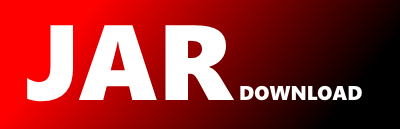
com.google.cloud.compute.v1.BackendBucketCdnPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* Message containing Cloud CDN configuration for a backend bucket.
*
*
* Protobuf type {@code google.cloud.compute.v1.BackendBucketCdnPolicy}
*/
public final class BackendBucketCdnPolicy extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.BackendBucketCdnPolicy)
BackendBucketCdnPolicyOrBuilder {
private static final long serialVersionUID = 0L;
// Use BackendBucketCdnPolicy.newBuilder() to construct.
private BackendBucketCdnPolicy(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BackendBucketCdnPolicy() {
bypassCacheOnRequestHeaders_ = java.util.Collections.emptyList();
cacheMode_ = "";
negativeCachingPolicy_ = java.util.Collections.emptyList();
signedUrlKeyNames_ = com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new BackendBucketCdnPolicy();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_BackendBucketCdnPolicy_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_BackendBucketCdnPolicy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.BackendBucketCdnPolicy.class,
com.google.cloud.compute.v1.BackendBucketCdnPolicy.Builder.class);
}
/**
*
*
*
* Specifies the cache setting for all responses from this backend. The possible values are: USE_ORIGIN_HEADERS Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server. FORCE_CACHE_ALL Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content. CACHE_ALL_STATIC Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached. If no value is provided for cdnPolicy.cacheMode, it defaults to CACHE_ALL_STATIC.
*
*
* Protobuf enum {@code google.cloud.compute.v1.BackendBucketCdnPolicy.CacheMode}
*/
public enum CacheMode implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_CACHE_MODE = 0;
*/
UNDEFINED_CACHE_MODE(0),
/**
*
*
*
* Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached.
*
*
* CACHE_ALL_STATIC = 355027945;
*/
CACHE_ALL_STATIC(355027945),
/**
*
*
*
* Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content.
*
*
* FORCE_CACHE_ALL = 486026928;
*/
FORCE_CACHE_ALL(486026928),
/** INVALID_CACHE_MODE = 381295560;
*/
INVALID_CACHE_MODE(381295560),
/**
*
*
*
* Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server.
*
*
* USE_ORIGIN_HEADERS = 55380261;
*/
USE_ORIGIN_HEADERS(55380261),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_CACHE_MODE = 0;
*/
public static final int UNDEFINED_CACHE_MODE_VALUE = 0;
/**
*
*
*
* Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached.
*
*
* CACHE_ALL_STATIC = 355027945;
*/
public static final int CACHE_ALL_STATIC_VALUE = 355027945;
/**
*
*
*
* Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content.
*
*
* FORCE_CACHE_ALL = 486026928;
*/
public static final int FORCE_CACHE_ALL_VALUE = 486026928;
/** INVALID_CACHE_MODE = 381295560;
*/
public static final int INVALID_CACHE_MODE_VALUE = 381295560;
/**
*
*
*
* Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server.
*
*
* USE_ORIGIN_HEADERS = 55380261;
*/
public static final int USE_ORIGIN_HEADERS_VALUE = 55380261;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CacheMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static CacheMode forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_CACHE_MODE;
case 355027945:
return CACHE_ALL_STATIC;
case 486026928:
return FORCE_CACHE_ALL;
case 381295560:
return INVALID_CACHE_MODE;
case 55380261:
return USE_ORIGIN_HEADERS;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public CacheMode findValueByNumber(int number) {
return CacheMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.BackendBucketCdnPolicy.getDescriptor()
.getEnumTypes()
.get(0);
}
private static final CacheMode[] VALUES = values();
public static CacheMode valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private CacheMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.BackendBucketCdnPolicy.CacheMode)
}
private int bitField0_;
public static final int BYPASS_CACHE_ON_REQUEST_HEADERS_FIELD_NUMBER = 486203082;
@SuppressWarnings("serial")
private java.util.List<
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader>
bypassCacheOnRequestHeaders_;
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
@java.lang.Override
public java.util.List<
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader>
getBypassCacheOnRequestHeadersList() {
return bypassCacheOnRequestHeaders_;
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
@java.lang.Override
public java.util.List<
? extends
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeaderOrBuilder>
getBypassCacheOnRequestHeadersOrBuilderList() {
return bypassCacheOnRequestHeaders_;
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
@java.lang.Override
public int getBypassCacheOnRequestHeadersCount() {
return bypassCacheOnRequestHeaders_.size();
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader
getBypassCacheOnRequestHeaders(int index) {
return bypassCacheOnRequestHeaders_.get(index);
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeaderOrBuilder
getBypassCacheOnRequestHeadersOrBuilder(int index) {
return bypassCacheOnRequestHeaders_.get(index);
}
public static final int CACHE_KEY_POLICY_FIELD_NUMBER = 159263727;
private com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cacheKeyPolicy_;
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*
* @return Whether the cacheKeyPolicy field is set.
*/
@java.lang.Override
public boolean hasCacheKeyPolicy() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*
* @return The cacheKeyPolicy.
*/
@java.lang.Override
public com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy getCacheKeyPolicy() {
return cacheKeyPolicy_ == null
? com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy.getDefaultInstance()
: cacheKeyPolicy_;
}
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicyOrBuilder
getCacheKeyPolicyOrBuilder() {
return cacheKeyPolicy_ == null
? com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy.getDefaultInstance()
: cacheKeyPolicy_;
}
public static final int CACHE_MODE_FIELD_NUMBER = 28877888;
@SuppressWarnings("serial")
private volatile java.lang.Object cacheMode_ = "";
/**
*
*
*
* Specifies the cache setting for all responses from this backend. The possible values are: USE_ORIGIN_HEADERS Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server. FORCE_CACHE_ALL Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content. CACHE_ALL_STATIC Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached. If no value is provided for cdnPolicy.cacheMode, it defaults to CACHE_ALL_STATIC.
* Check the CacheMode enum for the list of possible values.
*
*
* optional string cache_mode = 28877888;
*
* @return Whether the cacheMode field is set.
*/
@java.lang.Override
public boolean hasCacheMode() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Specifies the cache setting for all responses from this backend. The possible values are: USE_ORIGIN_HEADERS Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server. FORCE_CACHE_ALL Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content. CACHE_ALL_STATIC Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached. If no value is provided for cdnPolicy.cacheMode, it defaults to CACHE_ALL_STATIC.
* Check the CacheMode enum for the list of possible values.
*
*
* optional string cache_mode = 28877888;
*
* @return The cacheMode.
*/
@java.lang.Override
public java.lang.String getCacheMode() {
java.lang.Object ref = cacheMode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cacheMode_ = s;
return s;
}
}
/**
*
*
*
* Specifies the cache setting for all responses from this backend. The possible values are: USE_ORIGIN_HEADERS Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server. FORCE_CACHE_ALL Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content. CACHE_ALL_STATIC Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached. If no value is provided for cdnPolicy.cacheMode, it defaults to CACHE_ALL_STATIC.
* Check the CacheMode enum for the list of possible values.
*
*
* optional string cache_mode = 28877888;
*
* @return The bytes for cacheMode.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCacheModeBytes() {
java.lang.Object ref = cacheMode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
cacheMode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLIENT_TTL_FIELD_NUMBER = 29034360;
private int clientTtl_ = 0;
/**
*
*
*
* Specifies a separate client (e.g. browser client) maximum TTL. This is used to clamp the max-age (or Expires) value sent to the client. With FORCE_CACHE_ALL, the lesser of client_ttl and default_ttl is used for the response max-age directive, along with a "public" directive. For cacheable content in CACHE_ALL_STATIC mode, client_ttl clamps the max-age from the origin (if specified), or else sets the response max-age directive to the lesser of the client_ttl and default_ttl, and also ensures a "public" cache-control directive is present. If a client TTL is not specified, a default value (1 hour) will be used. The maximum allowed value is 31,622,400s (1 year).
*
*
* optional int32 client_ttl = 29034360;
*
* @return Whether the clientTtl field is set.
*/
@java.lang.Override
public boolean hasClientTtl() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Specifies a separate client (e.g. browser client) maximum TTL. This is used to clamp the max-age (or Expires) value sent to the client. With FORCE_CACHE_ALL, the lesser of client_ttl and default_ttl is used for the response max-age directive, along with a "public" directive. For cacheable content in CACHE_ALL_STATIC mode, client_ttl clamps the max-age from the origin (if specified), or else sets the response max-age directive to the lesser of the client_ttl and default_ttl, and also ensures a "public" cache-control directive is present. If a client TTL is not specified, a default value (1 hour) will be used. The maximum allowed value is 31,622,400s (1 year).
*
*
* optional int32 client_ttl = 29034360;
*
* @return The clientTtl.
*/
@java.lang.Override
public int getClientTtl() {
return clientTtl_;
}
public static final int DEFAULT_TTL_FIELD_NUMBER = 100253422;
private int defaultTtl_ = 0;
/**
*
*
*
* Specifies the default TTL for cached content served by this origin for responses that do not have an existing valid TTL (max-age or s-max-age). Setting a TTL of "0" means "always revalidate". The value of defaultTTL cannot be set to a value greater than that of maxTTL, but can be equal. When the cacheMode is set to FORCE_CACHE_ALL, the defaultTTL will overwrite the TTL set in all responses. The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 default_ttl = 100253422;
*
* @return Whether the defaultTtl field is set.
*/
@java.lang.Override
public boolean hasDefaultTtl() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Specifies the default TTL for cached content served by this origin for responses that do not have an existing valid TTL (max-age or s-max-age). Setting a TTL of "0" means "always revalidate". The value of defaultTTL cannot be set to a value greater than that of maxTTL, but can be equal. When the cacheMode is set to FORCE_CACHE_ALL, the defaultTTL will overwrite the TTL set in all responses. The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 default_ttl = 100253422;
*
* @return The defaultTtl.
*/
@java.lang.Override
public int getDefaultTtl() {
return defaultTtl_;
}
public static final int MAX_TTL_FIELD_NUMBER = 307578001;
private int maxTtl_ = 0;
/**
*
*
*
* Specifies the maximum allowed TTL for cached content served by this origin. Cache directives that attempt to set a max-age or s-maxage higher than this, or an Expires header more than maxTTL seconds in the future will be capped at the value of maxTTL, as if it were the value of an s-maxage Cache-Control directive. Headers sent to the client will not be modified. Setting a TTL of "0" means "always revalidate". The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 max_ttl = 307578001;
*
* @return Whether the maxTtl field is set.
*/
@java.lang.Override
public boolean hasMaxTtl() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Specifies the maximum allowed TTL for cached content served by this origin. Cache directives that attempt to set a max-age or s-maxage higher than this, or an Expires header more than maxTTL seconds in the future will be capped at the value of maxTTL, as if it were the value of an s-maxage Cache-Control directive. Headers sent to the client will not be modified. Setting a TTL of "0" means "always revalidate". The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 max_ttl = 307578001;
*
* @return The maxTtl.
*/
@java.lang.Override
public int getMaxTtl() {
return maxTtl_;
}
public static final int NEGATIVE_CACHING_FIELD_NUMBER = 336110005;
private boolean negativeCaching_ = false;
/**
*
*
*
* Negative caching allows per-status code TTLs to be set, in order to apply fine-grained caching for common errors or redirects. This can reduce the load on your origin and improve end-user experience by reducing response latency. When the cache mode is set to CACHE_ALL_STATIC or USE_ORIGIN_HEADERS, negative caching applies to responses with the specified response code that lack any Cache-Control, Expires, or Pragma: no-cache directives. When the cache mode is set to FORCE_CACHE_ALL, negative caching applies to all responses with the specified response code, and override any caching headers. By default, Cloud CDN will apply the following default TTLs to these status codes: HTTP 300 (Multiple Choice), 301, 308 (Permanent Redirects): 10m HTTP 404 (Not Found), 410 (Gone), 451 (Unavailable For Legal Reasons): 120s HTTP 405 (Method Not Found), 421 (Misdirected Request), 501 (Not Implemented): 60s. These defaults can be overridden in negative_caching_policy.
*
*
* optional bool negative_caching = 336110005;
*
* @return Whether the negativeCaching field is set.
*/
@java.lang.Override
public boolean hasNegativeCaching() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Negative caching allows per-status code TTLs to be set, in order to apply fine-grained caching for common errors or redirects. This can reduce the load on your origin and improve end-user experience by reducing response latency. When the cache mode is set to CACHE_ALL_STATIC or USE_ORIGIN_HEADERS, negative caching applies to responses with the specified response code that lack any Cache-Control, Expires, or Pragma: no-cache directives. When the cache mode is set to FORCE_CACHE_ALL, negative caching applies to all responses with the specified response code, and override any caching headers. By default, Cloud CDN will apply the following default TTLs to these status codes: HTTP 300 (Multiple Choice), 301, 308 (Permanent Redirects): 10m HTTP 404 (Not Found), 410 (Gone), 451 (Unavailable For Legal Reasons): 120s HTTP 405 (Method Not Found), 421 (Misdirected Request), 501 (Not Implemented): 60s. These defaults can be overridden in negative_caching_policy.
*
*
* optional bool negative_caching = 336110005;
*
* @return The negativeCaching.
*/
@java.lang.Override
public boolean getNegativeCaching() {
return negativeCaching_;
}
public static final int NEGATIVE_CACHING_POLICY_FIELD_NUMBER = 155359996;
@SuppressWarnings("serial")
private java.util.List
negativeCachingPolicy_;
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
@java.lang.Override
public java.util.List
getNegativeCachingPolicyList() {
return negativeCachingPolicy_;
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
@java.lang.Override
public java.util.List<
? extends
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicyOrBuilder>
getNegativeCachingPolicyOrBuilderList() {
return negativeCachingPolicy_;
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
@java.lang.Override
public int getNegativeCachingPolicyCount() {
return negativeCachingPolicy_.size();
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy
getNegativeCachingPolicy(int index) {
return negativeCachingPolicy_.get(index);
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicyOrBuilder
getNegativeCachingPolicyOrBuilder(int index) {
return negativeCachingPolicy_.get(index);
}
public static final int REQUEST_COALESCING_FIELD_NUMBER = 532808276;
private boolean requestCoalescing_ = false;
/**
*
*
*
* If true then Cloud CDN will combine multiple concurrent cache fill requests into a small number of requests to the origin.
*
*
* optional bool request_coalescing = 532808276;
*
* @return Whether the requestCoalescing field is set.
*/
@java.lang.Override
public boolean hasRequestCoalescing() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* If true then Cloud CDN will combine multiple concurrent cache fill requests into a small number of requests to the origin.
*
*
* optional bool request_coalescing = 532808276;
*
* @return The requestCoalescing.
*/
@java.lang.Override
public boolean getRequestCoalescing() {
return requestCoalescing_;
}
public static final int SERVE_WHILE_STALE_FIELD_NUMBER = 236682203;
private int serveWhileStale_ = 0;
/**
*
*
*
* Serve existing content from the cache (if available) when revalidating content with the origin, or when an error is encountered when refreshing the cache. This setting defines the default "max-stale" duration for any cached responses that do not specify a max-stale directive. Stale responses that exceed the TTL configured here will not be served. The default limit (max-stale) is 86400s (1 day), which will allow stale content to be served up to this limit beyond the max-age (or s-max-age) of a cached response. The maximum allowed value is 604800 (1 week). Set this to zero (0) to disable serve-while-stale.
*
*
* optional int32 serve_while_stale = 236682203;
*
* @return Whether the serveWhileStale field is set.
*/
@java.lang.Override
public boolean hasServeWhileStale() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Serve existing content from the cache (if available) when revalidating content with the origin, or when an error is encountered when refreshing the cache. This setting defines the default "max-stale" duration for any cached responses that do not specify a max-stale directive. Stale responses that exceed the TTL configured here will not be served. The default limit (max-stale) is 86400s (1 day), which will allow stale content to be served up to this limit beyond the max-age (or s-max-age) of a cached response. The maximum allowed value is 604800 (1 week). Set this to zero (0) to disable serve-while-stale.
*
*
* optional int32 serve_while_stale = 236682203;
*
* @return The serveWhileStale.
*/
@java.lang.Override
public int getServeWhileStale() {
return serveWhileStale_;
}
public static final int SIGNED_URL_CACHE_MAX_AGE_SEC_FIELD_NUMBER = 269374534;
private long signedUrlCacheMaxAgeSec_ = 0L;
/**
*
*
*
* Maximum number of seconds the response to a signed URL request will be considered fresh. After this time period, the response will be revalidated before being served. Defaults to 1hr (3600s). When serving responses to signed URL requests, Cloud CDN will internally behave as though all responses from this backend had a "Cache-Control: public, max-age=[TTL]" header, regardless of any existing Cache-Control header. The actual headers served in responses will not be altered.
*
*
* optional int64 signed_url_cache_max_age_sec = 269374534;
*
* @return Whether the signedUrlCacheMaxAgeSec field is set.
*/
@java.lang.Override
public boolean hasSignedUrlCacheMaxAgeSec() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Maximum number of seconds the response to a signed URL request will be considered fresh. After this time period, the response will be revalidated before being served. Defaults to 1hr (3600s). When serving responses to signed URL requests, Cloud CDN will internally behave as though all responses from this backend had a "Cache-Control: public, max-age=[TTL]" header, regardless of any existing Cache-Control header. The actual headers served in responses will not be altered.
*
*
* optional int64 signed_url_cache_max_age_sec = 269374534;
*
* @return The signedUrlCacheMaxAgeSec.
*/
@java.lang.Override
public long getSignedUrlCacheMaxAgeSec() {
return signedUrlCacheMaxAgeSec_;
}
public static final int SIGNED_URL_KEY_NAMES_FIELD_NUMBER = 371848885;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList signedUrlKeyNames_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @return A list containing the signedUrlKeyNames.
*/
public com.google.protobuf.ProtocolStringList getSignedUrlKeyNamesList() {
return signedUrlKeyNames_;
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @return The count of signedUrlKeyNames.
*/
public int getSignedUrlKeyNamesCount() {
return signedUrlKeyNames_.size();
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @param index The index of the element to return.
* @return The signedUrlKeyNames at the given index.
*/
public java.lang.String getSignedUrlKeyNames(int index) {
return signedUrlKeyNames_.get(index);
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @param index The index of the value to return.
* @return The bytes of the signedUrlKeyNames at the given index.
*/
public com.google.protobuf.ByteString getSignedUrlKeyNamesBytes(int index) {
return signedUrlKeyNames_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 28877888, cacheMode_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt32(29034360, clientTtl_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(100253422, defaultTtl_);
}
for (int i = 0; i < negativeCachingPolicy_.size(); i++) {
output.writeMessage(155359996, negativeCachingPolicy_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(159263727, getCacheKeyPolicy());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeInt32(236682203, serveWhileStale_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeInt64(269374534, signedUrlCacheMaxAgeSec_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt32(307578001, maxTtl_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeBool(336110005, negativeCaching_);
}
for (int i = 0; i < signedUrlKeyNames_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 371848885, signedUrlKeyNames_.getRaw(i));
}
for (int i = 0; i < bypassCacheOnRequestHeaders_.size(); i++) {
output.writeMessage(486203082, bypassCacheOnRequestHeaders_.get(i));
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeBool(532808276, requestCoalescing_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(28877888, cacheMode_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(29034360, clientTtl_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(100253422, defaultTtl_);
}
for (int i = 0; i < negativeCachingPolicy_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
155359996, negativeCachingPolicy_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(159263727, getCacheKeyPolicy());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(236682203, serveWhileStale_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeInt64Size(
269374534, signedUrlCacheMaxAgeSec_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(307578001, maxTtl_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(336110005, negativeCaching_);
}
{
int dataSize = 0;
for (int i = 0; i < signedUrlKeyNames_.size(); i++) {
dataSize += computeStringSizeNoTag(signedUrlKeyNames_.getRaw(i));
}
size += dataSize;
size += 5 * getSignedUrlKeyNamesList().size();
}
for (int i = 0; i < bypassCacheOnRequestHeaders_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
486203082, bypassCacheOnRequestHeaders_.get(i));
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(532808276, requestCoalescing_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.BackendBucketCdnPolicy)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.BackendBucketCdnPolicy other =
(com.google.cloud.compute.v1.BackendBucketCdnPolicy) obj;
if (!getBypassCacheOnRequestHeadersList().equals(other.getBypassCacheOnRequestHeadersList()))
return false;
if (hasCacheKeyPolicy() != other.hasCacheKeyPolicy()) return false;
if (hasCacheKeyPolicy()) {
if (!getCacheKeyPolicy().equals(other.getCacheKeyPolicy())) return false;
}
if (hasCacheMode() != other.hasCacheMode()) return false;
if (hasCacheMode()) {
if (!getCacheMode().equals(other.getCacheMode())) return false;
}
if (hasClientTtl() != other.hasClientTtl()) return false;
if (hasClientTtl()) {
if (getClientTtl() != other.getClientTtl()) return false;
}
if (hasDefaultTtl() != other.hasDefaultTtl()) return false;
if (hasDefaultTtl()) {
if (getDefaultTtl() != other.getDefaultTtl()) return false;
}
if (hasMaxTtl() != other.hasMaxTtl()) return false;
if (hasMaxTtl()) {
if (getMaxTtl() != other.getMaxTtl()) return false;
}
if (hasNegativeCaching() != other.hasNegativeCaching()) return false;
if (hasNegativeCaching()) {
if (getNegativeCaching() != other.getNegativeCaching()) return false;
}
if (!getNegativeCachingPolicyList().equals(other.getNegativeCachingPolicyList())) return false;
if (hasRequestCoalescing() != other.hasRequestCoalescing()) return false;
if (hasRequestCoalescing()) {
if (getRequestCoalescing() != other.getRequestCoalescing()) return false;
}
if (hasServeWhileStale() != other.hasServeWhileStale()) return false;
if (hasServeWhileStale()) {
if (getServeWhileStale() != other.getServeWhileStale()) return false;
}
if (hasSignedUrlCacheMaxAgeSec() != other.hasSignedUrlCacheMaxAgeSec()) return false;
if (hasSignedUrlCacheMaxAgeSec()) {
if (getSignedUrlCacheMaxAgeSec() != other.getSignedUrlCacheMaxAgeSec()) return false;
}
if (!getSignedUrlKeyNamesList().equals(other.getSignedUrlKeyNamesList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getBypassCacheOnRequestHeadersCount() > 0) {
hash = (37 * hash) + BYPASS_CACHE_ON_REQUEST_HEADERS_FIELD_NUMBER;
hash = (53 * hash) + getBypassCacheOnRequestHeadersList().hashCode();
}
if (hasCacheKeyPolicy()) {
hash = (37 * hash) + CACHE_KEY_POLICY_FIELD_NUMBER;
hash = (53 * hash) + getCacheKeyPolicy().hashCode();
}
if (hasCacheMode()) {
hash = (37 * hash) + CACHE_MODE_FIELD_NUMBER;
hash = (53 * hash) + getCacheMode().hashCode();
}
if (hasClientTtl()) {
hash = (37 * hash) + CLIENT_TTL_FIELD_NUMBER;
hash = (53 * hash) + getClientTtl();
}
if (hasDefaultTtl()) {
hash = (37 * hash) + DEFAULT_TTL_FIELD_NUMBER;
hash = (53 * hash) + getDefaultTtl();
}
if (hasMaxTtl()) {
hash = (37 * hash) + MAX_TTL_FIELD_NUMBER;
hash = (53 * hash) + getMaxTtl();
}
if (hasNegativeCaching()) {
hash = (37 * hash) + NEGATIVE_CACHING_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getNegativeCaching());
}
if (getNegativeCachingPolicyCount() > 0) {
hash = (37 * hash) + NEGATIVE_CACHING_POLICY_FIELD_NUMBER;
hash = (53 * hash) + getNegativeCachingPolicyList().hashCode();
}
if (hasRequestCoalescing()) {
hash = (37 * hash) + REQUEST_COALESCING_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getRequestCoalescing());
}
if (hasServeWhileStale()) {
hash = (37 * hash) + SERVE_WHILE_STALE_FIELD_NUMBER;
hash = (53 * hash) + getServeWhileStale();
}
if (hasSignedUrlCacheMaxAgeSec()) {
hash = (37 * hash) + SIGNED_URL_CACHE_MAX_AGE_SEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getSignedUrlCacheMaxAgeSec());
}
if (getSignedUrlKeyNamesCount() > 0) {
hash = (37 * hash) + SIGNED_URL_KEY_NAMES_FIELD_NUMBER;
hash = (53 * hash) + getSignedUrlKeyNamesList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.BackendBucketCdnPolicy prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Message containing Cloud CDN configuration for a backend bucket.
*
*
* Protobuf type {@code google.cloud.compute.v1.BackendBucketCdnPolicy}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.BackendBucketCdnPolicy)
com.google.cloud.compute.v1.BackendBucketCdnPolicyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_BackendBucketCdnPolicy_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_BackendBucketCdnPolicy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.BackendBucketCdnPolicy.class,
com.google.cloud.compute.v1.BackendBucketCdnPolicy.Builder.class);
}
// Construct using com.google.cloud.compute.v1.BackendBucketCdnPolicy.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getBypassCacheOnRequestHeadersFieldBuilder();
getCacheKeyPolicyFieldBuilder();
getNegativeCachingPolicyFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (bypassCacheOnRequestHeadersBuilder_ == null) {
bypassCacheOnRequestHeaders_ = java.util.Collections.emptyList();
} else {
bypassCacheOnRequestHeaders_ = null;
bypassCacheOnRequestHeadersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
cacheKeyPolicy_ = null;
if (cacheKeyPolicyBuilder_ != null) {
cacheKeyPolicyBuilder_.dispose();
cacheKeyPolicyBuilder_ = null;
}
cacheMode_ = "";
clientTtl_ = 0;
defaultTtl_ = 0;
maxTtl_ = 0;
negativeCaching_ = false;
if (negativeCachingPolicyBuilder_ == null) {
negativeCachingPolicy_ = java.util.Collections.emptyList();
} else {
negativeCachingPolicy_ = null;
negativeCachingPolicyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
requestCoalescing_ = false;
serveWhileStale_ = 0;
signedUrlCacheMaxAgeSec_ = 0L;
signedUrlKeyNames_ = com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_BackendBucketCdnPolicy_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.BackendBucketCdnPolicy getDefaultInstanceForType() {
return com.google.cloud.compute.v1.BackendBucketCdnPolicy.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.BackendBucketCdnPolicy build() {
com.google.cloud.compute.v1.BackendBucketCdnPolicy result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.BackendBucketCdnPolicy buildPartial() {
com.google.cloud.compute.v1.BackendBucketCdnPolicy result =
new com.google.cloud.compute.v1.BackendBucketCdnPolicy(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(
com.google.cloud.compute.v1.BackendBucketCdnPolicy result) {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
bypassCacheOnRequestHeaders_ =
java.util.Collections.unmodifiableList(bypassCacheOnRequestHeaders_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.bypassCacheOnRequestHeaders_ = bypassCacheOnRequestHeaders_;
} else {
result.bypassCacheOnRequestHeaders_ = bypassCacheOnRequestHeadersBuilder_.build();
}
if (negativeCachingPolicyBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
negativeCachingPolicy_ = java.util.Collections.unmodifiableList(negativeCachingPolicy_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.negativeCachingPolicy_ = negativeCachingPolicy_;
} else {
result.negativeCachingPolicy_ = negativeCachingPolicyBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.compute.v1.BackendBucketCdnPolicy result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.cacheKeyPolicy_ =
cacheKeyPolicyBuilder_ == null ? cacheKeyPolicy_ : cacheKeyPolicyBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.cacheMode_ = cacheMode_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.clientTtl_ = clientTtl_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.defaultTtl_ = defaultTtl_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.maxTtl_ = maxTtl_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.negativeCaching_ = negativeCaching_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.requestCoalescing_ = requestCoalescing_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.serveWhileStale_ = serveWhileStale_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.signedUrlCacheMaxAgeSec_ = signedUrlCacheMaxAgeSec_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
signedUrlKeyNames_.makeImmutable();
result.signedUrlKeyNames_ = signedUrlKeyNames_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.BackendBucketCdnPolicy) {
return mergeFrom((com.google.cloud.compute.v1.BackendBucketCdnPolicy) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.BackendBucketCdnPolicy other) {
if (other == com.google.cloud.compute.v1.BackendBucketCdnPolicy.getDefaultInstance())
return this;
if (bypassCacheOnRequestHeadersBuilder_ == null) {
if (!other.bypassCacheOnRequestHeaders_.isEmpty()) {
if (bypassCacheOnRequestHeaders_.isEmpty()) {
bypassCacheOnRequestHeaders_ = other.bypassCacheOnRequestHeaders_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureBypassCacheOnRequestHeadersIsMutable();
bypassCacheOnRequestHeaders_.addAll(other.bypassCacheOnRequestHeaders_);
}
onChanged();
}
} else {
if (!other.bypassCacheOnRequestHeaders_.isEmpty()) {
if (bypassCacheOnRequestHeadersBuilder_.isEmpty()) {
bypassCacheOnRequestHeadersBuilder_.dispose();
bypassCacheOnRequestHeadersBuilder_ = null;
bypassCacheOnRequestHeaders_ = other.bypassCacheOnRequestHeaders_;
bitField0_ = (bitField0_ & ~0x00000001);
bypassCacheOnRequestHeadersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getBypassCacheOnRequestHeadersFieldBuilder()
: null;
} else {
bypassCacheOnRequestHeadersBuilder_.addAllMessages(other.bypassCacheOnRequestHeaders_);
}
}
}
if (other.hasCacheKeyPolicy()) {
mergeCacheKeyPolicy(other.getCacheKeyPolicy());
}
if (other.hasCacheMode()) {
cacheMode_ = other.cacheMode_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasClientTtl()) {
setClientTtl(other.getClientTtl());
}
if (other.hasDefaultTtl()) {
setDefaultTtl(other.getDefaultTtl());
}
if (other.hasMaxTtl()) {
setMaxTtl(other.getMaxTtl());
}
if (other.hasNegativeCaching()) {
setNegativeCaching(other.getNegativeCaching());
}
if (negativeCachingPolicyBuilder_ == null) {
if (!other.negativeCachingPolicy_.isEmpty()) {
if (negativeCachingPolicy_.isEmpty()) {
negativeCachingPolicy_ = other.negativeCachingPolicy_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureNegativeCachingPolicyIsMutable();
negativeCachingPolicy_.addAll(other.negativeCachingPolicy_);
}
onChanged();
}
} else {
if (!other.negativeCachingPolicy_.isEmpty()) {
if (negativeCachingPolicyBuilder_.isEmpty()) {
negativeCachingPolicyBuilder_.dispose();
negativeCachingPolicyBuilder_ = null;
negativeCachingPolicy_ = other.negativeCachingPolicy_;
bitField0_ = (bitField0_ & ~0x00000080);
negativeCachingPolicyBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getNegativeCachingPolicyFieldBuilder()
: null;
} else {
negativeCachingPolicyBuilder_.addAllMessages(other.negativeCachingPolicy_);
}
}
}
if (other.hasRequestCoalescing()) {
setRequestCoalescing(other.getRequestCoalescing());
}
if (other.hasServeWhileStale()) {
setServeWhileStale(other.getServeWhileStale());
}
if (other.hasSignedUrlCacheMaxAgeSec()) {
setSignedUrlCacheMaxAgeSec(other.getSignedUrlCacheMaxAgeSec());
}
if (!other.signedUrlKeyNames_.isEmpty()) {
if (signedUrlKeyNames_.isEmpty()) {
signedUrlKeyNames_ = other.signedUrlKeyNames_;
bitField0_ |= 0x00000800;
} else {
ensureSignedUrlKeyNamesIsMutable();
signedUrlKeyNames_.addAll(other.signedUrlKeyNames_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 231023106:
{
cacheMode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 231023106
case 232274880:
{
clientTtl_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 232274880
case 802027376:
{
defaultTtl_ = input.readInt32();
bitField0_ |= 0x00000010;
break;
} // case 802027376
case 1242879970:
{
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy m =
input.readMessage(
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy
.parser(),
extensionRegistry);
if (negativeCachingPolicyBuilder_ == null) {
ensureNegativeCachingPolicyIsMutable();
negativeCachingPolicy_.add(m);
} else {
negativeCachingPolicyBuilder_.addMessage(m);
}
break;
} // case 1242879970
case 1274109818:
{
input.readMessage(getCacheKeyPolicyFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 1274109818
case 1893457624:
{
serveWhileStale_ = input.readInt32();
bitField0_ |= 0x00000200;
break;
} // case 1893457624
case -2139971024:
{
signedUrlCacheMaxAgeSec_ = input.readInt64();
bitField0_ |= 0x00000400;
break;
} // case -2139971024
case -1834343288:
{
maxTtl_ = input.readInt32();
bitField0_ |= 0x00000020;
break;
} // case -1834343288
case -1606087256:
{
negativeCaching_ = input.readBool();
bitField0_ |= 0x00000040;
break;
} // case -1606087256
case -1320176214:
{
java.lang.String s = input.readStringRequireUtf8();
ensureSignedUrlKeyNamesIsMutable();
signedUrlKeyNames_.add(s);
break;
} // case -1320176214
case -405342638:
{
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader m =
input.readMessage(
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader
.parser(),
extensionRegistry);
if (bypassCacheOnRequestHeadersBuilder_ == null) {
ensureBypassCacheOnRequestHeadersIsMutable();
bypassCacheOnRequestHeaders_.add(m);
} else {
bypassCacheOnRequestHeadersBuilder_.addMessage(m);
}
break;
} // case -405342638
case -32501088:
{
requestCoalescing_ = input.readBool();
bitField0_ |= 0x00000100;
break;
} // case -32501088
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List<
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader>
bypassCacheOnRequestHeaders_ = java.util.Collections.emptyList();
private void ensureBypassCacheOnRequestHeadersIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
bypassCacheOnRequestHeaders_ =
new java.util.ArrayList<
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader>(
bypassCacheOnRequestHeaders_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader,
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader.Builder,
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeaderOrBuilder>
bypassCacheOnRequestHeadersBuilder_;
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public java.util.List<
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader>
getBypassCacheOnRequestHeadersList() {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
return java.util.Collections.unmodifiableList(bypassCacheOnRequestHeaders_);
} else {
return bypassCacheOnRequestHeadersBuilder_.getMessageList();
}
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public int getBypassCacheOnRequestHeadersCount() {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
return bypassCacheOnRequestHeaders_.size();
} else {
return bypassCacheOnRequestHeadersBuilder_.getCount();
}
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader
getBypassCacheOnRequestHeaders(int index) {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
return bypassCacheOnRequestHeaders_.get(index);
} else {
return bypassCacheOnRequestHeadersBuilder_.getMessage(index);
}
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public Builder setBypassCacheOnRequestHeaders(
int index,
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader value) {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBypassCacheOnRequestHeadersIsMutable();
bypassCacheOnRequestHeaders_.set(index, value);
onChanged();
} else {
bypassCacheOnRequestHeadersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public Builder setBypassCacheOnRequestHeaders(
int index,
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader.Builder
builderForValue) {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
ensureBypassCacheOnRequestHeadersIsMutable();
bypassCacheOnRequestHeaders_.set(index, builderForValue.build());
onChanged();
} else {
bypassCacheOnRequestHeadersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public Builder addBypassCacheOnRequestHeaders(
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader value) {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBypassCacheOnRequestHeadersIsMutable();
bypassCacheOnRequestHeaders_.add(value);
onChanged();
} else {
bypassCacheOnRequestHeadersBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public Builder addBypassCacheOnRequestHeaders(
int index,
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader value) {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBypassCacheOnRequestHeadersIsMutable();
bypassCacheOnRequestHeaders_.add(index, value);
onChanged();
} else {
bypassCacheOnRequestHeadersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public Builder addBypassCacheOnRequestHeaders(
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader.Builder
builderForValue) {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
ensureBypassCacheOnRequestHeadersIsMutable();
bypassCacheOnRequestHeaders_.add(builderForValue.build());
onChanged();
} else {
bypassCacheOnRequestHeadersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public Builder addBypassCacheOnRequestHeaders(
int index,
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader.Builder
builderForValue) {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
ensureBypassCacheOnRequestHeadersIsMutable();
bypassCacheOnRequestHeaders_.add(index, builderForValue.build());
onChanged();
} else {
bypassCacheOnRequestHeadersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public Builder addAllBypassCacheOnRequestHeaders(
java.lang.Iterable<
? extends
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader>
values) {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
ensureBypassCacheOnRequestHeadersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, bypassCacheOnRequestHeaders_);
onChanged();
} else {
bypassCacheOnRequestHeadersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public Builder clearBypassCacheOnRequestHeaders() {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
bypassCacheOnRequestHeaders_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
bypassCacheOnRequestHeadersBuilder_.clear();
}
return this;
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public Builder removeBypassCacheOnRequestHeaders(int index) {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
ensureBypassCacheOnRequestHeadersIsMutable();
bypassCacheOnRequestHeaders_.remove(index);
onChanged();
} else {
bypassCacheOnRequestHeadersBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader.Builder
getBypassCacheOnRequestHeadersBuilder(int index) {
return getBypassCacheOnRequestHeadersFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeaderOrBuilder
getBypassCacheOnRequestHeadersOrBuilder(int index) {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
return bypassCacheOnRequestHeaders_.get(index);
} else {
return bypassCacheOnRequestHeadersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public java.util.List<
? extends
com.google.cloud.compute.v1
.BackendBucketCdnPolicyBypassCacheOnRequestHeaderOrBuilder>
getBypassCacheOnRequestHeadersOrBuilderList() {
if (bypassCacheOnRequestHeadersBuilder_ != null) {
return bypassCacheOnRequestHeadersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(bypassCacheOnRequestHeaders_);
}
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader.Builder
addBypassCacheOnRequestHeadersBuilder() {
return getBypassCacheOnRequestHeadersFieldBuilder()
.addBuilder(
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader
.getDefaultInstance());
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader.Builder
addBypassCacheOnRequestHeadersBuilder(int index) {
return getBypassCacheOnRequestHeadersFieldBuilder()
.addBuilder(
index,
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader
.getDefaultInstance());
}
/**
*
*
*
* Bypass the cache when the specified request headers are matched - e.g. Pragma or Authorization headers. Up to 5 headers can be specified. The cache is bypassed for all cdnPolicy.cacheMode settings.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader bypass_cache_on_request_headers = 486203082;
*
*/
public java.util.List<
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader.Builder>
getBypassCacheOnRequestHeadersBuilderList() {
return getBypassCacheOnRequestHeadersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader,
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader.Builder,
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeaderOrBuilder>
getBypassCacheOnRequestHeadersFieldBuilder() {
if (bypassCacheOnRequestHeadersBuilder_ == null) {
bypassCacheOnRequestHeadersBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader,
com.google.cloud.compute.v1.BackendBucketCdnPolicyBypassCacheOnRequestHeader
.Builder,
com.google.cloud.compute.v1
.BackendBucketCdnPolicyBypassCacheOnRequestHeaderOrBuilder>(
bypassCacheOnRequestHeaders_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
bypassCacheOnRequestHeaders_ = null;
}
return bypassCacheOnRequestHeadersBuilder_;
}
private com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cacheKeyPolicy_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy,
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy.Builder,
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicyOrBuilder>
cacheKeyPolicyBuilder_;
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*
* @return Whether the cacheKeyPolicy field is set.
*/
public boolean hasCacheKeyPolicy() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*
* @return The cacheKeyPolicy.
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy getCacheKeyPolicy() {
if (cacheKeyPolicyBuilder_ == null) {
return cacheKeyPolicy_ == null
? com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy.getDefaultInstance()
: cacheKeyPolicy_;
} else {
return cacheKeyPolicyBuilder_.getMessage();
}
}
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*/
public Builder setCacheKeyPolicy(
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy value) {
if (cacheKeyPolicyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cacheKeyPolicy_ = value;
} else {
cacheKeyPolicyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*/
public Builder setCacheKeyPolicy(
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy.Builder builderForValue) {
if (cacheKeyPolicyBuilder_ == null) {
cacheKeyPolicy_ = builderForValue.build();
} else {
cacheKeyPolicyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*/
public Builder mergeCacheKeyPolicy(
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy value) {
if (cacheKeyPolicyBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)
&& cacheKeyPolicy_ != null
&& cacheKeyPolicy_
!= com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy
.getDefaultInstance()) {
getCacheKeyPolicyBuilder().mergeFrom(value);
} else {
cacheKeyPolicy_ = value;
}
} else {
cacheKeyPolicyBuilder_.mergeFrom(value);
}
if (cacheKeyPolicy_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*/
public Builder clearCacheKeyPolicy() {
bitField0_ = (bitField0_ & ~0x00000002);
cacheKeyPolicy_ = null;
if (cacheKeyPolicyBuilder_ != null) {
cacheKeyPolicyBuilder_.dispose();
cacheKeyPolicyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy.Builder
getCacheKeyPolicyBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getCacheKeyPolicyFieldBuilder().getBuilder();
}
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicyOrBuilder
getCacheKeyPolicyOrBuilder() {
if (cacheKeyPolicyBuilder_ != null) {
return cacheKeyPolicyBuilder_.getMessageOrBuilder();
} else {
return cacheKeyPolicy_ == null
? com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy.getDefaultInstance()
: cacheKeyPolicy_;
}
}
/**
*
*
*
* The CacheKeyPolicy for this CdnPolicy.
*
*
*
* optional .google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy cache_key_policy = 159263727;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy,
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy.Builder,
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicyOrBuilder>
getCacheKeyPolicyFieldBuilder() {
if (cacheKeyPolicyBuilder_ == null) {
cacheKeyPolicyBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy,
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicy.Builder,
com.google.cloud.compute.v1.BackendBucketCdnPolicyCacheKeyPolicyOrBuilder>(
getCacheKeyPolicy(), getParentForChildren(), isClean());
cacheKeyPolicy_ = null;
}
return cacheKeyPolicyBuilder_;
}
private java.lang.Object cacheMode_ = "";
/**
*
*
*
* Specifies the cache setting for all responses from this backend. The possible values are: USE_ORIGIN_HEADERS Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server. FORCE_CACHE_ALL Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content. CACHE_ALL_STATIC Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached. If no value is provided for cdnPolicy.cacheMode, it defaults to CACHE_ALL_STATIC.
* Check the CacheMode enum for the list of possible values.
*
*
* optional string cache_mode = 28877888;
*
* @return Whether the cacheMode field is set.
*/
public boolean hasCacheMode() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Specifies the cache setting for all responses from this backend. The possible values are: USE_ORIGIN_HEADERS Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server. FORCE_CACHE_ALL Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content. CACHE_ALL_STATIC Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached. If no value is provided for cdnPolicy.cacheMode, it defaults to CACHE_ALL_STATIC.
* Check the CacheMode enum for the list of possible values.
*
*
* optional string cache_mode = 28877888;
*
* @return The cacheMode.
*/
public java.lang.String getCacheMode() {
java.lang.Object ref = cacheMode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cacheMode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Specifies the cache setting for all responses from this backend. The possible values are: USE_ORIGIN_HEADERS Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server. FORCE_CACHE_ALL Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content. CACHE_ALL_STATIC Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached. If no value is provided for cdnPolicy.cacheMode, it defaults to CACHE_ALL_STATIC.
* Check the CacheMode enum for the list of possible values.
*
*
* optional string cache_mode = 28877888;
*
* @return The bytes for cacheMode.
*/
public com.google.protobuf.ByteString getCacheModeBytes() {
java.lang.Object ref = cacheMode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
cacheMode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Specifies the cache setting for all responses from this backend. The possible values are: USE_ORIGIN_HEADERS Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server. FORCE_CACHE_ALL Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content. CACHE_ALL_STATIC Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached. If no value is provided for cdnPolicy.cacheMode, it defaults to CACHE_ALL_STATIC.
* Check the CacheMode enum for the list of possible values.
*
*
* optional string cache_mode = 28877888;
*
* @param value The cacheMode to set.
* @return This builder for chaining.
*/
public Builder setCacheMode(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
cacheMode_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Specifies the cache setting for all responses from this backend. The possible values are: USE_ORIGIN_HEADERS Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server. FORCE_CACHE_ALL Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content. CACHE_ALL_STATIC Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached. If no value is provided for cdnPolicy.cacheMode, it defaults to CACHE_ALL_STATIC.
* Check the CacheMode enum for the list of possible values.
*
*
* optional string cache_mode = 28877888;
*
* @return This builder for chaining.
*/
public Builder clearCacheMode() {
cacheMode_ = getDefaultInstance().getCacheMode();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* Specifies the cache setting for all responses from this backend. The possible values are: USE_ORIGIN_HEADERS Requires the origin to set valid caching headers to cache content. Responses without these headers will not be cached at Google's edge, and will require a full trip to the origin on every request, potentially impacting performance and increasing load on the origin server. FORCE_CACHE_ALL Cache all content, ignoring any "private", "no-store" or "no-cache" directives in Cache-Control response headers. Warning: this may result in Cloud CDN caching private, per-user (user identifiable) content. CACHE_ALL_STATIC Automatically cache static content, including common image formats, media (video and audio), and web assets (JavaScript and CSS). Requests and responses that are marked as uncacheable, as well as dynamic content (including HTML), will not be cached. If no value is provided for cdnPolicy.cacheMode, it defaults to CACHE_ALL_STATIC.
* Check the CacheMode enum for the list of possible values.
*
*
* optional string cache_mode = 28877888;
*
* @param value The bytes for cacheMode to set.
* @return This builder for chaining.
*/
public Builder setCacheModeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
cacheMode_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private int clientTtl_;
/**
*
*
*
* Specifies a separate client (e.g. browser client) maximum TTL. This is used to clamp the max-age (or Expires) value sent to the client. With FORCE_CACHE_ALL, the lesser of client_ttl and default_ttl is used for the response max-age directive, along with a "public" directive. For cacheable content in CACHE_ALL_STATIC mode, client_ttl clamps the max-age from the origin (if specified), or else sets the response max-age directive to the lesser of the client_ttl and default_ttl, and also ensures a "public" cache-control directive is present. If a client TTL is not specified, a default value (1 hour) will be used. The maximum allowed value is 31,622,400s (1 year).
*
*
* optional int32 client_ttl = 29034360;
*
* @return Whether the clientTtl field is set.
*/
@java.lang.Override
public boolean hasClientTtl() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Specifies a separate client (e.g. browser client) maximum TTL. This is used to clamp the max-age (or Expires) value sent to the client. With FORCE_CACHE_ALL, the lesser of client_ttl and default_ttl is used for the response max-age directive, along with a "public" directive. For cacheable content in CACHE_ALL_STATIC mode, client_ttl clamps the max-age from the origin (if specified), or else sets the response max-age directive to the lesser of the client_ttl and default_ttl, and also ensures a "public" cache-control directive is present. If a client TTL is not specified, a default value (1 hour) will be used. The maximum allowed value is 31,622,400s (1 year).
*
*
* optional int32 client_ttl = 29034360;
*
* @return The clientTtl.
*/
@java.lang.Override
public int getClientTtl() {
return clientTtl_;
}
/**
*
*
*
* Specifies a separate client (e.g. browser client) maximum TTL. This is used to clamp the max-age (or Expires) value sent to the client. With FORCE_CACHE_ALL, the lesser of client_ttl and default_ttl is used for the response max-age directive, along with a "public" directive. For cacheable content in CACHE_ALL_STATIC mode, client_ttl clamps the max-age from the origin (if specified), or else sets the response max-age directive to the lesser of the client_ttl and default_ttl, and also ensures a "public" cache-control directive is present. If a client TTL is not specified, a default value (1 hour) will be used. The maximum allowed value is 31,622,400s (1 year).
*
*
* optional int32 client_ttl = 29034360;
*
* @param value The clientTtl to set.
* @return This builder for chaining.
*/
public Builder setClientTtl(int value) {
clientTtl_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Specifies a separate client (e.g. browser client) maximum TTL. This is used to clamp the max-age (or Expires) value sent to the client. With FORCE_CACHE_ALL, the lesser of client_ttl and default_ttl is used for the response max-age directive, along with a "public" directive. For cacheable content in CACHE_ALL_STATIC mode, client_ttl clamps the max-age from the origin (if specified), or else sets the response max-age directive to the lesser of the client_ttl and default_ttl, and also ensures a "public" cache-control directive is present. If a client TTL is not specified, a default value (1 hour) will be used. The maximum allowed value is 31,622,400s (1 year).
*
*
* optional int32 client_ttl = 29034360;
*
* @return This builder for chaining.
*/
public Builder clearClientTtl() {
bitField0_ = (bitField0_ & ~0x00000008);
clientTtl_ = 0;
onChanged();
return this;
}
private int defaultTtl_;
/**
*
*
*
* Specifies the default TTL for cached content served by this origin for responses that do not have an existing valid TTL (max-age or s-max-age). Setting a TTL of "0" means "always revalidate". The value of defaultTTL cannot be set to a value greater than that of maxTTL, but can be equal. When the cacheMode is set to FORCE_CACHE_ALL, the defaultTTL will overwrite the TTL set in all responses. The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 default_ttl = 100253422;
*
* @return Whether the defaultTtl field is set.
*/
@java.lang.Override
public boolean hasDefaultTtl() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Specifies the default TTL for cached content served by this origin for responses that do not have an existing valid TTL (max-age or s-max-age). Setting a TTL of "0" means "always revalidate". The value of defaultTTL cannot be set to a value greater than that of maxTTL, but can be equal. When the cacheMode is set to FORCE_CACHE_ALL, the defaultTTL will overwrite the TTL set in all responses. The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 default_ttl = 100253422;
*
* @return The defaultTtl.
*/
@java.lang.Override
public int getDefaultTtl() {
return defaultTtl_;
}
/**
*
*
*
* Specifies the default TTL for cached content served by this origin for responses that do not have an existing valid TTL (max-age or s-max-age). Setting a TTL of "0" means "always revalidate". The value of defaultTTL cannot be set to a value greater than that of maxTTL, but can be equal. When the cacheMode is set to FORCE_CACHE_ALL, the defaultTTL will overwrite the TTL set in all responses. The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 default_ttl = 100253422;
*
* @param value The defaultTtl to set.
* @return This builder for chaining.
*/
public Builder setDefaultTtl(int value) {
defaultTtl_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Specifies the default TTL for cached content served by this origin for responses that do not have an existing valid TTL (max-age or s-max-age). Setting a TTL of "0" means "always revalidate". The value of defaultTTL cannot be set to a value greater than that of maxTTL, but can be equal. When the cacheMode is set to FORCE_CACHE_ALL, the defaultTTL will overwrite the TTL set in all responses. The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 default_ttl = 100253422;
*
* @return This builder for chaining.
*/
public Builder clearDefaultTtl() {
bitField0_ = (bitField0_ & ~0x00000010);
defaultTtl_ = 0;
onChanged();
return this;
}
private int maxTtl_;
/**
*
*
*
* Specifies the maximum allowed TTL for cached content served by this origin. Cache directives that attempt to set a max-age or s-maxage higher than this, or an Expires header more than maxTTL seconds in the future will be capped at the value of maxTTL, as if it were the value of an s-maxage Cache-Control directive. Headers sent to the client will not be modified. Setting a TTL of "0" means "always revalidate". The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 max_ttl = 307578001;
*
* @return Whether the maxTtl field is set.
*/
@java.lang.Override
public boolean hasMaxTtl() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Specifies the maximum allowed TTL for cached content served by this origin. Cache directives that attempt to set a max-age or s-maxage higher than this, or an Expires header more than maxTTL seconds in the future will be capped at the value of maxTTL, as if it were the value of an s-maxage Cache-Control directive. Headers sent to the client will not be modified. Setting a TTL of "0" means "always revalidate". The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 max_ttl = 307578001;
*
* @return The maxTtl.
*/
@java.lang.Override
public int getMaxTtl() {
return maxTtl_;
}
/**
*
*
*
* Specifies the maximum allowed TTL for cached content served by this origin. Cache directives that attempt to set a max-age or s-maxage higher than this, or an Expires header more than maxTTL seconds in the future will be capped at the value of maxTTL, as if it were the value of an s-maxage Cache-Control directive. Headers sent to the client will not be modified. Setting a TTL of "0" means "always revalidate". The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 max_ttl = 307578001;
*
* @param value The maxTtl to set.
* @return This builder for chaining.
*/
public Builder setMaxTtl(int value) {
maxTtl_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Specifies the maximum allowed TTL for cached content served by this origin. Cache directives that attempt to set a max-age or s-maxage higher than this, or an Expires header more than maxTTL seconds in the future will be capped at the value of maxTTL, as if it were the value of an s-maxage Cache-Control directive. Headers sent to the client will not be modified. Setting a TTL of "0" means "always revalidate". The maximum allowed value is 31,622,400s (1 year), noting that infrequently accessed objects may be evicted from the cache before the defined TTL.
*
*
* optional int32 max_ttl = 307578001;
*
* @return This builder for chaining.
*/
public Builder clearMaxTtl() {
bitField0_ = (bitField0_ & ~0x00000020);
maxTtl_ = 0;
onChanged();
return this;
}
private boolean negativeCaching_;
/**
*
*
*
* Negative caching allows per-status code TTLs to be set, in order to apply fine-grained caching for common errors or redirects. This can reduce the load on your origin and improve end-user experience by reducing response latency. When the cache mode is set to CACHE_ALL_STATIC or USE_ORIGIN_HEADERS, negative caching applies to responses with the specified response code that lack any Cache-Control, Expires, or Pragma: no-cache directives. When the cache mode is set to FORCE_CACHE_ALL, negative caching applies to all responses with the specified response code, and override any caching headers. By default, Cloud CDN will apply the following default TTLs to these status codes: HTTP 300 (Multiple Choice), 301, 308 (Permanent Redirects): 10m HTTP 404 (Not Found), 410 (Gone), 451 (Unavailable For Legal Reasons): 120s HTTP 405 (Method Not Found), 421 (Misdirected Request), 501 (Not Implemented): 60s. These defaults can be overridden in negative_caching_policy.
*
*
* optional bool negative_caching = 336110005;
*
* @return Whether the negativeCaching field is set.
*/
@java.lang.Override
public boolean hasNegativeCaching() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Negative caching allows per-status code TTLs to be set, in order to apply fine-grained caching for common errors or redirects. This can reduce the load on your origin and improve end-user experience by reducing response latency. When the cache mode is set to CACHE_ALL_STATIC or USE_ORIGIN_HEADERS, negative caching applies to responses with the specified response code that lack any Cache-Control, Expires, or Pragma: no-cache directives. When the cache mode is set to FORCE_CACHE_ALL, negative caching applies to all responses with the specified response code, and override any caching headers. By default, Cloud CDN will apply the following default TTLs to these status codes: HTTP 300 (Multiple Choice), 301, 308 (Permanent Redirects): 10m HTTP 404 (Not Found), 410 (Gone), 451 (Unavailable For Legal Reasons): 120s HTTP 405 (Method Not Found), 421 (Misdirected Request), 501 (Not Implemented): 60s. These defaults can be overridden in negative_caching_policy.
*
*
* optional bool negative_caching = 336110005;
*
* @return The negativeCaching.
*/
@java.lang.Override
public boolean getNegativeCaching() {
return negativeCaching_;
}
/**
*
*
*
* Negative caching allows per-status code TTLs to be set, in order to apply fine-grained caching for common errors or redirects. This can reduce the load on your origin and improve end-user experience by reducing response latency. When the cache mode is set to CACHE_ALL_STATIC or USE_ORIGIN_HEADERS, negative caching applies to responses with the specified response code that lack any Cache-Control, Expires, or Pragma: no-cache directives. When the cache mode is set to FORCE_CACHE_ALL, negative caching applies to all responses with the specified response code, and override any caching headers. By default, Cloud CDN will apply the following default TTLs to these status codes: HTTP 300 (Multiple Choice), 301, 308 (Permanent Redirects): 10m HTTP 404 (Not Found), 410 (Gone), 451 (Unavailable For Legal Reasons): 120s HTTP 405 (Method Not Found), 421 (Misdirected Request), 501 (Not Implemented): 60s. These defaults can be overridden in negative_caching_policy.
*
*
* optional bool negative_caching = 336110005;
*
* @param value The negativeCaching to set.
* @return This builder for chaining.
*/
public Builder setNegativeCaching(boolean value) {
negativeCaching_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Negative caching allows per-status code TTLs to be set, in order to apply fine-grained caching for common errors or redirects. This can reduce the load on your origin and improve end-user experience by reducing response latency. When the cache mode is set to CACHE_ALL_STATIC or USE_ORIGIN_HEADERS, negative caching applies to responses with the specified response code that lack any Cache-Control, Expires, or Pragma: no-cache directives. When the cache mode is set to FORCE_CACHE_ALL, negative caching applies to all responses with the specified response code, and override any caching headers. By default, Cloud CDN will apply the following default TTLs to these status codes: HTTP 300 (Multiple Choice), 301, 308 (Permanent Redirects): 10m HTTP 404 (Not Found), 410 (Gone), 451 (Unavailable For Legal Reasons): 120s HTTP 405 (Method Not Found), 421 (Misdirected Request), 501 (Not Implemented): 60s. These defaults can be overridden in negative_caching_policy.
*
*
* optional bool negative_caching = 336110005;
*
* @return This builder for chaining.
*/
public Builder clearNegativeCaching() {
bitField0_ = (bitField0_ & ~0x00000040);
negativeCaching_ = false;
onChanged();
return this;
}
private java.util.List
negativeCachingPolicy_ = java.util.Collections.emptyList();
private void ensureNegativeCachingPolicyIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
negativeCachingPolicy_ =
new java.util.ArrayList<
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy>(
negativeCachingPolicy_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy,
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy.Builder,
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicyOrBuilder>
negativeCachingPolicyBuilder_;
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public java.util.List
getNegativeCachingPolicyList() {
if (negativeCachingPolicyBuilder_ == null) {
return java.util.Collections.unmodifiableList(negativeCachingPolicy_);
} else {
return negativeCachingPolicyBuilder_.getMessageList();
}
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public int getNegativeCachingPolicyCount() {
if (negativeCachingPolicyBuilder_ == null) {
return negativeCachingPolicy_.size();
} else {
return negativeCachingPolicyBuilder_.getCount();
}
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy
getNegativeCachingPolicy(int index) {
if (negativeCachingPolicyBuilder_ == null) {
return negativeCachingPolicy_.get(index);
} else {
return negativeCachingPolicyBuilder_.getMessage(index);
}
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public Builder setNegativeCachingPolicy(
int index, com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy value) {
if (negativeCachingPolicyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNegativeCachingPolicyIsMutable();
negativeCachingPolicy_.set(index, value);
onChanged();
} else {
negativeCachingPolicyBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public Builder setNegativeCachingPolicy(
int index,
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy.Builder
builderForValue) {
if (negativeCachingPolicyBuilder_ == null) {
ensureNegativeCachingPolicyIsMutable();
negativeCachingPolicy_.set(index, builderForValue.build());
onChanged();
} else {
negativeCachingPolicyBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public Builder addNegativeCachingPolicy(
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy value) {
if (negativeCachingPolicyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNegativeCachingPolicyIsMutable();
negativeCachingPolicy_.add(value);
onChanged();
} else {
negativeCachingPolicyBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public Builder addNegativeCachingPolicy(
int index, com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy value) {
if (negativeCachingPolicyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNegativeCachingPolicyIsMutable();
negativeCachingPolicy_.add(index, value);
onChanged();
} else {
negativeCachingPolicyBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public Builder addNegativeCachingPolicy(
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy.Builder
builderForValue) {
if (negativeCachingPolicyBuilder_ == null) {
ensureNegativeCachingPolicyIsMutable();
negativeCachingPolicy_.add(builderForValue.build());
onChanged();
} else {
negativeCachingPolicyBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public Builder addNegativeCachingPolicy(
int index,
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy.Builder
builderForValue) {
if (negativeCachingPolicyBuilder_ == null) {
ensureNegativeCachingPolicyIsMutable();
negativeCachingPolicy_.add(index, builderForValue.build());
onChanged();
} else {
negativeCachingPolicyBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public Builder addAllNegativeCachingPolicy(
java.lang.Iterable<
? extends com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy>
values) {
if (negativeCachingPolicyBuilder_ == null) {
ensureNegativeCachingPolicyIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, negativeCachingPolicy_);
onChanged();
} else {
negativeCachingPolicyBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public Builder clearNegativeCachingPolicy() {
if (negativeCachingPolicyBuilder_ == null) {
negativeCachingPolicy_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
negativeCachingPolicyBuilder_.clear();
}
return this;
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public Builder removeNegativeCachingPolicy(int index) {
if (negativeCachingPolicyBuilder_ == null) {
ensureNegativeCachingPolicyIsMutable();
negativeCachingPolicy_.remove(index);
onChanged();
} else {
negativeCachingPolicyBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy.Builder
getNegativeCachingPolicyBuilder(int index) {
return getNegativeCachingPolicyFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicyOrBuilder
getNegativeCachingPolicyOrBuilder(int index) {
if (negativeCachingPolicyBuilder_ == null) {
return negativeCachingPolicy_.get(index);
} else {
return negativeCachingPolicyBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public java.util.List<
? extends
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicyOrBuilder>
getNegativeCachingPolicyOrBuilderList() {
if (negativeCachingPolicyBuilder_ != null) {
return negativeCachingPolicyBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(negativeCachingPolicy_);
}
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy.Builder
addNegativeCachingPolicyBuilder() {
return getNegativeCachingPolicyFieldBuilder()
.addBuilder(
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy
.getDefaultInstance());
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy.Builder
addNegativeCachingPolicyBuilder(int index) {
return getNegativeCachingPolicyFieldBuilder()
.addBuilder(
index,
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy
.getDefaultInstance());
}
/**
*
*
*
* Sets a cache TTL for the specified HTTP status code. negative_caching must be enabled to configure negative_caching_policy. Omitting the policy and leaving negative_caching enabled will use Cloud CDN's default cache TTLs. Note that when specifying an explicit negative_caching_policy, you should take care to specify a cache TTL for all response codes that you wish to cache. Cloud CDN will not apply any default negative caching when a policy exists.
*
*
*
* repeated .google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy negative_caching_policy = 155359996;
*
*/
public java.util.List<
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy.Builder>
getNegativeCachingPolicyBuilderList() {
return getNegativeCachingPolicyFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy,
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy.Builder,
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicyOrBuilder>
getNegativeCachingPolicyFieldBuilder() {
if (negativeCachingPolicyBuilder_ == null) {
negativeCachingPolicyBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy,
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicy.Builder,
com.google.cloud.compute.v1.BackendBucketCdnPolicyNegativeCachingPolicyOrBuilder>(
negativeCachingPolicy_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
negativeCachingPolicy_ = null;
}
return negativeCachingPolicyBuilder_;
}
private boolean requestCoalescing_;
/**
*
*
*
* If true then Cloud CDN will combine multiple concurrent cache fill requests into a small number of requests to the origin.
*
*
* optional bool request_coalescing = 532808276;
*
* @return Whether the requestCoalescing field is set.
*/
@java.lang.Override
public boolean hasRequestCoalescing() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* If true then Cloud CDN will combine multiple concurrent cache fill requests into a small number of requests to the origin.
*
*
* optional bool request_coalescing = 532808276;
*
* @return The requestCoalescing.
*/
@java.lang.Override
public boolean getRequestCoalescing() {
return requestCoalescing_;
}
/**
*
*
*
* If true then Cloud CDN will combine multiple concurrent cache fill requests into a small number of requests to the origin.
*
*
* optional bool request_coalescing = 532808276;
*
* @param value The requestCoalescing to set.
* @return This builder for chaining.
*/
public Builder setRequestCoalescing(boolean value) {
requestCoalescing_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* If true then Cloud CDN will combine multiple concurrent cache fill requests into a small number of requests to the origin.
*
*
* optional bool request_coalescing = 532808276;
*
* @return This builder for chaining.
*/
public Builder clearRequestCoalescing() {
bitField0_ = (bitField0_ & ~0x00000100);
requestCoalescing_ = false;
onChanged();
return this;
}
private int serveWhileStale_;
/**
*
*
*
* Serve existing content from the cache (if available) when revalidating content with the origin, or when an error is encountered when refreshing the cache. This setting defines the default "max-stale" duration for any cached responses that do not specify a max-stale directive. Stale responses that exceed the TTL configured here will not be served. The default limit (max-stale) is 86400s (1 day), which will allow stale content to be served up to this limit beyond the max-age (or s-max-age) of a cached response. The maximum allowed value is 604800 (1 week). Set this to zero (0) to disable serve-while-stale.
*
*
* optional int32 serve_while_stale = 236682203;
*
* @return Whether the serveWhileStale field is set.
*/
@java.lang.Override
public boolean hasServeWhileStale() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Serve existing content from the cache (if available) when revalidating content with the origin, or when an error is encountered when refreshing the cache. This setting defines the default "max-stale" duration for any cached responses that do not specify a max-stale directive. Stale responses that exceed the TTL configured here will not be served. The default limit (max-stale) is 86400s (1 day), which will allow stale content to be served up to this limit beyond the max-age (or s-max-age) of a cached response. The maximum allowed value is 604800 (1 week). Set this to zero (0) to disable serve-while-stale.
*
*
* optional int32 serve_while_stale = 236682203;
*
* @return The serveWhileStale.
*/
@java.lang.Override
public int getServeWhileStale() {
return serveWhileStale_;
}
/**
*
*
*
* Serve existing content from the cache (if available) when revalidating content with the origin, or when an error is encountered when refreshing the cache. This setting defines the default "max-stale" duration for any cached responses that do not specify a max-stale directive. Stale responses that exceed the TTL configured here will not be served. The default limit (max-stale) is 86400s (1 day), which will allow stale content to be served up to this limit beyond the max-age (or s-max-age) of a cached response. The maximum allowed value is 604800 (1 week). Set this to zero (0) to disable serve-while-stale.
*
*
* optional int32 serve_while_stale = 236682203;
*
* @param value The serveWhileStale to set.
* @return This builder for chaining.
*/
public Builder setServeWhileStale(int value) {
serveWhileStale_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Serve existing content from the cache (if available) when revalidating content with the origin, or when an error is encountered when refreshing the cache. This setting defines the default "max-stale" duration for any cached responses that do not specify a max-stale directive. Stale responses that exceed the TTL configured here will not be served. The default limit (max-stale) is 86400s (1 day), which will allow stale content to be served up to this limit beyond the max-age (or s-max-age) of a cached response. The maximum allowed value is 604800 (1 week). Set this to zero (0) to disable serve-while-stale.
*
*
* optional int32 serve_while_stale = 236682203;
*
* @return This builder for chaining.
*/
public Builder clearServeWhileStale() {
bitField0_ = (bitField0_ & ~0x00000200);
serveWhileStale_ = 0;
onChanged();
return this;
}
private long signedUrlCacheMaxAgeSec_;
/**
*
*
*
* Maximum number of seconds the response to a signed URL request will be considered fresh. After this time period, the response will be revalidated before being served. Defaults to 1hr (3600s). When serving responses to signed URL requests, Cloud CDN will internally behave as though all responses from this backend had a "Cache-Control: public, max-age=[TTL]" header, regardless of any existing Cache-Control header. The actual headers served in responses will not be altered.
*
*
* optional int64 signed_url_cache_max_age_sec = 269374534;
*
* @return Whether the signedUrlCacheMaxAgeSec field is set.
*/
@java.lang.Override
public boolean hasSignedUrlCacheMaxAgeSec() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Maximum number of seconds the response to a signed URL request will be considered fresh. After this time period, the response will be revalidated before being served. Defaults to 1hr (3600s). When serving responses to signed URL requests, Cloud CDN will internally behave as though all responses from this backend had a "Cache-Control: public, max-age=[TTL]" header, regardless of any existing Cache-Control header. The actual headers served in responses will not be altered.
*
*
* optional int64 signed_url_cache_max_age_sec = 269374534;
*
* @return The signedUrlCacheMaxAgeSec.
*/
@java.lang.Override
public long getSignedUrlCacheMaxAgeSec() {
return signedUrlCacheMaxAgeSec_;
}
/**
*
*
*
* Maximum number of seconds the response to a signed URL request will be considered fresh. After this time period, the response will be revalidated before being served. Defaults to 1hr (3600s). When serving responses to signed URL requests, Cloud CDN will internally behave as though all responses from this backend had a "Cache-Control: public, max-age=[TTL]" header, regardless of any existing Cache-Control header. The actual headers served in responses will not be altered.
*
*
* optional int64 signed_url_cache_max_age_sec = 269374534;
*
* @param value The signedUrlCacheMaxAgeSec to set.
* @return This builder for chaining.
*/
public Builder setSignedUrlCacheMaxAgeSec(long value) {
signedUrlCacheMaxAgeSec_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Maximum number of seconds the response to a signed URL request will be considered fresh. After this time period, the response will be revalidated before being served. Defaults to 1hr (3600s). When serving responses to signed URL requests, Cloud CDN will internally behave as though all responses from this backend had a "Cache-Control: public, max-age=[TTL]" header, regardless of any existing Cache-Control header. The actual headers served in responses will not be altered.
*
*
* optional int64 signed_url_cache_max_age_sec = 269374534;
*
* @return This builder for chaining.
*/
public Builder clearSignedUrlCacheMaxAgeSec() {
bitField0_ = (bitField0_ & ~0x00000400);
signedUrlCacheMaxAgeSec_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList signedUrlKeyNames_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureSignedUrlKeyNamesIsMutable() {
if (!signedUrlKeyNames_.isModifiable()) {
signedUrlKeyNames_ = new com.google.protobuf.LazyStringArrayList(signedUrlKeyNames_);
}
bitField0_ |= 0x00000800;
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @return A list containing the signedUrlKeyNames.
*/
public com.google.protobuf.ProtocolStringList getSignedUrlKeyNamesList() {
signedUrlKeyNames_.makeImmutable();
return signedUrlKeyNames_;
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @return The count of signedUrlKeyNames.
*/
public int getSignedUrlKeyNamesCount() {
return signedUrlKeyNames_.size();
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @param index The index of the element to return.
* @return The signedUrlKeyNames at the given index.
*/
public java.lang.String getSignedUrlKeyNames(int index) {
return signedUrlKeyNames_.get(index);
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @param index The index of the value to return.
* @return The bytes of the signedUrlKeyNames at the given index.
*/
public com.google.protobuf.ByteString getSignedUrlKeyNamesBytes(int index) {
return signedUrlKeyNames_.getByteString(index);
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @param index The index to set the value at.
* @param value The signedUrlKeyNames to set.
* @return This builder for chaining.
*/
public Builder setSignedUrlKeyNames(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSignedUrlKeyNamesIsMutable();
signedUrlKeyNames_.set(index, value);
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @param value The signedUrlKeyNames to add.
* @return This builder for chaining.
*/
public Builder addSignedUrlKeyNames(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSignedUrlKeyNamesIsMutable();
signedUrlKeyNames_.add(value);
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @param values The signedUrlKeyNames to add.
* @return This builder for chaining.
*/
public Builder addAllSignedUrlKeyNames(java.lang.Iterable values) {
ensureSignedUrlKeyNamesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, signedUrlKeyNames_);
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @return This builder for chaining.
*/
public Builder clearSignedUrlKeyNames() {
signedUrlKeyNames_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000800);
;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Names of the keys for signing request URLs.
*
*
* repeated string signed_url_key_names = 371848885;
*
* @param value The bytes of the signedUrlKeyNames to add.
* @return This builder for chaining.
*/
public Builder addSignedUrlKeyNamesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSignedUrlKeyNamesIsMutable();
signedUrlKeyNames_.add(value);
bitField0_ |= 0x00000800;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.BackendBucketCdnPolicy)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.BackendBucketCdnPolicy)
private static final com.google.cloud.compute.v1.BackendBucketCdnPolicy DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.BackendBucketCdnPolicy();
}
public static com.google.cloud.compute.v1.BackendBucketCdnPolicy getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BackendBucketCdnPolicy parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.BackendBucketCdnPolicy getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy