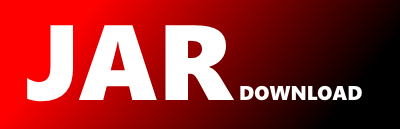
com.google.cloud.compute.v1.NetworkAttachment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* NetworkAttachments A network attachment resource ...
*
*
* Protobuf type {@code google.cloud.compute.v1.NetworkAttachment}
*/
public final class NetworkAttachment extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.NetworkAttachment)
NetworkAttachmentOrBuilder {
private static final long serialVersionUID = 0L;
// Use NetworkAttachment.newBuilder() to construct.
private NetworkAttachment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NetworkAttachment() {
connectionEndpoints_ = java.util.Collections.emptyList();
connectionPreference_ = "";
creationTimestamp_ = "";
description_ = "";
fingerprint_ = "";
kind_ = "";
name_ = "";
network_ = "";
producerAcceptLists_ = com.google.protobuf.LazyStringArrayList.emptyList();
producerRejectLists_ = com.google.protobuf.LazyStringArrayList.emptyList();
region_ = "";
selfLink_ = "";
selfLinkWithId_ = "";
subnetworks_ = com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new NetworkAttachment();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkAttachment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkAttachment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.NetworkAttachment.class,
com.google.cloud.compute.v1.NetworkAttachment.Builder.class);
}
/**
*
*
*
*
*
* Protobuf enum {@code google.cloud.compute.v1.NetworkAttachment.ConnectionPreference}
*/
public enum ConnectionPreference implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_CONNECTION_PREFERENCE = 0;
*/
UNDEFINED_CONNECTION_PREFERENCE(0),
/** ACCEPT_AUTOMATIC = 75250580;
*/
ACCEPT_AUTOMATIC(75250580),
/** ACCEPT_MANUAL = 373061341;
*/
ACCEPT_MANUAL(373061341),
/** INVALID = 530283991;
*/
INVALID(530283991),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_CONNECTION_PREFERENCE = 0;
*/
public static final int UNDEFINED_CONNECTION_PREFERENCE_VALUE = 0;
/** ACCEPT_AUTOMATIC = 75250580;
*/
public static final int ACCEPT_AUTOMATIC_VALUE = 75250580;
/** ACCEPT_MANUAL = 373061341;
*/
public static final int ACCEPT_MANUAL_VALUE = 373061341;
/** INVALID = 530283991;
*/
public static final int INVALID_VALUE = 530283991;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ConnectionPreference valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ConnectionPreference forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_CONNECTION_PREFERENCE;
case 75250580:
return ACCEPT_AUTOMATIC;
case 373061341:
return ACCEPT_MANUAL;
case 530283991:
return INVALID;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ConnectionPreference findValueByNumber(int number) {
return ConnectionPreference.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.NetworkAttachment.getDescriptor().getEnumTypes().get(0);
}
private static final ConnectionPreference[] VALUES = values();
public static ConnectionPreference valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ConnectionPreference(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.NetworkAttachment.ConnectionPreference)
}
private int bitField0_;
public static final int CONNECTION_ENDPOINTS_FIELD_NUMBER = 326078813;
@SuppressWarnings("serial")
private java.util.List
connectionEndpoints_;
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
@java.lang.Override
public java.util.List
getConnectionEndpointsList() {
return connectionEndpoints_;
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
@java.lang.Override
public java.util.List<
? extends com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpointOrBuilder>
getConnectionEndpointsOrBuilderList() {
return connectionEndpoints_;
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
@java.lang.Override
public int getConnectionEndpointsCount() {
return connectionEndpoints_.size();
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint getConnectionEndpoints(
int index) {
return connectionEndpoints_.get(index);
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpointOrBuilder
getConnectionEndpointsOrBuilder(int index) {
return connectionEndpoints_.get(index);
}
public static final int CONNECTION_PREFERENCE_FIELD_NUMBER = 285818076;
@SuppressWarnings("serial")
private volatile java.lang.Object connectionPreference_ = "";
/**
*
*
*
*
* Check the ConnectionPreference enum for the list of possible values.
*
*
* optional string connection_preference = 285818076;
*
* @return Whether the connectionPreference field is set.
*/
@java.lang.Override
public boolean hasConnectionPreference() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
*
* Check the ConnectionPreference enum for the list of possible values.
*
*
* optional string connection_preference = 285818076;
*
* @return The connectionPreference.
*/
@java.lang.Override
public java.lang.String getConnectionPreference() {
java.lang.Object ref = connectionPreference_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
connectionPreference_ = s;
return s;
}
}
/**
*
*
*
*
* Check the ConnectionPreference enum for the list of possible values.
*
*
* optional string connection_preference = 285818076;
*
* @return The bytes for connectionPreference.
*/
@java.lang.Override
public com.google.protobuf.ByteString getConnectionPreferenceBytes() {
java.lang.Object ref = connectionPreference_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
connectionPreference_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CREATION_TIMESTAMP_FIELD_NUMBER = 30525366;
@SuppressWarnings("serial")
private volatile java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
@java.lang.Override
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
@java.lang.Override
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 422937596;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FINGERPRINT_FIELD_NUMBER = 234678500;
@SuppressWarnings("serial")
private volatile java.lang.Object fingerprint_ = "";
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. An up-to-date fingerprint must be provided in order to patch.
*
*
* optional string fingerprint = 234678500;
*
* @return Whether the fingerprint field is set.
*/
@java.lang.Override
public boolean hasFingerprint() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. An up-to-date fingerprint must be provided in order to patch.
*
*
* optional string fingerprint = 234678500;
*
* @return The fingerprint.
*/
@java.lang.Override
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fingerprint_ = s;
return s;
}
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. An up-to-date fingerprint must be provided in order to patch.
*
*
* optional string fingerprint = 234678500;
*
* @return The bytes for fingerprint.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 3355;
private long id_ = 0L;
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int KIND_FIELD_NUMBER = 3292052;
@SuppressWarnings("serial")
private volatile java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
@java.lang.Override
public boolean hasKind() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
@java.lang.Override
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Type of the resource.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 3373707;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NETWORK_FIELD_NUMBER = 232872494;
@SuppressWarnings("serial")
private volatile java.lang.Object network_ = "";
/**
*
*
*
* [Output Only] The URL of the network which the Network Attachment belongs to. Practically it is inferred by fetching the network of the first subnetwork associated. Because it is required that all the subnetworks must be from the same network, it is assured that the Network Attachment belongs to the same network as all the subnetworks.
*
*
* optional string network = 232872494;
*
* @return Whether the network field is set.
*/
@java.lang.Override
public boolean hasNetwork() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* [Output Only] The URL of the network which the Network Attachment belongs to. Practically it is inferred by fetching the network of the first subnetwork associated. Because it is required that all the subnetworks must be from the same network, it is assured that the Network Attachment belongs to the same network as all the subnetworks.
*
*
* optional string network = 232872494;
*
* @return The network.
*/
@java.lang.Override
public java.lang.String getNetwork() {
java.lang.Object ref = network_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
network_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The URL of the network which the Network Attachment belongs to. Practically it is inferred by fetching the network of the first subnetwork associated. Because it is required that all the subnetworks must be from the same network, it is assured that the Network Attachment belongs to the same network as all the subnetworks.
*
*
* optional string network = 232872494;
*
* @return The bytes for network.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNetworkBytes() {
java.lang.Object ref = network_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
network_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PRODUCER_ACCEPT_LISTS_FIELD_NUMBER = 202804523;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList producerAcceptLists_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @return A list containing the producerAcceptLists.
*/
public com.google.protobuf.ProtocolStringList getProducerAcceptListsList() {
return producerAcceptLists_;
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @return The count of producerAcceptLists.
*/
public int getProducerAcceptListsCount() {
return producerAcceptLists_.size();
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @param index The index of the element to return.
* @return The producerAcceptLists at the given index.
*/
public java.lang.String getProducerAcceptLists(int index) {
return producerAcceptLists_.get(index);
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @param index The index of the value to return.
* @return The bytes of the producerAcceptLists at the given index.
*/
public com.google.protobuf.ByteString getProducerAcceptListsBytes(int index) {
return producerAcceptLists_.getByteString(index);
}
public static final int PRODUCER_REJECT_LISTS_FIELD_NUMBER = 4112002;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList producerRejectLists_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @return A list containing the producerRejectLists.
*/
public com.google.protobuf.ProtocolStringList getProducerRejectListsList() {
return producerRejectLists_;
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @return The count of producerRejectLists.
*/
public int getProducerRejectListsCount() {
return producerRejectLists_.size();
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @param index The index of the element to return.
* @return The producerRejectLists at the given index.
*/
public java.lang.String getProducerRejectLists(int index) {
return producerRejectLists_.get(index);
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @param index The index of the value to return.
* @return The bytes of the producerRejectLists at the given index.
*/
public com.google.protobuf.ByteString getProducerRejectListsBytes(int index) {
return producerRejectLists_.getByteString(index);
}
public static final int REGION_FIELD_NUMBER = 138946292;
@SuppressWarnings("serial")
private volatile java.lang.Object region_ = "";
/**
*
*
*
* [Output Only] URL of the region where the network attachment resides. This field applies only to the region resource. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return Whether the region field is set.
*/
@java.lang.Override
public boolean hasRegion() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* [Output Only] URL of the region where the network attachment resides. This field applies only to the region resource. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return The region.
*/
@java.lang.Override
public java.lang.String getRegion() {
java.lang.Object ref = region_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
region_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] URL of the region where the network attachment resides. This field applies only to the region resource. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return The bytes for region.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRegionBytes() {
java.lang.Object ref = region_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
region_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SELF_LINK_FIELD_NUMBER = 456214797;
@SuppressWarnings("serial")
private volatile java.lang.Object selfLink_ = "";
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return Whether the selfLink field is set.
*/
@java.lang.Override
public boolean hasSelfLink() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The selfLink.
*/
@java.lang.Override
public java.lang.String getSelfLink() {
java.lang.Object ref = selfLink_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLink_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The bytes for selfLink.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSelfLinkBytes() {
java.lang.Object ref = selfLink_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLink_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SELF_LINK_WITH_ID_FIELD_NUMBER = 44520962;
@SuppressWarnings("serial")
private volatile java.lang.Object selfLinkWithId_ = "";
/**
*
*
*
* [Output Only] Server-defined URL for this resource's resource id.
*
*
* optional string self_link_with_id = 44520962;
*
* @return Whether the selfLinkWithId field is set.
*/
@java.lang.Override
public boolean hasSelfLinkWithId() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* [Output Only] Server-defined URL for this resource's resource id.
*
*
* optional string self_link_with_id = 44520962;
*
* @return The selfLinkWithId.
*/
@java.lang.Override
public java.lang.String getSelfLinkWithId() {
java.lang.Object ref = selfLinkWithId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLinkWithId_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for this resource's resource id.
*
*
* optional string self_link_with_id = 44520962;
*
* @return The bytes for selfLinkWithId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSelfLinkWithIdBytes() {
java.lang.Object ref = selfLinkWithId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLinkWithId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBNETWORKS_FIELD_NUMBER = 415853125;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList subnetworks_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @return A list containing the subnetworks.
*/
public com.google.protobuf.ProtocolStringList getSubnetworksList() {
return subnetworks_;
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @return The count of subnetworks.
*/
public int getSubnetworksCount() {
return subnetworks_.size();
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @param index The index of the element to return.
* @return The subnetworks at the given index.
*/
public java.lang.String getSubnetworks(int index) {
return subnetworks_.get(index);
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @param index The index of the value to return.
* @return The bytes of the subnetworks at the given index.
*/
public com.google.protobuf.ByteString getSubnetworksBytes(int index) {
return subnetworks_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000010) != 0)) {
output.writeUInt64(3355, id_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3292052, kind_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3373707, name_);
}
for (int i = 0; i < producerRejectLists_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 4112002, producerRejectLists_.getRaw(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30525366, creationTimestamp_);
}
if (((bitField0_ & 0x00000400) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 44520962, selfLinkWithId_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 138946292, region_);
}
for (int i = 0; i < producerAcceptLists_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 202804523, producerAcceptLists_.getRaw(i));
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 232872494, network_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 234678500, fingerprint_);
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 285818076, connectionPreference_);
}
for (int i = 0; i < connectionEndpoints_.size(); i++) {
output.writeMessage(326078813, connectionEndpoints_.get(i));
}
for (int i = 0; i < subnetworks_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 415853125, subnetworks_.getRaw(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 422937596, description_);
}
if (((bitField0_ & 0x00000200) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 456214797, selfLink_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeUInt64Size(3355, id_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3292052, kind_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3373707, name_);
}
{
int dataSize = 0;
for (int i = 0; i < producerRejectLists_.size(); i++) {
dataSize += computeStringSizeNoTag(producerRejectLists_.getRaw(i));
}
size += dataSize;
size += 4 * getProducerRejectListsList().size();
}
if (((bitField0_ & 0x00000002) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(30525366, creationTimestamp_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(44520962, selfLinkWithId_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(138946292, region_);
}
{
int dataSize = 0;
for (int i = 0; i < producerAcceptLists_.size(); i++) {
dataSize += computeStringSizeNoTag(producerAcceptLists_.getRaw(i));
}
size += dataSize;
size += 5 * getProducerAcceptListsList().size();
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(232872494, network_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(234678500, fingerprint_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(
285818076, connectionPreference_);
}
for (int i = 0; i < connectionEndpoints_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
326078813, connectionEndpoints_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < subnetworks_.size(); i++) {
dataSize += computeStringSizeNoTag(subnetworks_.getRaw(i));
}
size += dataSize;
size += 5 * getSubnetworksList().size();
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(422937596, description_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(456214797, selfLink_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.NetworkAttachment)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.NetworkAttachment other =
(com.google.cloud.compute.v1.NetworkAttachment) obj;
if (!getConnectionEndpointsList().equals(other.getConnectionEndpointsList())) return false;
if (hasConnectionPreference() != other.hasConnectionPreference()) return false;
if (hasConnectionPreference()) {
if (!getConnectionPreference().equals(other.getConnectionPreference())) return false;
}
if (hasCreationTimestamp() != other.hasCreationTimestamp()) return false;
if (hasCreationTimestamp()) {
if (!getCreationTimestamp().equals(other.getCreationTimestamp())) return false;
}
if (hasDescription() != other.hasDescription()) return false;
if (hasDescription()) {
if (!getDescription().equals(other.getDescription())) return false;
}
if (hasFingerprint() != other.hasFingerprint()) return false;
if (hasFingerprint()) {
if (!getFingerprint().equals(other.getFingerprint())) return false;
}
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId() != other.getId()) return false;
}
if (hasKind() != other.hasKind()) return false;
if (hasKind()) {
if (!getKind().equals(other.getKind())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName().equals(other.getName())) return false;
}
if (hasNetwork() != other.hasNetwork()) return false;
if (hasNetwork()) {
if (!getNetwork().equals(other.getNetwork())) return false;
}
if (!getProducerAcceptListsList().equals(other.getProducerAcceptListsList())) return false;
if (!getProducerRejectListsList().equals(other.getProducerRejectListsList())) return false;
if (hasRegion() != other.hasRegion()) return false;
if (hasRegion()) {
if (!getRegion().equals(other.getRegion())) return false;
}
if (hasSelfLink() != other.hasSelfLink()) return false;
if (hasSelfLink()) {
if (!getSelfLink().equals(other.getSelfLink())) return false;
}
if (hasSelfLinkWithId() != other.hasSelfLinkWithId()) return false;
if (hasSelfLinkWithId()) {
if (!getSelfLinkWithId().equals(other.getSelfLinkWithId())) return false;
}
if (!getSubnetworksList().equals(other.getSubnetworksList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getConnectionEndpointsCount() > 0) {
hash = (37 * hash) + CONNECTION_ENDPOINTS_FIELD_NUMBER;
hash = (53 * hash) + getConnectionEndpointsList().hashCode();
}
if (hasConnectionPreference()) {
hash = (37 * hash) + CONNECTION_PREFERENCE_FIELD_NUMBER;
hash = (53 * hash) + getConnectionPreference().hashCode();
}
if (hasCreationTimestamp()) {
hash = (37 * hash) + CREATION_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getCreationTimestamp().hashCode();
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
if (hasFingerprint()) {
hash = (37 * hash) + FINGERPRINT_FIELD_NUMBER;
hash = (53 * hash) + getFingerprint().hashCode();
}
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getId());
}
if (hasKind()) {
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + getKind().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasNetwork()) {
hash = (37 * hash) + NETWORK_FIELD_NUMBER;
hash = (53 * hash) + getNetwork().hashCode();
}
if (getProducerAcceptListsCount() > 0) {
hash = (37 * hash) + PRODUCER_ACCEPT_LISTS_FIELD_NUMBER;
hash = (53 * hash) + getProducerAcceptListsList().hashCode();
}
if (getProducerRejectListsCount() > 0) {
hash = (37 * hash) + PRODUCER_REJECT_LISTS_FIELD_NUMBER;
hash = (53 * hash) + getProducerRejectListsList().hashCode();
}
if (hasRegion()) {
hash = (37 * hash) + REGION_FIELD_NUMBER;
hash = (53 * hash) + getRegion().hashCode();
}
if (hasSelfLink()) {
hash = (37 * hash) + SELF_LINK_FIELD_NUMBER;
hash = (53 * hash) + getSelfLink().hashCode();
}
if (hasSelfLinkWithId()) {
hash = (37 * hash) + SELF_LINK_WITH_ID_FIELD_NUMBER;
hash = (53 * hash) + getSelfLinkWithId().hashCode();
}
if (getSubnetworksCount() > 0) {
hash = (37 * hash) + SUBNETWORKS_FIELD_NUMBER;
hash = (53 * hash) + getSubnetworksList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.NetworkAttachment parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.NetworkAttachment parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkAttachment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.NetworkAttachment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkAttachment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.NetworkAttachment parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkAttachment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.NetworkAttachment parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkAttachment parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.NetworkAttachment parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkAttachment parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.NetworkAttachment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.NetworkAttachment prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* NetworkAttachments A network attachment resource ...
*
*
* Protobuf type {@code google.cloud.compute.v1.NetworkAttachment}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.NetworkAttachment)
com.google.cloud.compute.v1.NetworkAttachmentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkAttachment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkAttachment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.NetworkAttachment.class,
com.google.cloud.compute.v1.NetworkAttachment.Builder.class);
}
// Construct using com.google.cloud.compute.v1.NetworkAttachment.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (connectionEndpointsBuilder_ == null) {
connectionEndpoints_ = java.util.Collections.emptyList();
} else {
connectionEndpoints_ = null;
connectionEndpointsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
connectionPreference_ = "";
creationTimestamp_ = "";
description_ = "";
fingerprint_ = "";
id_ = 0L;
kind_ = "";
name_ = "";
network_ = "";
producerAcceptLists_ = com.google.protobuf.LazyStringArrayList.emptyList();
producerRejectLists_ = com.google.protobuf.LazyStringArrayList.emptyList();
region_ = "";
selfLink_ = "";
selfLinkWithId_ = "";
subnetworks_ = com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkAttachment_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkAttachment getDefaultInstanceForType() {
return com.google.cloud.compute.v1.NetworkAttachment.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkAttachment build() {
com.google.cloud.compute.v1.NetworkAttachment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkAttachment buildPartial() {
com.google.cloud.compute.v1.NetworkAttachment result =
new com.google.cloud.compute.v1.NetworkAttachment(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.cloud.compute.v1.NetworkAttachment result) {
if (connectionEndpointsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
connectionEndpoints_ = java.util.Collections.unmodifiableList(connectionEndpoints_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.connectionEndpoints_ = connectionEndpoints_;
} else {
result.connectionEndpoints_ = connectionEndpointsBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.compute.v1.NetworkAttachment result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.connectionPreference_ = connectionPreference_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.creationTimestamp_ = creationTimestamp_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.description_ = description_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.fingerprint_ = fingerprint_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.kind_ = kind_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.network_ = network_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
producerAcceptLists_.makeImmutable();
result.producerAcceptLists_ = producerAcceptLists_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
producerRejectLists_.makeImmutable();
result.producerRejectLists_ = producerRejectLists_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.region_ = region_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.selfLink_ = selfLink_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.selfLinkWithId_ = selfLinkWithId_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
subnetworks_.makeImmutable();
result.subnetworks_ = subnetworks_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.NetworkAttachment) {
return mergeFrom((com.google.cloud.compute.v1.NetworkAttachment) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.NetworkAttachment other) {
if (other == com.google.cloud.compute.v1.NetworkAttachment.getDefaultInstance()) return this;
if (connectionEndpointsBuilder_ == null) {
if (!other.connectionEndpoints_.isEmpty()) {
if (connectionEndpoints_.isEmpty()) {
connectionEndpoints_ = other.connectionEndpoints_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureConnectionEndpointsIsMutable();
connectionEndpoints_.addAll(other.connectionEndpoints_);
}
onChanged();
}
} else {
if (!other.connectionEndpoints_.isEmpty()) {
if (connectionEndpointsBuilder_.isEmpty()) {
connectionEndpointsBuilder_.dispose();
connectionEndpointsBuilder_ = null;
connectionEndpoints_ = other.connectionEndpoints_;
bitField0_ = (bitField0_ & ~0x00000001);
connectionEndpointsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getConnectionEndpointsFieldBuilder()
: null;
} else {
connectionEndpointsBuilder_.addAllMessages(other.connectionEndpoints_);
}
}
}
if (other.hasConnectionPreference()) {
connectionPreference_ = other.connectionPreference_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasCreationTimestamp()) {
creationTimestamp_ = other.creationTimestamp_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasDescription()) {
description_ = other.description_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasFingerprint()) {
fingerprint_ = other.fingerprint_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasKind()) {
kind_ = other.kind_;
bitField0_ |= 0x00000040;
onChanged();
}
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000080;
onChanged();
}
if (other.hasNetwork()) {
network_ = other.network_;
bitField0_ |= 0x00000100;
onChanged();
}
if (!other.producerAcceptLists_.isEmpty()) {
if (producerAcceptLists_.isEmpty()) {
producerAcceptLists_ = other.producerAcceptLists_;
bitField0_ |= 0x00000200;
} else {
ensureProducerAcceptListsIsMutable();
producerAcceptLists_.addAll(other.producerAcceptLists_);
}
onChanged();
}
if (!other.producerRejectLists_.isEmpty()) {
if (producerRejectLists_.isEmpty()) {
producerRejectLists_ = other.producerRejectLists_;
bitField0_ |= 0x00000400;
} else {
ensureProducerRejectListsIsMutable();
producerRejectLists_.addAll(other.producerRejectLists_);
}
onChanged();
}
if (other.hasRegion()) {
region_ = other.region_;
bitField0_ |= 0x00000800;
onChanged();
}
if (other.hasSelfLink()) {
selfLink_ = other.selfLink_;
bitField0_ |= 0x00001000;
onChanged();
}
if (other.hasSelfLinkWithId()) {
selfLinkWithId_ = other.selfLinkWithId_;
bitField0_ |= 0x00002000;
onChanged();
}
if (!other.subnetworks_.isEmpty()) {
if (subnetworks_.isEmpty()) {
subnetworks_ = other.subnetworks_;
bitField0_ |= 0x00004000;
} else {
ensureSubnetworksIsMutable();
subnetworks_.addAll(other.subnetworks_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 26840:
{
id_ = input.readUInt64();
bitField0_ |= 0x00000020;
break;
} // case 26840
case 26336418:
{
kind_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 26336418
case 26989658:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 26989658
case 32896018:
{
java.lang.String s = input.readStringRequireUtf8();
ensureProducerRejectListsIsMutable();
producerRejectLists_.add(s);
break;
} // case 32896018
case 244202930:
{
creationTimestamp_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 244202930
case 356167698:
{
selfLinkWithId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00002000;
break;
} // case 356167698
case 1111570338:
{
region_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000800;
break;
} // case 1111570338
case 1622436186:
{
java.lang.String s = input.readStringRequireUtf8();
ensureProducerAcceptListsIsMutable();
producerAcceptLists_.add(s);
break;
} // case 1622436186
case 1862979954:
{
network_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case 1862979954
case 1877428002:
{
fingerprint_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 1877428002
case -2008422686:
{
connectionPreference_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case -2008422686
case -1686336790:
{
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint m =
input.readMessage(
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.parser(),
extensionRegistry);
if (connectionEndpointsBuilder_ == null) {
ensureConnectionEndpointsIsMutable();
connectionEndpoints_.add(m);
} else {
connectionEndpointsBuilder_.addMessage(m);
}
break;
} // case -1686336790
case -968142294:
{
java.lang.String s = input.readStringRequireUtf8();
ensureSubnetworksIsMutable();
subnetworks_.add(s);
break;
} // case -968142294
case -911466526:
{
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case -911466526
case -645248918:
{
selfLink_ = input.readStringRequireUtf8();
bitField0_ |= 0x00001000;
break;
} // case -645248918
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List
connectionEndpoints_ = java.util.Collections.emptyList();
private void ensureConnectionEndpointsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
connectionEndpoints_ =
new java.util.ArrayList(
connectionEndpoints_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint,
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.Builder,
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpointOrBuilder>
connectionEndpointsBuilder_;
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public java.util.List
getConnectionEndpointsList() {
if (connectionEndpointsBuilder_ == null) {
return java.util.Collections.unmodifiableList(connectionEndpoints_);
} else {
return connectionEndpointsBuilder_.getMessageList();
}
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public int getConnectionEndpointsCount() {
if (connectionEndpointsBuilder_ == null) {
return connectionEndpoints_.size();
} else {
return connectionEndpointsBuilder_.getCount();
}
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint getConnectionEndpoints(
int index) {
if (connectionEndpointsBuilder_ == null) {
return connectionEndpoints_.get(index);
} else {
return connectionEndpointsBuilder_.getMessage(index);
}
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public Builder setConnectionEndpoints(
int index, com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint value) {
if (connectionEndpointsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConnectionEndpointsIsMutable();
connectionEndpoints_.set(index, value);
onChanged();
} else {
connectionEndpointsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public Builder setConnectionEndpoints(
int index,
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.Builder builderForValue) {
if (connectionEndpointsBuilder_ == null) {
ensureConnectionEndpointsIsMutable();
connectionEndpoints_.set(index, builderForValue.build());
onChanged();
} else {
connectionEndpointsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public Builder addConnectionEndpoints(
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint value) {
if (connectionEndpointsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConnectionEndpointsIsMutable();
connectionEndpoints_.add(value);
onChanged();
} else {
connectionEndpointsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public Builder addConnectionEndpoints(
int index, com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint value) {
if (connectionEndpointsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConnectionEndpointsIsMutable();
connectionEndpoints_.add(index, value);
onChanged();
} else {
connectionEndpointsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public Builder addConnectionEndpoints(
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.Builder builderForValue) {
if (connectionEndpointsBuilder_ == null) {
ensureConnectionEndpointsIsMutable();
connectionEndpoints_.add(builderForValue.build());
onChanged();
} else {
connectionEndpointsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public Builder addConnectionEndpoints(
int index,
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.Builder builderForValue) {
if (connectionEndpointsBuilder_ == null) {
ensureConnectionEndpointsIsMutable();
connectionEndpoints_.add(index, builderForValue.build());
onChanged();
} else {
connectionEndpointsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public Builder addAllConnectionEndpoints(
java.lang.Iterable extends com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint>
values) {
if (connectionEndpointsBuilder_ == null) {
ensureConnectionEndpointsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, connectionEndpoints_);
onChanged();
} else {
connectionEndpointsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public Builder clearConnectionEndpoints() {
if (connectionEndpointsBuilder_ == null) {
connectionEndpoints_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
connectionEndpointsBuilder_.clear();
}
return this;
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public Builder removeConnectionEndpoints(int index) {
if (connectionEndpointsBuilder_ == null) {
ensureConnectionEndpointsIsMutable();
connectionEndpoints_.remove(index);
onChanged();
} else {
connectionEndpointsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.Builder
getConnectionEndpointsBuilder(int index) {
return getConnectionEndpointsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpointOrBuilder
getConnectionEndpointsOrBuilder(int index) {
if (connectionEndpointsBuilder_ == null) {
return connectionEndpoints_.get(index);
} else {
return connectionEndpointsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public java.util.List<
? extends com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpointOrBuilder>
getConnectionEndpointsOrBuilderList() {
if (connectionEndpointsBuilder_ != null) {
return connectionEndpointsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(connectionEndpoints_);
}
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.Builder
addConnectionEndpointsBuilder() {
return getConnectionEndpointsFieldBuilder()
.addBuilder(
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.getDefaultInstance());
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.Builder
addConnectionEndpointsBuilder(int index) {
return getConnectionEndpointsFieldBuilder()
.addBuilder(
index,
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.getDefaultInstance());
}
/**
*
*
*
* [Output Only] An array of connections for all the producers connected to this network attachment.
*
*
*
* repeated .google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint connection_endpoints = 326078813;
*
*/
public java.util.List
getConnectionEndpointsBuilderList() {
return getConnectionEndpointsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint,
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.Builder,
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpointOrBuilder>
getConnectionEndpointsFieldBuilder() {
if (connectionEndpointsBuilder_ == null) {
connectionEndpointsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint,
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpoint.Builder,
com.google.cloud.compute.v1.NetworkAttachmentConnectedEndpointOrBuilder>(
connectionEndpoints_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
connectionEndpoints_ = null;
}
return connectionEndpointsBuilder_;
}
private java.lang.Object connectionPreference_ = "";
/**
*
*
*
*
* Check the ConnectionPreference enum for the list of possible values.
*
*
* optional string connection_preference = 285818076;
*
* @return Whether the connectionPreference field is set.
*/
public boolean hasConnectionPreference() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
*
* Check the ConnectionPreference enum for the list of possible values.
*
*
* optional string connection_preference = 285818076;
*
* @return The connectionPreference.
*/
public java.lang.String getConnectionPreference() {
java.lang.Object ref = connectionPreference_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
connectionPreference_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
*
* Check the ConnectionPreference enum for the list of possible values.
*
*
* optional string connection_preference = 285818076;
*
* @return The bytes for connectionPreference.
*/
public com.google.protobuf.ByteString getConnectionPreferenceBytes() {
java.lang.Object ref = connectionPreference_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
connectionPreference_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
*
* Check the ConnectionPreference enum for the list of possible values.
*
*
* optional string connection_preference = 285818076;
*
* @param value The connectionPreference to set.
* @return This builder for chaining.
*/
public Builder setConnectionPreference(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
connectionPreference_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
*
* Check the ConnectionPreference enum for the list of possible values.
*
*
* optional string connection_preference = 285818076;
*
* @return This builder for chaining.
*/
public Builder clearConnectionPreference() {
connectionPreference_ = getDefaultInstance().getConnectionPreference();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
*
* Check the ConnectionPreference enum for the list of possible values.
*
*
* optional string connection_preference = 285818076;
*
* @param value The bytes for connectionPreference to set.
* @return This builder for chaining.
*/
public Builder setConnectionPreferenceBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
connectionPreference_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestamp(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
creationTimestamp_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return This builder for chaining.
*/
public Builder clearCreationTimestamp() {
creationTimestamp_ = getDefaultInstance().getCreationTimestamp();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The bytes for creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestampBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
creationTimestamp_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return Whether the description field is set.
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The bytes for description.
*/
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object fingerprint_ = "";
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. An up-to-date fingerprint must be provided in order to patch.
*
*
* optional string fingerprint = 234678500;
*
* @return Whether the fingerprint field is set.
*/
public boolean hasFingerprint() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. An up-to-date fingerprint must be provided in order to patch.
*
*
* optional string fingerprint = 234678500;
*
* @return The fingerprint.
*/
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fingerprint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. An up-to-date fingerprint must be provided in order to patch.
*
*
* optional string fingerprint = 234678500;
*
* @return The bytes for fingerprint.
*/
public com.google.protobuf.ByteString getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. An up-to-date fingerprint must be provided in order to patch.
*
*
* optional string fingerprint = 234678500;
*
* @param value The fingerprint to set.
* @return This builder for chaining.
*/
public Builder setFingerprint(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
fingerprint_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. An up-to-date fingerprint must be provided in order to patch.
*
*
* optional string fingerprint = 234678500;
*
* @return This builder for chaining.
*/
public Builder clearFingerprint() {
fingerprint_ = getDefaultInstance().getFingerprint();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. An up-to-date fingerprint must be provided in order to patch.
*
*
* optional string fingerprint = 234678500;
*
* @param value The bytes for fingerprint to set.
* @return This builder for chaining.
*/
public Builder setFingerprintBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fingerprint_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private long id_;
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
id_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000020);
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
public boolean hasKind() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource.
*
*
* optional string kind = 3292052;
*
* @param value The kind to set.
* @return This builder for chaining.
*/
public Builder setKind(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource.
*
*
* optional string kind = 3292052;
*
* @return This builder for chaining.
*/
public Builder clearKind() {
kind_ = getDefaultInstance().getKind();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource.
*
*
* optional string kind = 3292052;
*
* @param value The bytes for kind to set.
* @return This builder for chaining.
*/
public Builder setKindBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
kind_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private java.lang.Object network_ = "";
/**
*
*
*
* [Output Only] The URL of the network which the Network Attachment belongs to. Practically it is inferred by fetching the network of the first subnetwork associated. Because it is required that all the subnetworks must be from the same network, it is assured that the Network Attachment belongs to the same network as all the subnetworks.
*
*
* optional string network = 232872494;
*
* @return Whether the network field is set.
*/
public boolean hasNetwork() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* [Output Only] The URL of the network which the Network Attachment belongs to. Practically it is inferred by fetching the network of the first subnetwork associated. Because it is required that all the subnetworks must be from the same network, it is assured that the Network Attachment belongs to the same network as all the subnetworks.
*
*
* optional string network = 232872494;
*
* @return The network.
*/
public java.lang.String getNetwork() {
java.lang.Object ref = network_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
network_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The URL of the network which the Network Attachment belongs to. Practically it is inferred by fetching the network of the first subnetwork associated. Because it is required that all the subnetworks must be from the same network, it is assured that the Network Attachment belongs to the same network as all the subnetworks.
*
*
* optional string network = 232872494;
*
* @return The bytes for network.
*/
public com.google.protobuf.ByteString getNetworkBytes() {
java.lang.Object ref = network_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
network_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The URL of the network which the Network Attachment belongs to. Practically it is inferred by fetching the network of the first subnetwork associated. Because it is required that all the subnetworks must be from the same network, it is assured that the Network Attachment belongs to the same network as all the subnetworks.
*
*
* optional string network = 232872494;
*
* @param value The network to set.
* @return This builder for chaining.
*/
public Builder setNetwork(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
network_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The URL of the network which the Network Attachment belongs to. Practically it is inferred by fetching the network of the first subnetwork associated. Because it is required that all the subnetworks must be from the same network, it is assured that the Network Attachment belongs to the same network as all the subnetworks.
*
*
* optional string network = 232872494;
*
* @return This builder for chaining.
*/
public Builder clearNetwork() {
network_ = getDefaultInstance().getNetwork();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The URL of the network which the Network Attachment belongs to. Practically it is inferred by fetching the network of the first subnetwork associated. Because it is required that all the subnetworks must be from the same network, it is assured that the Network Attachment belongs to the same network as all the subnetworks.
*
*
* optional string network = 232872494;
*
* @param value The bytes for network to set.
* @return This builder for chaining.
*/
public Builder setNetworkBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
network_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList producerAcceptLists_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureProducerAcceptListsIsMutable() {
if (!producerAcceptLists_.isModifiable()) {
producerAcceptLists_ = new com.google.protobuf.LazyStringArrayList(producerAcceptLists_);
}
bitField0_ |= 0x00000200;
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @return A list containing the producerAcceptLists.
*/
public com.google.protobuf.ProtocolStringList getProducerAcceptListsList() {
producerAcceptLists_.makeImmutable();
return producerAcceptLists_;
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @return The count of producerAcceptLists.
*/
public int getProducerAcceptListsCount() {
return producerAcceptLists_.size();
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @param index The index of the element to return.
* @return The producerAcceptLists at the given index.
*/
public java.lang.String getProducerAcceptLists(int index) {
return producerAcceptLists_.get(index);
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @param index The index of the value to return.
* @return The bytes of the producerAcceptLists at the given index.
*/
public com.google.protobuf.ByteString getProducerAcceptListsBytes(int index) {
return producerAcceptLists_.getByteString(index);
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @param index The index to set the value at.
* @param value The producerAcceptLists to set.
* @return This builder for chaining.
*/
public Builder setProducerAcceptLists(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureProducerAcceptListsIsMutable();
producerAcceptLists_.set(index, value);
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @param value The producerAcceptLists to add.
* @return This builder for chaining.
*/
public Builder addProducerAcceptLists(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureProducerAcceptListsIsMutable();
producerAcceptLists_.add(value);
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @param values The producerAcceptLists to add.
* @return This builder for chaining.
*/
public Builder addAllProducerAcceptLists(java.lang.Iterable values) {
ensureProducerAcceptListsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, producerAcceptLists_);
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @return This builder for chaining.
*/
public Builder clearProducerAcceptLists() {
producerAcceptLists_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
;
onChanged();
return this;
}
/**
*
*
*
* Projects that are allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_accept_lists = 202804523;
*
* @param value The bytes of the producerAcceptLists to add.
* @return This builder for chaining.
*/
public Builder addProducerAcceptListsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureProducerAcceptListsIsMutable();
producerAcceptLists_.add(value);
bitField0_ |= 0x00000200;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList producerRejectLists_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureProducerRejectListsIsMutable() {
if (!producerRejectLists_.isModifiable()) {
producerRejectLists_ = new com.google.protobuf.LazyStringArrayList(producerRejectLists_);
}
bitField0_ |= 0x00000400;
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @return A list containing the producerRejectLists.
*/
public com.google.protobuf.ProtocolStringList getProducerRejectListsList() {
producerRejectLists_.makeImmutable();
return producerRejectLists_;
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @return The count of producerRejectLists.
*/
public int getProducerRejectListsCount() {
return producerRejectLists_.size();
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @param index The index of the element to return.
* @return The producerRejectLists at the given index.
*/
public java.lang.String getProducerRejectLists(int index) {
return producerRejectLists_.get(index);
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @param index The index of the value to return.
* @return The bytes of the producerRejectLists at the given index.
*/
public com.google.protobuf.ByteString getProducerRejectListsBytes(int index) {
return producerRejectLists_.getByteString(index);
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @param index The index to set the value at.
* @param value The producerRejectLists to set.
* @return This builder for chaining.
*/
public Builder setProducerRejectLists(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureProducerRejectListsIsMutable();
producerRejectLists_.set(index, value);
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @param value The producerRejectLists to add.
* @return This builder for chaining.
*/
public Builder addProducerRejectLists(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureProducerRejectListsIsMutable();
producerRejectLists_.add(value);
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @param values The producerRejectLists to add.
* @return This builder for chaining.
*/
public Builder addAllProducerRejectLists(java.lang.Iterable values) {
ensureProducerRejectListsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, producerRejectLists_);
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @return This builder for chaining.
*/
public Builder clearProducerRejectLists() {
producerRejectLists_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
;
onChanged();
return this;
}
/**
*
*
*
* Projects that are not allowed to connect to this network attachment. The project can be specified using its id or number.
*
*
* repeated string producer_reject_lists = 4112002;
*
* @param value The bytes of the producerRejectLists to add.
* @return This builder for chaining.
*/
public Builder addProducerRejectListsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureProducerRejectListsIsMutable();
producerRejectLists_.add(value);
bitField0_ |= 0x00000400;
onChanged();
return this;
}
private java.lang.Object region_ = "";
/**
*
*
*
* [Output Only] URL of the region where the network attachment resides. This field applies only to the region resource. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return Whether the region field is set.
*/
public boolean hasRegion() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* [Output Only] URL of the region where the network attachment resides. This field applies only to the region resource. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return The region.
*/
public java.lang.String getRegion() {
java.lang.Object ref = region_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
region_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] URL of the region where the network attachment resides. This field applies only to the region resource. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return The bytes for region.
*/
public com.google.protobuf.ByteString getRegionBytes() {
java.lang.Object ref = region_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
region_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] URL of the region where the network attachment resides. This field applies only to the region resource. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @param value The region to set.
* @return This builder for chaining.
*/
public Builder setRegion(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
region_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] URL of the region where the network attachment resides. This field applies only to the region resource. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @return This builder for chaining.
*/
public Builder clearRegion() {
region_ = getDefaultInstance().getRegion();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] URL of the region where the network attachment resides. This field applies only to the region resource. You must specify this field as part of the HTTP request URL. It is not settable as a field in the request body.
*
*
* optional string region = 138946292;
*
* @param value The bytes for region to set.
* @return This builder for chaining.
*/
public Builder setRegionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
region_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
private java.lang.Object selfLink_ = "";
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return Whether the selfLink field is set.
*/
public boolean hasSelfLink() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The selfLink.
*/
public java.lang.String getSelfLink() {
java.lang.Object ref = selfLink_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLink_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The bytes for selfLink.
*/
public com.google.protobuf.ByteString getSelfLinkBytes() {
java.lang.Object ref = selfLink_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLink_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @param value The selfLink to set.
* @return This builder for chaining.
*/
public Builder setSelfLink(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
selfLink_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return This builder for chaining.
*/
public Builder clearSelfLink() {
selfLink_ = getDefaultInstance().getSelfLink();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @param value The bytes for selfLink to set.
* @return This builder for chaining.
*/
public Builder setSelfLinkBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
selfLink_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
private java.lang.Object selfLinkWithId_ = "";
/**
*
*
*
* [Output Only] Server-defined URL for this resource's resource id.
*
*
* optional string self_link_with_id = 44520962;
*
* @return Whether the selfLinkWithId field is set.
*/
public boolean hasSelfLinkWithId() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*
*
* [Output Only] Server-defined URL for this resource's resource id.
*
*
* optional string self_link_with_id = 44520962;
*
* @return The selfLinkWithId.
*/
public java.lang.String getSelfLinkWithId() {
java.lang.Object ref = selfLinkWithId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLinkWithId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for this resource's resource id.
*
*
* optional string self_link_with_id = 44520962;
*
* @return The bytes for selfLinkWithId.
*/
public com.google.protobuf.ByteString getSelfLinkWithIdBytes() {
java.lang.Object ref = selfLinkWithId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLinkWithId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for this resource's resource id.
*
*
* optional string self_link_with_id = 44520962;
*
* @param value The selfLinkWithId to set.
* @return This builder for chaining.
*/
public Builder setSelfLinkWithId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
selfLinkWithId_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined URL for this resource's resource id.
*
*
* optional string self_link_with_id = 44520962;
*
* @return This builder for chaining.
*/
public Builder clearSelfLinkWithId() {
selfLinkWithId_ = getDefaultInstance().getSelfLinkWithId();
bitField0_ = (bitField0_ & ~0x00002000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined URL for this resource's resource id.
*
*
* optional string self_link_with_id = 44520962;
*
* @param value The bytes for selfLinkWithId to set.
* @return This builder for chaining.
*/
public Builder setSelfLinkWithIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
selfLinkWithId_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList subnetworks_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureSubnetworksIsMutable() {
if (!subnetworks_.isModifiable()) {
subnetworks_ = new com.google.protobuf.LazyStringArrayList(subnetworks_);
}
bitField0_ |= 0x00004000;
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @return A list containing the subnetworks.
*/
public com.google.protobuf.ProtocolStringList getSubnetworksList() {
subnetworks_.makeImmutable();
return subnetworks_;
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @return The count of subnetworks.
*/
public int getSubnetworksCount() {
return subnetworks_.size();
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @param index The index of the element to return.
* @return The subnetworks at the given index.
*/
public java.lang.String getSubnetworks(int index) {
return subnetworks_.get(index);
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @param index The index of the value to return.
* @return The bytes of the subnetworks at the given index.
*/
public com.google.protobuf.ByteString getSubnetworksBytes(int index) {
return subnetworks_.getByteString(index);
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @param index The index to set the value at.
* @param value The subnetworks to set.
* @return This builder for chaining.
*/
public Builder setSubnetworks(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSubnetworksIsMutable();
subnetworks_.set(index, value);
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @param value The subnetworks to add.
* @return This builder for chaining.
*/
public Builder addSubnetworks(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSubnetworksIsMutable();
subnetworks_.add(value);
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @param values The subnetworks to add.
* @return This builder for chaining.
*/
public Builder addAllSubnetworks(java.lang.Iterable values) {
ensureSubnetworksIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, subnetworks_);
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @return This builder for chaining.
*/
public Builder clearSubnetworks() {
subnetworks_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00004000);
;
onChanged();
return this;
}
/**
*
*
*
* An array of URLs where each entry is the URL of a subnet provided by the service consumer to use for endpoints in the producers that connect to this network attachment.
*
*
* repeated string subnetworks = 415853125;
*
* @param value The bytes of the subnetworks to add.
* @return This builder for chaining.
*/
public Builder addSubnetworksBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSubnetworksIsMutable();
subnetworks_.add(value);
bitField0_ |= 0x00004000;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.NetworkAttachment)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.NetworkAttachment)
private static final com.google.cloud.compute.v1.NetworkAttachment DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.NetworkAttachment();
}
public static com.google.cloud.compute.v1.NetworkAttachment getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NetworkAttachment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkAttachment getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy