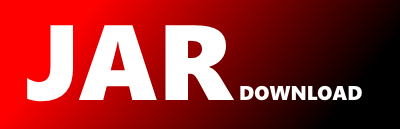
com.google.cloud.compute.v1.NetworkPeering Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* A network peering attached to a network resource. The message includes the peering name, peer network, peering state, and a flag indicating whether Google Compute Engine should automatically create routes for the peering.
*
*
* Protobuf type {@code google.cloud.compute.v1.NetworkPeering}
*/
public final class NetworkPeering extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.NetworkPeering)
NetworkPeeringOrBuilder {
private static final long serialVersionUID = 0L;
// Use NetworkPeering.newBuilder() to construct.
private NetworkPeering(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NetworkPeering() {
name_ = "";
network_ = "";
stackType_ = "";
state_ = "";
stateDetails_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new NetworkPeering();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkPeering_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkPeering_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.NetworkPeering.class,
com.google.cloud.compute.v1.NetworkPeering.Builder.class);
}
/**
*
*
*
* Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY.
*
*
* Protobuf enum {@code google.cloud.compute.v1.NetworkPeering.StackType}
*/
public enum StackType implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STACK_TYPE = 0;
*/
UNDEFINED_STACK_TYPE(0),
/**
*
*
*
* This Peering will allow IPv4 traffic and routes to be exchanged. Additionally if the matching peering is IPV4_IPV6, IPv6 traffic and routes will be exchanged as well.
*
*
* IPV4_IPV6 = 22197249;
*/
IPV4_IPV6(22197249),
/**
*
*
*
* This Peering will only allow IPv4 traffic and routes to be exchanged, even if the matching peering is IPV4_IPV6.
*
*
* IPV4_ONLY = 22373798;
*/
IPV4_ONLY(22373798),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STACK_TYPE = 0;
*/
public static final int UNDEFINED_STACK_TYPE_VALUE = 0;
/**
*
*
*
* This Peering will allow IPv4 traffic and routes to be exchanged. Additionally if the matching peering is IPV4_IPV6, IPv6 traffic and routes will be exchanged as well.
*
*
* IPV4_IPV6 = 22197249;
*/
public static final int IPV4_IPV6_VALUE = 22197249;
/**
*
*
*
* This Peering will only allow IPv4 traffic and routes to be exchanged, even if the matching peering is IPV4_IPV6.
*
*
* IPV4_ONLY = 22373798;
*/
public static final int IPV4_ONLY_VALUE = 22373798;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static StackType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static StackType forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_STACK_TYPE;
case 22197249:
return IPV4_IPV6;
case 22373798:
return IPV4_ONLY;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public StackType findValueByNumber(int number) {
return StackType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.NetworkPeering.getDescriptor().getEnumTypes().get(0);
}
private static final StackType[] VALUES = values();
public static StackType valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private StackType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.NetworkPeering.StackType)
}
/**
*
*
*
* [Output Only] State for the peering, either `ACTIVE` or `INACTIVE`. The peering is `ACTIVE` when there's a matching configuration in the peer network.
*
*
* Protobuf enum {@code google.cloud.compute.v1.NetworkPeering.State}
*/
public enum State implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STATE = 0;
*/
UNDEFINED_STATE(0),
/**
*
*
*
* Matching configuration exists on the peer.
*
*
* ACTIVE = 314733318;
*/
ACTIVE(314733318),
/**
*
*
*
* There is no matching configuration on the peer, including the case when peer does not exist.
*
*
* INACTIVE = 270421099;
*/
INACTIVE(270421099),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STATE = 0;
*/
public static final int UNDEFINED_STATE_VALUE = 0;
/**
*
*
*
* Matching configuration exists on the peer.
*
*
* ACTIVE = 314733318;
*/
public static final int ACTIVE_VALUE = 314733318;
/**
*
*
*
* There is no matching configuration on the peer, including the case when peer does not exist.
*
*
* INACTIVE = 270421099;
*/
public static final int INACTIVE_VALUE = 270421099;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static State valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static State forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_STATE;
case 314733318:
return ACTIVE;
case 270421099:
return INACTIVE;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public State findValueByNumber(int number) {
return State.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.NetworkPeering.getDescriptor().getEnumTypes().get(1);
}
private static final State[] VALUES = values();
public static State valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private State(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.NetworkPeering.State)
}
private int bitField0_;
public static final int AUTO_CREATE_ROUTES_FIELD_NUMBER = 57454941;
private boolean autoCreateRoutes_ = false;
/**
*
*
*
* This field will be deprecated soon. Use the exchange_subnet_routes field instead. Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool auto_create_routes = 57454941;
*
* @return Whether the autoCreateRoutes field is set.
*/
@java.lang.Override
public boolean hasAutoCreateRoutes() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* This field will be deprecated soon. Use the exchange_subnet_routes field instead. Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool auto_create_routes = 57454941;
*
* @return The autoCreateRoutes.
*/
@java.lang.Override
public boolean getAutoCreateRoutes() {
return autoCreateRoutes_;
}
public static final int EXCHANGE_SUBNET_ROUTES_FIELD_NUMBER = 26322256;
private boolean exchangeSubnetRoutes_ = false;
/**
*
*
*
* Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool exchange_subnet_routes = 26322256;
*
* @return Whether the exchangeSubnetRoutes field is set.
*/
@java.lang.Override
public boolean hasExchangeSubnetRoutes() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool exchange_subnet_routes = 26322256;
*
* @return The exchangeSubnetRoutes.
*/
@java.lang.Override
public boolean getExchangeSubnetRoutes() {
return exchangeSubnetRoutes_;
}
public static final int EXPORT_CUSTOM_ROUTES_FIELD_NUMBER = 60281485;
private boolean exportCustomRoutes_ = false;
/**
*
*
*
* Whether to export the custom routes to peer network. The default value is false.
*
*
* optional bool export_custom_routes = 60281485;
*
* @return Whether the exportCustomRoutes field is set.
*/
@java.lang.Override
public boolean hasExportCustomRoutes() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Whether to export the custom routes to peer network. The default value is false.
*
*
* optional bool export_custom_routes = 60281485;
*
* @return The exportCustomRoutes.
*/
@java.lang.Override
public boolean getExportCustomRoutes() {
return exportCustomRoutes_;
}
public static final int EXPORT_SUBNET_ROUTES_WITH_PUBLIC_IP_FIELD_NUMBER = 97940834;
private boolean exportSubnetRoutesWithPublicIp_ = false;
/**
*
*
*
* Whether subnet routes with public IP range are exported. The default value is true, all subnet routes are exported. IPv4 special-use ranges are always exported to peers and are not controlled by this field.
*
*
* optional bool export_subnet_routes_with_public_ip = 97940834;
*
* @return Whether the exportSubnetRoutesWithPublicIp field is set.
*/
@java.lang.Override
public boolean hasExportSubnetRoutesWithPublicIp() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Whether subnet routes with public IP range are exported. The default value is true, all subnet routes are exported. IPv4 special-use ranges are always exported to peers and are not controlled by this field.
*
*
* optional bool export_subnet_routes_with_public_ip = 97940834;
*
* @return The exportSubnetRoutesWithPublicIp.
*/
@java.lang.Override
public boolean getExportSubnetRoutesWithPublicIp() {
return exportSubnetRoutesWithPublicIp_;
}
public static final int IMPORT_CUSTOM_ROUTES_FIELD_NUMBER = 197982398;
private boolean importCustomRoutes_ = false;
/**
*
*
*
* Whether to import the custom routes from peer network. The default value is false.
*
*
* optional bool import_custom_routes = 197982398;
*
* @return Whether the importCustomRoutes field is set.
*/
@java.lang.Override
public boolean hasImportCustomRoutes() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Whether to import the custom routes from peer network. The default value is false.
*
*
* optional bool import_custom_routes = 197982398;
*
* @return The importCustomRoutes.
*/
@java.lang.Override
public boolean getImportCustomRoutes() {
return importCustomRoutes_;
}
public static final int IMPORT_SUBNET_ROUTES_WITH_PUBLIC_IP_FIELD_NUMBER = 14419729;
private boolean importSubnetRoutesWithPublicIp_ = false;
/**
*
*
*
* Whether subnet routes with public IP range are imported. The default value is false. IPv4 special-use ranges are always imported from peers and are not controlled by this field.
*
*
* optional bool import_subnet_routes_with_public_ip = 14419729;
*
* @return Whether the importSubnetRoutesWithPublicIp field is set.
*/
@java.lang.Override
public boolean hasImportSubnetRoutesWithPublicIp() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Whether subnet routes with public IP range are imported. The default value is false. IPv4 special-use ranges are always imported from peers and are not controlled by this field.
*
*
* optional bool import_subnet_routes_with_public_ip = 14419729;
*
* @return The importSubnetRoutesWithPublicIp.
*/
@java.lang.Override
public boolean getImportSubnetRoutesWithPublicIp() {
return importSubnetRoutesWithPublicIp_;
}
public static final int NAME_FIELD_NUMBER = 3373707;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* Name of this peering. Provided by the client when the peering is created. The name must comply with RFC1035. Specifically, the name must be 1-63 characters long and match regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be a lowercase letter, and all the following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Name of this peering. Provided by the client when the peering is created. The name must comply with RFC1035. Specifically, the name must be 1-63 characters long and match regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be a lowercase letter, and all the following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* Name of this peering. Provided by the client when the peering is created. The name must comply with RFC1035. Specifically, the name must be 1-63 characters long and match regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be a lowercase letter, and all the following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NETWORK_FIELD_NUMBER = 232872494;
@SuppressWarnings("serial")
private volatile java.lang.Object network_ = "";
/**
*
*
*
* The URL of the peer network. It can be either full URL or partial URL. The peer network may belong to a different project. If the partial URL does not contain project, it is assumed that the peer network is in the same project as the current network.
*
*
* optional string network = 232872494;
*
* @return Whether the network field is set.
*/
@java.lang.Override
public boolean hasNetwork() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The URL of the peer network. It can be either full URL or partial URL. The peer network may belong to a different project. If the partial URL does not contain project, it is assumed that the peer network is in the same project as the current network.
*
*
* optional string network = 232872494;
*
* @return The network.
*/
@java.lang.Override
public java.lang.String getNetwork() {
java.lang.Object ref = network_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
network_ = s;
return s;
}
}
/**
*
*
*
* The URL of the peer network. It can be either full URL or partial URL. The peer network may belong to a different project. If the partial URL does not contain project, it is assumed that the peer network is in the same project as the current network.
*
*
* optional string network = 232872494;
*
* @return The bytes for network.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNetworkBytes() {
java.lang.Object ref = network_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
network_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PEER_MTU_FIELD_NUMBER = 69584721;
private int peerMtu_ = 0;
/**
*
*
*
* Maximum Transmission Unit in bytes.
*
*
* optional int32 peer_mtu = 69584721;
*
* @return Whether the peerMtu field is set.
*/
@java.lang.Override
public boolean hasPeerMtu() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Maximum Transmission Unit in bytes.
*
*
* optional int32 peer_mtu = 69584721;
*
* @return The peerMtu.
*/
@java.lang.Override
public int getPeerMtu() {
return peerMtu_;
}
public static final int STACK_TYPE_FIELD_NUMBER = 425908881;
@SuppressWarnings("serial")
private volatile java.lang.Object stackType_ = "";
/**
*
*
*
* Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return Whether the stackType field is set.
*/
@java.lang.Override
public boolean hasStackType() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The stackType.
*/
@java.lang.Override
public java.lang.String getStackType() {
java.lang.Object ref = stackType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stackType_ = s;
return s;
}
}
/**
*
*
*
* Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The bytes for stackType.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStackTypeBytes() {
java.lang.Object ref = stackType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
stackType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATE_FIELD_NUMBER = 109757585;
@SuppressWarnings("serial")
private volatile java.lang.Object state_ = "";
/**
*
*
*
* [Output Only] State for the peering, either `ACTIVE` or `INACTIVE`. The peering is `ACTIVE` when there's a matching configuration in the peer network.
* Check the State enum for the list of possible values.
*
*
* optional string state = 109757585;
*
* @return Whether the state field is set.
*/
@java.lang.Override
public boolean hasState() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* [Output Only] State for the peering, either `ACTIVE` or `INACTIVE`. The peering is `ACTIVE` when there's a matching configuration in the peer network.
* Check the State enum for the list of possible values.
*
*
* optional string state = 109757585;
*
* @return The state.
*/
@java.lang.Override
public java.lang.String getState() {
java.lang.Object ref = state_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
state_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] State for the peering, either `ACTIVE` or `INACTIVE`. The peering is `ACTIVE` when there's a matching configuration in the peer network.
* Check the State enum for the list of possible values.
*
*
* optional string state = 109757585;
*
* @return The bytes for state.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStateBytes() {
java.lang.Object ref = state_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
state_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATE_DETAILS_FIELD_NUMBER = 95566996;
@SuppressWarnings("serial")
private volatile java.lang.Object stateDetails_ = "";
/**
*
*
*
* [Output Only] Details about the current state of the peering.
*
*
* optional string state_details = 95566996;
*
* @return Whether the stateDetails field is set.
*/
@java.lang.Override
public boolean hasStateDetails() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* [Output Only] Details about the current state of the peering.
*
*
* optional string state_details = 95566996;
*
* @return The stateDetails.
*/
@java.lang.Override
public java.lang.String getStateDetails() {
java.lang.Object ref = stateDetails_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stateDetails_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Details about the current state of the peering.
*
*
* optional string state_details = 95566996;
*
* @return The bytes for stateDetails.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStateDetailsBytes() {
java.lang.Object ref = stateDetails_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
stateDetails_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3373707, name_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeBool(14419729, importSubnetRoutesWithPublicIp_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBool(26322256, exchangeSubnetRoutes_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBool(57454941, autoCreateRoutes_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(60281485, exportCustomRoutes_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeInt32(69584721, peerMtu_);
}
if (((bitField0_ & 0x00000800) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 95566996, stateDetails_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBool(97940834, exportSubnetRoutesWithPublicIp_);
}
if (((bitField0_ & 0x00000400) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 109757585, state_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeBool(197982398, importCustomRoutes_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 232872494, network_);
}
if (((bitField0_ & 0x00000200) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 425908881, stackType_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3373707, name_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeBoolSize(
14419729, importSubnetRoutesWithPublicIp_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeBoolSize(26322256, exchangeSubnetRoutes_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(57454941, autoCreateRoutes_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(60281485, exportCustomRoutes_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(69584721, peerMtu_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(95566996, stateDetails_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeBoolSize(
97940834, exportSubnetRoutesWithPublicIp_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(109757585, state_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(197982398, importCustomRoutes_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(232872494, network_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(425908881, stackType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.NetworkPeering)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.NetworkPeering other =
(com.google.cloud.compute.v1.NetworkPeering) obj;
if (hasAutoCreateRoutes() != other.hasAutoCreateRoutes()) return false;
if (hasAutoCreateRoutes()) {
if (getAutoCreateRoutes() != other.getAutoCreateRoutes()) return false;
}
if (hasExchangeSubnetRoutes() != other.hasExchangeSubnetRoutes()) return false;
if (hasExchangeSubnetRoutes()) {
if (getExchangeSubnetRoutes() != other.getExchangeSubnetRoutes()) return false;
}
if (hasExportCustomRoutes() != other.hasExportCustomRoutes()) return false;
if (hasExportCustomRoutes()) {
if (getExportCustomRoutes() != other.getExportCustomRoutes()) return false;
}
if (hasExportSubnetRoutesWithPublicIp() != other.hasExportSubnetRoutesWithPublicIp())
return false;
if (hasExportSubnetRoutesWithPublicIp()) {
if (getExportSubnetRoutesWithPublicIp() != other.getExportSubnetRoutesWithPublicIp())
return false;
}
if (hasImportCustomRoutes() != other.hasImportCustomRoutes()) return false;
if (hasImportCustomRoutes()) {
if (getImportCustomRoutes() != other.getImportCustomRoutes()) return false;
}
if (hasImportSubnetRoutesWithPublicIp() != other.hasImportSubnetRoutesWithPublicIp())
return false;
if (hasImportSubnetRoutesWithPublicIp()) {
if (getImportSubnetRoutesWithPublicIp() != other.getImportSubnetRoutesWithPublicIp())
return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName().equals(other.getName())) return false;
}
if (hasNetwork() != other.hasNetwork()) return false;
if (hasNetwork()) {
if (!getNetwork().equals(other.getNetwork())) return false;
}
if (hasPeerMtu() != other.hasPeerMtu()) return false;
if (hasPeerMtu()) {
if (getPeerMtu() != other.getPeerMtu()) return false;
}
if (hasStackType() != other.hasStackType()) return false;
if (hasStackType()) {
if (!getStackType().equals(other.getStackType())) return false;
}
if (hasState() != other.hasState()) return false;
if (hasState()) {
if (!getState().equals(other.getState())) return false;
}
if (hasStateDetails() != other.hasStateDetails()) return false;
if (hasStateDetails()) {
if (!getStateDetails().equals(other.getStateDetails())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAutoCreateRoutes()) {
hash = (37 * hash) + AUTO_CREATE_ROUTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getAutoCreateRoutes());
}
if (hasExchangeSubnetRoutes()) {
hash = (37 * hash) + EXCHANGE_SUBNET_ROUTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getExchangeSubnetRoutes());
}
if (hasExportCustomRoutes()) {
hash = (37 * hash) + EXPORT_CUSTOM_ROUTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getExportCustomRoutes());
}
if (hasExportSubnetRoutesWithPublicIp()) {
hash = (37 * hash) + EXPORT_SUBNET_ROUTES_WITH_PUBLIC_IP_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashBoolean(getExportSubnetRoutesWithPublicIp());
}
if (hasImportCustomRoutes()) {
hash = (37 * hash) + IMPORT_CUSTOM_ROUTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getImportCustomRoutes());
}
if (hasImportSubnetRoutesWithPublicIp()) {
hash = (37 * hash) + IMPORT_SUBNET_ROUTES_WITH_PUBLIC_IP_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashBoolean(getImportSubnetRoutesWithPublicIp());
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasNetwork()) {
hash = (37 * hash) + NETWORK_FIELD_NUMBER;
hash = (53 * hash) + getNetwork().hashCode();
}
if (hasPeerMtu()) {
hash = (37 * hash) + PEER_MTU_FIELD_NUMBER;
hash = (53 * hash) + getPeerMtu();
}
if (hasStackType()) {
hash = (37 * hash) + STACK_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getStackType().hashCode();
}
if (hasState()) {
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + getState().hashCode();
}
if (hasStateDetails()) {
hash = (37 * hash) + STATE_DETAILS_FIELD_NUMBER;
hash = (53 * hash) + getStateDetails().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.NetworkPeering parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.NetworkPeering parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkPeering parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.NetworkPeering parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkPeering parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.NetworkPeering parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkPeering parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.NetworkPeering parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkPeering parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.NetworkPeering parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.NetworkPeering parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.NetworkPeering parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.NetworkPeering prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* A network peering attached to a network resource. The message includes the peering name, peer network, peering state, and a flag indicating whether Google Compute Engine should automatically create routes for the peering.
*
*
* Protobuf type {@code google.cloud.compute.v1.NetworkPeering}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.NetworkPeering)
com.google.cloud.compute.v1.NetworkPeeringOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkPeering_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkPeering_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.NetworkPeering.class,
com.google.cloud.compute.v1.NetworkPeering.Builder.class);
}
// Construct using com.google.cloud.compute.v1.NetworkPeering.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
autoCreateRoutes_ = false;
exchangeSubnetRoutes_ = false;
exportCustomRoutes_ = false;
exportSubnetRoutesWithPublicIp_ = false;
importCustomRoutes_ = false;
importSubnetRoutesWithPublicIp_ = false;
name_ = "";
network_ = "";
peerMtu_ = 0;
stackType_ = "";
state_ = "";
stateDetails_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_NetworkPeering_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkPeering getDefaultInstanceForType() {
return com.google.cloud.compute.v1.NetworkPeering.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkPeering build() {
com.google.cloud.compute.v1.NetworkPeering result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkPeering buildPartial() {
com.google.cloud.compute.v1.NetworkPeering result =
new com.google.cloud.compute.v1.NetworkPeering(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.compute.v1.NetworkPeering result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.autoCreateRoutes_ = autoCreateRoutes_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.exchangeSubnetRoutes_ = exchangeSubnetRoutes_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.exportCustomRoutes_ = exportCustomRoutes_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.exportSubnetRoutesWithPublicIp_ = exportSubnetRoutesWithPublicIp_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.importCustomRoutes_ = importCustomRoutes_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.importSubnetRoutesWithPublicIp_ = importSubnetRoutesWithPublicIp_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.network_ = network_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.peerMtu_ = peerMtu_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.stackType_ = stackType_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.state_ = state_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.stateDetails_ = stateDetails_;
to_bitField0_ |= 0x00000800;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.NetworkPeering) {
return mergeFrom((com.google.cloud.compute.v1.NetworkPeering) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.NetworkPeering other) {
if (other == com.google.cloud.compute.v1.NetworkPeering.getDefaultInstance()) return this;
if (other.hasAutoCreateRoutes()) {
setAutoCreateRoutes(other.getAutoCreateRoutes());
}
if (other.hasExchangeSubnetRoutes()) {
setExchangeSubnetRoutes(other.getExchangeSubnetRoutes());
}
if (other.hasExportCustomRoutes()) {
setExportCustomRoutes(other.getExportCustomRoutes());
}
if (other.hasExportSubnetRoutesWithPublicIp()) {
setExportSubnetRoutesWithPublicIp(other.getExportSubnetRoutesWithPublicIp());
}
if (other.hasImportCustomRoutes()) {
setImportCustomRoutes(other.getImportCustomRoutes());
}
if (other.hasImportSubnetRoutesWithPublicIp()) {
setImportSubnetRoutesWithPublicIp(other.getImportSubnetRoutesWithPublicIp());
}
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000040;
onChanged();
}
if (other.hasNetwork()) {
network_ = other.network_;
bitField0_ |= 0x00000080;
onChanged();
}
if (other.hasPeerMtu()) {
setPeerMtu(other.getPeerMtu());
}
if (other.hasStackType()) {
stackType_ = other.stackType_;
bitField0_ |= 0x00000200;
onChanged();
}
if (other.hasState()) {
state_ = other.state_;
bitField0_ |= 0x00000400;
onChanged();
}
if (other.hasStateDetails()) {
stateDetails_ = other.stateDetails_;
bitField0_ |= 0x00000800;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 26989658:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 26989658
case 115357832:
{
importSubnetRoutesWithPublicIp_ = input.readBool();
bitField0_ |= 0x00000020;
break;
} // case 115357832
case 210578048:
{
exchangeSubnetRoutes_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 210578048
case 459639528:
{
autoCreateRoutes_ = input.readBool();
bitField0_ |= 0x00000001;
break;
} // case 459639528
case 482251880:
{
exportCustomRoutes_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case 482251880
case 556677768:
{
peerMtu_ = input.readInt32();
bitField0_ |= 0x00000100;
break;
} // case 556677768
case 764535970:
{
stateDetails_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000800;
break;
} // case 764535970
case 783526672:
{
exportSubnetRoutesWithPublicIp_ = input.readBool();
bitField0_ |= 0x00000008;
break;
} // case 783526672
case 878060682:
{
state_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000400;
break;
} // case 878060682
case 1583859184:
{
importCustomRoutes_ = input.readBool();
bitField0_ |= 0x00000010;
break;
} // case 1583859184
case 1862979954:
{
network_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 1862979954
case -887696246:
{
stackType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case -887696246
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private boolean autoCreateRoutes_;
/**
*
*
*
* This field will be deprecated soon. Use the exchange_subnet_routes field instead. Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool auto_create_routes = 57454941;
*
* @return Whether the autoCreateRoutes field is set.
*/
@java.lang.Override
public boolean hasAutoCreateRoutes() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* This field will be deprecated soon. Use the exchange_subnet_routes field instead. Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool auto_create_routes = 57454941;
*
* @return The autoCreateRoutes.
*/
@java.lang.Override
public boolean getAutoCreateRoutes() {
return autoCreateRoutes_;
}
/**
*
*
*
* This field will be deprecated soon. Use the exchange_subnet_routes field instead. Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool auto_create_routes = 57454941;
*
* @param value The autoCreateRoutes to set.
* @return This builder for chaining.
*/
public Builder setAutoCreateRoutes(boolean value) {
autoCreateRoutes_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* This field will be deprecated soon. Use the exchange_subnet_routes field instead. Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool auto_create_routes = 57454941;
*
* @return This builder for chaining.
*/
public Builder clearAutoCreateRoutes() {
bitField0_ = (bitField0_ & ~0x00000001);
autoCreateRoutes_ = false;
onChanged();
return this;
}
private boolean exchangeSubnetRoutes_;
/**
*
*
*
* Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool exchange_subnet_routes = 26322256;
*
* @return Whether the exchangeSubnetRoutes field is set.
*/
@java.lang.Override
public boolean hasExchangeSubnetRoutes() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool exchange_subnet_routes = 26322256;
*
* @return The exchangeSubnetRoutes.
*/
@java.lang.Override
public boolean getExchangeSubnetRoutes() {
return exchangeSubnetRoutes_;
}
/**
*
*
*
* Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool exchange_subnet_routes = 26322256;
*
* @param value The exchangeSubnetRoutes to set.
* @return This builder for chaining.
*/
public Builder setExchangeSubnetRoutes(boolean value) {
exchangeSubnetRoutes_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Indicates whether full mesh connectivity is created and managed automatically between peered networks. Currently this field should always be true since Google Compute Engine will automatically create and manage subnetwork routes between two networks when peering state is ACTIVE.
*
*
* optional bool exchange_subnet_routes = 26322256;
*
* @return This builder for chaining.
*/
public Builder clearExchangeSubnetRoutes() {
bitField0_ = (bitField0_ & ~0x00000002);
exchangeSubnetRoutes_ = false;
onChanged();
return this;
}
private boolean exportCustomRoutes_;
/**
*
*
*
* Whether to export the custom routes to peer network. The default value is false.
*
*
* optional bool export_custom_routes = 60281485;
*
* @return Whether the exportCustomRoutes field is set.
*/
@java.lang.Override
public boolean hasExportCustomRoutes() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Whether to export the custom routes to peer network. The default value is false.
*
*
* optional bool export_custom_routes = 60281485;
*
* @return The exportCustomRoutes.
*/
@java.lang.Override
public boolean getExportCustomRoutes() {
return exportCustomRoutes_;
}
/**
*
*
*
* Whether to export the custom routes to peer network. The default value is false.
*
*
* optional bool export_custom_routes = 60281485;
*
* @param value The exportCustomRoutes to set.
* @return This builder for chaining.
*/
public Builder setExportCustomRoutes(boolean value) {
exportCustomRoutes_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Whether to export the custom routes to peer network. The default value is false.
*
*
* optional bool export_custom_routes = 60281485;
*
* @return This builder for chaining.
*/
public Builder clearExportCustomRoutes() {
bitField0_ = (bitField0_ & ~0x00000004);
exportCustomRoutes_ = false;
onChanged();
return this;
}
private boolean exportSubnetRoutesWithPublicIp_;
/**
*
*
*
* Whether subnet routes with public IP range are exported. The default value is true, all subnet routes are exported. IPv4 special-use ranges are always exported to peers and are not controlled by this field.
*
*
* optional bool export_subnet_routes_with_public_ip = 97940834;
*
* @return Whether the exportSubnetRoutesWithPublicIp field is set.
*/
@java.lang.Override
public boolean hasExportSubnetRoutesWithPublicIp() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Whether subnet routes with public IP range are exported. The default value is true, all subnet routes are exported. IPv4 special-use ranges are always exported to peers and are not controlled by this field.
*
*
* optional bool export_subnet_routes_with_public_ip = 97940834;
*
* @return The exportSubnetRoutesWithPublicIp.
*/
@java.lang.Override
public boolean getExportSubnetRoutesWithPublicIp() {
return exportSubnetRoutesWithPublicIp_;
}
/**
*
*
*
* Whether subnet routes with public IP range are exported. The default value is true, all subnet routes are exported. IPv4 special-use ranges are always exported to peers and are not controlled by this field.
*
*
* optional bool export_subnet_routes_with_public_ip = 97940834;
*
* @param value The exportSubnetRoutesWithPublicIp to set.
* @return This builder for chaining.
*/
public Builder setExportSubnetRoutesWithPublicIp(boolean value) {
exportSubnetRoutesWithPublicIp_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Whether subnet routes with public IP range are exported. The default value is true, all subnet routes are exported. IPv4 special-use ranges are always exported to peers and are not controlled by this field.
*
*
* optional bool export_subnet_routes_with_public_ip = 97940834;
*
* @return This builder for chaining.
*/
public Builder clearExportSubnetRoutesWithPublicIp() {
bitField0_ = (bitField0_ & ~0x00000008);
exportSubnetRoutesWithPublicIp_ = false;
onChanged();
return this;
}
private boolean importCustomRoutes_;
/**
*
*
*
* Whether to import the custom routes from peer network. The default value is false.
*
*
* optional bool import_custom_routes = 197982398;
*
* @return Whether the importCustomRoutes field is set.
*/
@java.lang.Override
public boolean hasImportCustomRoutes() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Whether to import the custom routes from peer network. The default value is false.
*
*
* optional bool import_custom_routes = 197982398;
*
* @return The importCustomRoutes.
*/
@java.lang.Override
public boolean getImportCustomRoutes() {
return importCustomRoutes_;
}
/**
*
*
*
* Whether to import the custom routes from peer network. The default value is false.
*
*
* optional bool import_custom_routes = 197982398;
*
* @param value The importCustomRoutes to set.
* @return This builder for chaining.
*/
public Builder setImportCustomRoutes(boolean value) {
importCustomRoutes_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Whether to import the custom routes from peer network. The default value is false.
*
*
* optional bool import_custom_routes = 197982398;
*
* @return This builder for chaining.
*/
public Builder clearImportCustomRoutes() {
bitField0_ = (bitField0_ & ~0x00000010);
importCustomRoutes_ = false;
onChanged();
return this;
}
private boolean importSubnetRoutesWithPublicIp_;
/**
*
*
*
* Whether subnet routes with public IP range are imported. The default value is false. IPv4 special-use ranges are always imported from peers and are not controlled by this field.
*
*
* optional bool import_subnet_routes_with_public_ip = 14419729;
*
* @return Whether the importSubnetRoutesWithPublicIp field is set.
*/
@java.lang.Override
public boolean hasImportSubnetRoutesWithPublicIp() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Whether subnet routes with public IP range are imported. The default value is false. IPv4 special-use ranges are always imported from peers and are not controlled by this field.
*
*
* optional bool import_subnet_routes_with_public_ip = 14419729;
*
* @return The importSubnetRoutesWithPublicIp.
*/
@java.lang.Override
public boolean getImportSubnetRoutesWithPublicIp() {
return importSubnetRoutesWithPublicIp_;
}
/**
*
*
*
* Whether subnet routes with public IP range are imported. The default value is false. IPv4 special-use ranges are always imported from peers and are not controlled by this field.
*
*
* optional bool import_subnet_routes_with_public_ip = 14419729;
*
* @param value The importSubnetRoutesWithPublicIp to set.
* @return This builder for chaining.
*/
public Builder setImportSubnetRoutesWithPublicIp(boolean value) {
importSubnetRoutesWithPublicIp_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Whether subnet routes with public IP range are imported. The default value is false. IPv4 special-use ranges are always imported from peers and are not controlled by this field.
*
*
* optional bool import_subnet_routes_with_public_ip = 14419729;
*
* @return This builder for chaining.
*/
public Builder clearImportSubnetRoutesWithPublicIp() {
bitField0_ = (bitField0_ & ~0x00000020);
importSubnetRoutesWithPublicIp_ = false;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
*
*
* Name of this peering. Provided by the client when the peering is created. The name must comply with RFC1035. Specifically, the name must be 1-63 characters long and match regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be a lowercase letter, and all the following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Name of this peering. Provided by the client when the peering is created. The name must comply with RFC1035. Specifically, the name must be 1-63 characters long and match regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be a lowercase letter, and all the following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Name of this peering. Provided by the client when the peering is created. The name must comply with RFC1035. Specifically, the name must be 1-63 characters long and match regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be a lowercase letter, and all the following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Name of this peering. Provided by the client when the peering is created. The name must comply with RFC1035. Specifically, the name must be 1-63 characters long and match regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be a lowercase letter, and all the following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Name of this peering. Provided by the client when the peering is created. The name must comply with RFC1035. Specifically, the name must be 1-63 characters long and match regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be a lowercase letter, and all the following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* Name of this peering. Provided by the client when the peering is created. The name must comply with RFC1035. Specifically, the name must be 1-63 characters long and match regular expression `[a-z]([-a-z0-9]*[a-z0-9])?`. The first character must be a lowercase letter, and all the following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object network_ = "";
/**
*
*
*
* The URL of the peer network. It can be either full URL or partial URL. The peer network may belong to a different project. If the partial URL does not contain project, it is assumed that the peer network is in the same project as the current network.
*
*
* optional string network = 232872494;
*
* @return Whether the network field is set.
*/
public boolean hasNetwork() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The URL of the peer network. It can be either full URL or partial URL. The peer network may belong to a different project. If the partial URL does not contain project, it is assumed that the peer network is in the same project as the current network.
*
*
* optional string network = 232872494;
*
* @return The network.
*/
public java.lang.String getNetwork() {
java.lang.Object ref = network_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
network_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The URL of the peer network. It can be either full URL or partial URL. The peer network may belong to a different project. If the partial URL does not contain project, it is assumed that the peer network is in the same project as the current network.
*
*
* optional string network = 232872494;
*
* @return The bytes for network.
*/
public com.google.protobuf.ByteString getNetworkBytes() {
java.lang.Object ref = network_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
network_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The URL of the peer network. It can be either full URL or partial URL. The peer network may belong to a different project. If the partial URL does not contain project, it is assumed that the peer network is in the same project as the current network.
*
*
* optional string network = 232872494;
*
* @param value The network to set.
* @return This builder for chaining.
*/
public Builder setNetwork(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
network_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* The URL of the peer network. It can be either full URL or partial URL. The peer network may belong to a different project. If the partial URL does not contain project, it is assumed that the peer network is in the same project as the current network.
*
*
* optional string network = 232872494;
*
* @return This builder for chaining.
*/
public Builder clearNetwork() {
network_ = getDefaultInstance().getNetwork();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
*
*
* The URL of the peer network. It can be either full URL or partial URL. The peer network may belong to a different project. If the partial URL does not contain project, it is assumed that the peer network is in the same project as the current network.
*
*
* optional string network = 232872494;
*
* @param value The bytes for network to set.
* @return This builder for chaining.
*/
public Builder setNetworkBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
network_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private int peerMtu_;
/**
*
*
*
* Maximum Transmission Unit in bytes.
*
*
* optional int32 peer_mtu = 69584721;
*
* @return Whether the peerMtu field is set.
*/
@java.lang.Override
public boolean hasPeerMtu() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Maximum Transmission Unit in bytes.
*
*
* optional int32 peer_mtu = 69584721;
*
* @return The peerMtu.
*/
@java.lang.Override
public int getPeerMtu() {
return peerMtu_;
}
/**
*
*
*
* Maximum Transmission Unit in bytes.
*
*
* optional int32 peer_mtu = 69584721;
*
* @param value The peerMtu to set.
* @return This builder for chaining.
*/
public Builder setPeerMtu(int value) {
peerMtu_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Maximum Transmission Unit in bytes.
*
*
* optional int32 peer_mtu = 69584721;
*
* @return This builder for chaining.
*/
public Builder clearPeerMtu() {
bitField0_ = (bitField0_ & ~0x00000100);
peerMtu_ = 0;
onChanged();
return this;
}
private java.lang.Object stackType_ = "";
/**
*
*
*
* Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return Whether the stackType field is set.
*/
public boolean hasStackType() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The stackType.
*/
public java.lang.String getStackType() {
java.lang.Object ref = stackType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stackType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The bytes for stackType.
*/
public com.google.protobuf.ByteString getStackTypeBytes() {
java.lang.Object ref = stackType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
stackType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @param value The stackType to set.
* @return This builder for chaining.
*/
public Builder setStackType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stackType_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return This builder for chaining.
*/
public Builder clearStackType() {
stackType_ = getDefaultInstance().getStackType();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
*
*
*
* Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @param value The bytes for stackType to set.
* @return This builder for chaining.
*/
public Builder setStackTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stackType_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
private java.lang.Object state_ = "";
/**
*
*
*
* [Output Only] State for the peering, either `ACTIVE` or `INACTIVE`. The peering is `ACTIVE` when there's a matching configuration in the peer network.
* Check the State enum for the list of possible values.
*
*
* optional string state = 109757585;
*
* @return Whether the state field is set.
*/
public boolean hasState() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* [Output Only] State for the peering, either `ACTIVE` or `INACTIVE`. The peering is `ACTIVE` when there's a matching configuration in the peer network.
* Check the State enum for the list of possible values.
*
*
* optional string state = 109757585;
*
* @return The state.
*/
public java.lang.String getState() {
java.lang.Object ref = state_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
state_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] State for the peering, either `ACTIVE` or `INACTIVE`. The peering is `ACTIVE` when there's a matching configuration in the peer network.
* Check the State enum for the list of possible values.
*
*
* optional string state = 109757585;
*
* @return The bytes for state.
*/
public com.google.protobuf.ByteString getStateBytes() {
java.lang.Object ref = state_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
state_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] State for the peering, either `ACTIVE` or `INACTIVE`. The peering is `ACTIVE` when there's a matching configuration in the peer network.
* Check the State enum for the list of possible values.
*
*
* optional string state = 109757585;
*
* @param value The state to set.
* @return This builder for chaining.
*/
public Builder setState(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
state_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] State for the peering, either `ACTIVE` or `INACTIVE`. The peering is `ACTIVE` when there's a matching configuration in the peer network.
* Check the State enum for the list of possible values.
*
*
* optional string state = 109757585;
*
* @return This builder for chaining.
*/
public Builder clearState() {
state_ = getDefaultInstance().getState();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] State for the peering, either `ACTIVE` or `INACTIVE`. The peering is `ACTIVE` when there's a matching configuration in the peer network.
* Check the State enum for the list of possible values.
*
*
* optional string state = 109757585;
*
* @param value The bytes for state to set.
* @return This builder for chaining.
*/
public Builder setStateBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
state_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
private java.lang.Object stateDetails_ = "";
/**
*
*
*
* [Output Only] Details about the current state of the peering.
*
*
* optional string state_details = 95566996;
*
* @return Whether the stateDetails field is set.
*/
public boolean hasStateDetails() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* [Output Only] Details about the current state of the peering.
*
*
* optional string state_details = 95566996;
*
* @return The stateDetails.
*/
public java.lang.String getStateDetails() {
java.lang.Object ref = stateDetails_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stateDetails_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Details about the current state of the peering.
*
*
* optional string state_details = 95566996;
*
* @return The bytes for stateDetails.
*/
public com.google.protobuf.ByteString getStateDetailsBytes() {
java.lang.Object ref = stateDetails_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
stateDetails_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Details about the current state of the peering.
*
*
* optional string state_details = 95566996;
*
* @param value The stateDetails to set.
* @return This builder for chaining.
*/
public Builder setStateDetails(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stateDetails_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Details about the current state of the peering.
*
*
* optional string state_details = 95566996;
*
* @return This builder for chaining.
*/
public Builder clearStateDetails() {
stateDetails_ = getDefaultInstance().getStateDetails();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Details about the current state of the peering.
*
*
* optional string state_details = 95566996;
*
* @param value The bytes for stateDetails to set.
* @return This builder for chaining.
*/
public Builder setStateDetailsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stateDetails_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.NetworkPeering)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.NetworkPeering)
private static final com.google.cloud.compute.v1.NetworkPeering DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.NetworkPeering();
}
public static com.google.cloud.compute.v1.NetworkPeering getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NetworkPeering parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.NetworkPeering getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy