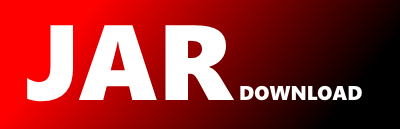
com.google.cloud.compute.v1.RouterBgpOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
public interface RouterBgpOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.compute.v1.RouterBgp)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* User-specified flag to indicate which mode to use for advertisement. The options are DEFAULT or CUSTOM.
* Check the AdvertiseMode enum for the list of possible values.
*
*
* optional string advertise_mode = 312134331;
*
* @return Whether the advertiseMode field is set.
*/
boolean hasAdvertiseMode();
/**
*
*
*
* User-specified flag to indicate which mode to use for advertisement. The options are DEFAULT or CUSTOM.
* Check the AdvertiseMode enum for the list of possible values.
*
*
* optional string advertise_mode = 312134331;
*
* @return The advertiseMode.
*/
java.lang.String getAdvertiseMode();
/**
*
*
*
* User-specified flag to indicate which mode to use for advertisement. The options are DEFAULT or CUSTOM.
* Check the AdvertiseMode enum for the list of possible values.
*
*
* optional string advertise_mode = 312134331;
*
* @return The bytes for advertiseMode.
*/
com.google.protobuf.ByteString getAdvertiseModeBytes();
/**
*
*
*
* User-specified list of prefix groups to advertise in custom mode. This field can only be populated if advertise_mode is CUSTOM and is advertised to all peers of the router. These groups will be advertised in addition to any specified prefixes. Leave this field blank to advertise no custom groups.
* Check the AdvertisedGroups enum for the list of possible values.
*
*
* repeated string advertised_groups = 21065526;
*
* @return A list containing the advertisedGroups.
*/
java.util.List getAdvertisedGroupsList();
/**
*
*
*
* User-specified list of prefix groups to advertise in custom mode. This field can only be populated if advertise_mode is CUSTOM and is advertised to all peers of the router. These groups will be advertised in addition to any specified prefixes. Leave this field blank to advertise no custom groups.
* Check the AdvertisedGroups enum for the list of possible values.
*
*
* repeated string advertised_groups = 21065526;
*
* @return The count of advertisedGroups.
*/
int getAdvertisedGroupsCount();
/**
*
*
*
* User-specified list of prefix groups to advertise in custom mode. This field can only be populated if advertise_mode is CUSTOM and is advertised to all peers of the router. These groups will be advertised in addition to any specified prefixes. Leave this field blank to advertise no custom groups.
* Check the AdvertisedGroups enum for the list of possible values.
*
*
* repeated string advertised_groups = 21065526;
*
* @param index The index of the element to return.
* @return The advertisedGroups at the given index.
*/
java.lang.String getAdvertisedGroups(int index);
/**
*
*
*
* User-specified list of prefix groups to advertise in custom mode. This field can only be populated if advertise_mode is CUSTOM and is advertised to all peers of the router. These groups will be advertised in addition to any specified prefixes. Leave this field blank to advertise no custom groups.
* Check the AdvertisedGroups enum for the list of possible values.
*
*
* repeated string advertised_groups = 21065526;
*
* @param index The index of the value to return.
* @return The bytes of the advertisedGroups at the given index.
*/
com.google.protobuf.ByteString getAdvertisedGroupsBytes(int index);
/**
*
*
*
* User-specified list of individual IP ranges to advertise in custom mode. This field can only be populated if advertise_mode is CUSTOM and is advertised to all peers of the router. These IP ranges will be advertised in addition to any specified groups. Leave this field blank to advertise no custom IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.RouterAdvertisedIpRange advertised_ip_ranges = 35449932;
*
*/
java.util.List getAdvertisedIpRangesList();
/**
*
*
*
* User-specified list of individual IP ranges to advertise in custom mode. This field can only be populated if advertise_mode is CUSTOM and is advertised to all peers of the router. These IP ranges will be advertised in addition to any specified groups. Leave this field blank to advertise no custom IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.RouterAdvertisedIpRange advertised_ip_ranges = 35449932;
*
*/
com.google.cloud.compute.v1.RouterAdvertisedIpRange getAdvertisedIpRanges(int index);
/**
*
*
*
* User-specified list of individual IP ranges to advertise in custom mode. This field can only be populated if advertise_mode is CUSTOM and is advertised to all peers of the router. These IP ranges will be advertised in addition to any specified groups. Leave this field blank to advertise no custom IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.RouterAdvertisedIpRange advertised_ip_ranges = 35449932;
*
*/
int getAdvertisedIpRangesCount();
/**
*
*
*
* User-specified list of individual IP ranges to advertise in custom mode. This field can only be populated if advertise_mode is CUSTOM and is advertised to all peers of the router. These IP ranges will be advertised in addition to any specified groups. Leave this field blank to advertise no custom IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.RouterAdvertisedIpRange advertised_ip_ranges = 35449932;
*
*/
java.util.List extends com.google.cloud.compute.v1.RouterAdvertisedIpRangeOrBuilder>
getAdvertisedIpRangesOrBuilderList();
/**
*
*
*
* User-specified list of individual IP ranges to advertise in custom mode. This field can only be populated if advertise_mode is CUSTOM and is advertised to all peers of the router. These IP ranges will be advertised in addition to any specified groups. Leave this field blank to advertise no custom IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.RouterAdvertisedIpRange advertised_ip_ranges = 35449932;
*
*/
com.google.cloud.compute.v1.RouterAdvertisedIpRangeOrBuilder getAdvertisedIpRangesOrBuilder(
int index);
/**
*
*
*
* Local BGP Autonomous System Number (ASN). Must be an RFC6996 private ASN, either 16-bit or 32-bit. The value will be fixed for this router resource. All VPN tunnels that link to this router will have the same local ASN.
*
*
* optional uint32 asn = 96892;
*
* @return Whether the asn field is set.
*/
boolean hasAsn();
/**
*
*
*
* Local BGP Autonomous System Number (ASN). Must be an RFC6996 private ASN, either 16-bit or 32-bit. The value will be fixed for this router resource. All VPN tunnels that link to this router will have the same local ASN.
*
*
* optional uint32 asn = 96892;
*
* @return The asn.
*/
int getAsn();
/**
*
*
*
* Explicitly specifies a range of valid BGP Identifiers for this Router. It is provided as a link-local IPv4 range (from 169.254.0.0/16), of size at least /30, even if the BGP sessions are over IPv6. It must not overlap with any IPv4 BGP session ranges. Other vendors commonly call this "router ID".
*
*
* optional string identifier_range = 501573159;
*
* @return Whether the identifierRange field is set.
*/
boolean hasIdentifierRange();
/**
*
*
*
* Explicitly specifies a range of valid BGP Identifiers for this Router. It is provided as a link-local IPv4 range (from 169.254.0.0/16), of size at least /30, even if the BGP sessions are over IPv6. It must not overlap with any IPv4 BGP session ranges. Other vendors commonly call this "router ID".
*
*
* optional string identifier_range = 501573159;
*
* @return The identifierRange.
*/
java.lang.String getIdentifierRange();
/**
*
*
*
* Explicitly specifies a range of valid BGP Identifiers for this Router. It is provided as a link-local IPv4 range (from 169.254.0.0/16), of size at least /30, even if the BGP sessions are over IPv6. It must not overlap with any IPv4 BGP session ranges. Other vendors commonly call this "router ID".
*
*
* optional string identifier_range = 501573159;
*
* @return The bytes for identifierRange.
*/
com.google.protobuf.ByteString getIdentifierRangeBytes();
/**
*
*
*
* The interval in seconds between BGP keepalive messages that are sent to the peer. Hold time is three times the interval at which keepalive messages are sent, and the hold time is the maximum number of seconds allowed to elapse between successive keepalive messages that BGP receives from a peer. BGP will use the smaller of either the local hold time value or the peer's hold time value as the hold time for the BGP connection between the two peers. If set, this value must be between 20 and 60. The default is 20.
*
*
* optional uint32 keepalive_interval = 276771516;
*
* @return Whether the keepaliveInterval field is set.
*/
boolean hasKeepaliveInterval();
/**
*
*
*
* The interval in seconds between BGP keepalive messages that are sent to the peer. Hold time is three times the interval at which keepalive messages are sent, and the hold time is the maximum number of seconds allowed to elapse between successive keepalive messages that BGP receives from a peer. BGP will use the smaller of either the local hold time value or the peer's hold time value as the hold time for the BGP connection between the two peers. If set, this value must be between 20 and 60. The default is 20.
*
*
* optional uint32 keepalive_interval = 276771516;
*
* @return The keepaliveInterval.
*/
int getKeepaliveInterval();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy