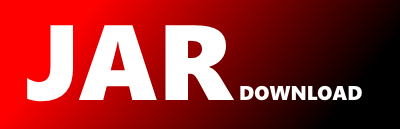
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* Specifies the mapping between the response code that will be returned along with the custom error content and the response code returned by the backend service.
*
*
* Protobuf type {@code google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule}
*/
public final class CustomErrorResponsePolicyCustomErrorResponseRule
extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule)
CustomErrorResponsePolicyCustomErrorResponseRuleOrBuilder {
private static final long serialVersionUID = 0L;
// Use CustomErrorResponsePolicyCustomErrorResponseRule.newBuilder() to construct.
private CustomErrorResponsePolicyCustomErrorResponseRule(
com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CustomErrorResponsePolicyCustomErrorResponseRule() {
matchResponseCodes_ = com.google.protobuf.LazyStringArrayList.emptyList();
path_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new CustomErrorResponsePolicyCustomErrorResponseRule();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_CustomErrorResponsePolicyCustomErrorResponseRule_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_CustomErrorResponsePolicyCustomErrorResponseRule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule.class,
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule.Builder
.class);
}
private int bitField0_;
public static final int MATCH_RESPONSE_CODES_FIELD_NUMBER = 104973410;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList matchResponseCodes_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @return A list containing the matchResponseCodes.
*/
public com.google.protobuf.ProtocolStringList getMatchResponseCodesList() {
return matchResponseCodes_;
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @return The count of matchResponseCodes.
*/
public int getMatchResponseCodesCount() {
return matchResponseCodes_.size();
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @param index The index of the element to return.
* @return The matchResponseCodes at the given index.
*/
public java.lang.String getMatchResponseCodes(int index) {
return matchResponseCodes_.get(index);
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @param index The index of the value to return.
* @return The bytes of the matchResponseCodes at the given index.
*/
public com.google.protobuf.ByteString getMatchResponseCodesBytes(int index) {
return matchResponseCodes_.getByteString(index);
}
public static final int OVERRIDE_RESPONSE_CODE_FIELD_NUMBER = 530328568;
private int overrideResponseCode_ = 0;
/**
*
*
*
* The HTTP status code returned with the response containing the custom error content. If overrideResponseCode is not supplied, the same response code returned by the original backend bucket or backend service is returned to the client.
*
*
* optional int32 override_response_code = 530328568;
*
* @return Whether the overrideResponseCode field is set.
*/
@java.lang.Override
public boolean hasOverrideResponseCode() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The HTTP status code returned with the response containing the custom error content. If overrideResponseCode is not supplied, the same response code returned by the original backend bucket or backend service is returned to the client.
*
*
* optional int32 override_response_code = 530328568;
*
* @return The overrideResponseCode.
*/
@java.lang.Override
public int getOverrideResponseCode() {
return overrideResponseCode_;
}
public static final int PATH_FIELD_NUMBER = 3433509;
@SuppressWarnings("serial")
private volatile java.lang.Object path_ = "";
/**
*
*
*
* The full path to a file within backendBucket . For example: /errors/defaultError.html path must start with a leading slash. path cannot have trailing slashes. If the file is not available in backendBucket or the load balancer cannot reach the BackendBucket, a simple Not Found Error is returned to the client. The value must be from 1 to 1024 characters
*
*
* optional string path = 3433509;
*
* @return Whether the path field is set.
*/
@java.lang.Override
public boolean hasPath() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The full path to a file within backendBucket . For example: /errors/defaultError.html path must start with a leading slash. path cannot have trailing slashes. If the file is not available in backendBucket or the load balancer cannot reach the BackendBucket, a simple Not Found Error is returned to the client. The value must be from 1 to 1024 characters
*
*
* optional string path = 3433509;
*
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
}
}
/**
*
*
*
* The full path to a file within backendBucket . For example: /errors/defaultError.html path must start with a leading slash. path cannot have trailing slashes. If the file is not available in backendBucket or the load balancer cannot reach the BackendBucket, a simple Not Found Error is returned to the client. The value must be from 1 to 1024 characters
*
*
* optional string path = 3433509;
*
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3433509, path_);
}
for (int i = 0; i < matchResponseCodes_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 104973410, matchResponseCodes_.getRaw(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(530328568, overrideResponseCode_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3433509, path_);
}
{
int dataSize = 0;
for (int i = 0; i < matchResponseCodes_.size(); i++) {
dataSize += computeStringSizeNoTag(matchResponseCodes_.getRaw(i));
}
size += dataSize;
size += 5 * getMatchResponseCodesList().size();
}
if (((bitField0_ & 0x00000001) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeInt32Size(530328568, overrideResponseCode_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj
instanceof com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule other =
(com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule) obj;
if (!getMatchResponseCodesList().equals(other.getMatchResponseCodesList())) return false;
if (hasOverrideResponseCode() != other.hasOverrideResponseCode()) return false;
if (hasOverrideResponseCode()) {
if (getOverrideResponseCode() != other.getOverrideResponseCode()) return false;
}
if (hasPath() != other.hasPath()) return false;
if (hasPath()) {
if (!getPath().equals(other.getPath())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getMatchResponseCodesCount() > 0) {
hash = (37 * hash) + MATCH_RESPONSE_CODES_FIELD_NUMBER;
hash = (53 * hash) + getMatchResponseCodesList().hashCode();
}
if (hasOverrideResponseCode()) {
hash = (37 * hash) + OVERRIDE_RESPONSE_CODE_FIELD_NUMBER;
hash = (53 * hash) + getOverrideResponseCode();
}
if (hasPath()) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseFrom(com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseFrom(byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseFrom(byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseDelimitedFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseFrom(com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Specifies the mapping between the response code that will be returned along with the custom error content and the response code returned by the backend service.
*
*
* Protobuf type {@code google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule)
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRuleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_CustomErrorResponsePolicyCustomErrorResponseRule_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_CustomErrorResponsePolicyCustomErrorResponseRule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule.class,
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule.Builder
.class);
}
// Construct using
// com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
matchResponseCodes_ = com.google.protobuf.LazyStringArrayList.emptyList();
overrideResponseCode_ = 0;
path_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_CustomErrorResponsePolicyCustomErrorResponseRule_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
getDefaultInstanceForType() {
return com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule build() {
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule result =
buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
buildPartial() {
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule result =
new com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
matchResponseCodes_.makeImmutable();
result.matchResponseCodes_ = matchResponseCodes_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.overrideResponseCode_ = overrideResponseCode_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.path_ = path_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other
instanceof com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule) {
return mergeFrom(
(com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule other) {
if (other
== com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
.getDefaultInstance()) return this;
if (!other.matchResponseCodes_.isEmpty()) {
if (matchResponseCodes_.isEmpty()) {
matchResponseCodes_ = other.matchResponseCodes_;
bitField0_ |= 0x00000001;
} else {
ensureMatchResponseCodesIsMutable();
matchResponseCodes_.addAll(other.matchResponseCodes_);
}
onChanged();
}
if (other.hasOverrideResponseCode()) {
setOverrideResponseCode(other.getOverrideResponseCode());
}
if (other.hasPath()) {
path_ = other.path_;
bitField0_ |= 0x00000004;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 27468074:
{
path_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 27468074
case 839787282:
{
java.lang.String s = input.readStringRequireUtf8();
ensureMatchResponseCodesIsMutable();
matchResponseCodes_.add(s);
break;
} // case 839787282
case -52338752:
{
overrideResponseCode_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case -52338752
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList matchResponseCodes_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureMatchResponseCodesIsMutable() {
if (!matchResponseCodes_.isModifiable()) {
matchResponseCodes_ = new com.google.protobuf.LazyStringArrayList(matchResponseCodes_);
}
bitField0_ |= 0x00000001;
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @return A list containing the matchResponseCodes.
*/
public com.google.protobuf.ProtocolStringList getMatchResponseCodesList() {
matchResponseCodes_.makeImmutable();
return matchResponseCodes_;
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @return The count of matchResponseCodes.
*/
public int getMatchResponseCodesCount() {
return matchResponseCodes_.size();
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @param index The index of the element to return.
* @return The matchResponseCodes at the given index.
*/
public java.lang.String getMatchResponseCodes(int index) {
return matchResponseCodes_.get(index);
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @param index The index of the value to return.
* @return The bytes of the matchResponseCodes at the given index.
*/
public com.google.protobuf.ByteString getMatchResponseCodesBytes(int index) {
return matchResponseCodes_.getByteString(index);
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @param index The index to set the value at.
* @param value The matchResponseCodes to set.
* @return This builder for chaining.
*/
public Builder setMatchResponseCodes(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureMatchResponseCodesIsMutable();
matchResponseCodes_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @param value The matchResponseCodes to add.
* @return This builder for chaining.
*/
public Builder addMatchResponseCodes(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureMatchResponseCodesIsMutable();
matchResponseCodes_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @param values The matchResponseCodes to add.
* @return This builder for chaining.
*/
public Builder addAllMatchResponseCodes(java.lang.Iterable values) {
ensureMatchResponseCodesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, matchResponseCodes_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @return This builder for chaining.
*/
public Builder clearMatchResponseCodes() {
matchResponseCodes_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
;
onChanged();
return this;
}
/**
*
*
*
* Valid values include: - A number between 400 and 599: For example 401 or 503, in which case the load balancer applies the policy if the error code exactly matches this value. - 5xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 500 to 599. - 4xx: Load Balancer will apply the policy if the backend service responds with any response code in the range of 400 to 499. Values must be unique within matchResponseCodes and across all errorResponseRules of CustomErrorResponsePolicy.
*
*
* repeated string match_response_codes = 104973410;
*
* @param value The bytes of the matchResponseCodes to add.
* @return This builder for chaining.
*/
public Builder addMatchResponseCodesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureMatchResponseCodesIsMutable();
matchResponseCodes_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int overrideResponseCode_;
/**
*
*
*
* The HTTP status code returned with the response containing the custom error content. If overrideResponseCode is not supplied, the same response code returned by the original backend bucket or backend service is returned to the client.
*
*
* optional int32 override_response_code = 530328568;
*
* @return Whether the overrideResponseCode field is set.
*/
@java.lang.Override
public boolean hasOverrideResponseCode() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The HTTP status code returned with the response containing the custom error content. If overrideResponseCode is not supplied, the same response code returned by the original backend bucket or backend service is returned to the client.
*
*
* optional int32 override_response_code = 530328568;
*
* @return The overrideResponseCode.
*/
@java.lang.Override
public int getOverrideResponseCode() {
return overrideResponseCode_;
}
/**
*
*
*
* The HTTP status code returned with the response containing the custom error content. If overrideResponseCode is not supplied, the same response code returned by the original backend bucket or backend service is returned to the client.
*
*
* optional int32 override_response_code = 530328568;
*
* @param value The overrideResponseCode to set.
* @return This builder for chaining.
*/
public Builder setOverrideResponseCode(int value) {
overrideResponseCode_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* The HTTP status code returned with the response containing the custom error content. If overrideResponseCode is not supplied, the same response code returned by the original backend bucket or backend service is returned to the client.
*
*
* optional int32 override_response_code = 530328568;
*
* @return This builder for chaining.
*/
public Builder clearOverrideResponseCode() {
bitField0_ = (bitField0_ & ~0x00000002);
overrideResponseCode_ = 0;
onChanged();
return this;
}
private java.lang.Object path_ = "";
/**
*
*
*
* The full path to a file within backendBucket . For example: /errors/defaultError.html path must start with a leading slash. path cannot have trailing slashes. If the file is not available in backendBucket or the load balancer cannot reach the BackendBucket, a simple Not Found Error is returned to the client. The value must be from 1 to 1024 characters
*
*
* optional string path = 3433509;
*
* @return Whether the path field is set.
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* The full path to a file within backendBucket . For example: /errors/defaultError.html path must start with a leading slash. path cannot have trailing slashes. If the file is not available in backendBucket or the load balancer cannot reach the BackendBucket, a simple Not Found Error is returned to the client. The value must be from 1 to 1024 characters
*
*
* optional string path = 3433509;
*
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The full path to a file within backendBucket . For example: /errors/defaultError.html path must start with a leading slash. path cannot have trailing slashes. If the file is not available in backendBucket or the load balancer cannot reach the BackendBucket, a simple Not Found Error is returned to the client. The value must be from 1 to 1024 characters
*
*
* optional string path = 3433509;
*
* @return The bytes for path.
*/
public com.google.protobuf.ByteString getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The full path to a file within backendBucket . For example: /errors/defaultError.html path must start with a leading slash. path cannot have trailing slashes. If the file is not available in backendBucket or the load balancer cannot reach the BackendBucket, a simple Not Found Error is returned to the client. The value must be from 1 to 1024 characters
*
*
* optional string path = 3433509;
*
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
path_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* The full path to a file within backendBucket . For example: /errors/defaultError.html path must start with a leading slash. path cannot have trailing slashes. If the file is not available in backendBucket or the load balancer cannot reach the BackendBucket, a simple Not Found Error is returned to the client. The value must be from 1 to 1024 characters
*
*
* optional string path = 3433509;
*
* @return This builder for chaining.
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* The full path to a file within backendBucket . For example: /errors/defaultError.html path must start with a leading slash. path cannot have trailing slashes. If the file is not available in backendBucket or the load balancer cannot reach the BackendBucket, a simple Not Found Error is returned to the client. The value must be from 1 to 1024 characters
*
*
* optional string path = 3433509;
*
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
path_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule)
private static final com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE =
new com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule();
}
public static com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER =
new com.google.protobuf.AbstractParser<
CustomErrorResponsePolicyCustomErrorResponseRule>() {
@java.lang.Override
public CustomErrorResponsePolicyCustomErrorResponseRule parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser
parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser
getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.CustomErrorResponsePolicyCustomErrorResponseRule
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy