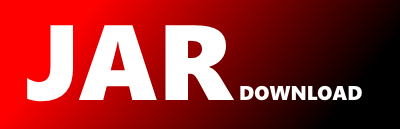
com.google.cloud.compute.v1.NetworkInterface Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* A network interface resource attached to an instance.
*
*
* Protobuf type {@code google.cloud.compute.v1.NetworkInterface}
*/
public final class NetworkInterface extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.NetworkInterface)
NetworkInterfaceOrBuilder {
private static final long serialVersionUID = 0L;
// Use NetworkInterface.newBuilder() to construct.
private NetworkInterface(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NetworkInterface() {
accessConfigs_ = java.util.Collections.emptyList();
aliasIpRanges_ = java.util.Collections.emptyList();
fingerprint_ = "";
ipv6AccessConfigs_ = java.util.Collections.emptyList();
ipv6AccessType_ = "";
ipv6Address_ = "";
kind_ = "";
name_ = "";
network_ = "";
networkAttachment_ = "";
networkIP_ = "";
nicType_ = "";
stackType_ = "";
subnetwork_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new NetworkInterface();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkInterface_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkInterface_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.NetworkInterface.class,
com.google.cloud.compute.v1.NetworkInterface.Builder.class);
}
/**
*
*
*
* [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed from the Internet. This field is always inherited from its subnetwork. Valid only if stackType is IPV4_IPV6.
*
*
* Protobuf enum {@code google.cloud.compute.v1.NetworkInterface.Ipv6AccessType}
*/
public enum Ipv6AccessType implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_IPV6_ACCESS_TYPE = 0;
*/
UNDEFINED_IPV6_ACCESS_TYPE(0),
/**
*
*
*
* This network interface can have external IPv6.
*
*
* EXTERNAL = 35607499;
*/
EXTERNAL(35607499),
/**
*
*
*
* This network interface can have internal IPv6.
*
*
* INTERNAL = 279295677;
*/
INTERNAL(279295677),
/** UNSPECIFIED_IPV6_ACCESS_TYPE = 313080613;
*/
UNSPECIFIED_IPV6_ACCESS_TYPE(313080613),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_IPV6_ACCESS_TYPE = 0;
*/
public static final int UNDEFINED_IPV6_ACCESS_TYPE_VALUE = 0;
/**
*
*
*
* This network interface can have external IPv6.
*
*
* EXTERNAL = 35607499;
*/
public static final int EXTERNAL_VALUE = 35607499;
/**
*
*
*
* This network interface can have internal IPv6.
*
*
* INTERNAL = 279295677;
*/
public static final int INTERNAL_VALUE = 279295677;
/** UNSPECIFIED_IPV6_ACCESS_TYPE = 313080613;
*/
public static final int UNSPECIFIED_IPV6_ACCESS_TYPE_VALUE = 313080613;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Ipv6AccessType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Ipv6AccessType forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_IPV6_ACCESS_TYPE;
case 35607499:
return EXTERNAL;
case 279295677:
return INTERNAL;
case 313080613:
return UNSPECIFIED_IPV6_ACCESS_TYPE;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Ipv6AccessType findValueByNumber(int number) {
return Ipv6AccessType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.NetworkInterface.getDescriptor().getEnumTypes().get(0);
}
private static final Ipv6AccessType[] VALUES = values();
public static Ipv6AccessType valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Ipv6AccessType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.NetworkInterface.Ipv6AccessType)
}
/**
*
*
*
* The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
*
*
* Protobuf enum {@code google.cloud.compute.v1.NetworkInterface.NicType}
*/
public enum NicType implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_NIC_TYPE = 0;
*/
UNDEFINED_NIC_TYPE(0),
/**
*
*
*
* GVNIC
*
*
* GVNIC = 68209305;
*/
GVNIC(68209305),
/**
*
*
*
* IDPF
*
*
* IDPF = 2242641;
*/
IDPF(2242641),
/**
*
*
*
* IRDMA
*
*
* IRDMA = 69927695;
*/
IRDMA(69927695),
/**
*
*
*
* MRDMA
*
*
* MRDMA = 73621779;
*/
MRDMA(73621779),
/**
*
*
*
* No type specified.
*
*
* UNSPECIFIED_NIC_TYPE = 67411801;
*/
UNSPECIFIED_NIC_TYPE(67411801),
/**
*
*
*
* VIRTIO
*
*
* VIRTIO_NET = 452123481;
*/
VIRTIO_NET(452123481),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_NIC_TYPE = 0;
*/
public static final int UNDEFINED_NIC_TYPE_VALUE = 0;
/**
*
*
*
* GVNIC
*
*
* GVNIC = 68209305;
*/
public static final int GVNIC_VALUE = 68209305;
/**
*
*
*
* IDPF
*
*
* IDPF = 2242641;
*/
public static final int IDPF_VALUE = 2242641;
/**
*
*
*
* IRDMA
*
*
* IRDMA = 69927695;
*/
public static final int IRDMA_VALUE = 69927695;
/**
*
*
*
* MRDMA
*
*
* MRDMA = 73621779;
*/
public static final int MRDMA_VALUE = 73621779;
/**
*
*
*
* No type specified.
*
*
* UNSPECIFIED_NIC_TYPE = 67411801;
*/
public static final int UNSPECIFIED_NIC_TYPE_VALUE = 67411801;
/**
*
*
*
* VIRTIO
*
*
* VIRTIO_NET = 452123481;
*/
public static final int VIRTIO_NET_VALUE = 452123481;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static NicType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static NicType forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_NIC_TYPE;
case 68209305:
return GVNIC;
case 2242641:
return IDPF;
case 69927695:
return IRDMA;
case 73621779:
return MRDMA;
case 67411801:
return UNSPECIFIED_NIC_TYPE;
case 452123481:
return VIRTIO_NET;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public NicType findValueByNumber(int number) {
return NicType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.NetworkInterface.getDescriptor().getEnumTypes().get(1);
}
private static final NicType[] VALUES = values();
public static NicType valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private NicType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.NetworkInterface.NicType)
}
/**
*
*
*
* The stack type for this network interface. To assign only IPv4 addresses, use IPV4_ONLY. To assign both IPv4 and IPv6 addresses, use IPV4_IPV6. If not specified, IPV4_ONLY is used. This field can be both set at instance creation and update network interface operations.
*
*
* Protobuf enum {@code google.cloud.compute.v1.NetworkInterface.StackType}
*/
public enum StackType implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STACK_TYPE = 0;
*/
UNDEFINED_STACK_TYPE(0),
/**
*
*
*
* The network interface can have both IPv4 and IPv6 addresses.
*
*
* IPV4_IPV6 = 22197249;
*/
IPV4_IPV6(22197249),
/**
*
*
*
* The network interface will only be assigned IPv4 addresses.
*
*
* IPV4_ONLY = 22373798;
*/
IPV4_ONLY(22373798),
/**
*
*
*
* The network interface will only be assigned IPv6 addresses.
*
*
* IPV6_ONLY = 79632100;
*/
IPV6_ONLY(79632100),
/** UNSPECIFIED_STACK_TYPE = 298084569;
*/
UNSPECIFIED_STACK_TYPE(298084569),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STACK_TYPE = 0;
*/
public static final int UNDEFINED_STACK_TYPE_VALUE = 0;
/**
*
*
*
* The network interface can have both IPv4 and IPv6 addresses.
*
*
* IPV4_IPV6 = 22197249;
*/
public static final int IPV4_IPV6_VALUE = 22197249;
/**
*
*
*
* The network interface will only be assigned IPv4 addresses.
*
*
* IPV4_ONLY = 22373798;
*/
public static final int IPV4_ONLY_VALUE = 22373798;
/**
*
*
*
* The network interface will only be assigned IPv6 addresses.
*
*
* IPV6_ONLY = 79632100;
*/
public static final int IPV6_ONLY_VALUE = 79632100;
/** UNSPECIFIED_STACK_TYPE = 298084569;
*/
public static final int UNSPECIFIED_STACK_TYPE_VALUE = 298084569;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static StackType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static StackType forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_STACK_TYPE;
case 22197249:
return IPV4_IPV6;
case 22373798:
return IPV4_ONLY;
case 79632100:
return IPV6_ONLY;
case 298084569:
return UNSPECIFIED_STACK_TYPE;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public StackType findValueByNumber(int number) {
return StackType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.NetworkInterface.getDescriptor().getEnumTypes().get(2);
}
private static final StackType[] VALUES = values();
public static StackType valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private StackType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.NetworkInterface.StackType)
}
private int bitField0_;
public static final int ACCESS_CONFIGS_FIELD_NUMBER = 111058326;
@SuppressWarnings("serial")
private java.util.List accessConfigs_;
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
@java.lang.Override
public java.util.List getAccessConfigsList() {
return accessConfigs_;
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.compute.v1.AccessConfigOrBuilder>
getAccessConfigsOrBuilderList() {
return accessConfigs_;
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
@java.lang.Override
public int getAccessConfigsCount() {
return accessConfigs_.size();
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
@java.lang.Override
public com.google.cloud.compute.v1.AccessConfig getAccessConfigs(int index) {
return accessConfigs_.get(index);
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
@java.lang.Override
public com.google.cloud.compute.v1.AccessConfigOrBuilder getAccessConfigsOrBuilder(int index) {
return accessConfigs_.get(index);
}
public static final int ALIAS_IP_RANGES_FIELD_NUMBER = 165085631;
@SuppressWarnings("serial")
private java.util.List aliasIpRanges_;
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
@java.lang.Override
public java.util.List getAliasIpRangesList() {
return aliasIpRanges_;
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.compute.v1.AliasIpRangeOrBuilder>
getAliasIpRangesOrBuilderList() {
return aliasIpRanges_;
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
@java.lang.Override
public int getAliasIpRangesCount() {
return aliasIpRanges_.size();
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
@java.lang.Override
public com.google.cloud.compute.v1.AliasIpRange getAliasIpRanges(int index) {
return aliasIpRanges_.get(index);
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
@java.lang.Override
public com.google.cloud.compute.v1.AliasIpRangeOrBuilder getAliasIpRangesOrBuilder(int index) {
return aliasIpRanges_.get(index);
}
public static final int FINGERPRINT_FIELD_NUMBER = 234678500;
@SuppressWarnings("serial")
private volatile java.lang.Object fingerprint_ = "";
/**
*
*
*
* Fingerprint hash of contents stored in this network interface. This field will be ignored when inserting an Instance or adding a NetworkInterface. An up-to-date fingerprint must be provided in order to update the NetworkInterface. The request will fail with error 400 Bad Request if the fingerprint is not provided, or 412 Precondition Failed if the fingerprint is out of date.
*
*
* optional string fingerprint = 234678500;
*
* @return Whether the fingerprint field is set.
*/
@java.lang.Override
public boolean hasFingerprint() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Fingerprint hash of contents stored in this network interface. This field will be ignored when inserting an Instance or adding a NetworkInterface. An up-to-date fingerprint must be provided in order to update the NetworkInterface. The request will fail with error 400 Bad Request if the fingerprint is not provided, or 412 Precondition Failed if the fingerprint is out of date.
*
*
* optional string fingerprint = 234678500;
*
* @return The fingerprint.
*/
@java.lang.Override
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fingerprint_ = s;
return s;
}
}
/**
*
*
*
* Fingerprint hash of contents stored in this network interface. This field will be ignored when inserting an Instance or adding a NetworkInterface. An up-to-date fingerprint must be provided in order to update the NetworkInterface. The request will fail with error 400 Bad Request if the fingerprint is not provided, or 412 Precondition Failed if the fingerprint is out of date.
*
*
* optional string fingerprint = 234678500;
*
* @return The bytes for fingerprint.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INTERNAL_IPV6_PREFIX_LENGTH_FIELD_NUMBER = 203833757;
private int internalIpv6PrefixLength_ = 0;
/**
*
*
*
* The prefix length of the primary internal IPv6 range.
*
*
* optional int32 internal_ipv6_prefix_length = 203833757;
*
* @return Whether the internalIpv6PrefixLength field is set.
*/
@java.lang.Override
public boolean hasInternalIpv6PrefixLength() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The prefix length of the primary internal IPv6 range.
*
*
* optional int32 internal_ipv6_prefix_length = 203833757;
*
* @return The internalIpv6PrefixLength.
*/
@java.lang.Override
public int getInternalIpv6PrefixLength() {
return internalIpv6PrefixLength_;
}
public static final int IPV6_ACCESS_CONFIGS_FIELD_NUMBER = 483472110;
@SuppressWarnings("serial")
private java.util.List ipv6AccessConfigs_;
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
@java.lang.Override
public java.util.List getIpv6AccessConfigsList() {
return ipv6AccessConfigs_;
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.compute.v1.AccessConfigOrBuilder>
getIpv6AccessConfigsOrBuilderList() {
return ipv6AccessConfigs_;
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
@java.lang.Override
public int getIpv6AccessConfigsCount() {
return ipv6AccessConfigs_.size();
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
@java.lang.Override
public com.google.cloud.compute.v1.AccessConfig getIpv6AccessConfigs(int index) {
return ipv6AccessConfigs_.get(index);
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
@java.lang.Override
public com.google.cloud.compute.v1.AccessConfigOrBuilder getIpv6AccessConfigsOrBuilder(
int index) {
return ipv6AccessConfigs_.get(index);
}
public static final int IPV6_ACCESS_TYPE_FIELD_NUMBER = 504658653;
@SuppressWarnings("serial")
private volatile java.lang.Object ipv6AccessType_ = "";
/**
*
*
*
* [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed from the Internet. This field is always inherited from its subnetwork. Valid only if stackType is IPV4_IPV6.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return Whether the ipv6AccessType field is set.
*/
@java.lang.Override
public boolean hasIpv6AccessType() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed from the Internet. This field is always inherited from its subnetwork. Valid only if stackType is IPV4_IPV6.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return The ipv6AccessType.
*/
@java.lang.Override
public java.lang.String getIpv6AccessType() {
java.lang.Object ref = ipv6AccessType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ipv6AccessType_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed from the Internet. This field is always inherited from its subnetwork. Valid only if stackType is IPV4_IPV6.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return The bytes for ipv6AccessType.
*/
@java.lang.Override
public com.google.protobuf.ByteString getIpv6AccessTypeBytes() {
java.lang.Object ref = ipv6AccessType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
ipv6AccessType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IPV6_ADDRESS_FIELD_NUMBER = 341563804;
@SuppressWarnings("serial")
private volatile java.lang.Object ipv6Address_ = "";
/**
*
*
*
* An IPv6 internal network address for this network interface. To use a static internal IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
*
*
* optional string ipv6_address = 341563804;
*
* @return Whether the ipv6Address field is set.
*/
@java.lang.Override
public boolean hasIpv6Address() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* An IPv6 internal network address for this network interface. To use a static internal IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
*
*
* optional string ipv6_address = 341563804;
*
* @return The ipv6Address.
*/
@java.lang.Override
public java.lang.String getIpv6Address() {
java.lang.Object ref = ipv6Address_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ipv6Address_ = s;
return s;
}
}
/**
*
*
*
* An IPv6 internal network address for this network interface. To use a static internal IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
*
*
* optional string ipv6_address = 341563804;
*
* @return The bytes for ipv6Address.
*/
@java.lang.Override
public com.google.protobuf.ByteString getIpv6AddressBytes() {
java.lang.Object ref = ipv6Address_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
ipv6Address_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KIND_FIELD_NUMBER = 3292052;
@SuppressWarnings("serial")
private volatile java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
@java.lang.Override
public boolean hasKind() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
@java.lang.Override
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 3373707;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* [Output Only] The name of the network interface, which is generated by the server. For a VM, the network interface uses the nicN naming format. Where N is a value between 0 and 7. The default interface value is nic0.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* [Output Only] The name of the network interface, which is generated by the server. For a VM, the network interface uses the nicN naming format. Where N is a value between 0 and 7. The default interface value is nic0.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The name of the network interface, which is generated by the server. For a VM, the network interface uses the nicN naming format. Where N is a value between 0 and 7. The default interface value is nic0.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NETWORK_FIELD_NUMBER = 232872494;
@SuppressWarnings("serial")
private volatile java.lang.Object network_ = "";
/**
*
*
*
* URL of the VPC network resource for this instance. When creating an instance, if neither the network nor the subnetwork is specified, the default network global/networks/default is used. If the selected project doesn't have the default network, you must specify a network or subnet. If the network is not specified but the subnetwork is specified, the network is inferred. If you specify this property, you can specify the network as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/global/networks/ network - projects/project/global/networks/network - global/networks/default
*
*
* optional string network = 232872494;
*
* @return Whether the network field is set.
*/
@java.lang.Override
public boolean hasNetwork() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* URL of the VPC network resource for this instance. When creating an instance, if neither the network nor the subnetwork is specified, the default network global/networks/default is used. If the selected project doesn't have the default network, you must specify a network or subnet. If the network is not specified but the subnetwork is specified, the network is inferred. If you specify this property, you can specify the network as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/global/networks/ network - projects/project/global/networks/network - global/networks/default
*
*
* optional string network = 232872494;
*
* @return The network.
*/
@java.lang.Override
public java.lang.String getNetwork() {
java.lang.Object ref = network_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
network_ = s;
return s;
}
}
/**
*
*
*
* URL of the VPC network resource for this instance. When creating an instance, if neither the network nor the subnetwork is specified, the default network global/networks/default is used. If the selected project doesn't have the default network, you must specify a network or subnet. If the network is not specified but the subnetwork is specified, the network is inferred. If you specify this property, you can specify the network as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/global/networks/ network - projects/project/global/networks/network - global/networks/default
*
*
* optional string network = 232872494;
*
* @return The bytes for network.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNetworkBytes() {
java.lang.Object ref = network_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
network_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NETWORK_ATTACHMENT_FIELD_NUMBER = 224644052;
@SuppressWarnings("serial")
private volatile java.lang.Object networkAttachment_ = "";
/**
*
*
*
* The URL of the network attachment that this interface should connect to in the following format: projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
*
*
* optional string network_attachment = 224644052;
*
* @return Whether the networkAttachment field is set.
*/
@java.lang.Override
public boolean hasNetworkAttachment() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The URL of the network attachment that this interface should connect to in the following format: projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
*
*
* optional string network_attachment = 224644052;
*
* @return The networkAttachment.
*/
@java.lang.Override
public java.lang.String getNetworkAttachment() {
java.lang.Object ref = networkAttachment_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
networkAttachment_ = s;
return s;
}
}
/**
*
*
*
* The URL of the network attachment that this interface should connect to in the following format: projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
*
*
* optional string network_attachment = 224644052;
*
* @return The bytes for networkAttachment.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNetworkAttachmentBytes() {
java.lang.Object ref = networkAttachment_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
networkAttachment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NETWORK_I_P_FIELD_NUMBER = 207181961;
@SuppressWarnings("serial")
private volatile java.lang.Object networkIP_ = "";
/**
*
*
*
* An IPv4 internal IP address to assign to the instance for this network interface. If not specified by the user, an unused internal IP is assigned by the system.
*
*
* optional string network_i_p = 207181961;
*
* @return Whether the networkIP field is set.
*/
@java.lang.Override
public boolean hasNetworkIP() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* An IPv4 internal IP address to assign to the instance for this network interface. If not specified by the user, an unused internal IP is assigned by the system.
*
*
* optional string network_i_p = 207181961;
*
* @return The networkIP.
*/
@java.lang.Override
public java.lang.String getNetworkIP() {
java.lang.Object ref = networkIP_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
networkIP_ = s;
return s;
}
}
/**
*
*
*
* An IPv4 internal IP address to assign to the instance for this network interface. If not specified by the user, an unused internal IP is assigned by the system.
*
*
* optional string network_i_p = 207181961;
*
* @return The bytes for networkIP.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNetworkIPBytes() {
java.lang.Object ref = networkIP_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
networkIP_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NIC_TYPE_FIELD_NUMBER = 59810577;
@SuppressWarnings("serial")
private volatile java.lang.Object nicType_ = "";
/**
*
*
*
* The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* Check the NicType enum for the list of possible values.
*
*
* optional string nic_type = 59810577;
*
* @return Whether the nicType field is set.
*/
@java.lang.Override
public boolean hasNicType() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* Check the NicType enum for the list of possible values.
*
*
* optional string nic_type = 59810577;
*
* @return The nicType.
*/
@java.lang.Override
public java.lang.String getNicType() {
java.lang.Object ref = nicType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nicType_ = s;
return s;
}
}
/**
*
*
*
* The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* Check the NicType enum for the list of possible values.
*
*
* optional string nic_type = 59810577;
*
* @return The bytes for nicType.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNicTypeBytes() {
java.lang.Object ref = nicType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
nicType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int QUEUE_COUNT_FIELD_NUMBER = 503708769;
private int queueCount_ = 0;
/**
*
*
*
* The networking queue count that's specified by users for the network interface. Both Rx and Tx queues will be set to this number. It'll be empty if not specified by the users.
*
*
* optional int32 queue_count = 503708769;
*
* @return Whether the queueCount field is set.
*/
@java.lang.Override
public boolean hasQueueCount() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* The networking queue count that's specified by users for the network interface. Both Rx and Tx queues will be set to this number. It'll be empty if not specified by the users.
*
*
* optional int32 queue_count = 503708769;
*
* @return The queueCount.
*/
@java.lang.Override
public int getQueueCount() {
return queueCount_;
}
public static final int STACK_TYPE_FIELD_NUMBER = 425908881;
@SuppressWarnings("serial")
private volatile java.lang.Object stackType_ = "";
/**
*
*
*
* The stack type for this network interface. To assign only IPv4 addresses, use IPV4_ONLY. To assign both IPv4 and IPv6 addresses, use IPV4_IPV6. If not specified, IPV4_ONLY is used. This field can be both set at instance creation and update network interface operations.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return Whether the stackType field is set.
*/
@java.lang.Override
public boolean hasStackType() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* The stack type for this network interface. To assign only IPv4 addresses, use IPV4_ONLY. To assign both IPv4 and IPv6 addresses, use IPV4_IPV6. If not specified, IPV4_ONLY is used. This field can be both set at instance creation and update network interface operations.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The stackType.
*/
@java.lang.Override
public java.lang.String getStackType() {
java.lang.Object ref = stackType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stackType_ = s;
return s;
}
}
/**
*
*
*
* The stack type for this network interface. To assign only IPv4 addresses, use IPV4_ONLY. To assign both IPv4 and IPv6 addresses, use IPV4_IPV6. If not specified, IPV4_ONLY is used. This field can be both set at instance creation and update network interface operations.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The bytes for stackType.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStackTypeBytes() {
java.lang.Object ref = stackType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
stackType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBNETWORK_FIELD_NUMBER = 307827694;
@SuppressWarnings("serial")
private volatile java.lang.Object subnetwork_ = "";
/**
*
*
*
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy mode, do not specify this field. If the network is in auto subnet mode, specifying the subnetwork is optional. If the network is in custom subnet mode, specifying the subnetwork is required. If you specify this field, you can specify the subnetwork as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/regions/region /subnetworks/subnetwork - regions/region/subnetworks/subnetwork
*
*
* optional string subnetwork = 307827694;
*
* @return Whether the subnetwork field is set.
*/
@java.lang.Override
public boolean hasSubnetwork() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy mode, do not specify this field. If the network is in auto subnet mode, specifying the subnetwork is optional. If the network is in custom subnet mode, specifying the subnetwork is required. If you specify this field, you can specify the subnetwork as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/regions/region /subnetworks/subnetwork - regions/region/subnetworks/subnetwork
*
*
* optional string subnetwork = 307827694;
*
* @return The subnetwork.
*/
@java.lang.Override
public java.lang.String getSubnetwork() {
java.lang.Object ref = subnetwork_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subnetwork_ = s;
return s;
}
}
/**
*
*
*
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy mode, do not specify this field. If the network is in auto subnet mode, specifying the subnetwork is optional. If the network is in custom subnet mode, specifying the subnetwork is required. If you specify this field, you can specify the subnetwork as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/regions/region /subnetworks/subnetwork - regions/region/subnetworks/subnetwork
*
*
* optional string subnetwork = 307827694;
*
* @return The bytes for subnetwork.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSubnetworkBytes() {
java.lang.Object ref = subnetwork_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
subnetwork_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3292052, kind_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3373707, name_);
}
if (((bitField0_ & 0x00000200) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 59810577, nicType_);
}
for (int i = 0; i < accessConfigs_.size(); i++) {
output.writeMessage(111058326, accessConfigs_.get(i));
}
for (int i = 0; i < aliasIpRanges_.size(); i++) {
output.writeMessage(165085631, aliasIpRanges_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(203833757, internalIpv6PrefixLength_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 207181961, networkIP_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 224644052, networkAttachment_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 232872494, network_);
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 234678500, fingerprint_);
}
if (((bitField0_ & 0x00001000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 307827694, subnetwork_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 341563804, ipv6Address_);
}
if (((bitField0_ & 0x00000800) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 425908881, stackType_);
}
for (int i = 0; i < ipv6AccessConfigs_.size(); i++) {
output.writeMessage(483472110, ipv6AccessConfigs_.get(i));
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeInt32(503708769, queueCount_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 504658653, ipv6AccessType_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3292052, kind_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3373707, name_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(59810577, nicType_);
}
for (int i = 0; i < accessConfigs_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
111058326, accessConfigs_.get(i));
}
for (int i = 0; i < aliasIpRanges_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
165085631, aliasIpRanges_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeInt32Size(
203833757, internalIpv6PrefixLength_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(207181961, networkIP_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(224644052, networkAttachment_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(232872494, network_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(234678500, fingerprint_);
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(307827694, subnetwork_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(341563804, ipv6Address_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(425908881, stackType_);
}
for (int i = 0; i < ipv6AccessConfigs_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
483472110, ipv6AccessConfigs_.get(i));
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(503708769, queueCount_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(504658653, ipv6AccessType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.NetworkInterface)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.NetworkInterface other =
(com.google.cloud.compute.v1.NetworkInterface) obj;
if (!getAccessConfigsList().equals(other.getAccessConfigsList())) return false;
if (!getAliasIpRangesList().equals(other.getAliasIpRangesList())) return false;
if (hasFingerprint() != other.hasFingerprint()) return false;
if (hasFingerprint()) {
if (!getFingerprint().equals(other.getFingerprint())) return false;
}
if (hasInternalIpv6PrefixLength() != other.hasInternalIpv6PrefixLength()) return false;
if (hasInternalIpv6PrefixLength()) {
if (getInternalIpv6PrefixLength() != other.getInternalIpv6PrefixLength()) return false;
}
if (!getIpv6AccessConfigsList().equals(other.getIpv6AccessConfigsList())) return false;
if (hasIpv6AccessType() != other.hasIpv6AccessType()) return false;
if (hasIpv6AccessType()) {
if (!getIpv6AccessType().equals(other.getIpv6AccessType())) return false;
}
if (hasIpv6Address() != other.hasIpv6Address()) return false;
if (hasIpv6Address()) {
if (!getIpv6Address().equals(other.getIpv6Address())) return false;
}
if (hasKind() != other.hasKind()) return false;
if (hasKind()) {
if (!getKind().equals(other.getKind())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName().equals(other.getName())) return false;
}
if (hasNetwork() != other.hasNetwork()) return false;
if (hasNetwork()) {
if (!getNetwork().equals(other.getNetwork())) return false;
}
if (hasNetworkAttachment() != other.hasNetworkAttachment()) return false;
if (hasNetworkAttachment()) {
if (!getNetworkAttachment().equals(other.getNetworkAttachment())) return false;
}
if (hasNetworkIP() != other.hasNetworkIP()) return false;
if (hasNetworkIP()) {
if (!getNetworkIP().equals(other.getNetworkIP())) return false;
}
if (hasNicType() != other.hasNicType()) return false;
if (hasNicType()) {
if (!getNicType().equals(other.getNicType())) return false;
}
if (hasQueueCount() != other.hasQueueCount()) return false;
if (hasQueueCount()) {
if (getQueueCount() != other.getQueueCount()) return false;
}
if (hasStackType() != other.hasStackType()) return false;
if (hasStackType()) {
if (!getStackType().equals(other.getStackType())) return false;
}
if (hasSubnetwork() != other.hasSubnetwork()) return false;
if (hasSubnetwork()) {
if (!getSubnetwork().equals(other.getSubnetwork())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getAccessConfigsCount() > 0) {
hash = (37 * hash) + ACCESS_CONFIGS_FIELD_NUMBER;
hash = (53 * hash) + getAccessConfigsList().hashCode();
}
if (getAliasIpRangesCount() > 0) {
hash = (37 * hash) + ALIAS_IP_RANGES_FIELD_NUMBER;
hash = (53 * hash) + getAliasIpRangesList().hashCode();
}
if (hasFingerprint()) {
hash = (37 * hash) + FINGERPRINT_FIELD_NUMBER;
hash = (53 * hash) + getFingerprint().hashCode();
}
if (hasInternalIpv6PrefixLength()) {
hash = (37 * hash) + INTERNAL_IPV6_PREFIX_LENGTH_FIELD_NUMBER;
hash = (53 * hash) + getInternalIpv6PrefixLength();
}
if (getIpv6AccessConfigsCount() > 0) {
hash = (37 * hash) + IPV6_ACCESS_CONFIGS_FIELD_NUMBER;
hash = (53 * hash) + getIpv6AccessConfigsList().hashCode();
}
if (hasIpv6AccessType()) {
hash = (37 * hash) + IPV6_ACCESS_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getIpv6AccessType().hashCode();
}
if (hasIpv6Address()) {
hash = (37 * hash) + IPV6_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getIpv6Address().hashCode();
}
if (hasKind()) {
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + getKind().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasNetwork()) {
hash = (37 * hash) + NETWORK_FIELD_NUMBER;
hash = (53 * hash) + getNetwork().hashCode();
}
if (hasNetworkAttachment()) {
hash = (37 * hash) + NETWORK_ATTACHMENT_FIELD_NUMBER;
hash = (53 * hash) + getNetworkAttachment().hashCode();
}
if (hasNetworkIP()) {
hash = (37 * hash) + NETWORK_I_P_FIELD_NUMBER;
hash = (53 * hash) + getNetworkIP().hashCode();
}
if (hasNicType()) {
hash = (37 * hash) + NIC_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getNicType().hashCode();
}
if (hasQueueCount()) {
hash = (37 * hash) + QUEUE_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getQueueCount();
}
if (hasStackType()) {
hash = (37 * hash) + STACK_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getStackType().hashCode();
}
if (hasSubnetwork()) {
hash = (37 * hash) + SUBNETWORK_FIELD_NUMBER;
hash = (53 * hash) + getSubnetwork().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.NetworkInterface parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.NetworkInterface parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkInterface parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.NetworkInterface parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkInterface parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.NetworkInterface parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkInterface parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.NetworkInterface parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkInterface parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.NetworkInterface parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkInterface parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.NetworkInterface parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.NetworkInterface prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* A network interface resource attached to an instance.
*
*
* Protobuf type {@code google.cloud.compute.v1.NetworkInterface}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.NetworkInterface)
com.google.cloud.compute.v1.NetworkInterfaceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkInterface_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkInterface_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.NetworkInterface.class,
com.google.cloud.compute.v1.NetworkInterface.Builder.class);
}
// Construct using com.google.cloud.compute.v1.NetworkInterface.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (accessConfigsBuilder_ == null) {
accessConfigs_ = java.util.Collections.emptyList();
} else {
accessConfigs_ = null;
accessConfigsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (aliasIpRangesBuilder_ == null) {
aliasIpRanges_ = java.util.Collections.emptyList();
} else {
aliasIpRanges_ = null;
aliasIpRangesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
fingerprint_ = "";
internalIpv6PrefixLength_ = 0;
if (ipv6AccessConfigsBuilder_ == null) {
ipv6AccessConfigs_ = java.util.Collections.emptyList();
} else {
ipv6AccessConfigs_ = null;
ipv6AccessConfigsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
ipv6AccessType_ = "";
ipv6Address_ = "";
kind_ = "";
name_ = "";
network_ = "";
networkAttachment_ = "";
networkIP_ = "";
nicType_ = "";
queueCount_ = 0;
stackType_ = "";
subnetwork_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkInterface_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkInterface getDefaultInstanceForType() {
return com.google.cloud.compute.v1.NetworkInterface.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkInterface build() {
com.google.cloud.compute.v1.NetworkInterface result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkInterface buildPartial() {
com.google.cloud.compute.v1.NetworkInterface result =
new com.google.cloud.compute.v1.NetworkInterface(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.cloud.compute.v1.NetworkInterface result) {
if (accessConfigsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
accessConfigs_ = java.util.Collections.unmodifiableList(accessConfigs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.accessConfigs_ = accessConfigs_;
} else {
result.accessConfigs_ = accessConfigsBuilder_.build();
}
if (aliasIpRangesBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
aliasIpRanges_ = java.util.Collections.unmodifiableList(aliasIpRanges_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.aliasIpRanges_ = aliasIpRanges_;
} else {
result.aliasIpRanges_ = aliasIpRangesBuilder_.build();
}
if (ipv6AccessConfigsBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
ipv6AccessConfigs_ = java.util.Collections.unmodifiableList(ipv6AccessConfigs_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.ipv6AccessConfigs_ = ipv6AccessConfigs_;
} else {
result.ipv6AccessConfigs_ = ipv6AccessConfigsBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.compute.v1.NetworkInterface result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.fingerprint_ = fingerprint_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.internalIpv6PrefixLength_ = internalIpv6PrefixLength_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.ipv6AccessType_ = ipv6AccessType_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.ipv6Address_ = ipv6Address_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.kind_ = kind_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.network_ = network_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.networkAttachment_ = networkAttachment_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.networkIP_ = networkIP_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.nicType_ = nicType_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.queueCount_ = queueCount_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.stackType_ = stackType_;
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.subnetwork_ = subnetwork_;
to_bitField0_ |= 0x00001000;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.NetworkInterface) {
return mergeFrom((com.google.cloud.compute.v1.NetworkInterface) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.NetworkInterface other) {
if (other == com.google.cloud.compute.v1.NetworkInterface.getDefaultInstance()) return this;
if (accessConfigsBuilder_ == null) {
if (!other.accessConfigs_.isEmpty()) {
if (accessConfigs_.isEmpty()) {
accessConfigs_ = other.accessConfigs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureAccessConfigsIsMutable();
accessConfigs_.addAll(other.accessConfigs_);
}
onChanged();
}
} else {
if (!other.accessConfigs_.isEmpty()) {
if (accessConfigsBuilder_.isEmpty()) {
accessConfigsBuilder_.dispose();
accessConfigsBuilder_ = null;
accessConfigs_ = other.accessConfigs_;
bitField0_ = (bitField0_ & ~0x00000001);
accessConfigsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getAccessConfigsFieldBuilder()
: null;
} else {
accessConfigsBuilder_.addAllMessages(other.accessConfigs_);
}
}
}
if (aliasIpRangesBuilder_ == null) {
if (!other.aliasIpRanges_.isEmpty()) {
if (aliasIpRanges_.isEmpty()) {
aliasIpRanges_ = other.aliasIpRanges_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureAliasIpRangesIsMutable();
aliasIpRanges_.addAll(other.aliasIpRanges_);
}
onChanged();
}
} else {
if (!other.aliasIpRanges_.isEmpty()) {
if (aliasIpRangesBuilder_.isEmpty()) {
aliasIpRangesBuilder_.dispose();
aliasIpRangesBuilder_ = null;
aliasIpRanges_ = other.aliasIpRanges_;
bitField0_ = (bitField0_ & ~0x00000002);
aliasIpRangesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getAliasIpRangesFieldBuilder()
: null;
} else {
aliasIpRangesBuilder_.addAllMessages(other.aliasIpRanges_);
}
}
}
if (other.hasFingerprint()) {
fingerprint_ = other.fingerprint_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasInternalIpv6PrefixLength()) {
setInternalIpv6PrefixLength(other.getInternalIpv6PrefixLength());
}
if (ipv6AccessConfigsBuilder_ == null) {
if (!other.ipv6AccessConfigs_.isEmpty()) {
if (ipv6AccessConfigs_.isEmpty()) {
ipv6AccessConfigs_ = other.ipv6AccessConfigs_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureIpv6AccessConfigsIsMutable();
ipv6AccessConfigs_.addAll(other.ipv6AccessConfigs_);
}
onChanged();
}
} else {
if (!other.ipv6AccessConfigs_.isEmpty()) {
if (ipv6AccessConfigsBuilder_.isEmpty()) {
ipv6AccessConfigsBuilder_.dispose();
ipv6AccessConfigsBuilder_ = null;
ipv6AccessConfigs_ = other.ipv6AccessConfigs_;
bitField0_ = (bitField0_ & ~0x00000010);
ipv6AccessConfigsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getIpv6AccessConfigsFieldBuilder()
: null;
} else {
ipv6AccessConfigsBuilder_.addAllMessages(other.ipv6AccessConfigs_);
}
}
}
if (other.hasIpv6AccessType()) {
ipv6AccessType_ = other.ipv6AccessType_;
bitField0_ |= 0x00000020;
onChanged();
}
if (other.hasIpv6Address()) {
ipv6Address_ = other.ipv6Address_;
bitField0_ |= 0x00000040;
onChanged();
}
if (other.hasKind()) {
kind_ = other.kind_;
bitField0_ |= 0x00000080;
onChanged();
}
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000100;
onChanged();
}
if (other.hasNetwork()) {
network_ = other.network_;
bitField0_ |= 0x00000200;
onChanged();
}
if (other.hasNetworkAttachment()) {
networkAttachment_ = other.networkAttachment_;
bitField0_ |= 0x00000400;
onChanged();
}
if (other.hasNetworkIP()) {
networkIP_ = other.networkIP_;
bitField0_ |= 0x00000800;
onChanged();
}
if (other.hasNicType()) {
nicType_ = other.nicType_;
bitField0_ |= 0x00001000;
onChanged();
}
if (other.hasQueueCount()) {
setQueueCount(other.getQueueCount());
}
if (other.hasStackType()) {
stackType_ = other.stackType_;
bitField0_ |= 0x00004000;
onChanged();
}
if (other.hasSubnetwork()) {
subnetwork_ = other.subnetwork_;
bitField0_ |= 0x00008000;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 26336418:
{
kind_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 26336418
case 26989658:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case 26989658
case 478484618:
{
nicType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00001000;
break;
} // case 478484618
case 888466610:
{
com.google.cloud.compute.v1.AccessConfig m =
input.readMessage(
com.google.cloud.compute.v1.AccessConfig.parser(), extensionRegistry);
if (accessConfigsBuilder_ == null) {
ensureAccessConfigsIsMutable();
accessConfigs_.add(m);
} else {
accessConfigsBuilder_.addMessage(m);
}
break;
} // case 888466610
case 1320685050:
{
com.google.cloud.compute.v1.AliasIpRange m =
input.readMessage(
com.google.cloud.compute.v1.AliasIpRange.parser(), extensionRegistry);
if (aliasIpRangesBuilder_ == null) {
ensureAliasIpRangesIsMutable();
aliasIpRanges_.add(m);
} else {
aliasIpRangesBuilder_.addMessage(m);
}
break;
} // case 1320685050
case 1630670056:
{
internalIpv6PrefixLength_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 1630670056
case 1657455690:
{
networkIP_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000800;
break;
} // case 1657455690
case 1797152418:
{
networkAttachment_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000400;
break;
} // case 1797152418
case 1862979954:
{
network_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case 1862979954
case 1877428002:
{
fingerprint_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 1877428002
case -1832345742:
{
subnetwork_ = input.readStringRequireUtf8();
bitField0_ |= 0x00008000;
break;
} // case -1832345742
case -1562456862:
{
ipv6Address_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case -1562456862
case -887696246:
{
stackType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00004000;
break;
} // case -887696246
case -427190414:
{
com.google.cloud.compute.v1.AccessConfig m =
input.readMessage(
com.google.cloud.compute.v1.AccessConfig.parser(), extensionRegistry);
if (ipv6AccessConfigsBuilder_ == null) {
ensureIpv6AccessConfigsIsMutable();
ipv6AccessConfigs_.add(m);
} else {
ipv6AccessConfigsBuilder_.addMessage(m);
}
break;
} // case -427190414
case -265297144:
{
queueCount_ = input.readInt32();
bitField0_ |= 0x00002000;
break;
} // case -265297144
case -257698070:
{
ipv6AccessType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case -257698070
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List accessConfigs_ =
java.util.Collections.emptyList();
private void ensureAccessConfigsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
accessConfigs_ =
new java.util.ArrayList(accessConfigs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AccessConfig,
com.google.cloud.compute.v1.AccessConfig.Builder,
com.google.cloud.compute.v1.AccessConfigOrBuilder>
accessConfigsBuilder_;
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public java.util.List getAccessConfigsList() {
if (accessConfigsBuilder_ == null) {
return java.util.Collections.unmodifiableList(accessConfigs_);
} else {
return accessConfigsBuilder_.getMessageList();
}
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public int getAccessConfigsCount() {
if (accessConfigsBuilder_ == null) {
return accessConfigs_.size();
} else {
return accessConfigsBuilder_.getCount();
}
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public com.google.cloud.compute.v1.AccessConfig getAccessConfigs(int index) {
if (accessConfigsBuilder_ == null) {
return accessConfigs_.get(index);
} else {
return accessConfigsBuilder_.getMessage(index);
}
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public Builder setAccessConfigs(int index, com.google.cloud.compute.v1.AccessConfig value) {
if (accessConfigsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAccessConfigsIsMutable();
accessConfigs_.set(index, value);
onChanged();
} else {
accessConfigsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public Builder setAccessConfigs(
int index, com.google.cloud.compute.v1.AccessConfig.Builder builderForValue) {
if (accessConfigsBuilder_ == null) {
ensureAccessConfigsIsMutable();
accessConfigs_.set(index, builderForValue.build());
onChanged();
} else {
accessConfigsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public Builder addAccessConfigs(com.google.cloud.compute.v1.AccessConfig value) {
if (accessConfigsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAccessConfigsIsMutable();
accessConfigs_.add(value);
onChanged();
} else {
accessConfigsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public Builder addAccessConfigs(int index, com.google.cloud.compute.v1.AccessConfig value) {
if (accessConfigsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAccessConfigsIsMutable();
accessConfigs_.add(index, value);
onChanged();
} else {
accessConfigsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public Builder addAccessConfigs(
com.google.cloud.compute.v1.AccessConfig.Builder builderForValue) {
if (accessConfigsBuilder_ == null) {
ensureAccessConfigsIsMutable();
accessConfigs_.add(builderForValue.build());
onChanged();
} else {
accessConfigsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public Builder addAccessConfigs(
int index, com.google.cloud.compute.v1.AccessConfig.Builder builderForValue) {
if (accessConfigsBuilder_ == null) {
ensureAccessConfigsIsMutable();
accessConfigs_.add(index, builderForValue.build());
onChanged();
} else {
accessConfigsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public Builder addAllAccessConfigs(
java.lang.Iterable extends com.google.cloud.compute.v1.AccessConfig> values) {
if (accessConfigsBuilder_ == null) {
ensureAccessConfigsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, accessConfigs_);
onChanged();
} else {
accessConfigsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public Builder clearAccessConfigs() {
if (accessConfigsBuilder_ == null) {
accessConfigs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
accessConfigsBuilder_.clear();
}
return this;
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public Builder removeAccessConfigs(int index) {
if (accessConfigsBuilder_ == null) {
ensureAccessConfigsIsMutable();
accessConfigs_.remove(index);
onChanged();
} else {
accessConfigsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public com.google.cloud.compute.v1.AccessConfig.Builder getAccessConfigsBuilder(int index) {
return getAccessConfigsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public com.google.cloud.compute.v1.AccessConfigOrBuilder getAccessConfigsOrBuilder(int index) {
if (accessConfigsBuilder_ == null) {
return accessConfigs_.get(index);
} else {
return accessConfigsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public java.util.List extends com.google.cloud.compute.v1.AccessConfigOrBuilder>
getAccessConfigsOrBuilderList() {
if (accessConfigsBuilder_ != null) {
return accessConfigsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(accessConfigs_);
}
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public com.google.cloud.compute.v1.AccessConfig.Builder addAccessConfigsBuilder() {
return getAccessConfigsFieldBuilder()
.addBuilder(com.google.cloud.compute.v1.AccessConfig.getDefaultInstance());
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public com.google.cloud.compute.v1.AccessConfig.Builder addAccessConfigsBuilder(int index) {
return getAccessConfigsFieldBuilder()
.addBuilder(index, com.google.cloud.compute.v1.AccessConfig.getDefaultInstance());
}
/**
*
*
*
* An array of configurations for this interface. Currently, only one access config, ONE_TO_ONE_NAT, is supported. If there are no accessConfigs specified, then this instance will have no external internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig access_configs = 111058326;
*/
public java.util.List
getAccessConfigsBuilderList() {
return getAccessConfigsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AccessConfig,
com.google.cloud.compute.v1.AccessConfig.Builder,
com.google.cloud.compute.v1.AccessConfigOrBuilder>
getAccessConfigsFieldBuilder() {
if (accessConfigsBuilder_ == null) {
accessConfigsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AccessConfig,
com.google.cloud.compute.v1.AccessConfig.Builder,
com.google.cloud.compute.v1.AccessConfigOrBuilder>(
accessConfigs_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
accessConfigs_ = null;
}
return accessConfigsBuilder_;
}
private java.util.List aliasIpRanges_ =
java.util.Collections.emptyList();
private void ensureAliasIpRangesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
aliasIpRanges_ =
new java.util.ArrayList(aliasIpRanges_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AliasIpRange,
com.google.cloud.compute.v1.AliasIpRange.Builder,
com.google.cloud.compute.v1.AliasIpRangeOrBuilder>
aliasIpRangesBuilder_;
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public java.util.List getAliasIpRangesList() {
if (aliasIpRangesBuilder_ == null) {
return java.util.Collections.unmodifiableList(aliasIpRanges_);
} else {
return aliasIpRangesBuilder_.getMessageList();
}
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public int getAliasIpRangesCount() {
if (aliasIpRangesBuilder_ == null) {
return aliasIpRanges_.size();
} else {
return aliasIpRangesBuilder_.getCount();
}
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public com.google.cloud.compute.v1.AliasIpRange getAliasIpRanges(int index) {
if (aliasIpRangesBuilder_ == null) {
return aliasIpRanges_.get(index);
} else {
return aliasIpRangesBuilder_.getMessage(index);
}
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public Builder setAliasIpRanges(int index, com.google.cloud.compute.v1.AliasIpRange value) {
if (aliasIpRangesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAliasIpRangesIsMutable();
aliasIpRanges_.set(index, value);
onChanged();
} else {
aliasIpRangesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public Builder setAliasIpRanges(
int index, com.google.cloud.compute.v1.AliasIpRange.Builder builderForValue) {
if (aliasIpRangesBuilder_ == null) {
ensureAliasIpRangesIsMutable();
aliasIpRanges_.set(index, builderForValue.build());
onChanged();
} else {
aliasIpRangesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public Builder addAliasIpRanges(com.google.cloud.compute.v1.AliasIpRange value) {
if (aliasIpRangesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAliasIpRangesIsMutable();
aliasIpRanges_.add(value);
onChanged();
} else {
aliasIpRangesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public Builder addAliasIpRanges(int index, com.google.cloud.compute.v1.AliasIpRange value) {
if (aliasIpRangesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAliasIpRangesIsMutable();
aliasIpRanges_.add(index, value);
onChanged();
} else {
aliasIpRangesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public Builder addAliasIpRanges(
com.google.cloud.compute.v1.AliasIpRange.Builder builderForValue) {
if (aliasIpRangesBuilder_ == null) {
ensureAliasIpRangesIsMutable();
aliasIpRanges_.add(builderForValue.build());
onChanged();
} else {
aliasIpRangesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public Builder addAliasIpRanges(
int index, com.google.cloud.compute.v1.AliasIpRange.Builder builderForValue) {
if (aliasIpRangesBuilder_ == null) {
ensureAliasIpRangesIsMutable();
aliasIpRanges_.add(index, builderForValue.build());
onChanged();
} else {
aliasIpRangesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public Builder addAllAliasIpRanges(
java.lang.Iterable extends com.google.cloud.compute.v1.AliasIpRange> values) {
if (aliasIpRangesBuilder_ == null) {
ensureAliasIpRangesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, aliasIpRanges_);
onChanged();
} else {
aliasIpRangesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public Builder clearAliasIpRanges() {
if (aliasIpRangesBuilder_ == null) {
aliasIpRanges_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
aliasIpRangesBuilder_.clear();
}
return this;
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public Builder removeAliasIpRanges(int index) {
if (aliasIpRangesBuilder_ == null) {
ensureAliasIpRangesIsMutable();
aliasIpRanges_.remove(index);
onChanged();
} else {
aliasIpRangesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public com.google.cloud.compute.v1.AliasIpRange.Builder getAliasIpRangesBuilder(int index) {
return getAliasIpRangesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public com.google.cloud.compute.v1.AliasIpRangeOrBuilder getAliasIpRangesOrBuilder(int index) {
if (aliasIpRangesBuilder_ == null) {
return aliasIpRanges_.get(index);
} else {
return aliasIpRangesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public java.util.List extends com.google.cloud.compute.v1.AliasIpRangeOrBuilder>
getAliasIpRangesOrBuilderList() {
if (aliasIpRangesBuilder_ != null) {
return aliasIpRangesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(aliasIpRanges_);
}
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public com.google.cloud.compute.v1.AliasIpRange.Builder addAliasIpRangesBuilder() {
return getAliasIpRangesFieldBuilder()
.addBuilder(com.google.cloud.compute.v1.AliasIpRange.getDefaultInstance());
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public com.google.cloud.compute.v1.AliasIpRange.Builder addAliasIpRangesBuilder(int index) {
return getAliasIpRangesFieldBuilder()
.addBuilder(index, com.google.cloud.compute.v1.AliasIpRange.getDefaultInstance());
}
/**
*
*
*
* An array of alias IP ranges for this network interface. You can only specify this field for network interfaces in VPC networks.
*
*
* repeated .google.cloud.compute.v1.AliasIpRange alias_ip_ranges = 165085631;
*/
public java.util.List
getAliasIpRangesBuilderList() {
return getAliasIpRangesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AliasIpRange,
com.google.cloud.compute.v1.AliasIpRange.Builder,
com.google.cloud.compute.v1.AliasIpRangeOrBuilder>
getAliasIpRangesFieldBuilder() {
if (aliasIpRangesBuilder_ == null) {
aliasIpRangesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AliasIpRange,
com.google.cloud.compute.v1.AliasIpRange.Builder,
com.google.cloud.compute.v1.AliasIpRangeOrBuilder>(
aliasIpRanges_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
aliasIpRanges_ = null;
}
return aliasIpRangesBuilder_;
}
private java.lang.Object fingerprint_ = "";
/**
*
*
*
* Fingerprint hash of contents stored in this network interface. This field will be ignored when inserting an Instance or adding a NetworkInterface. An up-to-date fingerprint must be provided in order to update the NetworkInterface. The request will fail with error 400 Bad Request if the fingerprint is not provided, or 412 Precondition Failed if the fingerprint is out of date.
*
*
* optional string fingerprint = 234678500;
*
* @return Whether the fingerprint field is set.
*/
public boolean hasFingerprint() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Fingerprint hash of contents stored in this network interface. This field will be ignored when inserting an Instance or adding a NetworkInterface. An up-to-date fingerprint must be provided in order to update the NetworkInterface. The request will fail with error 400 Bad Request if the fingerprint is not provided, or 412 Precondition Failed if the fingerprint is out of date.
*
*
* optional string fingerprint = 234678500;
*
* @return The fingerprint.
*/
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fingerprint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Fingerprint hash of contents stored in this network interface. This field will be ignored when inserting an Instance or adding a NetworkInterface. An up-to-date fingerprint must be provided in order to update the NetworkInterface. The request will fail with error 400 Bad Request if the fingerprint is not provided, or 412 Precondition Failed if the fingerprint is out of date.
*
*
* optional string fingerprint = 234678500;
*
* @return The bytes for fingerprint.
*/
public com.google.protobuf.ByteString getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Fingerprint hash of contents stored in this network interface. This field will be ignored when inserting an Instance or adding a NetworkInterface. An up-to-date fingerprint must be provided in order to update the NetworkInterface. The request will fail with error 400 Bad Request if the fingerprint is not provided, or 412 Precondition Failed if the fingerprint is out of date.
*
*
* optional string fingerprint = 234678500;
*
* @param value The fingerprint to set.
* @return This builder for chaining.
*/
public Builder setFingerprint(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
fingerprint_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Fingerprint hash of contents stored in this network interface. This field will be ignored when inserting an Instance or adding a NetworkInterface. An up-to-date fingerprint must be provided in order to update the NetworkInterface. The request will fail with error 400 Bad Request if the fingerprint is not provided, or 412 Precondition Failed if the fingerprint is out of date.
*
*
* optional string fingerprint = 234678500;
*
* @return This builder for chaining.
*/
public Builder clearFingerprint() {
fingerprint_ = getDefaultInstance().getFingerprint();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* Fingerprint hash of contents stored in this network interface. This field will be ignored when inserting an Instance or adding a NetworkInterface. An up-to-date fingerprint must be provided in order to update the NetworkInterface. The request will fail with error 400 Bad Request if the fingerprint is not provided, or 412 Precondition Failed if the fingerprint is out of date.
*
*
* optional string fingerprint = 234678500;
*
* @param value The bytes for fingerprint to set.
* @return This builder for chaining.
*/
public Builder setFingerprintBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fingerprint_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private int internalIpv6PrefixLength_;
/**
*
*
*
* The prefix length of the primary internal IPv6 range.
*
*
* optional int32 internal_ipv6_prefix_length = 203833757;
*
* @return Whether the internalIpv6PrefixLength field is set.
*/
@java.lang.Override
public boolean hasInternalIpv6PrefixLength() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The prefix length of the primary internal IPv6 range.
*
*
* optional int32 internal_ipv6_prefix_length = 203833757;
*
* @return The internalIpv6PrefixLength.
*/
@java.lang.Override
public int getInternalIpv6PrefixLength() {
return internalIpv6PrefixLength_;
}
/**
*
*
*
* The prefix length of the primary internal IPv6 range.
*
*
* optional int32 internal_ipv6_prefix_length = 203833757;
*
* @param value The internalIpv6PrefixLength to set.
* @return This builder for chaining.
*/
public Builder setInternalIpv6PrefixLength(int value) {
internalIpv6PrefixLength_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* The prefix length of the primary internal IPv6 range.
*
*
* optional int32 internal_ipv6_prefix_length = 203833757;
*
* @return This builder for chaining.
*/
public Builder clearInternalIpv6PrefixLength() {
bitField0_ = (bitField0_ & ~0x00000008);
internalIpv6PrefixLength_ = 0;
onChanged();
return this;
}
private java.util.List ipv6AccessConfigs_ =
java.util.Collections.emptyList();
private void ensureIpv6AccessConfigsIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
ipv6AccessConfigs_ =
new java.util.ArrayList(ipv6AccessConfigs_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AccessConfig,
com.google.cloud.compute.v1.AccessConfig.Builder,
com.google.cloud.compute.v1.AccessConfigOrBuilder>
ipv6AccessConfigsBuilder_;
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public java.util.List getIpv6AccessConfigsList() {
if (ipv6AccessConfigsBuilder_ == null) {
return java.util.Collections.unmodifiableList(ipv6AccessConfigs_);
} else {
return ipv6AccessConfigsBuilder_.getMessageList();
}
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public int getIpv6AccessConfigsCount() {
if (ipv6AccessConfigsBuilder_ == null) {
return ipv6AccessConfigs_.size();
} else {
return ipv6AccessConfigsBuilder_.getCount();
}
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public com.google.cloud.compute.v1.AccessConfig getIpv6AccessConfigs(int index) {
if (ipv6AccessConfigsBuilder_ == null) {
return ipv6AccessConfigs_.get(index);
} else {
return ipv6AccessConfigsBuilder_.getMessage(index);
}
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public Builder setIpv6AccessConfigs(int index, com.google.cloud.compute.v1.AccessConfig value) {
if (ipv6AccessConfigsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIpv6AccessConfigsIsMutable();
ipv6AccessConfigs_.set(index, value);
onChanged();
} else {
ipv6AccessConfigsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public Builder setIpv6AccessConfigs(
int index, com.google.cloud.compute.v1.AccessConfig.Builder builderForValue) {
if (ipv6AccessConfigsBuilder_ == null) {
ensureIpv6AccessConfigsIsMutable();
ipv6AccessConfigs_.set(index, builderForValue.build());
onChanged();
} else {
ipv6AccessConfigsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public Builder addIpv6AccessConfigs(com.google.cloud.compute.v1.AccessConfig value) {
if (ipv6AccessConfigsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIpv6AccessConfigsIsMutable();
ipv6AccessConfigs_.add(value);
onChanged();
} else {
ipv6AccessConfigsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public Builder addIpv6AccessConfigs(int index, com.google.cloud.compute.v1.AccessConfig value) {
if (ipv6AccessConfigsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIpv6AccessConfigsIsMutable();
ipv6AccessConfigs_.add(index, value);
onChanged();
} else {
ipv6AccessConfigsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public Builder addIpv6AccessConfigs(
com.google.cloud.compute.v1.AccessConfig.Builder builderForValue) {
if (ipv6AccessConfigsBuilder_ == null) {
ensureIpv6AccessConfigsIsMutable();
ipv6AccessConfigs_.add(builderForValue.build());
onChanged();
} else {
ipv6AccessConfigsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public Builder addIpv6AccessConfigs(
int index, com.google.cloud.compute.v1.AccessConfig.Builder builderForValue) {
if (ipv6AccessConfigsBuilder_ == null) {
ensureIpv6AccessConfigsIsMutable();
ipv6AccessConfigs_.add(index, builderForValue.build());
onChanged();
} else {
ipv6AccessConfigsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public Builder addAllIpv6AccessConfigs(
java.lang.Iterable extends com.google.cloud.compute.v1.AccessConfig> values) {
if (ipv6AccessConfigsBuilder_ == null) {
ensureIpv6AccessConfigsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, ipv6AccessConfigs_);
onChanged();
} else {
ipv6AccessConfigsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public Builder clearIpv6AccessConfigs() {
if (ipv6AccessConfigsBuilder_ == null) {
ipv6AccessConfigs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
ipv6AccessConfigsBuilder_.clear();
}
return this;
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public Builder removeIpv6AccessConfigs(int index) {
if (ipv6AccessConfigsBuilder_ == null) {
ensureIpv6AccessConfigsIsMutable();
ipv6AccessConfigs_.remove(index);
onChanged();
} else {
ipv6AccessConfigsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public com.google.cloud.compute.v1.AccessConfig.Builder getIpv6AccessConfigsBuilder(int index) {
return getIpv6AccessConfigsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public com.google.cloud.compute.v1.AccessConfigOrBuilder getIpv6AccessConfigsOrBuilder(
int index) {
if (ipv6AccessConfigsBuilder_ == null) {
return ipv6AccessConfigs_.get(index);
} else {
return ipv6AccessConfigsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public java.util.List extends com.google.cloud.compute.v1.AccessConfigOrBuilder>
getIpv6AccessConfigsOrBuilderList() {
if (ipv6AccessConfigsBuilder_ != null) {
return ipv6AccessConfigsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(ipv6AccessConfigs_);
}
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public com.google.cloud.compute.v1.AccessConfig.Builder addIpv6AccessConfigsBuilder() {
return getIpv6AccessConfigsFieldBuilder()
.addBuilder(com.google.cloud.compute.v1.AccessConfig.getDefaultInstance());
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public com.google.cloud.compute.v1.AccessConfig.Builder addIpv6AccessConfigsBuilder(int index) {
return getIpv6AccessConfigsFieldBuilder()
.addBuilder(index, com.google.cloud.compute.v1.AccessConfig.getDefaultInstance());
}
/**
*
*
*
* An array of IPv6 access configurations for this interface. Currently, only one IPv6 access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this instance will have no external IPv6 Internet access.
*
*
* repeated .google.cloud.compute.v1.AccessConfig ipv6_access_configs = 483472110;
*/
public java.util.List
getIpv6AccessConfigsBuilderList() {
return getIpv6AccessConfigsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AccessConfig,
com.google.cloud.compute.v1.AccessConfig.Builder,
com.google.cloud.compute.v1.AccessConfigOrBuilder>
getIpv6AccessConfigsFieldBuilder() {
if (ipv6AccessConfigsBuilder_ == null) {
ipv6AccessConfigsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.AccessConfig,
com.google.cloud.compute.v1.AccessConfig.Builder,
com.google.cloud.compute.v1.AccessConfigOrBuilder>(
ipv6AccessConfigs_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
ipv6AccessConfigs_ = null;
}
return ipv6AccessConfigsBuilder_;
}
private java.lang.Object ipv6AccessType_ = "";
/**
*
*
*
* [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed from the Internet. This field is always inherited from its subnetwork. Valid only if stackType is IPV4_IPV6.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return Whether the ipv6AccessType field is set.
*/
public boolean hasIpv6AccessType() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed from the Internet. This field is always inherited from its subnetwork. Valid only if stackType is IPV4_IPV6.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return The ipv6AccessType.
*/
public java.lang.String getIpv6AccessType() {
java.lang.Object ref = ipv6AccessType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ipv6AccessType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed from the Internet. This field is always inherited from its subnetwork. Valid only if stackType is IPV4_IPV6.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return The bytes for ipv6AccessType.
*/
public com.google.protobuf.ByteString getIpv6AccessTypeBytes() {
java.lang.Object ref = ipv6AccessType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
ipv6AccessType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed from the Internet. This field is always inherited from its subnetwork. Valid only if stackType is IPV4_IPV6.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @param value The ipv6AccessType to set.
* @return This builder for chaining.
*/
public Builder setIpv6AccessType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ipv6AccessType_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed from the Internet. This field is always inherited from its subnetwork. Valid only if stackType is IPV4_IPV6.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return This builder for chaining.
*/
public Builder clearIpv6AccessType() {
ipv6AccessType_ = getDefaultInstance().getIpv6AccessType();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed from the Internet. This field is always inherited from its subnetwork. Valid only if stackType is IPV4_IPV6.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @param value The bytes for ipv6AccessType to set.
* @return This builder for chaining.
*/
public Builder setIpv6AccessTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ipv6AccessType_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.lang.Object ipv6Address_ = "";
/**
*
*
*
* An IPv6 internal network address for this network interface. To use a static internal IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
*
*
* optional string ipv6_address = 341563804;
*
* @return Whether the ipv6Address field is set.
*/
public boolean hasIpv6Address() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* An IPv6 internal network address for this network interface. To use a static internal IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
*
*
* optional string ipv6_address = 341563804;
*
* @return The ipv6Address.
*/
public java.lang.String getIpv6Address() {
java.lang.Object ref = ipv6Address_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ipv6Address_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* An IPv6 internal network address for this network interface. To use a static internal IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
*
*
* optional string ipv6_address = 341563804;
*
* @return The bytes for ipv6Address.
*/
public com.google.protobuf.ByteString getIpv6AddressBytes() {
java.lang.Object ref = ipv6Address_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
ipv6Address_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* An IPv6 internal network address for this network interface. To use a static internal IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
*
*
* optional string ipv6_address = 341563804;
*
* @param value The ipv6Address to set.
* @return This builder for chaining.
*/
public Builder setIpv6Address(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ipv6Address_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* An IPv6 internal network address for this network interface. To use a static internal IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
*
*
* optional string ipv6_address = 341563804;
*
* @return This builder for chaining.
*/
public Builder clearIpv6Address() {
ipv6Address_ = getDefaultInstance().getIpv6Address();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* An IPv6 internal network address for this network interface. To use a static internal IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
*
*
* optional string ipv6_address = 341563804;
*
* @param value The bytes for ipv6Address to set.
* @return This builder for chaining.
*/
public Builder setIpv6AddressBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ipv6Address_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
public boolean hasKind() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
*
*
* optional string kind = 3292052;
*
* @param value The kind to set.
* @return This builder for chaining.
*/
public Builder setKind(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
*
*
* optional string kind = 3292052;
*
* @return This builder for chaining.
*/
public Builder clearKind() {
kind_ = getDefaultInstance().getKind();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#networkInterface for network interfaces.
*
*
* optional string kind = 3292052;
*
* @param value The bytes for kind to set.
* @return This builder for chaining.
*/
public Builder setKindBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
kind_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
*
*
* [Output Only] The name of the network interface, which is generated by the server. For a VM, the network interface uses the nicN naming format. Where N is a value between 0 and 7. The default interface value is nic0.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* [Output Only] The name of the network interface, which is generated by the server. For a VM, the network interface uses the nicN naming format. Where N is a value between 0 and 7. The default interface value is nic0.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The name of the network interface, which is generated by the server. For a VM, the network interface uses the nicN naming format. Where N is a value between 0 and 7. The default interface value is nic0.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The name of the network interface, which is generated by the server. For a VM, the network interface uses the nicN naming format. Where N is a value between 0 and 7. The default interface value is nic0.
*
*
* optional string name = 3373707;
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The name of the network interface, which is generated by the server. For a VM, the network interface uses the nicN naming format. Where N is a value between 0 and 7. The default interface value is nic0.
*
*
* optional string name = 3373707;
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The name of the network interface, which is generated by the server. For a VM, the network interface uses the nicN naming format. Where N is a value between 0 and 7. The default interface value is nic0.
*
*
* optional string name = 3373707;
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private java.lang.Object network_ = "";
/**
*
*
*
* URL of the VPC network resource for this instance. When creating an instance, if neither the network nor the subnetwork is specified, the default network global/networks/default is used. If the selected project doesn't have the default network, you must specify a network or subnet. If the network is not specified but the subnetwork is specified, the network is inferred. If you specify this property, you can specify the network as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/global/networks/ network - projects/project/global/networks/network - global/networks/default
*
*
* optional string network = 232872494;
*
* @return Whether the network field is set.
*/
public boolean hasNetwork() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* URL of the VPC network resource for this instance. When creating an instance, if neither the network nor the subnetwork is specified, the default network global/networks/default is used. If the selected project doesn't have the default network, you must specify a network or subnet. If the network is not specified but the subnetwork is specified, the network is inferred. If you specify this property, you can specify the network as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/global/networks/ network - projects/project/global/networks/network - global/networks/default
*
*
* optional string network = 232872494;
*
* @return The network.
*/
public java.lang.String getNetwork() {
java.lang.Object ref = network_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
network_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* URL of the VPC network resource for this instance. When creating an instance, if neither the network nor the subnetwork is specified, the default network global/networks/default is used. If the selected project doesn't have the default network, you must specify a network or subnet. If the network is not specified but the subnetwork is specified, the network is inferred. If you specify this property, you can specify the network as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/global/networks/ network - projects/project/global/networks/network - global/networks/default
*
*
* optional string network = 232872494;
*
* @return The bytes for network.
*/
public com.google.protobuf.ByteString getNetworkBytes() {
java.lang.Object ref = network_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
network_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* URL of the VPC network resource for this instance. When creating an instance, if neither the network nor the subnetwork is specified, the default network global/networks/default is used. If the selected project doesn't have the default network, you must specify a network or subnet. If the network is not specified but the subnetwork is specified, the network is inferred. If you specify this property, you can specify the network as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/global/networks/ network - projects/project/global/networks/network - global/networks/default
*
*
* optional string network = 232872494;
*
* @param value The network to set.
* @return This builder for chaining.
*/
public Builder setNetwork(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
network_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* URL of the VPC network resource for this instance. When creating an instance, if neither the network nor the subnetwork is specified, the default network global/networks/default is used. If the selected project doesn't have the default network, you must specify a network or subnet. If the network is not specified but the subnetwork is specified, the network is inferred. If you specify this property, you can specify the network as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/global/networks/ network - projects/project/global/networks/network - global/networks/default
*
*
* optional string network = 232872494;
*
* @return This builder for chaining.
*/
public Builder clearNetwork() {
network_ = getDefaultInstance().getNetwork();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
*
*
*
* URL of the VPC network resource for this instance. When creating an instance, if neither the network nor the subnetwork is specified, the default network global/networks/default is used. If the selected project doesn't have the default network, you must specify a network or subnet. If the network is not specified but the subnetwork is specified, the network is inferred. If you specify this property, you can specify the network as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/global/networks/ network - projects/project/global/networks/network - global/networks/default
*
*
* optional string network = 232872494;
*
* @param value The bytes for network to set.
* @return This builder for chaining.
*/
public Builder setNetworkBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
network_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
private java.lang.Object networkAttachment_ = "";
/**
*
*
*
* The URL of the network attachment that this interface should connect to in the following format: projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
*
*
* optional string network_attachment = 224644052;
*
* @return Whether the networkAttachment field is set.
*/
public boolean hasNetworkAttachment() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* The URL of the network attachment that this interface should connect to in the following format: projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
*
*
* optional string network_attachment = 224644052;
*
* @return The networkAttachment.
*/
public java.lang.String getNetworkAttachment() {
java.lang.Object ref = networkAttachment_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
networkAttachment_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The URL of the network attachment that this interface should connect to in the following format: projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
*
*
* optional string network_attachment = 224644052;
*
* @return The bytes for networkAttachment.
*/
public com.google.protobuf.ByteString getNetworkAttachmentBytes() {
java.lang.Object ref = networkAttachment_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
networkAttachment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The URL of the network attachment that this interface should connect to in the following format: projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
*
*
* optional string network_attachment = 224644052;
*
* @param value The networkAttachment to set.
* @return This builder for chaining.
*/
public Builder setNetworkAttachment(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
networkAttachment_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* The URL of the network attachment that this interface should connect to in the following format: projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
*
*
* optional string network_attachment = 224644052;
*
* @return This builder for chaining.
*/
public Builder clearNetworkAttachment() {
networkAttachment_ = getDefaultInstance().getNetworkAttachment();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
return this;
}
/**
*
*
*
* The URL of the network attachment that this interface should connect to in the following format: projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
*
*
* optional string network_attachment = 224644052;
*
* @param value The bytes for networkAttachment to set.
* @return This builder for chaining.
*/
public Builder setNetworkAttachmentBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
networkAttachment_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
private java.lang.Object networkIP_ = "";
/**
*
*
*
* An IPv4 internal IP address to assign to the instance for this network interface. If not specified by the user, an unused internal IP is assigned by the system.
*
*
* optional string network_i_p = 207181961;
*
* @return Whether the networkIP field is set.
*/
public boolean hasNetworkIP() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* An IPv4 internal IP address to assign to the instance for this network interface. If not specified by the user, an unused internal IP is assigned by the system.
*
*
* optional string network_i_p = 207181961;
*
* @return The networkIP.
*/
public java.lang.String getNetworkIP() {
java.lang.Object ref = networkIP_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
networkIP_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* An IPv4 internal IP address to assign to the instance for this network interface. If not specified by the user, an unused internal IP is assigned by the system.
*
*
* optional string network_i_p = 207181961;
*
* @return The bytes for networkIP.
*/
public com.google.protobuf.ByteString getNetworkIPBytes() {
java.lang.Object ref = networkIP_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
networkIP_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* An IPv4 internal IP address to assign to the instance for this network interface. If not specified by the user, an unused internal IP is assigned by the system.
*
*
* optional string network_i_p = 207181961;
*
* @param value The networkIP to set.
* @return This builder for chaining.
*/
public Builder setNetworkIP(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
networkIP_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* An IPv4 internal IP address to assign to the instance for this network interface. If not specified by the user, an unused internal IP is assigned by the system.
*
*
* optional string network_i_p = 207181961;
*
* @return This builder for chaining.
*/
public Builder clearNetworkIP() {
networkIP_ = getDefaultInstance().getNetworkIP();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
/**
*
*
*
* An IPv4 internal IP address to assign to the instance for this network interface. If not specified by the user, an unused internal IP is assigned by the system.
*
*
* optional string network_i_p = 207181961;
*
* @param value The bytes for networkIP to set.
* @return This builder for chaining.
*/
public Builder setNetworkIPBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
networkIP_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
private java.lang.Object nicType_ = "";
/**
*
*
*
* The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* Check the NicType enum for the list of possible values.
*
*
* optional string nic_type = 59810577;
*
* @return Whether the nicType field is set.
*/
public boolean hasNicType() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* Check the NicType enum for the list of possible values.
*
*
* optional string nic_type = 59810577;
*
* @return The nicType.
*/
public java.lang.String getNicType() {
java.lang.Object ref = nicType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nicType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* Check the NicType enum for the list of possible values.
*
*
* optional string nic_type = 59810577;
*
* @return The bytes for nicType.
*/
public com.google.protobuf.ByteString getNicTypeBytes() {
java.lang.Object ref = nicType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
nicType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* Check the NicType enum for the list of possible values.
*
*
* optional string nic_type = 59810577;
*
* @param value The nicType to set.
* @return This builder for chaining.
*/
public Builder setNicType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
nicType_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* Check the NicType enum for the list of possible values.
*
*
* optional string nic_type = 59810577;
*
* @return This builder for chaining.
*/
public Builder clearNicType() {
nicType_ = getDefaultInstance().getNicType();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
return this;
}
/**
*
*
*
* The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* Check the NicType enum for the list of possible values.
*
*
* optional string nic_type = 59810577;
*
* @param value The bytes for nicType to set.
* @return This builder for chaining.
*/
public Builder setNicTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
nicType_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
private int queueCount_;
/**
*
*
*
* The networking queue count that's specified by users for the network interface. Both Rx and Tx queues will be set to this number. It'll be empty if not specified by the users.
*
*
* optional int32 queue_count = 503708769;
*
* @return Whether the queueCount field is set.
*/
@java.lang.Override
public boolean hasQueueCount() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*
*
* The networking queue count that's specified by users for the network interface. Both Rx and Tx queues will be set to this number. It'll be empty if not specified by the users.
*
*
* optional int32 queue_count = 503708769;
*
* @return The queueCount.
*/
@java.lang.Override
public int getQueueCount() {
return queueCount_;
}
/**
*
*
*
* The networking queue count that's specified by users for the network interface. Both Rx and Tx queues will be set to this number. It'll be empty if not specified by the users.
*
*
* optional int32 queue_count = 503708769;
*
* @param value The queueCount to set.
* @return This builder for chaining.
*/
public Builder setQueueCount(int value) {
queueCount_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* The networking queue count that's specified by users for the network interface. Both Rx and Tx queues will be set to this number. It'll be empty if not specified by the users.
*
*
* optional int32 queue_count = 503708769;
*
* @return This builder for chaining.
*/
public Builder clearQueueCount() {
bitField0_ = (bitField0_ & ~0x00002000);
queueCount_ = 0;
onChanged();
return this;
}
private java.lang.Object stackType_ = "";
/**
*
*
*
* The stack type for this network interface. To assign only IPv4 addresses, use IPV4_ONLY. To assign both IPv4 and IPv6 addresses, use IPV4_IPV6. If not specified, IPV4_ONLY is used. This field can be both set at instance creation and update network interface operations.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return Whether the stackType field is set.
*/
public boolean hasStackType() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*
*
* The stack type for this network interface. To assign only IPv4 addresses, use IPV4_ONLY. To assign both IPv4 and IPv6 addresses, use IPV4_IPV6. If not specified, IPV4_ONLY is used. This field can be both set at instance creation and update network interface operations.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The stackType.
*/
public java.lang.String getStackType() {
java.lang.Object ref = stackType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stackType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The stack type for this network interface. To assign only IPv4 addresses, use IPV4_ONLY. To assign both IPv4 and IPv6 addresses, use IPV4_IPV6. If not specified, IPV4_ONLY is used. This field can be both set at instance creation and update network interface operations.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The bytes for stackType.
*/
public com.google.protobuf.ByteString getStackTypeBytes() {
java.lang.Object ref = stackType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
stackType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The stack type for this network interface. To assign only IPv4 addresses, use IPV4_ONLY. To assign both IPv4 and IPv6 addresses, use IPV4_IPV6. If not specified, IPV4_ONLY is used. This field can be both set at instance creation and update network interface operations.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @param value The stackType to set.
* @return This builder for chaining.
*/
public Builder setStackType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stackType_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* The stack type for this network interface. To assign only IPv4 addresses, use IPV4_ONLY. To assign both IPv4 and IPv6 addresses, use IPV4_IPV6. If not specified, IPV4_ONLY is used. This field can be both set at instance creation and update network interface operations.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return This builder for chaining.
*/
public Builder clearStackType() {
stackType_ = getDefaultInstance().getStackType();
bitField0_ = (bitField0_ & ~0x00004000);
onChanged();
return this;
}
/**
*
*
*
* The stack type for this network interface. To assign only IPv4 addresses, use IPV4_ONLY. To assign both IPv4 and IPv6 addresses, use IPV4_IPV6. If not specified, IPV4_ONLY is used. This field can be both set at instance creation and update network interface operations.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @param value The bytes for stackType to set.
* @return This builder for chaining.
*/
public Builder setStackTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stackType_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
private java.lang.Object subnetwork_ = "";
/**
*
*
*
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy mode, do not specify this field. If the network is in auto subnet mode, specifying the subnetwork is optional. If the network is in custom subnet mode, specifying the subnetwork is required. If you specify this field, you can specify the subnetwork as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/regions/region /subnetworks/subnetwork - regions/region/subnetworks/subnetwork
*
*
* optional string subnetwork = 307827694;
*
* @return Whether the subnetwork field is set.
*/
public boolean hasSubnetwork() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
*
*
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy mode, do not specify this field. If the network is in auto subnet mode, specifying the subnetwork is optional. If the network is in custom subnet mode, specifying the subnetwork is required. If you specify this field, you can specify the subnetwork as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/regions/region /subnetworks/subnetwork - regions/region/subnetworks/subnetwork
*
*
* optional string subnetwork = 307827694;
*
* @return The subnetwork.
*/
public java.lang.String getSubnetwork() {
java.lang.Object ref = subnetwork_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subnetwork_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy mode, do not specify this field. If the network is in auto subnet mode, specifying the subnetwork is optional. If the network is in custom subnet mode, specifying the subnetwork is required. If you specify this field, you can specify the subnetwork as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/regions/region /subnetworks/subnetwork - regions/region/subnetworks/subnetwork
*
*
* optional string subnetwork = 307827694;
*
* @return The bytes for subnetwork.
*/
public com.google.protobuf.ByteString getSubnetworkBytes() {
java.lang.Object ref = subnetwork_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
subnetwork_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy mode, do not specify this field. If the network is in auto subnet mode, specifying the subnetwork is optional. If the network is in custom subnet mode, specifying the subnetwork is required. If you specify this field, you can specify the subnetwork as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/regions/region /subnetworks/subnetwork - regions/region/subnetworks/subnetwork
*
*
* optional string subnetwork = 307827694;
*
* @param value The subnetwork to set.
* @return This builder for chaining.
*/
public Builder setSubnetwork(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
subnetwork_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy mode, do not specify this field. If the network is in auto subnet mode, specifying the subnetwork is optional. If the network is in custom subnet mode, specifying the subnetwork is required. If you specify this field, you can specify the subnetwork as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/regions/region /subnetworks/subnetwork - regions/region/subnetworks/subnetwork
*
*
* optional string subnetwork = 307827694;
*
* @return This builder for chaining.
*/
public Builder clearSubnetwork() {
subnetwork_ = getDefaultInstance().getSubnetwork();
bitField0_ = (bitField0_ & ~0x00008000);
onChanged();
return this;
}
/**
*
*
*
* The URL of the Subnetwork resource for this instance. If the network resource is in legacy mode, do not specify this field. If the network is in auto subnet mode, specifying the subnetwork is optional. If the network is in custom subnet mode, specifying the subnetwork is required. If you specify this field, you can specify the subnetwork as a full or partial URL. For example, the following are all valid URLs: - https://www.googleapis.com/compute/v1/projects/project/regions/region /subnetworks/subnetwork - regions/region/subnetworks/subnetwork
*
*
* optional string subnetwork = 307827694;
*
* @param value The bytes for subnetwork to set.
* @return This builder for chaining.
*/
public Builder setSubnetworkBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
subnetwork_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.NetworkInterface)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.NetworkInterface)
private static final com.google.cloud.compute.v1.NetworkInterface DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.NetworkInterface();
}
public static com.google.cloud.compute.v1.NetworkInterface getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NetworkInterface parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkInterface getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy