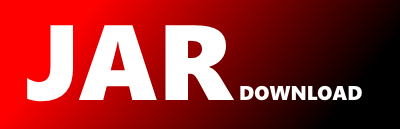
com.google.cloud.compute.v1.HTTPHealthCheck Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
*
*
* Protobuf type {@code google.cloud.compute.v1.HTTPHealthCheck}
*/
public final class HTTPHealthCheck extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.HTTPHealthCheck)
HTTPHealthCheckOrBuilder {
private static final long serialVersionUID = 0L;
// Use HTTPHealthCheck.newBuilder() to construct.
private HTTPHealthCheck(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HTTPHealthCheck() {
host_ = "";
portName_ = "";
portSpecification_ = "";
proxyHeader_ = "";
requestPath_ = "";
response_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new HTTPHealthCheck();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_HTTPHealthCheck_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_HTTPHealthCheck_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.HTTPHealthCheck.class,
com.google.cloud.compute.v1.HTTPHealthCheck.Builder.class);
}
/**
*
*
*
* Specifies how a port is selected for health checking. Can be one of the following values: USE_FIXED_PORT: Specifies a port number explicitly using the port field in the health check. Supported by backend services for passthrough load balancers and backend services for proxy load balancers. Also supported in legacy HTTP health checks for target pools. The health check supports all backends supported by the backend service provided the backend can be health checked. For example, GCE_VM_IP network endpoint groups, GCE_VM_IP_PORT network endpoint groups, and instance group backends. USE_NAMED_PORT: Not supported. USE_SERVING_PORT: Provides an indirect method of specifying the health check port by referring to the backend service. Only supported by backend services for proxy load balancers. Not supported by target pools. Not supported by backend services for pass-through load balancers. Supports all backends that can be health checked; for example, GCE_VM_IP_PORT network endpoint groups and instance group backends. For GCE_VM_IP_PORT network endpoint group backends, the health check uses the port number specified for each endpoint in the network endpoint group. For instance group backends, the health check uses the port number determined by looking up the backend service's named port in the instance group's list of named ports.
*
*
* Protobuf enum {@code google.cloud.compute.v1.HTTPHealthCheck.PortSpecification}
*/
public enum PortSpecification implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_PORT_SPECIFICATION = 0;
*/
UNDEFINED_PORT_SPECIFICATION(0),
/**
*
*
*
* The port number in the health check's port is used for health checking. Applies to network endpoint group and instance group backends.
*
*
* USE_FIXED_PORT = 190235748;
*/
USE_FIXED_PORT(190235748),
/**
*
*
*
* Not supported.
*
*
* USE_NAMED_PORT = 349300671;
*/
USE_NAMED_PORT(349300671),
/**
*
*
*
* For network endpoint group backends, the health check uses the port number specified on each endpoint in the network endpoint group. For instance group backends, the health check uses the port number specified for the backend service's named port defined in the instance group's named ports.
*
*
* USE_SERVING_PORT = 362637516;
*/
USE_SERVING_PORT(362637516),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_PORT_SPECIFICATION = 0;
*/
public static final int UNDEFINED_PORT_SPECIFICATION_VALUE = 0;
/**
*
*
*
* The port number in the health check's port is used for health checking. Applies to network endpoint group and instance group backends.
*
*
* USE_FIXED_PORT = 190235748;
*/
public static final int USE_FIXED_PORT_VALUE = 190235748;
/**
*
*
*
* Not supported.
*
*
* USE_NAMED_PORT = 349300671;
*/
public static final int USE_NAMED_PORT_VALUE = 349300671;
/**
*
*
*
* For network endpoint group backends, the health check uses the port number specified on each endpoint in the network endpoint group. For instance group backends, the health check uses the port number specified for the backend service's named port defined in the instance group's named ports.
*
*
* USE_SERVING_PORT = 362637516;
*/
public static final int USE_SERVING_PORT_VALUE = 362637516;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PortSpecification valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static PortSpecification forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_PORT_SPECIFICATION;
case 190235748:
return USE_FIXED_PORT;
case 349300671:
return USE_NAMED_PORT;
case 362637516:
return USE_SERVING_PORT;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public PortSpecification findValueByNumber(int number) {
return PortSpecification.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.HTTPHealthCheck.getDescriptor().getEnumTypes().get(0);
}
private static final PortSpecification[] VALUES = values();
public static PortSpecification valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private PortSpecification(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.HTTPHealthCheck.PortSpecification)
}
/**
*
*
*
* Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
*
*
* Protobuf enum {@code google.cloud.compute.v1.HTTPHealthCheck.ProxyHeader}
*/
public enum ProxyHeader implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_PROXY_HEADER = 0;
*/
UNDEFINED_PROXY_HEADER(0),
/** NONE = 2402104;
*/
NONE(2402104),
/** PROXY_V1 = 334352940;
*/
PROXY_V1(334352940),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_PROXY_HEADER = 0;
*/
public static final int UNDEFINED_PROXY_HEADER_VALUE = 0;
/** NONE = 2402104;
*/
public static final int NONE_VALUE = 2402104;
/** PROXY_V1 = 334352940;
*/
public static final int PROXY_V1_VALUE = 334352940;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProxyHeader valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ProxyHeader forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_PROXY_HEADER;
case 2402104:
return NONE;
case 334352940:
return PROXY_V1;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ProxyHeader findValueByNumber(int number) {
return ProxyHeader.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.HTTPHealthCheck.getDescriptor().getEnumTypes().get(1);
}
private static final ProxyHeader[] VALUES = values();
public static ProxyHeader valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ProxyHeader(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.HTTPHealthCheck.ProxyHeader)
}
private int bitField0_;
public static final int HOST_FIELD_NUMBER = 3208616;
@SuppressWarnings("serial")
private volatile java.lang.Object host_ = "";
/**
*
*
*
* The value of the host header in the HTTP health check request. If left empty (default value), the host header is set to the destination IP address to which health check packets are sent. The destination IP address depends on the type of load balancer. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#hc-packet-dest
*
*
* optional string host = 3208616;
*
* @return Whether the host field is set.
*/
@java.lang.Override
public boolean hasHost() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The value of the host header in the HTTP health check request. If left empty (default value), the host header is set to the destination IP address to which health check packets are sent. The destination IP address depends on the type of load balancer. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#hc-packet-dest
*
*
* optional string host = 3208616;
*
* @return The host.
*/
@java.lang.Override
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
host_ = s;
return s;
}
}
/**
*
*
*
* The value of the host header in the HTTP health check request. If left empty (default value), the host header is set to the destination IP address to which health check packets are sent. The destination IP address depends on the type of load balancer. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#hc-packet-dest
*
*
* optional string host = 3208616;
*
* @return The bytes for host.
*/
@java.lang.Override
public com.google.protobuf.ByteString getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PORT_FIELD_NUMBER = 3446913;
private int port_ = 0;
/**
*
*
*
* The TCP port number to which the health check prober sends packets. The default value is 80. Valid values are 1 through 65535.
*
*
* optional int32 port = 3446913;
*
* @return Whether the port field is set.
*/
@java.lang.Override
public boolean hasPort() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The TCP port number to which the health check prober sends packets. The default value is 80. Valid values are 1 through 65535.
*
*
* optional int32 port = 3446913;
*
* @return The port.
*/
@java.lang.Override
public int getPort() {
return port_;
}
public static final int PORT_NAME_FIELD_NUMBER = 41534345;
@SuppressWarnings("serial")
private volatile java.lang.Object portName_ = "";
/**
*
*
*
* Not supported.
*
*
* optional string port_name = 41534345;
*
* @return Whether the portName field is set.
*/
@java.lang.Override
public boolean hasPortName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Not supported.
*
*
* optional string port_name = 41534345;
*
* @return The portName.
*/
@java.lang.Override
public java.lang.String getPortName() {
java.lang.Object ref = portName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portName_ = s;
return s;
}
}
/**
*
*
*
* Not supported.
*
*
* optional string port_name = 41534345;
*
* @return The bytes for portName.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPortNameBytes() {
java.lang.Object ref = portName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
portName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PORT_SPECIFICATION_FIELD_NUMBER = 51590597;
@SuppressWarnings("serial")
private volatile java.lang.Object portSpecification_ = "";
/**
*
*
*
* Specifies how a port is selected for health checking. Can be one of the following values: USE_FIXED_PORT: Specifies a port number explicitly using the port field in the health check. Supported by backend services for passthrough load balancers and backend services for proxy load balancers. Also supported in legacy HTTP health checks for target pools. The health check supports all backends supported by the backend service provided the backend can be health checked. For example, GCE_VM_IP network endpoint groups, GCE_VM_IP_PORT network endpoint groups, and instance group backends. USE_NAMED_PORT: Not supported. USE_SERVING_PORT: Provides an indirect method of specifying the health check port by referring to the backend service. Only supported by backend services for proxy load balancers. Not supported by target pools. Not supported by backend services for pass-through load balancers. Supports all backends that can be health checked; for example, GCE_VM_IP_PORT network endpoint groups and instance group backends. For GCE_VM_IP_PORT network endpoint group backends, the health check uses the port number specified for each endpoint in the network endpoint group. For instance group backends, the health check uses the port number determined by looking up the backend service's named port in the instance group's list of named ports.
* Check the PortSpecification enum for the list of possible values.
*
*
* optional string port_specification = 51590597;
*
* @return Whether the portSpecification field is set.
*/
@java.lang.Override
public boolean hasPortSpecification() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Specifies how a port is selected for health checking. Can be one of the following values: USE_FIXED_PORT: Specifies a port number explicitly using the port field in the health check. Supported by backend services for passthrough load balancers and backend services for proxy load balancers. Also supported in legacy HTTP health checks for target pools. The health check supports all backends supported by the backend service provided the backend can be health checked. For example, GCE_VM_IP network endpoint groups, GCE_VM_IP_PORT network endpoint groups, and instance group backends. USE_NAMED_PORT: Not supported. USE_SERVING_PORT: Provides an indirect method of specifying the health check port by referring to the backend service. Only supported by backend services for proxy load balancers. Not supported by target pools. Not supported by backend services for pass-through load balancers. Supports all backends that can be health checked; for example, GCE_VM_IP_PORT network endpoint groups and instance group backends. For GCE_VM_IP_PORT network endpoint group backends, the health check uses the port number specified for each endpoint in the network endpoint group. For instance group backends, the health check uses the port number determined by looking up the backend service's named port in the instance group's list of named ports.
* Check the PortSpecification enum for the list of possible values.
*
*
* optional string port_specification = 51590597;
*
* @return The portSpecification.
*/
@java.lang.Override
public java.lang.String getPortSpecification() {
java.lang.Object ref = portSpecification_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portSpecification_ = s;
return s;
}
}
/**
*
*
*
* Specifies how a port is selected for health checking. Can be one of the following values: USE_FIXED_PORT: Specifies a port number explicitly using the port field in the health check. Supported by backend services for passthrough load balancers and backend services for proxy load balancers. Also supported in legacy HTTP health checks for target pools. The health check supports all backends supported by the backend service provided the backend can be health checked. For example, GCE_VM_IP network endpoint groups, GCE_VM_IP_PORT network endpoint groups, and instance group backends. USE_NAMED_PORT: Not supported. USE_SERVING_PORT: Provides an indirect method of specifying the health check port by referring to the backend service. Only supported by backend services for proxy load balancers. Not supported by target pools. Not supported by backend services for pass-through load balancers. Supports all backends that can be health checked; for example, GCE_VM_IP_PORT network endpoint groups and instance group backends. For GCE_VM_IP_PORT network endpoint group backends, the health check uses the port number specified for each endpoint in the network endpoint group. For instance group backends, the health check uses the port number determined by looking up the backend service's named port in the instance group's list of named ports.
* Check the PortSpecification enum for the list of possible values.
*
*
* optional string port_specification = 51590597;
*
* @return The bytes for portSpecification.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPortSpecificationBytes() {
java.lang.Object ref = portSpecification_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
portSpecification_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROXY_HEADER_FIELD_NUMBER = 160374142;
@SuppressWarnings("serial")
private volatile java.lang.Object proxyHeader_ = "";
/**
*
*
*
* Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
* Check the ProxyHeader enum for the list of possible values.
*
*
* optional string proxy_header = 160374142;
*
* @return Whether the proxyHeader field is set.
*/
@java.lang.Override
public boolean hasProxyHeader() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
* Check the ProxyHeader enum for the list of possible values.
*
*
* optional string proxy_header = 160374142;
*
* @return The proxyHeader.
*/
@java.lang.Override
public java.lang.String getProxyHeader() {
java.lang.Object ref = proxyHeader_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
proxyHeader_ = s;
return s;
}
}
/**
*
*
*
* Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
* Check the ProxyHeader enum for the list of possible values.
*
*
* optional string proxy_header = 160374142;
*
* @return The bytes for proxyHeader.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProxyHeaderBytes() {
java.lang.Object ref = proxyHeader_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
proxyHeader_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQUEST_PATH_FIELD_NUMBER = 229403605;
@SuppressWarnings("serial")
private volatile java.lang.Object requestPath_ = "";
/**
*
*
*
* The request path of the HTTP health check request. The default value is /. Must comply with RFC3986.
*
*
* optional string request_path = 229403605;
*
* @return Whether the requestPath field is set.
*/
@java.lang.Override
public boolean hasRequestPath() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* The request path of the HTTP health check request. The default value is /. Must comply with RFC3986.
*
*
* optional string request_path = 229403605;
*
* @return The requestPath.
*/
@java.lang.Override
public java.lang.String getRequestPath() {
java.lang.Object ref = requestPath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestPath_ = s;
return s;
}
}
/**
*
*
*
* The request path of the HTTP health check request. The default value is /. Must comply with RFC3986.
*
*
* optional string request_path = 229403605;
*
* @return The bytes for requestPath.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRequestPathBytes() {
java.lang.Object ref = requestPath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
requestPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RESPONSE_FIELD_NUMBER = 196547649;
@SuppressWarnings("serial")
private volatile java.lang.Object response_ = "";
/**
*
*
*
* Creates a content-based HTTP health check. In addition to the required HTTP 200 (OK) status code, you can configure the health check to pass only when the backend sends this specific ASCII response string within the first 1024 bytes of the HTTP response body. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#criteria-protocol-http
*
*
* optional string response = 196547649;
*
* @return Whether the response field is set.
*/
@java.lang.Override
public boolean hasResponse() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Creates a content-based HTTP health check. In addition to the required HTTP 200 (OK) status code, you can configure the health check to pass only when the backend sends this specific ASCII response string within the first 1024 bytes of the HTTP response body. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#criteria-protocol-http
*
*
* optional string response = 196547649;
*
* @return The response.
*/
@java.lang.Override
public java.lang.String getResponse() {
java.lang.Object ref = response_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
response_ = s;
return s;
}
}
/**
*
*
*
* Creates a content-based HTTP health check. In addition to the required HTTP 200 (OK) status code, you can configure the health check to pass only when the backend sends this specific ASCII response string within the first 1024 bytes of the HTTP response body. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#criteria-protocol-http
*
*
* optional string response = 196547649;
*
* @return The bytes for response.
*/
@java.lang.Override
public com.google.protobuf.ByteString getResponseBytes() {
java.lang.Object ref = response_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
response_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3208616, host_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(3446913, port_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 41534345, portName_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 51590597, portSpecification_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 160374142, proxyHeader_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 196547649, response_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 229403605, requestPath_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3208616, host_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(3446913, port_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(41534345, portName_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(51590597, portSpecification_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(160374142, proxyHeader_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(196547649, response_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(229403605, requestPath_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.HTTPHealthCheck)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.HTTPHealthCheck other =
(com.google.cloud.compute.v1.HTTPHealthCheck) obj;
if (hasHost() != other.hasHost()) return false;
if (hasHost()) {
if (!getHost().equals(other.getHost())) return false;
}
if (hasPort() != other.hasPort()) return false;
if (hasPort()) {
if (getPort() != other.getPort()) return false;
}
if (hasPortName() != other.hasPortName()) return false;
if (hasPortName()) {
if (!getPortName().equals(other.getPortName())) return false;
}
if (hasPortSpecification() != other.hasPortSpecification()) return false;
if (hasPortSpecification()) {
if (!getPortSpecification().equals(other.getPortSpecification())) return false;
}
if (hasProxyHeader() != other.hasProxyHeader()) return false;
if (hasProxyHeader()) {
if (!getProxyHeader().equals(other.getProxyHeader())) return false;
}
if (hasRequestPath() != other.hasRequestPath()) return false;
if (hasRequestPath()) {
if (!getRequestPath().equals(other.getRequestPath())) return false;
}
if (hasResponse() != other.hasResponse()) return false;
if (hasResponse()) {
if (!getResponse().equals(other.getResponse())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHost()) {
hash = (37 * hash) + HOST_FIELD_NUMBER;
hash = (53 * hash) + getHost().hashCode();
}
if (hasPort()) {
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
}
if (hasPortName()) {
hash = (37 * hash) + PORT_NAME_FIELD_NUMBER;
hash = (53 * hash) + getPortName().hashCode();
}
if (hasPortSpecification()) {
hash = (37 * hash) + PORT_SPECIFICATION_FIELD_NUMBER;
hash = (53 * hash) + getPortSpecification().hashCode();
}
if (hasProxyHeader()) {
hash = (37 * hash) + PROXY_HEADER_FIELD_NUMBER;
hash = (53 * hash) + getProxyHeader().hashCode();
}
if (hasRequestPath()) {
hash = (37 * hash) + REQUEST_PATH_FIELD_NUMBER;
hash = (53 * hash) + getRequestPath().hashCode();
}
if (hasResponse()) {
hash = (37 * hash) + RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getResponse().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.HTTPHealthCheck parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.HTTPHealthCheck prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
*
*
* Protobuf type {@code google.cloud.compute.v1.HTTPHealthCheck}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.HTTPHealthCheck)
com.google.cloud.compute.v1.HTTPHealthCheckOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_HTTPHealthCheck_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_HTTPHealthCheck_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.HTTPHealthCheck.class,
com.google.cloud.compute.v1.HTTPHealthCheck.Builder.class);
}
// Construct using com.google.cloud.compute.v1.HTTPHealthCheck.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
host_ = "";
port_ = 0;
portName_ = "";
portSpecification_ = "";
proxyHeader_ = "";
requestPath_ = "";
response_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_HTTPHealthCheck_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.HTTPHealthCheck getDefaultInstanceForType() {
return com.google.cloud.compute.v1.HTTPHealthCheck.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.HTTPHealthCheck build() {
com.google.cloud.compute.v1.HTTPHealthCheck result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.HTTPHealthCheck buildPartial() {
com.google.cloud.compute.v1.HTTPHealthCheck result =
new com.google.cloud.compute.v1.HTTPHealthCheck(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.compute.v1.HTTPHealthCheck result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.host_ = host_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.port_ = port_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.portName_ = portName_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.portSpecification_ = portSpecification_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.proxyHeader_ = proxyHeader_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.requestPath_ = requestPath_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.response_ = response_;
to_bitField0_ |= 0x00000040;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.HTTPHealthCheck) {
return mergeFrom((com.google.cloud.compute.v1.HTTPHealthCheck) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.HTTPHealthCheck other) {
if (other == com.google.cloud.compute.v1.HTTPHealthCheck.getDefaultInstance()) return this;
if (other.hasHost()) {
host_ = other.host_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasPort()) {
setPort(other.getPort());
}
if (other.hasPortName()) {
portName_ = other.portName_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasPortSpecification()) {
portSpecification_ = other.portSpecification_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasProxyHeader()) {
proxyHeader_ = other.proxyHeader_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasRequestPath()) {
requestPath_ = other.requestPath_;
bitField0_ |= 0x00000020;
onChanged();
}
if (other.hasResponse()) {
response_ = other.response_;
bitField0_ |= 0x00000040;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 25668930:
{
host_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 25668930
case 27575304:
{
port_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 27575304
case 332274762:
{
portName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 332274762
case 412724778:
{
portSpecification_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 412724778
case 1282993138:
{
proxyHeader_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 1282993138
case 1572381194:
{
response_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 1572381194
case 1835228842:
{
requestPath_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 1835228842
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object host_ = "";
/**
*
*
*
* The value of the host header in the HTTP health check request. If left empty (default value), the host header is set to the destination IP address to which health check packets are sent. The destination IP address depends on the type of load balancer. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#hc-packet-dest
*
*
* optional string host = 3208616;
*
* @return Whether the host field is set.
*/
public boolean hasHost() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The value of the host header in the HTTP health check request. If left empty (default value), the host header is set to the destination IP address to which health check packets are sent. The destination IP address depends on the type of load balancer. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#hc-packet-dest
*
*
* optional string host = 3208616;
*
* @return The host.
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
host_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The value of the host header in the HTTP health check request. If left empty (default value), the host header is set to the destination IP address to which health check packets are sent. The destination IP address depends on the type of load balancer. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#hc-packet-dest
*
*
* optional string host = 3208616;
*
* @return The bytes for host.
*/
public com.google.protobuf.ByteString getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The value of the host header in the HTTP health check request. If left empty (default value), the host header is set to the destination IP address to which health check packets are sent. The destination IP address depends on the type of load balancer. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#hc-packet-dest
*
*
* optional string host = 3208616;
*
* @param value The host to set.
* @return This builder for chaining.
*/
public Builder setHost(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
host_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The value of the host header in the HTTP health check request. If left empty (default value), the host header is set to the destination IP address to which health check packets are sent. The destination IP address depends on the type of load balancer. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#hc-packet-dest
*
*
* optional string host = 3208616;
*
* @return This builder for chaining.
*/
public Builder clearHost() {
host_ = getDefaultInstance().getHost();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* The value of the host header in the HTTP health check request. If left empty (default value), the host header is set to the destination IP address to which health check packets are sent. The destination IP address depends on the type of load balancer. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#hc-packet-dest
*
*
* optional string host = 3208616;
*
* @param value The bytes for host to set.
* @return This builder for chaining.
*/
public Builder setHostBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
host_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int port_;
/**
*
*
*
* The TCP port number to which the health check prober sends packets. The default value is 80. Valid values are 1 through 65535.
*
*
* optional int32 port = 3446913;
*
* @return Whether the port field is set.
*/
@java.lang.Override
public boolean hasPort() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The TCP port number to which the health check prober sends packets. The default value is 80. Valid values are 1 through 65535.
*
*
* optional int32 port = 3446913;
*
* @return The port.
*/
@java.lang.Override
public int getPort() {
return port_;
}
/**
*
*
*
* The TCP port number to which the health check prober sends packets. The default value is 80. Valid values are 1 through 65535.
*
*
* optional int32 port = 3446913;
*
* @param value The port to set.
* @return This builder for chaining.
*/
public Builder setPort(int value) {
port_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* The TCP port number to which the health check prober sends packets. The default value is 80. Valid values are 1 through 65535.
*
*
* optional int32 port = 3446913;
*
* @return This builder for chaining.
*/
public Builder clearPort() {
bitField0_ = (bitField0_ & ~0x00000002);
port_ = 0;
onChanged();
return this;
}
private java.lang.Object portName_ = "";
/**
*
*
*
* Not supported.
*
*
* optional string port_name = 41534345;
*
* @return Whether the portName field is set.
*/
public boolean hasPortName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Not supported.
*
*
* optional string port_name = 41534345;
*
* @return The portName.
*/
public java.lang.String getPortName() {
java.lang.Object ref = portName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Not supported.
*
*
* optional string port_name = 41534345;
*
* @return The bytes for portName.
*/
public com.google.protobuf.ByteString getPortNameBytes() {
java.lang.Object ref = portName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
portName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Not supported.
*
*
* optional string port_name = 41534345;
*
* @param value The portName to set.
* @return This builder for chaining.
*/
public Builder setPortName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portName_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Not supported.
*
*
* optional string port_name = 41534345;
*
* @return This builder for chaining.
*/
public Builder clearPortName() {
portName_ = getDefaultInstance().getPortName();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* Not supported.
*
*
* optional string port_name = 41534345;
*
* @param value The bytes for portName to set.
* @return This builder for chaining.
*/
public Builder setPortNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portName_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object portSpecification_ = "";
/**
*
*
*
* Specifies how a port is selected for health checking. Can be one of the following values: USE_FIXED_PORT: Specifies a port number explicitly using the port field in the health check. Supported by backend services for passthrough load balancers and backend services for proxy load balancers. Also supported in legacy HTTP health checks for target pools. The health check supports all backends supported by the backend service provided the backend can be health checked. For example, GCE_VM_IP network endpoint groups, GCE_VM_IP_PORT network endpoint groups, and instance group backends. USE_NAMED_PORT: Not supported. USE_SERVING_PORT: Provides an indirect method of specifying the health check port by referring to the backend service. Only supported by backend services for proxy load balancers. Not supported by target pools. Not supported by backend services for pass-through load balancers. Supports all backends that can be health checked; for example, GCE_VM_IP_PORT network endpoint groups and instance group backends. For GCE_VM_IP_PORT network endpoint group backends, the health check uses the port number specified for each endpoint in the network endpoint group. For instance group backends, the health check uses the port number determined by looking up the backend service's named port in the instance group's list of named ports.
* Check the PortSpecification enum for the list of possible values.
*
*
* optional string port_specification = 51590597;
*
* @return Whether the portSpecification field is set.
*/
public boolean hasPortSpecification() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Specifies how a port is selected for health checking. Can be one of the following values: USE_FIXED_PORT: Specifies a port number explicitly using the port field in the health check. Supported by backend services for passthrough load balancers and backend services for proxy load balancers. Also supported in legacy HTTP health checks for target pools. The health check supports all backends supported by the backend service provided the backend can be health checked. For example, GCE_VM_IP network endpoint groups, GCE_VM_IP_PORT network endpoint groups, and instance group backends. USE_NAMED_PORT: Not supported. USE_SERVING_PORT: Provides an indirect method of specifying the health check port by referring to the backend service. Only supported by backend services for proxy load balancers. Not supported by target pools. Not supported by backend services for pass-through load balancers. Supports all backends that can be health checked; for example, GCE_VM_IP_PORT network endpoint groups and instance group backends. For GCE_VM_IP_PORT network endpoint group backends, the health check uses the port number specified for each endpoint in the network endpoint group. For instance group backends, the health check uses the port number determined by looking up the backend service's named port in the instance group's list of named ports.
* Check the PortSpecification enum for the list of possible values.
*
*
* optional string port_specification = 51590597;
*
* @return The portSpecification.
*/
public java.lang.String getPortSpecification() {
java.lang.Object ref = portSpecification_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portSpecification_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Specifies how a port is selected for health checking. Can be one of the following values: USE_FIXED_PORT: Specifies a port number explicitly using the port field in the health check. Supported by backend services for passthrough load balancers and backend services for proxy load balancers. Also supported in legacy HTTP health checks for target pools. The health check supports all backends supported by the backend service provided the backend can be health checked. For example, GCE_VM_IP network endpoint groups, GCE_VM_IP_PORT network endpoint groups, and instance group backends. USE_NAMED_PORT: Not supported. USE_SERVING_PORT: Provides an indirect method of specifying the health check port by referring to the backend service. Only supported by backend services for proxy load balancers. Not supported by target pools. Not supported by backend services for pass-through load balancers. Supports all backends that can be health checked; for example, GCE_VM_IP_PORT network endpoint groups and instance group backends. For GCE_VM_IP_PORT network endpoint group backends, the health check uses the port number specified for each endpoint in the network endpoint group. For instance group backends, the health check uses the port number determined by looking up the backend service's named port in the instance group's list of named ports.
* Check the PortSpecification enum for the list of possible values.
*
*
* optional string port_specification = 51590597;
*
* @return The bytes for portSpecification.
*/
public com.google.protobuf.ByteString getPortSpecificationBytes() {
java.lang.Object ref = portSpecification_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
portSpecification_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Specifies how a port is selected for health checking. Can be one of the following values: USE_FIXED_PORT: Specifies a port number explicitly using the port field in the health check. Supported by backend services for passthrough load balancers and backend services for proxy load balancers. Also supported in legacy HTTP health checks for target pools. The health check supports all backends supported by the backend service provided the backend can be health checked. For example, GCE_VM_IP network endpoint groups, GCE_VM_IP_PORT network endpoint groups, and instance group backends. USE_NAMED_PORT: Not supported. USE_SERVING_PORT: Provides an indirect method of specifying the health check port by referring to the backend service. Only supported by backend services for proxy load balancers. Not supported by target pools. Not supported by backend services for pass-through load balancers. Supports all backends that can be health checked; for example, GCE_VM_IP_PORT network endpoint groups and instance group backends. For GCE_VM_IP_PORT network endpoint group backends, the health check uses the port number specified for each endpoint in the network endpoint group. For instance group backends, the health check uses the port number determined by looking up the backend service's named port in the instance group's list of named ports.
* Check the PortSpecification enum for the list of possible values.
*
*
* optional string port_specification = 51590597;
*
* @param value The portSpecification to set.
* @return This builder for chaining.
*/
public Builder setPortSpecification(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portSpecification_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Specifies how a port is selected for health checking. Can be one of the following values: USE_FIXED_PORT: Specifies a port number explicitly using the port field in the health check. Supported by backend services for passthrough load balancers and backend services for proxy load balancers. Also supported in legacy HTTP health checks for target pools. The health check supports all backends supported by the backend service provided the backend can be health checked. For example, GCE_VM_IP network endpoint groups, GCE_VM_IP_PORT network endpoint groups, and instance group backends. USE_NAMED_PORT: Not supported. USE_SERVING_PORT: Provides an indirect method of specifying the health check port by referring to the backend service. Only supported by backend services for proxy load balancers. Not supported by target pools. Not supported by backend services for pass-through load balancers. Supports all backends that can be health checked; for example, GCE_VM_IP_PORT network endpoint groups and instance group backends. For GCE_VM_IP_PORT network endpoint group backends, the health check uses the port number specified for each endpoint in the network endpoint group. For instance group backends, the health check uses the port number determined by looking up the backend service's named port in the instance group's list of named ports.
* Check the PortSpecification enum for the list of possible values.
*
*
* optional string port_specification = 51590597;
*
* @return This builder for chaining.
*/
public Builder clearPortSpecification() {
portSpecification_ = getDefaultInstance().getPortSpecification();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
*
*
* Specifies how a port is selected for health checking. Can be one of the following values: USE_FIXED_PORT: Specifies a port number explicitly using the port field in the health check. Supported by backend services for passthrough load balancers and backend services for proxy load balancers. Also supported in legacy HTTP health checks for target pools. The health check supports all backends supported by the backend service provided the backend can be health checked. For example, GCE_VM_IP network endpoint groups, GCE_VM_IP_PORT network endpoint groups, and instance group backends. USE_NAMED_PORT: Not supported. USE_SERVING_PORT: Provides an indirect method of specifying the health check port by referring to the backend service. Only supported by backend services for proxy load balancers. Not supported by target pools. Not supported by backend services for pass-through load balancers. Supports all backends that can be health checked; for example, GCE_VM_IP_PORT network endpoint groups and instance group backends. For GCE_VM_IP_PORT network endpoint group backends, the health check uses the port number specified for each endpoint in the network endpoint group. For instance group backends, the health check uses the port number determined by looking up the backend service's named port in the instance group's list of named ports.
* Check the PortSpecification enum for the list of possible values.
*
*
* optional string port_specification = 51590597;
*
* @param value The bytes for portSpecification to set.
* @return This builder for chaining.
*/
public Builder setPortSpecificationBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portSpecification_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object proxyHeader_ = "";
/**
*
*
*
* Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
* Check the ProxyHeader enum for the list of possible values.
*
*
* optional string proxy_header = 160374142;
*
* @return Whether the proxyHeader field is set.
*/
public boolean hasProxyHeader() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
* Check the ProxyHeader enum for the list of possible values.
*
*
* optional string proxy_header = 160374142;
*
* @return The proxyHeader.
*/
public java.lang.String getProxyHeader() {
java.lang.Object ref = proxyHeader_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
proxyHeader_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
* Check the ProxyHeader enum for the list of possible values.
*
*
* optional string proxy_header = 160374142;
*
* @return The bytes for proxyHeader.
*/
public com.google.protobuf.ByteString getProxyHeaderBytes() {
java.lang.Object ref = proxyHeader_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
proxyHeader_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
* Check the ProxyHeader enum for the list of possible values.
*
*
* optional string proxy_header = 160374142;
*
* @param value The proxyHeader to set.
* @return This builder for chaining.
*/
public Builder setProxyHeader(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
proxyHeader_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
* Check the ProxyHeader enum for the list of possible values.
*
*
* optional string proxy_header = 160374142;
*
* @return This builder for chaining.
*/
public Builder clearProxyHeader() {
proxyHeader_ = getDefaultInstance().getProxyHeader();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
*
*
* Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
* Check the ProxyHeader enum for the list of possible values.
*
*
* optional string proxy_header = 160374142;
*
* @param value The bytes for proxyHeader to set.
* @return This builder for chaining.
*/
public Builder setProxyHeaderBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
proxyHeader_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private java.lang.Object requestPath_ = "";
/**
*
*
*
* The request path of the HTTP health check request. The default value is /. Must comply with RFC3986.
*
*
* optional string request_path = 229403605;
*
* @return Whether the requestPath field is set.
*/
public boolean hasRequestPath() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* The request path of the HTTP health check request. The default value is /. Must comply with RFC3986.
*
*
* optional string request_path = 229403605;
*
* @return The requestPath.
*/
public java.lang.String getRequestPath() {
java.lang.Object ref = requestPath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestPath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The request path of the HTTP health check request. The default value is /. Must comply with RFC3986.
*
*
* optional string request_path = 229403605;
*
* @return The bytes for requestPath.
*/
public com.google.protobuf.ByteString getRequestPathBytes() {
java.lang.Object ref = requestPath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
requestPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The request path of the HTTP health check request. The default value is /. Must comply with RFC3986.
*
*
* optional string request_path = 229403605;
*
* @param value The requestPath to set.
* @return This builder for chaining.
*/
public Builder setRequestPath(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
requestPath_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* The request path of the HTTP health check request. The default value is /. Must comply with RFC3986.
*
*
* optional string request_path = 229403605;
*
* @return This builder for chaining.
*/
public Builder clearRequestPath() {
requestPath_ = getDefaultInstance().getRequestPath();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
*
*
* The request path of the HTTP health check request. The default value is /. Must comply with RFC3986.
*
*
* optional string request_path = 229403605;
*
* @param value The bytes for requestPath to set.
* @return This builder for chaining.
*/
public Builder setRequestPathBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
requestPath_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.lang.Object response_ = "";
/**
*
*
*
* Creates a content-based HTTP health check. In addition to the required HTTP 200 (OK) status code, you can configure the health check to pass only when the backend sends this specific ASCII response string within the first 1024 bytes of the HTTP response body. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#criteria-protocol-http
*
*
* optional string response = 196547649;
*
* @return Whether the response field is set.
*/
public boolean hasResponse() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Creates a content-based HTTP health check. In addition to the required HTTP 200 (OK) status code, you can configure the health check to pass only when the backend sends this specific ASCII response string within the first 1024 bytes of the HTTP response body. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#criteria-protocol-http
*
*
* optional string response = 196547649;
*
* @return The response.
*/
public java.lang.String getResponse() {
java.lang.Object ref = response_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
response_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Creates a content-based HTTP health check. In addition to the required HTTP 200 (OK) status code, you can configure the health check to pass only when the backend sends this specific ASCII response string within the first 1024 bytes of the HTTP response body. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#criteria-protocol-http
*
*
* optional string response = 196547649;
*
* @return The bytes for response.
*/
public com.google.protobuf.ByteString getResponseBytes() {
java.lang.Object ref = response_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
response_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Creates a content-based HTTP health check. In addition to the required HTTP 200 (OK) status code, you can configure the health check to pass only when the backend sends this specific ASCII response string within the first 1024 bytes of the HTTP response body. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#criteria-protocol-http
*
*
* optional string response = 196547649;
*
* @param value The response to set.
* @return This builder for chaining.
*/
public Builder setResponse(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
response_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Creates a content-based HTTP health check. In addition to the required HTTP 200 (OK) status code, you can configure the health check to pass only when the backend sends this specific ASCII response string within the first 1024 bytes of the HTTP response body. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#criteria-protocol-http
*
*
* optional string response = 196547649;
*
* @return This builder for chaining.
*/
public Builder clearResponse() {
response_ = getDefaultInstance().getResponse();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* Creates a content-based HTTP health check. In addition to the required HTTP 200 (OK) status code, you can configure the health check to pass only when the backend sends this specific ASCII response string within the first 1024 bytes of the HTTP response body. For details, see: https://cloud.google.com/load-balancing/docs/health-check-concepts#criteria-protocol-http
*
*
* optional string response = 196547649;
*
* @param value The bytes for response to set.
* @return This builder for chaining.
*/
public Builder setResponseBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
response_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.HTTPHealthCheck)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.HTTPHealthCheck)
private static final com.google.cloud.compute.v1.HTTPHealthCheck DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.HTTPHealthCheck();
}
public static com.google.cloud.compute.v1.HTTPHealthCheck getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HTTPHealthCheck parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.HTTPHealthCheck getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy