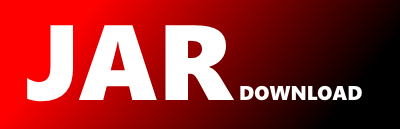
com.google.cloud.compute.v1.InsertSecurityPolicyRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* A request message for SecurityPolicies.Insert. See the method description for details.
*
*
* Protobuf type {@code google.cloud.compute.v1.InsertSecurityPolicyRequest}
*/
public final class InsertSecurityPolicyRequest extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.InsertSecurityPolicyRequest)
InsertSecurityPolicyRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use InsertSecurityPolicyRequest.newBuilder() to construct.
private InsertSecurityPolicyRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InsertSecurityPolicyRequest() {
project_ = "";
requestId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new InsertSecurityPolicyRequest();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_InsertSecurityPolicyRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_InsertSecurityPolicyRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.InsertSecurityPolicyRequest.class,
com.google.cloud.compute.v1.InsertSecurityPolicyRequest.Builder.class);
}
private int bitField0_;
public static final int PROJECT_FIELD_NUMBER = 227560217;
@SuppressWarnings("serial")
private volatile java.lang.Object project_ = "";
/**
*
*
*
* Project ID for this request.
*
*
*
* string project = 227560217 [(.google.api.field_behavior) = REQUIRED, (.google.cloud.operation_request_field) = "project"];
*
*
* @return The project.
*/
@java.lang.Override
public java.lang.String getProject() {
java.lang.Object ref = project_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
project_ = s;
return s;
}
}
/**
*
*
*
* Project ID for this request.
*
*
*
* string project = 227560217 [(.google.api.field_behavior) = REQUIRED, (.google.cloud.operation_request_field) = "project"];
*
*
* @return The bytes for project.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProjectBytes() {
java.lang.Object ref = project_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
project_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQUEST_ID_FIELD_NUMBER = 37109963;
@SuppressWarnings("serial")
private volatile java.lang.Object requestId_ = "";
/**
*
*
*
* An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*
* optional string request_id = 37109963;
*
* @return Whether the requestId field is set.
*/
@java.lang.Override
public boolean hasRequestId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*
* optional string request_id = 37109963;
*
* @return The requestId.
*/
@java.lang.Override
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
}
}
/**
*
*
*
* An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*
* optional string request_id = 37109963;
*
* @return The bytes for requestId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SECURITY_POLICY_RESOURCE_FIELD_NUMBER = 216159612;
private com.google.cloud.compute.v1.SecurityPolicy securityPolicyResource_;
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the securityPolicyResource field is set.
*/
@java.lang.Override
public boolean hasSecurityPolicyResource() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The securityPolicyResource.
*/
@java.lang.Override
public com.google.cloud.compute.v1.SecurityPolicy getSecurityPolicyResource() {
return securityPolicyResource_ == null
? com.google.cloud.compute.v1.SecurityPolicy.getDefaultInstance()
: securityPolicyResource_;
}
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.SecurityPolicyOrBuilder getSecurityPolicyResourceOrBuilder() {
return securityPolicyResource_ == null
? com.google.cloud.compute.v1.SecurityPolicy.getDefaultInstance()
: securityPolicyResource_;
}
public static final int VALIDATE_ONLY_FIELD_NUMBER = 242744629;
private boolean validateOnly_ = false;
/**
*
*
*
* If true, the request will not be committed.
*
*
* optional bool validate_only = 242744629;
*
* @return Whether the validateOnly field is set.
*/
@java.lang.Override
public boolean hasValidateOnly() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* If true, the request will not be committed.
*
*
* optional bool validate_only = 242744629;
*
* @return The validateOnly.
*/
@java.lang.Override
public boolean getValidateOnly() {
return validateOnly_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 37109963, requestId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(216159612, getSecurityPolicyResource());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(project_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 227560217, project_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(242744629, validateOnly_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(37109963, requestId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
216159612, getSecurityPolicyResource());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(project_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(227560217, project_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(242744629, validateOnly_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.InsertSecurityPolicyRequest)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.InsertSecurityPolicyRequest other =
(com.google.cloud.compute.v1.InsertSecurityPolicyRequest) obj;
if (!getProject().equals(other.getProject())) return false;
if (hasRequestId() != other.hasRequestId()) return false;
if (hasRequestId()) {
if (!getRequestId().equals(other.getRequestId())) return false;
}
if (hasSecurityPolicyResource() != other.hasSecurityPolicyResource()) return false;
if (hasSecurityPolicyResource()) {
if (!getSecurityPolicyResource().equals(other.getSecurityPolicyResource())) return false;
}
if (hasValidateOnly() != other.hasValidateOnly()) return false;
if (hasValidateOnly()) {
if (getValidateOnly() != other.getValidateOnly()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PROJECT_FIELD_NUMBER;
hash = (53 * hash) + getProject().hashCode();
if (hasRequestId()) {
hash = (37 * hash) + REQUEST_ID_FIELD_NUMBER;
hash = (53 * hash) + getRequestId().hashCode();
}
if (hasSecurityPolicyResource()) {
hash = (37 * hash) + SECURITY_POLICY_RESOURCE_FIELD_NUMBER;
hash = (53 * hash) + getSecurityPolicyResource().hashCode();
}
if (hasValidateOnly()) {
hash = (37 * hash) + VALIDATE_ONLY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getValidateOnly());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.compute.v1.InsertSecurityPolicyRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* A request message for SecurityPolicies.Insert. See the method description for details.
*
*
* Protobuf type {@code google.cloud.compute.v1.InsertSecurityPolicyRequest}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.InsertSecurityPolicyRequest)
com.google.cloud.compute.v1.InsertSecurityPolicyRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_InsertSecurityPolicyRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_InsertSecurityPolicyRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.InsertSecurityPolicyRequest.class,
com.google.cloud.compute.v1.InsertSecurityPolicyRequest.Builder.class);
}
// Construct using com.google.cloud.compute.v1.InsertSecurityPolicyRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getSecurityPolicyResourceFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
project_ = "";
requestId_ = "";
securityPolicyResource_ = null;
if (securityPolicyResourceBuilder_ != null) {
securityPolicyResourceBuilder_.dispose();
securityPolicyResourceBuilder_ = null;
}
validateOnly_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_InsertSecurityPolicyRequest_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.InsertSecurityPolicyRequest getDefaultInstanceForType() {
return com.google.cloud.compute.v1.InsertSecurityPolicyRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.InsertSecurityPolicyRequest build() {
com.google.cloud.compute.v1.InsertSecurityPolicyRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.InsertSecurityPolicyRequest buildPartial() {
com.google.cloud.compute.v1.InsertSecurityPolicyRequest result =
new com.google.cloud.compute.v1.InsertSecurityPolicyRequest(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.compute.v1.InsertSecurityPolicyRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.project_ = project_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.requestId_ = requestId_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.securityPolicyResource_ =
securityPolicyResourceBuilder_ == null
? securityPolicyResource_
: securityPolicyResourceBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.validateOnly_ = validateOnly_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.InsertSecurityPolicyRequest) {
return mergeFrom((com.google.cloud.compute.v1.InsertSecurityPolicyRequest) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.InsertSecurityPolicyRequest other) {
if (other == com.google.cloud.compute.v1.InsertSecurityPolicyRequest.getDefaultInstance())
return this;
if (!other.getProject().isEmpty()) {
project_ = other.project_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasRequestId()) {
requestId_ = other.requestId_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasSecurityPolicyResource()) {
mergeSecurityPolicyResource(other.getSecurityPolicyResource());
}
if (other.hasValidateOnly()) {
setValidateOnly(other.getValidateOnly());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 296879706:
{
requestId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 296879706
case 1729276898:
{
input.readMessage(
getSecurityPolicyResourceFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 1729276898
case 1820481738:
{
project_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 1820481738
case 1941957032:
{
validateOnly_ = input.readBool();
bitField0_ |= 0x00000008;
break;
} // case 1941957032
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object project_ = "";
/**
*
*
*
* Project ID for this request.
*
*
*
* string project = 227560217 [(.google.api.field_behavior) = REQUIRED, (.google.cloud.operation_request_field) = "project"];
*
*
* @return The project.
*/
public java.lang.String getProject() {
java.lang.Object ref = project_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
project_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Project ID for this request.
*
*
*
* string project = 227560217 [(.google.api.field_behavior) = REQUIRED, (.google.cloud.operation_request_field) = "project"];
*
*
* @return The bytes for project.
*/
public com.google.protobuf.ByteString getProjectBytes() {
java.lang.Object ref = project_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
project_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Project ID for this request.
*
*
*
* string project = 227560217 [(.google.api.field_behavior) = REQUIRED, (.google.cloud.operation_request_field) = "project"];
*
*
* @param value The project to set.
* @return This builder for chaining.
*/
public Builder setProject(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
project_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Project ID for this request.
*
*
*
* string project = 227560217 [(.google.api.field_behavior) = REQUIRED, (.google.cloud.operation_request_field) = "project"];
*
*
* @return This builder for chaining.
*/
public Builder clearProject() {
project_ = getDefaultInstance().getProject();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* Project ID for this request.
*
*
*
* string project = 227560217 [(.google.api.field_behavior) = REQUIRED, (.google.cloud.operation_request_field) = "project"];
*
*
* @param value The bytes for project to set.
* @return This builder for chaining.
*/
public Builder setProjectBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
project_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object requestId_ = "";
/**
*
*
*
* An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*
* optional string request_id = 37109963;
*
* @return Whether the requestId field is set.
*/
public boolean hasRequestId() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*
* optional string request_id = 37109963;
*
* @return The requestId.
*/
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*
* optional string request_id = 37109963;
*
* @return The bytes for requestId.
*/
public com.google.protobuf.ByteString getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*
* optional string request_id = 37109963;
*
* @param value The requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
requestId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*
* optional string request_id = 37109963;
*
* @return This builder for chaining.
*/
public Builder clearRequestId() {
requestId_ = getDefaultInstance().getRequestId();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*
* optional string request_id = 37109963;
*
* @param value The bytes for requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
requestId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.cloud.compute.v1.SecurityPolicy securityPolicyResource_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.SecurityPolicy,
com.google.cloud.compute.v1.SecurityPolicy.Builder,
com.google.cloud.compute.v1.SecurityPolicyOrBuilder>
securityPolicyResourceBuilder_;
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the securityPolicyResource field is set.
*/
public boolean hasSecurityPolicyResource() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The securityPolicyResource.
*/
public com.google.cloud.compute.v1.SecurityPolicy getSecurityPolicyResource() {
if (securityPolicyResourceBuilder_ == null) {
return securityPolicyResource_ == null
? com.google.cloud.compute.v1.SecurityPolicy.getDefaultInstance()
: securityPolicyResource_;
} else {
return securityPolicyResourceBuilder_.getMessage();
}
}
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setSecurityPolicyResource(com.google.cloud.compute.v1.SecurityPolicy value) {
if (securityPolicyResourceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
securityPolicyResource_ = value;
} else {
securityPolicyResourceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setSecurityPolicyResource(
com.google.cloud.compute.v1.SecurityPolicy.Builder builderForValue) {
if (securityPolicyResourceBuilder_ == null) {
securityPolicyResource_ = builderForValue.build();
} else {
securityPolicyResourceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder mergeSecurityPolicyResource(com.google.cloud.compute.v1.SecurityPolicy value) {
if (securityPolicyResourceBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)
&& securityPolicyResource_ != null
&& securityPolicyResource_
!= com.google.cloud.compute.v1.SecurityPolicy.getDefaultInstance()) {
getSecurityPolicyResourceBuilder().mergeFrom(value);
} else {
securityPolicyResource_ = value;
}
} else {
securityPolicyResourceBuilder_.mergeFrom(value);
}
if (securityPolicyResource_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder clearSecurityPolicyResource() {
bitField0_ = (bitField0_ & ~0x00000004);
securityPolicyResource_ = null;
if (securityPolicyResourceBuilder_ != null) {
securityPolicyResourceBuilder_.dispose();
securityPolicyResourceBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.cloud.compute.v1.SecurityPolicy.Builder getSecurityPolicyResourceBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getSecurityPolicyResourceFieldBuilder().getBuilder();
}
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.cloud.compute.v1.SecurityPolicyOrBuilder
getSecurityPolicyResourceOrBuilder() {
if (securityPolicyResourceBuilder_ != null) {
return securityPolicyResourceBuilder_.getMessageOrBuilder();
} else {
return securityPolicyResource_ == null
? com.google.cloud.compute.v1.SecurityPolicy.getDefaultInstance()
: securityPolicyResource_;
}
}
/**
*
*
*
* The body resource for this request
*
*
*
* .google.cloud.compute.v1.SecurityPolicy security_policy_resource = 216159612 [(.google.api.field_behavior) = REQUIRED];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.SecurityPolicy,
com.google.cloud.compute.v1.SecurityPolicy.Builder,
com.google.cloud.compute.v1.SecurityPolicyOrBuilder>
getSecurityPolicyResourceFieldBuilder() {
if (securityPolicyResourceBuilder_ == null) {
securityPolicyResourceBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.SecurityPolicy,
com.google.cloud.compute.v1.SecurityPolicy.Builder,
com.google.cloud.compute.v1.SecurityPolicyOrBuilder>(
getSecurityPolicyResource(), getParentForChildren(), isClean());
securityPolicyResource_ = null;
}
return securityPolicyResourceBuilder_;
}
private boolean validateOnly_;
/**
*
*
*
* If true, the request will not be committed.
*
*
* optional bool validate_only = 242744629;
*
* @return Whether the validateOnly field is set.
*/
@java.lang.Override
public boolean hasValidateOnly() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* If true, the request will not be committed.
*
*
* optional bool validate_only = 242744629;
*
* @return The validateOnly.
*/
@java.lang.Override
public boolean getValidateOnly() {
return validateOnly_;
}
/**
*
*
*
* If true, the request will not be committed.
*
*
* optional bool validate_only = 242744629;
*
* @param value The validateOnly to set.
* @return This builder for chaining.
*/
public Builder setValidateOnly(boolean value) {
validateOnly_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* If true, the request will not be committed.
*
*
* optional bool validate_only = 242744629;
*
* @return This builder for chaining.
*/
public Builder clearValidateOnly() {
bitField0_ = (bitField0_ & ~0x00000008);
validateOnly_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.InsertSecurityPolicyRequest)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.InsertSecurityPolicyRequest)
private static final com.google.cloud.compute.v1.InsertSecurityPolicyRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.InsertSecurityPolicyRequest();
}
public static com.google.cloud.compute.v1.InsertSecurityPolicyRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public InsertSecurityPolicyRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.InsertSecurityPolicyRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy