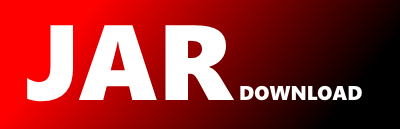
com.google.cloud.compute.v1.HttpHeaderMatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* matchRule criteria for request header matches.
*
*
* Protobuf type {@code google.cloud.compute.v1.HttpHeaderMatch}
*/
public final class HttpHeaderMatch extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.HttpHeaderMatch)
HttpHeaderMatchOrBuilder {
private static final long serialVersionUID = 0L;
// Use HttpHeaderMatch.newBuilder() to construct.
private HttpHeaderMatch(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HttpHeaderMatch() {
exactMatch_ = "";
headerName_ = "";
prefixMatch_ = "";
regexMatch_ = "";
suffixMatch_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new HttpHeaderMatch();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_HttpHeaderMatch_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_HttpHeaderMatch_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.HttpHeaderMatch.class,
com.google.cloud.compute.v1.HttpHeaderMatch.Builder.class);
}
private int bitField0_;
public static final int EXACT_MATCH_FIELD_NUMBER = 457641093;
@SuppressWarnings("serial")
private volatile java.lang.Object exactMatch_ = "";
/**
*
*
*
* The value should exactly match contents of exactMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string exact_match = 457641093;
*
* @return Whether the exactMatch field is set.
*/
@java.lang.Override
public boolean hasExactMatch() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The value should exactly match contents of exactMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string exact_match = 457641093;
*
* @return The exactMatch.
*/
@java.lang.Override
public java.lang.String getExactMatch() {
java.lang.Object ref = exactMatch_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exactMatch_ = s;
return s;
}
}
/**
*
*
*
* The value should exactly match contents of exactMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string exact_match = 457641093;
*
* @return The bytes for exactMatch.
*/
@java.lang.Override
public com.google.protobuf.ByteString getExactMatchBytes() {
java.lang.Object ref = exactMatch_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
exactMatch_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HEADER_NAME_FIELD_NUMBER = 110223613;
@SuppressWarnings("serial")
private volatile java.lang.Object headerName_ = "";
/**
*
*
*
* The name of the HTTP header to match. For matching against the HTTP request's authority, use a headerMatch with the header name ":authority". For matching a request's method, use the headerName ":method". When the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to true, only non-binary user-specified custom metadata and the `content-type` header are supported. The following transport-level headers cannot be used in header matching rules: `:authority`, `:method`, `:path`, `:scheme`, `user-agent`, `accept-encoding`, `content-encoding`, `grpc-accept-encoding`, `grpc-encoding`, `grpc-previous-rpc-attempts`, `grpc-tags-bin`, `grpc-timeout` and `grpc-trace-bin`.
*
*
* optional string header_name = 110223613;
*
* @return Whether the headerName field is set.
*/
@java.lang.Override
public boolean hasHeaderName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The name of the HTTP header to match. For matching against the HTTP request's authority, use a headerMatch with the header name ":authority". For matching a request's method, use the headerName ":method". When the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to true, only non-binary user-specified custom metadata and the `content-type` header are supported. The following transport-level headers cannot be used in header matching rules: `:authority`, `:method`, `:path`, `:scheme`, `user-agent`, `accept-encoding`, `content-encoding`, `grpc-accept-encoding`, `grpc-encoding`, `grpc-previous-rpc-attempts`, `grpc-tags-bin`, `grpc-timeout` and `grpc-trace-bin`.
*
*
* optional string header_name = 110223613;
*
* @return The headerName.
*/
@java.lang.Override
public java.lang.String getHeaderName() {
java.lang.Object ref = headerName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
headerName_ = s;
return s;
}
}
/**
*
*
*
* The name of the HTTP header to match. For matching against the HTTP request's authority, use a headerMatch with the header name ":authority". For matching a request's method, use the headerName ":method". When the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to true, only non-binary user-specified custom metadata and the `content-type` header are supported. The following transport-level headers cannot be used in header matching rules: `:authority`, `:method`, `:path`, `:scheme`, `user-agent`, `accept-encoding`, `content-encoding`, `grpc-accept-encoding`, `grpc-encoding`, `grpc-previous-rpc-attempts`, `grpc-tags-bin`, `grpc-timeout` and `grpc-trace-bin`.
*
*
* optional string header_name = 110223613;
*
* @return The bytes for headerName.
*/
@java.lang.Override
public com.google.protobuf.ByteString getHeaderNameBytes() {
java.lang.Object ref = headerName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
headerName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INVERT_MATCH_FIELD_NUMBER = 501130268;
private boolean invertMatch_ = false;
/**
*
*
*
* If set to false, the headerMatch is considered a match if the preceding match criteria are met. If set to true, the headerMatch is considered a match if the preceding match criteria are NOT met. The default setting is false.
*
*
* optional bool invert_match = 501130268;
*
* @return Whether the invertMatch field is set.
*/
@java.lang.Override
public boolean hasInvertMatch() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* If set to false, the headerMatch is considered a match if the preceding match criteria are met. If set to true, the headerMatch is considered a match if the preceding match criteria are NOT met. The default setting is false.
*
*
* optional bool invert_match = 501130268;
*
* @return The invertMatch.
*/
@java.lang.Override
public boolean getInvertMatch() {
return invertMatch_;
}
public static final int PREFIX_MATCH_FIELD_NUMBER = 257898968;
@SuppressWarnings("serial")
private volatile java.lang.Object prefixMatch_ = "";
/**
*
*
*
* The value of the header must start with the contents of prefixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string prefix_match = 257898968;
*
* @return Whether the prefixMatch field is set.
*/
@java.lang.Override
public boolean hasPrefixMatch() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The value of the header must start with the contents of prefixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string prefix_match = 257898968;
*
* @return The prefixMatch.
*/
@java.lang.Override
public java.lang.String getPrefixMatch() {
java.lang.Object ref = prefixMatch_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
prefixMatch_ = s;
return s;
}
}
/**
*
*
*
* The value of the header must start with the contents of prefixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string prefix_match = 257898968;
*
* @return The bytes for prefixMatch.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPrefixMatchBytes() {
java.lang.Object ref = prefixMatch_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
prefixMatch_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PRESENT_MATCH_FIELD_NUMBER = 67435841;
private boolean presentMatch_ = false;
/**
*
*
*
* A header with the contents of headerName must exist. The match takes place whether or not the request's header has a value. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional bool present_match = 67435841;
*
* @return Whether the presentMatch field is set.
*/
@java.lang.Override
public boolean hasPresentMatch() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* A header with the contents of headerName must exist. The match takes place whether or not the request's header has a value. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional bool present_match = 67435841;
*
* @return The presentMatch.
*/
@java.lang.Override
public boolean getPresentMatch() {
return presentMatch_;
}
public static final int RANGE_MATCH_FIELD_NUMBER = 97244227;
private com.google.cloud.compute.v1.Int64RangeMatch rangeMatch_;
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*
* @return Whether the rangeMatch field is set.
*/
@java.lang.Override
public boolean hasRangeMatch() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*
* @return The rangeMatch.
*/
@java.lang.Override
public com.google.cloud.compute.v1.Int64RangeMatch getRangeMatch() {
return rangeMatch_ == null
? com.google.cloud.compute.v1.Int64RangeMatch.getDefaultInstance()
: rangeMatch_;
}
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*/
@java.lang.Override
public com.google.cloud.compute.v1.Int64RangeMatchOrBuilder getRangeMatchOrBuilder() {
return rangeMatch_ == null
? com.google.cloud.compute.v1.Int64RangeMatch.getDefaultInstance()
: rangeMatch_;
}
public static final int REGEX_MATCH_FIELD_NUMBER = 107387853;
@SuppressWarnings("serial")
private volatile java.lang.Object regexMatch_ = "";
/**
*
*
*
* The value of the header must match the regular expression specified in regexMatch. For more information about regular expression syntax, see Syntax. For matching against a port specified in the HTTP request, use a headerMatch with headerName set to PORT and a regular expression that satisfies the RFC2616 Host header's port specifier. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. Regular expressions can only be used when the loadBalancingScheme is set to INTERNAL_SELF_MANAGED.
*
*
* optional string regex_match = 107387853;
*
* @return Whether the regexMatch field is set.
*/
@java.lang.Override
public boolean hasRegexMatch() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* The value of the header must match the regular expression specified in regexMatch. For more information about regular expression syntax, see Syntax. For matching against a port specified in the HTTP request, use a headerMatch with headerName set to PORT and a regular expression that satisfies the RFC2616 Host header's port specifier. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. Regular expressions can only be used when the loadBalancingScheme is set to INTERNAL_SELF_MANAGED.
*
*
* optional string regex_match = 107387853;
*
* @return The regexMatch.
*/
@java.lang.Override
public java.lang.String getRegexMatch() {
java.lang.Object ref = regexMatch_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
regexMatch_ = s;
return s;
}
}
/**
*
*
*
* The value of the header must match the regular expression specified in regexMatch. For more information about regular expression syntax, see Syntax. For matching against a port specified in the HTTP request, use a headerMatch with headerName set to PORT and a regular expression that satisfies the RFC2616 Host header's port specifier. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. Regular expressions can only be used when the loadBalancingScheme is set to INTERNAL_SELF_MANAGED.
*
*
* optional string regex_match = 107387853;
*
* @return The bytes for regexMatch.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRegexMatchBytes() {
java.lang.Object ref = regexMatch_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
regexMatch_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUFFIX_MATCH_FIELD_NUMBER = 426488663;
@SuppressWarnings("serial")
private volatile java.lang.Object suffixMatch_ = "";
/**
*
*
*
* The value of the header must end with the contents of suffixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string suffix_match = 426488663;
*
* @return Whether the suffixMatch field is set.
*/
@java.lang.Override
public boolean hasSuffixMatch() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The value of the header must end with the contents of suffixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string suffix_match = 426488663;
*
* @return The suffixMatch.
*/
@java.lang.Override
public java.lang.String getSuffixMatch() {
java.lang.Object ref = suffixMatch_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
suffixMatch_ = s;
return s;
}
}
/**
*
*
*
* The value of the header must end with the contents of suffixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string suffix_match = 426488663;
*
* @return The bytes for suffixMatch.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSuffixMatchBytes() {
java.lang.Object ref = suffixMatch_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
suffixMatch_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000010) != 0)) {
output.writeBool(67435841, presentMatch_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(97244227, getRangeMatch());
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 107387853, regexMatch_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 110223613, headerName_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 257898968, prefixMatch_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 426488663, suffixMatch_);
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 457641093, exactMatch_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(501130268, invertMatch_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(67435841, presentMatch_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(97244227, getRangeMatch());
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(107387853, regexMatch_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(110223613, headerName_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(257898968, prefixMatch_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(426488663, suffixMatch_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(457641093, exactMatch_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(501130268, invertMatch_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.HttpHeaderMatch)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.HttpHeaderMatch other =
(com.google.cloud.compute.v1.HttpHeaderMatch) obj;
if (hasExactMatch() != other.hasExactMatch()) return false;
if (hasExactMatch()) {
if (!getExactMatch().equals(other.getExactMatch())) return false;
}
if (hasHeaderName() != other.hasHeaderName()) return false;
if (hasHeaderName()) {
if (!getHeaderName().equals(other.getHeaderName())) return false;
}
if (hasInvertMatch() != other.hasInvertMatch()) return false;
if (hasInvertMatch()) {
if (getInvertMatch() != other.getInvertMatch()) return false;
}
if (hasPrefixMatch() != other.hasPrefixMatch()) return false;
if (hasPrefixMatch()) {
if (!getPrefixMatch().equals(other.getPrefixMatch())) return false;
}
if (hasPresentMatch() != other.hasPresentMatch()) return false;
if (hasPresentMatch()) {
if (getPresentMatch() != other.getPresentMatch()) return false;
}
if (hasRangeMatch() != other.hasRangeMatch()) return false;
if (hasRangeMatch()) {
if (!getRangeMatch().equals(other.getRangeMatch())) return false;
}
if (hasRegexMatch() != other.hasRegexMatch()) return false;
if (hasRegexMatch()) {
if (!getRegexMatch().equals(other.getRegexMatch())) return false;
}
if (hasSuffixMatch() != other.hasSuffixMatch()) return false;
if (hasSuffixMatch()) {
if (!getSuffixMatch().equals(other.getSuffixMatch())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasExactMatch()) {
hash = (37 * hash) + EXACT_MATCH_FIELD_NUMBER;
hash = (53 * hash) + getExactMatch().hashCode();
}
if (hasHeaderName()) {
hash = (37 * hash) + HEADER_NAME_FIELD_NUMBER;
hash = (53 * hash) + getHeaderName().hashCode();
}
if (hasInvertMatch()) {
hash = (37 * hash) + INVERT_MATCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getInvertMatch());
}
if (hasPrefixMatch()) {
hash = (37 * hash) + PREFIX_MATCH_FIELD_NUMBER;
hash = (53 * hash) + getPrefixMatch().hashCode();
}
if (hasPresentMatch()) {
hash = (37 * hash) + PRESENT_MATCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getPresentMatch());
}
if (hasRangeMatch()) {
hash = (37 * hash) + RANGE_MATCH_FIELD_NUMBER;
hash = (53 * hash) + getRangeMatch().hashCode();
}
if (hasRegexMatch()) {
hash = (37 * hash) + REGEX_MATCH_FIELD_NUMBER;
hash = (53 * hash) + getRegexMatch().hashCode();
}
if (hasSuffixMatch()) {
hash = (37 * hash) + SUFFIX_MATCH_FIELD_NUMBER;
hash = (53 * hash) + getSuffixMatch().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.HttpHeaderMatch parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.HttpHeaderMatch prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* matchRule criteria for request header matches.
*
*
* Protobuf type {@code google.cloud.compute.v1.HttpHeaderMatch}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.HttpHeaderMatch)
com.google.cloud.compute.v1.HttpHeaderMatchOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_HttpHeaderMatch_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_HttpHeaderMatch_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.HttpHeaderMatch.class,
com.google.cloud.compute.v1.HttpHeaderMatch.Builder.class);
}
// Construct using com.google.cloud.compute.v1.HttpHeaderMatch.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getRangeMatchFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
exactMatch_ = "";
headerName_ = "";
invertMatch_ = false;
prefixMatch_ = "";
presentMatch_ = false;
rangeMatch_ = null;
if (rangeMatchBuilder_ != null) {
rangeMatchBuilder_.dispose();
rangeMatchBuilder_ = null;
}
regexMatch_ = "";
suffixMatch_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_HttpHeaderMatch_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.HttpHeaderMatch getDefaultInstanceForType() {
return com.google.cloud.compute.v1.HttpHeaderMatch.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.HttpHeaderMatch build() {
com.google.cloud.compute.v1.HttpHeaderMatch result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.HttpHeaderMatch buildPartial() {
com.google.cloud.compute.v1.HttpHeaderMatch result =
new com.google.cloud.compute.v1.HttpHeaderMatch(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.compute.v1.HttpHeaderMatch result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.exactMatch_ = exactMatch_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.headerName_ = headerName_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.invertMatch_ = invertMatch_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.prefixMatch_ = prefixMatch_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.presentMatch_ = presentMatch_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.rangeMatch_ = rangeMatchBuilder_ == null ? rangeMatch_ : rangeMatchBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.regexMatch_ = regexMatch_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.suffixMatch_ = suffixMatch_;
to_bitField0_ |= 0x00000080;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.HttpHeaderMatch) {
return mergeFrom((com.google.cloud.compute.v1.HttpHeaderMatch) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.HttpHeaderMatch other) {
if (other == com.google.cloud.compute.v1.HttpHeaderMatch.getDefaultInstance()) return this;
if (other.hasExactMatch()) {
exactMatch_ = other.exactMatch_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasHeaderName()) {
headerName_ = other.headerName_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasInvertMatch()) {
setInvertMatch(other.getInvertMatch());
}
if (other.hasPrefixMatch()) {
prefixMatch_ = other.prefixMatch_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasPresentMatch()) {
setPresentMatch(other.getPresentMatch());
}
if (other.hasRangeMatch()) {
mergeRangeMatch(other.getRangeMatch());
}
if (other.hasRegexMatch()) {
regexMatch_ = other.regexMatch_;
bitField0_ |= 0x00000040;
onChanged();
}
if (other.hasSuffixMatch()) {
suffixMatch_ = other.suffixMatch_;
bitField0_ |= 0x00000080;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 539486728:
{
presentMatch_ = input.readBool();
bitField0_ |= 0x00000010;
break;
} // case 539486728
case 777953818:
{
input.readMessage(getRangeMatchFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 777953818
case 859102826:
{
regexMatch_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 859102826
case 881788906:
{
headerName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 881788906
case 2063191746:
{
prefixMatch_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 2063191746
case -883057990:
{
suffixMatch_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case -883057990
case -633838550:
{
exactMatch_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case -633838550
case -285925152:
{
invertMatch_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case -285925152
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object exactMatch_ = "";
/**
*
*
*
* The value should exactly match contents of exactMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string exact_match = 457641093;
*
* @return Whether the exactMatch field is set.
*/
public boolean hasExactMatch() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The value should exactly match contents of exactMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string exact_match = 457641093;
*
* @return The exactMatch.
*/
public java.lang.String getExactMatch() {
java.lang.Object ref = exactMatch_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exactMatch_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The value should exactly match contents of exactMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string exact_match = 457641093;
*
* @return The bytes for exactMatch.
*/
public com.google.protobuf.ByteString getExactMatchBytes() {
java.lang.Object ref = exactMatch_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
exactMatch_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The value should exactly match contents of exactMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string exact_match = 457641093;
*
* @param value The exactMatch to set.
* @return This builder for chaining.
*/
public Builder setExactMatch(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
exactMatch_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The value should exactly match contents of exactMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string exact_match = 457641093;
*
* @return This builder for chaining.
*/
public Builder clearExactMatch() {
exactMatch_ = getDefaultInstance().getExactMatch();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* The value should exactly match contents of exactMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string exact_match = 457641093;
*
* @param value The bytes for exactMatch to set.
* @return This builder for chaining.
*/
public Builder setExactMatchBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
exactMatch_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object headerName_ = "";
/**
*
*
*
* The name of the HTTP header to match. For matching against the HTTP request's authority, use a headerMatch with the header name ":authority". For matching a request's method, use the headerName ":method". When the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to true, only non-binary user-specified custom metadata and the `content-type` header are supported. The following transport-level headers cannot be used in header matching rules: `:authority`, `:method`, `:path`, `:scheme`, `user-agent`, `accept-encoding`, `content-encoding`, `grpc-accept-encoding`, `grpc-encoding`, `grpc-previous-rpc-attempts`, `grpc-tags-bin`, `grpc-timeout` and `grpc-trace-bin`.
*
*
* optional string header_name = 110223613;
*
* @return Whether the headerName field is set.
*/
public boolean hasHeaderName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The name of the HTTP header to match. For matching against the HTTP request's authority, use a headerMatch with the header name ":authority". For matching a request's method, use the headerName ":method". When the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to true, only non-binary user-specified custom metadata and the `content-type` header are supported. The following transport-level headers cannot be used in header matching rules: `:authority`, `:method`, `:path`, `:scheme`, `user-agent`, `accept-encoding`, `content-encoding`, `grpc-accept-encoding`, `grpc-encoding`, `grpc-previous-rpc-attempts`, `grpc-tags-bin`, `grpc-timeout` and `grpc-trace-bin`.
*
*
* optional string header_name = 110223613;
*
* @return The headerName.
*/
public java.lang.String getHeaderName() {
java.lang.Object ref = headerName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
headerName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The name of the HTTP header to match. For matching against the HTTP request's authority, use a headerMatch with the header name ":authority". For matching a request's method, use the headerName ":method". When the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to true, only non-binary user-specified custom metadata and the `content-type` header are supported. The following transport-level headers cannot be used in header matching rules: `:authority`, `:method`, `:path`, `:scheme`, `user-agent`, `accept-encoding`, `content-encoding`, `grpc-accept-encoding`, `grpc-encoding`, `grpc-previous-rpc-attempts`, `grpc-tags-bin`, `grpc-timeout` and `grpc-trace-bin`.
*
*
* optional string header_name = 110223613;
*
* @return The bytes for headerName.
*/
public com.google.protobuf.ByteString getHeaderNameBytes() {
java.lang.Object ref = headerName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
headerName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The name of the HTTP header to match. For matching against the HTTP request's authority, use a headerMatch with the header name ":authority". For matching a request's method, use the headerName ":method". When the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to true, only non-binary user-specified custom metadata and the `content-type` header are supported. The following transport-level headers cannot be used in header matching rules: `:authority`, `:method`, `:path`, `:scheme`, `user-agent`, `accept-encoding`, `content-encoding`, `grpc-accept-encoding`, `grpc-encoding`, `grpc-previous-rpc-attempts`, `grpc-tags-bin`, `grpc-timeout` and `grpc-trace-bin`.
*
*
* optional string header_name = 110223613;
*
* @param value The headerName to set.
* @return This builder for chaining.
*/
public Builder setHeaderName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
headerName_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* The name of the HTTP header to match. For matching against the HTTP request's authority, use a headerMatch with the header name ":authority". For matching a request's method, use the headerName ":method". When the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to true, only non-binary user-specified custom metadata and the `content-type` header are supported. The following transport-level headers cannot be used in header matching rules: `:authority`, `:method`, `:path`, `:scheme`, `user-agent`, `accept-encoding`, `content-encoding`, `grpc-accept-encoding`, `grpc-encoding`, `grpc-previous-rpc-attempts`, `grpc-tags-bin`, `grpc-timeout` and `grpc-trace-bin`.
*
*
* optional string header_name = 110223613;
*
* @return This builder for chaining.
*/
public Builder clearHeaderName() {
headerName_ = getDefaultInstance().getHeaderName();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* The name of the HTTP header to match. For matching against the HTTP request's authority, use a headerMatch with the header name ":authority". For matching a request's method, use the headerName ":method". When the URL map is bound to a target gRPC proxy that has the validateForProxyless field set to true, only non-binary user-specified custom metadata and the `content-type` header are supported. The following transport-level headers cannot be used in header matching rules: `:authority`, `:method`, `:path`, `:scheme`, `user-agent`, `accept-encoding`, `content-encoding`, `grpc-accept-encoding`, `grpc-encoding`, `grpc-previous-rpc-attempts`, `grpc-tags-bin`, `grpc-timeout` and `grpc-trace-bin`.
*
*
* optional string header_name = 110223613;
*
* @param value The bytes for headerName to set.
* @return This builder for chaining.
*/
public Builder setHeaderNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
headerName_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private boolean invertMatch_;
/**
*
*
*
* If set to false, the headerMatch is considered a match if the preceding match criteria are met. If set to true, the headerMatch is considered a match if the preceding match criteria are NOT met. The default setting is false.
*
*
* optional bool invert_match = 501130268;
*
* @return Whether the invertMatch field is set.
*/
@java.lang.Override
public boolean hasInvertMatch() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* If set to false, the headerMatch is considered a match if the preceding match criteria are met. If set to true, the headerMatch is considered a match if the preceding match criteria are NOT met. The default setting is false.
*
*
* optional bool invert_match = 501130268;
*
* @return The invertMatch.
*/
@java.lang.Override
public boolean getInvertMatch() {
return invertMatch_;
}
/**
*
*
*
* If set to false, the headerMatch is considered a match if the preceding match criteria are met. If set to true, the headerMatch is considered a match if the preceding match criteria are NOT met. The default setting is false.
*
*
* optional bool invert_match = 501130268;
*
* @param value The invertMatch to set.
* @return This builder for chaining.
*/
public Builder setInvertMatch(boolean value) {
invertMatch_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* If set to false, the headerMatch is considered a match if the preceding match criteria are met. If set to true, the headerMatch is considered a match if the preceding match criteria are NOT met. The default setting is false.
*
*
* optional bool invert_match = 501130268;
*
* @return This builder for chaining.
*/
public Builder clearInvertMatch() {
bitField0_ = (bitField0_ & ~0x00000004);
invertMatch_ = false;
onChanged();
return this;
}
private java.lang.Object prefixMatch_ = "";
/**
*
*
*
* The value of the header must start with the contents of prefixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string prefix_match = 257898968;
*
* @return Whether the prefixMatch field is set.
*/
public boolean hasPrefixMatch() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The value of the header must start with the contents of prefixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string prefix_match = 257898968;
*
* @return The prefixMatch.
*/
public java.lang.String getPrefixMatch() {
java.lang.Object ref = prefixMatch_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
prefixMatch_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The value of the header must start with the contents of prefixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string prefix_match = 257898968;
*
* @return The bytes for prefixMatch.
*/
public com.google.protobuf.ByteString getPrefixMatchBytes() {
java.lang.Object ref = prefixMatch_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
prefixMatch_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The value of the header must start with the contents of prefixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string prefix_match = 257898968;
*
* @param value The prefixMatch to set.
* @return This builder for chaining.
*/
public Builder setPrefixMatch(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
prefixMatch_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* The value of the header must start with the contents of prefixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string prefix_match = 257898968;
*
* @return This builder for chaining.
*/
public Builder clearPrefixMatch() {
prefixMatch_ = getDefaultInstance().getPrefixMatch();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
*
*
* The value of the header must start with the contents of prefixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string prefix_match = 257898968;
*
* @param value The bytes for prefixMatch to set.
* @return This builder for chaining.
*/
public Builder setPrefixMatchBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
prefixMatch_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private boolean presentMatch_;
/**
*
*
*
* A header with the contents of headerName must exist. The match takes place whether or not the request's header has a value. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional bool present_match = 67435841;
*
* @return Whether the presentMatch field is set.
*/
@java.lang.Override
public boolean hasPresentMatch() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* A header with the contents of headerName must exist. The match takes place whether or not the request's header has a value. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional bool present_match = 67435841;
*
* @return The presentMatch.
*/
@java.lang.Override
public boolean getPresentMatch() {
return presentMatch_;
}
/**
*
*
*
* A header with the contents of headerName must exist. The match takes place whether or not the request's header has a value. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional bool present_match = 67435841;
*
* @param value The presentMatch to set.
* @return This builder for chaining.
*/
public Builder setPresentMatch(boolean value) {
presentMatch_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* A header with the contents of headerName must exist. The match takes place whether or not the request's header has a value. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional bool present_match = 67435841;
*
* @return This builder for chaining.
*/
public Builder clearPresentMatch() {
bitField0_ = (bitField0_ & ~0x00000010);
presentMatch_ = false;
onChanged();
return this;
}
private com.google.cloud.compute.v1.Int64RangeMatch rangeMatch_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.Int64RangeMatch,
com.google.cloud.compute.v1.Int64RangeMatch.Builder,
com.google.cloud.compute.v1.Int64RangeMatchOrBuilder>
rangeMatchBuilder_;
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*
* @return Whether the rangeMatch field is set.
*/
public boolean hasRangeMatch() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*
* @return The rangeMatch.
*/
public com.google.cloud.compute.v1.Int64RangeMatch getRangeMatch() {
if (rangeMatchBuilder_ == null) {
return rangeMatch_ == null
? com.google.cloud.compute.v1.Int64RangeMatch.getDefaultInstance()
: rangeMatch_;
} else {
return rangeMatchBuilder_.getMessage();
}
}
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*/
public Builder setRangeMatch(com.google.cloud.compute.v1.Int64RangeMatch value) {
if (rangeMatchBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rangeMatch_ = value;
} else {
rangeMatchBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*/
public Builder setRangeMatch(
com.google.cloud.compute.v1.Int64RangeMatch.Builder builderForValue) {
if (rangeMatchBuilder_ == null) {
rangeMatch_ = builderForValue.build();
} else {
rangeMatchBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*/
public Builder mergeRangeMatch(com.google.cloud.compute.v1.Int64RangeMatch value) {
if (rangeMatchBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)
&& rangeMatch_ != null
&& rangeMatch_ != com.google.cloud.compute.v1.Int64RangeMatch.getDefaultInstance()) {
getRangeMatchBuilder().mergeFrom(value);
} else {
rangeMatch_ = value;
}
} else {
rangeMatchBuilder_.mergeFrom(value);
}
if (rangeMatch_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*/
public Builder clearRangeMatch() {
bitField0_ = (bitField0_ & ~0x00000020);
rangeMatch_ = null;
if (rangeMatchBuilder_ != null) {
rangeMatchBuilder_.dispose();
rangeMatchBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*/
public com.google.cloud.compute.v1.Int64RangeMatch.Builder getRangeMatchBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getRangeMatchFieldBuilder().getBuilder();
}
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*/
public com.google.cloud.compute.v1.Int64RangeMatchOrBuilder getRangeMatchOrBuilder() {
if (rangeMatchBuilder_ != null) {
return rangeMatchBuilder_.getMessageOrBuilder();
} else {
return rangeMatch_ == null
? com.google.cloud.compute.v1.Int64RangeMatch.getDefaultInstance()
: rangeMatch_;
}
}
/**
*
*
*
* The header value must be an integer and its value must be in the range specified in rangeMatch. If the header does not contain an integer, number or is empty, the match fails. For example for a range [-5, 0] - -3 will match. - 0 will not match. - 0.25 will not match. - -3someString will not match. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. rangeMatch is not supported for load balancers that have loadBalancingScheme set to EXTERNAL.
*
*
* optional .google.cloud.compute.v1.Int64RangeMatch range_match = 97244227;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.Int64RangeMatch,
com.google.cloud.compute.v1.Int64RangeMatch.Builder,
com.google.cloud.compute.v1.Int64RangeMatchOrBuilder>
getRangeMatchFieldBuilder() {
if (rangeMatchBuilder_ == null) {
rangeMatchBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.Int64RangeMatch,
com.google.cloud.compute.v1.Int64RangeMatch.Builder,
com.google.cloud.compute.v1.Int64RangeMatchOrBuilder>(
getRangeMatch(), getParentForChildren(), isClean());
rangeMatch_ = null;
}
return rangeMatchBuilder_;
}
private java.lang.Object regexMatch_ = "";
/**
*
*
*
* The value of the header must match the regular expression specified in regexMatch. For more information about regular expression syntax, see Syntax. For matching against a port specified in the HTTP request, use a headerMatch with headerName set to PORT and a regular expression that satisfies the RFC2616 Host header's port specifier. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. Regular expressions can only be used when the loadBalancingScheme is set to INTERNAL_SELF_MANAGED.
*
*
* optional string regex_match = 107387853;
*
* @return Whether the regexMatch field is set.
*/
public boolean hasRegexMatch() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* The value of the header must match the regular expression specified in regexMatch. For more information about regular expression syntax, see Syntax. For matching against a port specified in the HTTP request, use a headerMatch with headerName set to PORT and a regular expression that satisfies the RFC2616 Host header's port specifier. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. Regular expressions can only be used when the loadBalancingScheme is set to INTERNAL_SELF_MANAGED.
*
*
* optional string regex_match = 107387853;
*
* @return The regexMatch.
*/
public java.lang.String getRegexMatch() {
java.lang.Object ref = regexMatch_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
regexMatch_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The value of the header must match the regular expression specified in regexMatch. For more information about regular expression syntax, see Syntax. For matching against a port specified in the HTTP request, use a headerMatch with headerName set to PORT and a regular expression that satisfies the RFC2616 Host header's port specifier. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. Regular expressions can only be used when the loadBalancingScheme is set to INTERNAL_SELF_MANAGED.
*
*
* optional string regex_match = 107387853;
*
* @return The bytes for regexMatch.
*/
public com.google.protobuf.ByteString getRegexMatchBytes() {
java.lang.Object ref = regexMatch_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
regexMatch_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The value of the header must match the regular expression specified in regexMatch. For more information about regular expression syntax, see Syntax. For matching against a port specified in the HTTP request, use a headerMatch with headerName set to PORT and a regular expression that satisfies the RFC2616 Host header's port specifier. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. Regular expressions can only be used when the loadBalancingScheme is set to INTERNAL_SELF_MANAGED.
*
*
* optional string regex_match = 107387853;
*
* @param value The regexMatch to set.
* @return This builder for chaining.
*/
public Builder setRegexMatch(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
regexMatch_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* The value of the header must match the regular expression specified in regexMatch. For more information about regular expression syntax, see Syntax. For matching against a port specified in the HTTP request, use a headerMatch with headerName set to PORT and a regular expression that satisfies the RFC2616 Host header's port specifier. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. Regular expressions can only be used when the loadBalancingScheme is set to INTERNAL_SELF_MANAGED.
*
*
* optional string regex_match = 107387853;
*
* @return This builder for chaining.
*/
public Builder clearRegexMatch() {
regexMatch_ = getDefaultInstance().getRegexMatch();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* The value of the header must match the regular expression specified in regexMatch. For more information about regular expression syntax, see Syntax. For matching against a port specified in the HTTP request, use a headerMatch with headerName set to PORT and a regular expression that satisfies the RFC2616 Host header's port specifier. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set. Regular expressions can only be used when the loadBalancingScheme is set to INTERNAL_SELF_MANAGED.
*
*
* optional string regex_match = 107387853;
*
* @param value The bytes for regexMatch to set.
* @return This builder for chaining.
*/
public Builder setRegexMatchBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
regexMatch_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object suffixMatch_ = "";
/**
*
*
*
* The value of the header must end with the contents of suffixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string suffix_match = 426488663;
*
* @return Whether the suffixMatch field is set.
*/
public boolean hasSuffixMatch() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The value of the header must end with the contents of suffixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string suffix_match = 426488663;
*
* @return The suffixMatch.
*/
public java.lang.String getSuffixMatch() {
java.lang.Object ref = suffixMatch_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
suffixMatch_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The value of the header must end with the contents of suffixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string suffix_match = 426488663;
*
* @return The bytes for suffixMatch.
*/
public com.google.protobuf.ByteString getSuffixMatchBytes() {
java.lang.Object ref = suffixMatch_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
suffixMatch_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The value of the header must end with the contents of suffixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string suffix_match = 426488663;
*
* @param value The suffixMatch to set.
* @return This builder for chaining.
*/
public Builder setSuffixMatch(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
suffixMatch_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* The value of the header must end with the contents of suffixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string suffix_match = 426488663;
*
* @return This builder for chaining.
*/
public Builder clearSuffixMatch() {
suffixMatch_ = getDefaultInstance().getSuffixMatch();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
*
*
* The value of the header must end with the contents of suffixMatch. Only one of exactMatch, prefixMatch, suffixMatch, regexMatch, presentMatch or rangeMatch must be set.
*
*
* optional string suffix_match = 426488663;
*
* @param value The bytes for suffixMatch to set.
* @return This builder for chaining.
*/
public Builder setSuffixMatchBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
suffixMatch_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.HttpHeaderMatch)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.HttpHeaderMatch)
private static final com.google.cloud.compute.v1.HttpHeaderMatch DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.HttpHeaderMatch();
}
public static com.google.cloud.compute.v1.HttpHeaderMatch getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HttpHeaderMatch parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.HttpHeaderMatch getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy