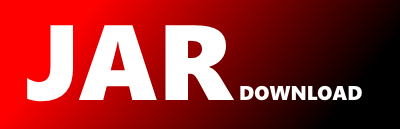
com.google.cloud.compute.v1.AutoscalingPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* Cloud Autoscaler policy.
*
*
* Protobuf type {@code google.cloud.compute.v1.AutoscalingPolicy}
*/
public final class AutoscalingPolicy extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.AutoscalingPolicy)
AutoscalingPolicyOrBuilder {
private static final long serialVersionUID = 0L;
// Use AutoscalingPolicy.newBuilder() to construct.
private AutoscalingPolicy(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AutoscalingPolicy() {
customMetricUtilizations_ = java.util.Collections.emptyList();
mode_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new AutoscalingPolicy();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_AutoscalingPolicy_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 355416580:
return internalGetScalingSchedules();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_AutoscalingPolicy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.AutoscalingPolicy.class,
com.google.cloud.compute.v1.AutoscalingPolicy.Builder.class);
}
/**
*
*
*
* Defines the operating mode for this policy. The following modes are available: - OFF: Disables the autoscaler but maintains its configuration. - ONLY_SCALE_OUT: Restricts the autoscaler to add VM instances only. - ON: Enables all autoscaler activities according to its policy. For more information, see "Turning off or restricting an autoscaler"
*
*
* Protobuf enum {@code google.cloud.compute.v1.AutoscalingPolicy.Mode}
*/
public enum Mode implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_MODE = 0;
*/
UNDEFINED_MODE(0),
/**
*
*
*
* Do not automatically scale the MIG in or out. The recommended_size field contains the size of MIG that would be set if the actuation mode was enabled.
*
*
* OFF = 78159;
*/
OFF(78159),
/**
*
*
*
* Automatically scale the MIG in and out according to the policy.
*
*
* ON = 2527;
*/
ON(2527),
/**
*
*
*
* Automatically create VMs according to the policy, but do not scale the MIG in.
*
*
* ONLY_SCALE_OUT = 152713670;
*/
ONLY_SCALE_OUT(152713670),
/**
*
*
*
* Automatically create VMs according to the policy, but do not scale the MIG in.
*
*
* ONLY_UP = 478095374;
*/
ONLY_UP(478095374),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_MODE = 0;
*/
public static final int UNDEFINED_MODE_VALUE = 0;
/**
*
*
*
* Do not automatically scale the MIG in or out. The recommended_size field contains the size of MIG that would be set if the actuation mode was enabled.
*
*
* OFF = 78159;
*/
public static final int OFF_VALUE = 78159;
/**
*
*
*
* Automatically scale the MIG in and out according to the policy.
*
*
* ON = 2527;
*/
public static final int ON_VALUE = 2527;
/**
*
*
*
* Automatically create VMs according to the policy, but do not scale the MIG in.
*
*
* ONLY_SCALE_OUT = 152713670;
*/
public static final int ONLY_SCALE_OUT_VALUE = 152713670;
/**
*
*
*
* Automatically create VMs according to the policy, but do not scale the MIG in.
*
*
* ONLY_UP = 478095374;
*/
public static final int ONLY_UP_VALUE = 478095374;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Mode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Mode forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_MODE;
case 78159:
return OFF;
case 2527:
return ON;
case 152713670:
return ONLY_SCALE_OUT;
case 478095374:
return ONLY_UP;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Mode findValueByNumber(int number) {
return Mode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.AutoscalingPolicy.getDescriptor().getEnumTypes().get(0);
}
private static final Mode[] VALUES = values();
public static Mode valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Mode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.AutoscalingPolicy.Mode)
}
private int bitField0_;
public static final int COOL_DOWN_PERIOD_SEC_FIELD_NUMBER = 107692954;
private int coolDownPeriodSec_ = 0;
/**
*
*
*
* The number of seconds that your application takes to initialize on a VM instance. This is referred to as the [initialization period](/compute/docs/autoscaler#cool_down_period). Specifying an accurate initialization period improves autoscaler decisions. For example, when scaling out, the autoscaler ignores data from VMs that are still initializing because those VMs might not yet represent normal usage of your application. The default initialization period is 60 seconds. Initialization periods might vary because of numerous factors. We recommend that you test how long your application takes to initialize. To do this, create a VM and time your application's startup process.
*
*
* optional int32 cool_down_period_sec = 107692954;
*
* @return Whether the coolDownPeriodSec field is set.
*/
@java.lang.Override
public boolean hasCoolDownPeriodSec() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The number of seconds that your application takes to initialize on a VM instance. This is referred to as the [initialization period](/compute/docs/autoscaler#cool_down_period). Specifying an accurate initialization period improves autoscaler decisions. For example, when scaling out, the autoscaler ignores data from VMs that are still initializing because those VMs might not yet represent normal usage of your application. The default initialization period is 60 seconds. Initialization periods might vary because of numerous factors. We recommend that you test how long your application takes to initialize. To do this, create a VM and time your application's startup process.
*
*
* optional int32 cool_down_period_sec = 107692954;
*
* @return The coolDownPeriodSec.
*/
@java.lang.Override
public int getCoolDownPeriodSec() {
return coolDownPeriodSec_;
}
public static final int CPU_UTILIZATION_FIELD_NUMBER = 381211147;
private com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpuUtilization_;
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*
* @return Whether the cpuUtilization field is set.
*/
@java.lang.Override
public boolean hasCpuUtilization() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*
* @return The cpuUtilization.
*/
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization getCpuUtilization() {
return cpuUtilization_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization.getDefaultInstance()
: cpuUtilization_;
}
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilizationOrBuilder
getCpuUtilizationOrBuilder() {
return cpuUtilization_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization.getDefaultInstance()
: cpuUtilization_;
}
public static final int CUSTOM_METRIC_UTILIZATIONS_FIELD_NUMBER = 131972850;
@SuppressWarnings("serial")
private java.util.List
customMetricUtilizations_;
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
@java.lang.Override
public java.util.List
getCustomMetricUtilizationsList() {
return customMetricUtilizations_;
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
@java.lang.Override
public java.util.List<
? extends com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilizationOrBuilder>
getCustomMetricUtilizationsOrBuilderList() {
return customMetricUtilizations_;
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
@java.lang.Override
public int getCustomMetricUtilizationsCount() {
return customMetricUtilizations_.size();
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization
getCustomMetricUtilizations(int index) {
return customMetricUtilizations_.get(index);
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilizationOrBuilder
getCustomMetricUtilizationsOrBuilder(int index) {
return customMetricUtilizations_.get(index);
}
public static final int LOAD_BALANCING_UTILIZATION_FIELD_NUMBER = 429746403;
private com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization
loadBalancingUtilization_;
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*
* @return Whether the loadBalancingUtilization field is set.
*/
@java.lang.Override
public boolean hasLoadBalancingUtilization() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*
* @return The loadBalancingUtilization.
*/
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization
getLoadBalancingUtilization() {
return loadBalancingUtilization_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization.getDefaultInstance()
: loadBalancingUtilization_;
}
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilizationOrBuilder
getLoadBalancingUtilizationOrBuilder() {
return loadBalancingUtilization_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization.getDefaultInstance()
: loadBalancingUtilization_;
}
public static final int MAX_NUM_REPLICAS_FIELD_NUMBER = 62327375;
private int maxNumReplicas_ = 0;
/**
*
*
*
* The maximum number of instances that the autoscaler can scale out to. This is required when creating or updating an autoscaler. The maximum number of replicas must not be lower than minimal number of replicas.
*
*
* optional int32 max_num_replicas = 62327375;
*
* @return Whether the maxNumReplicas field is set.
*/
@java.lang.Override
public boolean hasMaxNumReplicas() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The maximum number of instances that the autoscaler can scale out to. This is required when creating or updating an autoscaler. The maximum number of replicas must not be lower than minimal number of replicas.
*
*
* optional int32 max_num_replicas = 62327375;
*
* @return The maxNumReplicas.
*/
@java.lang.Override
public int getMaxNumReplicas() {
return maxNumReplicas_;
}
public static final int MIN_NUM_REPLICAS_FIELD_NUMBER = 535329825;
private int minNumReplicas_ = 0;
/**
*
*
*
* The minimum number of replicas that the autoscaler can scale in to. This cannot be less than 0. If not provided, autoscaler chooses a default value depending on maximum number of instances allowed.
*
*
* optional int32 min_num_replicas = 535329825;
*
* @return Whether the minNumReplicas field is set.
*/
@java.lang.Override
public boolean hasMinNumReplicas() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* The minimum number of replicas that the autoscaler can scale in to. This cannot be less than 0. If not provided, autoscaler chooses a default value depending on maximum number of instances allowed.
*
*
* optional int32 min_num_replicas = 535329825;
*
* @return The minNumReplicas.
*/
@java.lang.Override
public int getMinNumReplicas() {
return minNumReplicas_;
}
public static final int MODE_FIELD_NUMBER = 3357091;
@SuppressWarnings("serial")
private volatile java.lang.Object mode_ = "";
/**
*
*
*
* Defines the operating mode for this policy. The following modes are available: - OFF: Disables the autoscaler but maintains its configuration. - ONLY_SCALE_OUT: Restricts the autoscaler to add VM instances only. - ON: Enables all autoscaler activities according to its policy. For more information, see "Turning off or restricting an autoscaler"
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return Whether the mode field is set.
*/
@java.lang.Override
public boolean hasMode() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Defines the operating mode for this policy. The following modes are available: - OFF: Disables the autoscaler but maintains its configuration. - ONLY_SCALE_OUT: Restricts the autoscaler to add VM instances only. - ON: Enables all autoscaler activities according to its policy. For more information, see "Turning off or restricting an autoscaler"
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return The mode.
*/
@java.lang.Override
public java.lang.String getMode() {
java.lang.Object ref = mode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mode_ = s;
return s;
}
}
/**
*
*
*
* Defines the operating mode for this policy. The following modes are available: - OFF: Disables the autoscaler but maintains its configuration. - ONLY_SCALE_OUT: Restricts the autoscaler to add VM instances only. - ON: Enables all autoscaler activities according to its policy. For more information, see "Turning off or restricting an autoscaler"
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return The bytes for mode.
*/
@java.lang.Override
public com.google.protobuf.ByteString getModeBytes() {
java.lang.Object ref = mode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
mode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCALE_IN_CONTROL_FIELD_NUMBER = 527670872;
private com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl scaleInControl_;
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*
* @return Whether the scaleInControl field is set.
*/
@java.lang.Override
public boolean hasScaleInControl() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*
* @return The scaleInControl.
*/
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl getScaleInControl() {
return scaleInControl_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl.getDefaultInstance()
: scaleInControl_;
}
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicyScaleInControlOrBuilder
getScaleInControlOrBuilder() {
return scaleInControl_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl.getDefaultInstance()
: scaleInControl_;
}
public static final int SCALING_SCHEDULES_FIELD_NUMBER = 355416580;
private static final class ScalingSchedulesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
defaultEntry =
com.google.protobuf.MapEntry
.
newDefaultInstance(
com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_AutoscalingPolicy_ScalingSchedulesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule
.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
scalingSchedules_;
private com.google.protobuf.MapField<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
internalGetScalingSchedules() {
if (scalingSchedules_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ScalingSchedulesDefaultEntryHolder.defaultEntry);
}
return scalingSchedules_;
}
public int getScalingSchedulesCount() {
return internalGetScalingSchedules().getMap().size();
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
@java.lang.Override
public boolean containsScalingSchedules(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetScalingSchedules().getMap().containsKey(key);
}
/** Use {@link #getScalingSchedulesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
getScalingSchedules() {
return getScalingSchedulesMap();
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
@java.lang.Override
public java.util.Map<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
getScalingSchedulesMap() {
return internalGetScalingSchedules().getMap();
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
@java.lang.Override
public /* nullable */ com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule
getScalingSchedulesOrDefault(
java.lang.String key,
/* nullable */
com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetScalingSchedules().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule getScalingSchedulesOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetScalingSchedules().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3357091, mode_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(62327375, maxNumReplicas_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(107692954, coolDownPeriodSec_);
}
for (int i = 0; i < customMetricUtilizations_.size(); i++) {
output.writeMessage(131972850, customMetricUtilizations_.get(i));
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output,
internalGetScalingSchedules(),
ScalingSchedulesDefaultEntryHolder.defaultEntry,
355416580);
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(381211147, getCpuUtilization());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(429746403, getLoadBalancingUtilization());
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(527670872, getScaleInControl());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt32(535329825, minNumReplicas_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3357091, mode_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(62327375, maxNumReplicas_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(107692954, coolDownPeriodSec_);
}
for (int i = 0; i < customMetricUtilizations_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
131972850, customMetricUtilizations_.get(i));
}
for (java.util.Map.Entry<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
entry : internalGetScalingSchedules().getMap().entrySet()) {
com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
scalingSchedules__ =
ScalingSchedulesDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(355416580, scalingSchedules__);
}
if (((bitField0_ & 0x00000002) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(381211147, getCpuUtilization());
}
if (((bitField0_ & 0x00000004) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
429746403, getLoadBalancingUtilization());
}
if (((bitField0_ & 0x00000040) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(527670872, getScaleInControl());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(535329825, minNumReplicas_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.AutoscalingPolicy)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.AutoscalingPolicy other =
(com.google.cloud.compute.v1.AutoscalingPolicy) obj;
if (hasCoolDownPeriodSec() != other.hasCoolDownPeriodSec()) return false;
if (hasCoolDownPeriodSec()) {
if (getCoolDownPeriodSec() != other.getCoolDownPeriodSec()) return false;
}
if (hasCpuUtilization() != other.hasCpuUtilization()) return false;
if (hasCpuUtilization()) {
if (!getCpuUtilization().equals(other.getCpuUtilization())) return false;
}
if (!getCustomMetricUtilizationsList().equals(other.getCustomMetricUtilizationsList()))
return false;
if (hasLoadBalancingUtilization() != other.hasLoadBalancingUtilization()) return false;
if (hasLoadBalancingUtilization()) {
if (!getLoadBalancingUtilization().equals(other.getLoadBalancingUtilization())) return false;
}
if (hasMaxNumReplicas() != other.hasMaxNumReplicas()) return false;
if (hasMaxNumReplicas()) {
if (getMaxNumReplicas() != other.getMaxNumReplicas()) return false;
}
if (hasMinNumReplicas() != other.hasMinNumReplicas()) return false;
if (hasMinNumReplicas()) {
if (getMinNumReplicas() != other.getMinNumReplicas()) return false;
}
if (hasMode() != other.hasMode()) return false;
if (hasMode()) {
if (!getMode().equals(other.getMode())) return false;
}
if (hasScaleInControl() != other.hasScaleInControl()) return false;
if (hasScaleInControl()) {
if (!getScaleInControl().equals(other.getScaleInControl())) return false;
}
if (!internalGetScalingSchedules().equals(other.internalGetScalingSchedules())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCoolDownPeriodSec()) {
hash = (37 * hash) + COOL_DOWN_PERIOD_SEC_FIELD_NUMBER;
hash = (53 * hash) + getCoolDownPeriodSec();
}
if (hasCpuUtilization()) {
hash = (37 * hash) + CPU_UTILIZATION_FIELD_NUMBER;
hash = (53 * hash) + getCpuUtilization().hashCode();
}
if (getCustomMetricUtilizationsCount() > 0) {
hash = (37 * hash) + CUSTOM_METRIC_UTILIZATIONS_FIELD_NUMBER;
hash = (53 * hash) + getCustomMetricUtilizationsList().hashCode();
}
if (hasLoadBalancingUtilization()) {
hash = (37 * hash) + LOAD_BALANCING_UTILIZATION_FIELD_NUMBER;
hash = (53 * hash) + getLoadBalancingUtilization().hashCode();
}
if (hasMaxNumReplicas()) {
hash = (37 * hash) + MAX_NUM_REPLICAS_FIELD_NUMBER;
hash = (53 * hash) + getMaxNumReplicas();
}
if (hasMinNumReplicas()) {
hash = (37 * hash) + MIN_NUM_REPLICAS_FIELD_NUMBER;
hash = (53 * hash) + getMinNumReplicas();
}
if (hasMode()) {
hash = (37 * hash) + MODE_FIELD_NUMBER;
hash = (53 * hash) + getMode().hashCode();
}
if (hasScaleInControl()) {
hash = (37 * hash) + SCALE_IN_CONTROL_FIELD_NUMBER;
hash = (53 * hash) + getScaleInControl().hashCode();
}
if (!internalGetScalingSchedules().getMap().isEmpty()) {
hash = (37 * hash) + SCALING_SCHEDULES_FIELD_NUMBER;
hash = (53 * hash) + internalGetScalingSchedules().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.AutoscalingPolicy parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.AutoscalingPolicy prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Cloud Autoscaler policy.
*
*
* Protobuf type {@code google.cloud.compute.v1.AutoscalingPolicy}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.AutoscalingPolicy)
com.google.cloud.compute.v1.AutoscalingPolicyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_AutoscalingPolicy_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 355416580:
return internalGetScalingSchedules();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 355416580:
return internalGetMutableScalingSchedules();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_AutoscalingPolicy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.AutoscalingPolicy.class,
com.google.cloud.compute.v1.AutoscalingPolicy.Builder.class);
}
// Construct using com.google.cloud.compute.v1.AutoscalingPolicy.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getCpuUtilizationFieldBuilder();
getCustomMetricUtilizationsFieldBuilder();
getLoadBalancingUtilizationFieldBuilder();
getScaleInControlFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
coolDownPeriodSec_ = 0;
cpuUtilization_ = null;
if (cpuUtilizationBuilder_ != null) {
cpuUtilizationBuilder_.dispose();
cpuUtilizationBuilder_ = null;
}
if (customMetricUtilizationsBuilder_ == null) {
customMetricUtilizations_ = java.util.Collections.emptyList();
} else {
customMetricUtilizations_ = null;
customMetricUtilizationsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
loadBalancingUtilization_ = null;
if (loadBalancingUtilizationBuilder_ != null) {
loadBalancingUtilizationBuilder_.dispose();
loadBalancingUtilizationBuilder_ = null;
}
maxNumReplicas_ = 0;
minNumReplicas_ = 0;
mode_ = "";
scaleInControl_ = null;
if (scaleInControlBuilder_ != null) {
scaleInControlBuilder_.dispose();
scaleInControlBuilder_ = null;
}
internalGetMutableScalingSchedules().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_AutoscalingPolicy_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicy getDefaultInstanceForType() {
return com.google.cloud.compute.v1.AutoscalingPolicy.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicy build() {
com.google.cloud.compute.v1.AutoscalingPolicy result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicy buildPartial() {
com.google.cloud.compute.v1.AutoscalingPolicy result =
new com.google.cloud.compute.v1.AutoscalingPolicy(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.cloud.compute.v1.AutoscalingPolicy result) {
if (customMetricUtilizationsBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
customMetricUtilizations_ =
java.util.Collections.unmodifiableList(customMetricUtilizations_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.customMetricUtilizations_ = customMetricUtilizations_;
} else {
result.customMetricUtilizations_ = customMetricUtilizationsBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.compute.v1.AutoscalingPolicy result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.coolDownPeriodSec_ = coolDownPeriodSec_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.cpuUtilization_ =
cpuUtilizationBuilder_ == null ? cpuUtilization_ : cpuUtilizationBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.loadBalancingUtilization_ =
loadBalancingUtilizationBuilder_ == null
? loadBalancingUtilization_
: loadBalancingUtilizationBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.maxNumReplicas_ = maxNumReplicas_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.minNumReplicas_ = minNumReplicas_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.mode_ = mode_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.scaleInControl_ =
scaleInControlBuilder_ == null ? scaleInControl_ : scaleInControlBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.scalingSchedules_ =
internalGetScalingSchedules().build(ScalingSchedulesDefaultEntryHolder.defaultEntry);
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.AutoscalingPolicy) {
return mergeFrom((com.google.cloud.compute.v1.AutoscalingPolicy) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.AutoscalingPolicy other) {
if (other == com.google.cloud.compute.v1.AutoscalingPolicy.getDefaultInstance()) return this;
if (other.hasCoolDownPeriodSec()) {
setCoolDownPeriodSec(other.getCoolDownPeriodSec());
}
if (other.hasCpuUtilization()) {
mergeCpuUtilization(other.getCpuUtilization());
}
if (customMetricUtilizationsBuilder_ == null) {
if (!other.customMetricUtilizations_.isEmpty()) {
if (customMetricUtilizations_.isEmpty()) {
customMetricUtilizations_ = other.customMetricUtilizations_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureCustomMetricUtilizationsIsMutable();
customMetricUtilizations_.addAll(other.customMetricUtilizations_);
}
onChanged();
}
} else {
if (!other.customMetricUtilizations_.isEmpty()) {
if (customMetricUtilizationsBuilder_.isEmpty()) {
customMetricUtilizationsBuilder_.dispose();
customMetricUtilizationsBuilder_ = null;
customMetricUtilizations_ = other.customMetricUtilizations_;
bitField0_ = (bitField0_ & ~0x00000004);
customMetricUtilizationsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getCustomMetricUtilizationsFieldBuilder()
: null;
} else {
customMetricUtilizationsBuilder_.addAllMessages(other.customMetricUtilizations_);
}
}
}
if (other.hasLoadBalancingUtilization()) {
mergeLoadBalancingUtilization(other.getLoadBalancingUtilization());
}
if (other.hasMaxNumReplicas()) {
setMaxNumReplicas(other.getMaxNumReplicas());
}
if (other.hasMinNumReplicas()) {
setMinNumReplicas(other.getMinNumReplicas());
}
if (other.hasMode()) {
mode_ = other.mode_;
bitField0_ |= 0x00000040;
onChanged();
}
if (other.hasScaleInControl()) {
mergeScaleInControl(other.getScaleInControl());
}
internalGetMutableScalingSchedules().mergeFrom(other.internalGetScalingSchedules());
bitField0_ |= 0x00000100;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 26856730:
{
mode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 26856730
case 498619000:
{
maxNumReplicas_ = input.readInt32();
bitField0_ |= 0x00000010;
break;
} // case 498619000
case 861543632:
{
coolDownPeriodSec_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 861543632
case 1055782802:
{
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization m =
input.readMessage(
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization
.parser(),
extensionRegistry);
if (customMetricUtilizationsBuilder_ == null) {
ensureCustomMetricUtilizationsIsMutable();
customMetricUtilizations_.add(m);
} else {
customMetricUtilizationsBuilder_.addMessage(m);
}
break;
} // case 1055782802
case -1451634654:
{
com.google.protobuf.MapEntry<
java.lang.String,
com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
scalingSchedules__ =
input.readMessage(
ScalingSchedulesDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableScalingSchedules()
.ensureBuilderMap()
.put(scalingSchedules__.getKey(), scalingSchedules__.getValue());
bitField0_ |= 0x00000100;
break;
} // case -1451634654
case -1245278118:
{
input.readMessage(getCpuUtilizationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case -1245278118
case -856996070:
{
input.readMessage(
getLoadBalancingUtilizationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case -856996070
case -73600318:
{
input.readMessage(getScaleInControlFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case -73600318
case -12328696:
{
minNumReplicas_ = input.readInt32();
bitField0_ |= 0x00000020;
break;
} // case -12328696
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int coolDownPeriodSec_;
/**
*
*
*
* The number of seconds that your application takes to initialize on a VM instance. This is referred to as the [initialization period](/compute/docs/autoscaler#cool_down_period). Specifying an accurate initialization period improves autoscaler decisions. For example, when scaling out, the autoscaler ignores data from VMs that are still initializing because those VMs might not yet represent normal usage of your application. The default initialization period is 60 seconds. Initialization periods might vary because of numerous factors. We recommend that you test how long your application takes to initialize. To do this, create a VM and time your application's startup process.
*
*
* optional int32 cool_down_period_sec = 107692954;
*
* @return Whether the coolDownPeriodSec field is set.
*/
@java.lang.Override
public boolean hasCoolDownPeriodSec() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The number of seconds that your application takes to initialize on a VM instance. This is referred to as the [initialization period](/compute/docs/autoscaler#cool_down_period). Specifying an accurate initialization period improves autoscaler decisions. For example, when scaling out, the autoscaler ignores data from VMs that are still initializing because those VMs might not yet represent normal usage of your application. The default initialization period is 60 seconds. Initialization periods might vary because of numerous factors. We recommend that you test how long your application takes to initialize. To do this, create a VM and time your application's startup process.
*
*
* optional int32 cool_down_period_sec = 107692954;
*
* @return The coolDownPeriodSec.
*/
@java.lang.Override
public int getCoolDownPeriodSec() {
return coolDownPeriodSec_;
}
/**
*
*
*
* The number of seconds that your application takes to initialize on a VM instance. This is referred to as the [initialization period](/compute/docs/autoscaler#cool_down_period). Specifying an accurate initialization period improves autoscaler decisions. For example, when scaling out, the autoscaler ignores data from VMs that are still initializing because those VMs might not yet represent normal usage of your application. The default initialization period is 60 seconds. Initialization periods might vary because of numerous factors. We recommend that you test how long your application takes to initialize. To do this, create a VM and time your application's startup process.
*
*
* optional int32 cool_down_period_sec = 107692954;
*
* @param value The coolDownPeriodSec to set.
* @return This builder for chaining.
*/
public Builder setCoolDownPeriodSec(int value) {
coolDownPeriodSec_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The number of seconds that your application takes to initialize on a VM instance. This is referred to as the [initialization period](/compute/docs/autoscaler#cool_down_period). Specifying an accurate initialization period improves autoscaler decisions. For example, when scaling out, the autoscaler ignores data from VMs that are still initializing because those VMs might not yet represent normal usage of your application. The default initialization period is 60 seconds. Initialization periods might vary because of numerous factors. We recommend that you test how long your application takes to initialize. To do this, create a VM and time your application's startup process.
*
*
* optional int32 cool_down_period_sec = 107692954;
*
* @return This builder for chaining.
*/
public Builder clearCoolDownPeriodSec() {
bitField0_ = (bitField0_ & ~0x00000001);
coolDownPeriodSec_ = 0;
onChanged();
return this;
}
private com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpuUtilization_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization,
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilizationOrBuilder>
cpuUtilizationBuilder_;
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*
* @return Whether the cpuUtilization field is set.
*/
public boolean hasCpuUtilization() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*
* @return The cpuUtilization.
*/
public com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization getCpuUtilization() {
if (cpuUtilizationBuilder_ == null) {
return cpuUtilization_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization.getDefaultInstance()
: cpuUtilization_;
} else {
return cpuUtilizationBuilder_.getMessage();
}
}
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*/
public Builder setCpuUtilization(
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization value) {
if (cpuUtilizationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cpuUtilization_ = value;
} else {
cpuUtilizationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*/
public Builder setCpuUtilization(
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization.Builder builderForValue) {
if (cpuUtilizationBuilder_ == null) {
cpuUtilization_ = builderForValue.build();
} else {
cpuUtilizationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*/
public Builder mergeCpuUtilization(
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization value) {
if (cpuUtilizationBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)
&& cpuUtilization_ != null
&& cpuUtilization_
!= com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization
.getDefaultInstance()) {
getCpuUtilizationBuilder().mergeFrom(value);
} else {
cpuUtilization_ = value;
}
} else {
cpuUtilizationBuilder_.mergeFrom(value);
}
if (cpuUtilization_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*/
public Builder clearCpuUtilization() {
bitField0_ = (bitField0_ & ~0x00000002);
cpuUtilization_ = null;
if (cpuUtilizationBuilder_ != null) {
cpuUtilizationBuilder_.dispose();
cpuUtilizationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization.Builder
getCpuUtilizationBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getCpuUtilizationFieldBuilder().getBuilder();
}
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilizationOrBuilder
getCpuUtilizationOrBuilder() {
if (cpuUtilizationBuilder_ != null) {
return cpuUtilizationBuilder_.getMessageOrBuilder();
} else {
return cpuUtilization_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization.getDefaultInstance()
: cpuUtilization_;
}
}
/**
*
*
*
* Defines the CPU utilization policy that allows the autoscaler to scale based on the average CPU utilization of a managed instance group.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyCpuUtilization cpu_utilization = 381211147;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization,
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilizationOrBuilder>
getCpuUtilizationFieldBuilder() {
if (cpuUtilizationBuilder_ == null) {
cpuUtilizationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization,
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilization.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyCpuUtilizationOrBuilder>(
getCpuUtilization(), getParentForChildren(), isClean());
cpuUtilization_ = null;
}
return cpuUtilizationBuilder_;
}
private java.util.List
customMetricUtilizations_ = java.util.Collections.emptyList();
private void ensureCustomMetricUtilizationsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
customMetricUtilizations_ =
new java.util.ArrayList<
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization>(
customMetricUtilizations_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization,
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilizationOrBuilder>
customMetricUtilizationsBuilder_;
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public java.util.List
getCustomMetricUtilizationsList() {
if (customMetricUtilizationsBuilder_ == null) {
return java.util.Collections.unmodifiableList(customMetricUtilizations_);
} else {
return customMetricUtilizationsBuilder_.getMessageList();
}
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public int getCustomMetricUtilizationsCount() {
if (customMetricUtilizationsBuilder_ == null) {
return customMetricUtilizations_.size();
} else {
return customMetricUtilizationsBuilder_.getCount();
}
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization
getCustomMetricUtilizations(int index) {
if (customMetricUtilizationsBuilder_ == null) {
return customMetricUtilizations_.get(index);
} else {
return customMetricUtilizationsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public Builder setCustomMetricUtilizations(
int index, com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization value) {
if (customMetricUtilizationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCustomMetricUtilizationsIsMutable();
customMetricUtilizations_.set(index, value);
onChanged();
} else {
customMetricUtilizationsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public Builder setCustomMetricUtilizations(
int index,
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization.Builder
builderForValue) {
if (customMetricUtilizationsBuilder_ == null) {
ensureCustomMetricUtilizationsIsMutable();
customMetricUtilizations_.set(index, builderForValue.build());
onChanged();
} else {
customMetricUtilizationsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public Builder addCustomMetricUtilizations(
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization value) {
if (customMetricUtilizationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCustomMetricUtilizationsIsMutable();
customMetricUtilizations_.add(value);
onChanged();
} else {
customMetricUtilizationsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public Builder addCustomMetricUtilizations(
int index, com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization value) {
if (customMetricUtilizationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCustomMetricUtilizationsIsMutable();
customMetricUtilizations_.add(index, value);
onChanged();
} else {
customMetricUtilizationsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public Builder addCustomMetricUtilizations(
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization.Builder
builderForValue) {
if (customMetricUtilizationsBuilder_ == null) {
ensureCustomMetricUtilizationsIsMutable();
customMetricUtilizations_.add(builderForValue.build());
onChanged();
} else {
customMetricUtilizationsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public Builder addCustomMetricUtilizations(
int index,
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization.Builder
builderForValue) {
if (customMetricUtilizationsBuilder_ == null) {
ensureCustomMetricUtilizationsIsMutable();
customMetricUtilizations_.add(index, builderForValue.build());
onChanged();
} else {
customMetricUtilizationsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public Builder addAllCustomMetricUtilizations(
java.lang.Iterable<
? extends com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization>
values) {
if (customMetricUtilizationsBuilder_ == null) {
ensureCustomMetricUtilizationsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, customMetricUtilizations_);
onChanged();
} else {
customMetricUtilizationsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public Builder clearCustomMetricUtilizations() {
if (customMetricUtilizationsBuilder_ == null) {
customMetricUtilizations_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
customMetricUtilizationsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public Builder removeCustomMetricUtilizations(int index) {
if (customMetricUtilizationsBuilder_ == null) {
ensureCustomMetricUtilizationsIsMutable();
customMetricUtilizations_.remove(index);
onChanged();
} else {
customMetricUtilizationsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization.Builder
getCustomMetricUtilizationsBuilder(int index) {
return getCustomMetricUtilizationsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilizationOrBuilder
getCustomMetricUtilizationsOrBuilder(int index) {
if (customMetricUtilizationsBuilder_ == null) {
return customMetricUtilizations_.get(index);
} else {
return customMetricUtilizationsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public java.util.List<
? extends com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilizationOrBuilder>
getCustomMetricUtilizationsOrBuilderList() {
if (customMetricUtilizationsBuilder_ != null) {
return customMetricUtilizationsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(customMetricUtilizations_);
}
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization.Builder
addCustomMetricUtilizationsBuilder() {
return getCustomMetricUtilizationsFieldBuilder()
.addBuilder(
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization
.getDefaultInstance());
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization.Builder
addCustomMetricUtilizationsBuilder(int index) {
return getCustomMetricUtilizationsFieldBuilder()
.addBuilder(
index,
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization
.getDefaultInstance());
}
/**
*
*
*
* Configuration parameters of autoscaling based on a custom metric.
*
*
*
* repeated .google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization custom_metric_utilizations = 131972850;
*
*/
public java.util.List<
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization.Builder>
getCustomMetricUtilizationsBuilderList() {
return getCustomMetricUtilizationsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization,
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilizationOrBuilder>
getCustomMetricUtilizationsFieldBuilder() {
if (customMetricUtilizationsBuilder_ == null) {
customMetricUtilizationsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization,
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilization.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyCustomMetricUtilizationOrBuilder>(
customMetricUtilizations_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
customMetricUtilizations_ = null;
}
return customMetricUtilizationsBuilder_;
}
private com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization
loadBalancingUtilization_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization,
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilizationOrBuilder>
loadBalancingUtilizationBuilder_;
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*
* @return Whether the loadBalancingUtilization field is set.
*/
public boolean hasLoadBalancingUtilization() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*
* @return The loadBalancingUtilization.
*/
public com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization
getLoadBalancingUtilization() {
if (loadBalancingUtilizationBuilder_ == null) {
return loadBalancingUtilization_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization
.getDefaultInstance()
: loadBalancingUtilization_;
} else {
return loadBalancingUtilizationBuilder_.getMessage();
}
}
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*/
public Builder setLoadBalancingUtilization(
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization value) {
if (loadBalancingUtilizationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
loadBalancingUtilization_ = value;
} else {
loadBalancingUtilizationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*/
public Builder setLoadBalancingUtilization(
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization.Builder
builderForValue) {
if (loadBalancingUtilizationBuilder_ == null) {
loadBalancingUtilization_ = builderForValue.build();
} else {
loadBalancingUtilizationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*/
public Builder mergeLoadBalancingUtilization(
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization value) {
if (loadBalancingUtilizationBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& loadBalancingUtilization_ != null
&& loadBalancingUtilization_
!= com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization
.getDefaultInstance()) {
getLoadBalancingUtilizationBuilder().mergeFrom(value);
} else {
loadBalancingUtilization_ = value;
}
} else {
loadBalancingUtilizationBuilder_.mergeFrom(value);
}
if (loadBalancingUtilization_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*/
public Builder clearLoadBalancingUtilization() {
bitField0_ = (bitField0_ & ~0x00000008);
loadBalancingUtilization_ = null;
if (loadBalancingUtilizationBuilder_ != null) {
loadBalancingUtilizationBuilder_.dispose();
loadBalancingUtilizationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization.Builder
getLoadBalancingUtilizationBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getLoadBalancingUtilizationFieldBuilder().getBuilder();
}
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilizationOrBuilder
getLoadBalancingUtilizationOrBuilder() {
if (loadBalancingUtilizationBuilder_ != null) {
return loadBalancingUtilizationBuilder_.getMessageOrBuilder();
} else {
return loadBalancingUtilization_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization
.getDefaultInstance()
: loadBalancingUtilization_;
}
}
/**
*
*
*
* Configuration parameters of autoscaling based on load balancer.
*
*
*
* optional .google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization load_balancing_utilization = 429746403;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization,
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilizationOrBuilder>
getLoadBalancingUtilizationFieldBuilder() {
if (loadBalancingUtilizationBuilder_ == null) {
loadBalancingUtilizationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization,
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilization.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyLoadBalancingUtilizationOrBuilder>(
getLoadBalancingUtilization(), getParentForChildren(), isClean());
loadBalancingUtilization_ = null;
}
return loadBalancingUtilizationBuilder_;
}
private int maxNumReplicas_;
/**
*
*
*
* The maximum number of instances that the autoscaler can scale out to. This is required when creating or updating an autoscaler. The maximum number of replicas must not be lower than minimal number of replicas.
*
*
* optional int32 max_num_replicas = 62327375;
*
* @return Whether the maxNumReplicas field is set.
*/
@java.lang.Override
public boolean hasMaxNumReplicas() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* The maximum number of instances that the autoscaler can scale out to. This is required when creating or updating an autoscaler. The maximum number of replicas must not be lower than minimal number of replicas.
*
*
* optional int32 max_num_replicas = 62327375;
*
* @return The maxNumReplicas.
*/
@java.lang.Override
public int getMaxNumReplicas() {
return maxNumReplicas_;
}
/**
*
*
*
* The maximum number of instances that the autoscaler can scale out to. This is required when creating or updating an autoscaler. The maximum number of replicas must not be lower than minimal number of replicas.
*
*
* optional int32 max_num_replicas = 62327375;
*
* @param value The maxNumReplicas to set.
* @return This builder for chaining.
*/
public Builder setMaxNumReplicas(int value) {
maxNumReplicas_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* The maximum number of instances that the autoscaler can scale out to. This is required when creating or updating an autoscaler. The maximum number of replicas must not be lower than minimal number of replicas.
*
*
* optional int32 max_num_replicas = 62327375;
*
* @return This builder for chaining.
*/
public Builder clearMaxNumReplicas() {
bitField0_ = (bitField0_ & ~0x00000010);
maxNumReplicas_ = 0;
onChanged();
return this;
}
private int minNumReplicas_;
/**
*
*
*
* The minimum number of replicas that the autoscaler can scale in to. This cannot be less than 0. If not provided, autoscaler chooses a default value depending on maximum number of instances allowed.
*
*
* optional int32 min_num_replicas = 535329825;
*
* @return Whether the minNumReplicas field is set.
*/
@java.lang.Override
public boolean hasMinNumReplicas() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* The minimum number of replicas that the autoscaler can scale in to. This cannot be less than 0. If not provided, autoscaler chooses a default value depending on maximum number of instances allowed.
*
*
* optional int32 min_num_replicas = 535329825;
*
* @return The minNumReplicas.
*/
@java.lang.Override
public int getMinNumReplicas() {
return minNumReplicas_;
}
/**
*
*
*
* The minimum number of replicas that the autoscaler can scale in to. This cannot be less than 0. If not provided, autoscaler chooses a default value depending on maximum number of instances allowed.
*
*
* optional int32 min_num_replicas = 535329825;
*
* @param value The minNumReplicas to set.
* @return This builder for chaining.
*/
public Builder setMinNumReplicas(int value) {
minNumReplicas_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* The minimum number of replicas that the autoscaler can scale in to. This cannot be less than 0. If not provided, autoscaler chooses a default value depending on maximum number of instances allowed.
*
*
* optional int32 min_num_replicas = 535329825;
*
* @return This builder for chaining.
*/
public Builder clearMinNumReplicas() {
bitField0_ = (bitField0_ & ~0x00000020);
minNumReplicas_ = 0;
onChanged();
return this;
}
private java.lang.Object mode_ = "";
/**
*
*
*
* Defines the operating mode for this policy. The following modes are available: - OFF: Disables the autoscaler but maintains its configuration. - ONLY_SCALE_OUT: Restricts the autoscaler to add VM instances only. - ON: Enables all autoscaler activities according to its policy. For more information, see "Turning off or restricting an autoscaler"
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return Whether the mode field is set.
*/
public boolean hasMode() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Defines the operating mode for this policy. The following modes are available: - OFF: Disables the autoscaler but maintains its configuration. - ONLY_SCALE_OUT: Restricts the autoscaler to add VM instances only. - ON: Enables all autoscaler activities according to its policy. For more information, see "Turning off or restricting an autoscaler"
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return The mode.
*/
public java.lang.String getMode() {
java.lang.Object ref = mode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Defines the operating mode for this policy. The following modes are available: - OFF: Disables the autoscaler but maintains its configuration. - ONLY_SCALE_OUT: Restricts the autoscaler to add VM instances only. - ON: Enables all autoscaler activities according to its policy. For more information, see "Turning off or restricting an autoscaler"
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return The bytes for mode.
*/
public com.google.protobuf.ByteString getModeBytes() {
java.lang.Object ref = mode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
mode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Defines the operating mode for this policy. The following modes are available: - OFF: Disables the autoscaler but maintains its configuration. - ONLY_SCALE_OUT: Restricts the autoscaler to add VM instances only. - ON: Enables all autoscaler activities according to its policy. For more information, see "Turning off or restricting an autoscaler"
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @param value The mode to set.
* @return This builder for chaining.
*/
public Builder setMode(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
mode_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Defines the operating mode for this policy. The following modes are available: - OFF: Disables the autoscaler but maintains its configuration. - ONLY_SCALE_OUT: Restricts the autoscaler to add VM instances only. - ON: Enables all autoscaler activities according to its policy. For more information, see "Turning off or restricting an autoscaler"
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return This builder for chaining.
*/
public Builder clearMode() {
mode_ = getDefaultInstance().getMode();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* Defines the operating mode for this policy. The following modes are available: - OFF: Disables the autoscaler but maintains its configuration. - ONLY_SCALE_OUT: Restricts the autoscaler to add VM instances only. - ON: Enables all autoscaler activities according to its policy. For more information, see "Turning off or restricting an autoscaler"
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @param value The bytes for mode to set.
* @return This builder for chaining.
*/
public Builder setModeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
mode_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl scaleInControl_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl,
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControlOrBuilder>
scaleInControlBuilder_;
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*
* @return Whether the scaleInControl field is set.
*/
public boolean hasScaleInControl() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*
* @return The scaleInControl.
*/
public com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl getScaleInControl() {
if (scaleInControlBuilder_ == null) {
return scaleInControl_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl.getDefaultInstance()
: scaleInControl_;
} else {
return scaleInControlBuilder_.getMessage();
}
}
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*/
public Builder setScaleInControl(
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl value) {
if (scaleInControlBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scaleInControl_ = value;
} else {
scaleInControlBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*/
public Builder setScaleInControl(
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl.Builder builderForValue) {
if (scaleInControlBuilder_ == null) {
scaleInControl_ = builderForValue.build();
} else {
scaleInControlBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*/
public Builder mergeScaleInControl(
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl value) {
if (scaleInControlBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)
&& scaleInControl_ != null
&& scaleInControl_
!= com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl
.getDefaultInstance()) {
getScaleInControlBuilder().mergeFrom(value);
} else {
scaleInControl_ = value;
}
} else {
scaleInControlBuilder_.mergeFrom(value);
}
if (scaleInControl_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*/
public Builder clearScaleInControl() {
bitField0_ = (bitField0_ & ~0x00000080);
scaleInControl_ = null;
if (scaleInControlBuilder_ != null) {
scaleInControlBuilder_.dispose();
scaleInControlBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl.Builder
getScaleInControlBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getScaleInControlFieldBuilder().getBuilder();
}
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyScaleInControlOrBuilder
getScaleInControlOrBuilder() {
if (scaleInControlBuilder_ != null) {
return scaleInControlBuilder_.getMessageOrBuilder();
} else {
return scaleInControl_ == null
? com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl.getDefaultInstance()
: scaleInControl_;
}
}
/**
*
* optional .google.cloud.compute.v1.AutoscalingPolicyScaleInControl scale_in_control = 527670872;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl,
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControlOrBuilder>
getScaleInControlFieldBuilder() {
if (scaleInControlBuilder_ == null) {
scaleInControlBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl,
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControl.Builder,
com.google.cloud.compute.v1.AutoscalingPolicyScaleInControlOrBuilder>(
getScaleInControl(), getParentForChildren(), isClean());
scaleInControl_ = null;
}
return scaleInControlBuilder_;
}
private static final class ScalingSchedulesConverter
implements com.google.protobuf.MapFieldBuilder.Converter<
java.lang.String,
com.google.cloud.compute.v1.AutoscalingPolicyScalingScheduleOrBuilder,
com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> {
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule build(
com.google.cloud.compute.v1.AutoscalingPolicyScalingScheduleOrBuilder val) {
if (val instanceof com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule) {
return (com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule) val;
}
return ((com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
defaultEntry() {
return ScalingSchedulesDefaultEntryHolder.defaultEntry;
}
};
private static final ScalingSchedulesConverter scalingSchedulesConverter =
new ScalingSchedulesConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.compute.v1.AutoscalingPolicyScalingScheduleOrBuilder,
com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule,
com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule.Builder>
scalingSchedules_;
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.compute.v1.AutoscalingPolicyScalingScheduleOrBuilder,
com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule,
com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule.Builder>
internalGetScalingSchedules() {
if (scalingSchedules_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(scalingSchedulesConverter);
}
return scalingSchedules_;
}
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.compute.v1.AutoscalingPolicyScalingScheduleOrBuilder,
com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule,
com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule.Builder>
internalGetMutableScalingSchedules() {
if (scalingSchedules_ == null) {
scalingSchedules_ = new com.google.protobuf.MapFieldBuilder<>(scalingSchedulesConverter);
}
bitField0_ |= 0x00000100;
onChanged();
return scalingSchedules_;
}
public int getScalingSchedulesCount() {
return internalGetScalingSchedules().ensureBuilderMap().size();
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
@java.lang.Override
public boolean containsScalingSchedules(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetScalingSchedules().ensureBuilderMap().containsKey(key);
}
/** Use {@link #getScalingSchedulesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
getScalingSchedules() {
return getScalingSchedulesMap();
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
@java.lang.Override
public java.util.Map<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
getScalingSchedulesMap() {
return internalGetScalingSchedules().getImmutableMap();
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
@java.lang.Override
public /* nullable */ com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule
getScalingSchedulesOrDefault(
java.lang.String key,
/* nullable */
com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map<
java.lang.String,
com.google.cloud.compute.v1.AutoscalingPolicyScalingScheduleOrBuilder>
map = internalGetMutableScalingSchedules().ensureBuilderMap();
return map.containsKey(key) ? scalingSchedulesConverter.build(map.get(key)) : defaultValue;
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule getScalingSchedulesOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map<
java.lang.String,
com.google.cloud.compute.v1.AutoscalingPolicyScalingScheduleOrBuilder>
map = internalGetMutableScalingSchedules().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return scalingSchedulesConverter.build(map.get(key));
}
public Builder clearScalingSchedules() {
bitField0_ = (bitField0_ & ~0x00000100);
internalGetMutableScalingSchedules().clear();
return this;
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
public Builder removeScalingSchedules(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableScalingSchedules().ensureBuilderMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
getMutableScalingSchedules() {
bitField0_ |= 0x00000100;
return internalGetMutableScalingSchedules().ensureMessageMap();
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
public Builder putScalingSchedules(
java.lang.String key, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableScalingSchedules().ensureBuilderMap().put(key, value);
bitField0_ |= 0x00000100;
return this;
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
public Builder putAllScalingSchedules(
java.util.Map<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
values) {
for (java.util.Map.Entry<
java.lang.String, com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule>
e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableScalingSchedules().ensureBuilderMap().putAll(values);
bitField0_ |= 0x00000100;
return this;
}
/**
*
*
*
* Scaling schedules defined for an autoscaler. Multiple schedules can be set on an autoscaler, and they can overlap. During overlapping periods the greatest min_required_replicas of all scaling schedules is applied. Up to 128 scaling schedules are allowed.
*
*
*
* map<string, .google.cloud.compute.v1.AutoscalingPolicyScalingSchedule> scaling_schedules = 355416580;
*
*/
public com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule.Builder
putScalingSchedulesBuilderIfAbsent(java.lang.String key) {
java.util.Map<
java.lang.String,
com.google.cloud.compute.v1.AutoscalingPolicyScalingScheduleOrBuilder>
builderMap = internalGetMutableScalingSchedules().ensureBuilderMap();
com.google.cloud.compute.v1.AutoscalingPolicyScalingScheduleOrBuilder entry =
builderMap.get(key);
if (entry == null) {
entry = com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule) {
entry = ((com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.cloud.compute.v1.AutoscalingPolicyScalingSchedule.Builder) entry;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.AutoscalingPolicy)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.AutoscalingPolicy)
private static final com.google.cloud.compute.v1.AutoscalingPolicy DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.AutoscalingPolicy();
}
public static com.google.cloud.compute.v1.AutoscalingPolicy getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AutoscalingPolicy parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.AutoscalingPolicy getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy