRuntimeChannel().hashCode();
hash = (37 * hash) + THREADSAFE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getThreadsafe());
hash = (37 * hash) + VM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getVm());
hash = (37 * hash) + APP_ENGINE_APIS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getAppEngineApis());
if (!internalGetBetaSettings().getMap().isEmpty()) {
hash = (37 * hash) + BETA_SETTINGS_FIELD_NUMBER;
hash = (53 * hash) + internalGetBetaSettings().hashCode();
}
hash = (37 * hash) + ENV_FIELD_NUMBER;
hash = (53 * hash) + getEnv().hashCode();
hash = (37 * hash) + SERVING_STATUS_FIELD_NUMBER;
hash = (53 * hash) + servingStatus_;
hash = (37 * hash) + CREATED_BY_FIELD_NUMBER;
hash = (53 * hash) + getCreatedBy().hashCode();
if (hasCreateTime()) {
hash = (37 * hash) + CREATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getCreateTime().hashCode();
}
hash = (37 * hash) + DISK_USAGE_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getDiskUsageBytes());
hash = (37 * hash) + RUNTIME_API_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getRuntimeApiVersion().hashCode();
hash = (37 * hash) + RUNTIME_MAIN_EXECUTABLE_PATH_FIELD_NUMBER;
hash = (53 * hash) + getRuntimeMainExecutablePath().hashCode();
hash = (37 * hash) + SERVICE_ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getServiceAccount().hashCode();
if (getHandlersCount() > 0) {
hash = (37 * hash) + HANDLERS_FIELD_NUMBER;
hash = (53 * hash) + getHandlersList().hashCode();
}
if (getErrorHandlersCount() > 0) {
hash = (37 * hash) + ERROR_HANDLERS_FIELD_NUMBER;
hash = (53 * hash) + getErrorHandlersList().hashCode();
}
if (getLibrariesCount() > 0) {
hash = (37 * hash) + LIBRARIES_FIELD_NUMBER;
hash = (53 * hash) + getLibrariesList().hashCode();
}
if (hasApiConfig()) {
hash = (37 * hash) + API_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getApiConfig().hashCode();
}
if (!internalGetEnvVariables().getMap().isEmpty()) {
hash = (37 * hash) + ENV_VARIABLES_FIELD_NUMBER;
hash = (53 * hash) + internalGetEnvVariables().hashCode();
}
if (!internalGetBuildEnvVariables().getMap().isEmpty()) {
hash = (37 * hash) + BUILD_ENV_VARIABLES_FIELD_NUMBER;
hash = (53 * hash) + internalGetBuildEnvVariables().hashCode();
}
if (hasDefaultExpiration()) {
hash = (37 * hash) + DEFAULT_EXPIRATION_FIELD_NUMBER;
hash = (53 * hash) + getDefaultExpiration().hashCode();
}
if (hasHealthCheck()) {
hash = (37 * hash) + HEALTH_CHECK_FIELD_NUMBER;
hash = (53 * hash) + getHealthCheck().hashCode();
}
if (hasReadinessCheck()) {
hash = (37 * hash) + READINESS_CHECK_FIELD_NUMBER;
hash = (53 * hash) + getReadinessCheck().hashCode();
}
if (hasLivenessCheck()) {
hash = (37 * hash) + LIVENESS_CHECK_FIELD_NUMBER;
hash = (53 * hash) + getLivenessCheck().hashCode();
}
hash = (37 * hash) + NOBUILD_FILES_REGEX_FIELD_NUMBER;
hash = (53 * hash) + getNobuildFilesRegex().hashCode();
if (hasDeployment()) {
hash = (37 * hash) + DEPLOYMENT_FIELD_NUMBER;
hash = (53 * hash) + getDeployment().hashCode();
}
hash = (37 * hash) + VERSION_URL_FIELD_NUMBER;
hash = (53 * hash) + getVersionUrl().hashCode();
if (hasEndpointsApiService()) {
hash = (37 * hash) + ENDPOINTS_API_SERVICE_FIELD_NUMBER;
hash = (53 * hash) + getEndpointsApiService().hashCode();
}
if (hasEntrypoint()) {
hash = (37 * hash) + ENTRYPOINT_FIELD_NUMBER;
hash = (53 * hash) + getEntrypoint().hashCode();
}
if (hasVpcAccessConnector()) {
hash = (37 * hash) + VPC_ACCESS_CONNECTOR_FIELD_NUMBER;
hash = (53 * hash) + getVpcAccessConnector().hashCode();
}
switch (scalingCase_) {
case 3:
hash = (37 * hash) + AUTOMATIC_SCALING_FIELD_NUMBER;
hash = (53 * hash) + getAutomaticScaling().hashCode();
break;
case 4:
hash = (37 * hash) + BASIC_SCALING_FIELD_NUMBER;
hash = (53 * hash) + getBasicScaling().hashCode();
break;
case 5:
hash = (37 * hash) + MANUAL_SCALING_FIELD_NUMBER;
hash = (53 * hash) + getManualScaling().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.v1.Version parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.v1.Version parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.v1.Version parseFrom(com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.v1.Version parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.v1.Version parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.v1.Version parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.v1.Version parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.appengine.v1.Version parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.appengine.v1.Version parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.v1.Version parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.appengine.v1.Version parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.appengine.v1.Version parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.v1.Version prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* A Version resource is a specific set of source code and configuration files
* that are deployed into a service.
*
*
* Protobuf type {@code google.appengine.v1.Version}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.appengine.v1.Version)
com.google.appengine.v1.VersionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.appengine.v1.VersionProto
.internal_static_google_appengine_v1_Version_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 13:
return internalGetBetaSettings();
case 104:
return internalGetEnvVariables();
case 125:
return internalGetBuildEnvVariables();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 13:
return internalGetMutableBetaSettings();
case 104:
return internalGetMutableEnvVariables();
case 125:
return internalGetMutableBuildEnvVariables();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.v1.VersionProto
.internal_static_google_appengine_v1_Version_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.v1.Version.class, com.google.appengine.v1.Version.Builder.class);
}
// Construct using com.google.appengine.v1.Version.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getNetworkFieldBuilder();
getResourcesFieldBuilder();
getCreateTimeFieldBuilder();
getHandlersFieldBuilder();
getErrorHandlersFieldBuilder();
getLibrariesFieldBuilder();
getApiConfigFieldBuilder();
getDefaultExpirationFieldBuilder();
getHealthCheckFieldBuilder();
getReadinessCheckFieldBuilder();
getLivenessCheckFieldBuilder();
getDeploymentFieldBuilder();
getEndpointsApiServiceFieldBuilder();
getEntrypointFieldBuilder();
getVpcAccessConnectorFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
bitField1_ = 0;
name_ = "";
id_ = "";
if (automaticScalingBuilder_ != null) {
automaticScalingBuilder_.clear();
}
if (basicScalingBuilder_ != null) {
basicScalingBuilder_.clear();
}
if (manualScalingBuilder_ != null) {
manualScalingBuilder_.clear();
}
inboundServices_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
instanceClass_ = "";
network_ = null;
if (networkBuilder_ != null) {
networkBuilder_.dispose();
networkBuilder_ = null;
}
zones_ = com.google.protobuf.LazyStringArrayList.emptyList();
resources_ = null;
if (resourcesBuilder_ != null) {
resourcesBuilder_.dispose();
resourcesBuilder_ = null;
}
runtime_ = "";
runtimeChannel_ = "";
threadsafe_ = false;
vm_ = false;
appEngineApis_ = false;
internalGetMutableBetaSettings().clear();
env_ = "";
servingStatus_ = 0;
createdBy_ = "";
createTime_ = null;
if (createTimeBuilder_ != null) {
createTimeBuilder_.dispose();
createTimeBuilder_ = null;
}
diskUsageBytes_ = 0L;
runtimeApiVersion_ = "";
runtimeMainExecutablePath_ = "";
serviceAccount_ = "";
if (handlersBuilder_ == null) {
handlers_ = java.util.Collections.emptyList();
} else {
handlers_ = null;
handlersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x01000000);
if (errorHandlersBuilder_ == null) {
errorHandlers_ = java.util.Collections.emptyList();
} else {
errorHandlers_ = null;
errorHandlersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x02000000);
if (librariesBuilder_ == null) {
libraries_ = java.util.Collections.emptyList();
} else {
libraries_ = null;
librariesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x04000000);
apiConfig_ = null;
if (apiConfigBuilder_ != null) {
apiConfigBuilder_.dispose();
apiConfigBuilder_ = null;
}
internalGetMutableEnvVariables().clear();
internalGetMutableBuildEnvVariables().clear();
defaultExpiration_ = null;
if (defaultExpirationBuilder_ != null) {
defaultExpirationBuilder_.dispose();
defaultExpirationBuilder_ = null;
}
healthCheck_ = null;
if (healthCheckBuilder_ != null) {
healthCheckBuilder_.dispose();
healthCheckBuilder_ = null;
}
readinessCheck_ = null;
if (readinessCheckBuilder_ != null) {
readinessCheckBuilder_.dispose();
readinessCheckBuilder_ = null;
}
livenessCheck_ = null;
if (livenessCheckBuilder_ != null) {
livenessCheckBuilder_.dispose();
livenessCheckBuilder_ = null;
}
nobuildFilesRegex_ = "";
deployment_ = null;
if (deploymentBuilder_ != null) {
deploymentBuilder_.dispose();
deploymentBuilder_ = null;
}
versionUrl_ = "";
endpointsApiService_ = null;
if (endpointsApiServiceBuilder_ != null) {
endpointsApiServiceBuilder_.dispose();
endpointsApiServiceBuilder_ = null;
}
entrypoint_ = null;
if (entrypointBuilder_ != null) {
entrypointBuilder_.dispose();
entrypointBuilder_ = null;
}
vpcAccessConnector_ = null;
if (vpcAccessConnectorBuilder_ != null) {
vpcAccessConnectorBuilder_.dispose();
vpcAccessConnectorBuilder_ = null;
}
scalingCase_ = 0;
scaling_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.appengine.v1.VersionProto
.internal_static_google_appengine_v1_Version_descriptor;
}
@java.lang.Override
public com.google.appengine.v1.Version getDefaultInstanceForType() {
return com.google.appengine.v1.Version.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.v1.Version build() {
com.google.appengine.v1.Version result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.v1.Version buildPartial() {
com.google.appengine.v1.Version result = new com.google.appengine.v1.Version(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
if (bitField1_ != 0) {
buildPartial1(result);
}
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.appengine.v1.Version result) {
if (((bitField0_ & 0x00000020) != 0)) {
inboundServices_ = java.util.Collections.unmodifiableList(inboundServices_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.inboundServices_ = inboundServices_;
if (handlersBuilder_ == null) {
if (((bitField0_ & 0x01000000) != 0)) {
handlers_ = java.util.Collections.unmodifiableList(handlers_);
bitField0_ = (bitField0_ & ~0x01000000);
}
result.handlers_ = handlers_;
} else {
result.handlers_ = handlersBuilder_.build();
}
if (errorHandlersBuilder_ == null) {
if (((bitField0_ & 0x02000000) != 0)) {
errorHandlers_ = java.util.Collections.unmodifiableList(errorHandlers_);
bitField0_ = (bitField0_ & ~0x02000000);
}
result.errorHandlers_ = errorHandlers_;
} else {
result.errorHandlers_ = errorHandlersBuilder_.build();
}
if (librariesBuilder_ == null) {
if (((bitField0_ & 0x04000000) != 0)) {
libraries_ = java.util.Collections.unmodifiableList(libraries_);
bitField0_ = (bitField0_ & ~0x04000000);
}
result.libraries_ = libraries_;
} else {
result.libraries_ = librariesBuilder_.build();
}
}
private void buildPartial0(com.google.appengine.v1.Version result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.id_ = id_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.instanceClass_ = instanceClass_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000080) != 0)) {
result.network_ = networkBuilder_ == null ? network_ : networkBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
zones_.makeImmutable();
result.zones_ = zones_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.resources_ = resourcesBuilder_ == null ? resources_ : resourcesBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.runtime_ = runtime_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.runtimeChannel_ = runtimeChannel_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.threadsafe_ = threadsafe_;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.vm_ = vm_;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.appEngineApis_ = appEngineApis_;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.betaSettings_ = internalGetBetaSettings();
result.betaSettings_.makeImmutable();
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.env_ = env_;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.servingStatus_ = servingStatus_;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.createdBy_ = createdBy_;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.createTime_ = createTimeBuilder_ == null ? createTime_ : createTimeBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.diskUsageBytes_ = diskUsageBytes_;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.runtimeApiVersion_ = runtimeApiVersion_;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.runtimeMainExecutablePath_ = runtimeMainExecutablePath_;
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.serviceAccount_ = serviceAccount_;
}
if (((from_bitField0_ & 0x08000000) != 0)) {
result.apiConfig_ = apiConfigBuilder_ == null ? apiConfig_ : apiConfigBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x10000000) != 0)) {
result.envVariables_ = internalGetEnvVariables();
result.envVariables_.makeImmutable();
}
if (((from_bitField0_ & 0x20000000) != 0)) {
result.buildEnvVariables_ = internalGetBuildEnvVariables();
result.buildEnvVariables_.makeImmutable();
}
if (((from_bitField0_ & 0x40000000) != 0)) {
result.defaultExpiration_ =
defaultExpirationBuilder_ == null
? defaultExpiration_
: defaultExpirationBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x80000000) != 0)) {
result.healthCheck_ =
healthCheckBuilder_ == null ? healthCheck_ : healthCheckBuilder_.build();
to_bitField0_ |= 0x00000020;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartial1(com.google.appengine.v1.Version result) {
int from_bitField1_ = bitField1_;
int to_bitField0_ = 0;
if (((from_bitField1_ & 0x00000001) != 0)) {
result.readinessCheck_ =
readinessCheckBuilder_ == null ? readinessCheck_ : readinessCheckBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField1_ & 0x00000002) != 0)) {
result.livenessCheck_ =
livenessCheckBuilder_ == null ? livenessCheck_ : livenessCheckBuilder_.build();
to_bitField0_ |= 0x00000080;
}
if (((from_bitField1_ & 0x00000004) != 0)) {
result.nobuildFilesRegex_ = nobuildFilesRegex_;
}
if (((from_bitField1_ & 0x00000008) != 0)) {
result.deployment_ = deploymentBuilder_ == null ? deployment_ : deploymentBuilder_.build();
to_bitField0_ |= 0x00000100;
}
if (((from_bitField1_ & 0x00000010) != 0)) {
result.versionUrl_ = versionUrl_;
}
if (((from_bitField1_ & 0x00000020) != 0)) {
result.endpointsApiService_ =
endpointsApiServiceBuilder_ == null
? endpointsApiService_
: endpointsApiServiceBuilder_.build();
to_bitField0_ |= 0x00000200;
}
if (((from_bitField1_ & 0x00000040) != 0)) {
result.entrypoint_ = entrypointBuilder_ == null ? entrypoint_ : entrypointBuilder_.build();
to_bitField0_ |= 0x00000400;
}
if (((from_bitField1_ & 0x00000080) != 0)) {
result.vpcAccessConnector_ =
vpcAccessConnectorBuilder_ == null
? vpcAccessConnector_
: vpcAccessConnectorBuilder_.build();
to_bitField0_ |= 0x00000800;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartialOneofs(com.google.appengine.v1.Version result) {
result.scalingCase_ = scalingCase_;
result.scaling_ = this.scaling_;
if (scalingCase_ == 3 && automaticScalingBuilder_ != null) {
result.scaling_ = automaticScalingBuilder_.build();
}
if (scalingCase_ == 4 && basicScalingBuilder_ != null) {
result.scaling_ = basicScalingBuilder_.build();
}
if (scalingCase_ == 5 && manualScalingBuilder_ != null) {
result.scaling_ = manualScalingBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.v1.Version) {
return mergeFrom((com.google.appengine.v1.Version) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.v1.Version other) {
if (other == com.google.appengine.v1.Version.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getId().isEmpty()) {
id_ = other.id_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.inboundServices_.isEmpty()) {
if (inboundServices_.isEmpty()) {
inboundServices_ = other.inboundServices_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureInboundServicesIsMutable();
inboundServices_.addAll(other.inboundServices_);
}
onChanged();
}
if (!other.getInstanceClass().isEmpty()) {
instanceClass_ = other.instanceClass_;
bitField0_ |= 0x00000040;
onChanged();
}
if (other.hasNetwork()) {
mergeNetwork(other.getNetwork());
}
if (!other.zones_.isEmpty()) {
if (zones_.isEmpty()) {
zones_ = other.zones_;
bitField0_ |= 0x00000100;
} else {
ensureZonesIsMutable();
zones_.addAll(other.zones_);
}
onChanged();
}
if (other.hasResources()) {
mergeResources(other.getResources());
}
if (!other.getRuntime().isEmpty()) {
runtime_ = other.runtime_;
bitField0_ |= 0x00000400;
onChanged();
}
if (!other.getRuntimeChannel().isEmpty()) {
runtimeChannel_ = other.runtimeChannel_;
bitField0_ |= 0x00000800;
onChanged();
}
if (other.getThreadsafe() != false) {
setThreadsafe(other.getThreadsafe());
}
if (other.getVm() != false) {
setVm(other.getVm());
}
if (other.getAppEngineApis() != false) {
setAppEngineApis(other.getAppEngineApis());
}
internalGetMutableBetaSettings().mergeFrom(other.internalGetBetaSettings());
bitField0_ |= 0x00008000;
if (!other.getEnv().isEmpty()) {
env_ = other.env_;
bitField0_ |= 0x00010000;
onChanged();
}
if (other.servingStatus_ != 0) {
setServingStatusValue(other.getServingStatusValue());
}
if (!other.getCreatedBy().isEmpty()) {
createdBy_ = other.createdBy_;
bitField0_ |= 0x00040000;
onChanged();
}
if (other.hasCreateTime()) {
mergeCreateTime(other.getCreateTime());
}
if (other.getDiskUsageBytes() != 0L) {
setDiskUsageBytes(other.getDiskUsageBytes());
}
if (!other.getRuntimeApiVersion().isEmpty()) {
runtimeApiVersion_ = other.runtimeApiVersion_;
bitField0_ |= 0x00200000;
onChanged();
}
if (!other.getRuntimeMainExecutablePath().isEmpty()) {
runtimeMainExecutablePath_ = other.runtimeMainExecutablePath_;
bitField0_ |= 0x00400000;
onChanged();
}
if (!other.getServiceAccount().isEmpty()) {
serviceAccount_ = other.serviceAccount_;
bitField0_ |= 0x00800000;
onChanged();
}
if (handlersBuilder_ == null) {
if (!other.handlers_.isEmpty()) {
if (handlers_.isEmpty()) {
handlers_ = other.handlers_;
bitField0_ = (bitField0_ & ~0x01000000);
} else {
ensureHandlersIsMutable();
handlers_.addAll(other.handlers_);
}
onChanged();
}
} else {
if (!other.handlers_.isEmpty()) {
if (handlersBuilder_.isEmpty()) {
handlersBuilder_.dispose();
handlersBuilder_ = null;
handlers_ = other.handlers_;
bitField0_ = (bitField0_ & ~0x01000000);
handlersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getHandlersFieldBuilder()
: null;
} else {
handlersBuilder_.addAllMessages(other.handlers_);
}
}
}
if (errorHandlersBuilder_ == null) {
if (!other.errorHandlers_.isEmpty()) {
if (errorHandlers_.isEmpty()) {
errorHandlers_ = other.errorHandlers_;
bitField0_ = (bitField0_ & ~0x02000000);
} else {
ensureErrorHandlersIsMutable();
errorHandlers_.addAll(other.errorHandlers_);
}
onChanged();
}
} else {
if (!other.errorHandlers_.isEmpty()) {
if (errorHandlersBuilder_.isEmpty()) {
errorHandlersBuilder_.dispose();
errorHandlersBuilder_ = null;
errorHandlers_ = other.errorHandlers_;
bitField0_ = (bitField0_ & ~0x02000000);
errorHandlersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getErrorHandlersFieldBuilder()
: null;
} else {
errorHandlersBuilder_.addAllMessages(other.errorHandlers_);
}
}
}
if (librariesBuilder_ == null) {
if (!other.libraries_.isEmpty()) {
if (libraries_.isEmpty()) {
libraries_ = other.libraries_;
bitField0_ = (bitField0_ & ~0x04000000);
} else {
ensureLibrariesIsMutable();
libraries_.addAll(other.libraries_);
}
onChanged();
}
} else {
if (!other.libraries_.isEmpty()) {
if (librariesBuilder_.isEmpty()) {
librariesBuilder_.dispose();
librariesBuilder_ = null;
libraries_ = other.libraries_;
bitField0_ = (bitField0_ & ~0x04000000);
librariesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getLibrariesFieldBuilder()
: null;
} else {
librariesBuilder_.addAllMessages(other.libraries_);
}
}
}
if (other.hasApiConfig()) {
mergeApiConfig(other.getApiConfig());
}
internalGetMutableEnvVariables().mergeFrom(other.internalGetEnvVariables());
bitField0_ |= 0x10000000;
internalGetMutableBuildEnvVariables().mergeFrom(other.internalGetBuildEnvVariables());
bitField0_ |= 0x20000000;
if (other.hasDefaultExpiration()) {
mergeDefaultExpiration(other.getDefaultExpiration());
}
if (other.hasHealthCheck()) {
mergeHealthCheck(other.getHealthCheck());
}
if (other.hasReadinessCheck()) {
mergeReadinessCheck(other.getReadinessCheck());
}
if (other.hasLivenessCheck()) {
mergeLivenessCheck(other.getLivenessCheck());
}
if (!other.getNobuildFilesRegex().isEmpty()) {
nobuildFilesRegex_ = other.nobuildFilesRegex_;
bitField1_ |= 0x00000004;
onChanged();
}
if (other.hasDeployment()) {
mergeDeployment(other.getDeployment());
}
if (!other.getVersionUrl().isEmpty()) {
versionUrl_ = other.versionUrl_;
bitField1_ |= 0x00000010;
onChanged();
}
if (other.hasEndpointsApiService()) {
mergeEndpointsApiService(other.getEndpointsApiService());
}
if (other.hasEntrypoint()) {
mergeEntrypoint(other.getEntrypoint());
}
if (other.hasVpcAccessConnector()) {
mergeVpcAccessConnector(other.getVpcAccessConnector());
}
switch (other.getScalingCase()) {
case AUTOMATIC_SCALING:
{
mergeAutomaticScaling(other.getAutomaticScaling());
break;
}
case BASIC_SCALING:
{
mergeBasicScaling(other.getBasicScaling());
break;
}
case MANUAL_SCALING:
{
mergeManualScaling(other.getManualScaling());
break;
}
case SCALING_NOT_SET:
{
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
id_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26:
{
input.readMessage(
getAutomaticScalingFieldBuilder().getBuilder(), extensionRegistry);
scalingCase_ = 3;
break;
} // case 26
case 34:
{
input.readMessage(getBasicScalingFieldBuilder().getBuilder(), extensionRegistry);
scalingCase_ = 4;
break;
} // case 34
case 42:
{
input.readMessage(getManualScalingFieldBuilder().getBuilder(), extensionRegistry);
scalingCase_ = 5;
break;
} // case 42
case 48:
{
int tmpRaw = input.readEnum();
ensureInboundServicesIsMutable();
inboundServices_.add(tmpRaw);
break;
} // case 48
case 50:
{
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while (input.getBytesUntilLimit() > 0) {
int tmpRaw = input.readEnum();
ensureInboundServicesIsMutable();
inboundServices_.add(tmpRaw);
}
input.popLimit(oldLimit);
break;
} // case 50
case 58:
{
instanceClass_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 58
case 66:
{
input.readMessage(getNetworkFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 66
case 74:
{
input.readMessage(getResourcesFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000200;
break;
} // case 74
case 82:
{
runtime_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000400;
break;
} // case 82
case 88:
{
threadsafe_ = input.readBool();
bitField0_ |= 0x00001000;
break;
} // case 88
case 96:
{
vm_ = input.readBool();
bitField0_ |= 0x00002000;
break;
} // case 96
case 106:
{
com.google.protobuf.MapEntry betaSettings__ =
input.readMessage(
BetaSettingsDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableBetaSettings()
.getMutableMap()
.put(betaSettings__.getKey(), betaSettings__.getValue());
bitField0_ |= 0x00008000;
break;
} // case 106
case 114:
{
env_ = input.readStringRequireUtf8();
bitField0_ |= 0x00010000;
break;
} // case 114
case 120:
{
servingStatus_ = input.readEnum();
bitField0_ |= 0x00020000;
break;
} // case 120
case 130:
{
createdBy_ = input.readStringRequireUtf8();
bitField0_ |= 0x00040000;
break;
} // case 130
case 138:
{
input.readMessage(getCreateTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00080000;
break;
} // case 138
case 144:
{
diskUsageBytes_ = input.readInt64();
bitField0_ |= 0x00100000;
break;
} // case 144
case 170:
{
runtimeApiVersion_ = input.readStringRequireUtf8();
bitField0_ |= 0x00200000;
break;
} // case 170
case 178:
{
runtimeMainExecutablePath_ = input.readStringRequireUtf8();
bitField0_ |= 0x00400000;
break;
} // case 178
case 802:
{
com.google.appengine.v1.UrlMap m =
input.readMessage(com.google.appengine.v1.UrlMap.parser(), extensionRegistry);
if (handlersBuilder_ == null) {
ensureHandlersIsMutable();
handlers_.add(m);
} else {
handlersBuilder_.addMessage(m);
}
break;
} // case 802
case 810:
{
com.google.appengine.v1.ErrorHandler m =
input.readMessage(
com.google.appengine.v1.ErrorHandler.parser(), extensionRegistry);
if (errorHandlersBuilder_ == null) {
ensureErrorHandlersIsMutable();
errorHandlers_.add(m);
} else {
errorHandlersBuilder_.addMessage(m);
}
break;
} // case 810
case 818:
{
com.google.appengine.v1.Library m =
input.readMessage(com.google.appengine.v1.Library.parser(), extensionRegistry);
if (librariesBuilder_ == null) {
ensureLibrariesIsMutable();
libraries_.add(m);
} else {
librariesBuilder_.addMessage(m);
}
break;
} // case 818
case 826:
{
input.readMessage(getApiConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x08000000;
break;
} // case 826
case 834:
{
com.google.protobuf.MapEntry envVariables__ =
input.readMessage(
EnvVariablesDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableEnvVariables()
.getMutableMap()
.put(envVariables__.getKey(), envVariables__.getValue());
bitField0_ |= 0x10000000;
break;
} // case 834
case 842:
{
input.readMessage(
getDefaultExpirationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x40000000;
break;
} // case 842
case 850:
{
input.readMessage(getHealthCheckFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x80000000;
break;
} // case 850
case 858:
{
nobuildFilesRegex_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000004;
break;
} // case 858
case 866:
{
input.readMessage(getDeploymentFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000008;
break;
} // case 866
case 874:
{
versionUrl_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000010;
break;
} // case 874
case 882:
{
input.readMessage(
getEndpointsApiServiceFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000020;
break;
} // case 882
case 898:
{
input.readMessage(getReadinessCheckFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000001;
break;
} // case 898
case 906:
{
input.readMessage(getLivenessCheckFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000002;
break;
} // case 906
case 938:
{
runtimeChannel_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000800;
break;
} // case 938
case 946:
{
java.lang.String s = input.readStringRequireUtf8();
ensureZonesIsMutable();
zones_.add(s);
break;
} // case 946
case 970:
{
input.readMessage(
getVpcAccessConnectorFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000080;
break;
} // case 970
case 978:
{
input.readMessage(getEntrypointFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000040;
break;
} // case 978
case 1002:
{
com.google.protobuf.MapEntry
buildEnvVariables__ =
input.readMessage(
BuildEnvVariablesDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableBuildEnvVariables()
.getMutableMap()
.put(buildEnvVariables__.getKey(), buildEnvVariables__.getValue());
bitField0_ |= 0x20000000;
break;
} // case 1002
case 1018:
{
serviceAccount_ = input.readStringRequireUtf8();
bitField0_ |= 0x00800000;
break;
} // case 1018
case 1024:
{
appEngineApis_ = input.readBool();
bitField0_ |= 0x00004000;
break;
} // case 1024
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int scalingCase_ = 0;
private java.lang.Object scaling_;
public ScalingCase getScalingCase() {
return ScalingCase.forNumber(scalingCase_);
}
public Builder clearScaling() {
scalingCase_ = 0;
scaling_ = null;
onChanged();
return this;
}
private int bitField0_;
private int bitField1_;
private java.lang.Object name_ = "";
/**
*
*
*
* Full path to the Version resource in the API. Example:
* `apps/myapp/services/default/versions/v1`.
*
* @OutputOnly
*
*
* string name = 1;
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Full path to the Version resource in the API. Example:
* `apps/myapp/services/default/versions/v1`.
*
* @OutputOnly
*
*
* string name = 1;
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Full path to the Version resource in the API. Example:
* `apps/myapp/services/default/versions/v1`.
*
* @OutputOnly
*
*
* string name = 1;
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Full path to the Version resource in the API. Example:
* `apps/myapp/services/default/versions/v1`.
*
* @OutputOnly
*
*
* string name = 1;
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* Full path to the Version resource in the API. Example:
* `apps/myapp/services/default/versions/v1`.
*
* @OutputOnly
*
*
* string name = 1;
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object id_ = "";
/**
*
*
*
* Relative name of the version within the service. Example: `v1`.
* Version names can contain only lowercase letters, numbers, or hyphens.
* Reserved names: "default", "latest", and any name with the prefix "ah-".
*
*
* string id = 2;
*
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Relative name of the version within the service. Example: `v1`.
* Version names can contain only lowercase letters, numbers, or hyphens.
* Reserved names: "default", "latest", and any name with the prefix "ah-".
*
*
* string id = 2;
*
* @return The bytes for id.
*/
public com.google.protobuf.ByteString getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Relative name of the version within the service. Example: `v1`.
* Version names can contain only lowercase letters, numbers, or hyphens.
* Reserved names: "default", "latest", and any name with the prefix "ah-".
*
*
* string id = 2;
*
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Relative name of the version within the service. Example: `v1`.
* Version names can contain only lowercase letters, numbers, or hyphens.
* Reserved names: "default", "latest", and any name with the prefix "ah-".
*
*
* string id = 2;
*
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* Relative name of the version within the service. Example: `v1`.
* Version names can contain only lowercase letters, numbers, or hyphens.
* Reserved names: "default", "latest", and any name with the prefix "ah-".
*
*
* string id = 2;
*
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.AutomaticScaling,
com.google.appengine.v1.AutomaticScaling.Builder,
com.google.appengine.v1.AutomaticScalingOrBuilder>
automaticScalingBuilder_;
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*
* @return Whether the automaticScaling field is set.
*/
@java.lang.Override
public boolean hasAutomaticScaling() {
return scalingCase_ == 3;
}
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*
* @return The automaticScaling.
*/
@java.lang.Override
public com.google.appengine.v1.AutomaticScaling getAutomaticScaling() {
if (automaticScalingBuilder_ == null) {
if (scalingCase_ == 3) {
return (com.google.appengine.v1.AutomaticScaling) scaling_;
}
return com.google.appengine.v1.AutomaticScaling.getDefaultInstance();
} else {
if (scalingCase_ == 3) {
return automaticScalingBuilder_.getMessage();
}
return com.google.appengine.v1.AutomaticScaling.getDefaultInstance();
}
}
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*/
public Builder setAutomaticScaling(com.google.appengine.v1.AutomaticScaling value) {
if (automaticScalingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scaling_ = value;
onChanged();
} else {
automaticScalingBuilder_.setMessage(value);
}
scalingCase_ = 3;
return this;
}
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*/
public Builder setAutomaticScaling(
com.google.appengine.v1.AutomaticScaling.Builder builderForValue) {
if (automaticScalingBuilder_ == null) {
scaling_ = builderForValue.build();
onChanged();
} else {
automaticScalingBuilder_.setMessage(builderForValue.build());
}
scalingCase_ = 3;
return this;
}
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*/
public Builder mergeAutomaticScaling(com.google.appengine.v1.AutomaticScaling value) {
if (automaticScalingBuilder_ == null) {
if (scalingCase_ == 3
&& scaling_ != com.google.appengine.v1.AutomaticScaling.getDefaultInstance()) {
scaling_ =
com.google.appengine.v1.AutomaticScaling.newBuilder(
(com.google.appengine.v1.AutomaticScaling) scaling_)
.mergeFrom(value)
.buildPartial();
} else {
scaling_ = value;
}
onChanged();
} else {
if (scalingCase_ == 3) {
automaticScalingBuilder_.mergeFrom(value);
} else {
automaticScalingBuilder_.setMessage(value);
}
}
scalingCase_ = 3;
return this;
}
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*/
public Builder clearAutomaticScaling() {
if (automaticScalingBuilder_ == null) {
if (scalingCase_ == 3) {
scalingCase_ = 0;
scaling_ = null;
onChanged();
}
} else {
if (scalingCase_ == 3) {
scalingCase_ = 0;
scaling_ = null;
}
automaticScalingBuilder_.clear();
}
return this;
}
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*/
public com.google.appengine.v1.AutomaticScaling.Builder getAutomaticScalingBuilder() {
return getAutomaticScalingFieldBuilder().getBuilder();
}
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*/
@java.lang.Override
public com.google.appengine.v1.AutomaticScalingOrBuilder getAutomaticScalingOrBuilder() {
if ((scalingCase_ == 3) && (automaticScalingBuilder_ != null)) {
return automaticScalingBuilder_.getMessageOrBuilder();
} else {
if (scalingCase_ == 3) {
return (com.google.appengine.v1.AutomaticScaling) scaling_;
}
return com.google.appengine.v1.AutomaticScaling.getDefaultInstance();
}
}
/**
*
*
*
* Automatic scaling is based on request rate, response latencies, and other
* application metrics. Instances are dynamically created and destroyed as
* needed in order to handle traffic.
*
*
* .google.appengine.v1.AutomaticScaling automatic_scaling = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.AutomaticScaling,
com.google.appengine.v1.AutomaticScaling.Builder,
com.google.appengine.v1.AutomaticScalingOrBuilder>
getAutomaticScalingFieldBuilder() {
if (automaticScalingBuilder_ == null) {
if (!(scalingCase_ == 3)) {
scaling_ = com.google.appengine.v1.AutomaticScaling.getDefaultInstance();
}
automaticScalingBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.AutomaticScaling,
com.google.appengine.v1.AutomaticScaling.Builder,
com.google.appengine.v1.AutomaticScalingOrBuilder>(
(com.google.appengine.v1.AutomaticScaling) scaling_,
getParentForChildren(),
isClean());
scaling_ = null;
}
scalingCase_ = 3;
onChanged();
return automaticScalingBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.BasicScaling,
com.google.appengine.v1.BasicScaling.Builder,
com.google.appengine.v1.BasicScalingOrBuilder>
basicScalingBuilder_;
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*
* @return Whether the basicScaling field is set.
*/
@java.lang.Override
public boolean hasBasicScaling() {
return scalingCase_ == 4;
}
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*
* @return The basicScaling.
*/
@java.lang.Override
public com.google.appengine.v1.BasicScaling getBasicScaling() {
if (basicScalingBuilder_ == null) {
if (scalingCase_ == 4) {
return (com.google.appengine.v1.BasicScaling) scaling_;
}
return com.google.appengine.v1.BasicScaling.getDefaultInstance();
} else {
if (scalingCase_ == 4) {
return basicScalingBuilder_.getMessage();
}
return com.google.appengine.v1.BasicScaling.getDefaultInstance();
}
}
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*/
public Builder setBasicScaling(com.google.appengine.v1.BasicScaling value) {
if (basicScalingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scaling_ = value;
onChanged();
} else {
basicScalingBuilder_.setMessage(value);
}
scalingCase_ = 4;
return this;
}
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*/
public Builder setBasicScaling(com.google.appengine.v1.BasicScaling.Builder builderForValue) {
if (basicScalingBuilder_ == null) {
scaling_ = builderForValue.build();
onChanged();
} else {
basicScalingBuilder_.setMessage(builderForValue.build());
}
scalingCase_ = 4;
return this;
}
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*/
public Builder mergeBasicScaling(com.google.appengine.v1.BasicScaling value) {
if (basicScalingBuilder_ == null) {
if (scalingCase_ == 4
&& scaling_ != com.google.appengine.v1.BasicScaling.getDefaultInstance()) {
scaling_ =
com.google.appengine.v1.BasicScaling.newBuilder(
(com.google.appengine.v1.BasicScaling) scaling_)
.mergeFrom(value)
.buildPartial();
} else {
scaling_ = value;
}
onChanged();
} else {
if (scalingCase_ == 4) {
basicScalingBuilder_.mergeFrom(value);
} else {
basicScalingBuilder_.setMessage(value);
}
}
scalingCase_ = 4;
return this;
}
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*/
public Builder clearBasicScaling() {
if (basicScalingBuilder_ == null) {
if (scalingCase_ == 4) {
scalingCase_ = 0;
scaling_ = null;
onChanged();
}
} else {
if (scalingCase_ == 4) {
scalingCase_ = 0;
scaling_ = null;
}
basicScalingBuilder_.clear();
}
return this;
}
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*/
public com.google.appengine.v1.BasicScaling.Builder getBasicScalingBuilder() {
return getBasicScalingFieldBuilder().getBuilder();
}
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*/
@java.lang.Override
public com.google.appengine.v1.BasicScalingOrBuilder getBasicScalingOrBuilder() {
if ((scalingCase_ == 4) && (basicScalingBuilder_ != null)) {
return basicScalingBuilder_.getMessageOrBuilder();
} else {
if (scalingCase_ == 4) {
return (com.google.appengine.v1.BasicScaling) scaling_;
}
return com.google.appengine.v1.BasicScaling.getDefaultInstance();
}
}
/**
*
*
*
* A service with basic scaling will create an instance when the application
* receives a request. The instance will be turned down when the app becomes
* idle. Basic scaling is ideal for work that is intermittent or driven by
* user activity.
*
*
* .google.appengine.v1.BasicScaling basic_scaling = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.BasicScaling,
com.google.appengine.v1.BasicScaling.Builder,
com.google.appengine.v1.BasicScalingOrBuilder>
getBasicScalingFieldBuilder() {
if (basicScalingBuilder_ == null) {
if (!(scalingCase_ == 4)) {
scaling_ = com.google.appengine.v1.BasicScaling.getDefaultInstance();
}
basicScalingBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.BasicScaling,
com.google.appengine.v1.BasicScaling.Builder,
com.google.appengine.v1.BasicScalingOrBuilder>(
(com.google.appengine.v1.BasicScaling) scaling_, getParentForChildren(), isClean());
scaling_ = null;
}
scalingCase_ = 4;
onChanged();
return basicScalingBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.ManualScaling,
com.google.appengine.v1.ManualScaling.Builder,
com.google.appengine.v1.ManualScalingOrBuilder>
manualScalingBuilder_;
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*
* @return Whether the manualScaling field is set.
*/
@java.lang.Override
public boolean hasManualScaling() {
return scalingCase_ == 5;
}
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*
* @return The manualScaling.
*/
@java.lang.Override
public com.google.appengine.v1.ManualScaling getManualScaling() {
if (manualScalingBuilder_ == null) {
if (scalingCase_ == 5) {
return (com.google.appengine.v1.ManualScaling) scaling_;
}
return com.google.appengine.v1.ManualScaling.getDefaultInstance();
} else {
if (scalingCase_ == 5) {
return manualScalingBuilder_.getMessage();
}
return com.google.appengine.v1.ManualScaling.getDefaultInstance();
}
}
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*/
public Builder setManualScaling(com.google.appengine.v1.ManualScaling value) {
if (manualScalingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scaling_ = value;
onChanged();
} else {
manualScalingBuilder_.setMessage(value);
}
scalingCase_ = 5;
return this;
}
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*/
public Builder setManualScaling(com.google.appengine.v1.ManualScaling.Builder builderForValue) {
if (manualScalingBuilder_ == null) {
scaling_ = builderForValue.build();
onChanged();
} else {
manualScalingBuilder_.setMessage(builderForValue.build());
}
scalingCase_ = 5;
return this;
}
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*/
public Builder mergeManualScaling(com.google.appengine.v1.ManualScaling value) {
if (manualScalingBuilder_ == null) {
if (scalingCase_ == 5
&& scaling_ != com.google.appengine.v1.ManualScaling.getDefaultInstance()) {
scaling_ =
com.google.appengine.v1.ManualScaling.newBuilder(
(com.google.appengine.v1.ManualScaling) scaling_)
.mergeFrom(value)
.buildPartial();
} else {
scaling_ = value;
}
onChanged();
} else {
if (scalingCase_ == 5) {
manualScalingBuilder_.mergeFrom(value);
} else {
manualScalingBuilder_.setMessage(value);
}
}
scalingCase_ = 5;
return this;
}
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*/
public Builder clearManualScaling() {
if (manualScalingBuilder_ == null) {
if (scalingCase_ == 5) {
scalingCase_ = 0;
scaling_ = null;
onChanged();
}
} else {
if (scalingCase_ == 5) {
scalingCase_ = 0;
scaling_ = null;
}
manualScalingBuilder_.clear();
}
return this;
}
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*/
public com.google.appengine.v1.ManualScaling.Builder getManualScalingBuilder() {
return getManualScalingFieldBuilder().getBuilder();
}
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*/
@java.lang.Override
public com.google.appengine.v1.ManualScalingOrBuilder getManualScalingOrBuilder() {
if ((scalingCase_ == 5) && (manualScalingBuilder_ != null)) {
return manualScalingBuilder_.getMessageOrBuilder();
} else {
if (scalingCase_ == 5) {
return (com.google.appengine.v1.ManualScaling) scaling_;
}
return com.google.appengine.v1.ManualScaling.getDefaultInstance();
}
}
/**
*
*
*
* A service with manual scaling runs continuously, allowing you to perform
* complex initialization and rely on the state of its memory over time.
* Manually scaled versions are sometimes referred to as "backends".
*
*
* .google.appengine.v1.ManualScaling manual_scaling = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.ManualScaling,
com.google.appengine.v1.ManualScaling.Builder,
com.google.appengine.v1.ManualScalingOrBuilder>
getManualScalingFieldBuilder() {
if (manualScalingBuilder_ == null) {
if (!(scalingCase_ == 5)) {
scaling_ = com.google.appengine.v1.ManualScaling.getDefaultInstance();
}
manualScalingBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.ManualScaling,
com.google.appengine.v1.ManualScaling.Builder,
com.google.appengine.v1.ManualScalingOrBuilder>(
(com.google.appengine.v1.ManualScaling) scaling_,
getParentForChildren(),
isClean());
scaling_ = null;
}
scalingCase_ = 5;
onChanged();
return manualScalingBuilder_;
}
private java.util.List inboundServices_ = java.util.Collections.emptyList();
private void ensureInboundServicesIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
inboundServices_ = new java.util.ArrayList(inboundServices_);
bitField0_ |= 0x00000020;
}
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @return A list containing the inboundServices.
*/
public java.util.List getInboundServicesList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.appengine.v1.InboundServiceType>(
inboundServices_, inboundServices_converter_);
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @return The count of inboundServices.
*/
public int getInboundServicesCount() {
return inboundServices_.size();
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @param index The index of the element to return.
* @return The inboundServices at the given index.
*/
public com.google.appengine.v1.InboundServiceType getInboundServices(int index) {
return inboundServices_converter_.convert(inboundServices_.get(index));
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @param index The index to set the value at.
* @param value The inboundServices to set.
* @return This builder for chaining.
*/
public Builder setInboundServices(int index, com.google.appengine.v1.InboundServiceType value) {
if (value == null) {
throw new NullPointerException();
}
ensureInboundServicesIsMutable();
inboundServices_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @param value The inboundServices to add.
* @return This builder for chaining.
*/
public Builder addInboundServices(com.google.appengine.v1.InboundServiceType value) {
if (value == null) {
throw new NullPointerException();
}
ensureInboundServicesIsMutable();
inboundServices_.add(value.getNumber());
onChanged();
return this;
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @param values The inboundServices to add.
* @return This builder for chaining.
*/
public Builder addAllInboundServices(
java.lang.Iterable extends com.google.appengine.v1.InboundServiceType> values) {
ensureInboundServicesIsMutable();
for (com.google.appengine.v1.InboundServiceType value : values) {
inboundServices_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @return This builder for chaining.
*/
public Builder clearInboundServices() {
inboundServices_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @return A list containing the enum numeric values on the wire for inboundServices.
*/
public java.util.List getInboundServicesValueList() {
return java.util.Collections.unmodifiableList(inboundServices_);
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @param index The index of the value to return.
* @return The enum numeric value on the wire of inboundServices at the given index.
*/
public int getInboundServicesValue(int index) {
return inboundServices_.get(index);
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @param index The index to set the value at.
* @param value The enum numeric value on the wire for inboundServices to set.
* @return This builder for chaining.
*/
public Builder setInboundServicesValue(int index, int value) {
ensureInboundServicesIsMutable();
inboundServices_.set(index, value);
onChanged();
return this;
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @param value The enum numeric value on the wire for inboundServices to add.
* @return This builder for chaining.
*/
public Builder addInboundServicesValue(int value) {
ensureInboundServicesIsMutable();
inboundServices_.add(value);
onChanged();
return this;
}
/**
*
*
*
* Before an application can receive email or XMPP messages, the application
* must be configured to enable the service.
*
*
* repeated .google.appengine.v1.InboundServiceType inbound_services = 6;
*
* @param values The enum numeric values on the wire for inboundServices to add.
* @return This builder for chaining.
*/
public Builder addAllInboundServicesValue(java.lang.Iterable values) {
ensureInboundServicesIsMutable();
for (int value : values) {
inboundServices_.add(value);
}
onChanged();
return this;
}
private java.lang.Object instanceClass_ = "";
/**
*
*
*
* Instance class that is used to run this version. Valid values are:
*
* * AutomaticScaling: `F1`, `F2`, `F4`, `F4_1G`
* * ManualScaling or BasicScaling: `B1`, `B2`, `B4`, `B8`, `B4_1G`
*
* Defaults to `F1` for AutomaticScaling and `B1` for ManualScaling or
* BasicScaling.
*
*
* string instance_class = 7;
*
* @return The instanceClass.
*/
public java.lang.String getInstanceClass() {
java.lang.Object ref = instanceClass_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
instanceClass_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Instance class that is used to run this version. Valid values are:
*
* * AutomaticScaling: `F1`, `F2`, `F4`, `F4_1G`
* * ManualScaling or BasicScaling: `B1`, `B2`, `B4`, `B8`, `B4_1G`
*
* Defaults to `F1` for AutomaticScaling and `B1` for ManualScaling or
* BasicScaling.
*
*
* string instance_class = 7;
*
* @return The bytes for instanceClass.
*/
public com.google.protobuf.ByteString getInstanceClassBytes() {
java.lang.Object ref = instanceClass_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
instanceClass_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Instance class that is used to run this version. Valid values are:
*
* * AutomaticScaling: `F1`, `F2`, `F4`, `F4_1G`
* * ManualScaling or BasicScaling: `B1`, `B2`, `B4`, `B8`, `B4_1G`
*
* Defaults to `F1` for AutomaticScaling and `B1` for ManualScaling or
* BasicScaling.
*
*
* string instance_class = 7;
*
* @param value The instanceClass to set.
* @return This builder for chaining.
*/
public Builder setInstanceClass(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
instanceClass_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Instance class that is used to run this version. Valid values are:
*
* * AutomaticScaling: `F1`, `F2`, `F4`, `F4_1G`
* * ManualScaling or BasicScaling: `B1`, `B2`, `B4`, `B8`, `B4_1G`
*
* Defaults to `F1` for AutomaticScaling and `B1` for ManualScaling or
* BasicScaling.
*
*
* string instance_class = 7;
*
* @return This builder for chaining.
*/
public Builder clearInstanceClass() {
instanceClass_ = getDefaultInstance().getInstanceClass();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* Instance class that is used to run this version. Valid values are:
*
* * AutomaticScaling: `F1`, `F2`, `F4`, `F4_1G`
* * ManualScaling or BasicScaling: `B1`, `B2`, `B4`, `B8`, `B4_1G`
*
* Defaults to `F1` for AutomaticScaling and `B1` for ManualScaling or
* BasicScaling.
*
*
* string instance_class = 7;
*
* @param value The bytes for instanceClass to set.
* @return This builder for chaining.
*/
public Builder setInstanceClassBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
instanceClass_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private com.google.appengine.v1.Network network_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Network,
com.google.appengine.v1.Network.Builder,
com.google.appengine.v1.NetworkOrBuilder>
networkBuilder_;
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*
* @return Whether the network field is set.
*/
public boolean hasNetwork() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*
* @return The network.
*/
public com.google.appengine.v1.Network getNetwork() {
if (networkBuilder_ == null) {
return network_ == null ? com.google.appengine.v1.Network.getDefaultInstance() : network_;
} else {
return networkBuilder_.getMessage();
}
}
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*/
public Builder setNetwork(com.google.appengine.v1.Network value) {
if (networkBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
network_ = value;
} else {
networkBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*/
public Builder setNetwork(com.google.appengine.v1.Network.Builder builderForValue) {
if (networkBuilder_ == null) {
network_ = builderForValue.build();
} else {
networkBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*/
public Builder mergeNetwork(com.google.appengine.v1.Network value) {
if (networkBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)
&& network_ != null
&& network_ != com.google.appengine.v1.Network.getDefaultInstance()) {
getNetworkBuilder().mergeFrom(value);
} else {
network_ = value;
}
} else {
networkBuilder_.mergeFrom(value);
}
if (network_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*/
public Builder clearNetwork() {
bitField0_ = (bitField0_ & ~0x00000080);
network_ = null;
if (networkBuilder_ != null) {
networkBuilder_.dispose();
networkBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*/
public com.google.appengine.v1.Network.Builder getNetworkBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getNetworkFieldBuilder().getBuilder();
}
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*/
public com.google.appengine.v1.NetworkOrBuilder getNetworkOrBuilder() {
if (networkBuilder_ != null) {
return networkBuilder_.getMessageOrBuilder();
} else {
return network_ == null ? com.google.appengine.v1.Network.getDefaultInstance() : network_;
}
}
/**
*
*
*
* Extra network settings.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Network network = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Network,
com.google.appengine.v1.Network.Builder,
com.google.appengine.v1.NetworkOrBuilder>
getNetworkFieldBuilder() {
if (networkBuilder_ == null) {
networkBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Network,
com.google.appengine.v1.Network.Builder,
com.google.appengine.v1.NetworkOrBuilder>(
getNetwork(), getParentForChildren(), isClean());
network_ = null;
}
return networkBuilder_;
}
private com.google.protobuf.LazyStringArrayList zones_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureZonesIsMutable() {
if (!zones_.isModifiable()) {
zones_ = new com.google.protobuf.LazyStringArrayList(zones_);
}
bitField0_ |= 0x00000100;
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @return A list containing the zones.
*/
public com.google.protobuf.ProtocolStringList getZonesList() {
zones_.makeImmutable();
return zones_;
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @return The count of zones.
*/
public int getZonesCount() {
return zones_.size();
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @param index The index of the element to return.
* @return The zones at the given index.
*/
public java.lang.String getZones(int index) {
return zones_.get(index);
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @param index The index of the value to return.
* @return The bytes of the zones at the given index.
*/
public com.google.protobuf.ByteString getZonesBytes(int index) {
return zones_.getByteString(index);
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @param index The index to set the value at.
* @param value The zones to set.
* @return This builder for chaining.
*/
public Builder setZones(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureZonesIsMutable();
zones_.set(index, value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @param value The zones to add.
* @return This builder for chaining.
*/
public Builder addZones(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureZonesIsMutable();
zones_.add(value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @param values The zones to add.
* @return This builder for chaining.
*/
public Builder addAllZones(java.lang.Iterable values) {
ensureZonesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, zones_);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @return This builder for chaining.
*/
public Builder clearZones() {
zones_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
;
onChanged();
return this;
}
/**
*
*
*
* The Google Compute Engine zones that are supported by this version in the
* App Engine flexible environment. Deprecated.
*
*
* repeated string zones = 118;
*
* @param value The bytes of the zones to add.
* @return This builder for chaining.
*/
public Builder addZonesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureZonesIsMutable();
zones_.add(value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private com.google.appengine.v1.Resources resources_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Resources,
com.google.appengine.v1.Resources.Builder,
com.google.appengine.v1.ResourcesOrBuilder>
resourcesBuilder_;
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*
* @return Whether the resources field is set.
*/
public boolean hasResources() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*
* @return The resources.
*/
public com.google.appengine.v1.Resources getResources() {
if (resourcesBuilder_ == null) {
return resources_ == null
? com.google.appengine.v1.Resources.getDefaultInstance()
: resources_;
} else {
return resourcesBuilder_.getMessage();
}
}
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*/
public Builder setResources(com.google.appengine.v1.Resources value) {
if (resourcesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resources_ = value;
} else {
resourcesBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*/
public Builder setResources(com.google.appengine.v1.Resources.Builder builderForValue) {
if (resourcesBuilder_ == null) {
resources_ = builderForValue.build();
} else {
resourcesBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*/
public Builder mergeResources(com.google.appengine.v1.Resources value) {
if (resourcesBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)
&& resources_ != null
&& resources_ != com.google.appengine.v1.Resources.getDefaultInstance()) {
getResourcesBuilder().mergeFrom(value);
} else {
resources_ = value;
}
} else {
resourcesBuilder_.mergeFrom(value);
}
if (resources_ != null) {
bitField0_ |= 0x00000200;
onChanged();
}
return this;
}
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*/
public Builder clearResources() {
bitField0_ = (bitField0_ & ~0x00000200);
resources_ = null;
if (resourcesBuilder_ != null) {
resourcesBuilder_.dispose();
resourcesBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*/
public com.google.appengine.v1.Resources.Builder getResourcesBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getResourcesFieldBuilder().getBuilder();
}
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*/
public com.google.appengine.v1.ResourcesOrBuilder getResourcesOrBuilder() {
if (resourcesBuilder_ != null) {
return resourcesBuilder_.getMessageOrBuilder();
} else {
return resources_ == null
? com.google.appengine.v1.Resources.getDefaultInstance()
: resources_;
}
}
/**
*
*
*
* Machine resources for this version.
* Only applicable in the App Engine flexible environment.
*
*
* .google.appengine.v1.Resources resources = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Resources,
com.google.appengine.v1.Resources.Builder,
com.google.appengine.v1.ResourcesOrBuilder>
getResourcesFieldBuilder() {
if (resourcesBuilder_ == null) {
resourcesBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Resources,
com.google.appengine.v1.Resources.Builder,
com.google.appengine.v1.ResourcesOrBuilder>(
getResources(), getParentForChildren(), isClean());
resources_ = null;
}
return resourcesBuilder_;
}
private java.lang.Object runtime_ = "";
/**
*
*
*
* Desired runtime. Example: `python27`.
*
*
* string runtime = 10;
*
* @return The runtime.
*/
public java.lang.String getRuntime() {
java.lang.Object ref = runtime_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runtime_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Desired runtime. Example: `python27`.
*
*
* string runtime = 10;
*
* @return The bytes for runtime.
*/
public com.google.protobuf.ByteString getRuntimeBytes() {
java.lang.Object ref = runtime_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
runtime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Desired runtime. Example: `python27`.
*
*
* string runtime = 10;
*
* @param value The runtime to set.
* @return This builder for chaining.
*/
public Builder setRuntime(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
runtime_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Desired runtime. Example: `python27`.
*
*
* string runtime = 10;
*
* @return This builder for chaining.
*/
public Builder clearRuntime() {
runtime_ = getDefaultInstance().getRuntime();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
return this;
}
/**
*
*
*
* Desired runtime. Example: `python27`.
*
*
* string runtime = 10;
*
* @param value The bytes for runtime to set.
* @return This builder for chaining.
*/
public Builder setRuntimeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
runtime_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
private java.lang.Object runtimeChannel_ = "";
/**
*
*
*
* The channel of the runtime to use. Only available for some
* runtimes. Defaults to the `default` channel.
*
*
* string runtime_channel = 117;
*
* @return The runtimeChannel.
*/
public java.lang.String getRuntimeChannel() {
java.lang.Object ref = runtimeChannel_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runtimeChannel_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The channel of the runtime to use. Only available for some
* runtimes. Defaults to the `default` channel.
*
*
* string runtime_channel = 117;
*
* @return The bytes for runtimeChannel.
*/
public com.google.protobuf.ByteString getRuntimeChannelBytes() {
java.lang.Object ref = runtimeChannel_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
runtimeChannel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The channel of the runtime to use. Only available for some
* runtimes. Defaults to the `default` channel.
*
*
* string runtime_channel = 117;
*
* @param value The runtimeChannel to set.
* @return This builder for chaining.
*/
public Builder setRuntimeChannel(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
runtimeChannel_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* The channel of the runtime to use. Only available for some
* runtimes. Defaults to the `default` channel.
*
*
* string runtime_channel = 117;
*
* @return This builder for chaining.
*/
public Builder clearRuntimeChannel() {
runtimeChannel_ = getDefaultInstance().getRuntimeChannel();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
/**
*
*
*
* The channel of the runtime to use. Only available for some
* runtimes. Defaults to the `default` channel.
*
*
* string runtime_channel = 117;
*
* @param value The bytes for runtimeChannel to set.
* @return This builder for chaining.
*/
public Builder setRuntimeChannelBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
runtimeChannel_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
private boolean threadsafe_;
/**
*
*
*
* Whether multiple requests can be dispatched to this version at once.
*
*
* bool threadsafe = 11;
*
* @return The threadsafe.
*/
@java.lang.Override
public boolean getThreadsafe() {
return threadsafe_;
}
/**
*
*
*
* Whether multiple requests can be dispatched to this version at once.
*
*
* bool threadsafe = 11;
*
* @param value The threadsafe to set.
* @return This builder for chaining.
*/
public Builder setThreadsafe(boolean value) {
threadsafe_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* Whether multiple requests can be dispatched to this version at once.
*
*
* bool threadsafe = 11;
*
* @return This builder for chaining.
*/
public Builder clearThreadsafe() {
bitField0_ = (bitField0_ & ~0x00001000);
threadsafe_ = false;
onChanged();
return this;
}
private boolean vm_;
/**
*
*
*
* Whether to deploy this version in a container on a virtual machine.
*
*
* bool vm = 12;
*
* @return The vm.
*/
@java.lang.Override
public boolean getVm() {
return vm_;
}
/**
*
*
*
* Whether to deploy this version in a container on a virtual machine.
*
*
* bool vm = 12;
*
* @param value The vm to set.
* @return This builder for chaining.
*/
public Builder setVm(boolean value) {
vm_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* Whether to deploy this version in a container on a virtual machine.
*
*
* bool vm = 12;
*
* @return This builder for chaining.
*/
public Builder clearVm() {
bitField0_ = (bitField0_ & ~0x00002000);
vm_ = false;
onChanged();
return this;
}
private boolean appEngineApis_;
/**
*
*
*
* Allows App Engine second generation runtimes to access the legacy bundled
* services.
*
*
* bool app_engine_apis = 128;
*
* @return The appEngineApis.
*/
@java.lang.Override
public boolean getAppEngineApis() {
return appEngineApis_;
}
/**
*
*
*
* Allows App Engine second generation runtimes to access the legacy bundled
* services.
*
*
* bool app_engine_apis = 128;
*
* @param value The appEngineApis to set.
* @return This builder for chaining.
*/
public Builder setAppEngineApis(boolean value) {
appEngineApis_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* Allows App Engine second generation runtimes to access the legacy bundled
* services.
*
*
* bool app_engine_apis = 128;
*
* @return This builder for chaining.
*/
public Builder clearAppEngineApis() {
bitField0_ = (bitField0_ & ~0x00004000);
appEngineApis_ = false;
onChanged();
return this;
}
private com.google.protobuf.MapField betaSettings_;
private com.google.protobuf.MapField
internalGetBetaSettings() {
if (betaSettings_ == null) {
return com.google.protobuf.MapField.emptyMapField(
BetaSettingsDefaultEntryHolder.defaultEntry);
}
return betaSettings_;
}
private com.google.protobuf.MapField
internalGetMutableBetaSettings() {
if (betaSettings_ == null) {
betaSettings_ =
com.google.protobuf.MapField.newMapField(BetaSettingsDefaultEntryHolder.defaultEntry);
}
if (!betaSettings_.isMutable()) {
betaSettings_ = betaSettings_.copy();
}
bitField0_ |= 0x00008000;
onChanged();
return betaSettings_;
}
public int getBetaSettingsCount() {
return internalGetBetaSettings().getMap().size();
}
/**
*
*
*
* Metadata settings that are supplied to this version to enable
* beta runtime features.
*
*
* map<string, string> beta_settings = 13;
*/
@java.lang.Override
public boolean containsBetaSettings(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetBetaSettings().getMap().containsKey(key);
}
/** Use {@link #getBetaSettingsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getBetaSettings() {
return getBetaSettingsMap();
}
/**
*
*
*
* Metadata settings that are supplied to this version to enable
* beta runtime features.
*
*
* map<string, string> beta_settings = 13;
*/
@java.lang.Override
public java.util.Map getBetaSettingsMap() {
return internalGetBetaSettings().getMap();
}
/**
*
*
*
* Metadata settings that are supplied to this version to enable
* beta runtime features.
*
*
* map<string, string> beta_settings = 13;
*/
@java.lang.Override
public /* nullable */ java.lang.String getBetaSettingsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetBetaSettings().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Metadata settings that are supplied to this version to enable
* beta runtime features.
*
*
* map<string, string> beta_settings = 13;
*/
@java.lang.Override
public java.lang.String getBetaSettingsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetBetaSettings().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearBetaSettings() {
bitField0_ = (bitField0_ & ~0x00008000);
internalGetMutableBetaSettings().getMutableMap().clear();
return this;
}
/**
*
*
*
* Metadata settings that are supplied to this version to enable
* beta runtime features.
*
*
* map<string, string> beta_settings = 13;
*/
public Builder removeBetaSettings(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableBetaSettings().getMutableMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map getMutableBetaSettings() {
bitField0_ |= 0x00008000;
return internalGetMutableBetaSettings().getMutableMap();
}
/**
*
*
*
* Metadata settings that are supplied to this version to enable
* beta runtime features.
*
*
* map<string, string> beta_settings = 13;
*/
public Builder putBetaSettings(java.lang.String key, java.lang.String value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableBetaSettings().getMutableMap().put(key, value);
bitField0_ |= 0x00008000;
return this;
}
/**
*
*
*
* Metadata settings that are supplied to this version to enable
* beta runtime features.
*
*
* map<string, string> beta_settings = 13;
*/
public Builder putAllBetaSettings(java.util.Map values) {
internalGetMutableBetaSettings().getMutableMap().putAll(values);
bitField0_ |= 0x00008000;
return this;
}
private java.lang.Object env_ = "";
/**
*
*
*
* App Engine execution environment for this version.
*
* Defaults to `standard`.
*
*
* string env = 14;
*
* @return The env.
*/
public java.lang.String getEnv() {
java.lang.Object ref = env_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
env_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* App Engine execution environment for this version.
*
* Defaults to `standard`.
*
*
* string env = 14;
*
* @return The bytes for env.
*/
public com.google.protobuf.ByteString getEnvBytes() {
java.lang.Object ref = env_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
env_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* App Engine execution environment for this version.
*
* Defaults to `standard`.
*
*
* string env = 14;
*
* @param value The env to set.
* @return This builder for chaining.
*/
public Builder setEnv(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
env_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* App Engine execution environment for this version.
*
* Defaults to `standard`.
*
*
* string env = 14;
*
* @return This builder for chaining.
*/
public Builder clearEnv() {
env_ = getDefaultInstance().getEnv();
bitField0_ = (bitField0_ & ~0x00010000);
onChanged();
return this;
}
/**
*
*
*
* App Engine execution environment for this version.
*
* Defaults to `standard`.
*
*
* string env = 14;
*
* @param value The bytes for env to set.
* @return This builder for chaining.
*/
public Builder setEnvBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
env_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
private int servingStatus_ = 0;
/**
*
*
*
* Current serving status of this version. Only the versions with a
* `SERVING` status create instances and can be billed.
*
* `SERVING_STATUS_UNSPECIFIED` is an invalid value. Defaults to `SERVING`.
*
*
* .google.appengine.v1.ServingStatus serving_status = 15;
*
* @return The enum numeric value on the wire for servingStatus.
*/
@java.lang.Override
public int getServingStatusValue() {
return servingStatus_;
}
/**
*
*
*
* Current serving status of this version. Only the versions with a
* `SERVING` status create instances and can be billed.
*
* `SERVING_STATUS_UNSPECIFIED` is an invalid value. Defaults to `SERVING`.
*
*
* .google.appengine.v1.ServingStatus serving_status = 15;
*
* @param value The enum numeric value on the wire for servingStatus to set.
* @return This builder for chaining.
*/
public Builder setServingStatusValue(int value) {
servingStatus_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
*
*
* Current serving status of this version. Only the versions with a
* `SERVING` status create instances and can be billed.
*
* `SERVING_STATUS_UNSPECIFIED` is an invalid value. Defaults to `SERVING`.
*
*
* .google.appengine.v1.ServingStatus serving_status = 15;
*
* @return The servingStatus.
*/
@java.lang.Override
public com.google.appengine.v1.ServingStatus getServingStatus() {
com.google.appengine.v1.ServingStatus result =
com.google.appengine.v1.ServingStatus.forNumber(servingStatus_);
return result == null ? com.google.appengine.v1.ServingStatus.UNRECOGNIZED : result;
}
/**
*
*
*
* Current serving status of this version. Only the versions with a
* `SERVING` status create instances and can be billed.
*
* `SERVING_STATUS_UNSPECIFIED` is an invalid value. Defaults to `SERVING`.
*
*
* .google.appengine.v1.ServingStatus serving_status = 15;
*
* @param value The servingStatus to set.
* @return This builder for chaining.
*/
public Builder setServingStatus(com.google.appengine.v1.ServingStatus value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
servingStatus_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
*
* Current serving status of this version. Only the versions with a
* `SERVING` status create instances and can be billed.
*
* `SERVING_STATUS_UNSPECIFIED` is an invalid value. Defaults to `SERVING`.
*
*
* .google.appengine.v1.ServingStatus serving_status = 15;
*
* @return This builder for chaining.
*/
public Builder clearServingStatus() {
bitField0_ = (bitField0_ & ~0x00020000);
servingStatus_ = 0;
onChanged();
return this;
}
private java.lang.Object createdBy_ = "";
/**
*
*
*
* Email address of the user who created this version.
*
* @OutputOnly
*
*
* string created_by = 16;
*
* @return The createdBy.
*/
public java.lang.String getCreatedBy() {
java.lang.Object ref = createdBy_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
createdBy_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Email address of the user who created this version.
*
* @OutputOnly
*
*
* string created_by = 16;
*
* @return The bytes for createdBy.
*/
public com.google.protobuf.ByteString getCreatedByBytes() {
java.lang.Object ref = createdBy_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
createdBy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Email address of the user who created this version.
*
* @OutputOnly
*
*
* string created_by = 16;
*
* @param value The createdBy to set.
* @return This builder for chaining.
*/
public Builder setCreatedBy(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
createdBy_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
*
*
* Email address of the user who created this version.
*
* @OutputOnly
*
*
* string created_by = 16;
*
* @return This builder for chaining.
*/
public Builder clearCreatedBy() {
createdBy_ = getDefaultInstance().getCreatedBy();
bitField0_ = (bitField0_ & ~0x00040000);
onChanged();
return this;
}
/**
*
*
*
* Email address of the user who created this version.
*
* @OutputOnly
*
*
* string created_by = 16;
*
* @param value The bytes for createdBy to set.
* @return This builder for chaining.
*/
public Builder setCreatedByBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
createdBy_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
private com.google.protobuf.Timestamp createTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
createTimeBuilder_;
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*
* @return Whether the createTime field is set.
*/
public boolean hasCreateTime() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*
* @return The createTime.
*/
public com.google.protobuf.Timestamp getCreateTime() {
if (createTimeBuilder_ == null) {
return createTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: createTime_;
} else {
return createTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*/
public Builder setCreateTime(com.google.protobuf.Timestamp value) {
if (createTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
createTime_ = value;
} else {
createTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*/
public Builder setCreateTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (createTimeBuilder_ == null) {
createTime_ = builderForValue.build();
} else {
createTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*/
public Builder mergeCreateTime(com.google.protobuf.Timestamp value) {
if (createTimeBuilder_ == null) {
if (((bitField0_ & 0x00080000) != 0)
&& createTime_ != null
&& createTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getCreateTimeBuilder().mergeFrom(value);
} else {
createTime_ = value;
}
} else {
createTimeBuilder_.mergeFrom(value);
}
if (createTime_ != null) {
bitField0_ |= 0x00080000;
onChanged();
}
return this;
}
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*/
public Builder clearCreateTime() {
bitField0_ = (bitField0_ & ~0x00080000);
createTime_ = null;
if (createTimeBuilder_ != null) {
createTimeBuilder_.dispose();
createTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*/
public com.google.protobuf.Timestamp.Builder getCreateTimeBuilder() {
bitField0_ |= 0x00080000;
onChanged();
return getCreateTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*/
public com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder() {
if (createTimeBuilder_ != null) {
return createTimeBuilder_.getMessageOrBuilder();
} else {
return createTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: createTime_;
}
}
/**
*
*
*
* Time that this version was created.
*
* @OutputOnly
*
*
* .google.protobuf.Timestamp create_time = 17;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getCreateTimeFieldBuilder() {
if (createTimeBuilder_ == null) {
createTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getCreateTime(), getParentForChildren(), isClean());
createTime_ = null;
}
return createTimeBuilder_;
}
private long diskUsageBytes_;
/**
*
*
*
* Total size in bytes of all the files that are included in this version
* and currently hosted on the App Engine disk.
*
* @OutputOnly
*
*
* int64 disk_usage_bytes = 18;
*
* @return The diskUsageBytes.
*/
@java.lang.Override
public long getDiskUsageBytes() {
return diskUsageBytes_;
}
/**
*
*
*
* Total size in bytes of all the files that are included in this version
* and currently hosted on the App Engine disk.
*
* @OutputOnly
*
*
* int64 disk_usage_bytes = 18;
*
* @param value The diskUsageBytes to set.
* @return This builder for chaining.
*/
public Builder setDiskUsageBytes(long value) {
diskUsageBytes_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
*
*
* Total size in bytes of all the files that are included in this version
* and currently hosted on the App Engine disk.
*
* @OutputOnly
*
*
* int64 disk_usage_bytes = 18;
*
* @return This builder for chaining.
*/
public Builder clearDiskUsageBytes() {
bitField0_ = (bitField0_ & ~0x00100000);
diskUsageBytes_ = 0L;
onChanged();
return this;
}
private java.lang.Object runtimeApiVersion_ = "";
/**
*
*
*
* The version of the API in the given runtime environment. Please see the
* app.yaml reference for valid values at
* https://cloud.google.com/appengine/docs/standard/<language>/config/appref
*
*
* string runtime_api_version = 21;
*
* @return The runtimeApiVersion.
*/
public java.lang.String getRuntimeApiVersion() {
java.lang.Object ref = runtimeApiVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runtimeApiVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The version of the API in the given runtime environment. Please see the
* app.yaml reference for valid values at
* https://cloud.google.com/appengine/docs/standard/<language>/config/appref
*
*
* string runtime_api_version = 21;
*
* @return The bytes for runtimeApiVersion.
*/
public com.google.protobuf.ByteString getRuntimeApiVersionBytes() {
java.lang.Object ref = runtimeApiVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
runtimeApiVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The version of the API in the given runtime environment. Please see the
* app.yaml reference for valid values at
* https://cloud.google.com/appengine/docs/standard/<language>/config/appref
*
*
* string runtime_api_version = 21;
*
* @param value The runtimeApiVersion to set.
* @return This builder for chaining.
*/
public Builder setRuntimeApiVersion(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
runtimeApiVersion_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
*
*
* The version of the API in the given runtime environment. Please see the
* app.yaml reference for valid values at
* https://cloud.google.com/appengine/docs/standard/<language>/config/appref
*
*
* string runtime_api_version = 21;
*
* @return This builder for chaining.
*/
public Builder clearRuntimeApiVersion() {
runtimeApiVersion_ = getDefaultInstance().getRuntimeApiVersion();
bitField0_ = (bitField0_ & ~0x00200000);
onChanged();
return this;
}
/**
*
*
*
* The version of the API in the given runtime environment. Please see the
* app.yaml reference for valid values at
* https://cloud.google.com/appengine/docs/standard/<language>/config/appref
*
*
* string runtime_api_version = 21;
*
* @param value The bytes for runtimeApiVersion to set.
* @return This builder for chaining.
*/
public Builder setRuntimeApiVersionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
runtimeApiVersion_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
private java.lang.Object runtimeMainExecutablePath_ = "";
/**
*
*
*
* The path or name of the app's main executable.
*
*
* string runtime_main_executable_path = 22;
*
* @return The runtimeMainExecutablePath.
*/
public java.lang.String getRuntimeMainExecutablePath() {
java.lang.Object ref = runtimeMainExecutablePath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runtimeMainExecutablePath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The path or name of the app's main executable.
*
*
* string runtime_main_executable_path = 22;
*
* @return The bytes for runtimeMainExecutablePath.
*/
public com.google.protobuf.ByteString getRuntimeMainExecutablePathBytes() {
java.lang.Object ref = runtimeMainExecutablePath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
runtimeMainExecutablePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The path or name of the app's main executable.
*
*
* string runtime_main_executable_path = 22;
*
* @param value The runtimeMainExecutablePath to set.
* @return This builder for chaining.
*/
public Builder setRuntimeMainExecutablePath(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
runtimeMainExecutablePath_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
*
*
* The path or name of the app's main executable.
*
*
* string runtime_main_executable_path = 22;
*
* @return This builder for chaining.
*/
public Builder clearRuntimeMainExecutablePath() {
runtimeMainExecutablePath_ = getDefaultInstance().getRuntimeMainExecutablePath();
bitField0_ = (bitField0_ & ~0x00400000);
onChanged();
return this;
}
/**
*
*
*
* The path or name of the app's main executable.
*
*
* string runtime_main_executable_path = 22;
*
* @param value The bytes for runtimeMainExecutablePath to set.
* @return This builder for chaining.
*/
public Builder setRuntimeMainExecutablePathBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
runtimeMainExecutablePath_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
private java.lang.Object serviceAccount_ = "";
/**
*
*
*
* The identity that the deployed version will run as.
* Admin API will use the App Engine Appspot service account as default if
* this field is neither provided in app.yaml file nor through CLI flag.
*
*
* string service_account = 127;
*
* @return The serviceAccount.
*/
public java.lang.String getServiceAccount() {
java.lang.Object ref = serviceAccount_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serviceAccount_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The identity that the deployed version will run as.
* Admin API will use the App Engine Appspot service account as default if
* this field is neither provided in app.yaml file nor through CLI flag.
*
*
* string service_account = 127;
*
* @return The bytes for serviceAccount.
*/
public com.google.protobuf.ByteString getServiceAccountBytes() {
java.lang.Object ref = serviceAccount_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
serviceAccount_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The identity that the deployed version will run as.
* Admin API will use the App Engine Appspot service account as default if
* this field is neither provided in app.yaml file nor through CLI flag.
*
*
* string service_account = 127;
*
* @param value The serviceAccount to set.
* @return This builder for chaining.
*/
public Builder setServiceAccount(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
serviceAccount_ = value;
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
*
*
* The identity that the deployed version will run as.
* Admin API will use the App Engine Appspot service account as default if
* this field is neither provided in app.yaml file nor through CLI flag.
*
*
* string service_account = 127;
*
* @return This builder for chaining.
*/
public Builder clearServiceAccount() {
serviceAccount_ = getDefaultInstance().getServiceAccount();
bitField0_ = (bitField0_ & ~0x00800000);
onChanged();
return this;
}
/**
*
*
*
* The identity that the deployed version will run as.
* Admin API will use the App Engine Appspot service account as default if
* this field is neither provided in app.yaml file nor through CLI flag.
*
*
* string service_account = 127;
*
* @param value The bytes for serviceAccount to set.
* @return This builder for chaining.
*/
public Builder setServiceAccountBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
serviceAccount_ = value;
bitField0_ |= 0x00800000;
onChanged();
return this;
}
private java.util.List handlers_ =
java.util.Collections.emptyList();
private void ensureHandlersIsMutable() {
if (!((bitField0_ & 0x01000000) != 0)) {
handlers_ = new java.util.ArrayList(handlers_);
bitField0_ |= 0x01000000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.v1.UrlMap,
com.google.appengine.v1.UrlMap.Builder,
com.google.appengine.v1.UrlMapOrBuilder>
handlersBuilder_;
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public java.util.List getHandlersList() {
if (handlersBuilder_ == null) {
return java.util.Collections.unmodifiableList(handlers_);
} else {
return handlersBuilder_.getMessageList();
}
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public int getHandlersCount() {
if (handlersBuilder_ == null) {
return handlers_.size();
} else {
return handlersBuilder_.getCount();
}
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public com.google.appengine.v1.UrlMap getHandlers(int index) {
if (handlersBuilder_ == null) {
return handlers_.get(index);
} else {
return handlersBuilder_.getMessage(index);
}
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public Builder setHandlers(int index, com.google.appengine.v1.UrlMap value) {
if (handlersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHandlersIsMutable();
handlers_.set(index, value);
onChanged();
} else {
handlersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public Builder setHandlers(int index, com.google.appengine.v1.UrlMap.Builder builderForValue) {
if (handlersBuilder_ == null) {
ensureHandlersIsMutable();
handlers_.set(index, builderForValue.build());
onChanged();
} else {
handlersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public Builder addHandlers(com.google.appengine.v1.UrlMap value) {
if (handlersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHandlersIsMutable();
handlers_.add(value);
onChanged();
} else {
handlersBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public Builder addHandlers(int index, com.google.appengine.v1.UrlMap value) {
if (handlersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHandlersIsMutable();
handlers_.add(index, value);
onChanged();
} else {
handlersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public Builder addHandlers(com.google.appengine.v1.UrlMap.Builder builderForValue) {
if (handlersBuilder_ == null) {
ensureHandlersIsMutable();
handlers_.add(builderForValue.build());
onChanged();
} else {
handlersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public Builder addHandlers(int index, com.google.appengine.v1.UrlMap.Builder builderForValue) {
if (handlersBuilder_ == null) {
ensureHandlersIsMutable();
handlers_.add(index, builderForValue.build());
onChanged();
} else {
handlersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public Builder addAllHandlers(
java.lang.Iterable extends com.google.appengine.v1.UrlMap> values) {
if (handlersBuilder_ == null) {
ensureHandlersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, handlers_);
onChanged();
} else {
handlersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public Builder clearHandlers() {
if (handlersBuilder_ == null) {
handlers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x01000000);
onChanged();
} else {
handlersBuilder_.clear();
}
return this;
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public Builder removeHandlers(int index) {
if (handlersBuilder_ == null) {
ensureHandlersIsMutable();
handlers_.remove(index);
onChanged();
} else {
handlersBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public com.google.appengine.v1.UrlMap.Builder getHandlersBuilder(int index) {
return getHandlersFieldBuilder().getBuilder(index);
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public com.google.appengine.v1.UrlMapOrBuilder getHandlersOrBuilder(int index) {
if (handlersBuilder_ == null) {
return handlers_.get(index);
} else {
return handlersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public java.util.List extends com.google.appengine.v1.UrlMapOrBuilder>
getHandlersOrBuilderList() {
if (handlersBuilder_ != null) {
return handlersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(handlers_);
}
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public com.google.appengine.v1.UrlMap.Builder addHandlersBuilder() {
return getHandlersFieldBuilder()
.addBuilder(com.google.appengine.v1.UrlMap.getDefaultInstance());
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public com.google.appengine.v1.UrlMap.Builder addHandlersBuilder(int index) {
return getHandlersFieldBuilder()
.addBuilder(index, com.google.appengine.v1.UrlMap.getDefaultInstance());
}
/**
*
*
*
* An ordered list of URL-matching patterns that should be applied to incoming
* requests. The first matching URL handles the request and other request
* handlers are not attempted.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.UrlMap handlers = 100;
*/
public java.util.List getHandlersBuilderList() {
return getHandlersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.v1.UrlMap,
com.google.appengine.v1.UrlMap.Builder,
com.google.appengine.v1.UrlMapOrBuilder>
getHandlersFieldBuilder() {
if (handlersBuilder_ == null) {
handlersBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.v1.UrlMap,
com.google.appengine.v1.UrlMap.Builder,
com.google.appengine.v1.UrlMapOrBuilder>(
handlers_, ((bitField0_ & 0x01000000) != 0), getParentForChildren(), isClean());
handlers_ = null;
}
return handlersBuilder_;
}
private java.util.List errorHandlers_ =
java.util.Collections.emptyList();
private void ensureErrorHandlersIsMutable() {
if (!((bitField0_ & 0x02000000) != 0)) {
errorHandlers_ =
new java.util.ArrayList(errorHandlers_);
bitField0_ |= 0x02000000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.v1.ErrorHandler,
com.google.appengine.v1.ErrorHandler.Builder,
com.google.appengine.v1.ErrorHandlerOrBuilder>
errorHandlersBuilder_;
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public java.util.List getErrorHandlersList() {
if (errorHandlersBuilder_ == null) {
return java.util.Collections.unmodifiableList(errorHandlers_);
} else {
return errorHandlersBuilder_.getMessageList();
}
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public int getErrorHandlersCount() {
if (errorHandlersBuilder_ == null) {
return errorHandlers_.size();
} else {
return errorHandlersBuilder_.getCount();
}
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public com.google.appengine.v1.ErrorHandler getErrorHandlers(int index) {
if (errorHandlersBuilder_ == null) {
return errorHandlers_.get(index);
} else {
return errorHandlersBuilder_.getMessage(index);
}
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public Builder setErrorHandlers(int index, com.google.appengine.v1.ErrorHandler value) {
if (errorHandlersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureErrorHandlersIsMutable();
errorHandlers_.set(index, value);
onChanged();
} else {
errorHandlersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public Builder setErrorHandlers(
int index, com.google.appengine.v1.ErrorHandler.Builder builderForValue) {
if (errorHandlersBuilder_ == null) {
ensureErrorHandlersIsMutable();
errorHandlers_.set(index, builderForValue.build());
onChanged();
} else {
errorHandlersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public Builder addErrorHandlers(com.google.appengine.v1.ErrorHandler value) {
if (errorHandlersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureErrorHandlersIsMutable();
errorHandlers_.add(value);
onChanged();
} else {
errorHandlersBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public Builder addErrorHandlers(int index, com.google.appengine.v1.ErrorHandler value) {
if (errorHandlersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureErrorHandlersIsMutable();
errorHandlers_.add(index, value);
onChanged();
} else {
errorHandlersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public Builder addErrorHandlers(com.google.appengine.v1.ErrorHandler.Builder builderForValue) {
if (errorHandlersBuilder_ == null) {
ensureErrorHandlersIsMutable();
errorHandlers_.add(builderForValue.build());
onChanged();
} else {
errorHandlersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public Builder addErrorHandlers(
int index, com.google.appengine.v1.ErrorHandler.Builder builderForValue) {
if (errorHandlersBuilder_ == null) {
ensureErrorHandlersIsMutable();
errorHandlers_.add(index, builderForValue.build());
onChanged();
} else {
errorHandlersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public Builder addAllErrorHandlers(
java.lang.Iterable extends com.google.appengine.v1.ErrorHandler> values) {
if (errorHandlersBuilder_ == null) {
ensureErrorHandlersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, errorHandlers_);
onChanged();
} else {
errorHandlersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public Builder clearErrorHandlers() {
if (errorHandlersBuilder_ == null) {
errorHandlers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x02000000);
onChanged();
} else {
errorHandlersBuilder_.clear();
}
return this;
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public Builder removeErrorHandlers(int index) {
if (errorHandlersBuilder_ == null) {
ensureErrorHandlersIsMutable();
errorHandlers_.remove(index);
onChanged();
} else {
errorHandlersBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public com.google.appengine.v1.ErrorHandler.Builder getErrorHandlersBuilder(int index) {
return getErrorHandlersFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public com.google.appengine.v1.ErrorHandlerOrBuilder getErrorHandlersOrBuilder(int index) {
if (errorHandlersBuilder_ == null) {
return errorHandlers_.get(index);
} else {
return errorHandlersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public java.util.List extends com.google.appengine.v1.ErrorHandlerOrBuilder>
getErrorHandlersOrBuilderList() {
if (errorHandlersBuilder_ != null) {
return errorHandlersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(errorHandlers_);
}
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public com.google.appengine.v1.ErrorHandler.Builder addErrorHandlersBuilder() {
return getErrorHandlersFieldBuilder()
.addBuilder(com.google.appengine.v1.ErrorHandler.getDefaultInstance());
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public com.google.appengine.v1.ErrorHandler.Builder addErrorHandlersBuilder(int index) {
return getErrorHandlersFieldBuilder()
.addBuilder(index, com.google.appengine.v1.ErrorHandler.getDefaultInstance());
}
/**
*
*
*
* Custom static error pages. Limited to 10KB per page.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.ErrorHandler error_handlers = 101;
*/
public java.util.List
getErrorHandlersBuilderList() {
return getErrorHandlersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.v1.ErrorHandler,
com.google.appengine.v1.ErrorHandler.Builder,
com.google.appengine.v1.ErrorHandlerOrBuilder>
getErrorHandlersFieldBuilder() {
if (errorHandlersBuilder_ == null) {
errorHandlersBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.v1.ErrorHandler,
com.google.appengine.v1.ErrorHandler.Builder,
com.google.appengine.v1.ErrorHandlerOrBuilder>(
errorHandlers_,
((bitField0_ & 0x02000000) != 0),
getParentForChildren(),
isClean());
errorHandlers_ = null;
}
return errorHandlersBuilder_;
}
private java.util.List libraries_ =
java.util.Collections.emptyList();
private void ensureLibrariesIsMutable() {
if (!((bitField0_ & 0x04000000) != 0)) {
libraries_ = new java.util.ArrayList(libraries_);
bitField0_ |= 0x04000000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.v1.Library,
com.google.appengine.v1.Library.Builder,
com.google.appengine.v1.LibraryOrBuilder>
librariesBuilder_;
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public java.util.List getLibrariesList() {
if (librariesBuilder_ == null) {
return java.util.Collections.unmodifiableList(libraries_);
} else {
return librariesBuilder_.getMessageList();
}
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public int getLibrariesCount() {
if (librariesBuilder_ == null) {
return libraries_.size();
} else {
return librariesBuilder_.getCount();
}
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public com.google.appengine.v1.Library getLibraries(int index) {
if (librariesBuilder_ == null) {
return libraries_.get(index);
} else {
return librariesBuilder_.getMessage(index);
}
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public Builder setLibraries(int index, com.google.appengine.v1.Library value) {
if (librariesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLibrariesIsMutable();
libraries_.set(index, value);
onChanged();
} else {
librariesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public Builder setLibraries(
int index, com.google.appengine.v1.Library.Builder builderForValue) {
if (librariesBuilder_ == null) {
ensureLibrariesIsMutable();
libraries_.set(index, builderForValue.build());
onChanged();
} else {
librariesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public Builder addLibraries(com.google.appengine.v1.Library value) {
if (librariesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLibrariesIsMutable();
libraries_.add(value);
onChanged();
} else {
librariesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public Builder addLibraries(int index, com.google.appengine.v1.Library value) {
if (librariesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLibrariesIsMutable();
libraries_.add(index, value);
onChanged();
} else {
librariesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public Builder addLibraries(com.google.appengine.v1.Library.Builder builderForValue) {
if (librariesBuilder_ == null) {
ensureLibrariesIsMutable();
libraries_.add(builderForValue.build());
onChanged();
} else {
librariesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public Builder addLibraries(
int index, com.google.appengine.v1.Library.Builder builderForValue) {
if (librariesBuilder_ == null) {
ensureLibrariesIsMutable();
libraries_.add(index, builderForValue.build());
onChanged();
} else {
librariesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public Builder addAllLibraries(
java.lang.Iterable extends com.google.appengine.v1.Library> values) {
if (librariesBuilder_ == null) {
ensureLibrariesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, libraries_);
onChanged();
} else {
librariesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public Builder clearLibraries() {
if (librariesBuilder_ == null) {
libraries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x04000000);
onChanged();
} else {
librariesBuilder_.clear();
}
return this;
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public Builder removeLibraries(int index) {
if (librariesBuilder_ == null) {
ensureLibrariesIsMutable();
libraries_.remove(index);
onChanged();
} else {
librariesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public com.google.appengine.v1.Library.Builder getLibrariesBuilder(int index) {
return getLibrariesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public com.google.appengine.v1.LibraryOrBuilder getLibrariesOrBuilder(int index) {
if (librariesBuilder_ == null) {
return libraries_.get(index);
} else {
return librariesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public java.util.List extends com.google.appengine.v1.LibraryOrBuilder>
getLibrariesOrBuilderList() {
if (librariesBuilder_ != null) {
return librariesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(libraries_);
}
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public com.google.appengine.v1.Library.Builder addLibrariesBuilder() {
return getLibrariesFieldBuilder()
.addBuilder(com.google.appengine.v1.Library.getDefaultInstance());
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public com.google.appengine.v1.Library.Builder addLibrariesBuilder(int index) {
return getLibrariesFieldBuilder()
.addBuilder(index, com.google.appengine.v1.Library.getDefaultInstance());
}
/**
*
*
*
* Configuration for third-party Python runtime libraries that are required
* by the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* repeated .google.appengine.v1.Library libraries = 102;
*/
public java.util.List getLibrariesBuilderList() {
return getLibrariesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.v1.Library,
com.google.appengine.v1.Library.Builder,
com.google.appengine.v1.LibraryOrBuilder>
getLibrariesFieldBuilder() {
if (librariesBuilder_ == null) {
librariesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.v1.Library,
com.google.appengine.v1.Library.Builder,
com.google.appengine.v1.LibraryOrBuilder>(
libraries_, ((bitField0_ & 0x04000000) != 0), getParentForChildren(), isClean());
libraries_ = null;
}
return librariesBuilder_;
}
private com.google.appengine.v1.ApiConfigHandler apiConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.ApiConfigHandler,
com.google.appengine.v1.ApiConfigHandler.Builder,
com.google.appengine.v1.ApiConfigHandlerOrBuilder>
apiConfigBuilder_;
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*
* @return Whether the apiConfig field is set.
*/
public boolean hasApiConfig() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*
* @return The apiConfig.
*/
public com.google.appengine.v1.ApiConfigHandler getApiConfig() {
if (apiConfigBuilder_ == null) {
return apiConfig_ == null
? com.google.appengine.v1.ApiConfigHandler.getDefaultInstance()
: apiConfig_;
} else {
return apiConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*/
public Builder setApiConfig(com.google.appengine.v1.ApiConfigHandler value) {
if (apiConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
apiConfig_ = value;
} else {
apiConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*/
public Builder setApiConfig(com.google.appengine.v1.ApiConfigHandler.Builder builderForValue) {
if (apiConfigBuilder_ == null) {
apiConfig_ = builderForValue.build();
} else {
apiConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*/
public Builder mergeApiConfig(com.google.appengine.v1.ApiConfigHandler value) {
if (apiConfigBuilder_ == null) {
if (((bitField0_ & 0x08000000) != 0)
&& apiConfig_ != null
&& apiConfig_ != com.google.appengine.v1.ApiConfigHandler.getDefaultInstance()) {
getApiConfigBuilder().mergeFrom(value);
} else {
apiConfig_ = value;
}
} else {
apiConfigBuilder_.mergeFrom(value);
}
if (apiConfig_ != null) {
bitField0_ |= 0x08000000;
onChanged();
}
return this;
}
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*/
public Builder clearApiConfig() {
bitField0_ = (bitField0_ & ~0x08000000);
apiConfig_ = null;
if (apiConfigBuilder_ != null) {
apiConfigBuilder_.dispose();
apiConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*/
public com.google.appengine.v1.ApiConfigHandler.Builder getApiConfigBuilder() {
bitField0_ |= 0x08000000;
onChanged();
return getApiConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*/
public com.google.appengine.v1.ApiConfigHandlerOrBuilder getApiConfigOrBuilder() {
if (apiConfigBuilder_ != null) {
return apiConfigBuilder_.getMessageOrBuilder();
} else {
return apiConfig_ == null
? com.google.appengine.v1.ApiConfigHandler.getDefaultInstance()
: apiConfig_;
}
}
/**
*
*
*
* Serving configuration for
* [Google Cloud Endpoints](https://cloud.google.com/appengine/docs/python/endpoints/).
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ApiConfigHandler api_config = 103;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.ApiConfigHandler,
com.google.appengine.v1.ApiConfigHandler.Builder,
com.google.appengine.v1.ApiConfigHandlerOrBuilder>
getApiConfigFieldBuilder() {
if (apiConfigBuilder_ == null) {
apiConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.ApiConfigHandler,
com.google.appengine.v1.ApiConfigHandler.Builder,
com.google.appengine.v1.ApiConfigHandlerOrBuilder>(
getApiConfig(), getParentForChildren(), isClean());
apiConfig_ = null;
}
return apiConfigBuilder_;
}
private com.google.protobuf.MapField envVariables_;
private com.google.protobuf.MapField
internalGetEnvVariables() {
if (envVariables_ == null) {
return com.google.protobuf.MapField.emptyMapField(
EnvVariablesDefaultEntryHolder.defaultEntry);
}
return envVariables_;
}
private com.google.protobuf.MapField
internalGetMutableEnvVariables() {
if (envVariables_ == null) {
envVariables_ =
com.google.protobuf.MapField.newMapField(EnvVariablesDefaultEntryHolder.defaultEntry);
}
if (!envVariables_.isMutable()) {
envVariables_ = envVariables_.copy();
}
bitField0_ |= 0x10000000;
onChanged();
return envVariables_;
}
public int getEnvVariablesCount() {
return internalGetEnvVariables().getMap().size();
}
/**
*
*
*
* Environment variables available to the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> env_variables = 104;
*/
@java.lang.Override
public boolean containsEnvVariables(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetEnvVariables().getMap().containsKey(key);
}
/** Use {@link #getEnvVariablesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getEnvVariables() {
return getEnvVariablesMap();
}
/**
*
*
*
* Environment variables available to the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> env_variables = 104;
*/
@java.lang.Override
public java.util.Map getEnvVariablesMap() {
return internalGetEnvVariables().getMap();
}
/**
*
*
*
* Environment variables available to the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> env_variables = 104;
*/
@java.lang.Override
public /* nullable */ java.lang.String getEnvVariablesOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetEnvVariables().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Environment variables available to the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> env_variables = 104;
*/
@java.lang.Override
public java.lang.String getEnvVariablesOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetEnvVariables().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearEnvVariables() {
bitField0_ = (bitField0_ & ~0x10000000);
internalGetMutableEnvVariables().getMutableMap().clear();
return this;
}
/**
*
*
*
* Environment variables available to the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> env_variables = 104;
*/
public Builder removeEnvVariables(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableEnvVariables().getMutableMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map getMutableEnvVariables() {
bitField0_ |= 0x10000000;
return internalGetMutableEnvVariables().getMutableMap();
}
/**
*
*
*
* Environment variables available to the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> env_variables = 104;
*/
public Builder putEnvVariables(java.lang.String key, java.lang.String value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableEnvVariables().getMutableMap().put(key, value);
bitField0_ |= 0x10000000;
return this;
}
/**
*
*
*
* Environment variables available to the application.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> env_variables = 104;
*/
public Builder putAllEnvVariables(java.util.Map values) {
internalGetMutableEnvVariables().getMutableMap().putAll(values);
bitField0_ |= 0x10000000;
return this;
}
private com.google.protobuf.MapField buildEnvVariables_;
private com.google.protobuf.MapField
internalGetBuildEnvVariables() {
if (buildEnvVariables_ == null) {
return com.google.protobuf.MapField.emptyMapField(
BuildEnvVariablesDefaultEntryHolder.defaultEntry);
}
return buildEnvVariables_;
}
private com.google.protobuf.MapField
internalGetMutableBuildEnvVariables() {
if (buildEnvVariables_ == null) {
buildEnvVariables_ =
com.google.protobuf.MapField.newMapField(
BuildEnvVariablesDefaultEntryHolder.defaultEntry);
}
if (!buildEnvVariables_.isMutable()) {
buildEnvVariables_ = buildEnvVariables_.copy();
}
bitField0_ |= 0x20000000;
onChanged();
return buildEnvVariables_;
}
public int getBuildEnvVariablesCount() {
return internalGetBuildEnvVariables().getMap().size();
}
/**
*
*
*
* Environment variables available to the build environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> build_env_variables = 125;
*/
@java.lang.Override
public boolean containsBuildEnvVariables(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetBuildEnvVariables().getMap().containsKey(key);
}
/** Use {@link #getBuildEnvVariablesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getBuildEnvVariables() {
return getBuildEnvVariablesMap();
}
/**
*
*
*
* Environment variables available to the build environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> build_env_variables = 125;
*/
@java.lang.Override
public java.util.Map getBuildEnvVariablesMap() {
return internalGetBuildEnvVariables().getMap();
}
/**
*
*
*
* Environment variables available to the build environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> build_env_variables = 125;
*/
@java.lang.Override
public /* nullable */ java.lang.String getBuildEnvVariablesOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetBuildEnvVariables().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Environment variables available to the build environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> build_env_variables = 125;
*/
@java.lang.Override
public java.lang.String getBuildEnvVariablesOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetBuildEnvVariables().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearBuildEnvVariables() {
bitField0_ = (bitField0_ & ~0x20000000);
internalGetMutableBuildEnvVariables().getMutableMap().clear();
return this;
}
/**
*
*
*
* Environment variables available to the build environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> build_env_variables = 125;
*/
public Builder removeBuildEnvVariables(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableBuildEnvVariables().getMutableMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map getMutableBuildEnvVariables() {
bitField0_ |= 0x20000000;
return internalGetMutableBuildEnvVariables().getMutableMap();
}
/**
*
*
*
* Environment variables available to the build environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> build_env_variables = 125;
*/
public Builder putBuildEnvVariables(java.lang.String key, java.lang.String value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableBuildEnvVariables().getMutableMap().put(key, value);
bitField0_ |= 0x20000000;
return this;
}
/**
*
*
*
* Environment variables available to the build environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* map<string, string> build_env_variables = 125;
*/
public Builder putAllBuildEnvVariables(
java.util.Map values) {
internalGetMutableBuildEnvVariables().getMutableMap().putAll(values);
bitField0_ |= 0x20000000;
return this;
}
private com.google.protobuf.Duration defaultExpiration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
defaultExpirationBuilder_;
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*
* @return Whether the defaultExpiration field is set.
*/
public boolean hasDefaultExpiration() {
return ((bitField0_ & 0x40000000) != 0);
}
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*
* @return The defaultExpiration.
*/
public com.google.protobuf.Duration getDefaultExpiration() {
if (defaultExpirationBuilder_ == null) {
return defaultExpiration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: defaultExpiration_;
} else {
return defaultExpirationBuilder_.getMessage();
}
}
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*/
public Builder setDefaultExpiration(com.google.protobuf.Duration value) {
if (defaultExpirationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
defaultExpiration_ = value;
} else {
defaultExpirationBuilder_.setMessage(value);
}
bitField0_ |= 0x40000000;
onChanged();
return this;
}
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*/
public Builder setDefaultExpiration(com.google.protobuf.Duration.Builder builderForValue) {
if (defaultExpirationBuilder_ == null) {
defaultExpiration_ = builderForValue.build();
} else {
defaultExpirationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x40000000;
onChanged();
return this;
}
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*/
public Builder mergeDefaultExpiration(com.google.protobuf.Duration value) {
if (defaultExpirationBuilder_ == null) {
if (((bitField0_ & 0x40000000) != 0)
&& defaultExpiration_ != null
&& defaultExpiration_ != com.google.protobuf.Duration.getDefaultInstance()) {
getDefaultExpirationBuilder().mergeFrom(value);
} else {
defaultExpiration_ = value;
}
} else {
defaultExpirationBuilder_.mergeFrom(value);
}
if (defaultExpiration_ != null) {
bitField0_ |= 0x40000000;
onChanged();
}
return this;
}
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*/
public Builder clearDefaultExpiration() {
bitField0_ = (bitField0_ & ~0x40000000);
defaultExpiration_ = null;
if (defaultExpirationBuilder_ != null) {
defaultExpirationBuilder_.dispose();
defaultExpirationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*/
public com.google.protobuf.Duration.Builder getDefaultExpirationBuilder() {
bitField0_ |= 0x40000000;
onChanged();
return getDefaultExpirationFieldBuilder().getBuilder();
}
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*/
public com.google.protobuf.DurationOrBuilder getDefaultExpirationOrBuilder() {
if (defaultExpirationBuilder_ != null) {
return defaultExpirationBuilder_.getMessageOrBuilder();
} else {
return defaultExpiration_ == null
? com.google.protobuf.Duration.getDefaultInstance()
: defaultExpiration_;
}
}
/**
*
*
*
* Duration that static files should be cached by web proxies and browsers.
* Only applicable if the corresponding
* [StaticFilesHandler](https://cloud.google.com/appengine/docs/admin-api/reference/rest/v1/apps.services.versions#StaticFilesHandler)
* does not specify its own expiration time.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.protobuf.Duration default_expiration = 105;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getDefaultExpirationFieldBuilder() {
if (defaultExpirationBuilder_ == null) {
defaultExpirationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
getDefaultExpiration(), getParentForChildren(), isClean());
defaultExpiration_ = null;
}
return defaultExpirationBuilder_;
}
private com.google.appengine.v1.HealthCheck healthCheck_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.HealthCheck,
com.google.appengine.v1.HealthCheck.Builder,
com.google.appengine.v1.HealthCheckOrBuilder>
healthCheckBuilder_;
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*
* @return Whether the healthCheck field is set.
*/
public boolean hasHealthCheck() {
return ((bitField0_ & 0x80000000) != 0);
}
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*
* @return The healthCheck.
*/
public com.google.appengine.v1.HealthCheck getHealthCheck() {
if (healthCheckBuilder_ == null) {
return healthCheck_ == null
? com.google.appengine.v1.HealthCheck.getDefaultInstance()
: healthCheck_;
} else {
return healthCheckBuilder_.getMessage();
}
}
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*/
public Builder setHealthCheck(com.google.appengine.v1.HealthCheck value) {
if (healthCheckBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
healthCheck_ = value;
} else {
healthCheckBuilder_.setMessage(value);
}
bitField0_ |= 0x80000000;
onChanged();
return this;
}
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*/
public Builder setHealthCheck(com.google.appengine.v1.HealthCheck.Builder builderForValue) {
if (healthCheckBuilder_ == null) {
healthCheck_ = builderForValue.build();
} else {
healthCheckBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x80000000;
onChanged();
return this;
}
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*/
public Builder mergeHealthCheck(com.google.appengine.v1.HealthCheck value) {
if (healthCheckBuilder_ == null) {
if (((bitField0_ & 0x80000000) != 0)
&& healthCheck_ != null
&& healthCheck_ != com.google.appengine.v1.HealthCheck.getDefaultInstance()) {
getHealthCheckBuilder().mergeFrom(value);
} else {
healthCheck_ = value;
}
} else {
healthCheckBuilder_.mergeFrom(value);
}
if (healthCheck_ != null) {
bitField0_ |= 0x80000000;
onChanged();
}
return this;
}
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*/
public Builder clearHealthCheck() {
bitField0_ = (bitField0_ & ~0x80000000);
healthCheck_ = null;
if (healthCheckBuilder_ != null) {
healthCheckBuilder_.dispose();
healthCheckBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*/
public com.google.appengine.v1.HealthCheck.Builder getHealthCheckBuilder() {
bitField0_ |= 0x80000000;
onChanged();
return getHealthCheckFieldBuilder().getBuilder();
}
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*/
public com.google.appengine.v1.HealthCheckOrBuilder getHealthCheckOrBuilder() {
if (healthCheckBuilder_ != null) {
return healthCheckBuilder_.getMessageOrBuilder();
} else {
return healthCheck_ == null
? com.google.appengine.v1.HealthCheck.getDefaultInstance()
: healthCheck_;
}
}
/**
*
*
*
* Configures health checking for instances. Unhealthy instances are
* stopped and replaced with new instances.
* Only applicable in the App Engine flexible environment.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.HealthCheck health_check = 106;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.HealthCheck,
com.google.appengine.v1.HealthCheck.Builder,
com.google.appengine.v1.HealthCheckOrBuilder>
getHealthCheckFieldBuilder() {
if (healthCheckBuilder_ == null) {
healthCheckBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.HealthCheck,
com.google.appengine.v1.HealthCheck.Builder,
com.google.appengine.v1.HealthCheckOrBuilder>(
getHealthCheck(), getParentForChildren(), isClean());
healthCheck_ = null;
}
return healthCheckBuilder_;
}
private com.google.appengine.v1.ReadinessCheck readinessCheck_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.ReadinessCheck,
com.google.appengine.v1.ReadinessCheck.Builder,
com.google.appengine.v1.ReadinessCheckOrBuilder>
readinessCheckBuilder_;
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*
* @return Whether the readinessCheck field is set.
*/
public boolean hasReadinessCheck() {
return ((bitField1_ & 0x00000001) != 0);
}
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*
* @return The readinessCheck.
*/
public com.google.appengine.v1.ReadinessCheck getReadinessCheck() {
if (readinessCheckBuilder_ == null) {
return readinessCheck_ == null
? com.google.appengine.v1.ReadinessCheck.getDefaultInstance()
: readinessCheck_;
} else {
return readinessCheckBuilder_.getMessage();
}
}
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*/
public Builder setReadinessCheck(com.google.appengine.v1.ReadinessCheck value) {
if (readinessCheckBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
readinessCheck_ = value;
} else {
readinessCheckBuilder_.setMessage(value);
}
bitField1_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*/
public Builder setReadinessCheck(
com.google.appengine.v1.ReadinessCheck.Builder builderForValue) {
if (readinessCheckBuilder_ == null) {
readinessCheck_ = builderForValue.build();
} else {
readinessCheckBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*/
public Builder mergeReadinessCheck(com.google.appengine.v1.ReadinessCheck value) {
if (readinessCheckBuilder_ == null) {
if (((bitField1_ & 0x00000001) != 0)
&& readinessCheck_ != null
&& readinessCheck_ != com.google.appengine.v1.ReadinessCheck.getDefaultInstance()) {
getReadinessCheckBuilder().mergeFrom(value);
} else {
readinessCheck_ = value;
}
} else {
readinessCheckBuilder_.mergeFrom(value);
}
if (readinessCheck_ != null) {
bitField1_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*/
public Builder clearReadinessCheck() {
bitField1_ = (bitField1_ & ~0x00000001);
readinessCheck_ = null;
if (readinessCheckBuilder_ != null) {
readinessCheckBuilder_.dispose();
readinessCheckBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*/
public com.google.appengine.v1.ReadinessCheck.Builder getReadinessCheckBuilder() {
bitField1_ |= 0x00000001;
onChanged();
return getReadinessCheckFieldBuilder().getBuilder();
}
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*/
public com.google.appengine.v1.ReadinessCheckOrBuilder getReadinessCheckOrBuilder() {
if (readinessCheckBuilder_ != null) {
return readinessCheckBuilder_.getMessageOrBuilder();
} else {
return readinessCheck_ == null
? com.google.appengine.v1.ReadinessCheck.getDefaultInstance()
: readinessCheck_;
}
}
/**
*
*
*
* Configures readiness health checking for instances.
* Unhealthy instances are not put into the backend traffic rotation.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.ReadinessCheck readiness_check = 112;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.ReadinessCheck,
com.google.appengine.v1.ReadinessCheck.Builder,
com.google.appengine.v1.ReadinessCheckOrBuilder>
getReadinessCheckFieldBuilder() {
if (readinessCheckBuilder_ == null) {
readinessCheckBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.ReadinessCheck,
com.google.appengine.v1.ReadinessCheck.Builder,
com.google.appengine.v1.ReadinessCheckOrBuilder>(
getReadinessCheck(), getParentForChildren(), isClean());
readinessCheck_ = null;
}
return readinessCheckBuilder_;
}
private com.google.appengine.v1.LivenessCheck livenessCheck_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.LivenessCheck,
com.google.appengine.v1.LivenessCheck.Builder,
com.google.appengine.v1.LivenessCheckOrBuilder>
livenessCheckBuilder_;
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*
* @return Whether the livenessCheck field is set.
*/
public boolean hasLivenessCheck() {
return ((bitField1_ & 0x00000002) != 0);
}
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*
* @return The livenessCheck.
*/
public com.google.appengine.v1.LivenessCheck getLivenessCheck() {
if (livenessCheckBuilder_ == null) {
return livenessCheck_ == null
? com.google.appengine.v1.LivenessCheck.getDefaultInstance()
: livenessCheck_;
} else {
return livenessCheckBuilder_.getMessage();
}
}
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*/
public Builder setLivenessCheck(com.google.appengine.v1.LivenessCheck value) {
if (livenessCheckBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
livenessCheck_ = value;
} else {
livenessCheckBuilder_.setMessage(value);
}
bitField1_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*/
public Builder setLivenessCheck(com.google.appengine.v1.LivenessCheck.Builder builderForValue) {
if (livenessCheckBuilder_ == null) {
livenessCheck_ = builderForValue.build();
} else {
livenessCheckBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*/
public Builder mergeLivenessCheck(com.google.appengine.v1.LivenessCheck value) {
if (livenessCheckBuilder_ == null) {
if (((bitField1_ & 0x00000002) != 0)
&& livenessCheck_ != null
&& livenessCheck_ != com.google.appengine.v1.LivenessCheck.getDefaultInstance()) {
getLivenessCheckBuilder().mergeFrom(value);
} else {
livenessCheck_ = value;
}
} else {
livenessCheckBuilder_.mergeFrom(value);
}
if (livenessCheck_ != null) {
bitField1_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*/
public Builder clearLivenessCheck() {
bitField1_ = (bitField1_ & ~0x00000002);
livenessCheck_ = null;
if (livenessCheckBuilder_ != null) {
livenessCheckBuilder_.dispose();
livenessCheckBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*/
public com.google.appengine.v1.LivenessCheck.Builder getLivenessCheckBuilder() {
bitField1_ |= 0x00000002;
onChanged();
return getLivenessCheckFieldBuilder().getBuilder();
}
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*/
public com.google.appengine.v1.LivenessCheckOrBuilder getLivenessCheckOrBuilder() {
if (livenessCheckBuilder_ != null) {
return livenessCheckBuilder_.getMessageOrBuilder();
} else {
return livenessCheck_ == null
? com.google.appengine.v1.LivenessCheck.getDefaultInstance()
: livenessCheck_;
}
}
/**
*
*
*
* Configures liveness health checking for instances.
* Unhealthy instances are stopped and replaced with new instances
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.LivenessCheck liveness_check = 113;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.LivenessCheck,
com.google.appengine.v1.LivenessCheck.Builder,
com.google.appengine.v1.LivenessCheckOrBuilder>
getLivenessCheckFieldBuilder() {
if (livenessCheckBuilder_ == null) {
livenessCheckBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.LivenessCheck,
com.google.appengine.v1.LivenessCheck.Builder,
com.google.appengine.v1.LivenessCheckOrBuilder>(
getLivenessCheck(), getParentForChildren(), isClean());
livenessCheck_ = null;
}
return livenessCheckBuilder_;
}
private java.lang.Object nobuildFilesRegex_ = "";
/**
*
*
*
* Files that match this pattern will not be built into this version.
* Only applicable for Go runtimes.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* string nobuild_files_regex = 107;
*
* @return The nobuildFilesRegex.
*/
public java.lang.String getNobuildFilesRegex() {
java.lang.Object ref = nobuildFilesRegex_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nobuildFilesRegex_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Files that match this pattern will not be built into this version.
* Only applicable for Go runtimes.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* string nobuild_files_regex = 107;
*
* @return The bytes for nobuildFilesRegex.
*/
public com.google.protobuf.ByteString getNobuildFilesRegexBytes() {
java.lang.Object ref = nobuildFilesRegex_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
nobuildFilesRegex_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Files that match this pattern will not be built into this version.
* Only applicable for Go runtimes.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* string nobuild_files_regex = 107;
*
* @param value The nobuildFilesRegex to set.
* @return This builder for chaining.
*/
public Builder setNobuildFilesRegex(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
nobuildFilesRegex_ = value;
bitField1_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Files that match this pattern will not be built into this version.
* Only applicable for Go runtimes.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* string nobuild_files_regex = 107;
*
* @return This builder for chaining.
*/
public Builder clearNobuildFilesRegex() {
nobuildFilesRegex_ = getDefaultInstance().getNobuildFilesRegex();
bitField1_ = (bitField1_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* Files that match this pattern will not be built into this version.
* Only applicable for Go runtimes.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* string nobuild_files_regex = 107;
*
* @param value The bytes for nobuildFilesRegex to set.
* @return This builder for chaining.
*/
public Builder setNobuildFilesRegexBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
nobuildFilesRegex_ = value;
bitField1_ |= 0x00000004;
onChanged();
return this;
}
private com.google.appengine.v1.Deployment deployment_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Deployment,
com.google.appengine.v1.Deployment.Builder,
com.google.appengine.v1.DeploymentOrBuilder>
deploymentBuilder_;
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*
* @return Whether the deployment field is set.
*/
public boolean hasDeployment() {
return ((bitField1_ & 0x00000008) != 0);
}
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*
* @return The deployment.
*/
public com.google.appengine.v1.Deployment getDeployment() {
if (deploymentBuilder_ == null) {
return deployment_ == null
? com.google.appengine.v1.Deployment.getDefaultInstance()
: deployment_;
} else {
return deploymentBuilder_.getMessage();
}
}
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*/
public Builder setDeployment(com.google.appengine.v1.Deployment value) {
if (deploymentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
deployment_ = value;
} else {
deploymentBuilder_.setMessage(value);
}
bitField1_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*/
public Builder setDeployment(com.google.appengine.v1.Deployment.Builder builderForValue) {
if (deploymentBuilder_ == null) {
deployment_ = builderForValue.build();
} else {
deploymentBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*/
public Builder mergeDeployment(com.google.appengine.v1.Deployment value) {
if (deploymentBuilder_ == null) {
if (((bitField1_ & 0x00000008) != 0)
&& deployment_ != null
&& deployment_ != com.google.appengine.v1.Deployment.getDefaultInstance()) {
getDeploymentBuilder().mergeFrom(value);
} else {
deployment_ = value;
}
} else {
deploymentBuilder_.mergeFrom(value);
}
if (deployment_ != null) {
bitField1_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*/
public Builder clearDeployment() {
bitField1_ = (bitField1_ & ~0x00000008);
deployment_ = null;
if (deploymentBuilder_ != null) {
deploymentBuilder_.dispose();
deploymentBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*/
public com.google.appengine.v1.Deployment.Builder getDeploymentBuilder() {
bitField1_ |= 0x00000008;
onChanged();
return getDeploymentFieldBuilder().getBuilder();
}
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*/
public com.google.appengine.v1.DeploymentOrBuilder getDeploymentOrBuilder() {
if (deploymentBuilder_ != null) {
return deploymentBuilder_.getMessageOrBuilder();
} else {
return deployment_ == null
? com.google.appengine.v1.Deployment.getDefaultInstance()
: deployment_;
}
}
/**
*
*
*
* Code and application artifacts that make up this version.
*
* Only returned in `GET` requests if `view=FULL` is set.
*
*
* .google.appengine.v1.Deployment deployment = 108;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Deployment,
com.google.appengine.v1.Deployment.Builder,
com.google.appengine.v1.DeploymentOrBuilder>
getDeploymentFieldBuilder() {
if (deploymentBuilder_ == null) {
deploymentBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Deployment,
com.google.appengine.v1.Deployment.Builder,
com.google.appengine.v1.DeploymentOrBuilder>(
getDeployment(), getParentForChildren(), isClean());
deployment_ = null;
}
return deploymentBuilder_;
}
private java.lang.Object versionUrl_ = "";
/**
*
*
*
* Serving URL for this version. Example:
* "https://myversion-dot-myservice-dot-myapp.appspot.com"
*
* @OutputOnly
*
*
* string version_url = 109;
*
* @return The versionUrl.
*/
public java.lang.String getVersionUrl() {
java.lang.Object ref = versionUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
versionUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Serving URL for this version. Example:
* "https://myversion-dot-myservice-dot-myapp.appspot.com"
*
* @OutputOnly
*
*
* string version_url = 109;
*
* @return The bytes for versionUrl.
*/
public com.google.protobuf.ByteString getVersionUrlBytes() {
java.lang.Object ref = versionUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
versionUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Serving URL for this version. Example:
* "https://myversion-dot-myservice-dot-myapp.appspot.com"
*
* @OutputOnly
*
*
* string version_url = 109;
*
* @param value The versionUrl to set.
* @return This builder for chaining.
*/
public Builder setVersionUrl(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
versionUrl_ = value;
bitField1_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Serving URL for this version. Example:
* "https://myversion-dot-myservice-dot-myapp.appspot.com"
*
* @OutputOnly
*
*
* string version_url = 109;
*
* @return This builder for chaining.
*/
public Builder clearVersionUrl() {
versionUrl_ = getDefaultInstance().getVersionUrl();
bitField1_ = (bitField1_ & ~0x00000010);
onChanged();
return this;
}
/**
*
*
*
* Serving URL for this version. Example:
* "https://myversion-dot-myservice-dot-myapp.appspot.com"
*
* @OutputOnly
*
*
* string version_url = 109;
*
* @param value The bytes for versionUrl to set.
* @return This builder for chaining.
*/
public Builder setVersionUrlBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
versionUrl_ = value;
bitField1_ |= 0x00000010;
onChanged();
return this;
}
private com.google.appengine.v1.EndpointsApiService endpointsApiService_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.EndpointsApiService,
com.google.appengine.v1.EndpointsApiService.Builder,
com.google.appengine.v1.EndpointsApiServiceOrBuilder>
endpointsApiServiceBuilder_;
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*
* @return Whether the endpointsApiService field is set.
*/
public boolean hasEndpointsApiService() {
return ((bitField1_ & 0x00000020) != 0);
}
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*
* @return The endpointsApiService.
*/
public com.google.appengine.v1.EndpointsApiService getEndpointsApiService() {
if (endpointsApiServiceBuilder_ == null) {
return endpointsApiService_ == null
? com.google.appengine.v1.EndpointsApiService.getDefaultInstance()
: endpointsApiService_;
} else {
return endpointsApiServiceBuilder_.getMessage();
}
}
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*/
public Builder setEndpointsApiService(com.google.appengine.v1.EndpointsApiService value) {
if (endpointsApiServiceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
endpointsApiService_ = value;
} else {
endpointsApiServiceBuilder_.setMessage(value);
}
bitField1_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*/
public Builder setEndpointsApiService(
com.google.appengine.v1.EndpointsApiService.Builder builderForValue) {
if (endpointsApiServiceBuilder_ == null) {
endpointsApiService_ = builderForValue.build();
} else {
endpointsApiServiceBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*/
public Builder mergeEndpointsApiService(com.google.appengine.v1.EndpointsApiService value) {
if (endpointsApiServiceBuilder_ == null) {
if (((bitField1_ & 0x00000020) != 0)
&& endpointsApiService_ != null
&& endpointsApiService_
!= com.google.appengine.v1.EndpointsApiService.getDefaultInstance()) {
getEndpointsApiServiceBuilder().mergeFrom(value);
} else {
endpointsApiService_ = value;
}
} else {
endpointsApiServiceBuilder_.mergeFrom(value);
}
if (endpointsApiService_ != null) {
bitField1_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*/
public Builder clearEndpointsApiService() {
bitField1_ = (bitField1_ & ~0x00000020);
endpointsApiService_ = null;
if (endpointsApiServiceBuilder_ != null) {
endpointsApiServiceBuilder_.dispose();
endpointsApiServiceBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*/
public com.google.appengine.v1.EndpointsApiService.Builder getEndpointsApiServiceBuilder() {
bitField1_ |= 0x00000020;
onChanged();
return getEndpointsApiServiceFieldBuilder().getBuilder();
}
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*/
public com.google.appengine.v1.EndpointsApiServiceOrBuilder getEndpointsApiServiceOrBuilder() {
if (endpointsApiServiceBuilder_ != null) {
return endpointsApiServiceBuilder_.getMessageOrBuilder();
} else {
return endpointsApiService_ == null
? com.google.appengine.v1.EndpointsApiService.getDefaultInstance()
: endpointsApiService_;
}
}
/**
*
*
*
* Cloud Endpoints configuration.
*
* If endpoints_api_service is set, the Cloud Endpoints Extensible Service
* Proxy will be provided to serve the API implemented by the app.
*
*
* .google.appengine.v1.EndpointsApiService endpoints_api_service = 110;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.EndpointsApiService,
com.google.appengine.v1.EndpointsApiService.Builder,
com.google.appengine.v1.EndpointsApiServiceOrBuilder>
getEndpointsApiServiceFieldBuilder() {
if (endpointsApiServiceBuilder_ == null) {
endpointsApiServiceBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.EndpointsApiService,
com.google.appengine.v1.EndpointsApiService.Builder,
com.google.appengine.v1.EndpointsApiServiceOrBuilder>(
getEndpointsApiService(), getParentForChildren(), isClean());
endpointsApiService_ = null;
}
return endpointsApiServiceBuilder_;
}
private com.google.appengine.v1.Entrypoint entrypoint_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Entrypoint,
com.google.appengine.v1.Entrypoint.Builder,
com.google.appengine.v1.EntrypointOrBuilder>
entrypointBuilder_;
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*
* @return Whether the entrypoint field is set.
*/
public boolean hasEntrypoint() {
return ((bitField1_ & 0x00000040) != 0);
}
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*
* @return The entrypoint.
*/
public com.google.appengine.v1.Entrypoint getEntrypoint() {
if (entrypointBuilder_ == null) {
return entrypoint_ == null
? com.google.appengine.v1.Entrypoint.getDefaultInstance()
: entrypoint_;
} else {
return entrypointBuilder_.getMessage();
}
}
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*/
public Builder setEntrypoint(com.google.appengine.v1.Entrypoint value) {
if (entrypointBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
entrypoint_ = value;
} else {
entrypointBuilder_.setMessage(value);
}
bitField1_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*/
public Builder setEntrypoint(com.google.appengine.v1.Entrypoint.Builder builderForValue) {
if (entrypointBuilder_ == null) {
entrypoint_ = builderForValue.build();
} else {
entrypointBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*/
public Builder mergeEntrypoint(com.google.appengine.v1.Entrypoint value) {
if (entrypointBuilder_ == null) {
if (((bitField1_ & 0x00000040) != 0)
&& entrypoint_ != null
&& entrypoint_ != com.google.appengine.v1.Entrypoint.getDefaultInstance()) {
getEntrypointBuilder().mergeFrom(value);
} else {
entrypoint_ = value;
}
} else {
entrypointBuilder_.mergeFrom(value);
}
if (entrypoint_ != null) {
bitField1_ |= 0x00000040;
onChanged();
}
return this;
}
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*/
public Builder clearEntrypoint() {
bitField1_ = (bitField1_ & ~0x00000040);
entrypoint_ = null;
if (entrypointBuilder_ != null) {
entrypointBuilder_.dispose();
entrypointBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*/
public com.google.appengine.v1.Entrypoint.Builder getEntrypointBuilder() {
bitField1_ |= 0x00000040;
onChanged();
return getEntrypointFieldBuilder().getBuilder();
}
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*/
public com.google.appengine.v1.EntrypointOrBuilder getEntrypointOrBuilder() {
if (entrypointBuilder_ != null) {
return entrypointBuilder_.getMessageOrBuilder();
} else {
return entrypoint_ == null
? com.google.appengine.v1.Entrypoint.getDefaultInstance()
: entrypoint_;
}
}
/**
*
*
*
* The entrypoint for the application.
*
*
* .google.appengine.v1.Entrypoint entrypoint = 122;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Entrypoint,
com.google.appengine.v1.Entrypoint.Builder,
com.google.appengine.v1.EntrypointOrBuilder>
getEntrypointFieldBuilder() {
if (entrypointBuilder_ == null) {
entrypointBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.Entrypoint,
com.google.appengine.v1.Entrypoint.Builder,
com.google.appengine.v1.EntrypointOrBuilder>(
getEntrypoint(), getParentForChildren(), isClean());
entrypoint_ = null;
}
return entrypointBuilder_;
}
private com.google.appengine.v1.VpcAccessConnector vpcAccessConnector_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.VpcAccessConnector,
com.google.appengine.v1.VpcAccessConnector.Builder,
com.google.appengine.v1.VpcAccessConnectorOrBuilder>
vpcAccessConnectorBuilder_;
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*
* @return Whether the vpcAccessConnector field is set.
*/
public boolean hasVpcAccessConnector() {
return ((bitField1_ & 0x00000080) != 0);
}
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*
* @return The vpcAccessConnector.
*/
public com.google.appengine.v1.VpcAccessConnector getVpcAccessConnector() {
if (vpcAccessConnectorBuilder_ == null) {
return vpcAccessConnector_ == null
? com.google.appengine.v1.VpcAccessConnector.getDefaultInstance()
: vpcAccessConnector_;
} else {
return vpcAccessConnectorBuilder_.getMessage();
}
}
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*/
public Builder setVpcAccessConnector(com.google.appengine.v1.VpcAccessConnector value) {
if (vpcAccessConnectorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
vpcAccessConnector_ = value;
} else {
vpcAccessConnectorBuilder_.setMessage(value);
}
bitField1_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*/
public Builder setVpcAccessConnector(
com.google.appengine.v1.VpcAccessConnector.Builder builderForValue) {
if (vpcAccessConnectorBuilder_ == null) {
vpcAccessConnector_ = builderForValue.build();
} else {
vpcAccessConnectorBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*/
public Builder mergeVpcAccessConnector(com.google.appengine.v1.VpcAccessConnector value) {
if (vpcAccessConnectorBuilder_ == null) {
if (((bitField1_ & 0x00000080) != 0)
&& vpcAccessConnector_ != null
&& vpcAccessConnector_
!= com.google.appengine.v1.VpcAccessConnector.getDefaultInstance()) {
getVpcAccessConnectorBuilder().mergeFrom(value);
} else {
vpcAccessConnector_ = value;
}
} else {
vpcAccessConnectorBuilder_.mergeFrom(value);
}
if (vpcAccessConnector_ != null) {
bitField1_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*/
public Builder clearVpcAccessConnector() {
bitField1_ = (bitField1_ & ~0x00000080);
vpcAccessConnector_ = null;
if (vpcAccessConnectorBuilder_ != null) {
vpcAccessConnectorBuilder_.dispose();
vpcAccessConnectorBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*/
public com.google.appengine.v1.VpcAccessConnector.Builder getVpcAccessConnectorBuilder() {
bitField1_ |= 0x00000080;
onChanged();
return getVpcAccessConnectorFieldBuilder().getBuilder();
}
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*/
public com.google.appengine.v1.VpcAccessConnectorOrBuilder getVpcAccessConnectorOrBuilder() {
if (vpcAccessConnectorBuilder_ != null) {
return vpcAccessConnectorBuilder_.getMessageOrBuilder();
} else {
return vpcAccessConnector_ == null
? com.google.appengine.v1.VpcAccessConnector.getDefaultInstance()
: vpcAccessConnector_;
}
}
/**
*
*
*
* Enables VPC connectivity for standard apps.
*
*
* .google.appengine.v1.VpcAccessConnector vpc_access_connector = 121;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.VpcAccessConnector,
com.google.appengine.v1.VpcAccessConnector.Builder,
com.google.appengine.v1.VpcAccessConnectorOrBuilder>
getVpcAccessConnectorFieldBuilder() {
if (vpcAccessConnectorBuilder_ == null) {
vpcAccessConnectorBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.v1.VpcAccessConnector,
com.google.appengine.v1.VpcAccessConnector.Builder,
com.google.appengine.v1.VpcAccessConnectorOrBuilder>(
getVpcAccessConnector(), getParentForChildren(), isClean());
vpcAccessConnector_ = null;
}
return vpcAccessConnectorBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.appengine.v1.Version)
}
// @@protoc_insertion_point(class_scope:google.appengine.v1.Version)
private static final com.google.appengine.v1.Version DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.v1.Version();
}
public static com.google.appengine.v1.Version getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Version parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.v1.Version getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy