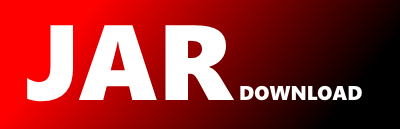
com.google.cloud.compute.v1.Disk Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* Represents a Persistent Disk resource. Google Compute Engine has two Disk resources: * [Zonal](/compute/docs/reference/rest/v1/disks) * [Regional](/compute/docs/reference/rest/v1/regionDisks) Persistent disks are required for running your VM instances. Create both boot and non-boot (data) persistent disks. For more information, read Persistent Disks. For more storage options, read Storage options. The disks resource represents a zonal persistent disk. For more information, read Zonal persistent disks. The regionDisks resource represents a regional persistent disk. For more information, read Regional resources.
*
*
* Protobuf type {@code google.cloud.compute.v1.Disk}
*/
public final class Disk extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.Disk)
DiskOrBuilder {
private static final long serialVersionUID = 0L;
// Use Disk.newBuilder() to construct.
private Disk(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Disk() {
accessMode_ = "";
architecture_ = "";
creationTimestamp_ = "";
description_ = "";
guestOsFeatures_ = java.util.Collections.emptyList();
kind_ = "";
labelFingerprint_ = "";
lastAttachTimestamp_ = "";
lastDetachTimestamp_ = "";
licenseCodes_ = emptyLongList();
licenses_ = com.google.protobuf.LazyStringArrayList.emptyList();
locationHint_ = "";
name_ = "";
options_ = "";
region_ = "";
replicaZones_ = com.google.protobuf.LazyStringArrayList.emptyList();
resourcePolicies_ = com.google.protobuf.LazyStringArrayList.emptyList();
selfLink_ = "";
sourceConsistencyGroupPolicy_ = "";
sourceConsistencyGroupPolicyId_ = "";
sourceDisk_ = "";
sourceDiskId_ = "";
sourceImage_ = "";
sourceImageId_ = "";
sourceInstantSnapshot_ = "";
sourceInstantSnapshotId_ = "";
sourceSnapshot_ = "";
sourceSnapshotId_ = "";
sourceStorageObject_ = "";
status_ = "";
storagePool_ = "";
type_ = "";
users_ = com.google.protobuf.LazyStringArrayList.emptyList();
zone_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Disk();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Disk_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 322925608:
return internalGetAsyncSecondaryDisks();
case 500195327:
return internalGetLabels();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Disk_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.Disk.class, com.google.cloud.compute.v1.Disk.Builder.class);
}
/**
*
*
*
* The access mode of the disk. - READ_WRITE_SINGLE: The default AccessMode, means the disk can be attached to single instance in RW mode. - READ_WRITE_MANY: The AccessMode means the disk can be attached to multiple instances in RW mode. - READ_ONLY_MANY: The AccessMode means the disk can be attached to multiple instances in RO mode. The AccessMode is only valid for Hyperdisk disk types.
*
*
* Protobuf enum {@code google.cloud.compute.v1.Disk.AccessMode}
*/
public enum AccessMode implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_ACCESS_MODE = 0;
*/
UNDEFINED_ACCESS_MODE(0),
/**
*
*
*
* The AccessMode means the disk can be attached to multiple instances in RO mode.
*
*
* READ_ONLY_MANY = 63460265;
*/
READ_ONLY_MANY(63460265),
/**
*
*
*
* The AccessMode means the disk can be attached to multiple instances in RW mode.
*
*
* READ_WRITE_MANY = 488743208;
*/
READ_WRITE_MANY(488743208),
/**
*
*
*
* The default AccessMode, means the disk can be attached to single instance in RW mode.
*
*
* READ_WRITE_SINGLE = 99323089;
*/
READ_WRITE_SINGLE(99323089),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_ACCESS_MODE = 0;
*/
public static final int UNDEFINED_ACCESS_MODE_VALUE = 0;
/**
*
*
*
* The AccessMode means the disk can be attached to multiple instances in RO mode.
*
*
* READ_ONLY_MANY = 63460265;
*/
public static final int READ_ONLY_MANY_VALUE = 63460265;
/**
*
*
*
* The AccessMode means the disk can be attached to multiple instances in RW mode.
*
*
* READ_WRITE_MANY = 488743208;
*/
public static final int READ_WRITE_MANY_VALUE = 488743208;
/**
*
*
*
* The default AccessMode, means the disk can be attached to single instance in RW mode.
*
*
* READ_WRITE_SINGLE = 99323089;
*/
public static final int READ_WRITE_SINGLE_VALUE = 99323089;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static AccessMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static AccessMode forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_ACCESS_MODE;
case 63460265:
return READ_ONLY_MANY;
case 488743208:
return READ_WRITE_MANY;
case 99323089:
return READ_WRITE_SINGLE;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public AccessMode findValueByNumber(int number) {
return AccessMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.Disk.getDescriptor().getEnumTypes().get(0);
}
private static final AccessMode[] VALUES = values();
public static AccessMode valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private AccessMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.Disk.AccessMode)
}
/**
*
*
*
* The architecture of the disk. Valid values are ARM64 or X86_64.
*
*
* Protobuf enum {@code google.cloud.compute.v1.Disk.Architecture}
*/
public enum Architecture implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_ARCHITECTURE = 0;
*/
UNDEFINED_ARCHITECTURE(0),
/**
*
*
*
* Default value indicating Architecture is not set.
*
*
* ARCHITECTURE_UNSPECIFIED = 394750507;
*/
ARCHITECTURE_UNSPECIFIED(394750507),
/**
*
*
*
* Machines with architecture ARM64
*
*
* ARM64 = 62547450;
*/
ARM64(62547450),
/**
*
*
*
* Machines with architecture X86_64
*
*
* X86_64 = 425300551;
*/
X86_64(425300551),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_ARCHITECTURE = 0;
*/
public static final int UNDEFINED_ARCHITECTURE_VALUE = 0;
/**
*
*
*
* Default value indicating Architecture is not set.
*
*
* ARCHITECTURE_UNSPECIFIED = 394750507;
*/
public static final int ARCHITECTURE_UNSPECIFIED_VALUE = 394750507;
/**
*
*
*
* Machines with architecture ARM64
*
*
* ARM64 = 62547450;
*/
public static final int ARM64_VALUE = 62547450;
/**
*
*
*
* Machines with architecture X86_64
*
*
* X86_64 = 425300551;
*/
public static final int X86_64_VALUE = 425300551;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Architecture valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Architecture forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_ARCHITECTURE;
case 394750507:
return ARCHITECTURE_UNSPECIFIED;
case 62547450:
return ARM64;
case 425300551:
return X86_64;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Architecture findValueByNumber(int number) {
return Architecture.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.Disk.getDescriptor().getEnumTypes().get(1);
}
private static final Architecture[] VALUES = values();
public static Architecture valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Architecture(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.Disk.Architecture)
}
/**
*
*
*
* [Output Only] The status of disk creation. - CREATING: Disk is provisioning. - RESTORING: Source data is being copied into the disk. - FAILED: Disk creation failed. - READY: Disk is ready for use. - DELETING: Disk is deleting.
*
*
* Protobuf enum {@code google.cloud.compute.v1.Disk.Status}
*/
public enum Status implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STATUS = 0;
*/
UNDEFINED_STATUS(0),
/**
*
*
*
* Disk is provisioning
*
*
* CREATING = 455564985;
*/
CREATING(455564985),
/**
*
*
*
* Disk is deleting.
*
*
* DELETING = 528602024;
*/
DELETING(528602024),
/**
*
*
*
* Disk creation failed.
*
*
* FAILED = 455706685;
*/
FAILED(455706685),
/**
*
*
*
* Disk is ready for use.
*
*
* READY = 77848963;
*/
READY(77848963),
/**
*
*
*
* Source data is being copied into the disk.
*
*
* RESTORING = 404263851;
*/
RESTORING(404263851),
/**
*
*
*
* Disk is currently unavailable and cannot be accessed, attached or detached.
*
*
* UNAVAILABLE = 413756464;
*/
UNAVAILABLE(413756464),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STATUS = 0;
*/
public static final int UNDEFINED_STATUS_VALUE = 0;
/**
*
*
*
* Disk is provisioning
*
*
* CREATING = 455564985;
*/
public static final int CREATING_VALUE = 455564985;
/**
*
*
*
* Disk is deleting.
*
*
* DELETING = 528602024;
*/
public static final int DELETING_VALUE = 528602024;
/**
*
*
*
* Disk creation failed.
*
*
* FAILED = 455706685;
*/
public static final int FAILED_VALUE = 455706685;
/**
*
*
*
* Disk is ready for use.
*
*
* READY = 77848963;
*/
public static final int READY_VALUE = 77848963;
/**
*
*
*
* Source data is being copied into the disk.
*
*
* RESTORING = 404263851;
*/
public static final int RESTORING_VALUE = 404263851;
/**
*
*
*
* Disk is currently unavailable and cannot be accessed, attached or detached.
*
*
* UNAVAILABLE = 413756464;
*/
public static final int UNAVAILABLE_VALUE = 413756464;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Status valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Status forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_STATUS;
case 455564985:
return CREATING;
case 528602024:
return DELETING;
case 455706685:
return FAILED;
case 77848963:
return READY;
case 404263851:
return RESTORING;
case 413756464:
return UNAVAILABLE;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Status findValueByNumber(int number) {
return Status.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.Disk.getDescriptor().getEnumTypes().get(2);
}
private static final Status[] VALUES = values();
public static Status valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Status(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.Disk.Status)
}
private int bitField0_;
private int bitField1_;
public static final int ACCESS_MODE_FIELD_NUMBER = 41155486;
@SuppressWarnings("serial")
private volatile java.lang.Object accessMode_ = "";
/**
*
*
*
* The access mode of the disk. - READ_WRITE_SINGLE: The default AccessMode, means the disk can be attached to single instance in RW mode. - READ_WRITE_MANY: The AccessMode means the disk can be attached to multiple instances in RW mode. - READ_ONLY_MANY: The AccessMode means the disk can be attached to multiple instances in RO mode. The AccessMode is only valid for Hyperdisk disk types.
* Check the AccessMode enum for the list of possible values.
*
*
* optional string access_mode = 41155486;
*
* @return Whether the accessMode field is set.
*/
@java.lang.Override
public boolean hasAccessMode() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The access mode of the disk. - READ_WRITE_SINGLE: The default AccessMode, means the disk can be attached to single instance in RW mode. - READ_WRITE_MANY: The AccessMode means the disk can be attached to multiple instances in RW mode. - READ_ONLY_MANY: The AccessMode means the disk can be attached to multiple instances in RO mode. The AccessMode is only valid for Hyperdisk disk types.
* Check the AccessMode enum for the list of possible values.
*
*
* optional string access_mode = 41155486;
*
* @return The accessMode.
*/
@java.lang.Override
public java.lang.String getAccessMode() {
java.lang.Object ref = accessMode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accessMode_ = s;
return s;
}
}
/**
*
*
*
* The access mode of the disk. - READ_WRITE_SINGLE: The default AccessMode, means the disk can be attached to single instance in RW mode. - READ_WRITE_MANY: The AccessMode means the disk can be attached to multiple instances in RW mode. - READ_ONLY_MANY: The AccessMode means the disk can be attached to multiple instances in RO mode. The AccessMode is only valid for Hyperdisk disk types.
* Check the AccessMode enum for the list of possible values.
*
*
* optional string access_mode = 41155486;
*
* @return The bytes for accessMode.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAccessModeBytes() {
java.lang.Object ref = accessMode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
accessMode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ARCHITECTURE_FIELD_NUMBER = 302803283;
@SuppressWarnings("serial")
private volatile java.lang.Object architecture_ = "";
/**
*
*
*
* The architecture of the disk. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return Whether the architecture field is set.
*/
@java.lang.Override
public boolean hasArchitecture() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The architecture of the disk. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return The architecture.
*/
@java.lang.Override
public java.lang.String getArchitecture() {
java.lang.Object ref = architecture_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
architecture_ = s;
return s;
}
}
/**
*
*
*
* The architecture of the disk. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return The bytes for architecture.
*/
@java.lang.Override
public com.google.protobuf.ByteString getArchitectureBytes() {
java.lang.Object ref = architecture_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
architecture_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ASYNC_PRIMARY_DISK_FIELD_NUMBER = 180517533;
private com.google.cloud.compute.v1.DiskAsyncReplication asyncPrimaryDisk_;
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*
* @return Whether the asyncPrimaryDisk field is set.
*/
@java.lang.Override
public boolean hasAsyncPrimaryDisk() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*
* @return The asyncPrimaryDisk.
*/
@java.lang.Override
public com.google.cloud.compute.v1.DiskAsyncReplication getAsyncPrimaryDisk() {
return asyncPrimaryDisk_ == null
? com.google.cloud.compute.v1.DiskAsyncReplication.getDefaultInstance()
: asyncPrimaryDisk_;
}
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.DiskAsyncReplicationOrBuilder getAsyncPrimaryDiskOrBuilder() {
return asyncPrimaryDisk_ == null
? com.google.cloud.compute.v1.DiskAsyncReplication.getDefaultInstance()
: asyncPrimaryDisk_;
}
public static final int ASYNC_SECONDARY_DISKS_FIELD_NUMBER = 322925608;
private static final class AsyncSecondaryDisksDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.compute.v1.DiskAsyncReplicationList>
defaultEntry =
com.google.protobuf.MapEntry
.
newDefaultInstance(
com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Disk_AsyncSecondaryDisksEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.cloud.compute.v1.DiskAsyncReplicationList.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.cloud.compute.v1.DiskAsyncReplicationList>
asyncSecondaryDisks_;
private com.google.protobuf.MapField<
java.lang.String, com.google.cloud.compute.v1.DiskAsyncReplicationList>
internalGetAsyncSecondaryDisks() {
if (asyncSecondaryDisks_ == null) {
return com.google.protobuf.MapField.emptyMapField(
AsyncSecondaryDisksDefaultEntryHolder.defaultEntry);
}
return asyncSecondaryDisks_;
}
public int getAsyncSecondaryDisksCount() {
return internalGetAsyncSecondaryDisks().getMap().size();
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
@java.lang.Override
public boolean containsAsyncSecondaryDisks(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetAsyncSecondaryDisks().getMap().containsKey(key);
}
/** Use {@link #getAsyncSecondaryDisksMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getAsyncSecondaryDisks() {
return getAsyncSecondaryDisksMap();
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
@java.lang.Override
public java.util.Map
getAsyncSecondaryDisksMap() {
return internalGetAsyncSecondaryDisks().getMap();
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
@java.lang.Override
public /* nullable */ com.google.cloud.compute.v1.DiskAsyncReplicationList
getAsyncSecondaryDisksOrDefault(
java.lang.String key,
/* nullable */
com.google.cloud.compute.v1.DiskAsyncReplicationList defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetAsyncSecondaryDisks().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.DiskAsyncReplicationList getAsyncSecondaryDisksOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetAsyncSecondaryDisks().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int CREATION_TIMESTAMP_FIELD_NUMBER = 30525366;
@SuppressWarnings("serial")
private volatile java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
@java.lang.Override
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
@java.lang.Override
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 422937596;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DISK_ENCRYPTION_KEY_FIELD_NUMBER = 271660677;
private com.google.cloud.compute.v1.CustomerEncryptionKey diskEncryptionKey_;
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*
* @return Whether the diskEncryptionKey field is set.
*/
@java.lang.Override
public boolean hasDiskEncryptionKey() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*
* @return The diskEncryptionKey.
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKey getDiskEncryptionKey() {
return diskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: diskEncryptionKey_;
}
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getDiskEncryptionKeyOrBuilder() {
return diskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: diskEncryptionKey_;
}
public static final int ENABLE_CONFIDENTIAL_COMPUTE_FIELD_NUMBER = 102135228;
private boolean enableConfidentialCompute_ = false;
/**
*
*
*
* Whether this disk is using confidential compute mode.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @return Whether the enableConfidentialCompute field is set.
*/
@java.lang.Override
public boolean hasEnableConfidentialCompute() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Whether this disk is using confidential compute mode.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @return The enableConfidentialCompute.
*/
@java.lang.Override
public boolean getEnableConfidentialCompute() {
return enableConfidentialCompute_;
}
public static final int GUEST_OS_FEATURES_FIELD_NUMBER = 79294545;
@SuppressWarnings("serial")
private java.util.List guestOsFeatures_;
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public java.util.List getGuestOsFeaturesList() {
return guestOsFeatures_;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
getGuestOsFeaturesOrBuilderList() {
return guestOsFeatures_;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public int getGuestOsFeaturesCount() {
return guestOsFeatures_.size();
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public com.google.cloud.compute.v1.GuestOsFeature getGuestOsFeatures(int index) {
return guestOsFeatures_.get(index);
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public com.google.cloud.compute.v1.GuestOsFeatureOrBuilder getGuestOsFeaturesOrBuilder(
int index) {
return guestOsFeatures_.get(index);
}
public static final int ID_FIELD_NUMBER = 3355;
private long id_ = 0L;
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int KIND_FIELD_NUMBER = 3292052;
@SuppressWarnings("serial")
private volatile java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#disk for disks.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
@java.lang.Override
public boolean hasKind() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#disk for disks.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
@java.lang.Override
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#disk for disks.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LABEL_FINGERPRINT_FIELD_NUMBER = 178124825;
@SuppressWarnings("serial")
private volatile java.lang.Object labelFingerprint_ = "";
/**
*
*
*
* A fingerprint for the labels being applied to this disk, which is essentially a hash of the labels set used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve a disk.
*
*
* optional string label_fingerprint = 178124825;
*
* @return Whether the labelFingerprint field is set.
*/
@java.lang.Override
public boolean hasLabelFingerprint() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* A fingerprint for the labels being applied to this disk, which is essentially a hash of the labels set used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve a disk.
*
*
* optional string label_fingerprint = 178124825;
*
* @return The labelFingerprint.
*/
@java.lang.Override
public java.lang.String getLabelFingerprint() {
java.lang.Object ref = labelFingerprint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
labelFingerprint_ = s;
return s;
}
}
/**
*
*
*
* A fingerprint for the labels being applied to this disk, which is essentially a hash of the labels set used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve a disk.
*
*
* optional string label_fingerprint = 178124825;
*
* @return The bytes for labelFingerprint.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLabelFingerprintBytes() {
java.lang.Object ref = labelFingerprint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
labelFingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LABELS_FIELD_NUMBER = 500195327;
private static final class LabelsDefaultEntryHolder {
static final com.google.protobuf.MapEntry defaultEntry =
com.google.protobuf.MapEntry.newDefaultInstance(
com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Disk_LabelsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField labels_;
private com.google.protobuf.MapField internalGetLabels() {
if (labels_ == null) {
return com.google.protobuf.MapField.emptyMapField(LabelsDefaultEntryHolder.defaultEntry);
}
return labels_;
}
public int getLabelsCount() {
return internalGetLabels().getMap().size();
}
/**
*
*
*
* Labels to apply to this disk. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public boolean containsLabels(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetLabels().getMap().containsKey(key);
}
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getLabels() {
return getLabelsMap();
}
/**
*
*
*
* Labels to apply to this disk. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public java.util.Map getLabelsMap() {
return internalGetLabels().getMap();
}
/**
*
*
*
* Labels to apply to this disk. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public /* nullable */ java.lang.String getLabelsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Labels to apply to this disk. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public java.lang.String getLabelsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int LAST_ATTACH_TIMESTAMP_FIELD_NUMBER = 42159653;
@SuppressWarnings("serial")
private volatile java.lang.Object lastAttachTimestamp_ = "";
/**
*
*
*
* [Output Only] Last attach timestamp in RFC3339 text format.
*
*
* optional string last_attach_timestamp = 42159653;
*
* @return Whether the lastAttachTimestamp field is set.
*/
@java.lang.Override
public boolean hasLastAttachTimestamp() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* [Output Only] Last attach timestamp in RFC3339 text format.
*
*
* optional string last_attach_timestamp = 42159653;
*
* @return The lastAttachTimestamp.
*/
@java.lang.Override
public java.lang.String getLastAttachTimestamp() {
java.lang.Object ref = lastAttachTimestamp_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
lastAttachTimestamp_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Last attach timestamp in RFC3339 text format.
*
*
* optional string last_attach_timestamp = 42159653;
*
* @return The bytes for lastAttachTimestamp.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLastAttachTimestampBytes() {
java.lang.Object ref = lastAttachTimestamp_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
lastAttachTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LAST_DETACH_TIMESTAMP_FIELD_NUMBER = 56471027;
@SuppressWarnings("serial")
private volatile java.lang.Object lastDetachTimestamp_ = "";
/**
*
*
*
* [Output Only] Last detach timestamp in RFC3339 text format.
*
*
* optional string last_detach_timestamp = 56471027;
*
* @return Whether the lastDetachTimestamp field is set.
*/
@java.lang.Override
public boolean hasLastDetachTimestamp() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* [Output Only] Last detach timestamp in RFC3339 text format.
*
*
* optional string last_detach_timestamp = 56471027;
*
* @return The lastDetachTimestamp.
*/
@java.lang.Override
public java.lang.String getLastDetachTimestamp() {
java.lang.Object ref = lastDetachTimestamp_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
lastDetachTimestamp_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Last detach timestamp in RFC3339 text format.
*
*
* optional string last_detach_timestamp = 56471027;
*
* @return The bytes for lastDetachTimestamp.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLastDetachTimestampBytes() {
java.lang.Object ref = lastDetachTimestamp_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
lastDetachTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LICENSE_CODES_FIELD_NUMBER = 45482664;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.LongList licenseCodes_ = emptyLongList();
/**
*
*
*
* Integer license codes indicating which licenses are attached to this disk.
*
*
* repeated int64 license_codes = 45482664;
*
* @return A list containing the licenseCodes.
*/
@java.lang.Override
public java.util.List getLicenseCodesList() {
return licenseCodes_;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this disk.
*
*
* repeated int64 license_codes = 45482664;
*
* @return The count of licenseCodes.
*/
public int getLicenseCodesCount() {
return licenseCodes_.size();
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this disk.
*
*
* repeated int64 license_codes = 45482664;
*
* @param index The index of the element to return.
* @return The licenseCodes at the given index.
*/
public long getLicenseCodes(int index) {
return licenseCodes_.getLong(index);
}
private int licenseCodesMemoizedSerializedSize = -1;
public static final int LICENSES_FIELD_NUMBER = 337642578;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList licenses_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @return A list containing the licenses.
*/
public com.google.protobuf.ProtocolStringList getLicensesList() {
return licenses_;
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @return The count of licenses.
*/
public int getLicensesCount() {
return licenses_.size();
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the element to return.
* @return The licenses at the given index.
*/
public java.lang.String getLicenses(int index) {
return licenses_.get(index);
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the value to return.
* @return The bytes of the licenses at the given index.
*/
public com.google.protobuf.ByteString getLicensesBytes(int index) {
return licenses_.getByteString(index);
}
public static final int LOCATION_HINT_FIELD_NUMBER = 350519505;
@SuppressWarnings("serial")
private volatile java.lang.Object locationHint_ = "";
/**
*
*
*
* An opaque location hint used to place the disk close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return Whether the locationHint field is set.
*/
@java.lang.Override
public boolean hasLocationHint() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* An opaque location hint used to place the disk close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return The locationHint.
*/
@java.lang.Override
public java.lang.String getLocationHint() {
java.lang.Object ref = locationHint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
locationHint_ = s;
return s;
}
}
/**
*
*
*
* An opaque location hint used to place the disk close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return The bytes for locationHint.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLocationHintBytes() {
java.lang.Object ref = locationHint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
locationHint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 3373707;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPTIONS_FIELD_NUMBER = 361137822;
@SuppressWarnings("serial")
private volatile java.lang.Object options_ = "";
/**
*
*
*
* Internal use only.
*
*
* optional string options = 361137822;
*
* @return Whether the options field is set.
*/
@java.lang.Override
public boolean hasOptions() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*
*
* Internal use only.
*
*
* optional string options = 361137822;
*
* @return The options.
*/
@java.lang.Override
public java.lang.String getOptions() {
java.lang.Object ref = options_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
options_ = s;
return s;
}
}
/**
*
*
*
* Internal use only.
*
*
* optional string options = 361137822;
*
* @return The bytes for options.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOptionsBytes() {
java.lang.Object ref = options_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
options_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PARAMS_FIELD_NUMBER = 78313862;
private com.google.cloud.compute.v1.DiskParams params_;
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*
* @return Whether the params field is set.
*/
@java.lang.Override
public boolean hasParams() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*
* @return The params.
*/
@java.lang.Override
public com.google.cloud.compute.v1.DiskParams getParams() {
return params_ == null ? com.google.cloud.compute.v1.DiskParams.getDefaultInstance() : params_;
}
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*/
@java.lang.Override
public com.google.cloud.compute.v1.DiskParamsOrBuilder getParamsOrBuilder() {
return params_ == null ? com.google.cloud.compute.v1.DiskParams.getDefaultInstance() : params_;
}
public static final int PHYSICAL_BLOCK_SIZE_BYTES_FIELD_NUMBER = 420007943;
private long physicalBlockSizeBytes_ = 0L;
/**
*
*
*
* Physical block size of the persistent disk, in bytes. If not present in a request, a default value is used. The currently supported size is 4096, other sizes may be added in the future. If an unsupported value is requested, the error message will list the supported values for the caller's project.
*
*
* optional int64 physical_block_size_bytes = 420007943;
*
* @return Whether the physicalBlockSizeBytes field is set.
*/
@java.lang.Override
public boolean hasPhysicalBlockSizeBytes() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
*
*
* Physical block size of the persistent disk, in bytes. If not present in a request, a default value is used. The currently supported size is 4096, other sizes may be added in the future. If an unsupported value is requested, the error message will list the supported values for the caller's project.
*
*
* optional int64 physical_block_size_bytes = 420007943;
*
* @return The physicalBlockSizeBytes.
*/
@java.lang.Override
public long getPhysicalBlockSizeBytes() {
return physicalBlockSizeBytes_;
}
public static final int PROVISIONED_IOPS_FIELD_NUMBER = 186769108;
private long provisionedIops_ = 0L;
/**
*
*
*
* Indicates how many IOPS to provision for the disk. This sets the number of I/O operations per second that the disk can handle. Values must be between 10,000 and 120,000. For more details, see the Extreme persistent disk documentation.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @return Whether the provisionedIops field is set.
*/
@java.lang.Override
public boolean hasProvisionedIops() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
*
*
* Indicates how many IOPS to provision for the disk. This sets the number of I/O operations per second that the disk can handle. Values must be between 10,000 and 120,000. For more details, see the Extreme persistent disk documentation.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @return The provisionedIops.
*/
@java.lang.Override
public long getProvisionedIops() {
return provisionedIops_;
}
public static final int PROVISIONED_THROUGHPUT_FIELD_NUMBER = 526524181;
private long provisionedThroughput_ = 0L;
/**
*
*
*
* Indicates how much throughput to provision for the disk. This sets the number of throughput mb per second that the disk can handle. Values must be greater than or equal to 1.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @return Whether the provisionedThroughput field is set.
*/
@java.lang.Override
public boolean hasProvisionedThroughput() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
*
*
* Indicates how much throughput to provision for the disk. This sets the number of throughput mb per second that the disk can handle. Values must be greater than or equal to 1.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @return The provisionedThroughput.
*/
@java.lang.Override
public long getProvisionedThroughput() {
return provisionedThroughput_;
}
public static final int REGION_FIELD_NUMBER = 138946292;
@SuppressWarnings("serial")
private volatile java.lang.Object region_ = "";
/**
*
*
*
* [Output Only] URL of the region where the disk resides. Only applicable for regional resources. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return Whether the region field is set.
*/
@java.lang.Override
public boolean hasRegion() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
*
*
* [Output Only] URL of the region where the disk resides. Only applicable for regional resources. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return The region.
*/
@java.lang.Override
public java.lang.String getRegion() {
java.lang.Object ref = region_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
region_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] URL of the region where the disk resides. Only applicable for regional resources. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return The bytes for region.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRegionBytes() {
java.lang.Object ref = region_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
region_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REPLICA_ZONES_FIELD_NUMBER = 48438272;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList replicaZones_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @return A list containing the replicaZones.
*/
public com.google.protobuf.ProtocolStringList getReplicaZonesList() {
return replicaZones_;
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @return The count of replicaZones.
*/
public int getReplicaZonesCount() {
return replicaZones_.size();
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @param index The index of the element to return.
* @return The replicaZones at the given index.
*/
public java.lang.String getReplicaZones(int index) {
return replicaZones_.get(index);
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @param index The index of the value to return.
* @return The bytes of the replicaZones at the given index.
*/
public com.google.protobuf.ByteString getReplicaZonesBytes(int index) {
return replicaZones_.getByteString(index);
}
public static final int RESOURCE_POLICIES_FIELD_NUMBER = 22220385;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList resourcePolicies_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @return A list containing the resourcePolicies.
*/
public com.google.protobuf.ProtocolStringList getResourcePoliciesList() {
return resourcePolicies_;
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @return The count of resourcePolicies.
*/
public int getResourcePoliciesCount() {
return resourcePolicies_.size();
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param index The index of the element to return.
* @return The resourcePolicies at the given index.
*/
public java.lang.String getResourcePolicies(int index) {
return resourcePolicies_.get(index);
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param index The index of the value to return.
* @return The bytes of the resourcePolicies at the given index.
*/
public com.google.protobuf.ByteString getResourcePoliciesBytes(int index) {
return resourcePolicies_.getByteString(index);
}
public static final int RESOURCE_STATUS_FIELD_NUMBER = 249429315;
private com.google.cloud.compute.v1.DiskResourceStatus resourceStatus_;
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*
* @return Whether the resourceStatus field is set.
*/
@java.lang.Override
public boolean hasResourceStatus() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*
* @return The resourceStatus.
*/
@java.lang.Override
public com.google.cloud.compute.v1.DiskResourceStatus getResourceStatus() {
return resourceStatus_ == null
? com.google.cloud.compute.v1.DiskResourceStatus.getDefaultInstance()
: resourceStatus_;
}
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*/
@java.lang.Override
public com.google.cloud.compute.v1.DiskResourceStatusOrBuilder getResourceStatusOrBuilder() {
return resourceStatus_ == null
? com.google.cloud.compute.v1.DiskResourceStatus.getDefaultInstance()
: resourceStatus_;
}
public static final int SATISFIES_PZI_FIELD_NUMBER = 480964257;
private boolean satisfiesPzi_ = false;
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @return Whether the satisfiesPzi field is set.
*/
@java.lang.Override
public boolean hasSatisfiesPzi() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @return The satisfiesPzi.
*/
@java.lang.Override
public boolean getSatisfiesPzi() {
return satisfiesPzi_;
}
public static final int SATISFIES_PZS_FIELD_NUMBER = 480964267;
private boolean satisfiesPzs_ = false;
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return Whether the satisfiesPzs field is set.
*/
@java.lang.Override
public boolean hasSatisfiesPzs() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return The satisfiesPzs.
*/
@java.lang.Override
public boolean getSatisfiesPzs() {
return satisfiesPzs_;
}
public static final int SELF_LINK_FIELD_NUMBER = 456214797;
@SuppressWarnings("serial")
private volatile java.lang.Object selfLink_ = "";
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return Whether the selfLink field is set.
*/
@java.lang.Override
public boolean hasSelfLink() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return The selfLink.
*/
@java.lang.Override
public java.lang.String getSelfLink() {
java.lang.Object ref = selfLink_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLink_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return The bytes for selfLink.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSelfLinkBytes() {
java.lang.Object ref = selfLink_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLink_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SIZE_GB_FIELD_NUMBER = 494929369;
private long sizeGb_ = 0L;
/**
*
*
*
* Size, in GB, of the persistent disk. You can specify this field when creating a persistent disk using the sourceImage, sourceSnapshot, or sourceDisk parameter, or specify it alone to create an empty persistent disk. If you specify this field along with a source, the value of sizeGb must not be less than the size of the source. Acceptable values are greater than 0.
*
*
* optional int64 size_gb = 494929369;
*
* @return Whether the sizeGb field is set.
*/
@java.lang.Override
public boolean hasSizeGb() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
*
*
*
* Size, in GB, of the persistent disk. You can specify this field when creating a persistent disk using the sourceImage, sourceSnapshot, or sourceDisk parameter, or specify it alone to create an empty persistent disk. If you specify this field along with a source, the value of sizeGb must not be less than the size of the source. Acceptable values are greater than 0.
*
*
* optional int64 size_gb = 494929369;
*
* @return The sizeGb.
*/
@java.lang.Override
public long getSizeGb() {
return sizeGb_;
}
public static final int SOURCE_CONSISTENCY_GROUP_POLICY_FIELD_NUMBER = 19616093;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceConsistencyGroupPolicy_ = "";
/**
*
*
*
* [Output Only] URL of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy = 19616093;
*
* @return Whether the sourceConsistencyGroupPolicy field is set.
*/
@java.lang.Override
public boolean hasSourceConsistencyGroupPolicy() {
return ((bitField0_ & 0x02000000) != 0);
}
/**
*
*
*
* [Output Only] URL of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy = 19616093;
*
* @return The sourceConsistencyGroupPolicy.
*/
@java.lang.Override
public java.lang.String getSourceConsistencyGroupPolicy() {
java.lang.Object ref = sourceConsistencyGroupPolicy_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceConsistencyGroupPolicy_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] URL of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy = 19616093;
*
* @return The bytes for sourceConsistencyGroupPolicy.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceConsistencyGroupPolicyBytes() {
java.lang.Object ref = sourceConsistencyGroupPolicy_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceConsistencyGroupPolicy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_CONSISTENCY_GROUP_POLICY_ID_FIELD_NUMBER = 267568957;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceConsistencyGroupPolicyId_ = "";
/**
*
*
*
* [Output Only] ID of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy_id = 267568957;
*
* @return Whether the sourceConsistencyGroupPolicyId field is set.
*/
@java.lang.Override
public boolean hasSourceConsistencyGroupPolicyId() {
return ((bitField0_ & 0x04000000) != 0);
}
/**
*
*
*
* [Output Only] ID of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy_id = 267568957;
*
* @return The sourceConsistencyGroupPolicyId.
*/
@java.lang.Override
public java.lang.String getSourceConsistencyGroupPolicyId() {
java.lang.Object ref = sourceConsistencyGroupPolicyId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceConsistencyGroupPolicyId_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] ID of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy_id = 267568957;
*
* @return The bytes for sourceConsistencyGroupPolicyId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceConsistencyGroupPolicyIdBytes() {
java.lang.Object ref = sourceConsistencyGroupPolicyId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceConsistencyGroupPolicyId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_DISK_FIELD_NUMBER = 451753793;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceDisk_ = "";
/**
*
*
*
* The source disk used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - https://www.googleapis.com/compute/v1/projects/project/regions/region /disks/disk - projects/project/zones/zone/disks/disk - projects/project/regions/region/disks/disk - zones/zone/disks/disk - regions/region/disks/disk
*
*
* optional string source_disk = 451753793;
*
* @return Whether the sourceDisk field is set.
*/
@java.lang.Override
public boolean hasSourceDisk() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
*
*
*
* The source disk used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - https://www.googleapis.com/compute/v1/projects/project/regions/region /disks/disk - projects/project/zones/zone/disks/disk - projects/project/regions/region/disks/disk - zones/zone/disks/disk - regions/region/disks/disk
*
*
* optional string source_disk = 451753793;
*
* @return The sourceDisk.
*/
@java.lang.Override
public java.lang.String getSourceDisk() {
java.lang.Object ref = sourceDisk_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceDisk_ = s;
return s;
}
}
/**
*
*
*
* The source disk used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - https://www.googleapis.com/compute/v1/projects/project/regions/region /disks/disk - projects/project/zones/zone/disks/disk - projects/project/regions/region/disks/disk - zones/zone/disks/disk - regions/region/disks/disk
*
*
* optional string source_disk = 451753793;
*
* @return The bytes for sourceDisk.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceDiskBytes() {
java.lang.Object ref = sourceDisk_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceDisk_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_DISK_ID_FIELD_NUMBER = 454190809;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceDiskId_ = "";
/**
*
*
*
* [Output Only] The unique ID of the disk used to create this disk. This value identifies the exact disk that was used to create this persistent disk. For example, if you created the persistent disk from a disk that was later deleted and recreated under the same name, the source disk ID would identify the exact version of the disk that was used.
*
*
* optional string source_disk_id = 454190809;
*
* @return Whether the sourceDiskId field is set.
*/
@java.lang.Override
public boolean hasSourceDiskId() {
return ((bitField0_ & 0x10000000) != 0);
}
/**
*
*
*
* [Output Only] The unique ID of the disk used to create this disk. This value identifies the exact disk that was used to create this persistent disk. For example, if you created the persistent disk from a disk that was later deleted and recreated under the same name, the source disk ID would identify the exact version of the disk that was used.
*
*
* optional string source_disk_id = 454190809;
*
* @return The sourceDiskId.
*/
@java.lang.Override
public java.lang.String getSourceDiskId() {
java.lang.Object ref = sourceDiskId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceDiskId_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The unique ID of the disk used to create this disk. This value identifies the exact disk that was used to create this persistent disk. For example, if you created the persistent disk from a disk that was later deleted and recreated under the same name, the source disk ID would identify the exact version of the disk that was used.
*
*
* optional string source_disk_id = 454190809;
*
* @return The bytes for sourceDiskId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceDiskIdBytes() {
java.lang.Object ref = sourceDiskId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceDiskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_IMAGE_FIELD_NUMBER = 50443319;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceImage_ = "";
/**
*
*
*
* The source image used to create this disk. If the source image is deleted, this field will not be set. To create a disk with one of the public operating system images, specify the image by its family name. For example, specify family/debian-9 to use the latest Debian 9 image: projects/debian-cloud/global/images/family/debian-9 Alternatively, use a specific version of a public operating system image: projects/debian-cloud/global/images/debian-9-stretch-vYYYYMMDD To create a disk with a custom image that you created, specify the image name in the following format: global/images/my-custom-image You can also specify a custom image by its image family, which returns the latest version of the image in that family. Replace the image name with family/family-name: global/images/family/my-image-family
*
*
* optional string source_image = 50443319;
*
* @return Whether the sourceImage field is set.
*/
@java.lang.Override
public boolean hasSourceImage() {
return ((bitField0_ & 0x20000000) != 0);
}
/**
*
*
*
* The source image used to create this disk. If the source image is deleted, this field will not be set. To create a disk with one of the public operating system images, specify the image by its family name. For example, specify family/debian-9 to use the latest Debian 9 image: projects/debian-cloud/global/images/family/debian-9 Alternatively, use a specific version of a public operating system image: projects/debian-cloud/global/images/debian-9-stretch-vYYYYMMDD To create a disk with a custom image that you created, specify the image name in the following format: global/images/my-custom-image You can also specify a custom image by its image family, which returns the latest version of the image in that family. Replace the image name with family/family-name: global/images/family/my-image-family
*
*
* optional string source_image = 50443319;
*
* @return The sourceImage.
*/
@java.lang.Override
public java.lang.String getSourceImage() {
java.lang.Object ref = sourceImage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceImage_ = s;
return s;
}
}
/**
*
*
*
* The source image used to create this disk. If the source image is deleted, this field will not be set. To create a disk with one of the public operating system images, specify the image by its family name. For example, specify family/debian-9 to use the latest Debian 9 image: projects/debian-cloud/global/images/family/debian-9 Alternatively, use a specific version of a public operating system image: projects/debian-cloud/global/images/debian-9-stretch-vYYYYMMDD To create a disk with a custom image that you created, specify the image name in the following format: global/images/my-custom-image You can also specify a custom image by its image family, which returns the latest version of the image in that family. Replace the image name with family/family-name: global/images/family/my-image-family
*
*
* optional string source_image = 50443319;
*
* @return The bytes for sourceImage.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceImageBytes() {
java.lang.Object ref = sourceImage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceImage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_IMAGE_ENCRYPTION_KEY_FIELD_NUMBER = 381503659;
private com.google.cloud.compute.v1.CustomerEncryptionKey sourceImageEncryptionKey_;
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*
* @return Whether the sourceImageEncryptionKey field is set.
*/
@java.lang.Override
public boolean hasSourceImageEncryptionKey() {
return ((bitField0_ & 0x40000000) != 0);
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*
* @return The sourceImageEncryptionKey.
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKey getSourceImageEncryptionKey() {
return sourceImageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceImageEncryptionKey_;
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getSourceImageEncryptionKeyOrBuilder() {
return sourceImageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceImageEncryptionKey_;
}
public static final int SOURCE_IMAGE_ID_FIELD_NUMBER = 55328291;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceImageId_ = "";
/**
*
*
*
* [Output Only] The ID value of the image used to create this disk. This value identifies the exact image that was used to create this persistent disk. For example, if you created the persistent disk from an image that was later deleted and recreated under the same name, the source image ID would identify the exact version of the image that was used.
*
*
* optional string source_image_id = 55328291;
*
* @return Whether the sourceImageId field is set.
*/
@java.lang.Override
public boolean hasSourceImageId() {
return ((bitField0_ & 0x80000000) != 0);
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this disk. This value identifies the exact image that was used to create this persistent disk. For example, if you created the persistent disk from an image that was later deleted and recreated under the same name, the source image ID would identify the exact version of the image that was used.
*
*
* optional string source_image_id = 55328291;
*
* @return The sourceImageId.
*/
@java.lang.Override
public java.lang.String getSourceImageId() {
java.lang.Object ref = sourceImageId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceImageId_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this disk. This value identifies the exact image that was used to create this persistent disk. For example, if you created the persistent disk from an image that was later deleted and recreated under the same name, the source image ID would identify the exact version of the image that was used.
*
*
* optional string source_image_id = 55328291;
*
* @return The bytes for sourceImageId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceImageIdBytes() {
java.lang.Object ref = sourceImageId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceImageId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_INSTANT_SNAPSHOT_FIELD_NUMBER = 219202054;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceInstantSnapshot_ = "";
/**
*
*
*
* The source instant snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /instantSnapshots/instantSnapshot - projects/project/zones/zone/instantSnapshots/instantSnapshot - zones/zone/instantSnapshots/instantSnapshot
*
*
* optional string source_instant_snapshot = 219202054;
*
* @return Whether the sourceInstantSnapshot field is set.
*/
@java.lang.Override
public boolean hasSourceInstantSnapshot() {
return ((bitField1_ & 0x00000001) != 0);
}
/**
*
*
*
* The source instant snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /instantSnapshots/instantSnapshot - projects/project/zones/zone/instantSnapshots/instantSnapshot - zones/zone/instantSnapshots/instantSnapshot
*
*
* optional string source_instant_snapshot = 219202054;
*
* @return The sourceInstantSnapshot.
*/
@java.lang.Override
public java.lang.String getSourceInstantSnapshot() {
java.lang.Object ref = sourceInstantSnapshot_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceInstantSnapshot_ = s;
return s;
}
}
/**
*
*
*
* The source instant snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /instantSnapshots/instantSnapshot - projects/project/zones/zone/instantSnapshots/instantSnapshot - zones/zone/instantSnapshots/instantSnapshot
*
*
* optional string source_instant_snapshot = 219202054;
*
* @return The bytes for sourceInstantSnapshot.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceInstantSnapshotBytes() {
java.lang.Object ref = sourceInstantSnapshot_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceInstantSnapshot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_INSTANT_SNAPSHOT_ID_FIELD_NUMBER = 287582708;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceInstantSnapshotId_ = "";
/**
*
*
*
* [Output Only] The unique ID of the instant snapshot used to create this disk. This value identifies the exact instant snapshot that was used to create this persistent disk. For example, if you created the persistent disk from an instant snapshot that was later deleted and recreated under the same name, the source instant snapshot ID would identify the exact version of the instant snapshot that was used.
*
*
* optional string source_instant_snapshot_id = 287582708;
*
* @return Whether the sourceInstantSnapshotId field is set.
*/
@java.lang.Override
public boolean hasSourceInstantSnapshotId() {
return ((bitField1_ & 0x00000002) != 0);
}
/**
*
*
*
* [Output Only] The unique ID of the instant snapshot used to create this disk. This value identifies the exact instant snapshot that was used to create this persistent disk. For example, if you created the persistent disk from an instant snapshot that was later deleted and recreated under the same name, the source instant snapshot ID would identify the exact version of the instant snapshot that was used.
*
*
* optional string source_instant_snapshot_id = 287582708;
*
* @return The sourceInstantSnapshotId.
*/
@java.lang.Override
public java.lang.String getSourceInstantSnapshotId() {
java.lang.Object ref = sourceInstantSnapshotId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceInstantSnapshotId_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The unique ID of the instant snapshot used to create this disk. This value identifies the exact instant snapshot that was used to create this persistent disk. For example, if you created the persistent disk from an instant snapshot that was later deleted and recreated under the same name, the source instant snapshot ID would identify the exact version of the instant snapshot that was used.
*
*
* optional string source_instant_snapshot_id = 287582708;
*
* @return The bytes for sourceInstantSnapshotId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceInstantSnapshotIdBytes() {
java.lang.Object ref = sourceInstantSnapshotId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceInstantSnapshotId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_SNAPSHOT_FIELD_NUMBER = 126061928;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceSnapshot_ = "";
/**
*
*
*
* The source snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project /global/snapshots/snapshot - projects/project/global/snapshots/snapshot - global/snapshots/snapshot
*
*
* optional string source_snapshot = 126061928;
*
* @return Whether the sourceSnapshot field is set.
*/
@java.lang.Override
public boolean hasSourceSnapshot() {
return ((bitField1_ & 0x00000004) != 0);
}
/**
*
*
*
* The source snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project /global/snapshots/snapshot - projects/project/global/snapshots/snapshot - global/snapshots/snapshot
*
*
* optional string source_snapshot = 126061928;
*
* @return The sourceSnapshot.
*/
@java.lang.Override
public java.lang.String getSourceSnapshot() {
java.lang.Object ref = sourceSnapshot_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceSnapshot_ = s;
return s;
}
}
/**
*
*
*
* The source snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project /global/snapshots/snapshot - projects/project/global/snapshots/snapshot - global/snapshots/snapshot
*
*
* optional string source_snapshot = 126061928;
*
* @return The bytes for sourceSnapshot.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceSnapshotBytes() {
java.lang.Object ref = sourceSnapshot_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceSnapshot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_SNAPSHOT_ENCRYPTION_KEY_FIELD_NUMBER = 303679322;
private com.google.cloud.compute.v1.CustomerEncryptionKey sourceSnapshotEncryptionKey_;
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*
* @return Whether the sourceSnapshotEncryptionKey field is set.
*/
@java.lang.Override
public boolean hasSourceSnapshotEncryptionKey() {
return ((bitField1_ & 0x00000008) != 0);
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*
* @return The sourceSnapshotEncryptionKey.
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKey getSourceSnapshotEncryptionKey() {
return sourceSnapshotEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceSnapshotEncryptionKey_;
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getSourceSnapshotEncryptionKeyOrBuilder() {
return sourceSnapshotEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceSnapshotEncryptionKey_;
}
public static final int SOURCE_SNAPSHOT_ID_FIELD_NUMBER = 98962258;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceSnapshotId_ = "";
/**
*
*
*
* [Output Only] The unique ID of the snapshot used to create this disk. This value identifies the exact snapshot that was used to create this persistent disk. For example, if you created the persistent disk from a snapshot that was later deleted and recreated under the same name, the source snapshot ID would identify the exact version of the snapshot that was used.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return Whether the sourceSnapshotId field is set.
*/
@java.lang.Override
public boolean hasSourceSnapshotId() {
return ((bitField1_ & 0x00000010) != 0);
}
/**
*
*
*
* [Output Only] The unique ID of the snapshot used to create this disk. This value identifies the exact snapshot that was used to create this persistent disk. For example, if you created the persistent disk from a snapshot that was later deleted and recreated under the same name, the source snapshot ID would identify the exact version of the snapshot that was used.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return The sourceSnapshotId.
*/
@java.lang.Override
public java.lang.String getSourceSnapshotId() {
java.lang.Object ref = sourceSnapshotId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceSnapshotId_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The unique ID of the snapshot used to create this disk. This value identifies the exact snapshot that was used to create this persistent disk. For example, if you created the persistent disk from a snapshot that was later deleted and recreated under the same name, the source snapshot ID would identify the exact version of the snapshot that was used.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return The bytes for sourceSnapshotId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceSnapshotIdBytes() {
java.lang.Object ref = sourceSnapshotId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceSnapshotId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_STORAGE_OBJECT_FIELD_NUMBER = 233052711;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceStorageObject_ = "";
/**
*
*
*
* The full Google Cloud Storage URI where the disk image is stored. This file must be a gzip-compressed tarball whose name ends in .tar.gz or virtual machine disk whose name ends in vmdk. Valid URIs may start with gs:// or https://storage.googleapis.com/. This flag is not optimized for creating multiple disks from a source storage object. To create many disks from a source storage object, use gcloud compute images import instead.
*
*
* optional string source_storage_object = 233052711;
*
* @return Whether the sourceStorageObject field is set.
*/
@java.lang.Override
public boolean hasSourceStorageObject() {
return ((bitField1_ & 0x00000020) != 0);
}
/**
*
*
*
* The full Google Cloud Storage URI where the disk image is stored. This file must be a gzip-compressed tarball whose name ends in .tar.gz or virtual machine disk whose name ends in vmdk. Valid URIs may start with gs:// or https://storage.googleapis.com/. This flag is not optimized for creating multiple disks from a source storage object. To create many disks from a source storage object, use gcloud compute images import instead.
*
*
* optional string source_storage_object = 233052711;
*
* @return The sourceStorageObject.
*/
@java.lang.Override
public java.lang.String getSourceStorageObject() {
java.lang.Object ref = sourceStorageObject_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceStorageObject_ = s;
return s;
}
}
/**
*
*
*
* The full Google Cloud Storage URI where the disk image is stored. This file must be a gzip-compressed tarball whose name ends in .tar.gz or virtual machine disk whose name ends in vmdk. Valid URIs may start with gs:// or https://storage.googleapis.com/. This flag is not optimized for creating multiple disks from a source storage object. To create many disks from a source storage object, use gcloud compute images import instead.
*
*
* optional string source_storage_object = 233052711;
*
* @return The bytes for sourceStorageObject.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceStorageObjectBytes() {
java.lang.Object ref = sourceStorageObject_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceStorageObject_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 181260274;
@SuppressWarnings("serial")
private volatile java.lang.Object status_ = "";
/**
*
*
*
* [Output Only] The status of disk creation. - CREATING: Disk is provisioning. - RESTORING: Source data is being copied into the disk. - FAILED: Disk creation failed. - READY: Disk is ready for use. - DELETING: Disk is deleting.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return ((bitField1_ & 0x00000040) != 0);
}
/**
*
*
*
* [Output Only] The status of disk creation. - CREATING: Disk is provisioning. - RESTORING: Source data is being copied into the disk. - FAILED: Disk creation failed. - READY: Disk is ready for use. - DELETING: Disk is deleting.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The status.
*/
@java.lang.Override
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
status_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The status of disk creation. - CREATING: Disk is provisioning. - RESTORING: Source data is being copied into the disk. - FAILED: Disk creation failed. - READY: Disk is ready for use. - DELETING: Disk is deleting.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The bytes for status.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STORAGE_POOL_FIELD_NUMBER = 360473440;
@SuppressWarnings("serial")
private volatile java.lang.Object storagePool_ = "";
/**
*
*
*
* The storage pool in which the new disk is created. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /storagePools/storagePool - projects/project/zones/zone/storagePools/storagePool - zones/zone/storagePools/storagePool
*
*
* optional string storage_pool = 360473440;
*
* @return Whether the storagePool field is set.
*/
@java.lang.Override
public boolean hasStoragePool() {
return ((bitField1_ & 0x00000080) != 0);
}
/**
*
*
*
* The storage pool in which the new disk is created. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /storagePools/storagePool - projects/project/zones/zone/storagePools/storagePool - zones/zone/storagePools/storagePool
*
*
* optional string storage_pool = 360473440;
*
* @return The storagePool.
*/
@java.lang.Override
public java.lang.String getStoragePool() {
java.lang.Object ref = storagePool_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
storagePool_ = s;
return s;
}
}
/**
*
*
*
* The storage pool in which the new disk is created. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /storagePools/storagePool - projects/project/zones/zone/storagePools/storagePool - zones/zone/storagePools/storagePool
*
*
* optional string storage_pool = 360473440;
*
* @return The bytes for storagePool.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStoragePoolBytes() {
java.lang.Object ref = storagePool_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
storagePool_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 3575610;
@SuppressWarnings("serial")
private volatile java.lang.Object type_ = "";
/**
*
*
*
* URL of the disk type resource describing which disk type to use to create the disk. Provide this when creating the disk. For example: projects/project /zones/zone/diskTypes/pd-ssd . See Persistent disk types.
*
*
* optional string type = 3575610;
*
* @return Whether the type field is set.
*/
@java.lang.Override
public boolean hasType() {
return ((bitField1_ & 0x00000100) != 0);
}
/**
*
*
*
* URL of the disk type resource describing which disk type to use to create the disk. Provide this when creating the disk. For example: projects/project /zones/zone/diskTypes/pd-ssd . See Persistent disk types.
*
*
* optional string type = 3575610;
*
* @return The type.
*/
@java.lang.Override
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
}
}
/**
*
*
*
* URL of the disk type resource describing which disk type to use to create the disk. Provide this when creating the disk. For example: projects/project /zones/zone/diskTypes/pd-ssd . See Persistent disk types.
*
*
* optional string type = 3575610;
*
* @return The bytes for type.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USERS_FIELD_NUMBER = 111578632;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList users_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @return A list containing the users.
*/
public com.google.protobuf.ProtocolStringList getUsersList() {
return users_;
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @return The count of users.
*/
public int getUsersCount() {
return users_.size();
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @param index The index of the element to return.
* @return The users at the given index.
*/
public java.lang.String getUsers(int index) {
return users_.get(index);
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @param index The index of the value to return.
* @return The bytes of the users at the given index.
*/
public com.google.protobuf.ByteString getUsersBytes(int index) {
return users_.getByteString(index);
}
public static final int ZONE_FIELD_NUMBER = 3744684;
@SuppressWarnings("serial")
private volatile java.lang.Object zone_ = "";
/**
*
*
*
* [Output Only] URL of the zone where the disk resides. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string zone = 3744684;
*
* @return Whether the zone field is set.
*/
@java.lang.Override
public boolean hasZone() {
return ((bitField1_ & 0x00000200) != 0);
}
/**
*
*
*
* [Output Only] URL of the zone where the disk resides. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string zone = 3744684;
*
* @return The zone.
*/
@java.lang.Override
public java.lang.String getZone() {
java.lang.Object ref = zone_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
zone_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] URL of the zone where the disk resides. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string zone = 3744684;
*
* @return The bytes for zone.
*/
@java.lang.Override
public com.google.protobuf.ByteString getZoneBytes() {
java.lang.Object ref = zone_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
zone_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000080) != 0)) {
output.writeUInt64(3355, id_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3292052, kind_);
}
if (((bitField0_ & 0x00002000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3373707, name_);
}
if (((bitField1_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3575610, type_);
}
if (((bitField1_ & 0x00000200) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3744684, zone_);
}
if (((bitField0_ & 0x02000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 19616093, sourceConsistencyGroupPolicy_);
}
for (int i = 0; i < resourcePolicies_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 22220385, resourcePolicies_.getRaw(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30525366, creationTimestamp_);
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 41155486, accessMode_);
}
if (((bitField0_ & 0x00000400) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 42159653, lastAttachTimestamp_);
}
if (getLicenseCodesList().size() > 0) {
output.writeUInt32NoTag(363861314);
output.writeUInt32NoTag(licenseCodesMemoizedSerializedSize);
}
for (int i = 0; i < licenseCodes_.size(); i++) {
output.writeInt64NoTag(licenseCodes_.getLong(i));
}
for (int i = 0; i < replicaZones_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 48438272, replicaZones_.getRaw(i));
}
if (((bitField0_ & 0x20000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 50443319, sourceImage_);
}
if (((bitField0_ & 0x80000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 55328291, sourceImageId_);
}
if (((bitField0_ & 0x00000800) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 56471027, lastDetachTimestamp_);
}
if (((bitField0_ & 0x00008000) != 0)) {
output.writeMessage(78313862, getParams());
}
for (int i = 0; i < guestOsFeatures_.size(); i++) {
output.writeMessage(79294545, guestOsFeatures_.get(i));
}
if (((bitField1_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 98962258, sourceSnapshotId_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeBool(102135228, enableConfidentialCompute_);
}
for (int i = 0; i < users_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 111578632, users_.getRaw(i));
}
if (((bitField1_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 126061928, sourceSnapshot_);
}
if (((bitField0_ & 0x00080000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 138946292, region_);
}
if (((bitField0_ & 0x00000200) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 178124825, labelFingerprint_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(180517533, getAsyncPrimaryDisk());
}
if (((bitField1_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 181260274, status_);
}
if (((bitField0_ & 0x00020000) != 0)) {
output.writeInt64(186769108, provisionedIops_);
}
if (((bitField1_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 219202054, sourceInstantSnapshot_);
}
if (((bitField1_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 233052711, sourceStorageObject_);
}
if (((bitField0_ & 0x00100000) != 0)) {
output.writeMessage(249429315, getResourceStatus());
}
if (((bitField0_ & 0x04000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 267568957, sourceConsistencyGroupPolicyId_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(271660677, getDiskEncryptionKey());
}
if (((bitField1_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 287582708, sourceInstantSnapshotId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 302803283, architecture_);
}
if (((bitField1_ & 0x00000008) != 0)) {
output.writeMessage(303679322, getSourceSnapshotEncryptionKey());
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output,
internalGetAsyncSecondaryDisks(),
AsyncSecondaryDisksDefaultEntryHolder.defaultEntry,
322925608);
for (int i = 0; i < licenses_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 337642578, licenses_.getRaw(i));
}
if (((bitField0_ & 0x00001000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 350519505, locationHint_);
}
if (((bitField1_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 360473440, storagePool_);
}
if (((bitField0_ & 0x00004000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 361137822, options_);
}
if (((bitField0_ & 0x40000000) != 0)) {
output.writeMessage(381503659, getSourceImageEncryptionKey());
}
if (((bitField0_ & 0x00010000) != 0)) {
output.writeInt64(420007943, physicalBlockSizeBytes_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 422937596, description_);
}
if (((bitField0_ & 0x08000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 451753793, sourceDisk_);
}
if (((bitField0_ & 0x10000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 454190809, sourceDiskId_);
}
if (((bitField0_ & 0x00800000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 456214797, selfLink_);
}
if (((bitField0_ & 0x00200000) != 0)) {
output.writeBool(480964257, satisfiesPzi_);
}
if (((bitField0_ & 0x00400000) != 0)) {
output.writeBool(480964267, satisfiesPzs_);
}
if (((bitField0_ & 0x01000000) != 0)) {
output.writeInt64(494929369, sizeGb_);
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetLabels(), LabelsDefaultEntryHolder.defaultEntry, 500195327);
if (((bitField0_ & 0x00040000) != 0)) {
output.writeInt64(526524181, provisionedThroughput_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeUInt64Size(3355, id_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3292052, kind_);
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3373707, name_);
}
if (((bitField1_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3575610, type_);
}
if (((bitField1_ & 0x00000200) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3744684, zone_);
}
if (((bitField0_ & 0x02000000) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(
19616093, sourceConsistencyGroupPolicy_);
}
{
int dataSize = 0;
for (int i = 0; i < resourcePolicies_.size(); i++) {
dataSize += computeStringSizeNoTag(resourcePolicies_.getRaw(i));
}
size += dataSize;
size += 4 * getResourcePoliciesList().size();
}
if (((bitField0_ & 0x00000008) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(30525366, creationTimestamp_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(41155486, accessMode_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(42159653, lastAttachTimestamp_);
}
{
int dataSize = 0;
for (int i = 0; i < licenseCodes_.size(); i++) {
dataSize +=
com.google.protobuf.CodedOutputStream.computeInt64SizeNoTag(licenseCodes_.getLong(i));
}
size += dataSize;
if (!getLicenseCodesList().isEmpty()) {
size += 5;
size += com.google.protobuf.CodedOutputStream.computeInt32SizeNoTag(dataSize);
}
licenseCodesMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < replicaZones_.size(); i++) {
dataSize += computeStringSizeNoTag(replicaZones_.getRaw(i));
}
size += dataSize;
size += 5 * getReplicaZonesList().size();
}
if (((bitField0_ & 0x20000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(50443319, sourceImage_);
}
if (((bitField0_ & 0x80000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(55328291, sourceImageId_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(56471027, lastDetachTimestamp_);
}
if (((bitField0_ & 0x00008000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(78313862, getParams());
}
for (int i = 0; i < guestOsFeatures_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
79294545, guestOsFeatures_.get(i));
}
if (((bitField1_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(98962258, sourceSnapshotId_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeBoolSize(
102135228, enableConfidentialCompute_);
}
{
int dataSize = 0;
for (int i = 0; i < users_.size(); i++) {
dataSize += computeStringSizeNoTag(users_.getRaw(i));
}
size += dataSize;
size += 5 * getUsersList().size();
}
if (((bitField1_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(126061928, sourceSnapshot_);
}
if (((bitField0_ & 0x00080000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(138946292, region_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(178124825, labelFingerprint_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
180517533, getAsyncPrimaryDisk());
}
if (((bitField1_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(181260274, status_);
}
if (((bitField0_ & 0x00020000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(186769108, provisionedIops_);
}
if (((bitField1_ & 0x00000001) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(
219202054, sourceInstantSnapshot_);
}
if (((bitField1_ & 0x00000020) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(233052711, sourceStorageObject_);
}
if (((bitField0_ & 0x00100000) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(249429315, getResourceStatus());
}
if (((bitField0_ & 0x04000000) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(
267568957, sourceConsistencyGroupPolicyId_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
271660677, getDiskEncryptionKey());
}
if (((bitField1_ & 0x00000002) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(
287582708, sourceInstantSnapshotId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(302803283, architecture_);
}
if (((bitField1_ & 0x00000008) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
303679322, getSourceSnapshotEncryptionKey());
}
for (java.util.Map.Entry
entry : internalGetAsyncSecondaryDisks().getMap().entrySet()) {
com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.compute.v1.DiskAsyncReplicationList>
asyncSecondaryDisks__ =
AsyncSecondaryDisksDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
322925608, asyncSecondaryDisks__);
}
{
int dataSize = 0;
for (int i = 0; i < licenses_.size(); i++) {
dataSize += computeStringSizeNoTag(licenses_.getRaw(i));
}
size += dataSize;
size += 5 * getLicensesList().size();
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(350519505, locationHint_);
}
if (((bitField1_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(360473440, storagePool_);
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(361137822, options_);
}
if (((bitField0_ & 0x40000000) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
381503659, getSourceImageEncryptionKey());
}
if (((bitField0_ & 0x00010000) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeInt64Size(
420007943, physicalBlockSizeBytes_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(422937596, description_);
}
if (((bitField0_ & 0x08000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(451753793, sourceDisk_);
}
if (((bitField0_ & 0x10000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(454190809, sourceDiskId_);
}
if (((bitField0_ & 0x00800000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(456214797, selfLink_);
}
if (((bitField0_ & 0x00200000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(480964257, satisfiesPzi_);
}
if (((bitField0_ & 0x00400000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(480964267, satisfiesPzs_);
}
if (((bitField0_ & 0x01000000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(494929369, sizeGb_);
}
for (java.util.Map.Entry entry :
internalGetLabels().getMap().entrySet()) {
com.google.protobuf.MapEntry labels__ =
LabelsDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(500195327, labels__);
}
if (((bitField0_ & 0x00040000) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeInt64Size(526524181, provisionedThroughput_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.Disk)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.Disk other = (com.google.cloud.compute.v1.Disk) obj;
if (hasAccessMode() != other.hasAccessMode()) return false;
if (hasAccessMode()) {
if (!getAccessMode().equals(other.getAccessMode())) return false;
}
if (hasArchitecture() != other.hasArchitecture()) return false;
if (hasArchitecture()) {
if (!getArchitecture().equals(other.getArchitecture())) return false;
}
if (hasAsyncPrimaryDisk() != other.hasAsyncPrimaryDisk()) return false;
if (hasAsyncPrimaryDisk()) {
if (!getAsyncPrimaryDisk().equals(other.getAsyncPrimaryDisk())) return false;
}
if (!internalGetAsyncSecondaryDisks().equals(other.internalGetAsyncSecondaryDisks()))
return false;
if (hasCreationTimestamp() != other.hasCreationTimestamp()) return false;
if (hasCreationTimestamp()) {
if (!getCreationTimestamp().equals(other.getCreationTimestamp())) return false;
}
if (hasDescription() != other.hasDescription()) return false;
if (hasDescription()) {
if (!getDescription().equals(other.getDescription())) return false;
}
if (hasDiskEncryptionKey() != other.hasDiskEncryptionKey()) return false;
if (hasDiskEncryptionKey()) {
if (!getDiskEncryptionKey().equals(other.getDiskEncryptionKey())) return false;
}
if (hasEnableConfidentialCompute() != other.hasEnableConfidentialCompute()) return false;
if (hasEnableConfidentialCompute()) {
if (getEnableConfidentialCompute() != other.getEnableConfidentialCompute()) return false;
}
if (!getGuestOsFeaturesList().equals(other.getGuestOsFeaturesList())) return false;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId() != other.getId()) return false;
}
if (hasKind() != other.hasKind()) return false;
if (hasKind()) {
if (!getKind().equals(other.getKind())) return false;
}
if (hasLabelFingerprint() != other.hasLabelFingerprint()) return false;
if (hasLabelFingerprint()) {
if (!getLabelFingerprint().equals(other.getLabelFingerprint())) return false;
}
if (!internalGetLabels().equals(other.internalGetLabels())) return false;
if (hasLastAttachTimestamp() != other.hasLastAttachTimestamp()) return false;
if (hasLastAttachTimestamp()) {
if (!getLastAttachTimestamp().equals(other.getLastAttachTimestamp())) return false;
}
if (hasLastDetachTimestamp() != other.hasLastDetachTimestamp()) return false;
if (hasLastDetachTimestamp()) {
if (!getLastDetachTimestamp().equals(other.getLastDetachTimestamp())) return false;
}
if (!getLicenseCodesList().equals(other.getLicenseCodesList())) return false;
if (!getLicensesList().equals(other.getLicensesList())) return false;
if (hasLocationHint() != other.hasLocationHint()) return false;
if (hasLocationHint()) {
if (!getLocationHint().equals(other.getLocationHint())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName().equals(other.getName())) return false;
}
if (hasOptions() != other.hasOptions()) return false;
if (hasOptions()) {
if (!getOptions().equals(other.getOptions())) return false;
}
if (hasParams() != other.hasParams()) return false;
if (hasParams()) {
if (!getParams().equals(other.getParams())) return false;
}
if (hasPhysicalBlockSizeBytes() != other.hasPhysicalBlockSizeBytes()) return false;
if (hasPhysicalBlockSizeBytes()) {
if (getPhysicalBlockSizeBytes() != other.getPhysicalBlockSizeBytes()) return false;
}
if (hasProvisionedIops() != other.hasProvisionedIops()) return false;
if (hasProvisionedIops()) {
if (getProvisionedIops() != other.getProvisionedIops()) return false;
}
if (hasProvisionedThroughput() != other.hasProvisionedThroughput()) return false;
if (hasProvisionedThroughput()) {
if (getProvisionedThroughput() != other.getProvisionedThroughput()) return false;
}
if (hasRegion() != other.hasRegion()) return false;
if (hasRegion()) {
if (!getRegion().equals(other.getRegion())) return false;
}
if (!getReplicaZonesList().equals(other.getReplicaZonesList())) return false;
if (!getResourcePoliciesList().equals(other.getResourcePoliciesList())) return false;
if (hasResourceStatus() != other.hasResourceStatus()) return false;
if (hasResourceStatus()) {
if (!getResourceStatus().equals(other.getResourceStatus())) return false;
}
if (hasSatisfiesPzi() != other.hasSatisfiesPzi()) return false;
if (hasSatisfiesPzi()) {
if (getSatisfiesPzi() != other.getSatisfiesPzi()) return false;
}
if (hasSatisfiesPzs() != other.hasSatisfiesPzs()) return false;
if (hasSatisfiesPzs()) {
if (getSatisfiesPzs() != other.getSatisfiesPzs()) return false;
}
if (hasSelfLink() != other.hasSelfLink()) return false;
if (hasSelfLink()) {
if (!getSelfLink().equals(other.getSelfLink())) return false;
}
if (hasSizeGb() != other.hasSizeGb()) return false;
if (hasSizeGb()) {
if (getSizeGb() != other.getSizeGb()) return false;
}
if (hasSourceConsistencyGroupPolicy() != other.hasSourceConsistencyGroupPolicy()) return false;
if (hasSourceConsistencyGroupPolicy()) {
if (!getSourceConsistencyGroupPolicy().equals(other.getSourceConsistencyGroupPolicy()))
return false;
}
if (hasSourceConsistencyGroupPolicyId() != other.hasSourceConsistencyGroupPolicyId())
return false;
if (hasSourceConsistencyGroupPolicyId()) {
if (!getSourceConsistencyGroupPolicyId().equals(other.getSourceConsistencyGroupPolicyId()))
return false;
}
if (hasSourceDisk() != other.hasSourceDisk()) return false;
if (hasSourceDisk()) {
if (!getSourceDisk().equals(other.getSourceDisk())) return false;
}
if (hasSourceDiskId() != other.hasSourceDiskId()) return false;
if (hasSourceDiskId()) {
if (!getSourceDiskId().equals(other.getSourceDiskId())) return false;
}
if (hasSourceImage() != other.hasSourceImage()) return false;
if (hasSourceImage()) {
if (!getSourceImage().equals(other.getSourceImage())) return false;
}
if (hasSourceImageEncryptionKey() != other.hasSourceImageEncryptionKey()) return false;
if (hasSourceImageEncryptionKey()) {
if (!getSourceImageEncryptionKey().equals(other.getSourceImageEncryptionKey())) return false;
}
if (hasSourceImageId() != other.hasSourceImageId()) return false;
if (hasSourceImageId()) {
if (!getSourceImageId().equals(other.getSourceImageId())) return false;
}
if (hasSourceInstantSnapshot() != other.hasSourceInstantSnapshot()) return false;
if (hasSourceInstantSnapshot()) {
if (!getSourceInstantSnapshot().equals(other.getSourceInstantSnapshot())) return false;
}
if (hasSourceInstantSnapshotId() != other.hasSourceInstantSnapshotId()) return false;
if (hasSourceInstantSnapshotId()) {
if (!getSourceInstantSnapshotId().equals(other.getSourceInstantSnapshotId())) return false;
}
if (hasSourceSnapshot() != other.hasSourceSnapshot()) return false;
if (hasSourceSnapshot()) {
if (!getSourceSnapshot().equals(other.getSourceSnapshot())) return false;
}
if (hasSourceSnapshotEncryptionKey() != other.hasSourceSnapshotEncryptionKey()) return false;
if (hasSourceSnapshotEncryptionKey()) {
if (!getSourceSnapshotEncryptionKey().equals(other.getSourceSnapshotEncryptionKey()))
return false;
}
if (hasSourceSnapshotId() != other.hasSourceSnapshotId()) return false;
if (hasSourceSnapshotId()) {
if (!getSourceSnapshotId().equals(other.getSourceSnapshotId())) return false;
}
if (hasSourceStorageObject() != other.hasSourceStorageObject()) return false;
if (hasSourceStorageObject()) {
if (!getSourceStorageObject().equals(other.getSourceStorageObject())) return false;
}
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus().equals(other.getStatus())) return false;
}
if (hasStoragePool() != other.hasStoragePool()) return false;
if (hasStoragePool()) {
if (!getStoragePool().equals(other.getStoragePool())) return false;
}
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (!getType().equals(other.getType())) return false;
}
if (!getUsersList().equals(other.getUsersList())) return false;
if (hasZone() != other.hasZone()) return false;
if (hasZone()) {
if (!getZone().equals(other.getZone())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccessMode()) {
hash = (37 * hash) + ACCESS_MODE_FIELD_NUMBER;
hash = (53 * hash) + getAccessMode().hashCode();
}
if (hasArchitecture()) {
hash = (37 * hash) + ARCHITECTURE_FIELD_NUMBER;
hash = (53 * hash) + getArchitecture().hashCode();
}
if (hasAsyncPrimaryDisk()) {
hash = (37 * hash) + ASYNC_PRIMARY_DISK_FIELD_NUMBER;
hash = (53 * hash) + getAsyncPrimaryDisk().hashCode();
}
if (!internalGetAsyncSecondaryDisks().getMap().isEmpty()) {
hash = (37 * hash) + ASYNC_SECONDARY_DISKS_FIELD_NUMBER;
hash = (53 * hash) + internalGetAsyncSecondaryDisks().hashCode();
}
if (hasCreationTimestamp()) {
hash = (37 * hash) + CREATION_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getCreationTimestamp().hashCode();
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
if (hasDiskEncryptionKey()) {
hash = (37 * hash) + DISK_ENCRYPTION_KEY_FIELD_NUMBER;
hash = (53 * hash) + getDiskEncryptionKey().hashCode();
}
if (hasEnableConfidentialCompute()) {
hash = (37 * hash) + ENABLE_CONFIDENTIAL_COMPUTE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getEnableConfidentialCompute());
}
if (getGuestOsFeaturesCount() > 0) {
hash = (37 * hash) + GUEST_OS_FEATURES_FIELD_NUMBER;
hash = (53 * hash) + getGuestOsFeaturesList().hashCode();
}
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getId());
}
if (hasKind()) {
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + getKind().hashCode();
}
if (hasLabelFingerprint()) {
hash = (37 * hash) + LABEL_FINGERPRINT_FIELD_NUMBER;
hash = (53 * hash) + getLabelFingerprint().hashCode();
}
if (!internalGetLabels().getMap().isEmpty()) {
hash = (37 * hash) + LABELS_FIELD_NUMBER;
hash = (53 * hash) + internalGetLabels().hashCode();
}
if (hasLastAttachTimestamp()) {
hash = (37 * hash) + LAST_ATTACH_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getLastAttachTimestamp().hashCode();
}
if (hasLastDetachTimestamp()) {
hash = (37 * hash) + LAST_DETACH_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getLastDetachTimestamp().hashCode();
}
if (getLicenseCodesCount() > 0) {
hash = (37 * hash) + LICENSE_CODES_FIELD_NUMBER;
hash = (53 * hash) + getLicenseCodesList().hashCode();
}
if (getLicensesCount() > 0) {
hash = (37 * hash) + LICENSES_FIELD_NUMBER;
hash = (53 * hash) + getLicensesList().hashCode();
}
if (hasLocationHint()) {
hash = (37 * hash) + LOCATION_HINT_FIELD_NUMBER;
hash = (53 * hash) + getLocationHint().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasOptions()) {
hash = (37 * hash) + OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + getOptions().hashCode();
}
if (hasParams()) {
hash = (37 * hash) + PARAMS_FIELD_NUMBER;
hash = (53 * hash) + getParams().hashCode();
}
if (hasPhysicalBlockSizeBytes()) {
hash = (37 * hash) + PHYSICAL_BLOCK_SIZE_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getPhysicalBlockSizeBytes());
}
if (hasProvisionedIops()) {
hash = (37 * hash) + PROVISIONED_IOPS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getProvisionedIops());
}
if (hasProvisionedThroughput()) {
hash = (37 * hash) + PROVISIONED_THROUGHPUT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getProvisionedThroughput());
}
if (hasRegion()) {
hash = (37 * hash) + REGION_FIELD_NUMBER;
hash = (53 * hash) + getRegion().hashCode();
}
if (getReplicaZonesCount() > 0) {
hash = (37 * hash) + REPLICA_ZONES_FIELD_NUMBER;
hash = (53 * hash) + getReplicaZonesList().hashCode();
}
if (getResourcePoliciesCount() > 0) {
hash = (37 * hash) + RESOURCE_POLICIES_FIELD_NUMBER;
hash = (53 * hash) + getResourcePoliciesList().hashCode();
}
if (hasResourceStatus()) {
hash = (37 * hash) + RESOURCE_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getResourceStatus().hashCode();
}
if (hasSatisfiesPzi()) {
hash = (37 * hash) + SATISFIES_PZI_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getSatisfiesPzi());
}
if (hasSatisfiesPzs()) {
hash = (37 * hash) + SATISFIES_PZS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getSatisfiesPzs());
}
if (hasSelfLink()) {
hash = (37 * hash) + SELF_LINK_FIELD_NUMBER;
hash = (53 * hash) + getSelfLink().hashCode();
}
if (hasSizeGb()) {
hash = (37 * hash) + SIZE_GB_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getSizeGb());
}
if (hasSourceConsistencyGroupPolicy()) {
hash = (37 * hash) + SOURCE_CONSISTENCY_GROUP_POLICY_FIELD_NUMBER;
hash = (53 * hash) + getSourceConsistencyGroupPolicy().hashCode();
}
if (hasSourceConsistencyGroupPolicyId()) {
hash = (37 * hash) + SOURCE_CONSISTENCY_GROUP_POLICY_ID_FIELD_NUMBER;
hash = (53 * hash) + getSourceConsistencyGroupPolicyId().hashCode();
}
if (hasSourceDisk()) {
hash = (37 * hash) + SOURCE_DISK_FIELD_NUMBER;
hash = (53 * hash) + getSourceDisk().hashCode();
}
if (hasSourceDiskId()) {
hash = (37 * hash) + SOURCE_DISK_ID_FIELD_NUMBER;
hash = (53 * hash) + getSourceDiskId().hashCode();
}
if (hasSourceImage()) {
hash = (37 * hash) + SOURCE_IMAGE_FIELD_NUMBER;
hash = (53 * hash) + getSourceImage().hashCode();
}
if (hasSourceImageEncryptionKey()) {
hash = (37 * hash) + SOURCE_IMAGE_ENCRYPTION_KEY_FIELD_NUMBER;
hash = (53 * hash) + getSourceImageEncryptionKey().hashCode();
}
if (hasSourceImageId()) {
hash = (37 * hash) + SOURCE_IMAGE_ID_FIELD_NUMBER;
hash = (53 * hash) + getSourceImageId().hashCode();
}
if (hasSourceInstantSnapshot()) {
hash = (37 * hash) + SOURCE_INSTANT_SNAPSHOT_FIELD_NUMBER;
hash = (53 * hash) + getSourceInstantSnapshot().hashCode();
}
if (hasSourceInstantSnapshotId()) {
hash = (37 * hash) + SOURCE_INSTANT_SNAPSHOT_ID_FIELD_NUMBER;
hash = (53 * hash) + getSourceInstantSnapshotId().hashCode();
}
if (hasSourceSnapshot()) {
hash = (37 * hash) + SOURCE_SNAPSHOT_FIELD_NUMBER;
hash = (53 * hash) + getSourceSnapshot().hashCode();
}
if (hasSourceSnapshotEncryptionKey()) {
hash = (37 * hash) + SOURCE_SNAPSHOT_ENCRYPTION_KEY_FIELD_NUMBER;
hash = (53 * hash) + getSourceSnapshotEncryptionKey().hashCode();
}
if (hasSourceSnapshotId()) {
hash = (37 * hash) + SOURCE_SNAPSHOT_ID_FIELD_NUMBER;
hash = (53 * hash) + getSourceSnapshotId().hashCode();
}
if (hasSourceStorageObject()) {
hash = (37 * hash) + SOURCE_STORAGE_OBJECT_FIELD_NUMBER;
hash = (53 * hash) + getSourceStorageObject().hashCode();
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (hasStoragePool()) {
hash = (37 * hash) + STORAGE_POOL_FIELD_NUMBER;
hash = (53 * hash) + getStoragePool().hashCode();
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
if (getUsersCount() > 0) {
hash = (37 * hash) + USERS_FIELD_NUMBER;
hash = (53 * hash) + getUsersList().hashCode();
}
if (hasZone()) {
hash = (37 * hash) + ZONE_FIELD_NUMBER;
hash = (53 * hash) + getZone().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.Disk parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Disk parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Disk parseFrom(com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Disk parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Disk parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Disk parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Disk parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Disk parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.Disk parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Disk parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.Disk parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Disk parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.Disk prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Represents a Persistent Disk resource. Google Compute Engine has two Disk resources: * [Zonal](/compute/docs/reference/rest/v1/disks) * [Regional](/compute/docs/reference/rest/v1/regionDisks) Persistent disks are required for running your VM instances. Create both boot and non-boot (data) persistent disks. For more information, read Persistent Disks. For more storage options, read Storage options. The disks resource represents a zonal persistent disk. For more information, read Zonal persistent disks. The regionDisks resource represents a regional persistent disk. For more information, read Regional resources.
*
*
* Protobuf type {@code google.cloud.compute.v1.Disk}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.Disk)
com.google.cloud.compute.v1.DiskOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Disk_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 322925608:
return internalGetAsyncSecondaryDisks();
case 500195327:
return internalGetLabels();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 322925608:
return internalGetMutableAsyncSecondaryDisks();
case 500195327:
return internalGetMutableLabels();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Disk_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.Disk.class,
com.google.cloud.compute.v1.Disk.Builder.class);
}
// Construct using com.google.cloud.compute.v1.Disk.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getAsyncPrimaryDiskFieldBuilder();
getDiskEncryptionKeyFieldBuilder();
getGuestOsFeaturesFieldBuilder();
getParamsFieldBuilder();
getResourceStatusFieldBuilder();
getSourceImageEncryptionKeyFieldBuilder();
getSourceSnapshotEncryptionKeyFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
bitField1_ = 0;
accessMode_ = "";
architecture_ = "";
asyncPrimaryDisk_ = null;
if (asyncPrimaryDiskBuilder_ != null) {
asyncPrimaryDiskBuilder_.dispose();
asyncPrimaryDiskBuilder_ = null;
}
internalGetMutableAsyncSecondaryDisks().clear();
creationTimestamp_ = "";
description_ = "";
diskEncryptionKey_ = null;
if (diskEncryptionKeyBuilder_ != null) {
diskEncryptionKeyBuilder_.dispose();
diskEncryptionKeyBuilder_ = null;
}
enableConfidentialCompute_ = false;
if (guestOsFeaturesBuilder_ == null) {
guestOsFeatures_ = java.util.Collections.emptyList();
} else {
guestOsFeatures_ = null;
guestOsFeaturesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
id_ = 0L;
kind_ = "";
labelFingerprint_ = "";
internalGetMutableLabels().clear();
lastAttachTimestamp_ = "";
lastDetachTimestamp_ = "";
licenseCodes_ = emptyLongList();
licenses_ = com.google.protobuf.LazyStringArrayList.emptyList();
locationHint_ = "";
name_ = "";
options_ = "";
params_ = null;
if (paramsBuilder_ != null) {
paramsBuilder_.dispose();
paramsBuilder_ = null;
}
physicalBlockSizeBytes_ = 0L;
provisionedIops_ = 0L;
provisionedThroughput_ = 0L;
region_ = "";
replicaZones_ = com.google.protobuf.LazyStringArrayList.emptyList();
resourcePolicies_ = com.google.protobuf.LazyStringArrayList.emptyList();
resourceStatus_ = null;
if (resourceStatusBuilder_ != null) {
resourceStatusBuilder_.dispose();
resourceStatusBuilder_ = null;
}
satisfiesPzi_ = false;
satisfiesPzs_ = false;
selfLink_ = "";
sizeGb_ = 0L;
sourceConsistencyGroupPolicy_ = "";
sourceConsistencyGroupPolicyId_ = "";
sourceDisk_ = "";
sourceDiskId_ = "";
sourceImage_ = "";
sourceImageEncryptionKey_ = null;
if (sourceImageEncryptionKeyBuilder_ != null) {
sourceImageEncryptionKeyBuilder_.dispose();
sourceImageEncryptionKeyBuilder_ = null;
}
sourceImageId_ = "";
sourceInstantSnapshot_ = "";
sourceInstantSnapshotId_ = "";
sourceSnapshot_ = "";
sourceSnapshotEncryptionKey_ = null;
if (sourceSnapshotEncryptionKeyBuilder_ != null) {
sourceSnapshotEncryptionKeyBuilder_.dispose();
sourceSnapshotEncryptionKeyBuilder_ = null;
}
sourceSnapshotId_ = "";
sourceStorageObject_ = "";
status_ = "";
storagePool_ = "";
type_ = "";
users_ = com.google.protobuf.LazyStringArrayList.emptyList();
zone_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Disk_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.Disk getDefaultInstanceForType() {
return com.google.cloud.compute.v1.Disk.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.Disk build() {
com.google.cloud.compute.v1.Disk result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.Disk buildPartial() {
com.google.cloud.compute.v1.Disk result = new com.google.cloud.compute.v1.Disk(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
if (bitField1_ != 0) {
buildPartial1(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.cloud.compute.v1.Disk result) {
if (guestOsFeaturesBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)) {
guestOsFeatures_ = java.util.Collections.unmodifiableList(guestOsFeatures_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.guestOsFeatures_ = guestOsFeatures_;
} else {
result.guestOsFeatures_ = guestOsFeaturesBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.compute.v1.Disk result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.accessMode_ = accessMode_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.architecture_ = architecture_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.asyncPrimaryDisk_ =
asyncPrimaryDiskBuilder_ == null ? asyncPrimaryDisk_ : asyncPrimaryDiskBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.asyncSecondaryDisks_ =
internalGetAsyncSecondaryDisks()
.build(AsyncSecondaryDisksDefaultEntryHolder.defaultEntry);
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.creationTimestamp_ = creationTimestamp_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.description_ = description_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.diskEncryptionKey_ =
diskEncryptionKeyBuilder_ == null
? diskEncryptionKey_
: diskEncryptionKeyBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.enableConfidentialCompute_ = enableConfidentialCompute_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.kind_ = kind_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.labelFingerprint_ = labelFingerprint_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.labels_ = internalGetLabels();
result.labels_.makeImmutable();
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.lastAttachTimestamp_ = lastAttachTimestamp_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.lastDetachTimestamp_ = lastDetachTimestamp_;
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
licenseCodes_.makeImmutable();
result.licenseCodes_ = licenseCodes_;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
licenses_.makeImmutable();
result.licenses_ = licenses_;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.locationHint_ = locationHint_;
to_bitField0_ |= 0x00001000;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00002000;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.options_ = options_;
to_bitField0_ |= 0x00004000;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.params_ = paramsBuilder_ == null ? params_ : paramsBuilder_.build();
to_bitField0_ |= 0x00008000;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.physicalBlockSizeBytes_ = physicalBlockSizeBytes_;
to_bitField0_ |= 0x00010000;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.provisionedIops_ = provisionedIops_;
to_bitField0_ |= 0x00020000;
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.provisionedThroughput_ = provisionedThroughput_;
to_bitField0_ |= 0x00040000;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
result.region_ = region_;
to_bitField0_ |= 0x00080000;
}
if (((from_bitField0_ & 0x02000000) != 0)) {
replicaZones_.makeImmutable();
result.replicaZones_ = replicaZones_;
}
if (((from_bitField0_ & 0x04000000) != 0)) {
resourcePolicies_.makeImmutable();
result.resourcePolicies_ = resourcePolicies_;
}
if (((from_bitField0_ & 0x08000000) != 0)) {
result.resourceStatus_ =
resourceStatusBuilder_ == null ? resourceStatus_ : resourceStatusBuilder_.build();
to_bitField0_ |= 0x00100000;
}
if (((from_bitField0_ & 0x10000000) != 0)) {
result.satisfiesPzi_ = satisfiesPzi_;
to_bitField0_ |= 0x00200000;
}
if (((from_bitField0_ & 0x20000000) != 0)) {
result.satisfiesPzs_ = satisfiesPzs_;
to_bitField0_ |= 0x00400000;
}
if (((from_bitField0_ & 0x40000000) != 0)) {
result.selfLink_ = selfLink_;
to_bitField0_ |= 0x00800000;
}
if (((from_bitField0_ & 0x80000000) != 0)) {
result.sizeGb_ = sizeGb_;
to_bitField0_ |= 0x01000000;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartial1(com.google.cloud.compute.v1.Disk result) {
int from_bitField1_ = bitField1_;
int to_bitField0_ = 0;
if (((from_bitField1_ & 0x00000001) != 0)) {
result.sourceConsistencyGroupPolicy_ = sourceConsistencyGroupPolicy_;
to_bitField0_ |= 0x02000000;
}
if (((from_bitField1_ & 0x00000002) != 0)) {
result.sourceConsistencyGroupPolicyId_ = sourceConsistencyGroupPolicyId_;
to_bitField0_ |= 0x04000000;
}
if (((from_bitField1_ & 0x00000004) != 0)) {
result.sourceDisk_ = sourceDisk_;
to_bitField0_ |= 0x08000000;
}
if (((from_bitField1_ & 0x00000008) != 0)) {
result.sourceDiskId_ = sourceDiskId_;
to_bitField0_ |= 0x10000000;
}
if (((from_bitField1_ & 0x00000010) != 0)) {
result.sourceImage_ = sourceImage_;
to_bitField0_ |= 0x20000000;
}
if (((from_bitField1_ & 0x00000020) != 0)) {
result.sourceImageEncryptionKey_ =
sourceImageEncryptionKeyBuilder_ == null
? sourceImageEncryptionKey_
: sourceImageEncryptionKeyBuilder_.build();
to_bitField0_ |= 0x40000000;
}
if (((from_bitField1_ & 0x00000040) != 0)) {
result.sourceImageId_ = sourceImageId_;
to_bitField0_ |= 0x80000000;
}
int to_bitField1_ = 0;
if (((from_bitField1_ & 0x00000080) != 0)) {
result.sourceInstantSnapshot_ = sourceInstantSnapshot_;
to_bitField1_ |= 0x00000001;
}
if (((from_bitField1_ & 0x00000100) != 0)) {
result.sourceInstantSnapshotId_ = sourceInstantSnapshotId_;
to_bitField1_ |= 0x00000002;
}
if (((from_bitField1_ & 0x00000200) != 0)) {
result.sourceSnapshot_ = sourceSnapshot_;
to_bitField1_ |= 0x00000004;
}
if (((from_bitField1_ & 0x00000400) != 0)) {
result.sourceSnapshotEncryptionKey_ =
sourceSnapshotEncryptionKeyBuilder_ == null
? sourceSnapshotEncryptionKey_
: sourceSnapshotEncryptionKeyBuilder_.build();
to_bitField1_ |= 0x00000008;
}
if (((from_bitField1_ & 0x00000800) != 0)) {
result.sourceSnapshotId_ = sourceSnapshotId_;
to_bitField1_ |= 0x00000010;
}
if (((from_bitField1_ & 0x00001000) != 0)) {
result.sourceStorageObject_ = sourceStorageObject_;
to_bitField1_ |= 0x00000020;
}
if (((from_bitField1_ & 0x00002000) != 0)) {
result.status_ = status_;
to_bitField1_ |= 0x00000040;
}
if (((from_bitField1_ & 0x00004000) != 0)) {
result.storagePool_ = storagePool_;
to_bitField1_ |= 0x00000080;
}
if (((from_bitField1_ & 0x00008000) != 0)) {
result.type_ = type_;
to_bitField1_ |= 0x00000100;
}
if (((from_bitField1_ & 0x00010000) != 0)) {
users_.makeImmutable();
result.users_ = users_;
}
if (((from_bitField1_ & 0x00020000) != 0)) {
result.zone_ = zone_;
to_bitField1_ |= 0x00000200;
}
result.bitField0_ |= to_bitField0_;
result.bitField1_ |= to_bitField1_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.Disk) {
return mergeFrom((com.google.cloud.compute.v1.Disk) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.Disk other) {
if (other == com.google.cloud.compute.v1.Disk.getDefaultInstance()) return this;
if (other.hasAccessMode()) {
accessMode_ = other.accessMode_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasArchitecture()) {
architecture_ = other.architecture_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasAsyncPrimaryDisk()) {
mergeAsyncPrimaryDisk(other.getAsyncPrimaryDisk());
}
internalGetMutableAsyncSecondaryDisks().mergeFrom(other.internalGetAsyncSecondaryDisks());
bitField0_ |= 0x00000008;
if (other.hasCreationTimestamp()) {
creationTimestamp_ = other.creationTimestamp_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasDescription()) {
description_ = other.description_;
bitField0_ |= 0x00000020;
onChanged();
}
if (other.hasDiskEncryptionKey()) {
mergeDiskEncryptionKey(other.getDiskEncryptionKey());
}
if (other.hasEnableConfidentialCompute()) {
setEnableConfidentialCompute(other.getEnableConfidentialCompute());
}
if (guestOsFeaturesBuilder_ == null) {
if (!other.guestOsFeatures_.isEmpty()) {
if (guestOsFeatures_.isEmpty()) {
guestOsFeatures_ = other.guestOsFeatures_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.addAll(other.guestOsFeatures_);
}
onChanged();
}
} else {
if (!other.guestOsFeatures_.isEmpty()) {
if (guestOsFeaturesBuilder_.isEmpty()) {
guestOsFeaturesBuilder_.dispose();
guestOsFeaturesBuilder_ = null;
guestOsFeatures_ = other.guestOsFeatures_;
bitField0_ = (bitField0_ & ~0x00000100);
guestOsFeaturesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getGuestOsFeaturesFieldBuilder()
: null;
} else {
guestOsFeaturesBuilder_.addAllMessages(other.guestOsFeatures_);
}
}
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasKind()) {
kind_ = other.kind_;
bitField0_ |= 0x00000400;
onChanged();
}
if (other.hasLabelFingerprint()) {
labelFingerprint_ = other.labelFingerprint_;
bitField0_ |= 0x00000800;
onChanged();
}
internalGetMutableLabels().mergeFrom(other.internalGetLabels());
bitField0_ |= 0x00001000;
if (other.hasLastAttachTimestamp()) {
lastAttachTimestamp_ = other.lastAttachTimestamp_;
bitField0_ |= 0x00002000;
onChanged();
}
if (other.hasLastDetachTimestamp()) {
lastDetachTimestamp_ = other.lastDetachTimestamp_;
bitField0_ |= 0x00004000;
onChanged();
}
if (!other.licenseCodes_.isEmpty()) {
if (licenseCodes_.isEmpty()) {
licenseCodes_ = other.licenseCodes_;
licenseCodes_.makeImmutable();
bitField0_ |= 0x00008000;
} else {
ensureLicenseCodesIsMutable();
licenseCodes_.addAll(other.licenseCodes_);
}
onChanged();
}
if (!other.licenses_.isEmpty()) {
if (licenses_.isEmpty()) {
licenses_ = other.licenses_;
bitField0_ |= 0x00010000;
} else {
ensureLicensesIsMutable();
licenses_.addAll(other.licenses_);
}
onChanged();
}
if (other.hasLocationHint()) {
locationHint_ = other.locationHint_;
bitField0_ |= 0x00020000;
onChanged();
}
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00040000;
onChanged();
}
if (other.hasOptions()) {
options_ = other.options_;
bitField0_ |= 0x00080000;
onChanged();
}
if (other.hasParams()) {
mergeParams(other.getParams());
}
if (other.hasPhysicalBlockSizeBytes()) {
setPhysicalBlockSizeBytes(other.getPhysicalBlockSizeBytes());
}
if (other.hasProvisionedIops()) {
setProvisionedIops(other.getProvisionedIops());
}
if (other.hasProvisionedThroughput()) {
setProvisionedThroughput(other.getProvisionedThroughput());
}
if (other.hasRegion()) {
region_ = other.region_;
bitField0_ |= 0x01000000;
onChanged();
}
if (!other.replicaZones_.isEmpty()) {
if (replicaZones_.isEmpty()) {
replicaZones_ = other.replicaZones_;
bitField0_ |= 0x02000000;
} else {
ensureReplicaZonesIsMutable();
replicaZones_.addAll(other.replicaZones_);
}
onChanged();
}
if (!other.resourcePolicies_.isEmpty()) {
if (resourcePolicies_.isEmpty()) {
resourcePolicies_ = other.resourcePolicies_;
bitField0_ |= 0x04000000;
} else {
ensureResourcePoliciesIsMutable();
resourcePolicies_.addAll(other.resourcePolicies_);
}
onChanged();
}
if (other.hasResourceStatus()) {
mergeResourceStatus(other.getResourceStatus());
}
if (other.hasSatisfiesPzi()) {
setSatisfiesPzi(other.getSatisfiesPzi());
}
if (other.hasSatisfiesPzs()) {
setSatisfiesPzs(other.getSatisfiesPzs());
}
if (other.hasSelfLink()) {
selfLink_ = other.selfLink_;
bitField0_ |= 0x40000000;
onChanged();
}
if (other.hasSizeGb()) {
setSizeGb(other.getSizeGb());
}
if (other.hasSourceConsistencyGroupPolicy()) {
sourceConsistencyGroupPolicy_ = other.sourceConsistencyGroupPolicy_;
bitField1_ |= 0x00000001;
onChanged();
}
if (other.hasSourceConsistencyGroupPolicyId()) {
sourceConsistencyGroupPolicyId_ = other.sourceConsistencyGroupPolicyId_;
bitField1_ |= 0x00000002;
onChanged();
}
if (other.hasSourceDisk()) {
sourceDisk_ = other.sourceDisk_;
bitField1_ |= 0x00000004;
onChanged();
}
if (other.hasSourceDiskId()) {
sourceDiskId_ = other.sourceDiskId_;
bitField1_ |= 0x00000008;
onChanged();
}
if (other.hasSourceImage()) {
sourceImage_ = other.sourceImage_;
bitField1_ |= 0x00000010;
onChanged();
}
if (other.hasSourceImageEncryptionKey()) {
mergeSourceImageEncryptionKey(other.getSourceImageEncryptionKey());
}
if (other.hasSourceImageId()) {
sourceImageId_ = other.sourceImageId_;
bitField1_ |= 0x00000040;
onChanged();
}
if (other.hasSourceInstantSnapshot()) {
sourceInstantSnapshot_ = other.sourceInstantSnapshot_;
bitField1_ |= 0x00000080;
onChanged();
}
if (other.hasSourceInstantSnapshotId()) {
sourceInstantSnapshotId_ = other.sourceInstantSnapshotId_;
bitField1_ |= 0x00000100;
onChanged();
}
if (other.hasSourceSnapshot()) {
sourceSnapshot_ = other.sourceSnapshot_;
bitField1_ |= 0x00000200;
onChanged();
}
if (other.hasSourceSnapshotEncryptionKey()) {
mergeSourceSnapshotEncryptionKey(other.getSourceSnapshotEncryptionKey());
}
if (other.hasSourceSnapshotId()) {
sourceSnapshotId_ = other.sourceSnapshotId_;
bitField1_ |= 0x00000800;
onChanged();
}
if (other.hasSourceStorageObject()) {
sourceStorageObject_ = other.sourceStorageObject_;
bitField1_ |= 0x00001000;
onChanged();
}
if (other.hasStatus()) {
status_ = other.status_;
bitField1_ |= 0x00002000;
onChanged();
}
if (other.hasStoragePool()) {
storagePool_ = other.storagePool_;
bitField1_ |= 0x00004000;
onChanged();
}
if (other.hasType()) {
type_ = other.type_;
bitField1_ |= 0x00008000;
onChanged();
}
if (!other.users_.isEmpty()) {
if (users_.isEmpty()) {
users_ = other.users_;
bitField1_ |= 0x00010000;
} else {
ensureUsersIsMutable();
users_.addAll(other.users_);
}
onChanged();
}
if (other.hasZone()) {
zone_ = other.zone_;
bitField1_ |= 0x00020000;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 26840:
{
id_ = input.readUInt64();
bitField0_ |= 0x00000200;
break;
} // case 26840
case 26336418:
{
kind_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000400;
break;
} // case 26336418
case 26989658:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00040000;
break;
} // case 26989658
case 28604882:
{
type_ = input.readStringRequireUtf8();
bitField1_ |= 0x00008000;
break;
} // case 28604882
case 29957474:
{
zone_ = input.readStringRequireUtf8();
bitField1_ |= 0x00020000;
break;
} // case 29957474
case 156928746:
{
sourceConsistencyGroupPolicy_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000001;
break;
} // case 156928746
case 177763082:
{
java.lang.String s = input.readStringRequireUtf8();
ensureResourcePoliciesIsMutable();
resourcePolicies_.add(s);
break;
} // case 177763082
case 244202930:
{
creationTimestamp_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 244202930
case 329243890:
{
accessMode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 329243890
case 337277226:
{
lastAttachTimestamp_ = input.readStringRequireUtf8();
bitField0_ |= 0x00002000;
break;
} // case 337277226
case 363861312:
{
long v = input.readInt64();
ensureLicenseCodesIsMutable();
licenseCodes_.addLong(v);
break;
} // case 363861312
case 363861314:
{
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensureLicenseCodesIsMutable();
while (input.getBytesUntilLimit() > 0) {
licenseCodes_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
} // case 363861314
case 387506178:
{
java.lang.String s = input.readStringRequireUtf8();
ensureReplicaZonesIsMutable();
replicaZones_.add(s);
break;
} // case 387506178
case 403546554:
{
sourceImage_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000010;
break;
} // case 403546554
case 442626330:
{
sourceImageId_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000040;
break;
} // case 442626330
case 451768218:
{
lastDetachTimestamp_ = input.readStringRequireUtf8();
bitField0_ |= 0x00004000;
break;
} // case 451768218
case 626510898:
{
input.readMessage(getParamsFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00100000;
break;
} // case 626510898
case 634356362:
{
com.google.cloud.compute.v1.GuestOsFeature m =
input.readMessage(
com.google.cloud.compute.v1.GuestOsFeature.parser(), extensionRegistry);
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(m);
} else {
guestOsFeaturesBuilder_.addMessage(m);
}
break;
} // case 634356362
case 791698066:
{
sourceSnapshotId_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000800;
break;
} // case 791698066
case 817081824:
{
enableConfidentialCompute_ = input.readBool();
bitField0_ |= 0x00000080;
break;
} // case 817081824
case 892629058:
{
java.lang.String s = input.readStringRequireUtf8();
ensureUsersIsMutable();
users_.add(s);
break;
} // case 892629058
case 1008495426:
{
sourceSnapshot_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000200;
break;
} // case 1008495426
case 1111570338:
{
region_ = input.readStringRequireUtf8();
bitField0_ |= 0x01000000;
break;
} // case 1111570338
case 1424998602:
{
labelFingerprint_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000800;
break;
} // case 1424998602
case 1444140266:
{
input.readMessage(
getAsyncPrimaryDiskFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 1444140266
case 1450082194:
{
status_ = input.readStringRequireUtf8();
bitField1_ |= 0x00002000;
break;
} // case 1450082194
case 1494152864:
{
provisionedIops_ = input.readInt64();
bitField0_ |= 0x00400000;
break;
} // case 1494152864
case 1753616434:
{
sourceInstantSnapshot_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000080;
break;
} // case 1753616434
case 1864421690:
{
sourceStorageObject_ = input.readStringRequireUtf8();
bitField1_ |= 0x00001000;
break;
} // case 1864421690
case 1995434522:
{
input.readMessage(getResourceStatusFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x08000000;
break;
} // case 1995434522
case 2140551658:
{
sourceConsistencyGroupPolicyId_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000002;
break;
} // case 2140551658
case -2121681878:
{
input.readMessage(
getDiskEncryptionKeyFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case -2121681878
case -1994305630:
{
sourceInstantSnapshotId_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000100;
break;
} // case -1994305630
case -1872541030:
{
architecture_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case -1872541030
case -1865532718:
{
input.readMessage(
getSourceSnapshotEncryptionKeyFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000400;
break;
} // case -1865532718
case -1711562430:
{
com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.compute.v1.DiskAsyncReplicationList>
asyncSecondaryDisks__ =
input.readMessage(
AsyncSecondaryDisksDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableAsyncSecondaryDisks()
.ensureBuilderMap()
.put(asyncSecondaryDisks__.getKey(), asyncSecondaryDisks__.getValue());
bitField0_ |= 0x00000008;
break;
} // case -1711562430
case -1593826670:
{
java.lang.String s = input.readStringRequireUtf8();
ensureLicensesIsMutable();
licenses_.add(s);
break;
} // case -1593826670
case -1490811254:
{
locationHint_ = input.readStringRequireUtf8();
bitField0_ |= 0x00020000;
break;
} // case -1490811254
case -1411179774:
{
storagePool_ = input.readStringRequireUtf8();
bitField1_ |= 0x00004000;
break;
} // case -1411179774
case -1405864718:
{
options_ = input.readStringRequireUtf8();
bitField0_ |= 0x00080000;
break;
} // case -1405864718
case -1242938022:
{
input.readMessage(
getSourceImageEncryptionKeyFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000020;
break;
} // case -1242938022
case -934903752:
{
physicalBlockSizeBytes_ = input.readInt64();
bitField0_ |= 0x00200000;
break;
} // case -934903752
case -911466526:
{
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case -911466526
case -680936950:
{
sourceDisk_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000004;
break;
} // case -680936950
case -661440822:
{
sourceDiskId_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000008;
break;
} // case -661440822
case -645248918:
{
selfLink_ = input.readStringRequireUtf8();
bitField0_ |= 0x40000000;
break;
} // case -645248918
case -447253240:
{
satisfiesPzi_ = input.readBool();
bitField0_ |= 0x10000000;
break;
} // case -447253240
case -447253160:
{
satisfiesPzs_ = input.readBool();
bitField0_ |= 0x20000000;
break;
} // case -447253160
case -335532344:
{
sizeGb_ = input.readInt64();
bitField0_ |= 0x80000000;
break;
} // case -335532344
case -293404678:
{
com.google.protobuf.MapEntry labels__ =
input.readMessage(
LabelsDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableLabels()
.getMutableMap()
.put(labels__.getKey(), labels__.getValue());
bitField0_ |= 0x00001000;
break;
} // case -293404678
case -82773848:
{
provisionedThroughput_ = input.readInt64();
bitField0_ |= 0x00800000;
break;
} // case -82773848
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int bitField1_;
private java.lang.Object accessMode_ = "";
/**
*
*
*
* The access mode of the disk. - READ_WRITE_SINGLE: The default AccessMode, means the disk can be attached to single instance in RW mode. - READ_WRITE_MANY: The AccessMode means the disk can be attached to multiple instances in RW mode. - READ_ONLY_MANY: The AccessMode means the disk can be attached to multiple instances in RO mode. The AccessMode is only valid for Hyperdisk disk types.
* Check the AccessMode enum for the list of possible values.
*
*
* optional string access_mode = 41155486;
*
* @return Whether the accessMode field is set.
*/
public boolean hasAccessMode() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The access mode of the disk. - READ_WRITE_SINGLE: The default AccessMode, means the disk can be attached to single instance in RW mode. - READ_WRITE_MANY: The AccessMode means the disk can be attached to multiple instances in RW mode. - READ_ONLY_MANY: The AccessMode means the disk can be attached to multiple instances in RO mode. The AccessMode is only valid for Hyperdisk disk types.
* Check the AccessMode enum for the list of possible values.
*
*
* optional string access_mode = 41155486;
*
* @return The accessMode.
*/
public java.lang.String getAccessMode() {
java.lang.Object ref = accessMode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accessMode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The access mode of the disk. - READ_WRITE_SINGLE: The default AccessMode, means the disk can be attached to single instance in RW mode. - READ_WRITE_MANY: The AccessMode means the disk can be attached to multiple instances in RW mode. - READ_ONLY_MANY: The AccessMode means the disk can be attached to multiple instances in RO mode. The AccessMode is only valid for Hyperdisk disk types.
* Check the AccessMode enum for the list of possible values.
*
*
* optional string access_mode = 41155486;
*
* @return The bytes for accessMode.
*/
public com.google.protobuf.ByteString getAccessModeBytes() {
java.lang.Object ref = accessMode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
accessMode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The access mode of the disk. - READ_WRITE_SINGLE: The default AccessMode, means the disk can be attached to single instance in RW mode. - READ_WRITE_MANY: The AccessMode means the disk can be attached to multiple instances in RW mode. - READ_ONLY_MANY: The AccessMode means the disk can be attached to multiple instances in RO mode. The AccessMode is only valid for Hyperdisk disk types.
* Check the AccessMode enum for the list of possible values.
*
*
* optional string access_mode = 41155486;
*
* @param value The accessMode to set.
* @return This builder for chaining.
*/
public Builder setAccessMode(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
accessMode_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The access mode of the disk. - READ_WRITE_SINGLE: The default AccessMode, means the disk can be attached to single instance in RW mode. - READ_WRITE_MANY: The AccessMode means the disk can be attached to multiple instances in RW mode. - READ_ONLY_MANY: The AccessMode means the disk can be attached to multiple instances in RO mode. The AccessMode is only valid for Hyperdisk disk types.
* Check the AccessMode enum for the list of possible values.
*
*
* optional string access_mode = 41155486;
*
* @return This builder for chaining.
*/
public Builder clearAccessMode() {
accessMode_ = getDefaultInstance().getAccessMode();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* The access mode of the disk. - READ_WRITE_SINGLE: The default AccessMode, means the disk can be attached to single instance in RW mode. - READ_WRITE_MANY: The AccessMode means the disk can be attached to multiple instances in RW mode. - READ_ONLY_MANY: The AccessMode means the disk can be attached to multiple instances in RO mode. The AccessMode is only valid for Hyperdisk disk types.
* Check the AccessMode enum for the list of possible values.
*
*
* optional string access_mode = 41155486;
*
* @param value The bytes for accessMode to set.
* @return This builder for chaining.
*/
public Builder setAccessModeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
accessMode_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object architecture_ = "";
/**
*
*
*
* The architecture of the disk. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return Whether the architecture field is set.
*/
public boolean hasArchitecture() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The architecture of the disk. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return The architecture.
*/
public java.lang.String getArchitecture() {
java.lang.Object ref = architecture_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
architecture_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The architecture of the disk. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return The bytes for architecture.
*/
public com.google.protobuf.ByteString getArchitectureBytes() {
java.lang.Object ref = architecture_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
architecture_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The architecture of the disk. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @param value The architecture to set.
* @return This builder for chaining.
*/
public Builder setArchitecture(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
architecture_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* The architecture of the disk. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return This builder for chaining.
*/
public Builder clearArchitecture() {
architecture_ = getDefaultInstance().getArchitecture();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* The architecture of the disk. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @param value The bytes for architecture to set.
* @return This builder for chaining.
*/
public Builder setArchitectureBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
architecture_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.cloud.compute.v1.DiskAsyncReplication asyncPrimaryDisk_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DiskAsyncReplication,
com.google.cloud.compute.v1.DiskAsyncReplication.Builder,
com.google.cloud.compute.v1.DiskAsyncReplicationOrBuilder>
asyncPrimaryDiskBuilder_;
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*
* @return Whether the asyncPrimaryDisk field is set.
*/
public boolean hasAsyncPrimaryDisk() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*
* @return The asyncPrimaryDisk.
*/
public com.google.cloud.compute.v1.DiskAsyncReplication getAsyncPrimaryDisk() {
if (asyncPrimaryDiskBuilder_ == null) {
return asyncPrimaryDisk_ == null
? com.google.cloud.compute.v1.DiskAsyncReplication.getDefaultInstance()
: asyncPrimaryDisk_;
} else {
return asyncPrimaryDiskBuilder_.getMessage();
}
}
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*/
public Builder setAsyncPrimaryDisk(com.google.cloud.compute.v1.DiskAsyncReplication value) {
if (asyncPrimaryDiskBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
asyncPrimaryDisk_ = value;
} else {
asyncPrimaryDiskBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*/
public Builder setAsyncPrimaryDisk(
com.google.cloud.compute.v1.DiskAsyncReplication.Builder builderForValue) {
if (asyncPrimaryDiskBuilder_ == null) {
asyncPrimaryDisk_ = builderForValue.build();
} else {
asyncPrimaryDiskBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*/
public Builder mergeAsyncPrimaryDisk(com.google.cloud.compute.v1.DiskAsyncReplication value) {
if (asyncPrimaryDiskBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)
&& asyncPrimaryDisk_ != null
&& asyncPrimaryDisk_
!= com.google.cloud.compute.v1.DiskAsyncReplication.getDefaultInstance()) {
getAsyncPrimaryDiskBuilder().mergeFrom(value);
} else {
asyncPrimaryDisk_ = value;
}
} else {
asyncPrimaryDiskBuilder_.mergeFrom(value);
}
if (asyncPrimaryDisk_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*/
public Builder clearAsyncPrimaryDisk() {
bitField0_ = (bitField0_ & ~0x00000004);
asyncPrimaryDisk_ = null;
if (asyncPrimaryDiskBuilder_ != null) {
asyncPrimaryDiskBuilder_.dispose();
asyncPrimaryDiskBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*/
public com.google.cloud.compute.v1.DiskAsyncReplication.Builder getAsyncPrimaryDiskBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getAsyncPrimaryDiskFieldBuilder().getBuilder();
}
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*/
public com.google.cloud.compute.v1.DiskAsyncReplicationOrBuilder
getAsyncPrimaryDiskOrBuilder() {
if (asyncPrimaryDiskBuilder_ != null) {
return asyncPrimaryDiskBuilder_.getMessageOrBuilder();
} else {
return asyncPrimaryDisk_ == null
? com.google.cloud.compute.v1.DiskAsyncReplication.getDefaultInstance()
: asyncPrimaryDisk_;
}
}
/**
*
*
*
* Disk asynchronously replicated into this disk.
*
*
* optional .google.cloud.compute.v1.DiskAsyncReplication async_primary_disk = 180517533;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DiskAsyncReplication,
com.google.cloud.compute.v1.DiskAsyncReplication.Builder,
com.google.cloud.compute.v1.DiskAsyncReplicationOrBuilder>
getAsyncPrimaryDiskFieldBuilder() {
if (asyncPrimaryDiskBuilder_ == null) {
asyncPrimaryDiskBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DiskAsyncReplication,
com.google.cloud.compute.v1.DiskAsyncReplication.Builder,
com.google.cloud.compute.v1.DiskAsyncReplicationOrBuilder>(
getAsyncPrimaryDisk(), getParentForChildren(), isClean());
asyncPrimaryDisk_ = null;
}
return asyncPrimaryDiskBuilder_;
}
private static final class AsyncSecondaryDisksConverter
implements com.google.protobuf.MapFieldBuilder.Converter<
java.lang.String,
com.google.cloud.compute.v1.DiskAsyncReplicationListOrBuilder,
com.google.cloud.compute.v1.DiskAsyncReplicationList> {
@java.lang.Override
public com.google.cloud.compute.v1.DiskAsyncReplicationList build(
com.google.cloud.compute.v1.DiskAsyncReplicationListOrBuilder val) {
if (val instanceof com.google.cloud.compute.v1.DiskAsyncReplicationList) {
return (com.google.cloud.compute.v1.DiskAsyncReplicationList) val;
}
return ((com.google.cloud.compute.v1.DiskAsyncReplicationList.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.compute.v1.DiskAsyncReplicationList>
defaultEntry() {
return AsyncSecondaryDisksDefaultEntryHolder.defaultEntry;
}
};
private static final AsyncSecondaryDisksConverter asyncSecondaryDisksConverter =
new AsyncSecondaryDisksConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.compute.v1.DiskAsyncReplicationListOrBuilder,
com.google.cloud.compute.v1.DiskAsyncReplicationList,
com.google.cloud.compute.v1.DiskAsyncReplicationList.Builder>
asyncSecondaryDisks_;
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.compute.v1.DiskAsyncReplicationListOrBuilder,
com.google.cloud.compute.v1.DiskAsyncReplicationList,
com.google.cloud.compute.v1.DiskAsyncReplicationList.Builder>
internalGetAsyncSecondaryDisks() {
if (asyncSecondaryDisks_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(asyncSecondaryDisksConverter);
}
return asyncSecondaryDisks_;
}
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.compute.v1.DiskAsyncReplicationListOrBuilder,
com.google.cloud.compute.v1.DiskAsyncReplicationList,
com.google.cloud.compute.v1.DiskAsyncReplicationList.Builder>
internalGetMutableAsyncSecondaryDisks() {
if (asyncSecondaryDisks_ == null) {
asyncSecondaryDisks_ =
new com.google.protobuf.MapFieldBuilder<>(asyncSecondaryDisksConverter);
}
bitField0_ |= 0x00000008;
onChanged();
return asyncSecondaryDisks_;
}
public int getAsyncSecondaryDisksCount() {
return internalGetAsyncSecondaryDisks().ensureBuilderMap().size();
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
@java.lang.Override
public boolean containsAsyncSecondaryDisks(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetAsyncSecondaryDisks().ensureBuilderMap().containsKey(key);
}
/** Use {@link #getAsyncSecondaryDisksMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getAsyncSecondaryDisks() {
return getAsyncSecondaryDisksMap();
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
@java.lang.Override
public java.util.Map
getAsyncSecondaryDisksMap() {
return internalGetAsyncSecondaryDisks().getImmutableMap();
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
@java.lang.Override
public /* nullable */ com.google.cloud.compute.v1.DiskAsyncReplicationList
getAsyncSecondaryDisksOrDefault(
java.lang.String key,
/* nullable */
com.google.cloud.compute.v1.DiskAsyncReplicationList defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetMutableAsyncSecondaryDisks().ensureBuilderMap();
return map.containsKey(key) ? asyncSecondaryDisksConverter.build(map.get(key)) : defaultValue;
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.DiskAsyncReplicationList getAsyncSecondaryDisksOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetMutableAsyncSecondaryDisks().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return asyncSecondaryDisksConverter.build(map.get(key));
}
public Builder clearAsyncSecondaryDisks() {
bitField0_ = (bitField0_ & ~0x00000008);
internalGetMutableAsyncSecondaryDisks().clear();
return this;
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
public Builder removeAsyncSecondaryDisks(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableAsyncSecondaryDisks().ensureBuilderMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map
getMutableAsyncSecondaryDisks() {
bitField0_ |= 0x00000008;
return internalGetMutableAsyncSecondaryDisks().ensureMessageMap();
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
public Builder putAsyncSecondaryDisks(
java.lang.String key, com.google.cloud.compute.v1.DiskAsyncReplicationList value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableAsyncSecondaryDisks().ensureBuilderMap().put(key, value);
bitField0_ |= 0x00000008;
return this;
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
public Builder putAllAsyncSecondaryDisks(
java.util.Map
values) {
for (java.util.Map.Entry<
java.lang.String, com.google.cloud.compute.v1.DiskAsyncReplicationList>
e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableAsyncSecondaryDisks().ensureBuilderMap().putAll(values);
bitField0_ |= 0x00000008;
return this;
}
/**
*
*
*
* [Output Only] A list of disks this disk is asynchronously replicated to.
*
*
*
* map<string, .google.cloud.compute.v1.DiskAsyncReplicationList> async_secondary_disks = 322925608;
*
*/
public com.google.cloud.compute.v1.DiskAsyncReplicationList.Builder
putAsyncSecondaryDisksBuilderIfAbsent(java.lang.String key) {
java.util.Map
builderMap = internalGetMutableAsyncSecondaryDisks().ensureBuilderMap();
com.google.cloud.compute.v1.DiskAsyncReplicationListOrBuilder entry = builderMap.get(key);
if (entry == null) {
entry = com.google.cloud.compute.v1.DiskAsyncReplicationList.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.cloud.compute.v1.DiskAsyncReplicationList) {
entry = ((com.google.cloud.compute.v1.DiskAsyncReplicationList) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.cloud.compute.v1.DiskAsyncReplicationList.Builder) entry;
}
private java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestamp(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
creationTimestamp_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return This builder for chaining.
*/
public Builder clearCreationTimestamp() {
creationTimestamp_ = getDefaultInstance().getCreationTimestamp();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The bytes for creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestampBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
creationTimestamp_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return Whether the description field is set.
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The bytes for description.
*/
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private com.google.cloud.compute.v1.CustomerEncryptionKey diskEncryptionKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
diskEncryptionKeyBuilder_;
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*
* @return Whether the diskEncryptionKey field is set.
*/
public boolean hasDiskEncryptionKey() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*
* @return The diskEncryptionKey.
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey getDiskEncryptionKey() {
if (diskEncryptionKeyBuilder_ == null) {
return diskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: diskEncryptionKey_;
} else {
return diskEncryptionKeyBuilder_.getMessage();
}
}
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public Builder setDiskEncryptionKey(com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (diskEncryptionKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
diskEncryptionKey_ = value;
} else {
diskEncryptionKeyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public Builder setDiskEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder builderForValue) {
if (diskEncryptionKeyBuilder_ == null) {
diskEncryptionKey_ = builderForValue.build();
} else {
diskEncryptionKeyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public Builder mergeDiskEncryptionKey(com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (diskEncryptionKeyBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)
&& diskEncryptionKey_ != null
&& diskEncryptionKey_
!= com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()) {
getDiskEncryptionKeyBuilder().mergeFrom(value);
} else {
diskEncryptionKey_ = value;
}
} else {
diskEncryptionKeyBuilder_.mergeFrom(value);
}
if (diskEncryptionKey_ != null) {
bitField0_ |= 0x00000040;
onChanged();
}
return this;
}
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public Builder clearDiskEncryptionKey() {
bitField0_ = (bitField0_ & ~0x00000040);
diskEncryptionKey_ = null;
if (diskEncryptionKeyBuilder_ != null) {
diskEncryptionKeyBuilder_.dispose();
diskEncryptionKeyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey.Builder getDiskEncryptionKeyBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getDiskEncryptionKeyFieldBuilder().getBuilder();
}
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getDiskEncryptionKeyOrBuilder() {
if (diskEncryptionKeyBuilder_ != null) {
return diskEncryptionKeyBuilder_.getMessageOrBuilder();
} else {
return diskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: diskEncryptionKey_;
}
}
/**
*
*
*
* Encrypts the disk using a customer-supplied encryption key or a customer-managed encryption key. Encryption keys do not protect access to metadata of the disk. After you encrypt a disk with a customer-supplied key, you must provide the same key if you use the disk later. For example, to create a disk snapshot, to create a disk image, to create a machine image, or to attach the disk to a virtual machine. After you encrypt a disk with a customer-managed key, the diskEncryptionKey.kmsKeyName is set to a key *version* name once the disk is created. The disk is encrypted with this version of the key. In the response, diskEncryptionKey.kmsKeyName appears in the following format: "diskEncryptionKey.kmsKeyName": "projects/kms_project_id/locations/region/keyRings/ key_region/cryptoKeys/key /cryptoKeysVersions/version If you do not provide an encryption key when creating the disk, then the disk is encrypted using an automatically generated key and you don't need to provide a key to use the disk later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
getDiskEncryptionKeyFieldBuilder() {
if (diskEncryptionKeyBuilder_ == null) {
diskEncryptionKeyBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>(
getDiskEncryptionKey(), getParentForChildren(), isClean());
diskEncryptionKey_ = null;
}
return diskEncryptionKeyBuilder_;
}
private boolean enableConfidentialCompute_;
/**
*
*
*
* Whether this disk is using confidential compute mode.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @return Whether the enableConfidentialCompute field is set.
*/
@java.lang.Override
public boolean hasEnableConfidentialCompute() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Whether this disk is using confidential compute mode.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @return The enableConfidentialCompute.
*/
@java.lang.Override
public boolean getEnableConfidentialCompute() {
return enableConfidentialCompute_;
}
/**
*
*
*
* Whether this disk is using confidential compute mode.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @param value The enableConfidentialCompute to set.
* @return This builder for chaining.
*/
public Builder setEnableConfidentialCompute(boolean value) {
enableConfidentialCompute_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Whether this disk is using confidential compute mode.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @return This builder for chaining.
*/
public Builder clearEnableConfidentialCompute() {
bitField0_ = (bitField0_ & ~0x00000080);
enableConfidentialCompute_ = false;
onChanged();
return this;
}
private java.util.List guestOsFeatures_ =
java.util.Collections.emptyList();
private void ensureGuestOsFeaturesIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
guestOsFeatures_ =
new java.util.ArrayList(guestOsFeatures_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.GuestOsFeature,
com.google.cloud.compute.v1.GuestOsFeature.Builder,
com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
guestOsFeaturesBuilder_;
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public java.util.List getGuestOsFeaturesList() {
if (guestOsFeaturesBuilder_ == null) {
return java.util.Collections.unmodifiableList(guestOsFeatures_);
} else {
return guestOsFeaturesBuilder_.getMessageList();
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public int getGuestOsFeaturesCount() {
if (guestOsFeaturesBuilder_ == null) {
return guestOsFeatures_.size();
} else {
return guestOsFeaturesBuilder_.getCount();
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature getGuestOsFeatures(int index) {
if (guestOsFeaturesBuilder_ == null) {
return guestOsFeatures_.get(index);
} else {
return guestOsFeaturesBuilder_.getMessage(index);
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder setGuestOsFeatures(int index, com.google.cloud.compute.v1.GuestOsFeature value) {
if (guestOsFeaturesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.set(index, value);
onChanged();
} else {
guestOsFeaturesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder setGuestOsFeatures(
int index, com.google.cloud.compute.v1.GuestOsFeature.Builder builderForValue) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.set(index, builderForValue.build());
onChanged();
} else {
guestOsFeaturesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(com.google.cloud.compute.v1.GuestOsFeature value) {
if (guestOsFeaturesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(value);
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(int index, com.google.cloud.compute.v1.GuestOsFeature value) {
if (guestOsFeaturesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(index, value);
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(
com.google.cloud.compute.v1.GuestOsFeature.Builder builderForValue) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(builderForValue.build());
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(
int index, com.google.cloud.compute.v1.GuestOsFeature.Builder builderForValue) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(index, builderForValue.build());
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addAllGuestOsFeatures(
java.lang.Iterable extends com.google.cloud.compute.v1.GuestOsFeature> values) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, guestOsFeatures_);
onChanged();
} else {
guestOsFeaturesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder clearGuestOsFeatures() {
if (guestOsFeaturesBuilder_ == null) {
guestOsFeatures_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
guestOsFeaturesBuilder_.clear();
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder removeGuestOsFeatures(int index) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.remove(index);
onChanged();
} else {
guestOsFeaturesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature.Builder getGuestOsFeaturesBuilder(int index) {
return getGuestOsFeaturesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeatureOrBuilder getGuestOsFeaturesOrBuilder(
int index) {
if (guestOsFeaturesBuilder_ == null) {
return guestOsFeatures_.get(index);
} else {
return guestOsFeaturesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public java.util.List extends com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
getGuestOsFeaturesOrBuilderList() {
if (guestOsFeaturesBuilder_ != null) {
return guestOsFeaturesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(guestOsFeatures_);
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature.Builder addGuestOsFeaturesBuilder() {
return getGuestOsFeaturesFieldBuilder()
.addBuilder(com.google.cloud.compute.v1.GuestOsFeature.getDefaultInstance());
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature.Builder addGuestOsFeaturesBuilder(int index) {
return getGuestOsFeaturesFieldBuilder()
.addBuilder(index, com.google.cloud.compute.v1.GuestOsFeature.getDefaultInstance());
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public java.util.List
getGuestOsFeaturesBuilderList() {
return getGuestOsFeaturesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.GuestOsFeature,
com.google.cloud.compute.v1.GuestOsFeature.Builder,
com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
getGuestOsFeaturesFieldBuilder() {
if (guestOsFeaturesBuilder_ == null) {
guestOsFeaturesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.GuestOsFeature,
com.google.cloud.compute.v1.GuestOsFeature.Builder,
com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>(
guestOsFeatures_,
((bitField0_ & 0x00000100) != 0),
getParentForChildren(),
isClean());
guestOsFeatures_ = null;
}
return guestOsFeaturesBuilder_;
}
private long id_;
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
id_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000200);
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#disk for disks.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
public boolean hasKind() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#disk for disks.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#disk for disks.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#disk for disks.
*
*
* optional string kind = 3292052;
*
* @param value The kind to set.
* @return This builder for chaining.
*/
public Builder setKind(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#disk for disks.
*
*
* optional string kind = 3292052;
*
* @return This builder for chaining.
*/
public Builder clearKind() {
kind_ = getDefaultInstance().getKind();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#disk for disks.
*
*
* optional string kind = 3292052;
*
* @param value The bytes for kind to set.
* @return This builder for chaining.
*/
public Builder setKindBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
kind_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
private java.lang.Object labelFingerprint_ = "";
/**
*
*
*
* A fingerprint for the labels being applied to this disk, which is essentially a hash of the labels set used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve a disk.
*
*
* optional string label_fingerprint = 178124825;
*
* @return Whether the labelFingerprint field is set.
*/
public boolean hasLabelFingerprint() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* A fingerprint for the labels being applied to this disk, which is essentially a hash of the labels set used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve a disk.
*
*
* optional string label_fingerprint = 178124825;
*
* @return The labelFingerprint.
*/
public java.lang.String getLabelFingerprint() {
java.lang.Object ref = labelFingerprint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
labelFingerprint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* A fingerprint for the labels being applied to this disk, which is essentially a hash of the labels set used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve a disk.
*
*
* optional string label_fingerprint = 178124825;
*
* @return The bytes for labelFingerprint.
*/
public com.google.protobuf.ByteString getLabelFingerprintBytes() {
java.lang.Object ref = labelFingerprint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
labelFingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* A fingerprint for the labels being applied to this disk, which is essentially a hash of the labels set used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve a disk.
*
*
* optional string label_fingerprint = 178124825;
*
* @param value The labelFingerprint to set.
* @return This builder for chaining.
*/
public Builder setLabelFingerprint(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
labelFingerprint_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* A fingerprint for the labels being applied to this disk, which is essentially a hash of the labels set used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve a disk.
*
*
* optional string label_fingerprint = 178124825;
*
* @return This builder for chaining.
*/
public Builder clearLabelFingerprint() {
labelFingerprint_ = getDefaultInstance().getLabelFingerprint();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
/**
*
*
*
* A fingerprint for the labels being applied to this disk, which is essentially a hash of the labels set used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve a disk.
*
*
* optional string label_fingerprint = 178124825;
*
* @param value The bytes for labelFingerprint to set.
* @return This builder for chaining.
*/
public Builder setLabelFingerprintBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
labelFingerprint_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
private com.google.protobuf.MapField labels_;
private com.google.protobuf.MapField internalGetLabels() {
if (labels_ == null) {
return com.google.protobuf.MapField.emptyMapField(LabelsDefaultEntryHolder.defaultEntry);
}
return labels_;
}
private com.google.protobuf.MapField
internalGetMutableLabels() {
if (labels_ == null) {
labels_ = com.google.protobuf.MapField.newMapField(LabelsDefaultEntryHolder.defaultEntry);
}
if (!labels_.isMutable()) {
labels_ = labels_.copy();
}
bitField0_ |= 0x00001000;
onChanged();
return labels_;
}
public int getLabelsCount() {
return internalGetLabels().getMap().size();
}
/**
*
*
*
* Labels to apply to this disk. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public boolean containsLabels(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetLabels().getMap().containsKey(key);
}
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getLabels() {
return getLabelsMap();
}
/**
*
*
*
* Labels to apply to this disk. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public java.util.Map getLabelsMap() {
return internalGetLabels().getMap();
}
/**
*
*
*
* Labels to apply to this disk. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public /* nullable */ java.lang.String getLabelsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Labels to apply to this disk. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public java.lang.String getLabelsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearLabels() {
bitField0_ = (bitField0_ & ~0x00001000);
internalGetMutableLabels().getMutableMap().clear();
return this;
}
/**
*
*
*
* Labels to apply to this disk. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
public Builder removeLabels(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableLabels().getMutableMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map getMutableLabels() {
bitField0_ |= 0x00001000;
return internalGetMutableLabels().getMutableMap();
}
/**
*
*
*
* Labels to apply to this disk. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
public Builder putLabels(java.lang.String key, java.lang.String value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableLabels().getMutableMap().put(key, value);
bitField0_ |= 0x00001000;
return this;
}
/**
*
*
*
* Labels to apply to this disk. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
public Builder putAllLabels(java.util.Map values) {
internalGetMutableLabels().getMutableMap().putAll(values);
bitField0_ |= 0x00001000;
return this;
}
private java.lang.Object lastAttachTimestamp_ = "";
/**
*
*
*
* [Output Only] Last attach timestamp in RFC3339 text format.
*
*
* optional string last_attach_timestamp = 42159653;
*
* @return Whether the lastAttachTimestamp field is set.
*/
public boolean hasLastAttachTimestamp() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*
*
* [Output Only] Last attach timestamp in RFC3339 text format.
*
*
* optional string last_attach_timestamp = 42159653;
*
* @return The lastAttachTimestamp.
*/
public java.lang.String getLastAttachTimestamp() {
java.lang.Object ref = lastAttachTimestamp_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
lastAttachTimestamp_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Last attach timestamp in RFC3339 text format.
*
*
* optional string last_attach_timestamp = 42159653;
*
* @return The bytes for lastAttachTimestamp.
*/
public com.google.protobuf.ByteString getLastAttachTimestampBytes() {
java.lang.Object ref = lastAttachTimestamp_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
lastAttachTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Last attach timestamp in RFC3339 text format.
*
*
* optional string last_attach_timestamp = 42159653;
*
* @param value The lastAttachTimestamp to set.
* @return This builder for chaining.
*/
public Builder setLastAttachTimestamp(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
lastAttachTimestamp_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Last attach timestamp in RFC3339 text format.
*
*
* optional string last_attach_timestamp = 42159653;
*
* @return This builder for chaining.
*/
public Builder clearLastAttachTimestamp() {
lastAttachTimestamp_ = getDefaultInstance().getLastAttachTimestamp();
bitField0_ = (bitField0_ & ~0x00002000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Last attach timestamp in RFC3339 text format.
*
*
* optional string last_attach_timestamp = 42159653;
*
* @param value The bytes for lastAttachTimestamp to set.
* @return This builder for chaining.
*/
public Builder setLastAttachTimestampBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
lastAttachTimestamp_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
private java.lang.Object lastDetachTimestamp_ = "";
/**
*
*
*
* [Output Only] Last detach timestamp in RFC3339 text format.
*
*
* optional string last_detach_timestamp = 56471027;
*
* @return Whether the lastDetachTimestamp field is set.
*/
public boolean hasLastDetachTimestamp() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*
*
* [Output Only] Last detach timestamp in RFC3339 text format.
*
*
* optional string last_detach_timestamp = 56471027;
*
* @return The lastDetachTimestamp.
*/
public java.lang.String getLastDetachTimestamp() {
java.lang.Object ref = lastDetachTimestamp_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
lastDetachTimestamp_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Last detach timestamp in RFC3339 text format.
*
*
* optional string last_detach_timestamp = 56471027;
*
* @return The bytes for lastDetachTimestamp.
*/
public com.google.protobuf.ByteString getLastDetachTimestampBytes() {
java.lang.Object ref = lastDetachTimestamp_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
lastDetachTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Last detach timestamp in RFC3339 text format.
*
*
* optional string last_detach_timestamp = 56471027;
*
* @param value The lastDetachTimestamp to set.
* @return This builder for chaining.
*/
public Builder setLastDetachTimestamp(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
lastDetachTimestamp_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Last detach timestamp in RFC3339 text format.
*
*
* optional string last_detach_timestamp = 56471027;
*
* @return This builder for chaining.
*/
public Builder clearLastDetachTimestamp() {
lastDetachTimestamp_ = getDefaultInstance().getLastDetachTimestamp();
bitField0_ = (bitField0_ & ~0x00004000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Last detach timestamp in RFC3339 text format.
*
*
* optional string last_detach_timestamp = 56471027;
*
* @param value The bytes for lastDetachTimestamp to set.
* @return This builder for chaining.
*/
public Builder setLastDetachTimestampBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
lastDetachTimestamp_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
private com.google.protobuf.Internal.LongList licenseCodes_ = emptyLongList();
private void ensureLicenseCodesIsMutable() {
if (!licenseCodes_.isModifiable()) {
licenseCodes_ = makeMutableCopy(licenseCodes_);
}
bitField0_ |= 0x00008000;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this disk.
*
*
* repeated int64 license_codes = 45482664;
*
* @return A list containing the licenseCodes.
*/
public java.util.List getLicenseCodesList() {
licenseCodes_.makeImmutable();
return licenseCodes_;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this disk.
*
*
* repeated int64 license_codes = 45482664;
*
* @return The count of licenseCodes.
*/
public int getLicenseCodesCount() {
return licenseCodes_.size();
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this disk.
*
*
* repeated int64 license_codes = 45482664;
*
* @param index The index of the element to return.
* @return The licenseCodes at the given index.
*/
public long getLicenseCodes(int index) {
return licenseCodes_.getLong(index);
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this disk.
*
*
* repeated int64 license_codes = 45482664;
*
* @param index The index to set the value at.
* @param value The licenseCodes to set.
* @return This builder for chaining.
*/
public Builder setLicenseCodes(int index, long value) {
ensureLicenseCodesIsMutable();
licenseCodes_.setLong(index, value);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this disk.
*
*
* repeated int64 license_codes = 45482664;
*
* @param value The licenseCodes to add.
* @return This builder for chaining.
*/
public Builder addLicenseCodes(long value) {
ensureLicenseCodesIsMutable();
licenseCodes_.addLong(value);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this disk.
*
*
* repeated int64 license_codes = 45482664;
*
* @param values The licenseCodes to add.
* @return This builder for chaining.
*/
public Builder addAllLicenseCodes(java.lang.Iterable extends java.lang.Long> values) {
ensureLicenseCodesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, licenseCodes_);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this disk.
*
*
* repeated int64 license_codes = 45482664;
*
* @return This builder for chaining.
*/
public Builder clearLicenseCodes() {
licenseCodes_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00008000);
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList licenses_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureLicensesIsMutable() {
if (!licenses_.isModifiable()) {
licenses_ = new com.google.protobuf.LazyStringArrayList(licenses_);
}
bitField0_ |= 0x00010000;
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @return A list containing the licenses.
*/
public com.google.protobuf.ProtocolStringList getLicensesList() {
licenses_.makeImmutable();
return licenses_;
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @return The count of licenses.
*/
public int getLicensesCount() {
return licenses_.size();
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the element to return.
* @return The licenses at the given index.
*/
public java.lang.String getLicenses(int index) {
return licenses_.get(index);
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the value to return.
* @return The bytes of the licenses at the given index.
*/
public com.google.protobuf.ByteString getLicensesBytes(int index) {
return licenses_.getByteString(index);
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @param index The index to set the value at.
* @param value The licenses to set.
* @return This builder for chaining.
*/
public Builder setLicenses(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLicensesIsMutable();
licenses_.set(index, value);
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @param value The licenses to add.
* @return This builder for chaining.
*/
public Builder addLicenses(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLicensesIsMutable();
licenses_.add(value);
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @param values The licenses to add.
* @return This builder for chaining.
*/
public Builder addAllLicenses(java.lang.Iterable values) {
ensureLicensesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, licenses_);
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @return This builder for chaining.
*/
public Builder clearLicenses() {
licenses_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00010000);
;
onChanged();
return this;
}
/**
*
*
*
* A list of publicly visible licenses. Reserved for Google's use.
*
*
* repeated string licenses = 337642578;
*
* @param value The bytes of the licenses to add.
* @return This builder for chaining.
*/
public Builder addLicensesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureLicensesIsMutable();
licenses_.add(value);
bitField0_ |= 0x00010000;
onChanged();
return this;
}
private java.lang.Object locationHint_ = "";
/**
*
*
*
* An opaque location hint used to place the disk close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return Whether the locationHint field is set.
*/
public boolean hasLocationHint() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
*
*
* An opaque location hint used to place the disk close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return The locationHint.
*/
public java.lang.String getLocationHint() {
java.lang.Object ref = locationHint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
locationHint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* An opaque location hint used to place the disk close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return The bytes for locationHint.
*/
public com.google.protobuf.ByteString getLocationHintBytes() {
java.lang.Object ref = locationHint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
locationHint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* An opaque location hint used to place the disk close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @param value The locationHint to set.
* @return This builder for chaining.
*/
public Builder setLocationHint(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
locationHint_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
*
*
* An opaque location hint used to place the disk close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return This builder for chaining.
*/
public Builder clearLocationHint() {
locationHint_ = getDefaultInstance().getLocationHint();
bitField0_ = (bitField0_ & ~0x00020000);
onChanged();
return this;
}
/**
*
*
*
* An opaque location hint used to place the disk close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @param value The bytes for locationHint to set.
* @return This builder for chaining.
*/
public Builder setLocationHintBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
locationHint_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00040000);
onChanged();
return this;
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
private java.lang.Object options_ = "";
/**
*
*
*
* Internal use only.
*
*
* optional string options = 361137822;
*
* @return Whether the options field is set.
*/
public boolean hasOptions() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
*
*
* Internal use only.
*
*
* optional string options = 361137822;
*
* @return The options.
*/
public java.lang.String getOptions() {
java.lang.Object ref = options_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
options_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Internal use only.
*
*
* optional string options = 361137822;
*
* @return The bytes for options.
*/
public com.google.protobuf.ByteString getOptionsBytes() {
java.lang.Object ref = options_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
options_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Internal use only.
*
*
* optional string options = 361137822;
*
* @param value The options to set.
* @return This builder for chaining.
*/
public Builder setOptions(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
options_ = value;
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
*
*
* Internal use only.
*
*
* optional string options = 361137822;
*
* @return This builder for chaining.
*/
public Builder clearOptions() {
options_ = getDefaultInstance().getOptions();
bitField0_ = (bitField0_ & ~0x00080000);
onChanged();
return this;
}
/**
*
*
*
* Internal use only.
*
*
* optional string options = 361137822;
*
* @param value The bytes for options to set.
* @return This builder for chaining.
*/
public Builder setOptionsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
options_ = value;
bitField0_ |= 0x00080000;
onChanged();
return this;
}
private com.google.cloud.compute.v1.DiskParams params_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DiskParams,
com.google.cloud.compute.v1.DiskParams.Builder,
com.google.cloud.compute.v1.DiskParamsOrBuilder>
paramsBuilder_;
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*
* @return Whether the params field is set.
*/
public boolean hasParams() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*
* @return The params.
*/
public com.google.cloud.compute.v1.DiskParams getParams() {
if (paramsBuilder_ == null) {
return params_ == null
? com.google.cloud.compute.v1.DiskParams.getDefaultInstance()
: params_;
} else {
return paramsBuilder_.getMessage();
}
}
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*/
public Builder setParams(com.google.cloud.compute.v1.DiskParams value) {
if (paramsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
params_ = value;
} else {
paramsBuilder_.setMessage(value);
}
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*/
public Builder setParams(com.google.cloud.compute.v1.DiskParams.Builder builderForValue) {
if (paramsBuilder_ == null) {
params_ = builderForValue.build();
} else {
paramsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*/
public Builder mergeParams(com.google.cloud.compute.v1.DiskParams value) {
if (paramsBuilder_ == null) {
if (((bitField0_ & 0x00100000) != 0)
&& params_ != null
&& params_ != com.google.cloud.compute.v1.DiskParams.getDefaultInstance()) {
getParamsBuilder().mergeFrom(value);
} else {
params_ = value;
}
} else {
paramsBuilder_.mergeFrom(value);
}
if (params_ != null) {
bitField0_ |= 0x00100000;
onChanged();
}
return this;
}
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*/
public Builder clearParams() {
bitField0_ = (bitField0_ & ~0x00100000);
params_ = null;
if (paramsBuilder_ != null) {
paramsBuilder_.dispose();
paramsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*/
public com.google.cloud.compute.v1.DiskParams.Builder getParamsBuilder() {
bitField0_ |= 0x00100000;
onChanged();
return getParamsFieldBuilder().getBuilder();
}
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*/
public com.google.cloud.compute.v1.DiskParamsOrBuilder getParamsOrBuilder() {
if (paramsBuilder_ != null) {
return paramsBuilder_.getMessageOrBuilder();
} else {
return params_ == null
? com.google.cloud.compute.v1.DiskParams.getDefaultInstance()
: params_;
}
}
/**
*
*
*
* Input only. [Input Only] Additional params passed with the request, but not persisted as part of resource payload.
*
*
* optional .google.cloud.compute.v1.DiskParams params = 78313862;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DiskParams,
com.google.cloud.compute.v1.DiskParams.Builder,
com.google.cloud.compute.v1.DiskParamsOrBuilder>
getParamsFieldBuilder() {
if (paramsBuilder_ == null) {
paramsBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DiskParams,
com.google.cloud.compute.v1.DiskParams.Builder,
com.google.cloud.compute.v1.DiskParamsOrBuilder>(
getParams(), getParentForChildren(), isClean());
params_ = null;
}
return paramsBuilder_;
}
private long physicalBlockSizeBytes_;
/**
*
*
*
* Physical block size of the persistent disk, in bytes. If not present in a request, a default value is used. The currently supported size is 4096, other sizes may be added in the future. If an unsupported value is requested, the error message will list the supported values for the caller's project.
*
*
* optional int64 physical_block_size_bytes = 420007943;
*
* @return Whether the physicalBlockSizeBytes field is set.
*/
@java.lang.Override
public boolean hasPhysicalBlockSizeBytes() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
*
*
*
* Physical block size of the persistent disk, in bytes. If not present in a request, a default value is used. The currently supported size is 4096, other sizes may be added in the future. If an unsupported value is requested, the error message will list the supported values for the caller's project.
*
*
* optional int64 physical_block_size_bytes = 420007943;
*
* @return The physicalBlockSizeBytes.
*/
@java.lang.Override
public long getPhysicalBlockSizeBytes() {
return physicalBlockSizeBytes_;
}
/**
*
*
*
* Physical block size of the persistent disk, in bytes. If not present in a request, a default value is used. The currently supported size is 4096, other sizes may be added in the future. If an unsupported value is requested, the error message will list the supported values for the caller's project.
*
*
* optional int64 physical_block_size_bytes = 420007943;
*
* @param value The physicalBlockSizeBytes to set.
* @return This builder for chaining.
*/
public Builder setPhysicalBlockSizeBytes(long value) {
physicalBlockSizeBytes_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
*
*
* Physical block size of the persistent disk, in bytes. If not present in a request, a default value is used. The currently supported size is 4096, other sizes may be added in the future. If an unsupported value is requested, the error message will list the supported values for the caller's project.
*
*
* optional int64 physical_block_size_bytes = 420007943;
*
* @return This builder for chaining.
*/
public Builder clearPhysicalBlockSizeBytes() {
bitField0_ = (bitField0_ & ~0x00200000);
physicalBlockSizeBytes_ = 0L;
onChanged();
return this;
}
private long provisionedIops_;
/**
*
*
*
* Indicates how many IOPS to provision for the disk. This sets the number of I/O operations per second that the disk can handle. Values must be between 10,000 and 120,000. For more details, see the Extreme persistent disk documentation.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @return Whether the provisionedIops field is set.
*/
@java.lang.Override
public boolean hasProvisionedIops() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
*
*
* Indicates how many IOPS to provision for the disk. This sets the number of I/O operations per second that the disk can handle. Values must be between 10,000 and 120,000. For more details, see the Extreme persistent disk documentation.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @return The provisionedIops.
*/
@java.lang.Override
public long getProvisionedIops() {
return provisionedIops_;
}
/**
*
*
*
* Indicates how many IOPS to provision for the disk. This sets the number of I/O operations per second that the disk can handle. Values must be between 10,000 and 120,000. For more details, see the Extreme persistent disk documentation.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @param value The provisionedIops to set.
* @return This builder for chaining.
*/
public Builder setProvisionedIops(long value) {
provisionedIops_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
*
*
* Indicates how many IOPS to provision for the disk. This sets the number of I/O operations per second that the disk can handle. Values must be between 10,000 and 120,000. For more details, see the Extreme persistent disk documentation.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @return This builder for chaining.
*/
public Builder clearProvisionedIops() {
bitField0_ = (bitField0_ & ~0x00400000);
provisionedIops_ = 0L;
onChanged();
return this;
}
private long provisionedThroughput_;
/**
*
*
*
* Indicates how much throughput to provision for the disk. This sets the number of throughput mb per second that the disk can handle. Values must be greater than or equal to 1.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @return Whether the provisionedThroughput field is set.
*/
@java.lang.Override
public boolean hasProvisionedThroughput() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
*
*
* Indicates how much throughput to provision for the disk. This sets the number of throughput mb per second that the disk can handle. Values must be greater than or equal to 1.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @return The provisionedThroughput.
*/
@java.lang.Override
public long getProvisionedThroughput() {
return provisionedThroughput_;
}
/**
*
*
*
* Indicates how much throughput to provision for the disk. This sets the number of throughput mb per second that the disk can handle. Values must be greater than or equal to 1.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @param value The provisionedThroughput to set.
* @return This builder for chaining.
*/
public Builder setProvisionedThroughput(long value) {
provisionedThroughput_ = value;
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
*
*
* Indicates how much throughput to provision for the disk. This sets the number of throughput mb per second that the disk can handle. Values must be greater than or equal to 1.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @return This builder for chaining.
*/
public Builder clearProvisionedThroughput() {
bitField0_ = (bitField0_ & ~0x00800000);
provisionedThroughput_ = 0L;
onChanged();
return this;
}
private java.lang.Object region_ = "";
/**
*
*
*
* [Output Only] URL of the region where the disk resides. Only applicable for regional resources. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return Whether the region field is set.
*/
public boolean hasRegion() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
*
*
*
* [Output Only] URL of the region where the disk resides. Only applicable for regional resources. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return The region.
*/
public java.lang.String getRegion() {
java.lang.Object ref = region_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
region_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] URL of the region where the disk resides. Only applicable for regional resources. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return The bytes for region.
*/
public com.google.protobuf.ByteString getRegionBytes() {
java.lang.Object ref = region_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
region_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] URL of the region where the disk resides. Only applicable for regional resources. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @param value The region to set.
* @return This builder for chaining.
*/
public Builder setRegion(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
region_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] URL of the region where the disk resides. Only applicable for regional resources. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return This builder for chaining.
*/
public Builder clearRegion() {
region_ = getDefaultInstance().getRegion();
bitField0_ = (bitField0_ & ~0x01000000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] URL of the region where the disk resides. Only applicable for regional resources. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @param value The bytes for region to set.
* @return This builder for chaining.
*/
public Builder setRegionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
region_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList replicaZones_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureReplicaZonesIsMutable() {
if (!replicaZones_.isModifiable()) {
replicaZones_ = new com.google.protobuf.LazyStringArrayList(replicaZones_);
}
bitField0_ |= 0x02000000;
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @return A list containing the replicaZones.
*/
public com.google.protobuf.ProtocolStringList getReplicaZonesList() {
replicaZones_.makeImmutable();
return replicaZones_;
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @return The count of replicaZones.
*/
public int getReplicaZonesCount() {
return replicaZones_.size();
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @param index The index of the element to return.
* @return The replicaZones at the given index.
*/
public java.lang.String getReplicaZones(int index) {
return replicaZones_.get(index);
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @param index The index of the value to return.
* @return The bytes of the replicaZones at the given index.
*/
public com.google.protobuf.ByteString getReplicaZonesBytes(int index) {
return replicaZones_.getByteString(index);
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @param index The index to set the value at.
* @param value The replicaZones to set.
* @return This builder for chaining.
*/
public Builder setReplicaZones(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureReplicaZonesIsMutable();
replicaZones_.set(index, value);
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @param value The replicaZones to add.
* @return This builder for chaining.
*/
public Builder addReplicaZones(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureReplicaZonesIsMutable();
replicaZones_.add(value);
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @param values The replicaZones to add.
* @return This builder for chaining.
*/
public Builder addAllReplicaZones(java.lang.Iterable values) {
ensureReplicaZonesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, replicaZones_);
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @return This builder for chaining.
*/
public Builder clearReplicaZones() {
replicaZones_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x02000000);
;
onChanged();
return this;
}
/**
*
*
*
* URLs of the zones where the disk should be replicated to. Only applicable for regional resources.
*
*
* repeated string replica_zones = 48438272;
*
* @param value The bytes of the replicaZones to add.
* @return This builder for chaining.
*/
public Builder addReplicaZonesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureReplicaZonesIsMutable();
replicaZones_.add(value);
bitField0_ |= 0x02000000;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList resourcePolicies_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureResourcePoliciesIsMutable() {
if (!resourcePolicies_.isModifiable()) {
resourcePolicies_ = new com.google.protobuf.LazyStringArrayList(resourcePolicies_);
}
bitField0_ |= 0x04000000;
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @return A list containing the resourcePolicies.
*/
public com.google.protobuf.ProtocolStringList getResourcePoliciesList() {
resourcePolicies_.makeImmutable();
return resourcePolicies_;
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @return The count of resourcePolicies.
*/
public int getResourcePoliciesCount() {
return resourcePolicies_.size();
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param index The index of the element to return.
* @return The resourcePolicies at the given index.
*/
public java.lang.String getResourcePolicies(int index) {
return resourcePolicies_.get(index);
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param index The index of the value to return.
* @return The bytes of the resourcePolicies at the given index.
*/
public com.google.protobuf.ByteString getResourcePoliciesBytes(int index) {
return resourcePolicies_.getByteString(index);
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param index The index to set the value at.
* @param value The resourcePolicies to set.
* @return This builder for chaining.
*/
public Builder setResourcePolicies(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureResourcePoliciesIsMutable();
resourcePolicies_.set(index, value);
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param value The resourcePolicies to add.
* @return This builder for chaining.
*/
public Builder addResourcePolicies(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureResourcePoliciesIsMutable();
resourcePolicies_.add(value);
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param values The resourcePolicies to add.
* @return This builder for chaining.
*/
public Builder addAllResourcePolicies(java.lang.Iterable values) {
ensureResourcePoliciesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, resourcePolicies_);
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @return This builder for chaining.
*/
public Builder clearResourcePolicies() {
resourcePolicies_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x04000000);
;
onChanged();
return this;
}
/**
*
*
*
* Resource policies applied to this disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param value The bytes of the resourcePolicies to add.
* @return This builder for chaining.
*/
public Builder addResourcePoliciesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureResourcePoliciesIsMutable();
resourcePolicies_.add(value);
bitField0_ |= 0x04000000;
onChanged();
return this;
}
private com.google.cloud.compute.v1.DiskResourceStatus resourceStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DiskResourceStatus,
com.google.cloud.compute.v1.DiskResourceStatus.Builder,
com.google.cloud.compute.v1.DiskResourceStatusOrBuilder>
resourceStatusBuilder_;
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*
*
* @return Whether the resourceStatus field is set.
*/
public boolean hasResourceStatus() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*
*
* @return The resourceStatus.
*/
public com.google.cloud.compute.v1.DiskResourceStatus getResourceStatus() {
if (resourceStatusBuilder_ == null) {
return resourceStatus_ == null
? com.google.cloud.compute.v1.DiskResourceStatus.getDefaultInstance()
: resourceStatus_;
} else {
return resourceStatusBuilder_.getMessage();
}
}
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*
*/
public Builder setResourceStatus(com.google.cloud.compute.v1.DiskResourceStatus value) {
if (resourceStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resourceStatus_ = value;
} else {
resourceStatusBuilder_.setMessage(value);
}
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*
*/
public Builder setResourceStatus(
com.google.cloud.compute.v1.DiskResourceStatus.Builder builderForValue) {
if (resourceStatusBuilder_ == null) {
resourceStatus_ = builderForValue.build();
} else {
resourceStatusBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*
*/
public Builder mergeResourceStatus(com.google.cloud.compute.v1.DiskResourceStatus value) {
if (resourceStatusBuilder_ == null) {
if (((bitField0_ & 0x08000000) != 0)
&& resourceStatus_ != null
&& resourceStatus_
!= com.google.cloud.compute.v1.DiskResourceStatus.getDefaultInstance()) {
getResourceStatusBuilder().mergeFrom(value);
} else {
resourceStatus_ = value;
}
} else {
resourceStatusBuilder_.mergeFrom(value);
}
if (resourceStatus_ != null) {
bitField0_ |= 0x08000000;
onChanged();
}
return this;
}
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*
*/
public Builder clearResourceStatus() {
bitField0_ = (bitField0_ & ~0x08000000);
resourceStatus_ = null;
if (resourceStatusBuilder_ != null) {
resourceStatusBuilder_.dispose();
resourceStatusBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*
*/
public com.google.cloud.compute.v1.DiskResourceStatus.Builder getResourceStatusBuilder() {
bitField0_ |= 0x08000000;
onChanged();
return getResourceStatusFieldBuilder().getBuilder();
}
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*
*/
public com.google.cloud.compute.v1.DiskResourceStatusOrBuilder getResourceStatusOrBuilder() {
if (resourceStatusBuilder_ != null) {
return resourceStatusBuilder_.getMessageOrBuilder();
} else {
return resourceStatus_ == null
? com.google.cloud.compute.v1.DiskResourceStatus.getDefaultInstance()
: resourceStatus_;
}
}
/**
*
*
*
* [Output Only] Status information for the disk resource.
*
*
* optional .google.cloud.compute.v1.DiskResourceStatus resource_status = 249429315;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DiskResourceStatus,
com.google.cloud.compute.v1.DiskResourceStatus.Builder,
com.google.cloud.compute.v1.DiskResourceStatusOrBuilder>
getResourceStatusFieldBuilder() {
if (resourceStatusBuilder_ == null) {
resourceStatusBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DiskResourceStatus,
com.google.cloud.compute.v1.DiskResourceStatus.Builder,
com.google.cloud.compute.v1.DiskResourceStatusOrBuilder>(
getResourceStatus(), getParentForChildren(), isClean());
resourceStatus_ = null;
}
return resourceStatusBuilder_;
}
private boolean satisfiesPzi_;
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @return Whether the satisfiesPzi field is set.
*/
@java.lang.Override
public boolean hasSatisfiesPzi() {
return ((bitField0_ & 0x10000000) != 0);
}
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @return The satisfiesPzi.
*/
@java.lang.Override
public boolean getSatisfiesPzi() {
return satisfiesPzi_;
}
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @param value The satisfiesPzi to set.
* @return This builder for chaining.
*/
public Builder setSatisfiesPzi(boolean value) {
satisfiesPzi_ = value;
bitField0_ |= 0x10000000;
onChanged();
return this;
}
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @return This builder for chaining.
*/
public Builder clearSatisfiesPzi() {
bitField0_ = (bitField0_ & ~0x10000000);
satisfiesPzi_ = false;
onChanged();
return this;
}
private boolean satisfiesPzs_;
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return Whether the satisfiesPzs field is set.
*/
@java.lang.Override
public boolean hasSatisfiesPzs() {
return ((bitField0_ & 0x20000000) != 0);
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return The satisfiesPzs.
*/
@java.lang.Override
public boolean getSatisfiesPzs() {
return satisfiesPzs_;
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @param value The satisfiesPzs to set.
* @return This builder for chaining.
*/
public Builder setSatisfiesPzs(boolean value) {
satisfiesPzs_ = value;
bitField0_ |= 0x20000000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return This builder for chaining.
*/
public Builder clearSatisfiesPzs() {
bitField0_ = (bitField0_ & ~0x20000000);
satisfiesPzs_ = false;
onChanged();
return this;
}
private java.lang.Object selfLink_ = "";
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return Whether the selfLink field is set.
*/
public boolean hasSelfLink() {
return ((bitField0_ & 0x40000000) != 0);
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return The selfLink.
*/
public java.lang.String getSelfLink() {
java.lang.Object ref = selfLink_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLink_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return The bytes for selfLink.
*/
public com.google.protobuf.ByteString getSelfLinkBytes() {
java.lang.Object ref = selfLink_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLink_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @param value The selfLink to set.
* @return This builder for chaining.
*/
public Builder setSelfLink(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
selfLink_ = value;
bitField0_ |= 0x40000000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return This builder for chaining.
*/
public Builder clearSelfLink() {
selfLink_ = getDefaultInstance().getSelfLink();
bitField0_ = (bitField0_ & ~0x40000000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @param value The bytes for selfLink to set.
* @return This builder for chaining.
*/
public Builder setSelfLinkBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
selfLink_ = value;
bitField0_ |= 0x40000000;
onChanged();
return this;
}
private long sizeGb_;
/**
*
*
*
* Size, in GB, of the persistent disk. You can specify this field when creating a persistent disk using the sourceImage, sourceSnapshot, or sourceDisk parameter, or specify it alone to create an empty persistent disk. If you specify this field along with a source, the value of sizeGb must not be less than the size of the source. Acceptable values are greater than 0.
*
*
* optional int64 size_gb = 494929369;
*
* @return Whether the sizeGb field is set.
*/
@java.lang.Override
public boolean hasSizeGb() {
return ((bitField0_ & 0x80000000) != 0);
}
/**
*
*
*
* Size, in GB, of the persistent disk. You can specify this field when creating a persistent disk using the sourceImage, sourceSnapshot, or sourceDisk parameter, or specify it alone to create an empty persistent disk. If you specify this field along with a source, the value of sizeGb must not be less than the size of the source. Acceptable values are greater than 0.
*
*
* optional int64 size_gb = 494929369;
*
* @return The sizeGb.
*/
@java.lang.Override
public long getSizeGb() {
return sizeGb_;
}
/**
*
*
*
* Size, in GB, of the persistent disk. You can specify this field when creating a persistent disk using the sourceImage, sourceSnapshot, or sourceDisk parameter, or specify it alone to create an empty persistent disk. If you specify this field along with a source, the value of sizeGb must not be less than the size of the source. Acceptable values are greater than 0.
*
*
* optional int64 size_gb = 494929369;
*
* @param value The sizeGb to set.
* @return This builder for chaining.
*/
public Builder setSizeGb(long value) {
sizeGb_ = value;
bitField0_ |= 0x80000000;
onChanged();
return this;
}
/**
*
*
*
* Size, in GB, of the persistent disk. You can specify this field when creating a persistent disk using the sourceImage, sourceSnapshot, or sourceDisk parameter, or specify it alone to create an empty persistent disk. If you specify this field along with a source, the value of sizeGb must not be less than the size of the source. Acceptable values are greater than 0.
*
*
* optional int64 size_gb = 494929369;
*
* @return This builder for chaining.
*/
public Builder clearSizeGb() {
bitField0_ = (bitField0_ & ~0x80000000);
sizeGb_ = 0L;
onChanged();
return this;
}
private java.lang.Object sourceConsistencyGroupPolicy_ = "";
/**
*
*
*
* [Output Only] URL of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy = 19616093;
*
* @return Whether the sourceConsistencyGroupPolicy field is set.
*/
public boolean hasSourceConsistencyGroupPolicy() {
return ((bitField1_ & 0x00000001) != 0);
}
/**
*
*
*
* [Output Only] URL of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy = 19616093;
*
* @return The sourceConsistencyGroupPolicy.
*/
public java.lang.String getSourceConsistencyGroupPolicy() {
java.lang.Object ref = sourceConsistencyGroupPolicy_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceConsistencyGroupPolicy_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] URL of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy = 19616093;
*
* @return The bytes for sourceConsistencyGroupPolicy.
*/
public com.google.protobuf.ByteString getSourceConsistencyGroupPolicyBytes() {
java.lang.Object ref = sourceConsistencyGroupPolicy_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceConsistencyGroupPolicy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] URL of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy = 19616093;
*
* @param value The sourceConsistencyGroupPolicy to set.
* @return This builder for chaining.
*/
public Builder setSourceConsistencyGroupPolicy(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceConsistencyGroupPolicy_ = value;
bitField1_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] URL of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy = 19616093;
*
* @return This builder for chaining.
*/
public Builder clearSourceConsistencyGroupPolicy() {
sourceConsistencyGroupPolicy_ = getDefaultInstance().getSourceConsistencyGroupPolicy();
bitField1_ = (bitField1_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] URL of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy = 19616093;
*
* @param value The bytes for sourceConsistencyGroupPolicy to set.
* @return This builder for chaining.
*/
public Builder setSourceConsistencyGroupPolicyBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceConsistencyGroupPolicy_ = value;
bitField1_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object sourceConsistencyGroupPolicyId_ = "";
/**
*
*
*
* [Output Only] ID of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy_id = 267568957;
*
* @return Whether the sourceConsistencyGroupPolicyId field is set.
*/
public boolean hasSourceConsistencyGroupPolicyId() {
return ((bitField1_ & 0x00000002) != 0);
}
/**
*
*
*
* [Output Only] ID of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy_id = 267568957;
*
* @return The sourceConsistencyGroupPolicyId.
*/
public java.lang.String getSourceConsistencyGroupPolicyId() {
java.lang.Object ref = sourceConsistencyGroupPolicyId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceConsistencyGroupPolicyId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] ID of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy_id = 267568957;
*
* @return The bytes for sourceConsistencyGroupPolicyId.
*/
public com.google.protobuf.ByteString getSourceConsistencyGroupPolicyIdBytes() {
java.lang.Object ref = sourceConsistencyGroupPolicyId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceConsistencyGroupPolicyId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] ID of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy_id = 267568957;
*
* @param value The sourceConsistencyGroupPolicyId to set.
* @return This builder for chaining.
*/
public Builder setSourceConsistencyGroupPolicyId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceConsistencyGroupPolicyId_ = value;
bitField1_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] ID of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy_id = 267568957;
*
* @return This builder for chaining.
*/
public Builder clearSourceConsistencyGroupPolicyId() {
sourceConsistencyGroupPolicyId_ = getDefaultInstance().getSourceConsistencyGroupPolicyId();
bitField1_ = (bitField1_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] ID of the DiskConsistencyGroupPolicy for a secondary disk that was created using a consistency group.
*
*
* optional string source_consistency_group_policy_id = 267568957;
*
* @param value The bytes for sourceConsistencyGroupPolicyId to set.
* @return This builder for chaining.
*/
public Builder setSourceConsistencyGroupPolicyIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceConsistencyGroupPolicyId_ = value;
bitField1_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object sourceDisk_ = "";
/**
*
*
*
* The source disk used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - https://www.googleapis.com/compute/v1/projects/project/regions/region /disks/disk - projects/project/zones/zone/disks/disk - projects/project/regions/region/disks/disk - zones/zone/disks/disk - regions/region/disks/disk
*
*
* optional string source_disk = 451753793;
*
* @return Whether the sourceDisk field is set.
*/
public boolean hasSourceDisk() {
return ((bitField1_ & 0x00000004) != 0);
}
/**
*
*
*
* The source disk used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - https://www.googleapis.com/compute/v1/projects/project/regions/region /disks/disk - projects/project/zones/zone/disks/disk - projects/project/regions/region/disks/disk - zones/zone/disks/disk - regions/region/disks/disk
*
*
* optional string source_disk = 451753793;
*
* @return The sourceDisk.
*/
public java.lang.String getSourceDisk() {
java.lang.Object ref = sourceDisk_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceDisk_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The source disk used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - https://www.googleapis.com/compute/v1/projects/project/regions/region /disks/disk - projects/project/zones/zone/disks/disk - projects/project/regions/region/disks/disk - zones/zone/disks/disk - regions/region/disks/disk
*
*
* optional string source_disk = 451753793;
*
* @return The bytes for sourceDisk.
*/
public com.google.protobuf.ByteString getSourceDiskBytes() {
java.lang.Object ref = sourceDisk_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceDisk_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The source disk used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - https://www.googleapis.com/compute/v1/projects/project/regions/region /disks/disk - projects/project/zones/zone/disks/disk - projects/project/regions/region/disks/disk - zones/zone/disks/disk - regions/region/disks/disk
*
*
* optional string source_disk = 451753793;
*
* @param value The sourceDisk to set.
* @return This builder for chaining.
*/
public Builder setSourceDisk(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceDisk_ = value;
bitField1_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* The source disk used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - https://www.googleapis.com/compute/v1/projects/project/regions/region /disks/disk - projects/project/zones/zone/disks/disk - projects/project/regions/region/disks/disk - zones/zone/disks/disk - regions/region/disks/disk
*
*
* optional string source_disk = 451753793;
*
* @return This builder for chaining.
*/
public Builder clearSourceDisk() {
sourceDisk_ = getDefaultInstance().getSourceDisk();
bitField1_ = (bitField1_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* The source disk used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - https://www.googleapis.com/compute/v1/projects/project/regions/region /disks/disk - projects/project/zones/zone/disks/disk - projects/project/regions/region/disks/disk - zones/zone/disks/disk - regions/region/disks/disk
*
*
* optional string source_disk = 451753793;
*
* @param value The bytes for sourceDisk to set.
* @return This builder for chaining.
*/
public Builder setSourceDiskBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceDisk_ = value;
bitField1_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object sourceDiskId_ = "";
/**
*
*
*
* [Output Only] The unique ID of the disk used to create this disk. This value identifies the exact disk that was used to create this persistent disk. For example, if you created the persistent disk from a disk that was later deleted and recreated under the same name, the source disk ID would identify the exact version of the disk that was used.
*
*
* optional string source_disk_id = 454190809;
*
* @return Whether the sourceDiskId field is set.
*/
public boolean hasSourceDiskId() {
return ((bitField1_ & 0x00000008) != 0);
}
/**
*
*
*
* [Output Only] The unique ID of the disk used to create this disk. This value identifies the exact disk that was used to create this persistent disk. For example, if you created the persistent disk from a disk that was later deleted and recreated under the same name, the source disk ID would identify the exact version of the disk that was used.
*
*
* optional string source_disk_id = 454190809;
*
* @return The sourceDiskId.
*/
public java.lang.String getSourceDiskId() {
java.lang.Object ref = sourceDiskId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceDiskId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The unique ID of the disk used to create this disk. This value identifies the exact disk that was used to create this persistent disk. For example, if you created the persistent disk from a disk that was later deleted and recreated under the same name, the source disk ID would identify the exact version of the disk that was used.
*
*
* optional string source_disk_id = 454190809;
*
* @return The bytes for sourceDiskId.
*/
public com.google.protobuf.ByteString getSourceDiskIdBytes() {
java.lang.Object ref = sourceDiskId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceDiskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The unique ID of the disk used to create this disk. This value identifies the exact disk that was used to create this persistent disk. For example, if you created the persistent disk from a disk that was later deleted and recreated under the same name, the source disk ID would identify the exact version of the disk that was used.
*
*
* optional string source_disk_id = 454190809;
*
* @param value The sourceDiskId to set.
* @return This builder for chaining.
*/
public Builder setSourceDiskId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceDiskId_ = value;
bitField1_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The unique ID of the disk used to create this disk. This value identifies the exact disk that was used to create this persistent disk. For example, if you created the persistent disk from a disk that was later deleted and recreated under the same name, the source disk ID would identify the exact version of the disk that was used.
*
*
* optional string source_disk_id = 454190809;
*
* @return This builder for chaining.
*/
public Builder clearSourceDiskId() {
sourceDiskId_ = getDefaultInstance().getSourceDiskId();
bitField1_ = (bitField1_ & ~0x00000008);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The unique ID of the disk used to create this disk. This value identifies the exact disk that was used to create this persistent disk. For example, if you created the persistent disk from a disk that was later deleted and recreated under the same name, the source disk ID would identify the exact version of the disk that was used.
*
*
* optional string source_disk_id = 454190809;
*
* @param value The bytes for sourceDiskId to set.
* @return This builder for chaining.
*/
public Builder setSourceDiskIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceDiskId_ = value;
bitField1_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object sourceImage_ = "";
/**
*
*
*
* The source image used to create this disk. If the source image is deleted, this field will not be set. To create a disk with one of the public operating system images, specify the image by its family name. For example, specify family/debian-9 to use the latest Debian 9 image: projects/debian-cloud/global/images/family/debian-9 Alternatively, use a specific version of a public operating system image: projects/debian-cloud/global/images/debian-9-stretch-vYYYYMMDD To create a disk with a custom image that you created, specify the image name in the following format: global/images/my-custom-image You can also specify a custom image by its image family, which returns the latest version of the image in that family. Replace the image name with family/family-name: global/images/family/my-image-family
*
*
* optional string source_image = 50443319;
*
* @return Whether the sourceImage field is set.
*/
public boolean hasSourceImage() {
return ((bitField1_ & 0x00000010) != 0);
}
/**
*
*
*
* The source image used to create this disk. If the source image is deleted, this field will not be set. To create a disk with one of the public operating system images, specify the image by its family name. For example, specify family/debian-9 to use the latest Debian 9 image: projects/debian-cloud/global/images/family/debian-9 Alternatively, use a specific version of a public operating system image: projects/debian-cloud/global/images/debian-9-stretch-vYYYYMMDD To create a disk with a custom image that you created, specify the image name in the following format: global/images/my-custom-image You can also specify a custom image by its image family, which returns the latest version of the image in that family. Replace the image name with family/family-name: global/images/family/my-image-family
*
*
* optional string source_image = 50443319;
*
* @return The sourceImage.
*/
public java.lang.String getSourceImage() {
java.lang.Object ref = sourceImage_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceImage_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The source image used to create this disk. If the source image is deleted, this field will not be set. To create a disk with one of the public operating system images, specify the image by its family name. For example, specify family/debian-9 to use the latest Debian 9 image: projects/debian-cloud/global/images/family/debian-9 Alternatively, use a specific version of a public operating system image: projects/debian-cloud/global/images/debian-9-stretch-vYYYYMMDD To create a disk with a custom image that you created, specify the image name in the following format: global/images/my-custom-image You can also specify a custom image by its image family, which returns the latest version of the image in that family. Replace the image name with family/family-name: global/images/family/my-image-family
*
*
* optional string source_image = 50443319;
*
* @return The bytes for sourceImage.
*/
public com.google.protobuf.ByteString getSourceImageBytes() {
java.lang.Object ref = sourceImage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceImage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The source image used to create this disk. If the source image is deleted, this field will not be set. To create a disk with one of the public operating system images, specify the image by its family name. For example, specify family/debian-9 to use the latest Debian 9 image: projects/debian-cloud/global/images/family/debian-9 Alternatively, use a specific version of a public operating system image: projects/debian-cloud/global/images/debian-9-stretch-vYYYYMMDD To create a disk with a custom image that you created, specify the image name in the following format: global/images/my-custom-image You can also specify a custom image by its image family, which returns the latest version of the image in that family. Replace the image name with family/family-name: global/images/family/my-image-family
*
*
* optional string source_image = 50443319;
*
* @param value The sourceImage to set.
* @return This builder for chaining.
*/
public Builder setSourceImage(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceImage_ = value;
bitField1_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* The source image used to create this disk. If the source image is deleted, this field will not be set. To create a disk with one of the public operating system images, specify the image by its family name. For example, specify family/debian-9 to use the latest Debian 9 image: projects/debian-cloud/global/images/family/debian-9 Alternatively, use a specific version of a public operating system image: projects/debian-cloud/global/images/debian-9-stretch-vYYYYMMDD To create a disk with a custom image that you created, specify the image name in the following format: global/images/my-custom-image You can also specify a custom image by its image family, which returns the latest version of the image in that family. Replace the image name with family/family-name: global/images/family/my-image-family
*
*
* optional string source_image = 50443319;
*
* @return This builder for chaining.
*/
public Builder clearSourceImage() {
sourceImage_ = getDefaultInstance().getSourceImage();
bitField1_ = (bitField1_ & ~0x00000010);
onChanged();
return this;
}
/**
*
*
*
* The source image used to create this disk. If the source image is deleted, this field will not be set. To create a disk with one of the public operating system images, specify the image by its family name. For example, specify family/debian-9 to use the latest Debian 9 image: projects/debian-cloud/global/images/family/debian-9 Alternatively, use a specific version of a public operating system image: projects/debian-cloud/global/images/debian-9-stretch-vYYYYMMDD To create a disk with a custom image that you created, specify the image name in the following format: global/images/my-custom-image You can also specify a custom image by its image family, which returns the latest version of the image in that family. Replace the image name with family/family-name: global/images/family/my-image-family
*
*
* optional string source_image = 50443319;
*
* @param value The bytes for sourceImage to set.
* @return This builder for chaining.
*/
public Builder setSourceImageBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceImage_ = value;
bitField1_ |= 0x00000010;
onChanged();
return this;
}
private com.google.cloud.compute.v1.CustomerEncryptionKey sourceImageEncryptionKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
sourceImageEncryptionKeyBuilder_;
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*
* @return Whether the sourceImageEncryptionKey field is set.
*/
public boolean hasSourceImageEncryptionKey() {
return ((bitField1_ & 0x00000020) != 0);
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*
* @return The sourceImageEncryptionKey.
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey getSourceImageEncryptionKey() {
if (sourceImageEncryptionKeyBuilder_ == null) {
return sourceImageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceImageEncryptionKey_;
} else {
return sourceImageEncryptionKeyBuilder_.getMessage();
}
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public Builder setSourceImageEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (sourceImageEncryptionKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sourceImageEncryptionKey_ = value;
} else {
sourceImageEncryptionKeyBuilder_.setMessage(value);
}
bitField1_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public Builder setSourceImageEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder builderForValue) {
if (sourceImageEncryptionKeyBuilder_ == null) {
sourceImageEncryptionKey_ = builderForValue.build();
} else {
sourceImageEncryptionKeyBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public Builder mergeSourceImageEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (sourceImageEncryptionKeyBuilder_ == null) {
if (((bitField1_ & 0x00000020) != 0)
&& sourceImageEncryptionKey_ != null
&& sourceImageEncryptionKey_
!= com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()) {
getSourceImageEncryptionKeyBuilder().mergeFrom(value);
} else {
sourceImageEncryptionKey_ = value;
}
} else {
sourceImageEncryptionKeyBuilder_.mergeFrom(value);
}
if (sourceImageEncryptionKey_ != null) {
bitField1_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public Builder clearSourceImageEncryptionKey() {
bitField1_ = (bitField1_ & ~0x00000020);
sourceImageEncryptionKey_ = null;
if (sourceImageEncryptionKeyBuilder_ != null) {
sourceImageEncryptionKeyBuilder_.dispose();
sourceImageEncryptionKeyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey.Builder
getSourceImageEncryptionKeyBuilder() {
bitField1_ |= 0x00000020;
onChanged();
return getSourceImageEncryptionKeyFieldBuilder().getBuilder();
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getSourceImageEncryptionKeyOrBuilder() {
if (sourceImageEncryptionKeyBuilder_ != null) {
return sourceImageEncryptionKeyBuilder_.getMessageOrBuilder();
} else {
return sourceImageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceImageEncryptionKey_;
}
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
getSourceImageEncryptionKeyFieldBuilder() {
if (sourceImageEncryptionKeyBuilder_ == null) {
sourceImageEncryptionKeyBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>(
getSourceImageEncryptionKey(), getParentForChildren(), isClean());
sourceImageEncryptionKey_ = null;
}
return sourceImageEncryptionKeyBuilder_;
}
private java.lang.Object sourceImageId_ = "";
/**
*
*
*
* [Output Only] The ID value of the image used to create this disk. This value identifies the exact image that was used to create this persistent disk. For example, if you created the persistent disk from an image that was later deleted and recreated under the same name, the source image ID would identify the exact version of the image that was used.
*
*
* optional string source_image_id = 55328291;
*
* @return Whether the sourceImageId field is set.
*/
public boolean hasSourceImageId() {
return ((bitField1_ & 0x00000040) != 0);
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this disk. This value identifies the exact image that was used to create this persistent disk. For example, if you created the persistent disk from an image that was later deleted and recreated under the same name, the source image ID would identify the exact version of the image that was used.
*
*
* optional string source_image_id = 55328291;
*
* @return The sourceImageId.
*/
public java.lang.String getSourceImageId() {
java.lang.Object ref = sourceImageId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceImageId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this disk. This value identifies the exact image that was used to create this persistent disk. For example, if you created the persistent disk from an image that was later deleted and recreated under the same name, the source image ID would identify the exact version of the image that was used.
*
*
* optional string source_image_id = 55328291;
*
* @return The bytes for sourceImageId.
*/
public com.google.protobuf.ByteString getSourceImageIdBytes() {
java.lang.Object ref = sourceImageId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceImageId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this disk. This value identifies the exact image that was used to create this persistent disk. For example, if you created the persistent disk from an image that was later deleted and recreated under the same name, the source image ID would identify the exact version of the image that was used.
*
*
* optional string source_image_id = 55328291;
*
* @param value The sourceImageId to set.
* @return This builder for chaining.
*/
public Builder setSourceImageId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceImageId_ = value;
bitField1_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this disk. This value identifies the exact image that was used to create this persistent disk. For example, if you created the persistent disk from an image that was later deleted and recreated under the same name, the source image ID would identify the exact version of the image that was used.
*
*
* optional string source_image_id = 55328291;
*
* @return This builder for chaining.
*/
public Builder clearSourceImageId() {
sourceImageId_ = getDefaultInstance().getSourceImageId();
bitField1_ = (bitField1_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this disk. This value identifies the exact image that was used to create this persistent disk. For example, if you created the persistent disk from an image that was later deleted and recreated under the same name, the source image ID would identify the exact version of the image that was used.
*
*
* optional string source_image_id = 55328291;
*
* @param value The bytes for sourceImageId to set.
* @return This builder for chaining.
*/
public Builder setSourceImageIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceImageId_ = value;
bitField1_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object sourceInstantSnapshot_ = "";
/**
*
*
*
* The source instant snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /instantSnapshots/instantSnapshot - projects/project/zones/zone/instantSnapshots/instantSnapshot - zones/zone/instantSnapshots/instantSnapshot
*
*
* optional string source_instant_snapshot = 219202054;
*
* @return Whether the sourceInstantSnapshot field is set.
*/
public boolean hasSourceInstantSnapshot() {
return ((bitField1_ & 0x00000080) != 0);
}
/**
*
*
*
* The source instant snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /instantSnapshots/instantSnapshot - projects/project/zones/zone/instantSnapshots/instantSnapshot - zones/zone/instantSnapshots/instantSnapshot
*
*
* optional string source_instant_snapshot = 219202054;
*
* @return The sourceInstantSnapshot.
*/
public java.lang.String getSourceInstantSnapshot() {
java.lang.Object ref = sourceInstantSnapshot_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceInstantSnapshot_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The source instant snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /instantSnapshots/instantSnapshot - projects/project/zones/zone/instantSnapshots/instantSnapshot - zones/zone/instantSnapshots/instantSnapshot
*
*
* optional string source_instant_snapshot = 219202054;
*
* @return The bytes for sourceInstantSnapshot.
*/
public com.google.protobuf.ByteString getSourceInstantSnapshotBytes() {
java.lang.Object ref = sourceInstantSnapshot_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceInstantSnapshot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The source instant snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /instantSnapshots/instantSnapshot - projects/project/zones/zone/instantSnapshots/instantSnapshot - zones/zone/instantSnapshots/instantSnapshot
*
*
* optional string source_instant_snapshot = 219202054;
*
* @param value The sourceInstantSnapshot to set.
* @return This builder for chaining.
*/
public Builder setSourceInstantSnapshot(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceInstantSnapshot_ = value;
bitField1_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* The source instant snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /instantSnapshots/instantSnapshot - projects/project/zones/zone/instantSnapshots/instantSnapshot - zones/zone/instantSnapshots/instantSnapshot
*
*
* optional string source_instant_snapshot = 219202054;
*
* @return This builder for chaining.
*/
public Builder clearSourceInstantSnapshot() {
sourceInstantSnapshot_ = getDefaultInstance().getSourceInstantSnapshot();
bitField1_ = (bitField1_ & ~0x00000080);
onChanged();
return this;
}
/**
*
*
*
* The source instant snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /instantSnapshots/instantSnapshot - projects/project/zones/zone/instantSnapshots/instantSnapshot - zones/zone/instantSnapshots/instantSnapshot
*
*
* optional string source_instant_snapshot = 219202054;
*
* @param value The bytes for sourceInstantSnapshot to set.
* @return This builder for chaining.
*/
public Builder setSourceInstantSnapshotBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceInstantSnapshot_ = value;
bitField1_ |= 0x00000080;
onChanged();
return this;
}
private java.lang.Object sourceInstantSnapshotId_ = "";
/**
*
*
*
* [Output Only] The unique ID of the instant snapshot used to create this disk. This value identifies the exact instant snapshot that was used to create this persistent disk. For example, if you created the persistent disk from an instant snapshot that was later deleted and recreated under the same name, the source instant snapshot ID would identify the exact version of the instant snapshot that was used.
*
*
* optional string source_instant_snapshot_id = 287582708;
*
* @return Whether the sourceInstantSnapshotId field is set.
*/
public boolean hasSourceInstantSnapshotId() {
return ((bitField1_ & 0x00000100) != 0);
}
/**
*
*
*
* [Output Only] The unique ID of the instant snapshot used to create this disk. This value identifies the exact instant snapshot that was used to create this persistent disk. For example, if you created the persistent disk from an instant snapshot that was later deleted and recreated under the same name, the source instant snapshot ID would identify the exact version of the instant snapshot that was used.
*
*
* optional string source_instant_snapshot_id = 287582708;
*
* @return The sourceInstantSnapshotId.
*/
public java.lang.String getSourceInstantSnapshotId() {
java.lang.Object ref = sourceInstantSnapshotId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceInstantSnapshotId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The unique ID of the instant snapshot used to create this disk. This value identifies the exact instant snapshot that was used to create this persistent disk. For example, if you created the persistent disk from an instant snapshot that was later deleted and recreated under the same name, the source instant snapshot ID would identify the exact version of the instant snapshot that was used.
*
*
* optional string source_instant_snapshot_id = 287582708;
*
* @return The bytes for sourceInstantSnapshotId.
*/
public com.google.protobuf.ByteString getSourceInstantSnapshotIdBytes() {
java.lang.Object ref = sourceInstantSnapshotId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceInstantSnapshotId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The unique ID of the instant snapshot used to create this disk. This value identifies the exact instant snapshot that was used to create this persistent disk. For example, if you created the persistent disk from an instant snapshot that was later deleted and recreated under the same name, the source instant snapshot ID would identify the exact version of the instant snapshot that was used.
*
*
* optional string source_instant_snapshot_id = 287582708;
*
* @param value The sourceInstantSnapshotId to set.
* @return This builder for chaining.
*/
public Builder setSourceInstantSnapshotId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceInstantSnapshotId_ = value;
bitField1_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The unique ID of the instant snapshot used to create this disk. This value identifies the exact instant snapshot that was used to create this persistent disk. For example, if you created the persistent disk from an instant snapshot that was later deleted and recreated under the same name, the source instant snapshot ID would identify the exact version of the instant snapshot that was used.
*
*
* optional string source_instant_snapshot_id = 287582708;
*
* @return This builder for chaining.
*/
public Builder clearSourceInstantSnapshotId() {
sourceInstantSnapshotId_ = getDefaultInstance().getSourceInstantSnapshotId();
bitField1_ = (bitField1_ & ~0x00000100);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The unique ID of the instant snapshot used to create this disk. This value identifies the exact instant snapshot that was used to create this persistent disk. For example, if you created the persistent disk from an instant snapshot that was later deleted and recreated under the same name, the source instant snapshot ID would identify the exact version of the instant snapshot that was used.
*
*
* optional string source_instant_snapshot_id = 287582708;
*
* @param value The bytes for sourceInstantSnapshotId to set.
* @return This builder for chaining.
*/
public Builder setSourceInstantSnapshotIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceInstantSnapshotId_ = value;
bitField1_ |= 0x00000100;
onChanged();
return this;
}
private java.lang.Object sourceSnapshot_ = "";
/**
*
*
*
* The source snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project /global/snapshots/snapshot - projects/project/global/snapshots/snapshot - global/snapshots/snapshot
*
*
* optional string source_snapshot = 126061928;
*
* @return Whether the sourceSnapshot field is set.
*/
public boolean hasSourceSnapshot() {
return ((bitField1_ & 0x00000200) != 0);
}
/**
*
*
*
* The source snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project /global/snapshots/snapshot - projects/project/global/snapshots/snapshot - global/snapshots/snapshot
*
*
* optional string source_snapshot = 126061928;
*
* @return The sourceSnapshot.
*/
public java.lang.String getSourceSnapshot() {
java.lang.Object ref = sourceSnapshot_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceSnapshot_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The source snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project /global/snapshots/snapshot - projects/project/global/snapshots/snapshot - global/snapshots/snapshot
*
*
* optional string source_snapshot = 126061928;
*
* @return The bytes for sourceSnapshot.
*/
public com.google.protobuf.ByteString getSourceSnapshotBytes() {
java.lang.Object ref = sourceSnapshot_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceSnapshot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The source snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project /global/snapshots/snapshot - projects/project/global/snapshots/snapshot - global/snapshots/snapshot
*
*
* optional string source_snapshot = 126061928;
*
* @param value The sourceSnapshot to set.
* @return This builder for chaining.
*/
public Builder setSourceSnapshot(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceSnapshot_ = value;
bitField1_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* The source snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project /global/snapshots/snapshot - projects/project/global/snapshots/snapshot - global/snapshots/snapshot
*
*
* optional string source_snapshot = 126061928;
*
* @return This builder for chaining.
*/
public Builder clearSourceSnapshot() {
sourceSnapshot_ = getDefaultInstance().getSourceSnapshot();
bitField1_ = (bitField1_ & ~0x00000200);
onChanged();
return this;
}
/**
*
*
*
* The source snapshot used to create this disk. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project /global/snapshots/snapshot - projects/project/global/snapshots/snapshot - global/snapshots/snapshot
*
*
* optional string source_snapshot = 126061928;
*
* @param value The bytes for sourceSnapshot to set.
* @return This builder for chaining.
*/
public Builder setSourceSnapshotBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceSnapshot_ = value;
bitField1_ |= 0x00000200;
onChanged();
return this;
}
private com.google.cloud.compute.v1.CustomerEncryptionKey sourceSnapshotEncryptionKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
sourceSnapshotEncryptionKeyBuilder_;
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*
* @return Whether the sourceSnapshotEncryptionKey field is set.
*/
public boolean hasSourceSnapshotEncryptionKey() {
return ((bitField1_ & 0x00000400) != 0);
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*
* @return The sourceSnapshotEncryptionKey.
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey getSourceSnapshotEncryptionKey() {
if (sourceSnapshotEncryptionKeyBuilder_ == null) {
return sourceSnapshotEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceSnapshotEncryptionKey_;
} else {
return sourceSnapshotEncryptionKeyBuilder_.getMessage();
}
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public Builder setSourceSnapshotEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (sourceSnapshotEncryptionKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sourceSnapshotEncryptionKey_ = value;
} else {
sourceSnapshotEncryptionKeyBuilder_.setMessage(value);
}
bitField1_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public Builder setSourceSnapshotEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder builderForValue) {
if (sourceSnapshotEncryptionKeyBuilder_ == null) {
sourceSnapshotEncryptionKey_ = builderForValue.build();
} else {
sourceSnapshotEncryptionKeyBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public Builder mergeSourceSnapshotEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (sourceSnapshotEncryptionKeyBuilder_ == null) {
if (((bitField1_ & 0x00000400) != 0)
&& sourceSnapshotEncryptionKey_ != null
&& sourceSnapshotEncryptionKey_
!= com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()) {
getSourceSnapshotEncryptionKeyBuilder().mergeFrom(value);
} else {
sourceSnapshotEncryptionKey_ = value;
}
} else {
sourceSnapshotEncryptionKeyBuilder_.mergeFrom(value);
}
if (sourceSnapshotEncryptionKey_ != null) {
bitField1_ |= 0x00000400;
onChanged();
}
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public Builder clearSourceSnapshotEncryptionKey() {
bitField1_ = (bitField1_ & ~0x00000400);
sourceSnapshotEncryptionKey_ = null;
if (sourceSnapshotEncryptionKeyBuilder_ != null) {
sourceSnapshotEncryptionKeyBuilder_.dispose();
sourceSnapshotEncryptionKeyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey.Builder
getSourceSnapshotEncryptionKeyBuilder() {
bitField1_ |= 0x00000400;
onChanged();
return getSourceSnapshotEncryptionKeyFieldBuilder().getBuilder();
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getSourceSnapshotEncryptionKeyOrBuilder() {
if (sourceSnapshotEncryptionKeyBuilder_ != null) {
return sourceSnapshotEncryptionKeyBuilder_.getMessageOrBuilder();
} else {
return sourceSnapshotEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceSnapshotEncryptionKey_;
}
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
getSourceSnapshotEncryptionKeyFieldBuilder() {
if (sourceSnapshotEncryptionKeyBuilder_ == null) {
sourceSnapshotEncryptionKeyBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>(
getSourceSnapshotEncryptionKey(), getParentForChildren(), isClean());
sourceSnapshotEncryptionKey_ = null;
}
return sourceSnapshotEncryptionKeyBuilder_;
}
private java.lang.Object sourceSnapshotId_ = "";
/**
*
*
*
* [Output Only] The unique ID of the snapshot used to create this disk. This value identifies the exact snapshot that was used to create this persistent disk. For example, if you created the persistent disk from a snapshot that was later deleted and recreated under the same name, the source snapshot ID would identify the exact version of the snapshot that was used.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return Whether the sourceSnapshotId field is set.
*/
public boolean hasSourceSnapshotId() {
return ((bitField1_ & 0x00000800) != 0);
}
/**
*
*
*
* [Output Only] The unique ID of the snapshot used to create this disk. This value identifies the exact snapshot that was used to create this persistent disk. For example, if you created the persistent disk from a snapshot that was later deleted and recreated under the same name, the source snapshot ID would identify the exact version of the snapshot that was used.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return The sourceSnapshotId.
*/
public java.lang.String getSourceSnapshotId() {
java.lang.Object ref = sourceSnapshotId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceSnapshotId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The unique ID of the snapshot used to create this disk. This value identifies the exact snapshot that was used to create this persistent disk. For example, if you created the persistent disk from a snapshot that was later deleted and recreated under the same name, the source snapshot ID would identify the exact version of the snapshot that was used.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return The bytes for sourceSnapshotId.
*/
public com.google.protobuf.ByteString getSourceSnapshotIdBytes() {
java.lang.Object ref = sourceSnapshotId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceSnapshotId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The unique ID of the snapshot used to create this disk. This value identifies the exact snapshot that was used to create this persistent disk. For example, if you created the persistent disk from a snapshot that was later deleted and recreated under the same name, the source snapshot ID would identify the exact version of the snapshot that was used.
*
*
* optional string source_snapshot_id = 98962258;
*
* @param value The sourceSnapshotId to set.
* @return This builder for chaining.
*/
public Builder setSourceSnapshotId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceSnapshotId_ = value;
bitField1_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The unique ID of the snapshot used to create this disk. This value identifies the exact snapshot that was used to create this persistent disk. For example, if you created the persistent disk from a snapshot that was later deleted and recreated under the same name, the source snapshot ID would identify the exact version of the snapshot that was used.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return This builder for chaining.
*/
public Builder clearSourceSnapshotId() {
sourceSnapshotId_ = getDefaultInstance().getSourceSnapshotId();
bitField1_ = (bitField1_ & ~0x00000800);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The unique ID of the snapshot used to create this disk. This value identifies the exact snapshot that was used to create this persistent disk. For example, if you created the persistent disk from a snapshot that was later deleted and recreated under the same name, the source snapshot ID would identify the exact version of the snapshot that was used.
*
*
* optional string source_snapshot_id = 98962258;
*
* @param value The bytes for sourceSnapshotId to set.
* @return This builder for chaining.
*/
public Builder setSourceSnapshotIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceSnapshotId_ = value;
bitField1_ |= 0x00000800;
onChanged();
return this;
}
private java.lang.Object sourceStorageObject_ = "";
/**
*
*
*
* The full Google Cloud Storage URI where the disk image is stored. This file must be a gzip-compressed tarball whose name ends in .tar.gz or virtual machine disk whose name ends in vmdk. Valid URIs may start with gs:// or https://storage.googleapis.com/. This flag is not optimized for creating multiple disks from a source storage object. To create many disks from a source storage object, use gcloud compute images import instead.
*
*
* optional string source_storage_object = 233052711;
*
* @return Whether the sourceStorageObject field is set.
*/
public boolean hasSourceStorageObject() {
return ((bitField1_ & 0x00001000) != 0);
}
/**
*
*
*
* The full Google Cloud Storage URI where the disk image is stored. This file must be a gzip-compressed tarball whose name ends in .tar.gz or virtual machine disk whose name ends in vmdk. Valid URIs may start with gs:// or https://storage.googleapis.com/. This flag is not optimized for creating multiple disks from a source storage object. To create many disks from a source storage object, use gcloud compute images import instead.
*
*
* optional string source_storage_object = 233052711;
*
* @return The sourceStorageObject.
*/
public java.lang.String getSourceStorageObject() {
java.lang.Object ref = sourceStorageObject_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceStorageObject_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The full Google Cloud Storage URI where the disk image is stored. This file must be a gzip-compressed tarball whose name ends in .tar.gz or virtual machine disk whose name ends in vmdk. Valid URIs may start with gs:// or https://storage.googleapis.com/. This flag is not optimized for creating multiple disks from a source storage object. To create many disks from a source storage object, use gcloud compute images import instead.
*
*
* optional string source_storage_object = 233052711;
*
* @return The bytes for sourceStorageObject.
*/
public com.google.protobuf.ByteString getSourceStorageObjectBytes() {
java.lang.Object ref = sourceStorageObject_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceStorageObject_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The full Google Cloud Storage URI where the disk image is stored. This file must be a gzip-compressed tarball whose name ends in .tar.gz or virtual machine disk whose name ends in vmdk. Valid URIs may start with gs:// or https://storage.googleapis.com/. This flag is not optimized for creating multiple disks from a source storage object. To create many disks from a source storage object, use gcloud compute images import instead.
*
*
* optional string source_storage_object = 233052711;
*
* @param value The sourceStorageObject to set.
* @return This builder for chaining.
*/
public Builder setSourceStorageObject(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceStorageObject_ = value;
bitField1_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* The full Google Cloud Storage URI where the disk image is stored. This file must be a gzip-compressed tarball whose name ends in .tar.gz or virtual machine disk whose name ends in vmdk. Valid URIs may start with gs:// or https://storage.googleapis.com/. This flag is not optimized for creating multiple disks from a source storage object. To create many disks from a source storage object, use gcloud compute images import instead.
*
*
* optional string source_storage_object = 233052711;
*
* @return This builder for chaining.
*/
public Builder clearSourceStorageObject() {
sourceStorageObject_ = getDefaultInstance().getSourceStorageObject();
bitField1_ = (bitField1_ & ~0x00001000);
onChanged();
return this;
}
/**
*
*
*
* The full Google Cloud Storage URI where the disk image is stored. This file must be a gzip-compressed tarball whose name ends in .tar.gz or virtual machine disk whose name ends in vmdk. Valid URIs may start with gs:// or https://storage.googleapis.com/. This flag is not optimized for creating multiple disks from a source storage object. To create many disks from a source storage object, use gcloud compute images import instead.
*
*
* optional string source_storage_object = 233052711;
*
* @param value The bytes for sourceStorageObject to set.
* @return This builder for chaining.
*/
public Builder setSourceStorageObjectBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceStorageObject_ = value;
bitField1_ |= 0x00001000;
onChanged();
return this;
}
private java.lang.Object status_ = "";
/**
*
*
*
* [Output Only] The status of disk creation. - CREATING: Disk is provisioning. - RESTORING: Source data is being copied into the disk. - FAILED: Disk creation failed. - READY: Disk is ready for use. - DELETING: Disk is deleting.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return ((bitField1_ & 0x00002000) != 0);
}
/**
*
*
*
* [Output Only] The status of disk creation. - CREATING: Disk is provisioning. - RESTORING: Source data is being copied into the disk. - FAILED: Disk creation failed. - READY: Disk is ready for use. - DELETING: Disk is deleting.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The status.
*/
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
status_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The status of disk creation. - CREATING: Disk is provisioning. - RESTORING: Source data is being copied into the disk. - FAILED: Disk creation failed. - READY: Disk is ready for use. - DELETING: Disk is deleting.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The bytes for status.
*/
public com.google.protobuf.ByteString getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The status of disk creation. - CREATING: Disk is provisioning. - RESTORING: Source data is being copied into the disk. - FAILED: Disk creation failed. - READY: Disk is ready for use. - DELETING: Disk is deleting.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
bitField1_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The status of disk creation. - CREATING: Disk is provisioning. - RESTORING: Source data is being copied into the disk. - FAILED: Disk creation failed. - READY: Disk is ready for use. - DELETING: Disk is deleting.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = getDefaultInstance().getStatus();
bitField1_ = (bitField1_ & ~0x00002000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The status of disk creation. - CREATING: Disk is provisioning. - RESTORING: Source data is being copied into the disk. - FAILED: Disk creation failed. - READY: Disk is ready for use. - DELETING: Disk is deleting.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @param value The bytes for status to set.
* @return This builder for chaining.
*/
public Builder setStatusBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
status_ = value;
bitField1_ |= 0x00002000;
onChanged();
return this;
}
private java.lang.Object storagePool_ = "";
/**
*
*
*
* The storage pool in which the new disk is created. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /storagePools/storagePool - projects/project/zones/zone/storagePools/storagePool - zones/zone/storagePools/storagePool
*
*
* optional string storage_pool = 360473440;
*
* @return Whether the storagePool field is set.
*/
public boolean hasStoragePool() {
return ((bitField1_ & 0x00004000) != 0);
}
/**
*
*
*
* The storage pool in which the new disk is created. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /storagePools/storagePool - projects/project/zones/zone/storagePools/storagePool - zones/zone/storagePools/storagePool
*
*
* optional string storage_pool = 360473440;
*
* @return The storagePool.
*/
public java.lang.String getStoragePool() {
java.lang.Object ref = storagePool_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
storagePool_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The storage pool in which the new disk is created. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /storagePools/storagePool - projects/project/zones/zone/storagePools/storagePool - zones/zone/storagePools/storagePool
*
*
* optional string storage_pool = 360473440;
*
* @return The bytes for storagePool.
*/
public com.google.protobuf.ByteString getStoragePoolBytes() {
java.lang.Object ref = storagePool_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
storagePool_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The storage pool in which the new disk is created. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /storagePools/storagePool - projects/project/zones/zone/storagePools/storagePool - zones/zone/storagePools/storagePool
*
*
* optional string storage_pool = 360473440;
*
* @param value The storagePool to set.
* @return This builder for chaining.
*/
public Builder setStoragePool(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
storagePool_ = value;
bitField1_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* The storage pool in which the new disk is created. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /storagePools/storagePool - projects/project/zones/zone/storagePools/storagePool - zones/zone/storagePools/storagePool
*
*
* optional string storage_pool = 360473440;
*
* @return This builder for chaining.
*/
public Builder clearStoragePool() {
storagePool_ = getDefaultInstance().getStoragePool();
bitField1_ = (bitField1_ & ~0x00004000);
onChanged();
return this;
}
/**
*
*
*
* The storage pool in which the new disk is created. You can provide this as a partial or full URL to the resource. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /storagePools/storagePool - projects/project/zones/zone/storagePools/storagePool - zones/zone/storagePools/storagePool
*
*
* optional string storage_pool = 360473440;
*
* @param value The bytes for storagePool to set.
* @return This builder for chaining.
*/
public Builder setStoragePoolBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
storagePool_ = value;
bitField1_ |= 0x00004000;
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
*
*
*
* URL of the disk type resource describing which disk type to use to create the disk. Provide this when creating the disk. For example: projects/project /zones/zone/diskTypes/pd-ssd . See Persistent disk types.
*
*
* optional string type = 3575610;
*
* @return Whether the type field is set.
*/
public boolean hasType() {
return ((bitField1_ & 0x00008000) != 0);
}
/**
*
*
*
* URL of the disk type resource describing which disk type to use to create the disk. Provide this when creating the disk. For example: projects/project /zones/zone/diskTypes/pd-ssd . See Persistent disk types.
*
*
* optional string type = 3575610;
*
* @return The type.
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* URL of the disk type resource describing which disk type to use to create the disk. Provide this when creating the disk. For example: projects/project /zones/zone/diskTypes/pd-ssd . See Persistent disk types.
*
*
* optional string type = 3575610;
*
* @return The bytes for type.
*/
public com.google.protobuf.ByteString getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* URL of the disk type resource describing which disk type to use to create the disk. Provide this when creating the disk. For example: projects/project /zones/zone/diskTypes/pd-ssd . See Persistent disk types.
*
*
* optional string type = 3575610;
*
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
bitField1_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* URL of the disk type resource describing which disk type to use to create the disk. Provide this when creating the disk. For example: projects/project /zones/zone/diskTypes/pd-ssd . See Persistent disk types.
*
*
* optional string type = 3575610;
*
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = getDefaultInstance().getType();
bitField1_ = (bitField1_ & ~0x00008000);
onChanged();
return this;
}
/**
*
*
*
* URL of the disk type resource describing which disk type to use to create the disk. Provide this when creating the disk. For example: projects/project /zones/zone/diskTypes/pd-ssd . See Persistent disk types.
*
*
* optional string type = 3575610;
*
* @param value The bytes for type to set.
* @return This builder for chaining.
*/
public Builder setTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
type_ = value;
bitField1_ |= 0x00008000;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList users_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureUsersIsMutable() {
if (!users_.isModifiable()) {
users_ = new com.google.protobuf.LazyStringArrayList(users_);
}
bitField1_ |= 0x00010000;
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @return A list containing the users.
*/
public com.google.protobuf.ProtocolStringList getUsersList() {
users_.makeImmutable();
return users_;
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @return The count of users.
*/
public int getUsersCount() {
return users_.size();
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @param index The index of the element to return.
* @return The users at the given index.
*/
public java.lang.String getUsers(int index) {
return users_.get(index);
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @param index The index of the value to return.
* @return The bytes of the users at the given index.
*/
public com.google.protobuf.ByteString getUsersBytes(int index) {
return users_.getByteString(index);
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @param index The index to set the value at.
* @param value The users to set.
* @return This builder for chaining.
*/
public Builder setUsers(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureUsersIsMutable();
users_.set(index, value);
bitField1_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @param value The users to add.
* @return This builder for chaining.
*/
public Builder addUsers(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureUsersIsMutable();
users_.add(value);
bitField1_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @param values The users to add.
* @return This builder for chaining.
*/
public Builder addAllUsers(java.lang.Iterable values) {
ensureUsersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, users_);
bitField1_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @return This builder for chaining.
*/
public Builder clearUsers() {
users_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField1_ = (bitField1_ & ~0x00010000);
;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Links to the users of the disk (attached instances) in form: projects/project/zones/zone/instances/instance
*
*
* repeated string users = 111578632;
*
* @param value The bytes of the users to add.
* @return This builder for chaining.
*/
public Builder addUsersBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureUsersIsMutable();
users_.add(value);
bitField1_ |= 0x00010000;
onChanged();
return this;
}
private java.lang.Object zone_ = "";
/**
*
*
*
* [Output Only] URL of the zone where the disk resides. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string zone = 3744684;
*
* @return Whether the zone field is set.
*/
public boolean hasZone() {
return ((bitField1_ & 0x00020000) != 0);
}
/**
*
*
*
* [Output Only] URL of the zone where the disk resides. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string zone = 3744684;
*
* @return The zone.
*/
public java.lang.String getZone() {
java.lang.Object ref = zone_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
zone_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] URL of the zone where the disk resides. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string zone = 3744684;
*
* @return The bytes for zone.
*/
public com.google.protobuf.ByteString getZoneBytes() {
java.lang.Object ref = zone_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
zone_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] URL of the zone where the disk resides. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string zone = 3744684;
*
* @param value The zone to set.
* @return This builder for chaining.
*/
public Builder setZone(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
zone_ = value;
bitField1_ |= 0x00020000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] URL of the zone where the disk resides. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string zone = 3744684;
*
* @return This builder for chaining.
*/
public Builder clearZone() {
zone_ = getDefaultInstance().getZone();
bitField1_ = (bitField1_ & ~0x00020000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] URL of the zone where the disk resides. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string zone = 3744684;
*
* @param value The bytes for zone to set.
* @return This builder for chaining.
*/
public Builder setZoneBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
zone_ = value;
bitField1_ |= 0x00020000;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.Disk)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.Disk)
private static final com.google.cloud.compute.v1.Disk DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.Disk();
}
public static com.google.cloud.compute.v1.Disk getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Disk parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.Disk getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy