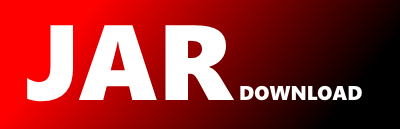
com.google.cloud.compute.v1.Image Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* Represents an Image resource. You can use images to create boot disks for your VM instances. For more information, read Images.
*
*
* Protobuf type {@code google.cloud.compute.v1.Image}
*/
public final class Image extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.Image)
ImageOrBuilder {
private static final long serialVersionUID = 0L;
// Use Image.newBuilder() to construct.
private Image(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Image() {
architecture_ = "";
creationTimestamp_ = "";
description_ = "";
family_ = "";
guestOsFeatures_ = java.util.Collections.emptyList();
kind_ = "";
labelFingerprint_ = "";
licenseCodes_ = emptyLongList();
licenses_ = com.google.protobuf.LazyStringArrayList.emptyList();
name_ = "";
selfLink_ = "";
sourceDisk_ = "";
sourceDiskId_ = "";
sourceImage_ = "";
sourceImageId_ = "";
sourceSnapshot_ = "";
sourceSnapshotId_ = "";
sourceType_ = "";
status_ = "";
storageLocations_ = com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Image();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Image_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 500195327:
return internalGetLabels();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Image_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.Image.class,
com.google.cloud.compute.v1.Image.Builder.class);
}
/**
*
*
*
* The architecture of the image. Valid values are ARM64 or X86_64.
*
*
* Protobuf enum {@code google.cloud.compute.v1.Image.Architecture}
*/
public enum Architecture implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_ARCHITECTURE = 0;
*/
UNDEFINED_ARCHITECTURE(0),
/**
*
*
*
* Default value indicating Architecture is not set.
*
*
* ARCHITECTURE_UNSPECIFIED = 394750507;
*/
ARCHITECTURE_UNSPECIFIED(394750507),
/**
*
*
*
* Machines with architecture ARM64
*
*
* ARM64 = 62547450;
*/
ARM64(62547450),
/**
*
*
*
* Machines with architecture X86_64
*
*
* X86_64 = 425300551;
*/
X86_64(425300551),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_ARCHITECTURE = 0;
*/
public static final int UNDEFINED_ARCHITECTURE_VALUE = 0;
/**
*
*
*
* Default value indicating Architecture is not set.
*
*
* ARCHITECTURE_UNSPECIFIED = 394750507;
*/
public static final int ARCHITECTURE_UNSPECIFIED_VALUE = 394750507;
/**
*
*
*
* Machines with architecture ARM64
*
*
* ARM64 = 62547450;
*/
public static final int ARM64_VALUE = 62547450;
/**
*
*
*
* Machines with architecture X86_64
*
*
* X86_64 = 425300551;
*/
public static final int X86_64_VALUE = 425300551;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Architecture valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Architecture forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_ARCHITECTURE;
case 394750507:
return ARCHITECTURE_UNSPECIFIED;
case 62547450:
return ARM64;
case 425300551:
return X86_64;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Architecture findValueByNumber(int number) {
return Architecture.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.Image.getDescriptor().getEnumTypes().get(0);
}
private static final Architecture[] VALUES = values();
public static Architecture valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Architecture(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.Image.Architecture)
}
/**
*
*
*
* The type of the image used to create this disk. The default and only valid value is RAW.
*
*
* Protobuf enum {@code google.cloud.compute.v1.Image.SourceType}
*/
public enum SourceType implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_SOURCE_TYPE = 0;
*/
UNDEFINED_SOURCE_TYPE(0),
/** RAW = 80904;
*/
RAW(80904),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_SOURCE_TYPE = 0;
*/
public static final int UNDEFINED_SOURCE_TYPE_VALUE = 0;
/** RAW = 80904;
*/
public static final int RAW_VALUE = 80904;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SourceType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static SourceType forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_SOURCE_TYPE;
case 80904:
return RAW;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public SourceType findValueByNumber(int number) {
return SourceType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.Image.getDescriptor().getEnumTypes().get(1);
}
private static final SourceType[] VALUES = values();
public static SourceType valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private SourceType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.Image.SourceType)
}
/**
*
*
*
* [Output Only] The status of the image. An image can be used to create other resources, such as instances, only after the image has been successfully created and the status is set to READY. Possible values are FAILED, PENDING, or READY.
*
*
* Protobuf enum {@code google.cloud.compute.v1.Image.Status}
*/
public enum Status implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STATUS = 0;
*/
UNDEFINED_STATUS(0),
/**
*
*
*
* Image is deleting.
*
*
* DELETING = 528602024;
*/
DELETING(528602024),
/**
*
*
*
* Image creation failed due to an error.
*
*
* FAILED = 455706685;
*/
FAILED(455706685),
/**
*
*
*
* Image hasn't been created as yet.
*
*
* PENDING = 35394935;
*/
PENDING(35394935),
/**
*
*
*
* Image has been successfully created.
*
*
* READY = 77848963;
*/
READY(77848963),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STATUS = 0;
*/
public static final int UNDEFINED_STATUS_VALUE = 0;
/**
*
*
*
* Image is deleting.
*
*
* DELETING = 528602024;
*/
public static final int DELETING_VALUE = 528602024;
/**
*
*
*
* Image creation failed due to an error.
*
*
* FAILED = 455706685;
*/
public static final int FAILED_VALUE = 455706685;
/**
*
*
*
* Image hasn't been created as yet.
*
*
* PENDING = 35394935;
*/
public static final int PENDING_VALUE = 35394935;
/**
*
*
*
* Image has been successfully created.
*
*
* READY = 77848963;
*/
public static final int READY_VALUE = 77848963;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Status valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Status forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_STATUS;
case 528602024:
return DELETING;
case 455706685:
return FAILED;
case 35394935:
return PENDING;
case 77848963:
return READY;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Status findValueByNumber(int number) {
return Status.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.Image.getDescriptor().getEnumTypes().get(2);
}
private static final Status[] VALUES = values();
public static Status valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Status(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.Image.Status)
}
private int bitField0_;
public static final int ARCHITECTURE_FIELD_NUMBER = 302803283;
@SuppressWarnings("serial")
private volatile java.lang.Object architecture_ = "";
/**
*
*
*
* The architecture of the image. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return Whether the architecture field is set.
*/
@java.lang.Override
public boolean hasArchitecture() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The architecture of the image. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return The architecture.
*/
@java.lang.Override
public java.lang.String getArchitecture() {
java.lang.Object ref = architecture_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
architecture_ = s;
return s;
}
}
/**
*
*
*
* The architecture of the image. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return The bytes for architecture.
*/
@java.lang.Override
public com.google.protobuf.ByteString getArchitectureBytes() {
java.lang.Object ref = architecture_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
architecture_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ARCHIVE_SIZE_BYTES_FIELD_NUMBER = 381093450;
private long archiveSizeBytes_ = 0L;
/**
*
*
*
* Size of the image tar.gz archive stored in Google Cloud Storage (in bytes).
*
*
* optional int64 archive_size_bytes = 381093450;
*
* @return Whether the archiveSizeBytes field is set.
*/
@java.lang.Override
public boolean hasArchiveSizeBytes() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Size of the image tar.gz archive stored in Google Cloud Storage (in bytes).
*
*
* optional int64 archive_size_bytes = 381093450;
*
* @return The archiveSizeBytes.
*/
@java.lang.Override
public long getArchiveSizeBytes() {
return archiveSizeBytes_;
}
public static final int CREATION_TIMESTAMP_FIELD_NUMBER = 30525366;
@SuppressWarnings("serial")
private volatile java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
@java.lang.Override
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
@java.lang.Override
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEPRECATED_FIELD_NUMBER = 515138995;
private com.google.cloud.compute.v1.DeprecationStatus deprecated_;
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*
* @return Whether the deprecated field is set.
*/
@java.lang.Override
public boolean hasDeprecated() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*
* @return The deprecated.
*/
@java.lang.Override
public com.google.cloud.compute.v1.DeprecationStatus getDeprecated() {
return deprecated_ == null
? com.google.cloud.compute.v1.DeprecationStatus.getDefaultInstance()
: deprecated_;
}
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*/
@java.lang.Override
public com.google.cloud.compute.v1.DeprecationStatusOrBuilder getDeprecatedOrBuilder() {
return deprecated_ == null
? com.google.cloud.compute.v1.DeprecationStatus.getDefaultInstance()
: deprecated_;
}
public static final int DESCRIPTION_FIELD_NUMBER = 422937596;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DISK_SIZE_GB_FIELD_NUMBER = 316263735;
private long diskSizeGb_ = 0L;
/**
*
*
*
* Size of the image when restored onto a persistent disk (in GB).
*
*
* optional int64 disk_size_gb = 316263735;
*
* @return Whether the diskSizeGb field is set.
*/
@java.lang.Override
public boolean hasDiskSizeGb() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Size of the image when restored onto a persistent disk (in GB).
*
*
* optional int64 disk_size_gb = 316263735;
*
* @return The diskSizeGb.
*/
@java.lang.Override
public long getDiskSizeGb() {
return diskSizeGb_;
}
public static final int ENABLE_CONFIDENTIAL_COMPUTE_FIELD_NUMBER = 102135228;
private boolean enableConfidentialCompute_ = false;
/**
*
*
*
* Whether this image is created from a confidential compute mode disk. [Output Only]: This field is not set by user, but from source disk.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @return Whether the enableConfidentialCompute field is set.
*/
@java.lang.Override
public boolean hasEnableConfidentialCompute() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Whether this image is created from a confidential compute mode disk. [Output Only]: This field is not set by user, but from source disk.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @return The enableConfidentialCompute.
*/
@java.lang.Override
public boolean getEnableConfidentialCompute() {
return enableConfidentialCompute_;
}
public static final int FAMILY_FIELD_NUMBER = 328751972;
@SuppressWarnings("serial")
private volatile java.lang.Object family_ = "";
/**
*
*
*
* The name of the image family to which this image belongs. The image family name can be from a publicly managed image family provided by Compute Engine, or from a custom image family you create. For example, centos-stream-9 is a publicly available image family. For more information, see Image family best practices. When creating disks, you can specify an image family instead of a specific image name. The image family always returns its latest image that is not deprecated. The name of the image family must comply with RFC1035.
*
*
* optional string family = 328751972;
*
* @return Whether the family field is set.
*/
@java.lang.Override
public boolean hasFamily() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The name of the image family to which this image belongs. The image family name can be from a publicly managed image family provided by Compute Engine, or from a custom image family you create. For example, centos-stream-9 is a publicly available image family. For more information, see Image family best practices. When creating disks, you can specify an image family instead of a specific image name. The image family always returns its latest image that is not deprecated. The name of the image family must comply with RFC1035.
*
*
* optional string family = 328751972;
*
* @return The family.
*/
@java.lang.Override
public java.lang.String getFamily() {
java.lang.Object ref = family_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
family_ = s;
return s;
}
}
/**
*
*
*
* The name of the image family to which this image belongs. The image family name can be from a publicly managed image family provided by Compute Engine, or from a custom image family you create. For example, centos-stream-9 is a publicly available image family. For more information, see Image family best practices. When creating disks, you can specify an image family instead of a specific image name. The image family always returns its latest image that is not deprecated. The name of the image family must comply with RFC1035.
*
*
* optional string family = 328751972;
*
* @return The bytes for family.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFamilyBytes() {
java.lang.Object ref = family_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
family_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GUEST_OS_FEATURES_FIELD_NUMBER = 79294545;
@SuppressWarnings("serial")
private java.util.List guestOsFeatures_;
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public java.util.List getGuestOsFeaturesList() {
return guestOsFeatures_;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
getGuestOsFeaturesOrBuilderList() {
return guestOsFeatures_;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public int getGuestOsFeaturesCount() {
return guestOsFeatures_.size();
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public com.google.cloud.compute.v1.GuestOsFeature getGuestOsFeatures(int index) {
return guestOsFeatures_.get(index);
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public com.google.cloud.compute.v1.GuestOsFeatureOrBuilder getGuestOsFeaturesOrBuilder(
int index) {
return guestOsFeatures_.get(index);
}
public static final int ID_FIELD_NUMBER = 3355;
private long id_ = 0L;
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int IMAGE_ENCRYPTION_KEY_FIELD_NUMBER = 379512583;
private com.google.cloud.compute.v1.CustomerEncryptionKey imageEncryptionKey_;
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*
* @return Whether the imageEncryptionKey field is set.
*/
@java.lang.Override
public boolean hasImageEncryptionKey() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*
* @return The imageEncryptionKey.
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKey getImageEncryptionKey() {
return imageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: imageEncryptionKey_;
}
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getImageEncryptionKeyOrBuilder() {
return imageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: imageEncryptionKey_;
}
public static final int KIND_FIELD_NUMBER = 3292052;
@SuppressWarnings("serial")
private volatile java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#image for images.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
@java.lang.Override
public boolean hasKind() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#image for images.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
@java.lang.Override
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#image for images.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LABEL_FINGERPRINT_FIELD_NUMBER = 178124825;
@SuppressWarnings("serial")
private volatile java.lang.Object labelFingerprint_ = "";
/**
*
*
*
* A fingerprint for the labels being applied to this image, which is essentially a hash of the labels used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an image.
*
*
* optional string label_fingerprint = 178124825;
*
* @return Whether the labelFingerprint field is set.
*/
@java.lang.Override
public boolean hasLabelFingerprint() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* A fingerprint for the labels being applied to this image, which is essentially a hash of the labels used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an image.
*
*
* optional string label_fingerprint = 178124825;
*
* @return The labelFingerprint.
*/
@java.lang.Override
public java.lang.String getLabelFingerprint() {
java.lang.Object ref = labelFingerprint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
labelFingerprint_ = s;
return s;
}
}
/**
*
*
*
* A fingerprint for the labels being applied to this image, which is essentially a hash of the labels used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an image.
*
*
* optional string label_fingerprint = 178124825;
*
* @return The bytes for labelFingerprint.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLabelFingerprintBytes() {
java.lang.Object ref = labelFingerprint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
labelFingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LABELS_FIELD_NUMBER = 500195327;
private static final class LabelsDefaultEntryHolder {
static final com.google.protobuf.MapEntry defaultEntry =
com.google.protobuf.MapEntry.newDefaultInstance(
com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Image_LabelsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField labels_;
private com.google.protobuf.MapField internalGetLabels() {
if (labels_ == null) {
return com.google.protobuf.MapField.emptyMapField(LabelsDefaultEntryHolder.defaultEntry);
}
return labels_;
}
public int getLabelsCount() {
return internalGetLabels().getMap().size();
}
/**
*
*
*
* Labels to apply to this image. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public boolean containsLabels(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetLabels().getMap().containsKey(key);
}
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getLabels() {
return getLabelsMap();
}
/**
*
*
*
* Labels to apply to this image. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public java.util.Map getLabelsMap() {
return internalGetLabels().getMap();
}
/**
*
*
*
* Labels to apply to this image. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public /* nullable */ java.lang.String getLabelsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Labels to apply to this image. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public java.lang.String getLabelsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int LICENSE_CODES_FIELD_NUMBER = 45482664;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.LongList licenseCodes_ = emptyLongList();
/**
*
*
*
* Integer license codes indicating which licenses are attached to this image.
*
*
* repeated int64 license_codes = 45482664;
*
* @return A list containing the licenseCodes.
*/
@java.lang.Override
public java.util.List getLicenseCodesList() {
return licenseCodes_;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this image.
*
*
* repeated int64 license_codes = 45482664;
*
* @return The count of licenseCodes.
*/
public int getLicenseCodesCount() {
return licenseCodes_.size();
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this image.
*
*
* repeated int64 license_codes = 45482664;
*
* @param index The index of the element to return.
* @return The licenseCodes at the given index.
*/
public long getLicenseCodes(int index) {
return licenseCodes_.getLong(index);
}
private int licenseCodesMemoizedSerializedSize = -1;
public static final int LICENSES_FIELD_NUMBER = 337642578;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList licenses_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @return A list containing the licenses.
*/
public com.google.protobuf.ProtocolStringList getLicensesList() {
return licenses_;
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @return The count of licenses.
*/
public int getLicensesCount() {
return licenses_.size();
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the element to return.
* @return The licenses at the given index.
*/
public java.lang.String getLicenses(int index) {
return licenses_.get(index);
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the value to return.
* @return The bytes of the licenses at the given index.
*/
public com.google.protobuf.ByteString getLicensesBytes(int index) {
return licenses_.getByteString(index);
}
public static final int NAME_FIELD_NUMBER = 3373707;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* Name of the resource; provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* Name of the resource; provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* Name of the resource; provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RAW_DISK_FIELD_NUMBER = 503113556;
private com.google.cloud.compute.v1.RawDisk rawDisk_;
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*
* @return Whether the rawDisk field is set.
*/
@java.lang.Override
public boolean hasRawDisk() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*
* @return The rawDisk.
*/
@java.lang.Override
public com.google.cloud.compute.v1.RawDisk getRawDisk() {
return rawDisk_ == null ? com.google.cloud.compute.v1.RawDisk.getDefaultInstance() : rawDisk_;
}
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*/
@java.lang.Override
public com.google.cloud.compute.v1.RawDiskOrBuilder getRawDiskOrBuilder() {
return rawDisk_ == null ? com.google.cloud.compute.v1.RawDisk.getDefaultInstance() : rawDisk_;
}
public static final int SATISFIES_PZI_FIELD_NUMBER = 480964257;
private boolean satisfiesPzi_ = false;
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @return Whether the satisfiesPzi field is set.
*/
@java.lang.Override
public boolean hasSatisfiesPzi() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @return The satisfiesPzi.
*/
@java.lang.Override
public boolean getSatisfiesPzi() {
return satisfiesPzi_;
}
public static final int SATISFIES_PZS_FIELD_NUMBER = 480964267;
private boolean satisfiesPzs_ = false;
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return Whether the satisfiesPzs field is set.
*/
@java.lang.Override
public boolean hasSatisfiesPzs() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return The satisfiesPzs.
*/
@java.lang.Override
public boolean getSatisfiesPzs() {
return satisfiesPzs_;
}
public static final int SELF_LINK_FIELD_NUMBER = 456214797;
@SuppressWarnings("serial")
private volatile java.lang.Object selfLink_ = "";
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return Whether the selfLink field is set.
*/
@java.lang.Override
public boolean hasSelfLink() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The selfLink.
*/
@java.lang.Override
public java.lang.String getSelfLink() {
java.lang.Object ref = selfLink_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLink_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The bytes for selfLink.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSelfLinkBytes() {
java.lang.Object ref = selfLink_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLink_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SHIELDED_INSTANCE_INITIAL_STATE_FIELD_NUMBER = 192356867;
private com.google.cloud.compute.v1.InitialStateConfig shieldedInstanceInitialState_;
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*
* @return Whether the shieldedInstanceInitialState field is set.
*/
@java.lang.Override
public boolean hasShieldedInstanceInitialState() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*
* @return The shieldedInstanceInitialState.
*/
@java.lang.Override
public com.google.cloud.compute.v1.InitialStateConfig getShieldedInstanceInitialState() {
return shieldedInstanceInitialState_ == null
? com.google.cloud.compute.v1.InitialStateConfig.getDefaultInstance()
: shieldedInstanceInitialState_;
}
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.InitialStateConfigOrBuilder
getShieldedInstanceInitialStateOrBuilder() {
return shieldedInstanceInitialState_ == null
? com.google.cloud.compute.v1.InitialStateConfig.getDefaultInstance()
: shieldedInstanceInitialState_;
}
public static final int SOURCE_DISK_FIELD_NUMBER = 451753793;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceDisk_ = "";
/**
*
*
*
* URL of the source disk used to create this image. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - projects/project/zones/zone/disks/disk - zones/zone/disks/disk In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_disk = 451753793;
*
* @return Whether the sourceDisk field is set.
*/
@java.lang.Override
public boolean hasSourceDisk() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
*
*
* URL of the source disk used to create this image. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - projects/project/zones/zone/disks/disk - zones/zone/disks/disk In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_disk = 451753793;
*
* @return The sourceDisk.
*/
@java.lang.Override
public java.lang.String getSourceDisk() {
java.lang.Object ref = sourceDisk_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceDisk_ = s;
return s;
}
}
/**
*
*
*
* URL of the source disk used to create this image. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - projects/project/zones/zone/disks/disk - zones/zone/disks/disk In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_disk = 451753793;
*
* @return The bytes for sourceDisk.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceDiskBytes() {
java.lang.Object ref = sourceDisk_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceDisk_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_DISK_ENCRYPTION_KEY_FIELD_NUMBER = 531501153;
private com.google.cloud.compute.v1.CustomerEncryptionKey sourceDiskEncryptionKey_;
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*
* @return Whether the sourceDiskEncryptionKey field is set.
*/
@java.lang.Override
public boolean hasSourceDiskEncryptionKey() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*
* @return The sourceDiskEncryptionKey.
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKey getSourceDiskEncryptionKey() {
return sourceDiskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceDiskEncryptionKey_;
}
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getSourceDiskEncryptionKeyOrBuilder() {
return sourceDiskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceDiskEncryptionKey_;
}
public static final int SOURCE_DISK_ID_FIELD_NUMBER = 454190809;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceDiskId_ = "";
/**
*
*
*
* [Output Only] The ID value of the disk used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given disk name.
*
*
* optional string source_disk_id = 454190809;
*
* @return Whether the sourceDiskId field is set.
*/
@java.lang.Override
public boolean hasSourceDiskId() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
*
*
* [Output Only] The ID value of the disk used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given disk name.
*
*
* optional string source_disk_id = 454190809;
*
* @return The sourceDiskId.
*/
@java.lang.Override
public java.lang.String getSourceDiskId() {
java.lang.Object ref = sourceDiskId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceDiskId_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The ID value of the disk used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given disk name.
*
*
* optional string source_disk_id = 454190809;
*
* @return The bytes for sourceDiskId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceDiskIdBytes() {
java.lang.Object ref = sourceDiskId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceDiskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_IMAGE_FIELD_NUMBER = 50443319;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceImage_ = "";
/**
*
*
*
* URL of the source image used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ images/image_name - projects/project_id/global/images/image_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_image = 50443319;
*
* @return Whether the sourceImage field is set.
*/
@java.lang.Override
public boolean hasSourceImage() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
*
*
*
* URL of the source image used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ images/image_name - projects/project_id/global/images/image_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_image = 50443319;
*
* @return The sourceImage.
*/
@java.lang.Override
public java.lang.String getSourceImage() {
java.lang.Object ref = sourceImage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceImage_ = s;
return s;
}
}
/**
*
*
*
* URL of the source image used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ images/image_name - projects/project_id/global/images/image_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_image = 50443319;
*
* @return The bytes for sourceImage.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceImageBytes() {
java.lang.Object ref = sourceImage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceImage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_IMAGE_ENCRYPTION_KEY_FIELD_NUMBER = 381503659;
private com.google.cloud.compute.v1.CustomerEncryptionKey sourceImageEncryptionKey_;
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*
* @return Whether the sourceImageEncryptionKey field is set.
*/
@java.lang.Override
public boolean hasSourceImageEncryptionKey() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*
* @return The sourceImageEncryptionKey.
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKey getSourceImageEncryptionKey() {
return sourceImageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceImageEncryptionKey_;
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getSourceImageEncryptionKeyOrBuilder() {
return sourceImageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceImageEncryptionKey_;
}
public static final int SOURCE_IMAGE_ID_FIELD_NUMBER = 55328291;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceImageId_ = "";
/**
*
*
*
* [Output Only] The ID value of the image used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given image name.
*
*
* optional string source_image_id = 55328291;
*
* @return Whether the sourceImageId field is set.
*/
@java.lang.Override
public boolean hasSourceImageId() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given image name.
*
*
* optional string source_image_id = 55328291;
*
* @return The sourceImageId.
*/
@java.lang.Override
public java.lang.String getSourceImageId() {
java.lang.Object ref = sourceImageId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceImageId_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given image name.
*
*
* optional string source_image_id = 55328291;
*
* @return The bytes for sourceImageId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceImageIdBytes() {
java.lang.Object ref = sourceImageId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceImageId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_SNAPSHOT_FIELD_NUMBER = 126061928;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceSnapshot_ = "";
/**
*
*
*
* URL of the source snapshot used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ snapshots/snapshot_name - projects/project_id/global/snapshots/snapshot_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_snapshot = 126061928;
*
* @return Whether the sourceSnapshot field is set.
*/
@java.lang.Override
public boolean hasSourceSnapshot() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
*
*
*
* URL of the source snapshot used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ snapshots/snapshot_name - projects/project_id/global/snapshots/snapshot_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_snapshot = 126061928;
*
* @return The sourceSnapshot.
*/
@java.lang.Override
public java.lang.String getSourceSnapshot() {
java.lang.Object ref = sourceSnapshot_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceSnapshot_ = s;
return s;
}
}
/**
*
*
*
* URL of the source snapshot used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ snapshots/snapshot_name - projects/project_id/global/snapshots/snapshot_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_snapshot = 126061928;
*
* @return The bytes for sourceSnapshot.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceSnapshotBytes() {
java.lang.Object ref = sourceSnapshot_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceSnapshot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_SNAPSHOT_ENCRYPTION_KEY_FIELD_NUMBER = 303679322;
private com.google.cloud.compute.v1.CustomerEncryptionKey sourceSnapshotEncryptionKey_;
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*
* @return Whether the sourceSnapshotEncryptionKey field is set.
*/
@java.lang.Override
public boolean hasSourceSnapshotEncryptionKey() {
return ((bitField0_ & 0x02000000) != 0);
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*
* @return The sourceSnapshotEncryptionKey.
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKey getSourceSnapshotEncryptionKey() {
return sourceSnapshotEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceSnapshotEncryptionKey_;
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getSourceSnapshotEncryptionKeyOrBuilder() {
return sourceSnapshotEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceSnapshotEncryptionKey_;
}
public static final int SOURCE_SNAPSHOT_ID_FIELD_NUMBER = 98962258;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceSnapshotId_ = "";
/**
*
*
*
* [Output Only] The ID value of the snapshot used to create this image. This value may be used to determine whether the snapshot was taken from the current or a previous instance of a given snapshot name.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return Whether the sourceSnapshotId field is set.
*/
@java.lang.Override
public boolean hasSourceSnapshotId() {
return ((bitField0_ & 0x04000000) != 0);
}
/**
*
*
*
* [Output Only] The ID value of the snapshot used to create this image. This value may be used to determine whether the snapshot was taken from the current or a previous instance of a given snapshot name.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return The sourceSnapshotId.
*/
@java.lang.Override
public java.lang.String getSourceSnapshotId() {
java.lang.Object ref = sourceSnapshotId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceSnapshotId_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The ID value of the snapshot used to create this image. This value may be used to determine whether the snapshot was taken from the current or a previous instance of a given snapshot name.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return The bytes for sourceSnapshotId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceSnapshotIdBytes() {
java.lang.Object ref = sourceSnapshotId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceSnapshotId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_TYPE_FIELD_NUMBER = 452245726;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceType_ = "";
/**
*
*
*
* The type of the image used to create this disk. The default and only valid value is RAW.
* Check the SourceType enum for the list of possible values.
*
*
* optional string source_type = 452245726;
*
* @return Whether the sourceType field is set.
*/
@java.lang.Override
public boolean hasSourceType() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
*
*
*
* The type of the image used to create this disk. The default and only valid value is RAW.
* Check the SourceType enum for the list of possible values.
*
*
* optional string source_type = 452245726;
*
* @return The sourceType.
*/
@java.lang.Override
public java.lang.String getSourceType() {
java.lang.Object ref = sourceType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceType_ = s;
return s;
}
}
/**
*
*
*
* The type of the image used to create this disk. The default and only valid value is RAW.
* Check the SourceType enum for the list of possible values.
*
*
* optional string source_type = 452245726;
*
* @return The bytes for sourceType.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceTypeBytes() {
java.lang.Object ref = sourceType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 181260274;
@SuppressWarnings("serial")
private volatile java.lang.Object status_ = "";
/**
*
*
*
* [Output Only] The status of the image. An image can be used to create other resources, such as instances, only after the image has been successfully created and the status is set to READY. Possible values are FAILED, PENDING, or READY.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return ((bitField0_ & 0x10000000) != 0);
}
/**
*
*
*
* [Output Only] The status of the image. An image can be used to create other resources, such as instances, only after the image has been successfully created and the status is set to READY. Possible values are FAILED, PENDING, or READY.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The status.
*/
@java.lang.Override
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
status_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The status of the image. An image can be used to create other resources, such as instances, only after the image has been successfully created and the status is set to READY. Possible values are FAILED, PENDING, or READY.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The bytes for status.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STORAGE_LOCATIONS_FIELD_NUMBER = 328005274;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList storageLocations_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @return A list containing the storageLocations.
*/
public com.google.protobuf.ProtocolStringList getStorageLocationsList() {
return storageLocations_;
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @return The count of storageLocations.
*/
public int getStorageLocationsCount() {
return storageLocations_.size();
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @param index The index of the element to return.
* @return The storageLocations at the given index.
*/
public java.lang.String getStorageLocations(int index) {
return storageLocations_.get(index);
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @param index The index of the value to return.
* @return The bytes of the storageLocations at the given index.
*/
public com.google.protobuf.ByteString getStorageLocationsBytes(int index) {
return storageLocations_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000100) != 0)) {
output.writeUInt64(3355, id_);
}
if (((bitField0_ & 0x00000400) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3292052, kind_);
}
if (((bitField0_ & 0x00001000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3373707, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30525366, creationTimestamp_);
}
if (getLicenseCodesList().size() > 0) {
output.writeUInt32NoTag(363861314);
output.writeUInt32NoTag(licenseCodesMemoizedSerializedSize);
}
for (int i = 0; i < licenseCodes_.size(); i++) {
output.writeInt64NoTag(licenseCodes_.getLong(i));
}
if (((bitField0_ & 0x00200000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 50443319, sourceImage_);
}
if (((bitField0_ & 0x00800000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 55328291, sourceImageId_);
}
for (int i = 0; i < guestOsFeatures_.size(); i++) {
output.writeMessage(79294545, guestOsFeatures_.get(i));
}
if (((bitField0_ & 0x04000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 98962258, sourceSnapshotId_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeBool(102135228, enableConfidentialCompute_);
}
if (((bitField0_ & 0x01000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 126061928, sourceSnapshot_);
}
if (((bitField0_ & 0x00000800) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 178124825, labelFingerprint_);
}
if (((bitField0_ & 0x10000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 181260274, status_);
}
if (((bitField0_ & 0x00020000) != 0)) {
output.writeMessage(192356867, getShieldedInstanceInitialState());
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 302803283, architecture_);
}
if (((bitField0_ & 0x02000000) != 0)) {
output.writeMessage(303679322, getSourceSnapshotEncryptionKey());
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt64(316263735, diskSizeGb_);
}
for (int i = 0; i < storageLocations_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 328005274, storageLocations_.getRaw(i));
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 328751972, family_);
}
for (int i = 0; i < licenses_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 337642578, licenses_.getRaw(i));
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeMessage(379512583, getImageEncryptionKey());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(381093450, archiveSizeBytes_);
}
if (((bitField0_ & 0x00400000) != 0)) {
output.writeMessage(381503659, getSourceImageEncryptionKey());
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 422937596, description_);
}
if (((bitField0_ & 0x00040000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 451753793, sourceDisk_);
}
if (((bitField0_ & 0x08000000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 452245726, sourceType_);
}
if (((bitField0_ & 0x00100000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 454190809, sourceDiskId_);
}
if (((bitField0_ & 0x00010000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 456214797, selfLink_);
}
if (((bitField0_ & 0x00004000) != 0)) {
output.writeBool(480964257, satisfiesPzi_);
}
if (((bitField0_ & 0x00008000) != 0)) {
output.writeBool(480964267, satisfiesPzs_);
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetLabels(), LabelsDefaultEntryHolder.defaultEntry, 500195327);
if (((bitField0_ & 0x00002000) != 0)) {
output.writeMessage(503113556, getRawDisk());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(515138995, getDeprecated());
}
if (((bitField0_ & 0x00080000) != 0)) {
output.writeMessage(531501153, getSourceDiskEncryptionKey());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeUInt64Size(3355, id_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3292052, kind_);
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3373707, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(30525366, creationTimestamp_);
}
{
int dataSize = 0;
for (int i = 0; i < licenseCodes_.size(); i++) {
dataSize +=
com.google.protobuf.CodedOutputStream.computeInt64SizeNoTag(licenseCodes_.getLong(i));
}
size += dataSize;
if (!getLicenseCodesList().isEmpty()) {
size += 5;
size += com.google.protobuf.CodedOutputStream.computeInt32SizeNoTag(dataSize);
}
licenseCodesMemoizedSerializedSize = dataSize;
}
if (((bitField0_ & 0x00200000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(50443319, sourceImage_);
}
if (((bitField0_ & 0x00800000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(55328291, sourceImageId_);
}
for (int i = 0; i < guestOsFeatures_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
79294545, guestOsFeatures_.get(i));
}
if (((bitField0_ & 0x04000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(98962258, sourceSnapshotId_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeBoolSize(
102135228, enableConfidentialCompute_);
}
if (((bitField0_ & 0x01000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(126061928, sourceSnapshot_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(178124825, labelFingerprint_);
}
if (((bitField0_ & 0x10000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(181260274, status_);
}
if (((bitField0_ & 0x00020000) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
192356867, getShieldedInstanceInitialState());
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(302803283, architecture_);
}
if (((bitField0_ & 0x02000000) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
303679322, getSourceSnapshotEncryptionKey());
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(316263735, diskSizeGb_);
}
{
int dataSize = 0;
for (int i = 0; i < storageLocations_.size(); i++) {
dataSize += computeStringSizeNoTag(storageLocations_.getRaw(i));
}
size += dataSize;
size += 5 * getStorageLocationsList().size();
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(328751972, family_);
}
{
int dataSize = 0;
for (int i = 0; i < licenses_.size(); i++) {
dataSize += computeStringSizeNoTag(licenses_.getRaw(i));
}
size += dataSize;
size += 5 * getLicensesList().size();
}
if (((bitField0_ & 0x00000200) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
379512583, getImageEncryptionKey());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(381093450, archiveSizeBytes_);
}
if (((bitField0_ & 0x00400000) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
381503659, getSourceImageEncryptionKey());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(422937596, description_);
}
if (((bitField0_ & 0x00040000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(451753793, sourceDisk_);
}
if (((bitField0_ & 0x08000000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(452245726, sourceType_);
}
if (((bitField0_ & 0x00100000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(454190809, sourceDiskId_);
}
if (((bitField0_ & 0x00010000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(456214797, selfLink_);
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(480964257, satisfiesPzi_);
}
if (((bitField0_ & 0x00008000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(480964267, satisfiesPzs_);
}
for (java.util.Map.Entry entry :
internalGetLabels().getMap().entrySet()) {
com.google.protobuf.MapEntry labels__ =
LabelsDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(500195327, labels__);
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(503113556, getRawDisk());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(515138995, getDeprecated());
}
if (((bitField0_ & 0x00080000) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
531501153, getSourceDiskEncryptionKey());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.Image)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.Image other = (com.google.cloud.compute.v1.Image) obj;
if (hasArchitecture() != other.hasArchitecture()) return false;
if (hasArchitecture()) {
if (!getArchitecture().equals(other.getArchitecture())) return false;
}
if (hasArchiveSizeBytes() != other.hasArchiveSizeBytes()) return false;
if (hasArchiveSizeBytes()) {
if (getArchiveSizeBytes() != other.getArchiveSizeBytes()) return false;
}
if (hasCreationTimestamp() != other.hasCreationTimestamp()) return false;
if (hasCreationTimestamp()) {
if (!getCreationTimestamp().equals(other.getCreationTimestamp())) return false;
}
if (hasDeprecated() != other.hasDeprecated()) return false;
if (hasDeprecated()) {
if (!getDeprecated().equals(other.getDeprecated())) return false;
}
if (hasDescription() != other.hasDescription()) return false;
if (hasDescription()) {
if (!getDescription().equals(other.getDescription())) return false;
}
if (hasDiskSizeGb() != other.hasDiskSizeGb()) return false;
if (hasDiskSizeGb()) {
if (getDiskSizeGb() != other.getDiskSizeGb()) return false;
}
if (hasEnableConfidentialCompute() != other.hasEnableConfidentialCompute()) return false;
if (hasEnableConfidentialCompute()) {
if (getEnableConfidentialCompute() != other.getEnableConfidentialCompute()) return false;
}
if (hasFamily() != other.hasFamily()) return false;
if (hasFamily()) {
if (!getFamily().equals(other.getFamily())) return false;
}
if (!getGuestOsFeaturesList().equals(other.getGuestOsFeaturesList())) return false;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId() != other.getId()) return false;
}
if (hasImageEncryptionKey() != other.hasImageEncryptionKey()) return false;
if (hasImageEncryptionKey()) {
if (!getImageEncryptionKey().equals(other.getImageEncryptionKey())) return false;
}
if (hasKind() != other.hasKind()) return false;
if (hasKind()) {
if (!getKind().equals(other.getKind())) return false;
}
if (hasLabelFingerprint() != other.hasLabelFingerprint()) return false;
if (hasLabelFingerprint()) {
if (!getLabelFingerprint().equals(other.getLabelFingerprint())) return false;
}
if (!internalGetLabels().equals(other.internalGetLabels())) return false;
if (!getLicenseCodesList().equals(other.getLicenseCodesList())) return false;
if (!getLicensesList().equals(other.getLicensesList())) return false;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName().equals(other.getName())) return false;
}
if (hasRawDisk() != other.hasRawDisk()) return false;
if (hasRawDisk()) {
if (!getRawDisk().equals(other.getRawDisk())) return false;
}
if (hasSatisfiesPzi() != other.hasSatisfiesPzi()) return false;
if (hasSatisfiesPzi()) {
if (getSatisfiesPzi() != other.getSatisfiesPzi()) return false;
}
if (hasSatisfiesPzs() != other.hasSatisfiesPzs()) return false;
if (hasSatisfiesPzs()) {
if (getSatisfiesPzs() != other.getSatisfiesPzs()) return false;
}
if (hasSelfLink() != other.hasSelfLink()) return false;
if (hasSelfLink()) {
if (!getSelfLink().equals(other.getSelfLink())) return false;
}
if (hasShieldedInstanceInitialState() != other.hasShieldedInstanceInitialState()) return false;
if (hasShieldedInstanceInitialState()) {
if (!getShieldedInstanceInitialState().equals(other.getShieldedInstanceInitialState()))
return false;
}
if (hasSourceDisk() != other.hasSourceDisk()) return false;
if (hasSourceDisk()) {
if (!getSourceDisk().equals(other.getSourceDisk())) return false;
}
if (hasSourceDiskEncryptionKey() != other.hasSourceDiskEncryptionKey()) return false;
if (hasSourceDiskEncryptionKey()) {
if (!getSourceDiskEncryptionKey().equals(other.getSourceDiskEncryptionKey())) return false;
}
if (hasSourceDiskId() != other.hasSourceDiskId()) return false;
if (hasSourceDiskId()) {
if (!getSourceDiskId().equals(other.getSourceDiskId())) return false;
}
if (hasSourceImage() != other.hasSourceImage()) return false;
if (hasSourceImage()) {
if (!getSourceImage().equals(other.getSourceImage())) return false;
}
if (hasSourceImageEncryptionKey() != other.hasSourceImageEncryptionKey()) return false;
if (hasSourceImageEncryptionKey()) {
if (!getSourceImageEncryptionKey().equals(other.getSourceImageEncryptionKey())) return false;
}
if (hasSourceImageId() != other.hasSourceImageId()) return false;
if (hasSourceImageId()) {
if (!getSourceImageId().equals(other.getSourceImageId())) return false;
}
if (hasSourceSnapshot() != other.hasSourceSnapshot()) return false;
if (hasSourceSnapshot()) {
if (!getSourceSnapshot().equals(other.getSourceSnapshot())) return false;
}
if (hasSourceSnapshotEncryptionKey() != other.hasSourceSnapshotEncryptionKey()) return false;
if (hasSourceSnapshotEncryptionKey()) {
if (!getSourceSnapshotEncryptionKey().equals(other.getSourceSnapshotEncryptionKey()))
return false;
}
if (hasSourceSnapshotId() != other.hasSourceSnapshotId()) return false;
if (hasSourceSnapshotId()) {
if (!getSourceSnapshotId().equals(other.getSourceSnapshotId())) return false;
}
if (hasSourceType() != other.hasSourceType()) return false;
if (hasSourceType()) {
if (!getSourceType().equals(other.getSourceType())) return false;
}
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus().equals(other.getStatus())) return false;
}
if (!getStorageLocationsList().equals(other.getStorageLocationsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasArchitecture()) {
hash = (37 * hash) + ARCHITECTURE_FIELD_NUMBER;
hash = (53 * hash) + getArchitecture().hashCode();
}
if (hasArchiveSizeBytes()) {
hash = (37 * hash) + ARCHIVE_SIZE_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getArchiveSizeBytes());
}
if (hasCreationTimestamp()) {
hash = (37 * hash) + CREATION_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getCreationTimestamp().hashCode();
}
if (hasDeprecated()) {
hash = (37 * hash) + DEPRECATED_FIELD_NUMBER;
hash = (53 * hash) + getDeprecated().hashCode();
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
if (hasDiskSizeGb()) {
hash = (37 * hash) + DISK_SIZE_GB_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getDiskSizeGb());
}
if (hasEnableConfidentialCompute()) {
hash = (37 * hash) + ENABLE_CONFIDENTIAL_COMPUTE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getEnableConfidentialCompute());
}
if (hasFamily()) {
hash = (37 * hash) + FAMILY_FIELD_NUMBER;
hash = (53 * hash) + getFamily().hashCode();
}
if (getGuestOsFeaturesCount() > 0) {
hash = (37 * hash) + GUEST_OS_FEATURES_FIELD_NUMBER;
hash = (53 * hash) + getGuestOsFeaturesList().hashCode();
}
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getId());
}
if (hasImageEncryptionKey()) {
hash = (37 * hash) + IMAGE_ENCRYPTION_KEY_FIELD_NUMBER;
hash = (53 * hash) + getImageEncryptionKey().hashCode();
}
if (hasKind()) {
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + getKind().hashCode();
}
if (hasLabelFingerprint()) {
hash = (37 * hash) + LABEL_FINGERPRINT_FIELD_NUMBER;
hash = (53 * hash) + getLabelFingerprint().hashCode();
}
if (!internalGetLabels().getMap().isEmpty()) {
hash = (37 * hash) + LABELS_FIELD_NUMBER;
hash = (53 * hash) + internalGetLabels().hashCode();
}
if (getLicenseCodesCount() > 0) {
hash = (37 * hash) + LICENSE_CODES_FIELD_NUMBER;
hash = (53 * hash) + getLicenseCodesList().hashCode();
}
if (getLicensesCount() > 0) {
hash = (37 * hash) + LICENSES_FIELD_NUMBER;
hash = (53 * hash) + getLicensesList().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasRawDisk()) {
hash = (37 * hash) + RAW_DISK_FIELD_NUMBER;
hash = (53 * hash) + getRawDisk().hashCode();
}
if (hasSatisfiesPzi()) {
hash = (37 * hash) + SATISFIES_PZI_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getSatisfiesPzi());
}
if (hasSatisfiesPzs()) {
hash = (37 * hash) + SATISFIES_PZS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getSatisfiesPzs());
}
if (hasSelfLink()) {
hash = (37 * hash) + SELF_LINK_FIELD_NUMBER;
hash = (53 * hash) + getSelfLink().hashCode();
}
if (hasShieldedInstanceInitialState()) {
hash = (37 * hash) + SHIELDED_INSTANCE_INITIAL_STATE_FIELD_NUMBER;
hash = (53 * hash) + getShieldedInstanceInitialState().hashCode();
}
if (hasSourceDisk()) {
hash = (37 * hash) + SOURCE_DISK_FIELD_NUMBER;
hash = (53 * hash) + getSourceDisk().hashCode();
}
if (hasSourceDiskEncryptionKey()) {
hash = (37 * hash) + SOURCE_DISK_ENCRYPTION_KEY_FIELD_NUMBER;
hash = (53 * hash) + getSourceDiskEncryptionKey().hashCode();
}
if (hasSourceDiskId()) {
hash = (37 * hash) + SOURCE_DISK_ID_FIELD_NUMBER;
hash = (53 * hash) + getSourceDiskId().hashCode();
}
if (hasSourceImage()) {
hash = (37 * hash) + SOURCE_IMAGE_FIELD_NUMBER;
hash = (53 * hash) + getSourceImage().hashCode();
}
if (hasSourceImageEncryptionKey()) {
hash = (37 * hash) + SOURCE_IMAGE_ENCRYPTION_KEY_FIELD_NUMBER;
hash = (53 * hash) + getSourceImageEncryptionKey().hashCode();
}
if (hasSourceImageId()) {
hash = (37 * hash) + SOURCE_IMAGE_ID_FIELD_NUMBER;
hash = (53 * hash) + getSourceImageId().hashCode();
}
if (hasSourceSnapshot()) {
hash = (37 * hash) + SOURCE_SNAPSHOT_FIELD_NUMBER;
hash = (53 * hash) + getSourceSnapshot().hashCode();
}
if (hasSourceSnapshotEncryptionKey()) {
hash = (37 * hash) + SOURCE_SNAPSHOT_ENCRYPTION_KEY_FIELD_NUMBER;
hash = (53 * hash) + getSourceSnapshotEncryptionKey().hashCode();
}
if (hasSourceSnapshotId()) {
hash = (37 * hash) + SOURCE_SNAPSHOT_ID_FIELD_NUMBER;
hash = (53 * hash) + getSourceSnapshotId().hashCode();
}
if (hasSourceType()) {
hash = (37 * hash) + SOURCE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getSourceType().hashCode();
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (getStorageLocationsCount() > 0) {
hash = (37 * hash) + STORAGE_LOCATIONS_FIELD_NUMBER;
hash = (53 * hash) + getStorageLocationsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.Image parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Image parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Image parseFrom(com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Image parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Image parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Image parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Image parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Image parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.Image parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Image parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.Image parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Image parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.Image prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Represents an Image resource. You can use images to create boot disks for your VM instances. For more information, read Images.
*
*
* Protobuf type {@code google.cloud.compute.v1.Image}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.Image)
com.google.cloud.compute.v1.ImageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Image_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 500195327:
return internalGetLabels();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 500195327:
return internalGetMutableLabels();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Image_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.Image.class,
com.google.cloud.compute.v1.Image.Builder.class);
}
// Construct using com.google.cloud.compute.v1.Image.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getDeprecatedFieldBuilder();
getGuestOsFeaturesFieldBuilder();
getImageEncryptionKeyFieldBuilder();
getRawDiskFieldBuilder();
getShieldedInstanceInitialStateFieldBuilder();
getSourceDiskEncryptionKeyFieldBuilder();
getSourceImageEncryptionKeyFieldBuilder();
getSourceSnapshotEncryptionKeyFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
bitField1_ = 0;
architecture_ = "";
archiveSizeBytes_ = 0L;
creationTimestamp_ = "";
deprecated_ = null;
if (deprecatedBuilder_ != null) {
deprecatedBuilder_.dispose();
deprecatedBuilder_ = null;
}
description_ = "";
diskSizeGb_ = 0L;
enableConfidentialCompute_ = false;
family_ = "";
if (guestOsFeaturesBuilder_ == null) {
guestOsFeatures_ = java.util.Collections.emptyList();
} else {
guestOsFeatures_ = null;
guestOsFeaturesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
id_ = 0L;
imageEncryptionKey_ = null;
if (imageEncryptionKeyBuilder_ != null) {
imageEncryptionKeyBuilder_.dispose();
imageEncryptionKeyBuilder_ = null;
}
kind_ = "";
labelFingerprint_ = "";
internalGetMutableLabels().clear();
licenseCodes_ = emptyLongList();
licenses_ = com.google.protobuf.LazyStringArrayList.emptyList();
name_ = "";
rawDisk_ = null;
if (rawDiskBuilder_ != null) {
rawDiskBuilder_.dispose();
rawDiskBuilder_ = null;
}
satisfiesPzi_ = false;
satisfiesPzs_ = false;
selfLink_ = "";
shieldedInstanceInitialState_ = null;
if (shieldedInstanceInitialStateBuilder_ != null) {
shieldedInstanceInitialStateBuilder_.dispose();
shieldedInstanceInitialStateBuilder_ = null;
}
sourceDisk_ = "";
sourceDiskEncryptionKey_ = null;
if (sourceDiskEncryptionKeyBuilder_ != null) {
sourceDiskEncryptionKeyBuilder_.dispose();
sourceDiskEncryptionKeyBuilder_ = null;
}
sourceDiskId_ = "";
sourceImage_ = "";
sourceImageEncryptionKey_ = null;
if (sourceImageEncryptionKeyBuilder_ != null) {
sourceImageEncryptionKeyBuilder_.dispose();
sourceImageEncryptionKeyBuilder_ = null;
}
sourceImageId_ = "";
sourceSnapshot_ = "";
sourceSnapshotEncryptionKey_ = null;
if (sourceSnapshotEncryptionKeyBuilder_ != null) {
sourceSnapshotEncryptionKeyBuilder_.dispose();
sourceSnapshotEncryptionKeyBuilder_ = null;
}
sourceSnapshotId_ = "";
sourceType_ = "";
status_ = "";
storageLocations_ = com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Image_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.Image getDefaultInstanceForType() {
return com.google.cloud.compute.v1.Image.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.Image build() {
com.google.cloud.compute.v1.Image result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.Image buildPartial() {
com.google.cloud.compute.v1.Image result = new com.google.cloud.compute.v1.Image(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
if (bitField1_ != 0) {
buildPartial1(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.cloud.compute.v1.Image result) {
if (guestOsFeaturesBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)) {
guestOsFeatures_ = java.util.Collections.unmodifiableList(guestOsFeatures_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.guestOsFeatures_ = guestOsFeatures_;
} else {
result.guestOsFeatures_ = guestOsFeaturesBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.compute.v1.Image result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.architecture_ = architecture_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.archiveSizeBytes_ = archiveSizeBytes_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.creationTimestamp_ = creationTimestamp_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.deprecated_ = deprecatedBuilder_ == null ? deprecated_ : deprecatedBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.description_ = description_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.diskSizeGb_ = diskSizeGb_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.enableConfidentialCompute_ = enableConfidentialCompute_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.family_ = family_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.imageEncryptionKey_ =
imageEncryptionKeyBuilder_ == null
? imageEncryptionKey_
: imageEncryptionKeyBuilder_.build();
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.kind_ = kind_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.labelFingerprint_ = labelFingerprint_;
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.labels_ = internalGetLabels();
result.labels_.makeImmutable();
}
if (((from_bitField0_ & 0x00004000) != 0)) {
licenseCodes_.makeImmutable();
result.licenseCodes_ = licenseCodes_;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
licenses_.makeImmutable();
result.licenses_ = licenses_;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00001000;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.rawDisk_ = rawDiskBuilder_ == null ? rawDisk_ : rawDiskBuilder_.build();
to_bitField0_ |= 0x00002000;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.satisfiesPzi_ = satisfiesPzi_;
to_bitField0_ |= 0x00004000;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.satisfiesPzs_ = satisfiesPzs_;
to_bitField0_ |= 0x00008000;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.selfLink_ = selfLink_;
to_bitField0_ |= 0x00010000;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.shieldedInstanceInitialState_ =
shieldedInstanceInitialStateBuilder_ == null
? shieldedInstanceInitialState_
: shieldedInstanceInitialStateBuilder_.build();
to_bitField0_ |= 0x00020000;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.sourceDisk_ = sourceDisk_;
to_bitField0_ |= 0x00040000;
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.sourceDiskEncryptionKey_ =
sourceDiskEncryptionKeyBuilder_ == null
? sourceDiskEncryptionKey_
: sourceDiskEncryptionKeyBuilder_.build();
to_bitField0_ |= 0x00080000;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
result.sourceDiskId_ = sourceDiskId_;
to_bitField0_ |= 0x00100000;
}
if (((from_bitField0_ & 0x02000000) != 0)) {
result.sourceImage_ = sourceImage_;
to_bitField0_ |= 0x00200000;
}
if (((from_bitField0_ & 0x04000000) != 0)) {
result.sourceImageEncryptionKey_ =
sourceImageEncryptionKeyBuilder_ == null
? sourceImageEncryptionKey_
: sourceImageEncryptionKeyBuilder_.build();
to_bitField0_ |= 0x00400000;
}
if (((from_bitField0_ & 0x08000000) != 0)) {
result.sourceImageId_ = sourceImageId_;
to_bitField0_ |= 0x00800000;
}
if (((from_bitField0_ & 0x10000000) != 0)) {
result.sourceSnapshot_ = sourceSnapshot_;
to_bitField0_ |= 0x01000000;
}
if (((from_bitField0_ & 0x20000000) != 0)) {
result.sourceSnapshotEncryptionKey_ =
sourceSnapshotEncryptionKeyBuilder_ == null
? sourceSnapshotEncryptionKey_
: sourceSnapshotEncryptionKeyBuilder_.build();
to_bitField0_ |= 0x02000000;
}
if (((from_bitField0_ & 0x40000000) != 0)) {
result.sourceSnapshotId_ = sourceSnapshotId_;
to_bitField0_ |= 0x04000000;
}
if (((from_bitField0_ & 0x80000000) != 0)) {
result.sourceType_ = sourceType_;
to_bitField0_ |= 0x08000000;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartial1(com.google.cloud.compute.v1.Image result) {
int from_bitField1_ = bitField1_;
int to_bitField0_ = 0;
if (((from_bitField1_ & 0x00000001) != 0)) {
result.status_ = status_;
to_bitField0_ |= 0x10000000;
}
if (((from_bitField1_ & 0x00000002) != 0)) {
storageLocations_.makeImmutable();
result.storageLocations_ = storageLocations_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.Image) {
return mergeFrom((com.google.cloud.compute.v1.Image) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.Image other) {
if (other == com.google.cloud.compute.v1.Image.getDefaultInstance()) return this;
if (other.hasArchitecture()) {
architecture_ = other.architecture_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasArchiveSizeBytes()) {
setArchiveSizeBytes(other.getArchiveSizeBytes());
}
if (other.hasCreationTimestamp()) {
creationTimestamp_ = other.creationTimestamp_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasDeprecated()) {
mergeDeprecated(other.getDeprecated());
}
if (other.hasDescription()) {
description_ = other.description_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasDiskSizeGb()) {
setDiskSizeGb(other.getDiskSizeGb());
}
if (other.hasEnableConfidentialCompute()) {
setEnableConfidentialCompute(other.getEnableConfidentialCompute());
}
if (other.hasFamily()) {
family_ = other.family_;
bitField0_ |= 0x00000080;
onChanged();
}
if (guestOsFeaturesBuilder_ == null) {
if (!other.guestOsFeatures_.isEmpty()) {
if (guestOsFeatures_.isEmpty()) {
guestOsFeatures_ = other.guestOsFeatures_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.addAll(other.guestOsFeatures_);
}
onChanged();
}
} else {
if (!other.guestOsFeatures_.isEmpty()) {
if (guestOsFeaturesBuilder_.isEmpty()) {
guestOsFeaturesBuilder_.dispose();
guestOsFeaturesBuilder_ = null;
guestOsFeatures_ = other.guestOsFeatures_;
bitField0_ = (bitField0_ & ~0x00000100);
guestOsFeaturesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getGuestOsFeaturesFieldBuilder()
: null;
} else {
guestOsFeaturesBuilder_.addAllMessages(other.guestOsFeatures_);
}
}
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasImageEncryptionKey()) {
mergeImageEncryptionKey(other.getImageEncryptionKey());
}
if (other.hasKind()) {
kind_ = other.kind_;
bitField0_ |= 0x00000800;
onChanged();
}
if (other.hasLabelFingerprint()) {
labelFingerprint_ = other.labelFingerprint_;
bitField0_ |= 0x00001000;
onChanged();
}
internalGetMutableLabels().mergeFrom(other.internalGetLabels());
bitField0_ |= 0x00002000;
if (!other.licenseCodes_.isEmpty()) {
if (licenseCodes_.isEmpty()) {
licenseCodes_ = other.licenseCodes_;
licenseCodes_.makeImmutable();
bitField0_ |= 0x00004000;
} else {
ensureLicenseCodesIsMutable();
licenseCodes_.addAll(other.licenseCodes_);
}
onChanged();
}
if (!other.licenses_.isEmpty()) {
if (licenses_.isEmpty()) {
licenses_ = other.licenses_;
bitField0_ |= 0x00008000;
} else {
ensureLicensesIsMutable();
licenses_.addAll(other.licenses_);
}
onChanged();
}
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00010000;
onChanged();
}
if (other.hasRawDisk()) {
mergeRawDisk(other.getRawDisk());
}
if (other.hasSatisfiesPzi()) {
setSatisfiesPzi(other.getSatisfiesPzi());
}
if (other.hasSatisfiesPzs()) {
setSatisfiesPzs(other.getSatisfiesPzs());
}
if (other.hasSelfLink()) {
selfLink_ = other.selfLink_;
bitField0_ |= 0x00100000;
onChanged();
}
if (other.hasShieldedInstanceInitialState()) {
mergeShieldedInstanceInitialState(other.getShieldedInstanceInitialState());
}
if (other.hasSourceDisk()) {
sourceDisk_ = other.sourceDisk_;
bitField0_ |= 0x00400000;
onChanged();
}
if (other.hasSourceDiskEncryptionKey()) {
mergeSourceDiskEncryptionKey(other.getSourceDiskEncryptionKey());
}
if (other.hasSourceDiskId()) {
sourceDiskId_ = other.sourceDiskId_;
bitField0_ |= 0x01000000;
onChanged();
}
if (other.hasSourceImage()) {
sourceImage_ = other.sourceImage_;
bitField0_ |= 0x02000000;
onChanged();
}
if (other.hasSourceImageEncryptionKey()) {
mergeSourceImageEncryptionKey(other.getSourceImageEncryptionKey());
}
if (other.hasSourceImageId()) {
sourceImageId_ = other.sourceImageId_;
bitField0_ |= 0x08000000;
onChanged();
}
if (other.hasSourceSnapshot()) {
sourceSnapshot_ = other.sourceSnapshot_;
bitField0_ |= 0x10000000;
onChanged();
}
if (other.hasSourceSnapshotEncryptionKey()) {
mergeSourceSnapshotEncryptionKey(other.getSourceSnapshotEncryptionKey());
}
if (other.hasSourceSnapshotId()) {
sourceSnapshotId_ = other.sourceSnapshotId_;
bitField0_ |= 0x40000000;
onChanged();
}
if (other.hasSourceType()) {
sourceType_ = other.sourceType_;
bitField0_ |= 0x80000000;
onChanged();
}
if (other.hasStatus()) {
status_ = other.status_;
bitField1_ |= 0x00000001;
onChanged();
}
if (!other.storageLocations_.isEmpty()) {
if (storageLocations_.isEmpty()) {
storageLocations_ = other.storageLocations_;
bitField1_ |= 0x00000002;
} else {
ensureStorageLocationsIsMutable();
storageLocations_.addAll(other.storageLocations_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 26840:
{
id_ = input.readUInt64();
bitField0_ |= 0x00000200;
break;
} // case 26840
case 26336418:
{
kind_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000800;
break;
} // case 26336418
case 26989658:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00010000;
break;
} // case 26989658
case 244202930:
{
creationTimestamp_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 244202930
case 363861312:
{
long v = input.readInt64();
ensureLicenseCodesIsMutable();
licenseCodes_.addLong(v);
break;
} // case 363861312
case 363861314:
{
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensureLicenseCodesIsMutable();
while (input.getBytesUntilLimit() > 0) {
licenseCodes_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
} // case 363861314
case 403546554:
{
sourceImage_ = input.readStringRequireUtf8();
bitField0_ |= 0x02000000;
break;
} // case 403546554
case 442626330:
{
sourceImageId_ = input.readStringRequireUtf8();
bitField0_ |= 0x08000000;
break;
} // case 442626330
case 634356362:
{
com.google.cloud.compute.v1.GuestOsFeature m =
input.readMessage(
com.google.cloud.compute.v1.GuestOsFeature.parser(), extensionRegistry);
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(m);
} else {
guestOsFeaturesBuilder_.addMessage(m);
}
break;
} // case 634356362
case 791698066:
{
sourceSnapshotId_ = input.readStringRequireUtf8();
bitField0_ |= 0x40000000;
break;
} // case 791698066
case 817081824:
{
enableConfidentialCompute_ = input.readBool();
bitField0_ |= 0x00000040;
break;
} // case 817081824
case 1008495426:
{
sourceSnapshot_ = input.readStringRequireUtf8();
bitField0_ |= 0x10000000;
break;
} // case 1008495426
case 1424998602:
{
labelFingerprint_ = input.readStringRequireUtf8();
bitField0_ |= 0x00001000;
break;
} // case 1424998602
case 1450082194:
{
status_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000001;
break;
} // case 1450082194
case 1538854938:
{
input.readMessage(
getShieldedInstanceInitialStateFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00200000;
break;
} // case 1538854938
case -1872541030:
{
architecture_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case -1872541030
case -1865532718:
{
input.readMessage(
getSourceSnapshotEncryptionKeyFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x20000000;
break;
} // case -1865532718
case -1764857416:
{
diskSizeGb_ = input.readInt64();
bitField0_ |= 0x00000020;
break;
} // case -1764857416
case -1670925102:
{
java.lang.String s = input.readStringRequireUtf8();
ensureStorageLocationsIsMutable();
storageLocations_.add(s);
break;
} // case -1670925102
case -1664951518:
{
family_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case -1664951518
case -1593826670:
{
java.lang.String s = input.readStringRequireUtf8();
ensureLicensesIsMutable();
licenses_.add(s);
break;
} // case -1593826670
case -1258866630:
{
input.readMessage(
getImageEncryptionKeyFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000400;
break;
} // case -1258866630
case -1246219696:
{
archiveSizeBytes_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case -1246219696
case -1242938022:
{
input.readMessage(
getSourceImageEncryptionKeyFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x04000000;
break;
} // case -1242938022
case -911466526:
{
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case -911466526
case -680936950:
{
sourceDisk_ = input.readStringRequireUtf8();
bitField0_ |= 0x00400000;
break;
} // case -680936950
case -677001486:
{
sourceType_ = input.readStringRequireUtf8();
bitField0_ |= 0x80000000;
break;
} // case -677001486
case -661440822:
{
sourceDiskId_ = input.readStringRequireUtf8();
bitField0_ |= 0x01000000;
break;
} // case -661440822
case -645248918:
{
selfLink_ = input.readStringRequireUtf8();
bitField0_ |= 0x00100000;
break;
} // case -645248918
case -447253240:
{
satisfiesPzi_ = input.readBool();
bitField0_ |= 0x00040000;
break;
} // case -447253240
case -447253160:
{
satisfiesPzs_ = input.readBool();
bitField0_ |= 0x00080000;
break;
} // case -447253160
case -293404678:
{
com.google.protobuf.MapEntry labels__ =
input.readMessage(
LabelsDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableLabels()
.getMutableMap()
.put(labels__.getKey(), labels__.getValue());
bitField0_ |= 0x00002000;
break;
} // case -293404678
case -270058846:
{
input.readMessage(getRawDiskFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00020000;
break;
} // case -270058846
case -173855334:
{
input.readMessage(getDeprecatedFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case -173855334
case -42958070:
{
input.readMessage(
getSourceDiskEncryptionKeyFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00800000;
break;
} // case -42958070
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int bitField1_;
private java.lang.Object architecture_ = "";
/**
*
*
*
* The architecture of the image. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return Whether the architecture field is set.
*/
public boolean hasArchitecture() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The architecture of the image. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return The architecture.
*/
public java.lang.String getArchitecture() {
java.lang.Object ref = architecture_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
architecture_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The architecture of the image. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return The bytes for architecture.
*/
public com.google.protobuf.ByteString getArchitectureBytes() {
java.lang.Object ref = architecture_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
architecture_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The architecture of the image. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @param value The architecture to set.
* @return This builder for chaining.
*/
public Builder setArchitecture(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
architecture_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The architecture of the image. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @return This builder for chaining.
*/
public Builder clearArchitecture() {
architecture_ = getDefaultInstance().getArchitecture();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* The architecture of the image. Valid values are ARM64 or X86_64.
* Check the Architecture enum for the list of possible values.
*
*
* optional string architecture = 302803283;
*
* @param value The bytes for architecture to set.
* @return This builder for chaining.
*/
public Builder setArchitectureBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
architecture_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private long archiveSizeBytes_;
/**
*
*
*
* Size of the image tar.gz archive stored in Google Cloud Storage (in bytes).
*
*
* optional int64 archive_size_bytes = 381093450;
*
* @return Whether the archiveSizeBytes field is set.
*/
@java.lang.Override
public boolean hasArchiveSizeBytes() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Size of the image tar.gz archive stored in Google Cloud Storage (in bytes).
*
*
* optional int64 archive_size_bytes = 381093450;
*
* @return The archiveSizeBytes.
*/
@java.lang.Override
public long getArchiveSizeBytes() {
return archiveSizeBytes_;
}
/**
*
*
*
* Size of the image tar.gz archive stored in Google Cloud Storage (in bytes).
*
*
* optional int64 archive_size_bytes = 381093450;
*
* @param value The archiveSizeBytes to set.
* @return This builder for chaining.
*/
public Builder setArchiveSizeBytes(long value) {
archiveSizeBytes_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Size of the image tar.gz archive stored in Google Cloud Storage (in bytes).
*
*
* optional int64 archive_size_bytes = 381093450;
*
* @return This builder for chaining.
*/
public Builder clearArchiveSizeBytes() {
bitField0_ = (bitField0_ & ~0x00000002);
archiveSizeBytes_ = 0L;
onChanged();
return this;
}
private java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestamp(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
creationTimestamp_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return This builder for chaining.
*/
public Builder clearCreationTimestamp() {
creationTimestamp_ = getDefaultInstance().getCreationTimestamp();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The bytes for creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestampBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
creationTimestamp_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.google.cloud.compute.v1.DeprecationStatus deprecated_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DeprecationStatus,
com.google.cloud.compute.v1.DeprecationStatus.Builder,
com.google.cloud.compute.v1.DeprecationStatusOrBuilder>
deprecatedBuilder_;
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*
* @return Whether the deprecated field is set.
*/
public boolean hasDeprecated() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*
* @return The deprecated.
*/
public com.google.cloud.compute.v1.DeprecationStatus getDeprecated() {
if (deprecatedBuilder_ == null) {
return deprecated_ == null
? com.google.cloud.compute.v1.DeprecationStatus.getDefaultInstance()
: deprecated_;
} else {
return deprecatedBuilder_.getMessage();
}
}
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*/
public Builder setDeprecated(com.google.cloud.compute.v1.DeprecationStatus value) {
if (deprecatedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
deprecated_ = value;
} else {
deprecatedBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*/
public Builder setDeprecated(
com.google.cloud.compute.v1.DeprecationStatus.Builder builderForValue) {
if (deprecatedBuilder_ == null) {
deprecated_ = builderForValue.build();
} else {
deprecatedBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*/
public Builder mergeDeprecated(com.google.cloud.compute.v1.DeprecationStatus value) {
if (deprecatedBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& deprecated_ != null
&& deprecated_ != com.google.cloud.compute.v1.DeprecationStatus.getDefaultInstance()) {
getDeprecatedBuilder().mergeFrom(value);
} else {
deprecated_ = value;
}
} else {
deprecatedBuilder_.mergeFrom(value);
}
if (deprecated_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*/
public Builder clearDeprecated() {
bitField0_ = (bitField0_ & ~0x00000008);
deprecated_ = null;
if (deprecatedBuilder_ != null) {
deprecatedBuilder_.dispose();
deprecatedBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*/
public com.google.cloud.compute.v1.DeprecationStatus.Builder getDeprecatedBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getDeprecatedFieldBuilder().getBuilder();
}
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*/
public com.google.cloud.compute.v1.DeprecationStatusOrBuilder getDeprecatedOrBuilder() {
if (deprecatedBuilder_ != null) {
return deprecatedBuilder_.getMessageOrBuilder();
} else {
return deprecated_ == null
? com.google.cloud.compute.v1.DeprecationStatus.getDefaultInstance()
: deprecated_;
}
}
/**
*
*
*
* The deprecation status associated with this image.
*
*
* optional .google.cloud.compute.v1.DeprecationStatus deprecated = 515138995;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DeprecationStatus,
com.google.cloud.compute.v1.DeprecationStatus.Builder,
com.google.cloud.compute.v1.DeprecationStatusOrBuilder>
getDeprecatedFieldBuilder() {
if (deprecatedBuilder_ == null) {
deprecatedBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.DeprecationStatus,
com.google.cloud.compute.v1.DeprecationStatus.Builder,
com.google.cloud.compute.v1.DeprecationStatusOrBuilder>(
getDeprecated(), getParentForChildren(), isClean());
deprecated_ = null;
}
return deprecatedBuilder_;
}
private java.lang.Object description_ = "";
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return Whether the description field is set.
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The bytes for description.
*/
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private long diskSizeGb_;
/**
*
*
*
* Size of the image when restored onto a persistent disk (in GB).
*
*
* optional int64 disk_size_gb = 316263735;
*
* @return Whether the diskSizeGb field is set.
*/
@java.lang.Override
public boolean hasDiskSizeGb() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Size of the image when restored onto a persistent disk (in GB).
*
*
* optional int64 disk_size_gb = 316263735;
*
* @return The diskSizeGb.
*/
@java.lang.Override
public long getDiskSizeGb() {
return diskSizeGb_;
}
/**
*
*
*
* Size of the image when restored onto a persistent disk (in GB).
*
*
* optional int64 disk_size_gb = 316263735;
*
* @param value The diskSizeGb to set.
* @return This builder for chaining.
*/
public Builder setDiskSizeGb(long value) {
diskSizeGb_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Size of the image when restored onto a persistent disk (in GB).
*
*
* optional int64 disk_size_gb = 316263735;
*
* @return This builder for chaining.
*/
public Builder clearDiskSizeGb() {
bitField0_ = (bitField0_ & ~0x00000020);
diskSizeGb_ = 0L;
onChanged();
return this;
}
private boolean enableConfidentialCompute_;
/**
*
*
*
* Whether this image is created from a confidential compute mode disk. [Output Only]: This field is not set by user, but from source disk.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @return Whether the enableConfidentialCompute field is set.
*/
@java.lang.Override
public boolean hasEnableConfidentialCompute() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Whether this image is created from a confidential compute mode disk. [Output Only]: This field is not set by user, but from source disk.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @return The enableConfidentialCompute.
*/
@java.lang.Override
public boolean getEnableConfidentialCompute() {
return enableConfidentialCompute_;
}
/**
*
*
*
* Whether this image is created from a confidential compute mode disk. [Output Only]: This field is not set by user, but from source disk.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @param value The enableConfidentialCompute to set.
* @return This builder for chaining.
*/
public Builder setEnableConfidentialCompute(boolean value) {
enableConfidentialCompute_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Whether this image is created from a confidential compute mode disk. [Output Only]: This field is not set by user, but from source disk.
*
*
* optional bool enable_confidential_compute = 102135228;
*
* @return This builder for chaining.
*/
public Builder clearEnableConfidentialCompute() {
bitField0_ = (bitField0_ & ~0x00000040);
enableConfidentialCompute_ = false;
onChanged();
return this;
}
private java.lang.Object family_ = "";
/**
*
*
*
* The name of the image family to which this image belongs. The image family name can be from a publicly managed image family provided by Compute Engine, or from a custom image family you create. For example, centos-stream-9 is a publicly available image family. For more information, see Image family best practices. When creating disks, you can specify an image family instead of a specific image name. The image family always returns its latest image that is not deprecated. The name of the image family must comply with RFC1035.
*
*
* optional string family = 328751972;
*
* @return Whether the family field is set.
*/
public boolean hasFamily() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The name of the image family to which this image belongs. The image family name can be from a publicly managed image family provided by Compute Engine, or from a custom image family you create. For example, centos-stream-9 is a publicly available image family. For more information, see Image family best practices. When creating disks, you can specify an image family instead of a specific image name. The image family always returns its latest image that is not deprecated. The name of the image family must comply with RFC1035.
*
*
* optional string family = 328751972;
*
* @return The family.
*/
public java.lang.String getFamily() {
java.lang.Object ref = family_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
family_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The name of the image family to which this image belongs. The image family name can be from a publicly managed image family provided by Compute Engine, or from a custom image family you create. For example, centos-stream-9 is a publicly available image family. For more information, see Image family best practices. When creating disks, you can specify an image family instead of a specific image name. The image family always returns its latest image that is not deprecated. The name of the image family must comply with RFC1035.
*
*
* optional string family = 328751972;
*
* @return The bytes for family.
*/
public com.google.protobuf.ByteString getFamilyBytes() {
java.lang.Object ref = family_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
family_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The name of the image family to which this image belongs. The image family name can be from a publicly managed image family provided by Compute Engine, or from a custom image family you create. For example, centos-stream-9 is a publicly available image family. For more information, see Image family best practices. When creating disks, you can specify an image family instead of a specific image name. The image family always returns its latest image that is not deprecated. The name of the image family must comply with RFC1035.
*
*
* optional string family = 328751972;
*
* @param value The family to set.
* @return This builder for chaining.
*/
public Builder setFamily(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
family_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* The name of the image family to which this image belongs. The image family name can be from a publicly managed image family provided by Compute Engine, or from a custom image family you create. For example, centos-stream-9 is a publicly available image family. For more information, see Image family best practices. When creating disks, you can specify an image family instead of a specific image name. The image family always returns its latest image that is not deprecated. The name of the image family must comply with RFC1035.
*
*
* optional string family = 328751972;
*
* @return This builder for chaining.
*/
public Builder clearFamily() {
family_ = getDefaultInstance().getFamily();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
*
*
* The name of the image family to which this image belongs. The image family name can be from a publicly managed image family provided by Compute Engine, or from a custom image family you create. For example, centos-stream-9 is a publicly available image family. For more information, see Image family best practices. When creating disks, you can specify an image family instead of a specific image name. The image family always returns its latest image that is not deprecated. The name of the image family must comply with RFC1035.
*
*
* optional string family = 328751972;
*
* @param value The bytes for family to set.
* @return This builder for chaining.
*/
public Builder setFamilyBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
family_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private java.util.List guestOsFeatures_ =
java.util.Collections.emptyList();
private void ensureGuestOsFeaturesIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
guestOsFeatures_ =
new java.util.ArrayList(guestOsFeatures_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.GuestOsFeature,
com.google.cloud.compute.v1.GuestOsFeature.Builder,
com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
guestOsFeaturesBuilder_;
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public java.util.List getGuestOsFeaturesList() {
if (guestOsFeaturesBuilder_ == null) {
return java.util.Collections.unmodifiableList(guestOsFeatures_);
} else {
return guestOsFeaturesBuilder_.getMessageList();
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public int getGuestOsFeaturesCount() {
if (guestOsFeaturesBuilder_ == null) {
return guestOsFeatures_.size();
} else {
return guestOsFeaturesBuilder_.getCount();
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature getGuestOsFeatures(int index) {
if (guestOsFeaturesBuilder_ == null) {
return guestOsFeatures_.get(index);
} else {
return guestOsFeaturesBuilder_.getMessage(index);
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder setGuestOsFeatures(int index, com.google.cloud.compute.v1.GuestOsFeature value) {
if (guestOsFeaturesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.set(index, value);
onChanged();
} else {
guestOsFeaturesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder setGuestOsFeatures(
int index, com.google.cloud.compute.v1.GuestOsFeature.Builder builderForValue) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.set(index, builderForValue.build());
onChanged();
} else {
guestOsFeaturesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(com.google.cloud.compute.v1.GuestOsFeature value) {
if (guestOsFeaturesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(value);
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(int index, com.google.cloud.compute.v1.GuestOsFeature value) {
if (guestOsFeaturesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(index, value);
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(
com.google.cloud.compute.v1.GuestOsFeature.Builder builderForValue) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(builderForValue.build());
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(
int index, com.google.cloud.compute.v1.GuestOsFeature.Builder builderForValue) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(index, builderForValue.build());
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addAllGuestOsFeatures(
java.lang.Iterable extends com.google.cloud.compute.v1.GuestOsFeature> values) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, guestOsFeatures_);
onChanged();
} else {
guestOsFeaturesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder clearGuestOsFeatures() {
if (guestOsFeaturesBuilder_ == null) {
guestOsFeatures_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
guestOsFeaturesBuilder_.clear();
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder removeGuestOsFeatures(int index) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.remove(index);
onChanged();
} else {
guestOsFeaturesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature.Builder getGuestOsFeaturesBuilder(int index) {
return getGuestOsFeaturesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeatureOrBuilder getGuestOsFeaturesOrBuilder(
int index) {
if (guestOsFeaturesBuilder_ == null) {
return guestOsFeatures_.get(index);
} else {
return guestOsFeaturesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public java.util.List extends com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
getGuestOsFeaturesOrBuilderList() {
if (guestOsFeaturesBuilder_ != null) {
return guestOsFeaturesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(guestOsFeatures_);
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature.Builder addGuestOsFeaturesBuilder() {
return getGuestOsFeaturesFieldBuilder()
.addBuilder(com.google.cloud.compute.v1.GuestOsFeature.getDefaultInstance());
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature.Builder addGuestOsFeaturesBuilder(int index) {
return getGuestOsFeaturesFieldBuilder()
.addBuilder(index, com.google.cloud.compute.v1.GuestOsFeature.getDefaultInstance());
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public java.util.List
getGuestOsFeaturesBuilderList() {
return getGuestOsFeaturesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.GuestOsFeature,
com.google.cloud.compute.v1.GuestOsFeature.Builder,
com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
getGuestOsFeaturesFieldBuilder() {
if (guestOsFeaturesBuilder_ == null) {
guestOsFeaturesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.GuestOsFeature,
com.google.cloud.compute.v1.GuestOsFeature.Builder,
com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>(
guestOsFeatures_,
((bitField0_ & 0x00000100) != 0),
getParentForChildren(),
isClean());
guestOsFeatures_ = null;
}
return guestOsFeaturesBuilder_;
}
private long id_;
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
id_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000200);
id_ = 0L;
onChanged();
return this;
}
private com.google.cloud.compute.v1.CustomerEncryptionKey imageEncryptionKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
imageEncryptionKeyBuilder_;
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*
* @return Whether the imageEncryptionKey field is set.
*/
public boolean hasImageEncryptionKey() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*
* @return The imageEncryptionKey.
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey getImageEncryptionKey() {
if (imageEncryptionKeyBuilder_ == null) {
return imageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: imageEncryptionKey_;
} else {
return imageEncryptionKeyBuilder_.getMessage();
}
}
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*/
public Builder setImageEncryptionKey(com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (imageEncryptionKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
imageEncryptionKey_ = value;
} else {
imageEncryptionKeyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*/
public Builder setImageEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder builderForValue) {
if (imageEncryptionKeyBuilder_ == null) {
imageEncryptionKey_ = builderForValue.build();
} else {
imageEncryptionKeyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*/
public Builder mergeImageEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (imageEncryptionKeyBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0)
&& imageEncryptionKey_ != null
&& imageEncryptionKey_
!= com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()) {
getImageEncryptionKeyBuilder().mergeFrom(value);
} else {
imageEncryptionKey_ = value;
}
} else {
imageEncryptionKeyBuilder_.mergeFrom(value);
}
if (imageEncryptionKey_ != null) {
bitField0_ |= 0x00000400;
onChanged();
}
return this;
}
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*/
public Builder clearImageEncryptionKey() {
bitField0_ = (bitField0_ & ~0x00000400);
imageEncryptionKey_ = null;
if (imageEncryptionKeyBuilder_ != null) {
imageEncryptionKeyBuilder_.dispose();
imageEncryptionKeyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey.Builder
getImageEncryptionKeyBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getImageEncryptionKeyFieldBuilder().getBuilder();
}
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getImageEncryptionKeyOrBuilder() {
if (imageEncryptionKeyBuilder_ != null) {
return imageEncryptionKeyBuilder_.getMessageOrBuilder();
} else {
return imageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: imageEncryptionKey_;
}
}
/**
*
*
*
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey image_encryption_key = 379512583;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
getImageEncryptionKeyFieldBuilder() {
if (imageEncryptionKeyBuilder_ == null) {
imageEncryptionKeyBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>(
getImageEncryptionKey(), getParentForChildren(), isClean());
imageEncryptionKey_ = null;
}
return imageEncryptionKeyBuilder_;
}
private java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#image for images.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
public boolean hasKind() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#image for images.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#image for images.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#image for images.
*
*
* optional string kind = 3292052;
*
* @param value The kind to set.
* @return This builder for chaining.
*/
public Builder setKind(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#image for images.
*
*
* optional string kind = 3292052;
*
* @return This builder for chaining.
*/
public Builder clearKind() {
kind_ = getDefaultInstance().getKind();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#image for images.
*
*
* optional string kind = 3292052;
*
* @param value The bytes for kind to set.
* @return This builder for chaining.
*/
public Builder setKindBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
kind_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
private java.lang.Object labelFingerprint_ = "";
/**
*
*
*
* A fingerprint for the labels being applied to this image, which is essentially a hash of the labels used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an image.
*
*
* optional string label_fingerprint = 178124825;
*
* @return Whether the labelFingerprint field is set.
*/
public boolean hasLabelFingerprint() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* A fingerprint for the labels being applied to this image, which is essentially a hash of the labels used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an image.
*
*
* optional string label_fingerprint = 178124825;
*
* @return The labelFingerprint.
*/
public java.lang.String getLabelFingerprint() {
java.lang.Object ref = labelFingerprint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
labelFingerprint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* A fingerprint for the labels being applied to this image, which is essentially a hash of the labels used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an image.
*
*
* optional string label_fingerprint = 178124825;
*
* @return The bytes for labelFingerprint.
*/
public com.google.protobuf.ByteString getLabelFingerprintBytes() {
java.lang.Object ref = labelFingerprint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
labelFingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* A fingerprint for the labels being applied to this image, which is essentially a hash of the labels used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an image.
*
*
* optional string label_fingerprint = 178124825;
*
* @param value The labelFingerprint to set.
* @return This builder for chaining.
*/
public Builder setLabelFingerprint(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
labelFingerprint_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* A fingerprint for the labels being applied to this image, which is essentially a hash of the labels used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an image.
*
*
* optional string label_fingerprint = 178124825;
*
* @return This builder for chaining.
*/
public Builder clearLabelFingerprint() {
labelFingerprint_ = getDefaultInstance().getLabelFingerprint();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
return this;
}
/**
*
*
*
* A fingerprint for the labels being applied to this image, which is essentially a hash of the labels used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an image.
*
*
* optional string label_fingerprint = 178124825;
*
* @param value The bytes for labelFingerprint to set.
* @return This builder for chaining.
*/
public Builder setLabelFingerprintBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
labelFingerprint_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
private com.google.protobuf.MapField labels_;
private com.google.protobuf.MapField internalGetLabels() {
if (labels_ == null) {
return com.google.protobuf.MapField.emptyMapField(LabelsDefaultEntryHolder.defaultEntry);
}
return labels_;
}
private com.google.protobuf.MapField
internalGetMutableLabels() {
if (labels_ == null) {
labels_ = com.google.protobuf.MapField.newMapField(LabelsDefaultEntryHolder.defaultEntry);
}
if (!labels_.isMutable()) {
labels_ = labels_.copy();
}
bitField0_ |= 0x00002000;
onChanged();
return labels_;
}
public int getLabelsCount() {
return internalGetLabels().getMap().size();
}
/**
*
*
*
* Labels to apply to this image. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public boolean containsLabels(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetLabels().getMap().containsKey(key);
}
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getLabels() {
return getLabelsMap();
}
/**
*
*
*
* Labels to apply to this image. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public java.util.Map getLabelsMap() {
return internalGetLabels().getMap();
}
/**
*
*
*
* Labels to apply to this image. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public /* nullable */ java.lang.String getLabelsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Labels to apply to this image. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
@java.lang.Override
public java.lang.String getLabelsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearLabels() {
bitField0_ = (bitField0_ & ~0x00002000);
internalGetMutableLabels().getMutableMap().clear();
return this;
}
/**
*
*
*
* Labels to apply to this image. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
public Builder removeLabels(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableLabels().getMutableMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map getMutableLabels() {
bitField0_ |= 0x00002000;
return internalGetMutableLabels().getMutableMap();
}
/**
*
*
*
* Labels to apply to this image. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
public Builder putLabels(java.lang.String key, java.lang.String value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableLabels().getMutableMap().put(key, value);
bitField0_ |= 0x00002000;
return this;
}
/**
*
*
*
* Labels to apply to this image. These can be later modified by the setLabels method.
*
*
* map<string, string> labels = 500195327;
*/
public Builder putAllLabels(java.util.Map values) {
internalGetMutableLabels().getMutableMap().putAll(values);
bitField0_ |= 0x00002000;
return this;
}
private com.google.protobuf.Internal.LongList licenseCodes_ = emptyLongList();
private void ensureLicenseCodesIsMutable() {
if (!licenseCodes_.isModifiable()) {
licenseCodes_ = makeMutableCopy(licenseCodes_);
}
bitField0_ |= 0x00004000;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this image.
*
*
* repeated int64 license_codes = 45482664;
*
* @return A list containing the licenseCodes.
*/
public java.util.List getLicenseCodesList() {
licenseCodes_.makeImmutable();
return licenseCodes_;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this image.
*
*
* repeated int64 license_codes = 45482664;
*
* @return The count of licenseCodes.
*/
public int getLicenseCodesCount() {
return licenseCodes_.size();
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this image.
*
*
* repeated int64 license_codes = 45482664;
*
* @param index The index of the element to return.
* @return The licenseCodes at the given index.
*/
public long getLicenseCodes(int index) {
return licenseCodes_.getLong(index);
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this image.
*
*
* repeated int64 license_codes = 45482664;
*
* @param index The index to set the value at.
* @param value The licenseCodes to set.
* @return This builder for chaining.
*/
public Builder setLicenseCodes(int index, long value) {
ensureLicenseCodesIsMutable();
licenseCodes_.setLong(index, value);
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this image.
*
*
* repeated int64 license_codes = 45482664;
*
* @param value The licenseCodes to add.
* @return This builder for chaining.
*/
public Builder addLicenseCodes(long value) {
ensureLicenseCodesIsMutable();
licenseCodes_.addLong(value);
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this image.
*
*
* repeated int64 license_codes = 45482664;
*
* @param values The licenseCodes to add.
* @return This builder for chaining.
*/
public Builder addAllLicenseCodes(java.lang.Iterable extends java.lang.Long> values) {
ensureLicenseCodesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, licenseCodes_);
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* Integer license codes indicating which licenses are attached to this image.
*
*
* repeated int64 license_codes = 45482664;
*
* @return This builder for chaining.
*/
public Builder clearLicenseCodes() {
licenseCodes_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00004000);
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList licenses_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureLicensesIsMutable() {
if (!licenses_.isModifiable()) {
licenses_ = new com.google.protobuf.LazyStringArrayList(licenses_);
}
bitField0_ |= 0x00008000;
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @return A list containing the licenses.
*/
public com.google.protobuf.ProtocolStringList getLicensesList() {
licenses_.makeImmutable();
return licenses_;
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @return The count of licenses.
*/
public int getLicensesCount() {
return licenses_.size();
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the element to return.
* @return The licenses at the given index.
*/
public java.lang.String getLicenses(int index) {
return licenses_.get(index);
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the value to return.
* @return The bytes of the licenses at the given index.
*/
public com.google.protobuf.ByteString getLicensesBytes(int index) {
return licenses_.getByteString(index);
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @param index The index to set the value at.
* @param value The licenses to set.
* @return This builder for chaining.
*/
public Builder setLicenses(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLicensesIsMutable();
licenses_.set(index, value);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @param value The licenses to add.
* @return This builder for chaining.
*/
public Builder addLicenses(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLicensesIsMutable();
licenses_.add(value);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @param values The licenses to add.
* @return This builder for chaining.
*/
public Builder addAllLicenses(java.lang.Iterable values) {
ensureLicensesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, licenses_);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @return This builder for chaining.
*/
public Builder clearLicenses() {
licenses_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00008000);
;
onChanged();
return this;
}
/**
*
*
*
* Any applicable license URI.
*
*
* repeated string licenses = 337642578;
*
* @param value The bytes of the licenses to add.
* @return This builder for chaining.
*/
public Builder addLicensesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureLicensesIsMutable();
licenses_.add(value);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
*
*
* Name of the resource; provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
*
*
* Name of the resource; provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Name of the resource; provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Name of the resource; provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* Name of the resource; provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00010000);
onChanged();
return this;
}
/**
*
*
*
* Name of the resource; provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
private com.google.cloud.compute.v1.RawDisk rawDisk_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.RawDisk,
com.google.cloud.compute.v1.RawDisk.Builder,
com.google.cloud.compute.v1.RawDiskOrBuilder>
rawDiskBuilder_;
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*
* @return Whether the rawDisk field is set.
*/
public boolean hasRawDisk() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*
* @return The rawDisk.
*/
public com.google.cloud.compute.v1.RawDisk getRawDisk() {
if (rawDiskBuilder_ == null) {
return rawDisk_ == null
? com.google.cloud.compute.v1.RawDisk.getDefaultInstance()
: rawDisk_;
} else {
return rawDiskBuilder_.getMessage();
}
}
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*/
public Builder setRawDisk(com.google.cloud.compute.v1.RawDisk value) {
if (rawDiskBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rawDisk_ = value;
} else {
rawDiskBuilder_.setMessage(value);
}
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*/
public Builder setRawDisk(com.google.cloud.compute.v1.RawDisk.Builder builderForValue) {
if (rawDiskBuilder_ == null) {
rawDisk_ = builderForValue.build();
} else {
rawDiskBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*/
public Builder mergeRawDisk(com.google.cloud.compute.v1.RawDisk value) {
if (rawDiskBuilder_ == null) {
if (((bitField0_ & 0x00020000) != 0)
&& rawDisk_ != null
&& rawDisk_ != com.google.cloud.compute.v1.RawDisk.getDefaultInstance()) {
getRawDiskBuilder().mergeFrom(value);
} else {
rawDisk_ = value;
}
} else {
rawDiskBuilder_.mergeFrom(value);
}
if (rawDisk_ != null) {
bitField0_ |= 0x00020000;
onChanged();
}
return this;
}
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*/
public Builder clearRawDisk() {
bitField0_ = (bitField0_ & ~0x00020000);
rawDisk_ = null;
if (rawDiskBuilder_ != null) {
rawDiskBuilder_.dispose();
rawDiskBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*/
public com.google.cloud.compute.v1.RawDisk.Builder getRawDiskBuilder() {
bitField0_ |= 0x00020000;
onChanged();
return getRawDiskFieldBuilder().getBuilder();
}
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*/
public com.google.cloud.compute.v1.RawDiskOrBuilder getRawDiskOrBuilder() {
if (rawDiskBuilder_ != null) {
return rawDiskBuilder_.getMessageOrBuilder();
} else {
return rawDisk_ == null
? com.google.cloud.compute.v1.RawDisk.getDefaultInstance()
: rawDisk_;
}
}
/**
*
*
*
* The parameters of the raw disk image.
*
*
* optional .google.cloud.compute.v1.RawDisk raw_disk = 503113556;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.RawDisk,
com.google.cloud.compute.v1.RawDisk.Builder,
com.google.cloud.compute.v1.RawDiskOrBuilder>
getRawDiskFieldBuilder() {
if (rawDiskBuilder_ == null) {
rawDiskBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.RawDisk,
com.google.cloud.compute.v1.RawDisk.Builder,
com.google.cloud.compute.v1.RawDiskOrBuilder>(
getRawDisk(), getParentForChildren(), isClean());
rawDisk_ = null;
}
return rawDiskBuilder_;
}
private boolean satisfiesPzi_;
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @return Whether the satisfiesPzi field is set.
*/
@java.lang.Override
public boolean hasSatisfiesPzi() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @return The satisfiesPzi.
*/
@java.lang.Override
public boolean getSatisfiesPzi() {
return satisfiesPzi_;
}
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @param value The satisfiesPzi to set.
* @return This builder for chaining.
*/
public Builder setSatisfiesPzi(boolean value) {
satisfiesPzi_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
*
*
* Output only. Reserved for future use.
*
*
* optional bool satisfies_pzi = 480964257;
*
* @return This builder for chaining.
*/
public Builder clearSatisfiesPzi() {
bitField0_ = (bitField0_ & ~0x00040000);
satisfiesPzi_ = false;
onChanged();
return this;
}
private boolean satisfiesPzs_;
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return Whether the satisfiesPzs field is set.
*/
@java.lang.Override
public boolean hasSatisfiesPzs() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return The satisfiesPzs.
*/
@java.lang.Override
public boolean getSatisfiesPzs() {
return satisfiesPzs_;
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @param value The satisfiesPzs to set.
* @return This builder for chaining.
*/
public Builder setSatisfiesPzs(boolean value) {
satisfiesPzs_ = value;
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return This builder for chaining.
*/
public Builder clearSatisfiesPzs() {
bitField0_ = (bitField0_ & ~0x00080000);
satisfiesPzs_ = false;
onChanged();
return this;
}
private java.lang.Object selfLink_ = "";
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return Whether the selfLink field is set.
*/
public boolean hasSelfLink() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The selfLink.
*/
public java.lang.String getSelfLink() {
java.lang.Object ref = selfLink_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLink_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The bytes for selfLink.
*/
public com.google.protobuf.ByteString getSelfLinkBytes() {
java.lang.Object ref = selfLink_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLink_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @param value The selfLink to set.
* @return This builder for chaining.
*/
public Builder setSelfLink(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
selfLink_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return This builder for chaining.
*/
public Builder clearSelfLink() {
selfLink_ = getDefaultInstance().getSelfLink();
bitField0_ = (bitField0_ & ~0x00100000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @param value The bytes for selfLink to set.
* @return This builder for chaining.
*/
public Builder setSelfLinkBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
selfLink_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
private com.google.cloud.compute.v1.InitialStateConfig shieldedInstanceInitialState_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.InitialStateConfig,
com.google.cloud.compute.v1.InitialStateConfig.Builder,
com.google.cloud.compute.v1.InitialStateConfigOrBuilder>
shieldedInstanceInitialStateBuilder_;
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*
* @return Whether the shieldedInstanceInitialState field is set.
*/
public boolean hasShieldedInstanceInitialState() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*
* @return The shieldedInstanceInitialState.
*/
public com.google.cloud.compute.v1.InitialStateConfig getShieldedInstanceInitialState() {
if (shieldedInstanceInitialStateBuilder_ == null) {
return shieldedInstanceInitialState_ == null
? com.google.cloud.compute.v1.InitialStateConfig.getDefaultInstance()
: shieldedInstanceInitialState_;
} else {
return shieldedInstanceInitialStateBuilder_.getMessage();
}
}
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*/
public Builder setShieldedInstanceInitialState(
com.google.cloud.compute.v1.InitialStateConfig value) {
if (shieldedInstanceInitialStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
shieldedInstanceInitialState_ = value;
} else {
shieldedInstanceInitialStateBuilder_.setMessage(value);
}
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*/
public Builder setShieldedInstanceInitialState(
com.google.cloud.compute.v1.InitialStateConfig.Builder builderForValue) {
if (shieldedInstanceInitialStateBuilder_ == null) {
shieldedInstanceInitialState_ = builderForValue.build();
} else {
shieldedInstanceInitialStateBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*/
public Builder mergeShieldedInstanceInitialState(
com.google.cloud.compute.v1.InitialStateConfig value) {
if (shieldedInstanceInitialStateBuilder_ == null) {
if (((bitField0_ & 0x00200000) != 0)
&& shieldedInstanceInitialState_ != null
&& shieldedInstanceInitialState_
!= com.google.cloud.compute.v1.InitialStateConfig.getDefaultInstance()) {
getShieldedInstanceInitialStateBuilder().mergeFrom(value);
} else {
shieldedInstanceInitialState_ = value;
}
} else {
shieldedInstanceInitialStateBuilder_.mergeFrom(value);
}
if (shieldedInstanceInitialState_ != null) {
bitField0_ |= 0x00200000;
onChanged();
}
return this;
}
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*/
public Builder clearShieldedInstanceInitialState() {
bitField0_ = (bitField0_ & ~0x00200000);
shieldedInstanceInitialState_ = null;
if (shieldedInstanceInitialStateBuilder_ != null) {
shieldedInstanceInitialStateBuilder_.dispose();
shieldedInstanceInitialStateBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*/
public com.google.cloud.compute.v1.InitialStateConfig.Builder
getShieldedInstanceInitialStateBuilder() {
bitField0_ |= 0x00200000;
onChanged();
return getShieldedInstanceInitialStateFieldBuilder().getBuilder();
}
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*/
public com.google.cloud.compute.v1.InitialStateConfigOrBuilder
getShieldedInstanceInitialStateOrBuilder() {
if (shieldedInstanceInitialStateBuilder_ != null) {
return shieldedInstanceInitialStateBuilder_.getMessageOrBuilder();
} else {
return shieldedInstanceInitialState_ == null
? com.google.cloud.compute.v1.InitialStateConfig.getDefaultInstance()
: shieldedInstanceInitialState_;
}
}
/**
*
*
*
* Set the secure boot keys of shielded instance.
*
*
*
* optional .google.cloud.compute.v1.InitialStateConfig shielded_instance_initial_state = 192356867;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.InitialStateConfig,
com.google.cloud.compute.v1.InitialStateConfig.Builder,
com.google.cloud.compute.v1.InitialStateConfigOrBuilder>
getShieldedInstanceInitialStateFieldBuilder() {
if (shieldedInstanceInitialStateBuilder_ == null) {
shieldedInstanceInitialStateBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.InitialStateConfig,
com.google.cloud.compute.v1.InitialStateConfig.Builder,
com.google.cloud.compute.v1.InitialStateConfigOrBuilder>(
getShieldedInstanceInitialState(), getParentForChildren(), isClean());
shieldedInstanceInitialState_ = null;
}
return shieldedInstanceInitialStateBuilder_;
}
private java.lang.Object sourceDisk_ = "";
/**
*
*
*
* URL of the source disk used to create this image. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - projects/project/zones/zone/disks/disk - zones/zone/disks/disk In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_disk = 451753793;
*
* @return Whether the sourceDisk field is set.
*/
public boolean hasSourceDisk() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
*
*
* URL of the source disk used to create this image. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - projects/project/zones/zone/disks/disk - zones/zone/disks/disk In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_disk = 451753793;
*
* @return The sourceDisk.
*/
public java.lang.String getSourceDisk() {
java.lang.Object ref = sourceDisk_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceDisk_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* URL of the source disk used to create this image. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - projects/project/zones/zone/disks/disk - zones/zone/disks/disk In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_disk = 451753793;
*
* @return The bytes for sourceDisk.
*/
public com.google.protobuf.ByteString getSourceDiskBytes() {
java.lang.Object ref = sourceDisk_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceDisk_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* URL of the source disk used to create this image. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - projects/project/zones/zone/disks/disk - zones/zone/disks/disk In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_disk = 451753793;
*
* @param value The sourceDisk to set.
* @return This builder for chaining.
*/
public Builder setSourceDisk(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceDisk_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
*
*
* URL of the source disk used to create this image. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - projects/project/zones/zone/disks/disk - zones/zone/disks/disk In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_disk = 451753793;
*
* @return This builder for chaining.
*/
public Builder clearSourceDisk() {
sourceDisk_ = getDefaultInstance().getSourceDisk();
bitField0_ = (bitField0_ & ~0x00400000);
onChanged();
return this;
}
/**
*
*
*
* URL of the source disk used to create this image. For example, the following are valid values: - https://www.googleapis.com/compute/v1/projects/project/zones/zone /disks/disk - projects/project/zones/zone/disks/disk - zones/zone/disks/disk In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_disk = 451753793;
*
* @param value The bytes for sourceDisk to set.
* @return This builder for chaining.
*/
public Builder setSourceDiskBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceDisk_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
private com.google.cloud.compute.v1.CustomerEncryptionKey sourceDiskEncryptionKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
sourceDiskEncryptionKeyBuilder_;
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*
* @return Whether the sourceDiskEncryptionKey field is set.
*/
public boolean hasSourceDiskEncryptionKey() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*
* @return The sourceDiskEncryptionKey.
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey getSourceDiskEncryptionKey() {
if (sourceDiskEncryptionKeyBuilder_ == null) {
return sourceDiskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceDiskEncryptionKey_;
} else {
return sourceDiskEncryptionKeyBuilder_.getMessage();
}
}
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*/
public Builder setSourceDiskEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (sourceDiskEncryptionKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sourceDiskEncryptionKey_ = value;
} else {
sourceDiskEncryptionKeyBuilder_.setMessage(value);
}
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*/
public Builder setSourceDiskEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder builderForValue) {
if (sourceDiskEncryptionKeyBuilder_ == null) {
sourceDiskEncryptionKey_ = builderForValue.build();
} else {
sourceDiskEncryptionKeyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*/
public Builder mergeSourceDiskEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (sourceDiskEncryptionKeyBuilder_ == null) {
if (((bitField0_ & 0x00800000) != 0)
&& sourceDiskEncryptionKey_ != null
&& sourceDiskEncryptionKey_
!= com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()) {
getSourceDiskEncryptionKeyBuilder().mergeFrom(value);
} else {
sourceDiskEncryptionKey_ = value;
}
} else {
sourceDiskEncryptionKeyBuilder_.mergeFrom(value);
}
if (sourceDiskEncryptionKey_ != null) {
bitField0_ |= 0x00800000;
onChanged();
}
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*/
public Builder clearSourceDiskEncryptionKey() {
bitField0_ = (bitField0_ & ~0x00800000);
sourceDiskEncryptionKey_ = null;
if (sourceDiskEncryptionKeyBuilder_ != null) {
sourceDiskEncryptionKeyBuilder_.dispose();
sourceDiskEncryptionKeyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey.Builder
getSourceDiskEncryptionKeyBuilder() {
bitField0_ |= 0x00800000;
onChanged();
return getSourceDiskEncryptionKeyFieldBuilder().getBuilder();
}
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getSourceDiskEncryptionKeyOrBuilder() {
if (sourceDiskEncryptionKeyBuilder_ != null) {
return sourceDiskEncryptionKeyBuilder_.getMessageOrBuilder();
} else {
return sourceDiskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceDiskEncryptionKey_;
}
}
/**
*
*
*
* The customer-supplied encryption key of the source disk. Required if the source disk is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_disk_encryption_key = 531501153;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
getSourceDiskEncryptionKeyFieldBuilder() {
if (sourceDiskEncryptionKeyBuilder_ == null) {
sourceDiskEncryptionKeyBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>(
getSourceDiskEncryptionKey(), getParentForChildren(), isClean());
sourceDiskEncryptionKey_ = null;
}
return sourceDiskEncryptionKeyBuilder_;
}
private java.lang.Object sourceDiskId_ = "";
/**
*
*
*
* [Output Only] The ID value of the disk used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given disk name.
*
*
* optional string source_disk_id = 454190809;
*
* @return Whether the sourceDiskId field is set.
*/
public boolean hasSourceDiskId() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
*
*
*
* [Output Only] The ID value of the disk used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given disk name.
*
*
* optional string source_disk_id = 454190809;
*
* @return The sourceDiskId.
*/
public java.lang.String getSourceDiskId() {
java.lang.Object ref = sourceDiskId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceDiskId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The ID value of the disk used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given disk name.
*
*
* optional string source_disk_id = 454190809;
*
* @return The bytes for sourceDiskId.
*/
public com.google.protobuf.ByteString getSourceDiskIdBytes() {
java.lang.Object ref = sourceDiskId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceDiskId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The ID value of the disk used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given disk name.
*
*
* optional string source_disk_id = 454190809;
*
* @param value The sourceDiskId to set.
* @return This builder for chaining.
*/
public Builder setSourceDiskId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceDiskId_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The ID value of the disk used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given disk name.
*
*
* optional string source_disk_id = 454190809;
*
* @return This builder for chaining.
*/
public Builder clearSourceDiskId() {
sourceDiskId_ = getDefaultInstance().getSourceDiskId();
bitField0_ = (bitField0_ & ~0x01000000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The ID value of the disk used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given disk name.
*
*
* optional string source_disk_id = 454190809;
*
* @param value The bytes for sourceDiskId to set.
* @return This builder for chaining.
*/
public Builder setSourceDiskIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceDiskId_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
private java.lang.Object sourceImage_ = "";
/**
*
*
*
* URL of the source image used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ images/image_name - projects/project_id/global/images/image_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_image = 50443319;
*
* @return Whether the sourceImage field is set.
*/
public boolean hasSourceImage() {
return ((bitField0_ & 0x02000000) != 0);
}
/**
*
*
*
* URL of the source image used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ images/image_name - projects/project_id/global/images/image_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_image = 50443319;
*
* @return The sourceImage.
*/
public java.lang.String getSourceImage() {
java.lang.Object ref = sourceImage_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceImage_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* URL of the source image used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ images/image_name - projects/project_id/global/images/image_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_image = 50443319;
*
* @return The bytes for sourceImage.
*/
public com.google.protobuf.ByteString getSourceImageBytes() {
java.lang.Object ref = sourceImage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceImage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* URL of the source image used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ images/image_name - projects/project_id/global/images/image_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_image = 50443319;
*
* @param value The sourceImage to set.
* @return This builder for chaining.
*/
public Builder setSourceImage(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceImage_ = value;
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
*
*
* URL of the source image used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ images/image_name - projects/project_id/global/images/image_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_image = 50443319;
*
* @return This builder for chaining.
*/
public Builder clearSourceImage() {
sourceImage_ = getDefaultInstance().getSourceImage();
bitField0_ = (bitField0_ & ~0x02000000);
onChanged();
return this;
}
/**
*
*
*
* URL of the source image used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ images/image_name - projects/project_id/global/images/image_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_image = 50443319;
*
* @param value The bytes for sourceImage to set.
* @return This builder for chaining.
*/
public Builder setSourceImageBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceImage_ = value;
bitField0_ |= 0x02000000;
onChanged();
return this;
}
private com.google.cloud.compute.v1.CustomerEncryptionKey sourceImageEncryptionKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
sourceImageEncryptionKeyBuilder_;
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*
* @return Whether the sourceImageEncryptionKey field is set.
*/
public boolean hasSourceImageEncryptionKey() {
return ((bitField0_ & 0x04000000) != 0);
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*
* @return The sourceImageEncryptionKey.
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey getSourceImageEncryptionKey() {
if (sourceImageEncryptionKeyBuilder_ == null) {
return sourceImageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceImageEncryptionKey_;
} else {
return sourceImageEncryptionKeyBuilder_.getMessage();
}
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public Builder setSourceImageEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (sourceImageEncryptionKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sourceImageEncryptionKey_ = value;
} else {
sourceImageEncryptionKeyBuilder_.setMessage(value);
}
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public Builder setSourceImageEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder builderForValue) {
if (sourceImageEncryptionKeyBuilder_ == null) {
sourceImageEncryptionKey_ = builderForValue.build();
} else {
sourceImageEncryptionKeyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public Builder mergeSourceImageEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (sourceImageEncryptionKeyBuilder_ == null) {
if (((bitField0_ & 0x04000000) != 0)
&& sourceImageEncryptionKey_ != null
&& sourceImageEncryptionKey_
!= com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()) {
getSourceImageEncryptionKeyBuilder().mergeFrom(value);
} else {
sourceImageEncryptionKey_ = value;
}
} else {
sourceImageEncryptionKeyBuilder_.mergeFrom(value);
}
if (sourceImageEncryptionKey_ != null) {
bitField0_ |= 0x04000000;
onChanged();
}
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public Builder clearSourceImageEncryptionKey() {
bitField0_ = (bitField0_ & ~0x04000000);
sourceImageEncryptionKey_ = null;
if (sourceImageEncryptionKeyBuilder_ != null) {
sourceImageEncryptionKeyBuilder_.dispose();
sourceImageEncryptionKeyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey.Builder
getSourceImageEncryptionKeyBuilder() {
bitField0_ |= 0x04000000;
onChanged();
return getSourceImageEncryptionKeyFieldBuilder().getBuilder();
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getSourceImageEncryptionKeyOrBuilder() {
if (sourceImageEncryptionKeyBuilder_ != null) {
return sourceImageEncryptionKeyBuilder_.getMessageOrBuilder();
} else {
return sourceImageEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceImageEncryptionKey_;
}
}
/**
*
*
*
* The customer-supplied encryption key of the source image. Required if the source image is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_image_encryption_key = 381503659;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
getSourceImageEncryptionKeyFieldBuilder() {
if (sourceImageEncryptionKeyBuilder_ == null) {
sourceImageEncryptionKeyBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>(
getSourceImageEncryptionKey(), getParentForChildren(), isClean());
sourceImageEncryptionKey_ = null;
}
return sourceImageEncryptionKeyBuilder_;
}
private java.lang.Object sourceImageId_ = "";
/**
*
*
*
* [Output Only] The ID value of the image used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given image name.
*
*
* optional string source_image_id = 55328291;
*
* @return Whether the sourceImageId field is set.
*/
public boolean hasSourceImageId() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given image name.
*
*
* optional string source_image_id = 55328291;
*
* @return The sourceImageId.
*/
public java.lang.String getSourceImageId() {
java.lang.Object ref = sourceImageId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceImageId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given image name.
*
*
* optional string source_image_id = 55328291;
*
* @return The bytes for sourceImageId.
*/
public com.google.protobuf.ByteString getSourceImageIdBytes() {
java.lang.Object ref = sourceImageId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceImageId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given image name.
*
*
* optional string source_image_id = 55328291;
*
* @param value The sourceImageId to set.
* @return This builder for chaining.
*/
public Builder setSourceImageId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceImageId_ = value;
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given image name.
*
*
* optional string source_image_id = 55328291;
*
* @return This builder for chaining.
*/
public Builder clearSourceImageId() {
sourceImageId_ = getDefaultInstance().getSourceImageId();
bitField0_ = (bitField0_ & ~0x08000000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The ID value of the image used to create this image. This value may be used to determine whether the image was taken from the current or a previous instance of a given image name.
*
*
* optional string source_image_id = 55328291;
*
* @param value The bytes for sourceImageId to set.
* @return This builder for chaining.
*/
public Builder setSourceImageIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceImageId_ = value;
bitField0_ |= 0x08000000;
onChanged();
return this;
}
private java.lang.Object sourceSnapshot_ = "";
/**
*
*
*
* URL of the source snapshot used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ snapshots/snapshot_name - projects/project_id/global/snapshots/snapshot_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_snapshot = 126061928;
*
* @return Whether the sourceSnapshot field is set.
*/
public boolean hasSourceSnapshot() {
return ((bitField0_ & 0x10000000) != 0);
}
/**
*
*
*
* URL of the source snapshot used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ snapshots/snapshot_name - projects/project_id/global/snapshots/snapshot_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_snapshot = 126061928;
*
* @return The sourceSnapshot.
*/
public java.lang.String getSourceSnapshot() {
java.lang.Object ref = sourceSnapshot_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceSnapshot_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* URL of the source snapshot used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ snapshots/snapshot_name - projects/project_id/global/snapshots/snapshot_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_snapshot = 126061928;
*
* @return The bytes for sourceSnapshot.
*/
public com.google.protobuf.ByteString getSourceSnapshotBytes() {
java.lang.Object ref = sourceSnapshot_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceSnapshot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* URL of the source snapshot used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ snapshots/snapshot_name - projects/project_id/global/snapshots/snapshot_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_snapshot = 126061928;
*
* @param value The sourceSnapshot to set.
* @return This builder for chaining.
*/
public Builder setSourceSnapshot(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceSnapshot_ = value;
bitField0_ |= 0x10000000;
onChanged();
return this;
}
/**
*
*
*
* URL of the source snapshot used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ snapshots/snapshot_name - projects/project_id/global/snapshots/snapshot_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_snapshot = 126061928;
*
* @return This builder for chaining.
*/
public Builder clearSourceSnapshot() {
sourceSnapshot_ = getDefaultInstance().getSourceSnapshot();
bitField0_ = (bitField0_ & ~0x10000000);
onChanged();
return this;
}
/**
*
*
*
* URL of the source snapshot used to create this image. The following are valid formats for the URL: - https://www.googleapis.com/compute/v1/projects/project_id/global/ snapshots/snapshot_name - projects/project_id/global/snapshots/snapshot_name In order to create an image, you must provide the full or partial URL of one of the following: - The rawDisk.source URL - The sourceDisk URL - The sourceImage URL - The sourceSnapshot URL
*
*
* optional string source_snapshot = 126061928;
*
* @param value The bytes for sourceSnapshot to set.
* @return This builder for chaining.
*/
public Builder setSourceSnapshotBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceSnapshot_ = value;
bitField0_ |= 0x10000000;
onChanged();
return this;
}
private com.google.cloud.compute.v1.CustomerEncryptionKey sourceSnapshotEncryptionKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
sourceSnapshotEncryptionKeyBuilder_;
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*
* @return Whether the sourceSnapshotEncryptionKey field is set.
*/
public boolean hasSourceSnapshotEncryptionKey() {
return ((bitField0_ & 0x20000000) != 0);
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*
* @return The sourceSnapshotEncryptionKey.
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey getSourceSnapshotEncryptionKey() {
if (sourceSnapshotEncryptionKeyBuilder_ == null) {
return sourceSnapshotEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceSnapshotEncryptionKey_;
} else {
return sourceSnapshotEncryptionKeyBuilder_.getMessage();
}
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public Builder setSourceSnapshotEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (sourceSnapshotEncryptionKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sourceSnapshotEncryptionKey_ = value;
} else {
sourceSnapshotEncryptionKeyBuilder_.setMessage(value);
}
bitField0_ |= 0x20000000;
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public Builder setSourceSnapshotEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder builderForValue) {
if (sourceSnapshotEncryptionKeyBuilder_ == null) {
sourceSnapshotEncryptionKey_ = builderForValue.build();
} else {
sourceSnapshotEncryptionKeyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x20000000;
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public Builder mergeSourceSnapshotEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (sourceSnapshotEncryptionKeyBuilder_ == null) {
if (((bitField0_ & 0x20000000) != 0)
&& sourceSnapshotEncryptionKey_ != null
&& sourceSnapshotEncryptionKey_
!= com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()) {
getSourceSnapshotEncryptionKeyBuilder().mergeFrom(value);
} else {
sourceSnapshotEncryptionKey_ = value;
}
} else {
sourceSnapshotEncryptionKeyBuilder_.mergeFrom(value);
}
if (sourceSnapshotEncryptionKey_ != null) {
bitField0_ |= 0x20000000;
onChanged();
}
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public Builder clearSourceSnapshotEncryptionKey() {
bitField0_ = (bitField0_ & ~0x20000000);
sourceSnapshotEncryptionKey_ = null;
if (sourceSnapshotEncryptionKeyBuilder_ != null) {
sourceSnapshotEncryptionKeyBuilder_.dispose();
sourceSnapshotEncryptionKeyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey.Builder
getSourceSnapshotEncryptionKeyBuilder() {
bitField0_ |= 0x20000000;
onChanged();
return getSourceSnapshotEncryptionKeyFieldBuilder().getBuilder();
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getSourceSnapshotEncryptionKeyOrBuilder() {
if (sourceSnapshotEncryptionKeyBuilder_ != null) {
return sourceSnapshotEncryptionKeyBuilder_.getMessageOrBuilder();
} else {
return sourceSnapshotEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: sourceSnapshotEncryptionKey_;
}
}
/**
*
*
*
* The customer-supplied encryption key of the source snapshot. Required if the source snapshot is protected by a customer-supplied encryption key.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey source_snapshot_encryption_key = 303679322;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
getSourceSnapshotEncryptionKeyFieldBuilder() {
if (sourceSnapshotEncryptionKeyBuilder_ == null) {
sourceSnapshotEncryptionKeyBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>(
getSourceSnapshotEncryptionKey(), getParentForChildren(), isClean());
sourceSnapshotEncryptionKey_ = null;
}
return sourceSnapshotEncryptionKeyBuilder_;
}
private java.lang.Object sourceSnapshotId_ = "";
/**
*
*
*
* [Output Only] The ID value of the snapshot used to create this image. This value may be used to determine whether the snapshot was taken from the current or a previous instance of a given snapshot name.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return Whether the sourceSnapshotId field is set.
*/
public boolean hasSourceSnapshotId() {
return ((bitField0_ & 0x40000000) != 0);
}
/**
*
*
*
* [Output Only] The ID value of the snapshot used to create this image. This value may be used to determine whether the snapshot was taken from the current or a previous instance of a given snapshot name.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return The sourceSnapshotId.
*/
public java.lang.String getSourceSnapshotId() {
java.lang.Object ref = sourceSnapshotId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceSnapshotId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The ID value of the snapshot used to create this image. This value may be used to determine whether the snapshot was taken from the current or a previous instance of a given snapshot name.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return The bytes for sourceSnapshotId.
*/
public com.google.protobuf.ByteString getSourceSnapshotIdBytes() {
java.lang.Object ref = sourceSnapshotId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceSnapshotId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The ID value of the snapshot used to create this image. This value may be used to determine whether the snapshot was taken from the current or a previous instance of a given snapshot name.
*
*
* optional string source_snapshot_id = 98962258;
*
* @param value The sourceSnapshotId to set.
* @return This builder for chaining.
*/
public Builder setSourceSnapshotId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceSnapshotId_ = value;
bitField0_ |= 0x40000000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The ID value of the snapshot used to create this image. This value may be used to determine whether the snapshot was taken from the current or a previous instance of a given snapshot name.
*
*
* optional string source_snapshot_id = 98962258;
*
* @return This builder for chaining.
*/
public Builder clearSourceSnapshotId() {
sourceSnapshotId_ = getDefaultInstance().getSourceSnapshotId();
bitField0_ = (bitField0_ & ~0x40000000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The ID value of the snapshot used to create this image. This value may be used to determine whether the snapshot was taken from the current or a previous instance of a given snapshot name.
*
*
* optional string source_snapshot_id = 98962258;
*
* @param value The bytes for sourceSnapshotId to set.
* @return This builder for chaining.
*/
public Builder setSourceSnapshotIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceSnapshotId_ = value;
bitField0_ |= 0x40000000;
onChanged();
return this;
}
private java.lang.Object sourceType_ = "";
/**
*
*
*
* The type of the image used to create this disk. The default and only valid value is RAW.
* Check the SourceType enum for the list of possible values.
*
*
* optional string source_type = 452245726;
*
* @return Whether the sourceType field is set.
*/
public boolean hasSourceType() {
return ((bitField0_ & 0x80000000) != 0);
}
/**
*
*
*
* The type of the image used to create this disk. The default and only valid value is RAW.
* Check the SourceType enum for the list of possible values.
*
*
* optional string source_type = 452245726;
*
* @return The sourceType.
*/
public java.lang.String getSourceType() {
java.lang.Object ref = sourceType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The type of the image used to create this disk. The default and only valid value is RAW.
* Check the SourceType enum for the list of possible values.
*
*
* optional string source_type = 452245726;
*
* @return The bytes for sourceType.
*/
public com.google.protobuf.ByteString getSourceTypeBytes() {
java.lang.Object ref = sourceType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sourceType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The type of the image used to create this disk. The default and only valid value is RAW.
* Check the SourceType enum for the list of possible values.
*
*
* optional string source_type = 452245726;
*
* @param value The sourceType to set.
* @return This builder for chaining.
*/
public Builder setSourceType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceType_ = value;
bitField0_ |= 0x80000000;
onChanged();
return this;
}
/**
*
*
*
* The type of the image used to create this disk. The default and only valid value is RAW.
* Check the SourceType enum for the list of possible values.
*
*
* optional string source_type = 452245726;
*
* @return This builder for chaining.
*/
public Builder clearSourceType() {
sourceType_ = getDefaultInstance().getSourceType();
bitField0_ = (bitField0_ & ~0x80000000);
onChanged();
return this;
}
/**
*
*
*
* The type of the image used to create this disk. The default and only valid value is RAW.
* Check the SourceType enum for the list of possible values.
*
*
* optional string source_type = 452245726;
*
* @param value The bytes for sourceType to set.
* @return This builder for chaining.
*/
public Builder setSourceTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceType_ = value;
bitField0_ |= 0x80000000;
onChanged();
return this;
}
private java.lang.Object status_ = "";
/**
*
*
*
* [Output Only] The status of the image. An image can be used to create other resources, such as instances, only after the image has been successfully created and the status is set to READY. Possible values are FAILED, PENDING, or READY.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return ((bitField1_ & 0x00000001) != 0);
}
/**
*
*
*
* [Output Only] The status of the image. An image can be used to create other resources, such as instances, only after the image has been successfully created and the status is set to READY. Possible values are FAILED, PENDING, or READY.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The status.
*/
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
status_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The status of the image. An image can be used to create other resources, such as instances, only after the image has been successfully created and the status is set to READY. Possible values are FAILED, PENDING, or READY.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The bytes for status.
*/
public com.google.protobuf.ByteString getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The status of the image. An image can be used to create other resources, such as instances, only after the image has been successfully created and the status is set to READY. Possible values are FAILED, PENDING, or READY.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
bitField1_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The status of the image. An image can be used to create other resources, such as instances, only after the image has been successfully created and the status is set to READY. Possible values are FAILED, PENDING, or READY.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = getDefaultInstance().getStatus();
bitField1_ = (bitField1_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The status of the image. An image can be used to create other resources, such as instances, only after the image has been successfully created and the status is set to READY. Possible values are FAILED, PENDING, or READY.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @param value The bytes for status to set.
* @return This builder for chaining.
*/
public Builder setStatusBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
status_ = value;
bitField1_ |= 0x00000001;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList storageLocations_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureStorageLocationsIsMutable() {
if (!storageLocations_.isModifiable()) {
storageLocations_ = new com.google.protobuf.LazyStringArrayList(storageLocations_);
}
bitField1_ |= 0x00000002;
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @return A list containing the storageLocations.
*/
public com.google.protobuf.ProtocolStringList getStorageLocationsList() {
storageLocations_.makeImmutable();
return storageLocations_;
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @return The count of storageLocations.
*/
public int getStorageLocationsCount() {
return storageLocations_.size();
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @param index The index of the element to return.
* @return The storageLocations at the given index.
*/
public java.lang.String getStorageLocations(int index) {
return storageLocations_.get(index);
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @param index The index of the value to return.
* @return The bytes of the storageLocations at the given index.
*/
public com.google.protobuf.ByteString getStorageLocationsBytes(int index) {
return storageLocations_.getByteString(index);
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @param index The index to set the value at.
* @param value The storageLocations to set.
* @return This builder for chaining.
*/
public Builder setStorageLocations(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureStorageLocationsIsMutable();
storageLocations_.set(index, value);
bitField1_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @param value The storageLocations to add.
* @return This builder for chaining.
*/
public Builder addStorageLocations(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureStorageLocationsIsMutable();
storageLocations_.add(value);
bitField1_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @param values The storageLocations to add.
* @return This builder for chaining.
*/
public Builder addAllStorageLocations(java.lang.Iterable values) {
ensureStorageLocationsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, storageLocations_);
bitField1_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @return This builder for chaining.
*/
public Builder clearStorageLocations() {
storageLocations_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField1_ = (bitField1_ & ~0x00000002);
;
onChanged();
return this;
}
/**
*
*
*
* Cloud Storage bucket storage location of the image (regional or multi-regional).
*
*
* repeated string storage_locations = 328005274;
*
* @param value The bytes of the storageLocations to add.
* @return This builder for chaining.
*/
public Builder addStorageLocationsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureStorageLocationsIsMutable();
storageLocations_.add(value);
bitField1_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.Image)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.Image)
private static final com.google.cloud.compute.v1.Image DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.Image();
}
public static com.google.cloud.compute.v1.Image getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Image parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.Image getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy