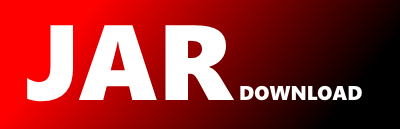
com.google.cloud.compute.v1.Reservation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* Represents a reservation resource. A reservation ensures that capacity is held in a specific zone even if the reserved VMs are not running. For more information, read Reserving zonal resources.
*
*
* Protobuf type {@code google.cloud.compute.v1.Reservation}
*/
public final class Reservation extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.Reservation)
ReservationOrBuilder {
private static final long serialVersionUID = 0L;
// Use Reservation.newBuilder() to construct.
private Reservation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Reservation() {
commitment_ = "";
creationTimestamp_ = "";
description_ = "";
kind_ = "";
name_ = "";
selfLink_ = "";
status_ = "";
zone_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Reservation();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Reservation_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 22220385:
return internalGetResourcePolicies();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Reservation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.Reservation.class,
com.google.cloud.compute.v1.Reservation.Builder.class);
}
/**
*
*
*
* [Output Only] The status of the reservation. - CREATING: Reservation resources are being allocated. - READY: Reservation resources have been allocated, and the reservation is ready for use. - DELETING: Reservation deletion is in progress. - UPDATING: Reservation update is in progress.
*
*
* Protobuf enum {@code google.cloud.compute.v1.Reservation.Status}
*/
public enum Status implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STATUS = 0;
*/
UNDEFINED_STATUS(0),
/**
*
*
*
* Reservation resources are being allocated.
*
*
* CREATING = 455564985;
*/
CREATING(455564985),
/**
*
*
*
* Reservation deletion is in progress.
*
*
* DELETING = 528602024;
*/
DELETING(528602024),
/** INVALID = 530283991;
*/
INVALID(530283991),
/**
*
*
*
* Reservation resources have been allocated, and the reservation is ready for use.
*
*
* READY = 77848963;
*/
READY(77848963),
/**
*
*
*
* Reservation update is in progress.
*
*
* UPDATING = 494614342;
*/
UPDATING(494614342),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STATUS = 0;
*/
public static final int UNDEFINED_STATUS_VALUE = 0;
/**
*
*
*
* Reservation resources are being allocated.
*
*
* CREATING = 455564985;
*/
public static final int CREATING_VALUE = 455564985;
/**
*
*
*
* Reservation deletion is in progress.
*
*
* DELETING = 528602024;
*/
public static final int DELETING_VALUE = 528602024;
/** INVALID = 530283991;
*/
public static final int INVALID_VALUE = 530283991;
/**
*
*
*
* Reservation resources have been allocated, and the reservation is ready for use.
*
*
* READY = 77848963;
*/
public static final int READY_VALUE = 77848963;
/**
*
*
*
* Reservation update is in progress.
*
*
* UPDATING = 494614342;
*/
public static final int UPDATING_VALUE = 494614342;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Status valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Status forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_STATUS;
case 455564985:
return CREATING;
case 528602024:
return DELETING;
case 530283991:
return INVALID;
case 77848963:
return READY;
case 494614342:
return UPDATING;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Status findValueByNumber(int number) {
return Status.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.Reservation.getDescriptor().getEnumTypes().get(0);
}
private static final Status[] VALUES = values();
public static Status valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Status(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.Reservation.Status)
}
private int bitField0_;
public static final int AGGREGATE_RESERVATION_FIELD_NUMBER = 291567948;
private com.google.cloud.compute.v1.AllocationAggregateReservation aggregateReservation_;
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*
* @return Whether the aggregateReservation field is set.
*/
@java.lang.Override
public boolean hasAggregateReservation() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*
* @return The aggregateReservation.
*/
@java.lang.Override
public com.google.cloud.compute.v1.AllocationAggregateReservation getAggregateReservation() {
return aggregateReservation_ == null
? com.google.cloud.compute.v1.AllocationAggregateReservation.getDefaultInstance()
: aggregateReservation_;
}
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.AllocationAggregateReservationOrBuilder
getAggregateReservationOrBuilder() {
return aggregateReservation_ == null
? com.google.cloud.compute.v1.AllocationAggregateReservation.getDefaultInstance()
: aggregateReservation_;
}
public static final int COMMITMENT_FIELD_NUMBER = 482134805;
@SuppressWarnings("serial")
private volatile java.lang.Object commitment_ = "";
/**
*
*
*
* [Output Only] Full or partial URL to a parent commitment. This field displays for reservations that are tied to a commitment.
*
*
* optional string commitment = 482134805;
*
* @return Whether the commitment field is set.
*/
@java.lang.Override
public boolean hasCommitment() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* [Output Only] Full or partial URL to a parent commitment. This field displays for reservations that are tied to a commitment.
*
*
* optional string commitment = 482134805;
*
* @return The commitment.
*/
@java.lang.Override
public java.lang.String getCommitment() {
java.lang.Object ref = commitment_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
commitment_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Full or partial URL to a parent commitment. This field displays for reservations that are tied to a commitment.
*
*
* optional string commitment = 482134805;
*
* @return The bytes for commitment.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCommitmentBytes() {
java.lang.Object ref = commitment_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
commitment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CREATION_TIMESTAMP_FIELD_NUMBER = 30525366;
@SuppressWarnings("serial")
private volatile java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
@java.lang.Override
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
@java.lang.Override
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 422937596;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 3355;
private long id_ = 0L;
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int KIND_FIELD_NUMBER = 3292052;
@SuppressWarnings("serial")
private volatile java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#reservations for reservations.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
@java.lang.Override
public boolean hasKind() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#reservations for reservations.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
@java.lang.Override
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#reservations for reservations.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 3373707;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* The name of the resource, provided by the client when initially creating the resource. The resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* The name of the resource, provided by the client when initially creating the resource. The resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* The name of the resource, provided by the client when initially creating the resource. The resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RESOURCE_POLICIES_FIELD_NUMBER = 22220385;
private static final class ResourcePoliciesDefaultEntryHolder {
static final com.google.protobuf.MapEntry defaultEntry =
com.google.protobuf.MapEntry.newDefaultInstance(
com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Reservation_ResourcePoliciesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField resourcePolicies_;
private com.google.protobuf.MapField
internalGetResourcePolicies() {
if (resourcePolicies_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ResourcePoliciesDefaultEntryHolder.defaultEntry);
}
return resourcePolicies_;
}
public int getResourcePoliciesCount() {
return internalGetResourcePolicies().getMap().size();
}
/**
*
*
*
* Resource policies to be added to this reservation. The key is defined by user, and the value is resource policy url. This is to define placement policy with reservation.
*
*
* map<string, string> resource_policies = 22220385;
*/
@java.lang.Override
public boolean containsResourcePolicies(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetResourcePolicies().getMap().containsKey(key);
}
/** Use {@link #getResourcePoliciesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getResourcePolicies() {
return getResourcePoliciesMap();
}
/**
*
*
*
* Resource policies to be added to this reservation. The key is defined by user, and the value is resource policy url. This is to define placement policy with reservation.
*
*
* map<string, string> resource_policies = 22220385;
*/
@java.lang.Override
public java.util.Map getResourcePoliciesMap() {
return internalGetResourcePolicies().getMap();
}
/**
*
*
*
* Resource policies to be added to this reservation. The key is defined by user, and the value is resource policy url. This is to define placement policy with reservation.
*
*
* map<string, string> resource_policies = 22220385;
*/
@java.lang.Override
public /* nullable */ java.lang.String getResourcePoliciesOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetResourcePolicies().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Resource policies to be added to this reservation. The key is defined by user, and the value is resource policy url. This is to define placement policy with reservation.
*
*
* map<string, string> resource_policies = 22220385;
*/
@java.lang.Override
public java.lang.String getResourcePoliciesOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetResourcePolicies().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int RESOURCE_STATUS_FIELD_NUMBER = 249429315;
private com.google.cloud.compute.v1.AllocationResourceStatus resourceStatus_;
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*
* @return Whether the resourceStatus field is set.
*/
@java.lang.Override
public boolean hasResourceStatus() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*
* @return The resourceStatus.
*/
@java.lang.Override
public com.google.cloud.compute.v1.AllocationResourceStatus getResourceStatus() {
return resourceStatus_ == null
? com.google.cloud.compute.v1.AllocationResourceStatus.getDefaultInstance()
: resourceStatus_;
}
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.AllocationResourceStatusOrBuilder
getResourceStatusOrBuilder() {
return resourceStatus_ == null
? com.google.cloud.compute.v1.AllocationResourceStatus.getDefaultInstance()
: resourceStatus_;
}
public static final int SATISFIES_PZS_FIELD_NUMBER = 480964267;
private boolean satisfiesPzs_ = false;
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return Whether the satisfiesPzs field is set.
*/
@java.lang.Override
public boolean hasSatisfiesPzs() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return The satisfiesPzs.
*/
@java.lang.Override
public boolean getSatisfiesPzs() {
return satisfiesPzs_;
}
public static final int SELF_LINK_FIELD_NUMBER = 456214797;
@SuppressWarnings("serial")
private volatile java.lang.Object selfLink_ = "";
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return Whether the selfLink field is set.
*/
@java.lang.Override
public boolean hasSelfLink() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return The selfLink.
*/
@java.lang.Override
public java.lang.String getSelfLink() {
java.lang.Object ref = selfLink_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLink_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return The bytes for selfLink.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSelfLinkBytes() {
java.lang.Object ref = selfLink_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLink_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SHARE_SETTINGS_FIELD_NUMBER = 266668163;
private com.google.cloud.compute.v1.ShareSettings shareSettings_;
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*
* @return Whether the shareSettings field is set.
*/
@java.lang.Override
public boolean hasShareSettings() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*
* @return The shareSettings.
*/
@java.lang.Override
public com.google.cloud.compute.v1.ShareSettings getShareSettings() {
return shareSettings_ == null
? com.google.cloud.compute.v1.ShareSettings.getDefaultInstance()
: shareSettings_;
}
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*/
@java.lang.Override
public com.google.cloud.compute.v1.ShareSettingsOrBuilder getShareSettingsOrBuilder() {
return shareSettings_ == null
? com.google.cloud.compute.v1.ShareSettings.getDefaultInstance()
: shareSettings_;
}
public static final int SPECIFIC_RESERVATION_FIELD_NUMBER = 404901951;
private com.google.cloud.compute.v1.AllocationSpecificSKUReservation specificReservation_;
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*
* @return Whether the specificReservation field is set.
*/
@java.lang.Override
public boolean hasSpecificReservation() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*
* @return The specificReservation.
*/
@java.lang.Override
public com.google.cloud.compute.v1.AllocationSpecificSKUReservation getSpecificReservation() {
return specificReservation_ == null
? com.google.cloud.compute.v1.AllocationSpecificSKUReservation.getDefaultInstance()
: specificReservation_;
}
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.AllocationSpecificSKUReservationOrBuilder
getSpecificReservationOrBuilder() {
return specificReservation_ == null
? com.google.cloud.compute.v1.AllocationSpecificSKUReservation.getDefaultInstance()
: specificReservation_;
}
public static final int SPECIFIC_RESERVATION_REQUIRED_FIELD_NUMBER = 226550687;
private boolean specificReservationRequired_ = false;
/**
*
*
*
* Indicates whether the reservation can be consumed by VMs with affinity for "any" reservation. If the field is set, then only VMs that target the reservation by name can consume from this reservation.
*
*
* optional bool specific_reservation_required = 226550687;
*
* @return Whether the specificReservationRequired field is set.
*/
@java.lang.Override
public boolean hasSpecificReservationRequired() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* Indicates whether the reservation can be consumed by VMs with affinity for "any" reservation. If the field is set, then only VMs that target the reservation by name can consume from this reservation.
*
*
* optional bool specific_reservation_required = 226550687;
*
* @return The specificReservationRequired.
*/
@java.lang.Override
public boolean getSpecificReservationRequired() {
return specificReservationRequired_;
}
public static final int STATUS_FIELD_NUMBER = 181260274;
@SuppressWarnings("serial")
private volatile java.lang.Object status_ = "";
/**
*
*
*
* [Output Only] The status of the reservation. - CREATING: Reservation resources are being allocated. - READY: Reservation resources have been allocated, and the reservation is ready for use. - DELETING: Reservation deletion is in progress. - UPDATING: Reservation update is in progress.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*
*
* [Output Only] The status of the reservation. - CREATING: Reservation resources are being allocated. - READY: Reservation resources have been allocated, and the reservation is ready for use. - DELETING: Reservation deletion is in progress. - UPDATING: Reservation update is in progress.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The status.
*/
@java.lang.Override
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
status_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The status of the reservation. - CREATING: Reservation resources are being allocated. - READY: Reservation resources have been allocated, and the reservation is ready for use. - DELETING: Reservation deletion is in progress. - UPDATING: Reservation update is in progress.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The bytes for status.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ZONE_FIELD_NUMBER = 3744684;
@SuppressWarnings("serial")
private volatile java.lang.Object zone_ = "";
/**
*
*
*
* Zone in which the reservation resides. A zone must be provided if the reservation is created within a commitment.
*
*
* optional string zone = 3744684;
*
* @return Whether the zone field is set.
*/
@java.lang.Override
public boolean hasZone() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*
*
* Zone in which the reservation resides. A zone must be provided if the reservation is created within a commitment.
*
*
* optional string zone = 3744684;
*
* @return The zone.
*/
@java.lang.Override
public java.lang.String getZone() {
java.lang.Object ref = zone_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
zone_ = s;
return s;
}
}
/**
*
*
*
* Zone in which the reservation resides. A zone must be provided if the reservation is created within a commitment.
*
*
* optional string zone = 3744684;
*
* @return The bytes for zone.
*/
@java.lang.Override
public com.google.protobuf.ByteString getZoneBytes() {
java.lang.Object ref = zone_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
zone_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000010) != 0)) {
output.writeUInt64(3355, id_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3292052, kind_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3373707, name_);
}
if (((bitField0_ & 0x00004000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3744684, zone_);
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output,
internalGetResourcePolicies(),
ResourcePoliciesDefaultEntryHolder.defaultEntry,
22220385);
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30525366, creationTimestamp_);
}
if (((bitField0_ & 0x00002000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 181260274, status_);
}
if (((bitField0_ & 0x00001000) != 0)) {
output.writeBool(226550687, specificReservationRequired_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeMessage(249429315, getResourceStatus());
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeMessage(266668163, getShareSettings());
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(291567948, getAggregateReservation());
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeMessage(404901951, getSpecificReservation());
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 422937596, description_);
}
if (((bitField0_ & 0x00000200) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 456214797, selfLink_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeBool(480964267, satisfiesPzs_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 482134805, commitment_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeUInt64Size(3355, id_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3292052, kind_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3373707, name_);
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3744684, zone_);
}
for (java.util.Map.Entry entry :
internalGetResourcePolicies().getMap().entrySet()) {
com.google.protobuf.MapEntry resourcePolicies__ =
ResourcePoliciesDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(22220385, resourcePolicies__);
}
if (((bitField0_ & 0x00000004) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(30525366, creationTimestamp_);
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(181260274, status_);
}
if (((bitField0_ & 0x00001000) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeBoolSize(
226550687, specificReservationRequired_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(249429315, getResourceStatus());
}
if (((bitField0_ & 0x00000400) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(266668163, getShareSettings());
}
if (((bitField0_ & 0x00000001) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
291567948, getAggregateReservation());
}
if (((bitField0_ & 0x00000800) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
404901951, getSpecificReservation());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(422937596, description_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(456214797, selfLink_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(480964267, satisfiesPzs_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(482134805, commitment_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.Reservation)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.Reservation other = (com.google.cloud.compute.v1.Reservation) obj;
if (hasAggregateReservation() != other.hasAggregateReservation()) return false;
if (hasAggregateReservation()) {
if (!getAggregateReservation().equals(other.getAggregateReservation())) return false;
}
if (hasCommitment() != other.hasCommitment()) return false;
if (hasCommitment()) {
if (!getCommitment().equals(other.getCommitment())) return false;
}
if (hasCreationTimestamp() != other.hasCreationTimestamp()) return false;
if (hasCreationTimestamp()) {
if (!getCreationTimestamp().equals(other.getCreationTimestamp())) return false;
}
if (hasDescription() != other.hasDescription()) return false;
if (hasDescription()) {
if (!getDescription().equals(other.getDescription())) return false;
}
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId() != other.getId()) return false;
}
if (hasKind() != other.hasKind()) return false;
if (hasKind()) {
if (!getKind().equals(other.getKind())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName().equals(other.getName())) return false;
}
if (!internalGetResourcePolicies().equals(other.internalGetResourcePolicies())) return false;
if (hasResourceStatus() != other.hasResourceStatus()) return false;
if (hasResourceStatus()) {
if (!getResourceStatus().equals(other.getResourceStatus())) return false;
}
if (hasSatisfiesPzs() != other.hasSatisfiesPzs()) return false;
if (hasSatisfiesPzs()) {
if (getSatisfiesPzs() != other.getSatisfiesPzs()) return false;
}
if (hasSelfLink() != other.hasSelfLink()) return false;
if (hasSelfLink()) {
if (!getSelfLink().equals(other.getSelfLink())) return false;
}
if (hasShareSettings() != other.hasShareSettings()) return false;
if (hasShareSettings()) {
if (!getShareSettings().equals(other.getShareSettings())) return false;
}
if (hasSpecificReservation() != other.hasSpecificReservation()) return false;
if (hasSpecificReservation()) {
if (!getSpecificReservation().equals(other.getSpecificReservation())) return false;
}
if (hasSpecificReservationRequired() != other.hasSpecificReservationRequired()) return false;
if (hasSpecificReservationRequired()) {
if (getSpecificReservationRequired() != other.getSpecificReservationRequired()) return false;
}
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus().equals(other.getStatus())) return false;
}
if (hasZone() != other.hasZone()) return false;
if (hasZone()) {
if (!getZone().equals(other.getZone())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAggregateReservation()) {
hash = (37 * hash) + AGGREGATE_RESERVATION_FIELD_NUMBER;
hash = (53 * hash) + getAggregateReservation().hashCode();
}
if (hasCommitment()) {
hash = (37 * hash) + COMMITMENT_FIELD_NUMBER;
hash = (53 * hash) + getCommitment().hashCode();
}
if (hasCreationTimestamp()) {
hash = (37 * hash) + CREATION_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getCreationTimestamp().hashCode();
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getId());
}
if (hasKind()) {
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + getKind().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (!internalGetResourcePolicies().getMap().isEmpty()) {
hash = (37 * hash) + RESOURCE_POLICIES_FIELD_NUMBER;
hash = (53 * hash) + internalGetResourcePolicies().hashCode();
}
if (hasResourceStatus()) {
hash = (37 * hash) + RESOURCE_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getResourceStatus().hashCode();
}
if (hasSatisfiesPzs()) {
hash = (37 * hash) + SATISFIES_PZS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getSatisfiesPzs());
}
if (hasSelfLink()) {
hash = (37 * hash) + SELF_LINK_FIELD_NUMBER;
hash = (53 * hash) + getSelfLink().hashCode();
}
if (hasShareSettings()) {
hash = (37 * hash) + SHARE_SETTINGS_FIELD_NUMBER;
hash = (53 * hash) + getShareSettings().hashCode();
}
if (hasSpecificReservation()) {
hash = (37 * hash) + SPECIFIC_RESERVATION_FIELD_NUMBER;
hash = (53 * hash) + getSpecificReservation().hashCode();
}
if (hasSpecificReservationRequired()) {
hash = (37 * hash) + SPECIFIC_RESERVATION_REQUIRED_FIELD_NUMBER;
hash =
(53 * hash) + com.google.protobuf.Internal.hashBoolean(getSpecificReservationRequired());
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (hasZone()) {
hash = (37 * hash) + ZONE_FIELD_NUMBER;
hash = (53 * hash) + getZone().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.Reservation parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Reservation parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Reservation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Reservation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Reservation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Reservation parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Reservation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Reservation parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.Reservation parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Reservation parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.Reservation parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Reservation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.Reservation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Represents a reservation resource. A reservation ensures that capacity is held in a specific zone even if the reserved VMs are not running. For more information, read Reserving zonal resources.
*
*
* Protobuf type {@code google.cloud.compute.v1.Reservation}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.Reservation)
com.google.cloud.compute.v1.ReservationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Reservation_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 22220385:
return internalGetResourcePolicies();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 22220385:
return internalGetMutableResourcePolicies();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Reservation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.Reservation.class,
com.google.cloud.compute.v1.Reservation.Builder.class);
}
// Construct using com.google.cloud.compute.v1.Reservation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getAggregateReservationFieldBuilder();
getResourceStatusFieldBuilder();
getShareSettingsFieldBuilder();
getSpecificReservationFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
aggregateReservation_ = null;
if (aggregateReservationBuilder_ != null) {
aggregateReservationBuilder_.dispose();
aggregateReservationBuilder_ = null;
}
commitment_ = "";
creationTimestamp_ = "";
description_ = "";
id_ = 0L;
kind_ = "";
name_ = "";
internalGetMutableResourcePolicies().clear();
resourceStatus_ = null;
if (resourceStatusBuilder_ != null) {
resourceStatusBuilder_.dispose();
resourceStatusBuilder_ = null;
}
satisfiesPzs_ = false;
selfLink_ = "";
shareSettings_ = null;
if (shareSettingsBuilder_ != null) {
shareSettingsBuilder_.dispose();
shareSettingsBuilder_ = null;
}
specificReservation_ = null;
if (specificReservationBuilder_ != null) {
specificReservationBuilder_.dispose();
specificReservationBuilder_ = null;
}
specificReservationRequired_ = false;
status_ = "";
zone_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Reservation_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.Reservation getDefaultInstanceForType() {
return com.google.cloud.compute.v1.Reservation.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.Reservation build() {
com.google.cloud.compute.v1.Reservation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.Reservation buildPartial() {
com.google.cloud.compute.v1.Reservation result =
new com.google.cloud.compute.v1.Reservation(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.compute.v1.Reservation result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.aggregateReservation_ =
aggregateReservationBuilder_ == null
? aggregateReservation_
: aggregateReservationBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.commitment_ = commitment_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.creationTimestamp_ = creationTimestamp_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.description_ = description_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.kind_ = kind_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.resourcePolicies_ = internalGetResourcePolicies();
result.resourcePolicies_.makeImmutable();
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.resourceStatus_ =
resourceStatusBuilder_ == null ? resourceStatus_ : resourceStatusBuilder_.build();
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.satisfiesPzs_ = satisfiesPzs_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.selfLink_ = selfLink_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.shareSettings_ =
shareSettingsBuilder_ == null ? shareSettings_ : shareSettingsBuilder_.build();
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.specificReservation_ =
specificReservationBuilder_ == null
? specificReservation_
: specificReservationBuilder_.build();
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.specificReservationRequired_ = specificReservationRequired_;
to_bitField0_ |= 0x00001000;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.status_ = status_;
to_bitField0_ |= 0x00002000;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.zone_ = zone_;
to_bitField0_ |= 0x00004000;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.Reservation) {
return mergeFrom((com.google.cloud.compute.v1.Reservation) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.Reservation other) {
if (other == com.google.cloud.compute.v1.Reservation.getDefaultInstance()) return this;
if (other.hasAggregateReservation()) {
mergeAggregateReservation(other.getAggregateReservation());
}
if (other.hasCommitment()) {
commitment_ = other.commitment_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasCreationTimestamp()) {
creationTimestamp_ = other.creationTimestamp_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasDescription()) {
description_ = other.description_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasKind()) {
kind_ = other.kind_;
bitField0_ |= 0x00000020;
onChanged();
}
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000040;
onChanged();
}
internalGetMutableResourcePolicies().mergeFrom(other.internalGetResourcePolicies());
bitField0_ |= 0x00000080;
if (other.hasResourceStatus()) {
mergeResourceStatus(other.getResourceStatus());
}
if (other.hasSatisfiesPzs()) {
setSatisfiesPzs(other.getSatisfiesPzs());
}
if (other.hasSelfLink()) {
selfLink_ = other.selfLink_;
bitField0_ |= 0x00000400;
onChanged();
}
if (other.hasShareSettings()) {
mergeShareSettings(other.getShareSettings());
}
if (other.hasSpecificReservation()) {
mergeSpecificReservation(other.getSpecificReservation());
}
if (other.hasSpecificReservationRequired()) {
setSpecificReservationRequired(other.getSpecificReservationRequired());
}
if (other.hasStatus()) {
status_ = other.status_;
bitField0_ |= 0x00004000;
onChanged();
}
if (other.hasZone()) {
zone_ = other.zone_;
bitField0_ |= 0x00008000;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 26840:
{
id_ = input.readUInt64();
bitField0_ |= 0x00000010;
break;
} // case 26840
case 26336418:
{
kind_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 26336418
case 26989658:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 26989658
case 29957474:
{
zone_ = input.readStringRequireUtf8();
bitField0_ |= 0x00008000;
break;
} // case 29957474
case 177763082:
{
com.google.protobuf.MapEntry
resourcePolicies__ =
input.readMessage(
ResourcePoliciesDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableResourcePolicies()
.getMutableMap()
.put(resourcePolicies__.getKey(), resourcePolicies__.getValue());
bitField0_ |= 0x00000080;
break;
} // case 177763082
case 244202930:
{
creationTimestamp_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 244202930
case 1450082194:
{
status_ = input.readStringRequireUtf8();
bitField0_ |= 0x00004000;
break;
} // case 1450082194
case 1812405496:
{
specificReservationRequired_ = input.readBool();
bitField0_ |= 0x00002000;
break;
} // case 1812405496
case 1995434522:
{
input.readMessage(getResourceStatusFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 1995434522
case 2133345306:
{
input.readMessage(getShareSettingsFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000800;
break;
} // case 2133345306
case -1962423710:
{
input.readMessage(
getAggregateReservationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case -1962423710
case -1055751686:
{
input.readMessage(
getSpecificReservationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00001000;
break;
} // case -1055751686
case -911466526:
{
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case -911466526
case -645248918:
{
selfLink_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000400;
break;
} // case -645248918
case -447253160:
{
satisfiesPzs_ = input.readBool();
bitField0_ |= 0x00000200;
break;
} // case -447253160
case -437888854:
{
commitment_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case -437888854
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.cloud.compute.v1.AllocationAggregateReservation aggregateReservation_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AllocationAggregateReservation,
com.google.cloud.compute.v1.AllocationAggregateReservation.Builder,
com.google.cloud.compute.v1.AllocationAggregateReservationOrBuilder>
aggregateReservationBuilder_;
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*
* @return Whether the aggregateReservation field is set.
*/
public boolean hasAggregateReservation() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*
* @return The aggregateReservation.
*/
public com.google.cloud.compute.v1.AllocationAggregateReservation getAggregateReservation() {
if (aggregateReservationBuilder_ == null) {
return aggregateReservation_ == null
? com.google.cloud.compute.v1.AllocationAggregateReservation.getDefaultInstance()
: aggregateReservation_;
} else {
return aggregateReservationBuilder_.getMessage();
}
}
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*/
public Builder setAggregateReservation(
com.google.cloud.compute.v1.AllocationAggregateReservation value) {
if (aggregateReservationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
aggregateReservation_ = value;
} else {
aggregateReservationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*/
public Builder setAggregateReservation(
com.google.cloud.compute.v1.AllocationAggregateReservation.Builder builderForValue) {
if (aggregateReservationBuilder_ == null) {
aggregateReservation_ = builderForValue.build();
} else {
aggregateReservationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*/
public Builder mergeAggregateReservation(
com.google.cloud.compute.v1.AllocationAggregateReservation value) {
if (aggregateReservationBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)
&& aggregateReservation_ != null
&& aggregateReservation_
!= com.google.cloud.compute.v1.AllocationAggregateReservation
.getDefaultInstance()) {
getAggregateReservationBuilder().mergeFrom(value);
} else {
aggregateReservation_ = value;
}
} else {
aggregateReservationBuilder_.mergeFrom(value);
}
if (aggregateReservation_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*/
public Builder clearAggregateReservation() {
bitField0_ = (bitField0_ & ~0x00000001);
aggregateReservation_ = null;
if (aggregateReservationBuilder_ != null) {
aggregateReservationBuilder_.dispose();
aggregateReservationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*/
public com.google.cloud.compute.v1.AllocationAggregateReservation.Builder
getAggregateReservationBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getAggregateReservationFieldBuilder().getBuilder();
}
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*/
public com.google.cloud.compute.v1.AllocationAggregateReservationOrBuilder
getAggregateReservationOrBuilder() {
if (aggregateReservationBuilder_ != null) {
return aggregateReservationBuilder_.getMessageOrBuilder();
} else {
return aggregateReservation_ == null
? com.google.cloud.compute.v1.AllocationAggregateReservation.getDefaultInstance()
: aggregateReservation_;
}
}
/**
*
*
*
* Reservation for aggregated resources, providing shape flexibility.
*
*
*
* optional .google.cloud.compute.v1.AllocationAggregateReservation aggregate_reservation = 291567948;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AllocationAggregateReservation,
com.google.cloud.compute.v1.AllocationAggregateReservation.Builder,
com.google.cloud.compute.v1.AllocationAggregateReservationOrBuilder>
getAggregateReservationFieldBuilder() {
if (aggregateReservationBuilder_ == null) {
aggregateReservationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AllocationAggregateReservation,
com.google.cloud.compute.v1.AllocationAggregateReservation.Builder,
com.google.cloud.compute.v1.AllocationAggregateReservationOrBuilder>(
getAggregateReservation(), getParentForChildren(), isClean());
aggregateReservation_ = null;
}
return aggregateReservationBuilder_;
}
private java.lang.Object commitment_ = "";
/**
*
*
*
* [Output Only] Full or partial URL to a parent commitment. This field displays for reservations that are tied to a commitment.
*
*
* optional string commitment = 482134805;
*
* @return Whether the commitment field is set.
*/
public boolean hasCommitment() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* [Output Only] Full or partial URL to a parent commitment. This field displays for reservations that are tied to a commitment.
*
*
* optional string commitment = 482134805;
*
* @return The commitment.
*/
public java.lang.String getCommitment() {
java.lang.Object ref = commitment_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
commitment_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Full or partial URL to a parent commitment. This field displays for reservations that are tied to a commitment.
*
*
* optional string commitment = 482134805;
*
* @return The bytes for commitment.
*/
public com.google.protobuf.ByteString getCommitmentBytes() {
java.lang.Object ref = commitment_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
commitment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Full or partial URL to a parent commitment. This field displays for reservations that are tied to a commitment.
*
*
* optional string commitment = 482134805;
*
* @param value The commitment to set.
* @return This builder for chaining.
*/
public Builder setCommitment(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
commitment_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Full or partial URL to a parent commitment. This field displays for reservations that are tied to a commitment.
*
*
* optional string commitment = 482134805;
*
* @return This builder for chaining.
*/
public Builder clearCommitment() {
commitment_ = getDefaultInstance().getCommitment();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Full or partial URL to a parent commitment. This field displays for reservations that are tied to a commitment.
*
*
* optional string commitment = 482134805;
*
* @param value The bytes for commitment to set.
* @return This builder for chaining.
*/
public Builder setCommitmentBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
commitment_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestamp(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
creationTimestamp_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return This builder for chaining.
*/
public Builder clearCreationTimestamp() {
creationTimestamp_ = getDefaultInstance().getCreationTimestamp();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The bytes for creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestampBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
creationTimestamp_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return Whether the description field is set.
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The bytes for description.
*/
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private long id_;
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
id_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The unique identifier for the resource. This identifier is defined by the server.
*
*
* optional uint64 id = 3355;
*
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000010);
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#reservations for reservations.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
public boolean hasKind() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#reservations for reservations.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#reservations for reservations.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#reservations for reservations.
*
*
* optional string kind = 3292052;
*
* @param value The kind to set.
* @return This builder for chaining.
*/
public Builder setKind(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#reservations for reservations.
*
*
* optional string kind = 3292052;
*
* @return This builder for chaining.
*/
public Builder clearKind() {
kind_ = getDefaultInstance().getKind();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#reservations for reservations.
*
*
* optional string kind = 3292052;
*
* @param value The bytes for kind to set.
* @return This builder for chaining.
*/
public Builder setKindBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
kind_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
*
*
* The name of the resource, provided by the client when initially creating the resource. The resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* The name of the resource, provided by the client when initially creating the resource. The resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The name of the resource, provided by the client when initially creating the resource. The resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The name of the resource, provided by the client when initially creating the resource. The resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* The name of the resource, provided by the client when initially creating the resource. The resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* The name of the resource, provided by the client when initially creating the resource. The resource name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private com.google.protobuf.MapField resourcePolicies_;
private com.google.protobuf.MapField
internalGetResourcePolicies() {
if (resourcePolicies_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ResourcePoliciesDefaultEntryHolder.defaultEntry);
}
return resourcePolicies_;
}
private com.google.protobuf.MapField
internalGetMutableResourcePolicies() {
if (resourcePolicies_ == null) {
resourcePolicies_ =
com.google.protobuf.MapField.newMapField(
ResourcePoliciesDefaultEntryHolder.defaultEntry);
}
if (!resourcePolicies_.isMutable()) {
resourcePolicies_ = resourcePolicies_.copy();
}
bitField0_ |= 0x00000080;
onChanged();
return resourcePolicies_;
}
public int getResourcePoliciesCount() {
return internalGetResourcePolicies().getMap().size();
}
/**
*
*
*
* Resource policies to be added to this reservation. The key is defined by user, and the value is resource policy url. This is to define placement policy with reservation.
*
*
* map<string, string> resource_policies = 22220385;
*/
@java.lang.Override
public boolean containsResourcePolicies(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetResourcePolicies().getMap().containsKey(key);
}
/** Use {@link #getResourcePoliciesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getResourcePolicies() {
return getResourcePoliciesMap();
}
/**
*
*
*
* Resource policies to be added to this reservation. The key is defined by user, and the value is resource policy url. This is to define placement policy with reservation.
*
*
* map<string, string> resource_policies = 22220385;
*/
@java.lang.Override
public java.util.Map getResourcePoliciesMap() {
return internalGetResourcePolicies().getMap();
}
/**
*
*
*
* Resource policies to be added to this reservation. The key is defined by user, and the value is resource policy url. This is to define placement policy with reservation.
*
*
* map<string, string> resource_policies = 22220385;
*/
@java.lang.Override
public /* nullable */ java.lang.String getResourcePoliciesOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetResourcePolicies().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Resource policies to be added to this reservation. The key is defined by user, and the value is resource policy url. This is to define placement policy with reservation.
*
*
* map<string, string> resource_policies = 22220385;
*/
@java.lang.Override
public java.lang.String getResourcePoliciesOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetResourcePolicies().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearResourcePolicies() {
bitField0_ = (bitField0_ & ~0x00000080);
internalGetMutableResourcePolicies().getMutableMap().clear();
return this;
}
/**
*
*
*
* Resource policies to be added to this reservation. The key is defined by user, and the value is resource policy url. This is to define placement policy with reservation.
*
*
* map<string, string> resource_policies = 22220385;
*/
public Builder removeResourcePolicies(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableResourcePolicies().getMutableMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map getMutableResourcePolicies() {
bitField0_ |= 0x00000080;
return internalGetMutableResourcePolicies().getMutableMap();
}
/**
*
*
*
* Resource policies to be added to this reservation. The key is defined by user, and the value is resource policy url. This is to define placement policy with reservation.
*
*
* map<string, string> resource_policies = 22220385;
*/
public Builder putResourcePolicies(java.lang.String key, java.lang.String value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableResourcePolicies().getMutableMap().put(key, value);
bitField0_ |= 0x00000080;
return this;
}
/**
*
*
*
* Resource policies to be added to this reservation. The key is defined by user, and the value is resource policy url. This is to define placement policy with reservation.
*
*
* map<string, string> resource_policies = 22220385;
*/
public Builder putAllResourcePolicies(
java.util.Map values) {
internalGetMutableResourcePolicies().getMutableMap().putAll(values);
bitField0_ |= 0x00000080;
return this;
}
private com.google.cloud.compute.v1.AllocationResourceStatus resourceStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AllocationResourceStatus,
com.google.cloud.compute.v1.AllocationResourceStatus.Builder,
com.google.cloud.compute.v1.AllocationResourceStatusOrBuilder>
resourceStatusBuilder_;
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*
* @return Whether the resourceStatus field is set.
*/
public boolean hasResourceStatus() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*
* @return The resourceStatus.
*/
public com.google.cloud.compute.v1.AllocationResourceStatus getResourceStatus() {
if (resourceStatusBuilder_ == null) {
return resourceStatus_ == null
? com.google.cloud.compute.v1.AllocationResourceStatus.getDefaultInstance()
: resourceStatus_;
} else {
return resourceStatusBuilder_.getMessage();
}
}
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*/
public Builder setResourceStatus(com.google.cloud.compute.v1.AllocationResourceStatus value) {
if (resourceStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resourceStatus_ = value;
} else {
resourceStatusBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*/
public Builder setResourceStatus(
com.google.cloud.compute.v1.AllocationResourceStatus.Builder builderForValue) {
if (resourceStatusBuilder_ == null) {
resourceStatus_ = builderForValue.build();
} else {
resourceStatusBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*/
public Builder mergeResourceStatus(com.google.cloud.compute.v1.AllocationResourceStatus value) {
if (resourceStatusBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)
&& resourceStatus_ != null
&& resourceStatus_
!= com.google.cloud.compute.v1.AllocationResourceStatus.getDefaultInstance()) {
getResourceStatusBuilder().mergeFrom(value);
} else {
resourceStatus_ = value;
}
} else {
resourceStatusBuilder_.mergeFrom(value);
}
if (resourceStatus_ != null) {
bitField0_ |= 0x00000100;
onChanged();
}
return this;
}
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*/
public Builder clearResourceStatus() {
bitField0_ = (bitField0_ & ~0x00000100);
resourceStatus_ = null;
if (resourceStatusBuilder_ != null) {
resourceStatusBuilder_.dispose();
resourceStatusBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*/
public com.google.cloud.compute.v1.AllocationResourceStatus.Builder getResourceStatusBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getResourceStatusFieldBuilder().getBuilder();
}
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*/
public com.google.cloud.compute.v1.AllocationResourceStatusOrBuilder
getResourceStatusOrBuilder() {
if (resourceStatusBuilder_ != null) {
return resourceStatusBuilder_.getMessageOrBuilder();
} else {
return resourceStatus_ == null
? com.google.cloud.compute.v1.AllocationResourceStatus.getDefaultInstance()
: resourceStatus_;
}
}
/**
*
*
*
* [Output Only] Status information for Reservation resource.
*
*
* optional .google.cloud.compute.v1.AllocationResourceStatus resource_status = 249429315;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AllocationResourceStatus,
com.google.cloud.compute.v1.AllocationResourceStatus.Builder,
com.google.cloud.compute.v1.AllocationResourceStatusOrBuilder>
getResourceStatusFieldBuilder() {
if (resourceStatusBuilder_ == null) {
resourceStatusBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AllocationResourceStatus,
com.google.cloud.compute.v1.AllocationResourceStatus.Builder,
com.google.cloud.compute.v1.AllocationResourceStatusOrBuilder>(
getResourceStatus(), getParentForChildren(), isClean());
resourceStatus_ = null;
}
return resourceStatusBuilder_;
}
private boolean satisfiesPzs_;
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return Whether the satisfiesPzs field is set.
*/
@java.lang.Override
public boolean hasSatisfiesPzs() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return The satisfiesPzs.
*/
@java.lang.Override
public boolean getSatisfiesPzs() {
return satisfiesPzs_;
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @param value The satisfiesPzs to set.
* @return This builder for chaining.
*/
public Builder setSatisfiesPzs(boolean value) {
satisfiesPzs_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Reserved for future use.
*
*
* optional bool satisfies_pzs = 480964267;
*
* @return This builder for chaining.
*/
public Builder clearSatisfiesPzs() {
bitField0_ = (bitField0_ & ~0x00000200);
satisfiesPzs_ = false;
onChanged();
return this;
}
private java.lang.Object selfLink_ = "";
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return Whether the selfLink field is set.
*/
public boolean hasSelfLink() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return The selfLink.
*/
public java.lang.String getSelfLink() {
java.lang.Object ref = selfLink_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLink_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return The bytes for selfLink.
*/
public com.google.protobuf.ByteString getSelfLinkBytes() {
java.lang.Object ref = selfLink_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLink_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @param value The selfLink to set.
* @return This builder for chaining.
*/
public Builder setSelfLink(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
selfLink_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @return This builder for chaining.
*/
public Builder clearSelfLink() {
selfLink_ = getDefaultInstance().getSelfLink();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined fully-qualified URL for this resource.
*
*
* optional string self_link = 456214797;
*
* @param value The bytes for selfLink to set.
* @return This builder for chaining.
*/
public Builder setSelfLinkBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
selfLink_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
private com.google.cloud.compute.v1.ShareSettings shareSettings_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.ShareSettings,
com.google.cloud.compute.v1.ShareSettings.Builder,
com.google.cloud.compute.v1.ShareSettingsOrBuilder>
shareSettingsBuilder_;
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*
* @return Whether the shareSettings field is set.
*/
public boolean hasShareSettings() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*
* @return The shareSettings.
*/
public com.google.cloud.compute.v1.ShareSettings getShareSettings() {
if (shareSettingsBuilder_ == null) {
return shareSettings_ == null
? com.google.cloud.compute.v1.ShareSettings.getDefaultInstance()
: shareSettings_;
} else {
return shareSettingsBuilder_.getMessage();
}
}
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*/
public Builder setShareSettings(com.google.cloud.compute.v1.ShareSettings value) {
if (shareSettingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
shareSettings_ = value;
} else {
shareSettingsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*/
public Builder setShareSettings(
com.google.cloud.compute.v1.ShareSettings.Builder builderForValue) {
if (shareSettingsBuilder_ == null) {
shareSettings_ = builderForValue.build();
} else {
shareSettingsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*/
public Builder mergeShareSettings(com.google.cloud.compute.v1.ShareSettings value) {
if (shareSettingsBuilder_ == null) {
if (((bitField0_ & 0x00000800) != 0)
&& shareSettings_ != null
&& shareSettings_ != com.google.cloud.compute.v1.ShareSettings.getDefaultInstance()) {
getShareSettingsBuilder().mergeFrom(value);
} else {
shareSettings_ = value;
}
} else {
shareSettingsBuilder_.mergeFrom(value);
}
if (shareSettings_ != null) {
bitField0_ |= 0x00000800;
onChanged();
}
return this;
}
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*/
public Builder clearShareSettings() {
bitField0_ = (bitField0_ & ~0x00000800);
shareSettings_ = null;
if (shareSettingsBuilder_ != null) {
shareSettingsBuilder_.dispose();
shareSettingsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*/
public com.google.cloud.compute.v1.ShareSettings.Builder getShareSettingsBuilder() {
bitField0_ |= 0x00000800;
onChanged();
return getShareSettingsFieldBuilder().getBuilder();
}
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*/
public com.google.cloud.compute.v1.ShareSettingsOrBuilder getShareSettingsOrBuilder() {
if (shareSettingsBuilder_ != null) {
return shareSettingsBuilder_.getMessageOrBuilder();
} else {
return shareSettings_ == null
? com.google.cloud.compute.v1.ShareSettings.getDefaultInstance()
: shareSettings_;
}
}
/**
*
*
*
* Specify share-settings to create a shared reservation. This property is optional. For more information about the syntax and options for this field and its subfields, see the guide for creating a shared reservation.
*
*
* optional .google.cloud.compute.v1.ShareSettings share_settings = 266668163;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.ShareSettings,
com.google.cloud.compute.v1.ShareSettings.Builder,
com.google.cloud.compute.v1.ShareSettingsOrBuilder>
getShareSettingsFieldBuilder() {
if (shareSettingsBuilder_ == null) {
shareSettingsBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.ShareSettings,
com.google.cloud.compute.v1.ShareSettings.Builder,
com.google.cloud.compute.v1.ShareSettingsOrBuilder>(
getShareSettings(), getParentForChildren(), isClean());
shareSettings_ = null;
}
return shareSettingsBuilder_;
}
private com.google.cloud.compute.v1.AllocationSpecificSKUReservation specificReservation_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AllocationSpecificSKUReservation,
com.google.cloud.compute.v1.AllocationSpecificSKUReservation.Builder,
com.google.cloud.compute.v1.AllocationSpecificSKUReservationOrBuilder>
specificReservationBuilder_;
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*
* @return Whether the specificReservation field is set.
*/
public boolean hasSpecificReservation() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*
* @return The specificReservation.
*/
public com.google.cloud.compute.v1.AllocationSpecificSKUReservation getSpecificReservation() {
if (specificReservationBuilder_ == null) {
return specificReservation_ == null
? com.google.cloud.compute.v1.AllocationSpecificSKUReservation.getDefaultInstance()
: specificReservation_;
} else {
return specificReservationBuilder_.getMessage();
}
}
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*/
public Builder setSpecificReservation(
com.google.cloud.compute.v1.AllocationSpecificSKUReservation value) {
if (specificReservationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
specificReservation_ = value;
} else {
specificReservationBuilder_.setMessage(value);
}
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*/
public Builder setSpecificReservation(
com.google.cloud.compute.v1.AllocationSpecificSKUReservation.Builder builderForValue) {
if (specificReservationBuilder_ == null) {
specificReservation_ = builderForValue.build();
} else {
specificReservationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*/
public Builder mergeSpecificReservation(
com.google.cloud.compute.v1.AllocationSpecificSKUReservation value) {
if (specificReservationBuilder_ == null) {
if (((bitField0_ & 0x00001000) != 0)
&& specificReservation_ != null
&& specificReservation_
!= com.google.cloud.compute.v1.AllocationSpecificSKUReservation
.getDefaultInstance()) {
getSpecificReservationBuilder().mergeFrom(value);
} else {
specificReservation_ = value;
}
} else {
specificReservationBuilder_.mergeFrom(value);
}
if (specificReservation_ != null) {
bitField0_ |= 0x00001000;
onChanged();
}
return this;
}
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*/
public Builder clearSpecificReservation() {
bitField0_ = (bitField0_ & ~0x00001000);
specificReservation_ = null;
if (specificReservationBuilder_ != null) {
specificReservationBuilder_.dispose();
specificReservationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*/
public com.google.cloud.compute.v1.AllocationSpecificSKUReservation.Builder
getSpecificReservationBuilder() {
bitField0_ |= 0x00001000;
onChanged();
return getSpecificReservationFieldBuilder().getBuilder();
}
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*/
public com.google.cloud.compute.v1.AllocationSpecificSKUReservationOrBuilder
getSpecificReservationOrBuilder() {
if (specificReservationBuilder_ != null) {
return specificReservationBuilder_.getMessageOrBuilder();
} else {
return specificReservation_ == null
? com.google.cloud.compute.v1.AllocationSpecificSKUReservation.getDefaultInstance()
: specificReservation_;
}
}
/**
*
*
*
* Reservation for instances with specific machine shapes.
*
*
*
* optional .google.cloud.compute.v1.AllocationSpecificSKUReservation specific_reservation = 404901951;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AllocationSpecificSKUReservation,
com.google.cloud.compute.v1.AllocationSpecificSKUReservation.Builder,
com.google.cloud.compute.v1.AllocationSpecificSKUReservationOrBuilder>
getSpecificReservationFieldBuilder() {
if (specificReservationBuilder_ == null) {
specificReservationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AllocationSpecificSKUReservation,
com.google.cloud.compute.v1.AllocationSpecificSKUReservation.Builder,
com.google.cloud.compute.v1.AllocationSpecificSKUReservationOrBuilder>(
getSpecificReservation(), getParentForChildren(), isClean());
specificReservation_ = null;
}
return specificReservationBuilder_;
}
private boolean specificReservationRequired_;
/**
*
*
*
* Indicates whether the reservation can be consumed by VMs with affinity for "any" reservation. If the field is set, then only VMs that target the reservation by name can consume from this reservation.
*
*
* optional bool specific_reservation_required = 226550687;
*
* @return Whether the specificReservationRequired field is set.
*/
@java.lang.Override
public boolean hasSpecificReservationRequired() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*
*
* Indicates whether the reservation can be consumed by VMs with affinity for "any" reservation. If the field is set, then only VMs that target the reservation by name can consume from this reservation.
*
*
* optional bool specific_reservation_required = 226550687;
*
* @return The specificReservationRequired.
*/
@java.lang.Override
public boolean getSpecificReservationRequired() {
return specificReservationRequired_;
}
/**
*
*
*
* Indicates whether the reservation can be consumed by VMs with affinity for "any" reservation. If the field is set, then only VMs that target the reservation by name can consume from this reservation.
*
*
* optional bool specific_reservation_required = 226550687;
*
* @param value The specificReservationRequired to set.
* @return This builder for chaining.
*/
public Builder setSpecificReservationRequired(boolean value) {
specificReservationRequired_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* Indicates whether the reservation can be consumed by VMs with affinity for "any" reservation. If the field is set, then only VMs that target the reservation by name can consume from this reservation.
*
*
* optional bool specific_reservation_required = 226550687;
*
* @return This builder for chaining.
*/
public Builder clearSpecificReservationRequired() {
bitField0_ = (bitField0_ & ~0x00002000);
specificReservationRequired_ = false;
onChanged();
return this;
}
private java.lang.Object status_ = "";
/**
*
*
*
* [Output Only] The status of the reservation. - CREATING: Reservation resources are being allocated. - READY: Reservation resources have been allocated, and the reservation is ready for use. - DELETING: Reservation deletion is in progress. - UPDATING: Reservation update is in progress.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*
*
* [Output Only] The status of the reservation. - CREATING: Reservation resources are being allocated. - READY: Reservation resources have been allocated, and the reservation is ready for use. - DELETING: Reservation deletion is in progress. - UPDATING: Reservation update is in progress.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The status.
*/
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
status_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The status of the reservation. - CREATING: Reservation resources are being allocated. - READY: Reservation resources have been allocated, and the reservation is ready for use. - DELETING: Reservation deletion is in progress. - UPDATING: Reservation update is in progress.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The bytes for status.
*/
public com.google.protobuf.ByteString getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The status of the reservation. - CREATING: Reservation resources are being allocated. - READY: Reservation resources have been allocated, and the reservation is ready for use. - DELETING: Reservation deletion is in progress. - UPDATING: Reservation update is in progress.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The status of the reservation. - CREATING: Reservation resources are being allocated. - READY: Reservation resources have been allocated, and the reservation is ready for use. - DELETING: Reservation deletion is in progress. - UPDATING: Reservation update is in progress.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = getDefaultInstance().getStatus();
bitField0_ = (bitField0_ & ~0x00004000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The status of the reservation. - CREATING: Reservation resources are being allocated. - READY: Reservation resources have been allocated, and the reservation is ready for use. - DELETING: Reservation deletion is in progress. - UPDATING: Reservation update is in progress.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @param value The bytes for status to set.
* @return This builder for chaining.
*/
public Builder setStatusBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
status_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
private java.lang.Object zone_ = "";
/**
*
*
*
* Zone in which the reservation resides. A zone must be provided if the reservation is created within a commitment.
*
*
* optional string zone = 3744684;
*
* @return Whether the zone field is set.
*/
public boolean hasZone() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
*
*
* Zone in which the reservation resides. A zone must be provided if the reservation is created within a commitment.
*
*
* optional string zone = 3744684;
*
* @return The zone.
*/
public java.lang.String getZone() {
java.lang.Object ref = zone_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
zone_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Zone in which the reservation resides. A zone must be provided if the reservation is created within a commitment.
*
*
* optional string zone = 3744684;
*
* @return The bytes for zone.
*/
public com.google.protobuf.ByteString getZoneBytes() {
java.lang.Object ref = zone_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
zone_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Zone in which the reservation resides. A zone must be provided if the reservation is created within a commitment.
*
*
* optional string zone = 3744684;
*
* @param value The zone to set.
* @return This builder for chaining.
*/
public Builder setZone(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
zone_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Zone in which the reservation resides. A zone must be provided if the reservation is created within a commitment.
*
*
* optional string zone = 3744684;
*
* @return This builder for chaining.
*/
public Builder clearZone() {
zone_ = getDefaultInstance().getZone();
bitField0_ = (bitField0_ & ~0x00008000);
onChanged();
return this;
}
/**
*
*
*
* Zone in which the reservation resides. A zone must be provided if the reservation is created within a commitment.
*
*
* optional string zone = 3744684;
*
* @param value The bytes for zone to set.
* @return This builder for chaining.
*/
public Builder setZoneBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
zone_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.Reservation)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.Reservation)
private static final com.google.cloud.compute.v1.Reservation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.Reservation();
}
public static com.google.cloud.compute.v1.Reservation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Reservation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.Reservation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy